5.7 ELMo#
!wget -nc --no-cache -O init.py -q https://raw.githubusercontent.com/rramosp/2021.deeplearning/main/content/init.py
import init; init.init(force_download=False);
replicating local resources
import sys
if 'google.colab' in sys.modules:
print ("setting tensorflow version in colab")
%tensorflow_version 1.x
import tensorflow as tf
tf.__version__
setting tensorflow version in colab
TensorFlow 1.x selected.
'1.15.2'
ELMo: Embeddings from Language Models#
Word embeddings such as word2vec or GloVe provides an exact meaning to words. Eventhough they provided a great improvement to many NLP task, such “constant” meaning was a major drawback of this word embeddings as the meaning of words changes based on context, and thus this wasn’t the best option for Language Modelling.
For instance, after we train word2vec/Glove on a corpus we get as output one vector representation for, say the word cell. So even if we had a sentence like “He went to the prison cell with his cell phone to extract blood cell samples from inmates”, where the word cell has different meanings based on the sentence context, these models just collapse them all into one vector for cell in their output source.
Unlike most widely used word embeddings ELMo word representations are functions of the entire input sentence, instead of the single word. They are computed on top of two-layer Bidirectional Language Models (biLMs) with character convolutions, as a linear function of the internal network states. Therefore, the same word can have different word vectors under different contexts.
Deep contextualized word representations
Character-level Convolutional Networks
from IPython.display import Image
#Image(filename='local/imgs/ELMo.gif', width=1200)
Image(open('local/imgs/ELMo.gif','rb').read())
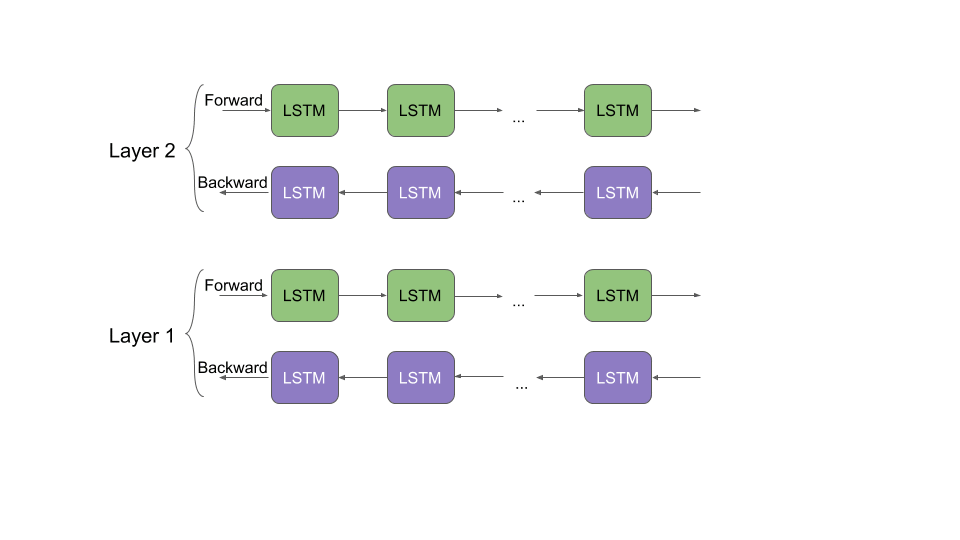
Given \(T\) tokens \((x_1,x_2,\cdots,x_T)\), a forward language model computes the probability of the sequence by modeling the probability of token \(x_k\) given the history \((x_1,\cdots, x_{k-1})\). This formulation has been addressed in the state of the art using many different approach, and more recently including some approximation based on Bidirectional Recurrent Networks.
ELMo is inspired in the Language Modelling problem, which has the advantage of being a self-supervised task.
A practical implication of this difference is that we can use word2vec and Glove vectors trained on a large corpus directly for downstream tasks. All we need is the vectors for the words. There is no need for the model itself that was used to train these vectors.
However, in the case of ELMo and BERT (we will see it in a forthcoming lecture), since they are context dependent, we need the model that was used to train the vectors even after training, since the models generate the vectors for a word based on context. source.
Let’s see how to define a simplified ELMo version from scratch:#
def Char_CNN(vocab_size, input_size = 120, embedding_size=32):
# parameter
conv_layers = [[8, 3, 2],
[8, 3, 2],
[8, 3, -1],#]
[8, 3, -1]]
fully_connected_layers = [64, 64]
dropout_p = 0.5
# Embedding layer Initialization
embedding_layer = Embedding(vocab_size + 1,
embedding_size,
input_length=input_size,mask_zero=True))
# Model Construction
# Input
inputs = Input(shape=(input_size,), name='input_c', dtype='int64') # shape=(?, 1014)
# Embedding
x = embedding_layer(inputs)
# Conv
for filter_num, filter_size, pooling_size in conv_layers:
x = Conv1D(filter_num, filter_size)(x)
x = Activation('relu')(x)
if pooling_size != -1:
x = MaxPooling1D(pool_size=pooling_size)(x) # Final shape=(None, 34, 256)
x = Flatten()(x) # (None, 8704)
# Fully connected layers
for dense_size in fully_connected_layers:
x = Dense(dense_size, activation='relu')(x) # dense_size == 1024
x = Dropout(dropout_p)(x)
Char_CNN_Embeddings = Model(inputs=inputs, outputs=x)
return Char_CNN_Embeddings
#Define model on top of Char_CNN: sentiment as input
Input_elmo = Input(shape=(input_text_charact_padded[0].shape[0],input_text_charact_padded[0].shape[1],), name='input_s')
CNN_Embeddings = Char_CNN(len(tk.word_index), input_size = word_max_length, embedding_size=32)
embedding = TimeDistributed(CNN_Embeddings)(Input_elmo)
x = Bidirectional(LSTM(units=128, return_sequences=True,
recurrent_dropout=0.2, dropout=0.2))(embedding)
x_rnn = Bidirectional(LSTM(units=128, return_sequences=True,
recurrent_dropout=0.2, dropout=0.2))(x)
x_add = add([x, x_rnn]) # residual connection to the first biLSTM
#----------------ELMo ends here -------------------------------------
#--------------------------------------------------------------------
x_dense = TimeDistributed(Dense(16, activation="relu"))(x_add)
out_1 = TimeDistributed(Dense(1, activation="sigmoid"))(x_dense)
model2 = Model(inputs=[Input_elmo], outputs=[out_1])
We can also use pre-trained ELMo from tensorflow hub repository.
Download the model#
Let’s load ELMo model. This will take some time because the model is over 350 Mb in size
import tensorflow_hub as hub
import tensorflow as tf
#gpus= tf.config.experimental.list_physical_devices('GPU')
#tf.config.experimental.set_memory_growth(gpus[0], True)
elmo_model = hub.Module("https://tfhub.dev/google/elmo/2", trainable=True)
# In TEnsorFlow 2 the statement should be somethin like this
#elmo_model = hub.KerasLayer("https://tfhub.dev/google/elmo/2")
embeddings = elmo_model(["i like green eggs and ham",
"would you eat them in a box"],
signature="default",
as_dict=True)["elmo"]
print(embeddings.shape)
INFO:tensorflow:Saver not created because there are no variables in the graph to restore
INFO:tensorflow:Saver not created because there are no variables in the graph to restore
(2, 7, 1024)
Name Entity Recognition (NER)#
NER is a sequential labeling problem where the aim is to label every word in a sentence of pargraph, according to a list of “entity” classes. This is different from part of Speech (POS) tagging, which explains how a word is used in a sentence For more information about POS.
Tipical labels in NER are:
import nltk
nltk.download('maxent_ne_chunker')
chunker=nltk.data.load(nltk.chunk._MULTICLASS_NE_CHUNKER)
sorted(chunker._tagger._classifier.labels())
[nltk_data] Downloading package maxent_ne_chunker to
[nltk_data] /root/nltk_data...
[nltk_data] Unzipping chunkers/maxent_ne_chunker.zip.
['B-FACILITY',
'B-GPE',
'B-GSP',
'B-LOCATION',
'B-ORGANIZATION',
'B-PERSON',
'I-FACILITY',
'I-GPE',
'I-GSP',
'I-LOCATION',
'I-ORGANIZATION',
'I-PERSON',
'O']
GPE stands for Geo-Political Entity, GSP stands for Geographical-Social-Political Entity. and there are other definitions of labels that include more classes Detailed info can be found here.
NER task is commonly viewed as a sequential prediction problem in which we aim at assigning the correct label for each token. Different ways of encoding information in a set of labels make different chunk representation. The two most popular schemes are BIO and BILOU source.
BIO stands for Beginning, Inside and Outside (of a text segment).
Similar but more detailed than BIO, BILOU encode the Beginning, the Inside and Last token of multi-token chunks while differentiate them from Unit-length chunks
import pandas as pd
import math
import numpy as np
import matplotlib.pyplot as plt
plt.style.use("ggplot")
data = pd.read_csv("local/data/ner_dataset.csv", encoding="latin1")
data = data.drop(['POS'], axis =1)
data = data.fillna(method="ffill")
data.tail(12)
Sentence # | Word | Tag | |
---|---|---|---|
1048563 | Sentence: 47958 | exploded | O |
1048564 | Sentence: 47958 | upon | O |
1048565 | Sentence: 47958 | impact | O |
1048566 | Sentence: 47958 | . | O |
1048567 | Sentence: 47959 | Indian | B-gpe |
1048568 | Sentence: 47959 | forces | O |
1048569 | Sentence: 47959 | said | O |
1048570 | Sentence: 47959 | they | O |
1048571 | Sentence: 47959 | responded | O |
1048572 | Sentence: 47959 | to | O |
1048573 | Sentence: 47959 | the | O |
1048574 | Sentence: 47959 | attack | O |
words = set(list(data['Word'].values))
words.add('PADword')
n_words = len(words)
n_words
35179
tags = list(set(data["Tag"].values))
n_tags = len(tags)
n_tags
17
tags
['B-org',
'I-org',
'B-art',
'I-geo',
'I-art',
'I-gpe',
'I-per',
'I-tim',
'B-nat',
'I-eve',
'B-geo',
'B-per',
'I-nat',
'B-gpe',
'O',
'B-tim',
'B-eve']
class SentenceGetter(object):
def __init__(self, data):
self.n_sent = 1
self.data = data
self.empty = False
agg_func = lambda s: [(w, t) for w, t in zip(s["Word"].values.tolist(),s["Tag"].values.tolist())]
self.grouped = self.data.groupby("Sentence #").apply(agg_func)
self.sentences = [s for s in self.grouped]
def get_next(self):
try:
s = self.grouped["Sentence: {}".format(self.n_sent)]
self.n_sent += 1
return s
except:
return None
getter = SentenceGetter(data)
sent = getter.get_next()
print(sent)
[('Thousands', 'O'), ('of', 'O'), ('demonstrators', 'O'), ('have', 'O'), ('marched', 'O'), ('through', 'O'), ('London', 'B-geo'), ('to', 'O'), ('protest', 'O'), ('the', 'O'), ('war', 'O'), ('in', 'O'), ('Iraq', 'B-geo'), ('and', 'O'), ('demand', 'O'), ('the', 'O'), ('withdrawal', 'O'), ('of', 'O'), ('British', 'B-gpe'), ('troops', 'O'), ('from', 'O'), ('that', 'O'), ('country', 'O'), ('.', 'O')]
sentences = getter.sentences
print(len(sentences))
47959
largest_sen = max(len(sen) for sen in sentences)
print('biggest sentence has {} words'.format(largest_sen))
biggest sentence has 104 words
%matplotlib inline
plt.hist([len(sen) for sen in sentences], bins= 50)
plt.show()
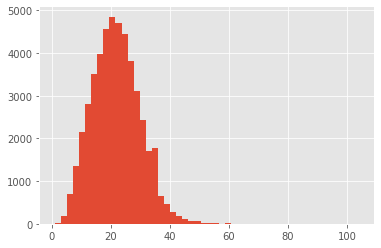
words2index = {w:i for i,w in enumerate(words)}
tags2index = {t:i for i,t in enumerate(tags)}
print(words2index['London'])
print(tags2index['B-geo'])
11193
10
ELMo embedding represents every word as 1024 feature vector. Therefore, in order to reduce the computational complexity and based on the former histogram, we can truncate the sequences to a maximum length of 50.
max_len = 50
X = [[w[0]for w in s] for s in sentences]
new_X = []
for seq in X:
new_seq = []
for i in range(max_len):
try:
new_seq.append(seq[i])
except:
new_seq.append("PADword")
new_X.append(new_seq)
new_X[15]
['Israeli',
'officials',
'say',
'Prime',
'Minister',
'Ariel',
'Sharon',
'will',
'undergo',
'a',
'medical',
'procedure',
'Thursday',
'to',
'close',
'a',
'tiny',
'hole',
'in',
'his',
'heart',
'discovered',
'during',
'treatment',
'for',
'a',
'minor',
'stroke',
'suffered',
'last',
'month',
'.',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword',
'PADword']
from keras.preprocessing.sequence import pad_sequences
y = [[tags2index[w[1]] for w in s] for s in sentences]
y = pad_sequences(maxlen=max_len, sequences=y, padding="post", value=tags2index["O"])
y[15]
Using TensorFlow backend.
array([13, 14, 14, 11, 6, 6, 6, 14, 14, 14, 14, 14, 15, 14, 14, 14, 14,
14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14,
14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14],
dtype=int32)
from sklearn.model_selection import train_test_split
X_tr, X_te, y_tr, y_te = train_test_split(new_X, y, test_size=0.1, random_state=2018)
batch_size = 32
import tensorflow as tf
import tensorflow_hub as hub
from keras import backend as K
sess = tf.Session()
K.set_session(sess)
sess.run(tf.global_variables_initializer())
sess.run(tf.tables_initializer())
This is how we have to define the layer in order to be used in the model (In TF 2.x this should not be necessary take a look to this guide):
def ElmoEmbedding(x):
return elmo_model(inputs={
"tokens": tf.squeeze(tf.cast(x, tf.string)),
"sequence_len": tf.constant(batch_size*[max_len])
},
signature="tokens",
as_dict=True)["elmo"]
from keras.models import Model, Input
from keras.layers.merge import add
from keras.layers import LSTM, Embedding, Dense, TimeDistributed, Dropout, Bidirectional, Lambda
Excercise 1: Complete the model arquitecture#
After the Embedding layer include two Bidirectional LSTM layers with 512 cells each. The second layer must be on top of the first one. As output layer include a Dense layer which takes as input the sum of the two Bidirectional layers output’s. Remember that in NER the output must be a sequence of same length as the input.
input_text = Input(shape=(max_len,), dtype=tf.string)
embedding = Lambda(ElmoEmbedding, output_shape=(max_len, 1024))(input_text)
x = Bidirectional(LSTM(units=512, return_sequences=True,
recurrent_dropout=0.2, dropout=0.2))(embedding)
x_rnn = Bidirectional(LSTM(units=512, return_sequences=True,
recurrent_dropout=0.2, dropout=0.2))(x)
x = add([x, x_rnn]) # residual connection to the first biLSTM
out = TimeDistributed(Dense(n_tags, activation="softmax"))(x)
INFO:tensorflow:Saver not created because there are no variables in the graph to restore
INFO:tensorflow:Saver not created because there are no variables in the graph to restore
model = Model(input_text, out)
model.compile(optimizer="adam", loss="sparse_categorical_crossentropy", metrics=["accuracy"])
Split data into training and validation subsets, use both during training to evaluate training and validation accuracies.
X_tr, X_val = X_tr[:1213*batch_size], X_tr[-135*batch_size:]
y_tr, y_val = y_tr[:1213*batch_size], y_tr[-135*batch_size:]
y_tr = y_tr.reshape(y_tr.shape[0], y_tr.shape[1], 1)
y_val = y_val.reshape(y_val.shape[0], y_val.shape[1], 1)
Train the model using a batch_size = 32 and 5 epochs. Use verbose=1 to see the evolution of the training process.
model.fit(np.array(X_tr), y_tr, validation_data=(np.array(X_val), y_val),
batch_size=batch_size, epochs=5, verbose=1)
Train on 38816 samples, validate on 4320 samples
Epoch 1/5
38816/38816 [==============================] - 542s 14ms/step - loss: 0.0584 - accuracy: 0.9828 - val_loss: 0.0415 - val_accuracy: 0.9867
Epoch 2/5
38816/38816 [==============================] - 542s 14ms/step - loss: 0.0413 - accuracy: 0.9867 - val_loss: 0.0329 - val_accuracy: 0.9889
Epoch 3/5
38816/38816 [==============================] - 542s 14ms/step - loss: 0.0347 - accuracy: 0.9884 - val_loss: 0.0267 - val_accuracy: 0.9907
Epoch 4/5
38816/38816 [==============================] - 540s 14ms/step - loss: 0.0291 - accuracy: 0.9899 - val_loss: 0.0208 - val_accuracy: 0.9927
Epoch 5/5
38816/38816 [==============================] - 541s 14ms/step - loss: 0.0242 - accuracy: 0.9914 - val_loss: 0.0169 - val_accuracy: 0.9943
<keras.callbacks.callbacks.History at 0x7f0a2f696a20>
!pip install seqeval
Collecting seqeval
Downloading https://files.pythonhosted.org/packages/34/91/068aca8d60ce56dd9ba4506850e876aba5e66a6f2f29aa223224b50df0de/seqeval-0.0.12.tar.gz
Requirement already satisfied: numpy>=1.14.0 in /usr/local/lib/python3.6/dist-packages (from seqeval) (1.18.3)
Requirement already satisfied: Keras>=2.2.4 in /usr/local/lib/python3.6/dist-packages (from seqeval) (2.3.1)
Requirement already satisfied: six>=1.9.0 in /usr/local/lib/python3.6/dist-packages (from Keras>=2.2.4->seqeval) (1.12.0)
Requirement already satisfied: pyyaml in /usr/local/lib/python3.6/dist-packages (from Keras>=2.2.4->seqeval) (3.13)
Requirement already satisfied: h5py in /usr/local/lib/python3.6/dist-packages (from Keras>=2.2.4->seqeval) (2.10.0)
Requirement already satisfied: scipy>=0.14 in /usr/local/lib/python3.6/dist-packages (from Keras>=2.2.4->seqeval) (1.4.1)
Requirement already satisfied: keras-preprocessing>=1.0.5 in /usr/local/lib/python3.6/dist-packages (from Keras>=2.2.4->seqeval) (1.1.0)
Requirement already satisfied: keras-applications>=1.0.6 in /usr/local/lib/python3.6/dist-packages (from Keras>=2.2.4->seqeval) (1.0.8)
Building wheels for collected packages: seqeval
Building wheel for seqeval (setup.py) ... ?25l?25hdone
Created wheel for seqeval: filename=seqeval-0.0.12-cp36-none-any.whl size=7424 sha256=b57be0c86874a72d5b1d198068cbc96e97ae0f762a26f7aa52c3ef7b099cf4b0
Stored in directory: /root/.cache/pip/wheels/4f/32/0a/df3b340a82583566975377d65e724895b3fad101a3fb729f68
Successfully built seqeval
Installing collected packages: seqeval
Successfully installed seqeval-0.0.12
from seqeval.metrics import precision_score, recall_score, f1_score, classification_report
X_te = X_te[:149*batch_size]
test_pred = model.predict(np.array(X_te), verbose=1)
4768/4768 [==============================] - 48s 10ms/step
idx2tag = {i: w for w, i in tags2index.items()}
def pred2label(pred):
out = []
for pred_i in pred:
out_i = []
for p in pred_i:
p_i = np.argmax(p)
out_i.append(idx2tag[p_i].replace("PADword", "O"))
out.append(out_i)
return out
def test2label(pred):
out = []
for pred_i in pred:
out_i = []
for p in pred_i:
out_i.append(idx2tag[p].replace("PADword", "O"))
out.append(out_i)
return out
pred_labels = pred2label(test_pred)
test_labels = test2label(y_te[:149*32])
print("F1-score: {:.1%}".format(f1_score(test_labels, pred_labels)))
F1-score: 82.6%
print(classification_report(test_labels, pred_labels))
precision recall f1-score support
geo 0.85 0.90 0.87 3720
per 0.74 0.77 0.76 1677
tim 0.86 0.86 0.86 2148
gpe 0.96 0.94 0.95 1591
org 0.69 0.70 0.70 2061
eve 0.22 0.36 0.27 33
art 0.38 0.10 0.16 49
nat 0.33 0.18 0.24 22
micro avg 0.82 0.84 0.83 11301
macro avg 0.82 0.84 0.83 11301
Let’s see the predictions for one sequence:
i = 390
p = model.predict(np.array(X_te[i:i+batch_size]))[0]
p = np.argmax(p, axis=-1)
print("{:15} {:5}: ({})".format("Word", "Pred", "True"))
print("="*30)
for w, true, pred in zip(X_te[i], y_te[i], p):
if w != "PADword":
print("{:15}:{:5} ({})".format(w, tags[pred], tags[true]))
Word Pred : (True)
==============================
Citing :O (O)
a :O (O)
draft :O (O)
report :O (O)
from :O (O)
the :O (O)
U.S. :B-org (B-org)
Government :I-org (I-org)
Accountability :I-org (O)
office :O (O)
, :O (O)
The :B-org (B-org)
New :I-org (I-org)
York :I-org (I-org)
Times :I-org (I-org)
said :O (O)
Saturday :B-tim (B-tim)
the :O (O)
losses :O (O)
amount :O (O)
to :O (O)
between :O (O)
1,00,000 :O (O)
and :O (O)
3,00,000 :O (O)
barrels :O (O)
a :O (O)
day :O (O)
of :O (O)
Iraq :B-geo (B-geo)
's :O (O)
declared :O (O)
oil :O (O)
production :O (O)
over :O (O)
the :O (O)
past :B-tim (B-tim)
four :I-tim (I-tim)
years :O (O)
. :O (O)
Lest’s compare the result with a simple Embedding layer instead of ELMo#
For the sake of comparison, create a model using a conventional Embedding layer, instead of ELMo model. We are going to use an output dimension of 1024 for the Embedding layer and 10000 words dictionary for tokanization.
class SentenceGetter(object):
def __init__(self, data):
self.n_sent = 1
self.data = data
self.empty = False
#agg_func = lambda s: [(w, t) for w, t in zip(s["Word"].values.tolist(),s["Tag"].values.tolist())]}
input_func = lambda s: " ".join(w for w in s["Word"].values.tolist())
ouput_func = lambda s: " ".join(t for t in s["Tag"].values.tolist())
self.in_grouped = self.data.groupby("Sentence #").apply(input_func)
self.out_grouped = self.data.groupby("Sentence #").apply(ouput_func)
self.input_sentences = [s for s in self.in_grouped]
self.output_targets = [s for s in self.out_grouped]
def get_next(self):
try:
i = self.in_grouped["Sentence: {}".format(self.n_sent)]
o = self.out_grouped["Sentence: {}".format(self.n_sent)]
self.n_sent += 1
return i,o
except:
return None
getter = SentenceGetter(data)
sent_input, out_put = getter.get_next()
sentences = getter.input_sentences
targets = getter.output_targets
print(len(sentences))
print(len(targets))
47959
47959
from keras.preprocessing.sequence import pad_sequences
y = [[tags2index[w] for w in s.split(' ')] for s in targets]
y = pad_sequences(maxlen=max_len, sequences=y, padding="post", value=tags2index["O"])
y[15]
array([13, 14, 14, 11, 6, 6, 6, 14, 14, 14, 14, 14, 15, 14, 14, 14, 14,
14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14,
14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14, 14],
dtype=int32)
from keras.preprocessing.text import Tokenizer
word_tokenizer = Tokenizer(num_words=10000)
# fit the tokenizer on the documents
word_tokenizer.fit_on_texts(sentences)
# summarize what was learned
print(word_tokenizer.word_counts)
print(word_tokenizer.document_count)
print(word_tokenizer.word_index)
print(word_tokenizer.word_docs)
# integer encode documents
encoded_docs = word_tokenizer.texts_to_sequences(sentences)
#print(encoded_docs)
OrderedDict([('thousands', 495), ('of', 26394), ('demonstrators', 132), ('have', 5487), ('marched', 65), ('through', 519), ('london', 280), ('to', 23322), ('protest', 238), ('the', 63916), ('war', 994), ('in', 28098), ('iraq', 1749), ('and', 20147), ('demand', 221), ('withdrawal', 154), ('british', 648), ('troops', 1202), ('from', 4557), ('that', 6437), ('country', 1948), ('iranian', 402), ('officials', 3390), ('say', 4178), ('they', 2397), ('expect', 66), ('get', 170), ('access', 127), ('sealed', 19), ('sensitive', 30), ('parts', 169), ('plant', 111), ('wednesday', 1258), ('after', 2740), ('an', 4236), ('iaea', 73), ('surveillance', 51), ('system', 267), ('begins', 51), ('functioning', 11), ('helicopter', 110), ('gunships', 18), ('saturday', 1152), ('pounded', 15), ('militant', 461), ('hideouts', 16), ('orakzai', 19), ('tribal', 170), ('region', 858), ('where', 658), ('many', 600), ('taliban', 288), ('militants', 1065), ('are', 3721), ('believed', 175), ('fled', 154), ('avoid', 82), ('earlier', 827), ('military', 2041), ('offensive', 154), ('nearby', 107), ('south', 1023), ('waziristan', 82), ('left', 352), ('a', 22785), ('tense', 17), ('hour', 129), ('long', 401), ('standoff', 31), ('with', 5448), ('riot', 46), ('police', 1868), ('u', 5472), ('n', 905), ('relief', 203), ('coordinator', 13), ('jan', 66), ('egeland', 14), ('said', 5331), ('sunday', 1215), ('s', 4563), ('indonesian', 114), ('australian', 121), ('helicopters', 69), ('ferrying', 2), ('out', 1093), ('food', 388), ('supplies', 163), ('remote', 99), ('areas', 309), ('western', 541), ('aceh', 56), ('province', 947), ('ground', 82), ('crews', 18), ('can', 511), ('not', 2710), ('reach', 124), ('mr', 3088), ('latest', 232), ('figures', 72), ('show', 222), ('1', 753), ('8', 264), ('million', 919), ('people', 2758), ('need', 147), ('assistance', 141), ('greatest', 19), ('indonesia', 221), ('sri', 144), ('lanka', 93), ('maldives', 6), ('india', 529), ('he', 4370), ('last', 1990), ('week', 1370), ("'s", 10923), ('tsunami', 157), ('massive', 144), ('underwater', 7), ('earthquake', 159), ('triggered', 75), ('it', 3823), ('has', 7216), ('affected', 92), ('millions', 144), ('asia', 242), ('africa', 356), ('some', 1110), ('27', 107), ('000', 1210), ('known', 335), ('dead', 360), ('aid', 487), ('is', 6750), ('being', 575), ('rushed', 23), ('but', 2520), ('official', 750), ('stressed', 50), ('bottlenecks', 2), ('lack', 107), ('infrastructure', 101), ('remain', 236), ('challenge', 54), ('lebanese', 217), ('politicians', 79), ('condemning', 18), ('friday', 1279), ('bomb', 720), ('blast', 338), ('christian', 97), ('neighborhood', 60), ('beirut', 96), ('as', 4226), ('attempt', 150), ('sow', 2), ('sectarian', 59), ('strife', 15), ('formerly', 26), ('torn', 54), ('string', 31), ('voiced', 22), ('their', 1798), ('anger', 24), ('while', 627), ('at', 4699), ('united', 2051), ('nations', 1036), ('summit', 245), ('new', 2155), ('york', 286), ('prime', 1135), ('minister', 1877), ('fouad', 3), ('siniora', 9), ('resolute', 1), ('preventing', 30), ('such', 423), ('attempts', 63), ('destroying', 30), ('spirit', 12), ('one', 1853), ('person', 141), ('was', 4881), ('killed', 2861), ('more', 2332), ('than', 1896), ('20', 413), ('others', 627), ('injured', 239), ('late', 570), ('which', 1633), ('took', 438), ('place', 381), ('on', 7137), ('residential', 15), ('street', 85), ('lebanon', 302), ('suffered', 102), ('series', 264), ('bombings', 243), ('since', 1358), ('explosion', 227), ('february', 263), ('former', 1020), ('rafik', 52), ('hariri', 105), ('other', 1527), ('syria', 294), ('widely', 65), ('accused', 490), ('involvement', 116), ('his', 3469), ('killing', 585), ('comes', 234), ('days', 529), ('before', 719), ('investigator', 16), ('detlev', 11), ('mehlis', 19), ('return', 262), ('damascus', 69), ('interview', 154), ('several', 915), ('syrian', 241), ('about', 1567), ('assassination', 131), ('global', 322), ('financial', 304), ('crisis', 257), ('iceland', 10), ('economy', 586), ('shambles', 1), ('israeli', 1044), ('ariel', 75), ('sharon', 159), ('will', 3404), ('undergo', 12), ('medical', 172), ('procedure', 23), ('thursday', 1333), ('close', 239), ('tiny', 19), ('hole', 10), ('heart', 71), ('discovered', 116), ('during', 1232), ('treatment', 106), ('for', 8586), ('minor', 32), ('stroke', 36), ('month', 1136), ('doctors', 102), ('describe', 14), ('birth', 32), ('defect', 4), ('partition', 4), ('between', 1050), ('upper', 40), ('chambers', 13), ('cardiac', 1), ('catheterization', 1), ('involves', 24), ('inserting', 1), ('catheter', 1), ('blood', 58), ('vessel', 53), ('into', 1159), ('umbrella', 6), ('like', 143), ('device', 40), ('plug', 1), ('make', 334), ('full', 143), ('recovery', 81), ('returned', 138), ('work', 362), ('december', 314), ('25', 259), ('emergency', 216), ('hospitalization', 4), ('caused', 217), ('any', 511), ('permanent', 87), ('damage', 120), ('designers', 3), ('first', 1142), ('private', 155), ('manned', 7), ('rocket', 158), ('burst', 13), ('space', 180), ('received', 164), ('10', 557), ('prize', 60), ('created', 75), ('promote', 59), ('tourism', 123), ('spaceshipone', 3), ('designer', 3), ('burt', 1), ('rutan', 1), ('accepted', 43), ('ansari', 1), ('x', 4), ('money', 221), ('trophy', 2), ('behalf', 21), ('team', 249), ('awards', 21), ('ceremony', 113), ('state', 1463), ('missouri', 9), ('win', 160), ('had', 1518), ('off', 565), ('twice', 45), ('two', 3011), ('period', 102), ('fly', 25), ('least', 1483), ('100', 223), ('kilometers', 285), ('above', 53), ('earth', 69), ('spacecraft', 17), ('made', 693), ('its', 2672), ('flights', 80), ('september', 321), ('early', 483), ('october', 249), ('lifting', 20), ('california', 95), ('mojave', 1), ('desert', 15), ('three', 1425), ('major', 436), ('banks', 73), ('collapsed', 52), ('unemployment', 109), ('soared', 24), ('value', 60), ('krona', 1), ('plunged', 36), ('vehicle', 193), ('carry', 84), ('pilot', 28), ('weight', 19), ('equivalent', 5), ('passengers', 72), ('financed', 7), ('paul', 131), ('allen', 10), ('co', 70), ('founder', 25), ('microsoft', 26), ('corporation', 45), ('north', 906), ('korea', 516), ('says', 4640), ('flooding', 65), ('by', 4541), ('typhoon', 47), ('wipha', 1), ('destroyed', 131), ('14', 200), ('homes', 187), ('09', 10), ('hectares', 15), ('crops', 34), ('news', 764), ('agency', 810), ('kcna', 8), ('reported', 486), ('monday', 1266), ('floods', 65), ('also', 2314), ('or', 934), ('damaged', 81), ('public', 375), ('buildings', 92), ('washed', 16), ('roads', 55), ('bridges', 11), ('railways', 4), ('report', 806), ('did', 412), ('mention', 16), ('deaths', 221), ('injuries', 103), ('most', 644), ('heavy', 190), ('rains', 86), ('occurred', 198), ('southwestern', 76), ('part', 639), ('including', 812), ('capital', 729), ('pyongyang', 130), ('severe', 69), ('600', 66), ('missing', 173), ('displaced', 95), ('00', 224), ('strong', 196), ('under', 754), ('ocean', 111), ('sumatra', 14), ('nias', 5), ('islands', 197), ('panic', 12), ('no', 1011), ('geological', 18), ('survey', 73), ('gave', 118), ('preliminary', 41), ('estimate', 29), ('strength', 39), ('tuesday', 1394), ('morning', 94), ('quake', 100), ('6', 212), ('7', 278), ('richter', 3), ('scale', 76), ('epicenter', 9), ('island', 305), ('geir', 2), ('haarde', 1), ('refused', 148), ('resign', 43), ('call', 191), ('elections', 768), ('cause', 113), ('march', 340), ('900', 20), ('both', 443), ('experienced', 38), ('countless', 2), ('earthquakes', 18), ('producing', 62), ('26', 142), ('death', 505), ('toll', 151), ('tragedy', 23), ('stands', 36), ('76', 28), ('28', 89), ('them', 618), ('nearly', 503), ('50', 288), ('still', 384), ('listed', 19), ('feared', 31), ('rap', 5), ('star', 60), ('snoop', 6), ('dogg', 6), ('five', 699), ('associates', 13), ('been', 2892), ('arrested', 467), ('britain', 335), ('disturbance', 4), ('heathrow', 6), ('airport', 113), ('told', 685), ('media', 398), ('musician', 16), ('who', 1981), ('born', 83), ('name', 122), ('calvin', 2), ('broadus', 2), ('members', 654), ('entourage', 3), ('were', 3520), ('held', 592), ('charges', 445), ('violent', 135), ('disorder', 9), ('affray', 1), ('group', 1324), ('waiting', 29), ('flight', 55), ('perform', 10), ('concert', 23), ('when', 1230), ('denied', 222), ('class', 29), ('lounge', 3), ('later', 514), ('threw', 50), ('bottles', 10), ('whisky', 1), ('duty', 35), ('free', 283), ('store', 29), ('scuffled', 6), ('member', 356), ('gang', 41), ('crips', 2), ('southern', 862), ('songs', 11), ('reflect', 25), ('gritty', 1), ('life', 172), ('streets', 88), ('blames', 32), ('economic', 740), ('calamity', 2), ('commercial', 60), ('bankers', 6), ('afghan', 834), ('president', 3452), ('hamid', 115), ('karzai', 150), ('fired', 277), ('high', 550), ('ranking', 41), ('spying', 43), ('countries', 815), ('warns', 27), ('would', 1160), ('spare', 14), ('anyone', 55), ('engages', 2), ('activity', 130), ('disclosure', 3), ('lunch', 12), ('meeting', 594), ('newly', 58), ('sworn', 36), ('parliament', 506), ('dismissed', 85), ('nor', 37), ('indicate', 61), ('action', 206), ('involved', 188), ('evidence', 136), ('against', 1257), ('even', 129), ('if', 718), ('punished', 14), ('found', 474), ('foreign', 1125), ('be', 2540), ('shown', 47), ('television', 286), ('put', 183), ('trial', 244), ('led', 657), ('afghanistan', 1043), ('taleban', 576), ('ousted', 145), ('2001', 233), ('won', 248), ('presidential', 396), ('election', 735), ('2004', 242), ('now', 391), ('waging', 14), ('insurgency', 126), ('administration', 293), ('four', 857), ('weeks', 337), ('un', 69), ('secretary', 545), ('general', 734), ('kofi', 101), ('annan', 164), ('trying', 282), ('broker', 12), ('deal', 365), ('kenyan', 64), ('government', 3262), ('mwai', 17), ('kibaki', 36), ('opposition', 586), ('raila', 4), ('odinga', 6), ('negotiations', 172), ('concentrated', 10), ('power', 543), ('sharing', 53), ('agreement', 430), ('transitional', 37), ('arrangement', 12), ('leading', 189), ('forced', 175), ('ask', 44), ('international', 1088), ('monetary', 55), ('fund', 121), ('multi', 48), ('billion', 384), ('dollar', 109), ('loan', 29), ('recent', 563), ('complimentary', 2), ('sets', 48), ('issues', 208), ('must', 285), ('addressed', 29), ('finalize', 8), ('detailed', 17), ('francois', 11), ('grignon', 1), ('director', 134), ('program', 605), ('icg', 1), ('telephone', 40), ('discuss', 268), ('stake', 19), ('voa', 237), ('reporter', 44), ('akwei', 1), ('thompson', 1), ('demonstrate', 13), ('stronger', 45), ('political', 736), ('tackle', 11), ('task', 22), ('legal', 136), ('constitutional', 126), ('reform', 144), ('needed', 137), ('transition', 35), ('because', 620), ('\x85', 4), ('\x94', 4), ('electoral', 104), ('dispute', 153), ('losers', 1), ('bush', 981), ('signed', 297), ('legislation', 100), ('require', 36), ('screening', 9), ('all', 793), ('air', 276), ('sea', 148), ('cargo', 43), ('provide', 173), ('cities', 149), ('deemed', 18), ('risk', 80), ('terrorist', 365), ('attack', 997), ('signing', 47), ('bill', 207), ('advisers', 5), ('counter', 84), ('terrorism', 299), ('homeland', 57), ('security', 1627), ('teams', 58), ('doing', 73), ('everything', 22), ('protect', 103), ('what', 569), ('called', 1023), ('dangerous', 68), ('enemy', 39), ('measures', 164), ('recommendations', 20), ('independent', 185), ('commission', 256), ('investigated', 31), ('11', 305), ('attacks', 1077), ('states', 1520), ('those', 572), ('include', 269), ('grant', 36), ('4', 254), ('given', 129), ('upgrade', 11), ('transit', 33), ('mandate', 31), ('bound', 37), ('planes', 61), ('ships', 69), ('within', 207), ('next', 626), ('years', 1021), ('burmese', 103), ('democracy', 236), ('advocate', 9), ('aung', 75), ('san', 121), ('suu', 65), ('kyi', 68), ('calling', 216), ('citizens', 155), ('her', 632), ('toward', 157), ('national', 755), ('reconciliation', 47), ('year', 2232), ('statement', 717), ('she', 558), ('asked', 194), ('struggle', 58), ('together', 108), ('strengths', 1), ('force', 432), ('words', 61), ('2011', 76), ('health', 591), ('experts', 178), ('cancer', 63), ('become', 171), ('world', 1349), ('2010', 218), ('overtaking', 2), ('disease', 201), ('65', 41), ('old', 523), ('democratic', 366), ('reforms', 153), ('burma', 319), ('released', 530), ('seven', 355), ('house', 600), ('arrest', 204), ('november', 268), ('13', 208), ('just', 311), ('rulers', 17), ('claimed', 283), ('overwhelming', 22), ('victory', 111), ('criticized', 191), ('decades', 159), ('establish', 50), ('social', 123), ('networks', 23), ('achieve', 21), ('well', 346), ('truly', 4), ('again', 158), ('leaders', 710), ('2', 481), ('200', 180), ('prisoners', 238), ('engage', 20), ('talks', 1006), ('clinton', 122), ('assembled', 7), ('activists', 155), ('academics', 4), ('address', 163), ('poverty', 109), ('warning', 143), ('conflict', 248), ('opening', 101), ('initiative', 51), ('conference', 267), ('coincides', 7), ('millennium', 22), ('assembly', 142), ('focus', 104), ('day', 942), ('secure', 59), ('concrete', 15), ('pledges', 22), ('significant', 96), ('problems', 178), ('simply', 18), ('talk', 32), ('organizers', 40), ('different', 51), ('forums', 1), ('participants', 20), ('required', 42), ('pledge', 45), ('ways', 79), ('back', 281), ('progress', 134), ('expected', 600), ('attendees', 4), ('speakers', 11), ('tony', 92), ('blair', 129), ('israel', 966), ('deputy', 180), ('shimon', 14), ('peres', 29), ('peruvian', 26), ('narrow', 23), ('gap', 30), ('candidates', 149), ('vying', 3), ('second', 484), ('spot', 21), ('ballot', 20), ('run', 352), ('tightened', 11), ('further', 201), ('organization', 461), ('issued', 297), ('factor', 17), ('behind', 121), ('growing', 150), ('deadliness', 1), ('rising', 114), ('cigarette', 6), ('smoking', 21), ('developing', 113), ('center', 268), ('alan', 19), ('garcia', 12), ('leads', 26), ('pro', 268), ('business', 181), ('congresswoman', 3), ('lourdes', 1), ('flores', 12), ('less', 132), ('96', 11), ('votes', 89), ('90', 96), ('percent', 677), ('counted', 27), ('surge', 35), ('attributed', 22), ('support', 470), ('among', 436), ('peruvians', 2), ('living', 128), ('abroad', 75), ('whose', 99), ('apparently', 76), ('starting', 47), ('impact', 59), ('tally', 14), ('candidate', 182), ('half', 217), ('vote', 506), ('april', 194), ('9', 191), ('30', 376), ('final', 242), ('results', 215), ('announced', 496), ('either', 61), ('take', 431), ('nationalist', 22), ('ollanta', 1), ('humala', 1), ('31', 103), ('chilean', 36), ('authorities', 1188), ('freed', 127), ('bail', 40), ('wife', 79), ('adult', 12), ('children', 300), ('dictator', 71), ('augusto', 12), ('pinochet', 32), ('detained', 329), ('tax', 147), ('evasion', 24), ('lucia', 7), ('hiriart', 4), ('investigation', 255), ('dollars', 158), ('kept', 43), ('bank', 479), ('accounts', 79), ('fifth', 74), ('child', 80), ('daughter', 46), ('charged', 184), ('whereabouts', 10), ('unknown', 57), ('located', 81), ('prohibited', 7), ('leaving', 114), ('indicted', 64), ('fraud', 135), ('allegedly', 118), ('hiding', 46), ('40', 233), ('smokers', 7), ('thought', 57), ('live', 88), ('china', 1026), ('alone', 29), ('faces', 114), ('human', 606), ('rights', 609), ('related', 130), ('rule', 169), ('mid', 95), ('1970s', 29), ('lawyers', 81), ('healthy', 20), ('enough', 130), ('stand', 71), ('court', 724), ('ordered', 221), ('fit', 13), ('do', 281), ('so', 377), ('rebel', 322), ('sources', 116), ('mexico', 209), ('female', 54), ('leader', 899), ('zapatista', 9), ('movement', 190), ('died', 595), ('subcomandante', 6), ('marcos', 8), ('comandante', 2), ('ramona', 2), ('saying', 785), ('lost', 143), ('fighter', 51), ('zapatistas', 2), ('piece', 23), ('announcement', 146), ('came', 392), ('stop', 299), ('chiapas', 4), ('six', 595), ('nationwide', 66), ('tour', 105), ('nature', 27), ('immediately', 154), ('clear', 212), ('rumored', 2), ('kidney', 15), ('once', 113), ('transplant', 5), ('mysterious', 4), ('tzotzil', 1), ('indian', 353), ('promoter', 3), ('women', 285), ('longtime', 25), ('appeared', 110), ('wearing', 33), ('black', 86), ('ski', 14), ('mask', 2), ('january', 391), ('emerged', 24), ('jungle', 10), ('hideout', 30), ('begin', 183), ('bid', 60), ('influence', 50), ('this', 1614), ('estimated', 127), ('12', 296), ('diagnosed', 20), ('form', 161), ('representatives', 125), ('washington', 555), ('based', 390), ('council', 473), ('american', 729), ('islamic', 419), ('relations', 240), ('appealed', 46), ('release', 306), ('kidnapped', 211), ('journalist', 119), ('jill', 17), ('carroll', 28), ('baghdad', 763), ('influential', 32), ('abductors', 16), ('unharmed', 37), ('documented', 5), ('record', 229), ('objective', 9), ('reporting', 56), ('respect', 40), ('iraqi', 1159), ('arab', 243), ('culture', 27), ('there', 823), ('word', 50), ('fate', 44), ('following', 476), ('threat', 168), ('kidnappers', 84), ('execute', 4), ('unless', 84), ('eight', 318), ('detainees', 172), ('custody', 123), ('meanwhile', 456), ('son', 87), ('senior', 232), ('defense', 495), ('ministry', 561), ('videotape', 44), ('al', 1230), ('arabiya', 9), ('threatened', 158), ('kill', 122), ('brigadier', 24), ('sabah', 8), ('abd', 3), ('karim', 5), ('forces', 1348), ('cooperating', 17), ('coalition', 496), ('muslim', 374), ('groups', 514), ('attending', 55), ('anti', 362), ('northern', 566), ('denounced', 39), ('declaration', 38), ('scholars', 8), ('clerics', 36), ('top', 537), ('seminary', 5), ('150', 72), ('darul', 1), ('uloom', 1), ('deoband', 1), ('uttar', 6), ('pradesh', 16), ('targeting', 77), ('innocent', 26), ('contradicts', 4), ('islam', 111), ('concept', 2), ('peace', 648), ('described', 102), ('religion', 25), ('mercy', 7), ('condemned', 115), ('kinds', 4), ('oppression', 3), ('violence', 759), ('pakistani', 490), ('launched', 196), ('hunt', 29), ('insurgents', 550), ('predicts', 10), ('die', 40), ('however', 274), ('ensure', 65), ('community', 169), ('harassed', 8), ('whenever', 8), ('link', 29), ('muslims', 168), ('studied', 6), ('madrassas', 5), ('religious', 155), ('schools', 83), ('spending', 130), ('lives', 114), ('bars', 13), ('having', 106), ('falsely', 1), ('acts', 29), ('terror', 151), ('gates', 47), ('norway', 76), ('pledged', 116), ('campaign', 225), ('vaccinate', 8), ('poorest', 41), ('donors', 59), ('hope', 100), ('750', 11), ('melinda', 12), ('foundation', 37), ('290', 3), ('spurs', 1), ('foundations', 4), ('governments', 127), ('invest', 16), ('alliance', 162), ('vaccines', 11), ('immunization', 4), ('hopes', 123), ('immunize', 2), ('2015', 2), ('estimates', 62), ('cost', 108), ('each', 236), ('diseases', 29), ('easily', 41), ('prevented', 39), ('basic', 44), ('spain', 180), ('begun', 112), ('24', 121), ('suspected', 539), ('qaida', 477), ('helping', 95), ('plan', 439), ('defendants', 51), ('madrid', 32), ('bulletproof', 1), ('glass', 11), ('predicted', 30), ('number', 475), ('soon', 139), ('greater', 84), ('aids', 114), ('tuberculosis', 12), ('malaria', 29), ('combined', 37), ('armed', 236), ('providing', 76), ('remodeled', 1), ('courtroom', 13), ('specially', 4), ('built', 68), ('trials', 30), ('multiple', 27), ('spanish', 133), ('cell', 55), ('imad', 5), ('eddin', 1), ('barakat', 2), ('yarkas', 1), ('organized', 53), ('suspects', 293), ('details', 173), ('finalized', 12), ('prosecutors', 131), ('asking', 47), ('sentenced', 96), ('60', 164), ('prison', 311), ('convicted', 110), ('european', 740), ('union', 671), ('observers', 52), ('preparing', 63), ('monitor', 53), ('palestinian', 1055), ('parliamentary', 270), ('west', 481), ('gaza', 559), ('strip', 253), ('despite', 254), ('kidnappings', 48), ('foreigners', 63), ('chief', 466), ('eu', 374), ('observer', 11), ('veronique', 1), ('de', 133), ('keyser', 2), ('making', 194), ('regular', 32), ('assessments', 2), ('taking', 199), ('necessary', 74), ('precautions', 10), ('commitment', 48), ('met', 336), ('adding', 30), ('bad', 55), ('sign', 99), ('send', 93), ('monitors', 53), ('workers', 410), ('ms', 179), ('spoke', 132), ('reporters', 329), ('jerusalem', 99), ('headed', 59), ('towns', 80), ('campaigning', 22), ('delay', 85), ('citing', 48), ('dire', 4), ('law', 286), ('order', 170), ('conditions', 135), ('refusal', 26), ('permit', 19), ('voting', 97), ('east', 377), ('patients', 50), ('may', 652), ('rise', 92), ('2030', 2), ('17', 193), ('dying', 14), ('delegation', 100), ('monitored', 16), ('liberia', 39), ('concerned', 94), ('act', 102), ('responsibly', 2), ('post', 258), ('process', 244), ('stays', 2), ('track', 47), ('jimmy', 7), ('carter', 24), ('benin', 21), ('nicephore', 2), ('soglo', 2), ('urged', 251), ('continue', 314), ('added', 142), ('extensive', 34), ('voter', 29), ('education', 111), ('runoff', 43), ('problem', 64), ('poll', 125), ('widespread', 82), ('understanding', 20), ('casting', 13), ('ballots', 58), ('cited', 41), ('ignorance', 2), ('choice', 24), ('joint', 173), ('institute', 33), ('includes', 121), ('europe', 275), ('america', 173), ('remarks', 87), ('gathered', 113), ('main', 266), ("shi'ite", 337), ('mosque', 118), ('kabul', 152), ('referred', 40), ('wants', 161), ('our', 45), ('destruction', 66), ('sheds', 2), ('refer', 26), ('similar', 152), ('offers', 20), ('rebuffed', 2), ('civil', 254), ('liberties', 23), ('obtained', 32), ('documents', 70), ('tried', 154), ('suppress', 6), ('reports', 738), ('abuse', 100), ('saw', 70), ('dramatic', 10), ('increase', 236), ('nato', 529), ('thousand', 73), ('coming', 115), ('months', 438), ('snows', 2), ('thaw', 7), ('mountain', 31), ('accessible', 2), ('french', 425), ('nine', 210), ('paris', 124), ('normandy', 3), ('crackdown', 100), ('planning', 121), ('france', 322), ('links', 87), ('algerian', 32), ('salafist', 6), ('combat', 110), ('gspc', 1), ('declared', 161), ('allegiance', 9), ('detainee', 23), ('escorted', 6), ('interrogation', 26), ('us', 210), ('guards', 100), ('camp', 186), ('ray', 13), ('guantanamo', 100), ('bay', 71), ('naval', 69), ('base', 239), ('pentagon', 60), ('cuba', 237), ('home', 421), ('tribunal', 125), ('determined', 42), ('combatants', 9), ('sent', 208), ('sudan', 375), ('another', 574), ('saudi', 210), ('arabia', 110), ('third', 319), ('jordan', 94), ('cleared', 32), ('types', 12), ('administrative', 26), ('review', 90), ('staff', 112), ('intelligence', 276), ('dia', 2), ('witnessed', 10), ('incidents', 82), ('assaulted', 8), ('deprived', 4), ('sleep', 4), ('humiliated', 1), ('eighth', 21), ('moroccan', 28), ('transferred', 48), ('yemen', 47), ('low', 153), ('income', 98), ('highly', 69), ('dependent', 40), ('declining', 42), ('oil', 1192), ('resources', 106), ('revenue', 63), ('petroleum', 74), ('roughly', 37), ('gdp', 182), ('70', 136), ('effects', 28), ('diversifying', 4), ('initiated', 12), ('2006', 210), ('designed', 68), ('bolster', 18), ('non', 176), ('sectors', 65), ('investment', 162), ('2009', 209), ('exported', 10), ('liquefied', 1), ('natural', 165), ('gas', 315), ('diversification', 7), ('effort', 227), ('established', 78), ('friends', 40), ('aims', 22), ('efforts', 420), ('towards', 58), ('august', 258), ('imf', 71), ('approved', 233), ('370', 5), ('these', 91), ('ambitious', 16), ('endeavors', 1), ('continues', 148), ('face', 227), ('difficult', 61), ('term', 269), ('challenges', 63), ('water', 232), ('population', 141), ('growth', 349), ('rate', 143), ('singapore', 49), ('founded', 31), ('trading', 114), ('colony', 33), ('1819', 2), ('joined', 116), ('malaysian', 20), ('federation', 52), ('1963', 9), ('separated', 14), ('became', 180), ('subsequently', 25), ('prosperous', 20), ('port', 143), ('busiest', 7), ('terms', 66), ('tonnage', 1), ('handled', 20), ('per', 174), ('capita', 34), ('equal', 21), ('included', 107), ('complaints', 36), ('personnel', 104), ('e', 81), ('mails', 16), ('special', 176), ('ruled', 159), ('thani', 3), ('family', 195), ('1800s', 1), ('qatar', 36), ('transformed', 10), ('itself', 62), ('poor', 179), ('protectorate', 14), ('noted', 45), ('mainly', 89), ('pearling', 1), ('revenues', 59), ('1980s', 53), ('1990s', 82), ('qatari', 2), ('crippled', 8), ('continuous', 5), ('siphoning', 4), ('amir', 13), ('1972', 12), ('current', 173), ('hamad', 5), ('bin', 101), ('khalifa', 3), ('overthrew', 18), ('him', 677), ('bloodless', 10), ('coup', 99), ('1995', 90), ('resolved', 36), ('longstanding', 6), ('border', 647), ('disputes', 37), ('bahrain', 25), ('2007', 186), ('enabled', 8), ('attain', 1), ('highest', 118), ('subsistence', 31), ('agriculture', 129), ('forestry', 10), ('remains', 217), ('backbone', 2), ('central', 483), ('african', 454), ('republic', 263), ('car', 408), ('outlying', 2), ('agricultural', 95), ('sector', 212), ('generates', 3), ('timber', 15), ('accounted', 12), ('16', 177), ('export', 106), ('earnings', 51), ('diamond', 16), ('industry', 169), ('important', 130), ('constraints', 4), ('development', 302), ('landlocked', 14), ('position', 71), ('transportation', 40), ('largely', 103), ('unskilled', 2), ('legacy', 5), ('misdirected', 1), ('macroeconomic', 15), ('policies', 123), ('factional', 16), ('fighting', 594), ('opponents', 70), ('drag', 6), ('revitalization', 1), ('aclu', 1), ('federal', 221), ('comply', 23), ('request', 112), ('freedom', 112), ('information', 312), ('distribution', 43), ('extraordinarily', 3), ('unequal', 4), ('grants', 17), ('only', 485), ('partially', 9), ('meet', 351), ('humanitarian', 118), ('needs', 92), ('peasant', 4), ('eagle', 31), ('captured', 191), ('trap', 8), ('much', 237), ('admiring', 1), ('bird', 530), ('set', 478), ('prove', 19), ('ungrateful', 1), ('deliverer', 1), ('seeing', 40), ('sitting', 14), ('wall', 62), ('safe', 67), ('flew', 34), ('talons', 6), ('snatched', 6), ('bundle', 2), ('head', 324), ('rose', 121), ('pursuit', 12), ('let', 56), ('fall', 89), ('up', 1108), ('man', 411), ('same', 235), ('find', 99), ('fallen', 38), ('pieces', 17), ('marveled', 1), ('service', 252), ('rendered', 2), ('ass', 34), ('fox', 96), ('entered', 103), ('partnership', 36), ('mutual', 12), ('protection', 74), ('went', 176), ('forest', 12), ('proceeded', 6), ('far', 179), ('lion', 55), ('imminent', 9), ('danger', 28), ('approached', 18), ('promised', 122), ('contrive', 3), ('capture', 47), ('harm', 23), ('then', 258), ('upon', 90), ('assuring', 5), ('deep', 51), ('pit', 1), ('arranged', 10), ('should', 384), ('georgian', 52), ('zurab', 3), ('zhvania', 5), ('supports', 41), ('ukraine', 212), ('upcoming', 80), ('ukrainian', 98), ('fair', 77), ('secured', 15), ('clutched', 1), ('attacked', 216), ('leisure', 3), ('never', 108), ('trust', 30), ('your', 68), ('mathematicians', 1), ('lose', 22), ('functions', 7), ('nostalgia', 1), ('grammar', 1), ('lesson', 4), ('you', 204), ('present', 76), ('past', 348), ('perfect', 7), ('my', 64), ('grandfather', 3), ('worked', 74), ('blacksmith', 1), ('shop', 14), ('boy', 63), ('used', 362), ('tell', 30), ('me', 41), ('how', 241), ('toughened', 1), ('himself', 142), ('could', 789), ('rigors', 1), ('blacksmithing', 1), ('outside', 320), ('5', 393), ('pound', 15), ('potato', 4), ('sack', 3), ('hand', 99), ('extend', 52), ('arms', 143), ('straight', 49), ('sides', 156), ('hold', 250), ('sacks', 4), ('finally', 33), ('got', 44), ('lift', 34), ('minutes', 59), ('eventually', 38), ('started', 87), ('putting', 34), ('potatoes', 1), ('advance', 42), ('copies', 9), ("'", 415), ('internal', 63), ('audit', 11), ('reportedly', 62), ('wasted', 2), ('overlooked', 1), ('overcharges', 1), ('contractors', 32), ('published', 155), ('audits', 1), ('criticize', 14), ('aide', 41), ('benon', 3), ('sevan', 8), ('office', 331), ('ran', 31), ('currently', 141), ('visiting', 77), ('comment', 112), ('voice', 56), ('portions', 15), ('times', 172), ('associated', 135), ('press', 229), ('reveal', 14), ('systematic', 5), ('corruption', 212), ('bribery', 6), ('named', 139), ('panel', 77), ('investigate', 56), ('allegations', 164), ('billions', 31), ('diverted', 8), ('saddam', 193), ('hussein', 136), ('regime', 89), ('corrupt', 15), ('1996', 65), ('help', 543), ('gulf', 162), ('volcker', 6), ('heads', 61), ('cut', 217), ('wrongdoing', 21), ('airstrikes', 35), ('sites', 66), ('artillery', 40), ('soldiers', 769), ('53', 37), ('wounded', 707), ('jaffna', 13), ('peninsula', 71), ('tamil', 84), ('tiger', 52), ('rebels', 698), ('provoked', 7), ('fire', 427), ('strikes', 113), ('lankan', 47), ('ship', 112), ('evacuate', 19), ('800', 50), ('civilians', 316), ('trapped', 42), ('area', 501), ('stranded', 23), ('expressed', 207), ('satisfaction', 3), ('visit', 439), ('began', 438), ('great', 93), ('interest', 121), ('georgia', 128), ('separate', 284), ('tens', 127), ('white', 319), ('pink', 4), ('bathed', 1), ('lighting', 7), ('attention', 55), ('fight', 169), ('breast', 13), ('barack', 98), ('obama', 244), ('networking', 6), ('site', 213), ('twitter', 13), ('executive', 83), ('mansion', 4), ('lit', 3), ('evening', 53), ('recognition', 25), ('awareness', 20), ('proclamation', 8), ('numerous', 45), ('aimed', 264), ('large', 290), ('ribbon', 1), ('hung', 5), ('society', 41), ('annually', 30), ('claims', 108), ('19', 120), ('worldwide', 110), ('someone', 25), ('dies', 6), ('traffic', 43), ('accident', 84), ('average', 66), ('every', 109), ('seconds', 42), ('level', 159), ('advisor', 34), ('condoleezza', 99), ('rice', 231), ('conclusion', 15), ('road', 131), ('safety', 99), ('priority', 24), ('2008', 253), ('plans', 376), ('issue', 174), ('amid', 105), ('calls', 252), ('tetiana', 2), ('koprowicz', 2), ('improve', 92), ('diplomat', 79), ('ismail', 37), ('haniyeh', 22), ('case', 251), ('bbc', 23), ('johnston', 8), ('consul', 2), ('richard', 38), ('makepeace', 1), ('hamas', 437), ('kidnapping', 65), ('boycott', 60), ('represent', 26), ('change', 166), ('policy', 201), ('focused', 38), ('resolution', 127), ('permanently', 11), ('time', 584), ('abduction', 23), ('quoted', 96), ('recognizes', 19), ('territorial', 35), ('integrity', 16), ('regards', 11), ('abkhazia', 26), ('russian', 556), ('enclave', 28), ('own', 180), ('affairs', 104), ('agreed', 316), ('missile', 172), ('range', 87), ('ballistic', 20), ('missiles', 106), ('short', 118), ('rockets', 92), ('robert', 94), ('counterpart', 67), ('ehud', 52), ('barak', 6), ('committee', 211), ('proposed', 142), ('spokesman', 732), ('geoff', 4), ('morrell', 1), ('able', 112), ('possibly', 30), ('iran', 1311), ('territories', 60), ('facing', 92), ('withdrawing', 37), ('territory', 194), ('2005', 178), ('venezuelan', 244), ('hugo', 154), ('chavez', 370), ('brakes', 1), ('socialist', 41), ('revolution', 50), ('voters', 125), ('rejected', 211), ('constitution', 278), ('weekly', 72), ('broadcast', 103), ('mistake', 26), ('try', 110), ('quicken', 1), ('pace', 27), ('turn', 58), ('venezuela', 368), ('haven', 17), ('evaluate', 1), ('referendum', 163), ('deciding', 9), ('proceed', 11), ('regional', 180), ('way', 237), ('consolidate', 7), ('party', 868), ('turned', 124), ('down', 542), ('package', 57), ('belarus', 45), ('criminal', 96), ('probes', 5), ('18', 195), ('over', 1304), ('alleged', 249), ('riots', 49), ('concludes', 6), ('vesna', 1), ('authoritarian', 10), ('15', 355), ('overall', 67), ('belarusian', 25), ('jailed', 60), ('wake', 37), ('protests', 288), ('re', 161), ('alexander', 43), ('lukashenko', 39), ('fourth', 122), ('consecutive', 25), ('capturing', 9), ('80', 147), ('polls', 112), ('closed', 126), ('fraudulent', 18), ('strongly', 62), ('nominee', 38), ('confirmation', 39), ('hearings', 28), ('heavily', 94), ('repairing', 6), ('alliances', 7), ('strained', 32), ('senate', 171), ('question', 48), ('hours', 219), ('nomination', 53), ('succeed', 31), ('colin', 24), ('powell', 43), ('spent', 81), ('testifying', 4), ('handling', 37), ('tough', 28), ('questions', 37), ('direction', 17), ('decision', 273), ('invade', 8), ('korean', 272), ('japanese', 219), ('protesters', 219), ('seoul', 46), ('stopping', 29), ('mock', 3), ('funeral', 69), ('junichiro', 26), ('koizumi', 43), ('steadfastly', 2), ('maintained', 29), ('believes', 63), ('declined', 97), ('give', 205), ('timetable', 28), ('goals', 32), ('spreading', 37), ('around', 307), ('globe', 11), ('building', 233), ('kashmir', 201), ('involving', 66), ('gunbattles', 11), ('erupted', 83), ('raided', 60), ('udhampur', 2), ('poonch', 3), ('districts', 36), ('clashes', 141), ('along', 306), ('incident', 272), ('youths', 21), ('crossfire', 5), ('battled', 17), ('kupwara', 4), ('district', 234), ('civilian', 190), ('residents', 207), ('manmohan', 39), ('singh', 79), ('kashmiri', 29), ('prominent', 66), ('separatists', 53), ('attend', 98), ('fast', 40), ('chain', 16), ('kfc', 5), ('famous', 25), ('fried', 4), ('chicken', 22), ('hundreds', 296), ('surrounded', 35), ('carried', 222), ('empty', 14), ('coffin', 13), ('picture', 24), ('park', 30), ('near', 912), ('embassy', 205), ('kentucky', 3), ('harlan', 1), ('david', 66), ('sanders', 4), ('better', 107), ('know', 51), ('patrons', 1), ('colonel', 44), ('restaurant', 21), ('brisk', 2), ('serves', 11), ('kabobs', 1), ('pizza', 1), ('alongside', 22), ('rahimgul', 1), ('sarawan', 1), ('inspired', 13), ('association', 98), ('ends', 27), ('brian', 15), ('narrates', 15), ('approve', 63), ('superpower', 2), ('benjamin', 22), ('netanyahu', 32), ('sales', 130), ('locally', 10), ('platforms', 4), ('newspaper', 337), ('increased', 195), ('research', 79), ('focusing', 24), ('mini', 5), ('satellites', 13), ('primary', 43), ('market', 249), ('quotes', 165), ('division', 29), ('capability', 11), ('carve', 1), ('total', 139), ('250', 45), ('companies', 223), ('possibility', 41), ('collaboration', 8), ('burned', 51), ('effigy', 3), ('ambassador', 175), ('toshiyuki', 1), ('takano', 1), ('front', 133), ('residence', 26), ('direct', 84), ('fell', 153), ('slightly', 53), ('commerce', 45), ('slipped', 9), ('0', 90), ('22', 117), ('contracted', 39), ('gives', 39), ('indication', 17), ('future', 197), ('inflows', 11), ('grew', 52), ('88', 23), ('64', 42), ('97', 12), ('although', 136), ('build', 152), ('investments', 37), ('slowing', 15), ('2003', 344), ('outbreak', 178), ('acute', 10), ('respiratory', 10), ('syndrome', 5), ('spring', 23), ('43', 25), ('qalat', 2), ('zabul', 37), ('patrol', 131), ('ambushed', 55), ('sniper', 9), ('machine', 22), ('guns', 25), ('weapons', 526), ('locations', 19), ('small', 255), ('propelled', 22), ('grenades', 41), ('flaming', 2), ('arrows', 1), ('failed', 214), ('nepal', 135), ('maoist', 76), ('ban', 204), ('kathmandu', 43), ('disrupted', 28), ('city', 1120), ('pleas', 4), ('mass', 145), ('demonstration', 40), ('demanding', 110), ('end', 466), ('increasingly', 55), ('bloody', 34), ('imposed', 112), ('killings', 86), ('escalated', 12), ('ahead', 255), ('deadline', 65), ('sher', 8), ('bahadur', 3), ('deuba', 3), ('maoists', 24), ('resume', 124), ('replace', 87), ('monarchy', 46), ('communist', 153), ('congressman', 30), ('tom', 27), ('conspiracy', 30), ('charge', 137), ('reinstated', 13), ('majority', 223), ('republican', 100), ('speaking', 188), ('indictment', 27), ('manufactured', 17), ('frivolous', 4), ('lawmaker', 48), ('longer', 63), ('comfortable', 4), ('sentiment', 11), ('runs', 58), ('controlled', 97), ('tokyo', 89), ('approval', 106), ('history', 87), ('books', 15), ('critics', 95), ('downplay', 2), ('japan', 371), ('wartime', 14), ('atrocities', 24), ('cnn', 37), ('christopher', 29), ('shays', 1), ('continual', 1), ('sometimes', 25), ('go', 184), ('beyond', 27), ('ethical', 8), ('stepped', 89), ('grand', 50), ('jury', 31), ('texas', 94), ('violating', 37), ('finance', 80), ('allegation', 7), ('centers', 61), ('misuse', 10), ('corporate', 11), ('donations', 25), ('rome', 43), ('discussed', 70), ('prices', 418), ('trade', 430), ('barriers', 15), ('reduced', 58), ('bans', 18), ('lifted', 43), ('commodities', 16), ('doubled', 14), ('corn', 6), ('wheat', 14), ('highs', 27), ('philippines', 45), ('haiti', 182), ('hard', 108), ('hit', 370), ('filipino', 4), ('haitian', 73), ('immigrants', 64), ('sending', 79), ('families', 110), ('deborah', 6), ('block', 59), ('story', 52), ('harder', 12), ('arabic', 28), ('network', 150), ('jazeera', 53), ('operate', 29), ('considering', 74), ('unmanned', 23), ('look', 40), ('allied', 35), ('renew', 22), ('visas', 9), ('employees', 83), ('conferences', 4), ('briefings', 5), ('tied', 25), ('move', 250), ('owns', 15), ('suspend', 50), ('obstacles', 13), ('closing', 43), ('doha', 10), ('ties', 181), ('municipal', 26), ('numbers', 44), ('threats', 84), ('separatist', 92), ('sporadic', 15), ('srinagar', 25), ('burnt', 1), ('tires', 6), ('pelted', 6), ('stones', 19), ('chose', 18), ('town', 523), ('councils', 17), ('stages', 16), ('29', 66), ('stage', 48), ('raul', 36), ('baduel', 2), ('caracas', 71), ('pilotless', 1), ('scores', 43), ('eastern', 403), ('bombing', 220), ('northwestern', 103), ('bombs', 127), ('crowded', 35), ('explosions', 71), ('almost', 148), ('daily', 105), ('blasts', 68), ('across', 319), ('officers', 272), ('sunni', 210), ('cleric', 85), ('clash', 104), ('backed', 184), ('helmand', 112), ('soldier', 251), ('fierce', 31), ('sangin', 6), ('dozens', 124), ('stormed', 47), ('checkpoint', 79), ('maintaining', 22), ('aging', 16), ('f', 26), ('jets', 47), ('positions', 66), ('dropping', 25), ('hotbed', 3), ('insurgent', 141), ('line', 121), ('islamist', 110), ('11th', 21), ('russia', 605), ('lower', 118), ('duma', 17), ('ratified', 19), ('treaties', 12), ('breakaway', 35), ('regions', 88), ('ossetia', 20), ('endorsements', 3), ('moscow', 216), ('keep', 129), ('lawmakers', 297), ('voted', 138), ('unanimously', 18), ('friendship', 9), ('formalize', 2), ('diplomatic', 130), ('recognized', 32), ('shortly', 86), ('swept', 45), ('reclaim', 6), ('invasion', 86), ('drew', 28), ('condemnations', 2), ('respond', 47), ('sanctions', 238), ('station', 208), ('sergei', 37), ('lavrov', 22), ('opposes', 33), ('deployment', 52), ('assume', 15), ('responsibility', 259), ('trouble', 26), ('blocking', 29), ('stem', 35), ('therapy', 5), ('successfully', 34), ('treated', 51), ('leukemia', 3), ('cancers', 1), ('bone', 3), ('marrow', 1), ('transplants', 2), ('study', 88), ('journal', 28), ('jama', 2), ('finds', 13), ('cells', 15), ('autoimmune', 1), ('alex', 4), ('villarreal', 1), ('forming', 35), ('rival', 117), ('labor', 177), ('gain', 59), ('pullout', 39), ('palestinians', 228), ('overnight', 58), ('egypt', 270), ('opened', 217), ('moving', 76), ('forbidden', 6), ('rafah', 22), ('men', 505), ('smugglers', 19), ('terrorists', 165), ('shot', 259), ('spotted', 17), ('hospital', 244), ('nuclear', 1104), ('stalled', 45), ('negotiators', 61), ('abu', 154), ('musab', 48), ('zarqawi', 72), ('holy', 89), ('claim', 116), ('web', 66), ('gunmen', 313), ('amer', 5), ('nayef', 1), ('officer', 159), ('dora', 2), ('gunned', 24), ('provincial', 114), ('governor', 143), ('bodyguards', 33), ('rode', 6), ('elsewhere', 131), ('armored', 24), ('struck', 129), ('roadside', 237), ('separately', 104), ('suicide', 420), ('bomber', 215), ('rammed', 18), ('forthcoming', 1), ('marks', 44), ('beginning', 83), ('phase', 25), ('responsible', 118), ('dialogue', 69), ("shi'ites", 48), ('arabs', 42), ('kurds', 53), ('difference', 6), ('success', 35), ('failure', 69), ('increasing', 81), ('ongoing', 73), ('violations', 56), ('pose', 20), ('key', 262), ('prepare', 40), ('positive', 85), ('determination', 10), ('quarterly', 8), ('beset', 4), ('formidable', 2), ('tehran', 340), ('kamal', 16), ('kharrazi', 11), ('always', 28), ('sought', 65), ('suspension', 41), ('uranium', 177), ('enrichment', 122), ('activities', 173), ('germany', 280), ('pressing', 33), ('pleaded', 58), ('hostages', 97), ('egyptian', 178), ('construction', 151), ('company', 420), ('bus', 120), ('driver', 80), ('busload', 1), ('wounding', 144), ('cairo', 96), ('firm', 87), ('mahmoud', 235), ('sweilam', 2), ('veteran', 16), ('surrendered', 30), ('deadly', 249), ('rampage', 10), ('driving', 48), ('suddenly', 15), ('stopped', 78), ('shooting', 92), ('automatic', 7), ('rifle', 12), ('investigating', 117), ('motive', 22), ('employers', 11), ('71', 13), ('jobs', 114), ('july', 285), ('indicating', 8), ('slow', 70), ('recover', 42), ('recession', 77), ('department', 377), ('shows', 114), ('stayed', 13), ('unchanged', 9), ('economists', 46), ('closely', 46), ('watch', 90), ('payrolls', 2), ('figure', 66), ('bulk', 15), ('hiring', 5), ('employment', 31), ('big', 38), ('indicator', 2), ('consumer', 88), ('unemployed', 7), ('worried', 28), ('job', 79), ('stability', 92), ('tend', 2), ('spend', 25), ('suspended', 116), ('good', 139), ('faith', 21), ('gesture', 17), ('decide', 50), ('whether', 221), ('due', 209), ('cuts', 68), ('census', 2), ('bureau', 49), ('44', 25), ('temporary', 43), ('hired', 11), ('couple', 35), ('conduct', 63), ('decade', 97), ('count', 30), ('invited', 35), ('join', 116), ('offering', 54), ('inexpensive', 4), ('caribbean', 56), ('offer', 124), ('elect', 67), ('rene', 21), ('preval', 42), ('inclusion', 7), ('petrocaribe', 2), ('generous', 5), ('payment', 25), ('options', 26), ('purchase', 30), ('donate', 12), ('diesel', 13), ('fuel', 249), ('use', 285), ('hospitals', 44), ('elected', 153), ('promises', 23), ('haitians', 10), ('inaugurated', 11), ('batons', 11), ('dispersed', 8), ('tibetan', 55), ('refugees', 114), ('monks', 16), ('chinese', 466), ('enriched', 29), ('loaded', 14), ('trucks', 41), ('vans', 1), ('detention', 132), ('demonstrations', 104), ('tibet', 61), ('lhasa', 13), ('400', 70), ('deeply', 26), ('arbitrary', 5), ('arrests', 75), ('detentions', 14), ('himalayas', 3), ('route', 40), ('tibetans', 16), ('fleeing', 23), ('21', 124), ('mostly', 156), ('policeman', 50), ('cabinet', 189), ('suhaila', 1), ('abed', 4), ('jaafar', 2), ('displacement', 3), ('migration', 7), ('escaped', 69), ('unhurt', 9), ('jack', 26), ('straw', 33), ('jalal', 46), ('talabani', 62), ('dominated', 73), ('atomic', 153), ('energy', 421), ('gholamreza', 3), ('aghazadeh', 4), ('europeans', 26), ('speed', 41), ('ibrahim', 50), ('jaafari', 27), ('choosing', 11), ('affair', 8), ('jordanian', 47), ('hostage', 72), ('studies', 20), ('tightly', 6), ('managing', 14), ('sugar', 40), ('helps', 13), ('diabetics', 2), ('complications', 4), ('type', 24), ('diabetes', 16), ('funded', 30), ('juvenile', 3), ('carol', 17), ('pearson', 16), ('forensic', 9), ('bosnia', 77), ('herzegovina', 40), ('exhuming', 2), ('grave', 43), ('contain', 53), ('bodies', 168), ('36', 50), ('balkan', 16), ('eyewitnesses', 3), ('bosnian', 107), ('serb', 98), ('victims', 274), ('garage', 2), ('village', 160), ('snagovo', 1), ('zvornik', 3), ('corpses', 8), ('buried', 45), ('already', 219), ('exhumed', 4), ('graves', 12), ('finding', 56), ('300', 111), ('skeletons', 2), ('today', 247), ('scientific', 22), ('achievements', 9), ('boys', 30), ('massacred', 2), ('srebrenica', 27), ('bandits', 7), ('taken', 249), ('southeast', 122), ('blocked', 52), ('vehicles', 97), ('abducting', 8), ('sistan', 3), ('baluchestan', 1), ('few', 150), ('available', 56), ('motorcycle', 24), ('riders', 6), ('parade', 25), ('rolling', 4), ('thunder', 3), ('memorial', 46), ('holiday', 99), ('honoring', 12), ('battle', 101), ('bikers', 1), ('vietnam', 93), ('veterans', 15), ('gathering', 51), ('lincoln', 4), ('musical', 15), ('tribute', 10), ('pop', 27), ('revere', 2), ('raiders', 4), ('singer', 48), ('nancy', 8), ('sinatra', 1), ('event', 103), ('specifically', 20), ('honors', 9), ('seeded', 48), ('lleyton', 4), ('hewitt', 6), ('australia', 139), ('dominik', 1), ('hrbaty', 2), ('slovakia', 26), ('advanced', 40), ('round', 162), ('hardcourt', 5), ('tennis', 65), ('championships', 20), ('adelaide', 2), ('coast', 300), ('louisiana', 30), ('braces', 1), ('onslaught', 2), ('hurricane', 262), ('katrina', 139), ('landfall', 17), ('dropped', 102), ('bouncing', 2), ('beat', 50), ('hernych', 2), ('czech', 69), ('04', 16), ('jun', 50), ('06', 136), ('feb', 56), ('apr', 47), ('easier', 24), ('topping', 3), ('rameez', 1), ('junaid', 2), ('mar', 55), ('07', 46), ('matches', 20), ('players', 45), ('james', 45), ('blake', 8), ('defeated', 62), ('alberto', 25), ('martin', 34), ('juan', 22), ('ignacio', 3), ('chela', 3), ('argentina', 73), ('upset', 25), ('losing', 19), ('ivo', 7), ('karlovic', 5), ('croatia', 64), ('03', 22), ('unseeded', 5), ('advancing', 14), ('mark', 82), ('philippoussis', 2), ('florian', 4), ('mayer', 8), ('andy', 12), ('murray', 7), ('andreas', 4), ('seppi', 2), ('italy', 133), ('frenchman', 6), ('florent', 2), ('serra', 3), ('jarkko', 4), ('nieminen', 5), ('finland', 41), ('danish', 56), ('player', 50), ('kenneth', 4), ('carlsen', 3), ('amnesty', 88), ('hiv', 72), ('devastating', 46), ('gender', 7), ('inequality', 8), ('contributing', 9), ('factors', 11), ('annie', 1), ('lennox', 1), ('highlighting', 10), ('charity', 31), ('tune', 3), ('sing', 6), ('trip', 183), ('learned', 17), ('affects', 11), ('especially', 61), ('pregnant', 7), ('mothers', 12), ('unborn', 3), ('does', 214), ('mandy', 4), ('clark', 11), ('authorizes', 8), ('coordinate', 20), ('disaster', 90), ('appropriate', 14), ('parishes', 1), ('drug', 246), ('raid', 139), ('athletes', 14), ('olympic', 158), ('games', 118), ('italian', 119), ('seized', 197), ('materials', 49), ('surprise', 26), ('sweep', 16), ('quarters', 22), ('austrian', 25), ('biathlon', 6), ('cross', 140), ('searched', 19), ('residences', 2), ('conducted', 76), ('unannounced', 8), ('competition', 41), ('tests', 129), ('skiers', 2), ('biathletes', 1), ('consistent', 9), ('doping', 20), ('laws', 85), ('treat', 16), ('offense', 8), ('probe', 133), ('equipment', 78), ('austria', 49), ('connected', 17), ('walter', 13), ('banned', 91), ('ioc', 18), ('turin', 31), ('olympics', 75), ('vancouver', 6), ('suspicion', 38), ('performing', 15), ('transfusions', 1), ('2002', 186), ('salt', 7), ('lake', 19), ('coach', 16), ('existence', 16), ('militias', 83), ('incompatible', 3), ('restoring', 20), ('sovereignty', 62), ('domination', 6), ('envoy', 92), ('terje', 6), ('roed', 11), ('larsen', 11), ('inability', 9), ('exert', 5), ('control', 356), ('rein', 6), ('stalling', 2), ('implementing', 18), ('1559', 3), ('disarming', 13), ('hezbollah', 137), ('32', 61), ('neighboring', 195), ('mississippi', 22), ('preparation', 14), ('storm', 230), ('evacuations', 5), ('lying', 22), ('delivered', 43), ('deployed', 78), ('camps', 54), ('hills', 5), ('loud', 5), ('leave', 145), ('surrender', 32), ('army', 516), ('contractor', 22), ('delhi', 86), ('17th', 34), ('century', 166), ('red', 102), ('fort', 19), ('ago', 346), ('muhammad', 47), ('arif', 2), ('handed', 68), ('sentence', 70), ('role', 188), ('2000', 117), ('sneaked', 1), ('complex', 37), ('palace', 24), ('mughal', 3), ('emperor', 15), ('shah', 24), ('jehan', 1), ('symbol', 12), ('independence', 286), ('speeches', 13), ('pronouncements', 1), ('formally', 87), ('extended', 65), ('informed', 33), ('congress', 238), ('poses', 10), ('continuing', 85), ('rigs', 5), ('evacuated', 34), ('atlantic', 48), ('season', 115), ('churned', 1), ('warm', 15), ('waters', 73), ('actions', 67), ('hostile', 37), ('interests', 47), ('existing', 30), ('renewed', 55), ('expire', 19), ('isolated', 22), ('assistant', 36), ('kurt', 6), ('campbell', 8), ('visited', 118), ('junta', 34), ('effectively', 17), ('exclude', 5), ('league', 80), ('accepting', 10), ('requests', 19), ('h1', 2), ('b', 27), ('specialty', 4), ('quota', 18), ('reached', 165), ('immigration', 63), ('services', 176), ('visa', 25), ('wait', 23), ('until', 357), ('allows', 45), ('hire', 7), ('skilled', 12), ('skills', 14), ('scientists', 97), ('engineers', 33), ('computer', 43), ('programmers', 1), ('granted', 58), ('normally', 13), ('185', 9), ('kilometer', 52), ('winds', 76), ('moved', 74), ('slowly', 33), ('northwest', 113), ('decides', 5), ('addition', 61), ('additional', 92), ('permits', 18), ('degree', 10), ('master', 11), ('doctorate', 3), ('college', 30), ('university', 68), ('taiwan', 191), ('chen', 43), ('shui', 19), ('bian', 21), ('strait', 13), ('willing', 52), ('guarantee', 11), ('appeal', 94), ('create', 100), ('code', 23), ('overtures', 3), ('speak', 40), ('taiwanese', 14), ('agrees', 10), ('inseparable', 3), ('seize', 20), ('makes', 60), ('moves', 43), ('diplomats', 122), ('allies', 95), ('push', 75), ('possible', 254), ('condition', 108), ('anonymity', 12), ('persuade', 13), ('compromise', 25), ('board', 123), ('governors', 15), ('vienna', 58), ('slammed', 14), ('florida', 82), ('accuses', 63), ('seeking', 166), ('denies', 116), ('obligations', 11), ('allow', 219), ('inspectors', 42), ('facilities', 128), ('bar', 16), ('visits', 52), ('refugee', 93), ('sparking', 22), ('shootout', 32), ('badly', 23), ('spokeswoman', 95), ('balata', 2), ('nablus', 23), ('pre', 56), ('dawn', 23), ('operation', 260), ('enforcing', 4), ('displays', 7), ('step', 112), ('imposing', 19), ('bloodshed', 13), ('finished', 53), ('local', 420), ('seen', 154), ('test', 141), ('clout', 2), ('unofficial', 9), ('ruling', 256), ('fatah', 151), ('abbas', 246), ('61', 18), ('104', 11), ('completed', 68), ('suppressing', 6), ('lead', 175), ('withdraw', 92), ('jericho', 16), ('marines', 77), ('operations', 275), ('iraqis', 127), ('kurdish', 231), ('sunnis', 24), ('sky', 9), ('ready', 111), ('depends', 31), ('rapidly', 31), ('infected', 108), ('virus', 353), ('spread', 141), ('provinces', 95), ('autonomous', 33), ('injected', 4), ('transmission', 17), ('situation', 185), ('exists', 7), ('malaysia', 48), ('warnings', 23), ('shigeru', 1), ('omi', 2), ('asian', 159), ('500', 160), ('records', 38), ('cambodia', 33), ('percentage', 23), ('enjoying', 8), ('christmas', 84), ('none', 28), ('appreciate', 1), ('folks', 1), ('victoria', 8), ('usually', 37), ('balmy', 1), ('unprecedented', 24), ('centimeters', 30), ('snow', 59), ('weather', 123), ('measurable', 2), ('snowfall', 5), ('1973', 18), ('enjoyed', 12), ('1917', 8), ('86', 9), ('snowman', 1), ('forecast', 20), ('sunny', 5), ('skies', 7), ('temperatures', 42), ('freezing', 24), ('kirkuk', 73), ('tuz', 4), ('khormato', 1), ('detonated', 74), ('samarra', 19), ('blew', 79), ('come', 195), ('pilgrims', 49), ('gather', 20), ('tight', 35), ('karbala', 25), ('arbaeen', 1), ('walked', 28), ('mourning', 24), ('imam', 17), ('centuries', 52), ('grandson', 6), ('prophet', 58), ('mohammad', 80), ('revered', 8), ('rain', 68), ('sandstorms', 2), ('battered', 14), ('hardest', 23), ('storms', 23), ('brought', 121), ('wind', 20), ('factory', 39), ('collapse', 57), ('alexandria', 6), ('collapses', 3), ('blamed', 177), ('follow', 57), ('rules', 64), ('relatively', 39), ('frequent', 46), ('reopened', 17), ('ports', 24), ('visibility', 4), ('accidents', 40), ('denver', 5), ('colorado', 11), ('limited', 86), ('shut', 81), ('travel', 161), ('snowstorms', 3), ('plains', 6), ('formal', 63), ('truce', 67), ('ending', 96), ('mountainous', 37), ('common', 97), ('highways', 13), ('postal', 10), ('delivery', 62), ('open', 181), ('runways', 2), ('delays', 22), ('airlines', 47), ('backlog', 1), ('travelers', 21), ('rushing', 7), ('sympathy', 4), ('solidarity', 31), ('poland', 103), ('krzysztof', 2), ('skubiszewski', 5), ('p', 28), ('j', 15), ('crowley', 5), ('paved', 6), ('eventual', 14), ('membership', 91), ('statesman', 3), ('visionary', 2), ('age', 73), ('83', 16), ('interrupted', 12), ('facto', 15), ('occupied', 71), ('communism', 8), ('1989', 60), ('1993', 46), ('geographic', 8), ('headquarters', 81), ('revealed', 25), ('extraordinary', 12), ('archaeological', 4), ('graveyards', 1), ('niger', 113), ('insight', 5), ('sahara', 21), ('green', 36), ('lush', 1), ('sisco', 17), ('ugandan', 63), ('joseph', 37), ('kony', 11), ('lieutenant', 43), ('chris', 15), ('magezi', 1), ('clashed', 49), ('commanded', 8), ('southwest', 42), ('juba', 8), ('fighters', 195), ('heading', 44), ('congo', 144), ('witnesses', 206), ('gunman', 24), ('pursue', 36), ('lord', 18), ('resistance', 66), ('warrants', 14), ('lra', 13), ('notorious', 20), ('brutality', 7), ('uganda', 62), ('uprising', 54), ('winning', 54), ('82', 16), ('activist', 33), ('triumfalnaya', 1), ('square', 63), ('right', 158), ('shouted', 8), ('slogans', 36), ('vladimir', 90), ('putin', 146), ('reversed', 11), ('dissident', 17), ('lyudmila', 3), ('alexeyeva', 1), ('recipients', 5), ('sakharov', 1), ('founding', 20), ('helsinki', 5), ('oldest', 14), ('organizations', 88), ('kurram', 7), ('authority', 150), ('attacking', 36), ('targets', 96), ('dismayed', 5), ('exercising', 4), ('assemble', 4), ('peacefully', 20), ('speech', 121), ('universal', 15), ('recognize', 56), ('defend', 48), ('shells', 17), ('impose', 44), ('strict', 24), ('swat', 39), ('valley', 66), ('allahabad', 1), ('eject', 1), ('loyal', 34), ('maulana', 5), ('fazlullah', 6), ('checkpoints', 20), ('closer', 42), ('truck', 85), ('gains', 32), ('islamists', 21), ('recaptured', 6), ('strategic', 47), ('peak', 15), ('fm', 11), ('radio', 192), ('angry', 37), ('demonstrated', 31), ('effect', 77), ('connecting', 3), ('jalalabad', 10), ('demanded', 95), ('bring', 148), ('skyrocketing', 3), ('setting', 43), ('aside', 24), ('buy', 78), ('kazakhstan', 46), ('pakistan', 897), ('afghans', 46), ('reliant', 3), ('imports', 73), ('recently', 222), ('slowed', 27), ('exports', 195), ('concerns', 196), ('herat', 20), ('believe', 130), ('abducted', 115), ('nepalese', 26), ('worker', 70), ('disappeared', 46), ('traveling', 99), ('adraskan', 1), ('ghraib', 46), ('prisoner', 49), ('scandal', 57), ('guilty', 104), ('dereliction', 6), ('specialist', 7), ('megan', 1), ('ambuhl', 1), ('plea', 24), ('summary', 1), ('martial', 15), ('carrying', 198), ('tourist', 51), ('cosmonauts', 3), ('docked', 8), ('demoted', 3), ('pay', 123), ('woman', 188), ('maryland', 15), ('plead', 6), ('stemming', 14), ('prevent', 142), ('faced', 41), ('photographs', 37), ('taunting', 2), ('humiliating', 4), ('naked', 7), ('condemnation', 12), ('burundi', 30), ('liberation', 77), ('bujumbura', 2), ('fnl', 2), ('robbing', 4), ('intervened', 10), ('accord', 82), ('broken', 41), ('connection', 114), ('ali', 156), ('haidari', 5), ('soyuzcapsule', 1), ('acting', 44), ('tip', 15), ('mosul', 108), ('guardsmen', 9), ('destabilize', 17), ('interim', 149), ('iyad', 26), ('allawi', 47), ('remanded', 2), ('suspect', 147), ('transport', 52), ('ismael', 2), ('abdurahman', 1), ('withholding', 5), ('helped', 113), ('patrolling', 15), ('56', 23), ('bombers', 90), ('scheduled', 306), ('extradition', 36), ('hearing', 61), ('hamdi', 7), ('issac', 7), ('osman', 15), ('fyodor', 1), ('yurchikhin', 1), ('oleg', 2), ('kotov', 1), ('software', 18), ('billionaire', 13), ('charles', 37), ('simonyi', 3), ('citizen', 46), ('ethiopian', 97), ('descent', 16), ('warlord', 7), ('abdul', 68), ('rashid', 23), ('dostum', 9), ('narrowly', 17), ('praying', 7), ('eid', 27), ('adha', 7), ('festival', 42), ('purportedly', 8), ('mullah', 39), ('omar', 65), ('radical', 55), ('lay', 35), ('exchange', 156), ('agencies', 117), ('enter', 76), ('defiant', 3), ('watchdog', 15), ('resolutions', 9), ('likes', 1), ('ahmadinejad', 140), ('overwhelmingly', 27), ('insists', 46), ('peaceful', 139), ('accuse', 62), ('paid', 88), ('privilege', 2), ('spaceflight', 1), ('comments', 176), ('pointed', 14), ('disarmed', 9), ('arsenal', 11), ('confirmed', 328), ('cooperation', 187), ('forward', 59), ('enrich', 19), ('generate', 25), ('electricity', 97), ('ghazni', 31), ('interpreter', 17), ('nationalities', 19), ('german', 203), ('mazar', 5), ('i', 201), ('sharif', 24), ('tajikistan', 16), ('stuffed', 3), ('explosives', 153), ('staged', 49), ('gourmet', 2), ('dinner', 21), ('eaten', 6), ('anniversary', 109), ('yuri', 11), ('gagarin', 1), ('1961', 7), ('khost', 34), ('ahmad', 40), ('khan', 77), ('midday', 8), ('prayers', 41), ('worshippers', 20), ('blame', 46), ('bilateral', 81), ('deepen', 4), ('opportunities', 24), ('16th', 32), ('relationship', 37), ('differences', 55), ('critical', 76), ('soviet', 122), ('crew', 91), ('mikhail', 35), ('tyurin', 1), ('astronauts', 40), ('miguel', 9), ('lopez', 29), ('alegria', 1), ('sunita', 1), ('williams', 29), ('friendly', 26), ('dozen', 51), ('baquba', 33), ('photos', 33), ('wanted', 156), ('showing', 62), ('hair', 16), ('cropped', 1), ('beard', 1), ('bulgaria', 27), ('nikolai', 5), ('svinarov', 1), ('probably', 42), ('bulgarian', 14), ('agent', 35), ('investigations', 20), ('toughen', 3), ('illegals', 1), ('chance', 50), ('limit', 41), ('debate', 56), ('controversial', 109), ('regarding', 24), ('implementation', 19), ('map', 30), ('middle', 214), ('provisions', 20), ('guest', 13), ('deport', 9), ('illegal', 166), ('fewer', 19), ('passes', 12), ('reconciled', 1), ('passed', 144), ('emphasized', 9), ('felony', 5), ('illegally', 65), ('sparked', 87), ('working', 223), ('raise', 89), ('rebuild', 43), ('inter', 20), ('train', 92), ('teachers', 39), ('adopt', 15), ('curriculum', 3), ('idb', 2), ('school', 133), ('enrolled', 2), ('classes', 12), ('luis', 46), ('moreno', 8), ('arrived', 164), ('improved', 54), ('ability', 36), ('read', 26), ('mail', 22), ('hear', 22), ('phone', 48), ('formed', 70), ('determine', 61), ('best', 83), ('done', 47), ('promoting', 35), ('values', 22), ('propagandists', 1), ('depicting', 12), ('hateful', 1), ('acknowledged', 43), ('decisions', 25), ('hindered', 8), ('diplomacy', 21), ('emerge', 11), ('proving', 4), ('merit', 3), ('los', 54), ('angeles', 52), ('forged', 10), ('nation', 411), ('ally', 53), ('cites', 16), ('khartoum', 55), ('provided', 104), ('shared', 25), ('data', 56), ('though', 65), ('list', 115), ('sponsors', 6), ('flown', 23), ('removed', 55), ('achieving', 7), ('vision', 12), ('palestine', 14), ('side', 79), ('osama', 65), ('laden', 102), ('decline', 65), ('3', 358), ('stay', 76), ('slowdown', 25), ('economies', 42), ('particularly', 61), ('weathering', 1), ('integrated', 5), ('benefited', 8), ('price', 172), ('increases', 30), ('diversify', 18), ('ease', 49), ('ups', 1), ('downs', 3), ('affect', 34), ('commodity', 15), ('markets', 93), ('hong', 105), ('kong', 99), ('charter', 36), ('carrier', 21), ('cr', 1), ('airways', 9), ('purchased', 19), ('boeing', 34), ('worth', 75), ('bought', 27), ('737', 8), ('airliners', 13), ('787s', 1), ('expand', 59), ('boosts', 4), ('manufacturer', 10), ('flag', 24), ('cathay', 1), ('pacific', 86), ('aircraft', 175), ('crack', 20), ('institutions', 55), ('status', 70), ('protesting', 50), ('broke', 147), ('stations', 87), ('curfew', 20), ('largest', 296), ('refineries', 28), ('pipeline', 73), ('worsen', 6), ('exploded', 173), ('sudanese', 148), ('readying', 3), ('rape', 39), ('murder', 101), ('darfur', 331), ('troubled', 68), ('justice', 193), ('mohamed', 56), ('yassin', 6), ('reuters', 77), ('agents', 55), ('abuses', 54), ('vowed', 73), ('crimes', 247), ('humanity', 44), ('punish', 22), ('consider', 83), ('proposal', 129), ('mustapha', 1), ('refuse', 8), ('measure', 121), ('manuscript', 3), ('composer', 2), ('ludwig', 1), ('van', 45), ('beethoven', 3), ('gross', 11), ('fuge', 1), ('sold', 59), ('auction', 17), ('sotheby', 3), ('auctioneers', 1), ('anonymous', 5), ('buyer', 5), ('library', 3), ('palmer', 3), ('theological', 1), ('philadelphia', 6), ('page', 18), ('document', 72), ('performed', 12), ('1826', 1), ('piano', 4), ('duet', 3), ('version', 21), ('quartet', 7), ('flat', 10), ('written', 32), ('brown', 46), ('ink', 2), ('annotations', 1), ('pencil', 2), ('crayon', 1), ('rediscovery', 1), ('complete', 63), ('reassessment', 1), ('music', 67), ('continued', 174), ('deaf', 1), ('wrote', 28), ('prior', 34), ('1827', 1), ('basque', 20), ('eta', 34), ('foiled', 13), ('plot', 73), ('resumes', 6), ('attackers', 73), ('1920', 3), ('brigades', 36), ('planned', 199), ('plague', 4), ('session', 86), ('testify', 9), ('torture', 80), ('140', 30), ('dujail', 19), ('1982', 26), ('john', 224), ('howard', 30), ('restricted', 18), ('classified', 21), ('material', 58), ('forcing', 51), ('repeatedly', 86), ('vital', 23), ('granting', 20), ('initially', 38), ('resisted', 10), ('reluctance', 3), ('share', 136), ('gara', 2), ('june', 254), ('restrictions', 70), ('deliver', 37), ('commitments', 16), ('cartoon', 14), ('paper', 45), ('prosecutor', 85), ('saeed', 3), ('mortazavi', 1), ('cartoonist', 4), ('mana', 1), ('neyestani', 1), ('editors', 4), ('poked', 1), ('fun', 5), ('ethnic', 197), ('azeris', 4), ('tabriz', 1), ('azerbaijan', 31), ('portrayed', 2), ('azeri', 6), ('cockroach', 1), ('rioting', 18), ('tear', 19), ('disperse', 21), ('language', 42), ('turkish', 227), ('target', 84), ('la', 18), ('peineta', 1), ('stadium', 30), ('centerpiece', 2), ('host', 76), ('2012', 30), ('jakarta', 51), ('comprehensive', 29), ('sustainable', 10), ('solution', 31), ('1976', 20), ('covers', 9), ('participation', 34), ('arrangements', 9), ('gained', 47), ('momentum', 8), ('devastated', 57), ('attacker', 35), ('protecting', 31), ('tensions', 115), ('yet', 146), ('turkmen', 7), ('competing', 21), ('rich', 79), ('maintains', 17), ('foothold', 1), ('jose', 76), ('rodriguez', 44), ('zapatero', 21), ('offered', 107), ('renounces', 6), ('yugoslavia', 25), ('brief', 44), ('croatian', 38), ('sanader', 3), ('attended', 55), ('postponed', 59), ('carla', 6), ('del', 27), ('ponte', 10), ('zagreb', 9), ('ante', 7), ('gotovina', 17), ('fully', 64), ('ministers', 197), ('warn', 26), ('compliance', 12), ('hague', 75), ('presence', 91), ('maintain', 64), ('boundary', 7), ('mission', 250), ('peacekeepers', 121), ('1960s', 17), ('1978', 12), ('confirm', 45), ('invaded', 14), ('search', 92), ('corp', 21), ('shareholders', 13), ('acquisition', 15), ('royal', 45), ('trustco', 1), ('ltd', 3), ('toronto', 5), ('212', 3), ('mi', 4), ('llion', 1), ('thrift', 4), ('holding', 148), ('expects', 56), ('obtain', 16), ('regulatory', 12), ('transaction', 8), ('kingdom', 65), ('historically', 8), ('played', 64), ('literature', 9), ('science', 29), ('zenith', 2), ('19th', 45), ('empire', 45), ('stretched', 5), ('surface', 35), ('20th', 35), ('uk', 61), ('seriously', 53), ('depleted', 2), ('wars', 56), ('irish', 29), ('dismantling', 13), ('rebuilding', 31), ('modern', 27), ('commonwealth', 21), ('pursues', 3), ('approach', 27), ('active', 45), ('scottish', 11), ('wales', 8), ('ireland', 30), ('1999', 80), ('cancelled', 5), ('tampa', 1), ('roger', 14), ('daltrey', 2), ('ill', 29), ('latter', 11), ('wrangling', 3), ('devolution', 1), ('explored', 7), ('initial', 59), ('colonizing', 1), ('costa', 41), ('rica', 31), ('proved', 13), ('unsuccessful', 13), ('combination', 13), ('mosquito', 8), ('infested', 3), ('swamps', 1), ('brutal', 21), ('heat', 20), ('natives', 3), ('pirate', 9), ('raids', 95), ('1563', 2), ('settlement', 90), ('cartago', 1), ('cooler', 3), ('fertile', 4), ('highlands', 4), ('remained', 55), ('1821', 6), ('jointly', 13), ('disintegrated', 1), ('1838', 3), ('proclaimed', 12), ('periods', 7), ('marred', 23), ('1949', 22), ('dissolved', 23), ('expanded', 36), ('technology', 110), ('industries', 45), ('standard', 28), ('63', 21), ('offstage', 1), ('song', 22), ('guitarist', 6), ('pete', 3), ('townshend', 2), ('crowd', 68), ('suffering', 64), ('bronchitis', 1), ('barely', 2), ('land', 181), ('ownership', 18), ('fishing', 88), ('trawling', 1), ('occur', 9), ('refuge', 24), ('mining', 67), ('alumina', 4), ('gold', 80), ('accounting', 14), ('85', 37), ('vulnerable', 28), ('mineral', 13), ('volatility', 2), ('ronald', 14), ('venetiaan', 1), ('inherited', 2), ('inflation', 75), ('fiscal', 69), ('deficit', 82), ('quickly', 66), ('implemented', 22), ('austerity', 9), ('raised', 95), ('taxes', 58), ('attempted', 41), ('tamed', 1), ('owing', 2), ('sizeable', 6), ('suriname', 10), ('projects', 94), ('bauxite', 6), ('netherlands', 77), ('belgium', 33), ('waned', 1), ('earned', 28), ('picked', 29), ('boosting', 33), ('budget', 114), ('devalued', 3), ('currency', 70), ('reduce', 97), ('cheered', 7), ('rescheduled', 8), ('prospects', 27), ('medium', 14), ('depend', 18), ('introduction', 6), ('structural', 18), ('liberalize', 5), ('carib', 3), ('indians', 14), ('inhabited', 11), ('grenada', 8), ('columbus', 16), ('1498', 1), ('uncolonized', 1), ('settled', 35), ('estates', 4), ('imported', 38), ('slaves', 17), ('1762', 1), ('vigorously', 3), ('production', 223), ('cacao', 1), ('surpassed', 6), ('crop', 19), ('nutmeg', 2), ('1967', 27), ('autonomy', 65), ('attained', 11), ('1974', 21), ('smallest', 7), ('hemisphere', 21), ('marxist', 16), ('1983', 15), ('ringleaders', 1), ('cuban', 152), ('reinstituted', 1), ('touring', 7), ('endless', 1), ('wire', 8), ('album', 20), ('ivan', 11), ('causing', 63), ('philosopher', 2), ('shore', 19), ('shipwreck', 1), ('drowned', 15), ('inveighed', 1), ('injustice', 4), ('providence', 2), ('sake', 7), ('perchance', 1), ('sailing', 11), ('persons', 16), ('perish', 2), ('indulging', 1), ('reflections', 2), ('whole', 23), ('ants', 6), ('nest', 4), ('standing', 41), ('climbed', 26), ('stung', 6), ('trampled', 4), ('foot', 28), ('mercury', 5), ('presented', 54), ('striking', 10), ('wand', 1), ('indeed', 6), ('yourself', 5), ('judge', 184), ('dealings', 5), ('hast', 1), ('thyself', 1), ('manner', 11), ('apartment', 32), ('tulkarem', 15), ('rami', 1), ('tayyah', 1), ('recruitment', 9), ('establishment', 20), ('shootings', 20), ('ahmed', 76), ('qureia', 24), ('hampering', 13), ('restart', 15), ('yasser', 21), ('arafat', 45), ('aggression', 15), ('sends', 11), ('message', 92), ('want', 168), ('things', 23), ('quiet', 18), ('band', 27), ('performs', 3), ('criticism', 108), ('joschka', 5), ('fischer', 8), ('churches', 27), ('simultaneous', 13), ('armenian', 12), ('chaldean', 2), ('planting', 15), ('casualties', 134), ('minority', 72), ('church', 110), ('extreme', 16), ('caution', 7), ('root', 21), ('concern', 152), ('result', 86), ('committed', 75), ('combating', 5), ('strike', 221), ('worsened', 9), ('alike', 6), ('committing', 21), ('serious', 108), ('uruzgan', 37), ('kandahar', 155), ('talking', 13), ('paintings', 4), ('contemporary', 5), ('art', 29), ('names', 33), ('pablo', 2), ('picasso', 1), ("o'keeffe", 1), ('vincent', 8), ('gogh', 7), ('often', 112), ('mind', 13), ('we', 63), ('body', 155), ('artwork', 4), ('artists', 10), ('primimoda', 1), ('gallery', 3), ('ndimyake', 1), ('mwakalyelye', 1), ('tells', 24), ('biggest', 69), ('maker', 24), ('chips', 2), ('investing', 9), ('intel', 13), ('chairman', 113), ('bangalore', 7), ('employs', 16), ('generally', 32), ('respected', 9), ('going', 61), ('stimulate', 7), ('innovation', 6), ('oriented', 14), ('overseas', 38), ('operating', 73), ('invested', 12), ('700', 57), ('barrett', 3), ('grow', 38), ('davis', 20), ('cup', 120), ('tournament', 85), ('hardcourts', 1), ('carson', 1), ('tie', 12), ('start', 124), ('title', 36), ('squad', 25), ('clay', 4), ('seville', 2), ('crown', 13), ('previous', 109), ('match', 104), ('winner', 73), ('romania', 27), ('criticizes', 8), ('ceasefire', 64), ('policemen', 137), ('sopore', 1), ('hurled', 14), ('indiscriminately', 6), ('mansoorain', 2), ('portion', 26), ('merger', 32), ('technologies', 10), ('crash', 93), ('moon', 56), ('smart', 7), ('lunar', 14), ('542', 1), ('utc', 11), ('volcanic', 8), ('plain', 4), ('excellence', 2), ('orbited', 1), ('manager', 15), ('gerhard', 27), ('schwehm', 1), ('justify', 9), ('excessive', 14), ('jammu', 13), ('solar', 21), ('electric', 19), ('propulsion', 2), ('interplanetary', 1), ('missions', 27), ('miniaturized', 1), ('instruments', 13), ('examine', 16), ('craft', 5), ('orbiting', 15), ('somalia', 270), ('orphanage', 3), ('mogadishu', 96), ('abdikadir', 1), ('yusuf', 16), ('kariye', 2), ('unidentified', 73), ('assailants', 30), ('lafoole', 1), ('endangering', 3), ('rely', 17), ('warned', 232), ('catastrophe', 13), ('describes', 17), ('recovering', 18), ('iranians', 24), ('receive', 65), ('senator', 124), ('joe', 15), ('lieberman', 14), ('intends', 21), ('introduced', 35), ('senators', 31), ('mccain', 48), ('lindsey', 2), ('graham', 5), ('authorize', 10), ('funds', 95), ('tools', 5), ('evade', 1), ('censorship', 10), ('online', 17), ('persian', 30), ('programming', 11), ('cover', 36), ('funding', 69), ('sponsored', 40), ('farda', 6), ('boost', 100), ('broadcaster', 14), ('wave', 56), ('satellite', 47), ('capacity', 54), ('cambodian', 18), ('clearing', 27), ('mines', 39), ('khmer', 10), ('rouge', 13), ('howes', 2), ('bristol', 1), ('england', 56), ('huon', 1), ('huot', 2), ('removing', 15), ('angor', 1), ('wat', 2), ('temple', 14), ('showed', 111), ('executed', 36), ('52', 39), ('puth', 1), ('lim', 1), ('notes', 25), ('minorities', 16), ('personal', 40), ('freedoms', 17), ('dedicated', 18), ('jews', 42), ('nazis', 9), ('ii', 113), ('dignitaries', 11), ('survivors', 72), ('nazi', 23), ('murdered', 19), ('berlin', 31), ('speaker', 55), ('wolfgang', 6), ('thierse', 1), ('memory', 8), ('holocaust', 26), ('alive', 33), ('hectare', 3), ('architect', 7), ('peter', 48), ('eisenman', 2), ('consists', 16), ('711', 1), ('unadorned', 1), ('slabs', 1), ('underground', 39), ('evokes', 2), ('rigid', 3), ('discipline', 11), ('arguments', 9), ('size', 35), ('design', 12), ('honor', 38), ('jewish', 138), ('norwegian', 35), ('interference', 19), ('210', 3), ('tomas', 6), ('archer', 2), ('continuously', 6), ('repeated', 55), ('similarly', 1), ('situations', 13), ('bangladesh', 72), ('extrajudicial', 2), ('politically', 35), ('motivated', 31), ('r', 5), ('c', 45), ('provides', 47), ('internally', 6), ('departure', 14), ('suspicious', 22), ('resist', 7), ('pressure', 145), ('peacekeeping', 124), ('volatile', 35), ('receiving', 54), ('jacques', 39), ('bernard', 18), ('farmhouse', 2), ('ransacked', 5), ('supported', 45), ('manipulating', 8), ('claiming', 32), ('outright', 10), ('internationally', 28), ('brokered', 26), ('divide', 14), ('blank', 6), ('proportionately', 2), ('boat', 64), ('37', 39), ('guerrillas', 45), ('encounters', 2), ('prevents', 5), ('mideast', 14), ('male', 23), ('swim', 6), ('ashore', 16), ('capsized', 14), ('surma', 1), ('river', 107), ('northeastern', 82), ('colliding', 3), ('sunamganj', 1), ('240', 8), ('northeast', 51), ('dhaka', 14), ('rivers', 21), ('lax', 2), ('regulations', 38), ('cayman', 10), ('tropical', 71), ('depression', 17), ('shape', 10), ('strengthen', 70), ('wilma', 19), ('21st', 10), ('tying', 3), ('1933', 3), ('daybreak', 2), ('centered', 36), ('325', 6), ('sustained', 41), ('55', 49), ('itv', 2), ('abide', 7), ('recognizing', 9), ('denouncing', 11), ('likely', 141), ('caymans', 1), ('jamaica', 15), ('forecasts', 5), ('reeling', 2), ('mine', 100), ('sailors', 14), ('batticaloa', 9), ('grenade', 48), ('parking', 8), ('compound', 64), ('damaging', 24), ('monitoring', 42), ('cease', 98), ('unarmed', 12), ('scandinavian', 3), ('reprimanded', 6), ('69', 16), ('concluded', 30), ('454', 5), ('esad', 1), ('bajramovic', 1), ('croat', 15), ('kevljani', 2), ('prijedor', 4), ('inmates', 39), ('omarska', 1), ('keraterm', 1), ('recovered', 55), ('himalayan', 26), ('220', 14), ('winter', 67), ('sneak', 3), ('joining', 30), ('manouchehr', 18), ('mottaki', 24), ('idea', 27), ('ambitions', 32), ('revolutionary', 65), ('yahya', 5), ('rahim', 10), ('safavi', 2), ('cbs', 13), ('dealing', 36), ('intervention', 17), ('option', 24), ('greetings', 10), ('celebrate', 36), ('occasion', 13), ('thanks', 18), ('blessings', 4), ('remember', 14), ('abraham', 4), ('loving', 5), ('god', 20), ('considered', 85), ('wrested', 1), ('legislature', 33), ('sacrifice', 13), ('commemorates', 6), ('willingness', 15), ('obedience', 1), ('according', 69), ('scripture', 1), ('allah', 2), ('exchanging', 6), ('gifts', 11), ('engaging', 10), ('worship', 5), ('hopeful', 23), ('talents', 1), ('generosity', 1), ('compassion', 7), ('cyprus', 46), ('treaty', 99), ('dimitris', 2), ('christofias', 2), ('mediterranean', 23), ('ratify', 14), ('49', 23), ('nicosia', 4), ('favor', 57), ('streamlining', 3), ('bureaucracy', 6), ('enact', 7), ('advocates', 21), ('unclear', 71), ('salvaged', 1), ('defeat', 60), ('designate', 14), ('lands', 20), ('ravaged', 19), ('concentrate', 5), ('creating', 34), ('withstand', 6), ('harsh', 28), ('commissioner', 43), ('antonio', 24), ('guterres', 5), ('remark', 15), ('zone', 75), ('reviewing', 14), ('materialize', 3), ('cold', 39), ('hunger', 44), ('muzaffarabad', 13), ('repay', 12), ('generously', 1), ('hosting', 13), ('toured', 10), ('miillion', 1), ('exhibition', 17), ('imperial', 11), ('museum', 40), ('marking', 48), ('centenary', 2), ('writer', 11), ('ian', 9), ('fleming', 3), ('secret', 113), ('bond', 8), ('burge', 2), ('exhibit', 6), ('titled', 5), ('eyes', 11), ('fallujah', 51), ('contains', 14), ('linked', 164), ('sattler', 2), ('mandelson', 6), ('textile', 30), ('shipments', 22), ('hardship', 3), ('embedded', 3), ('searching', 49), ('property', 31), ('ammunition', 28), ('letters', 20), ('mural', 1), ('pledging', 14), ('wired', 2), ('assault', 57), ('jerry', 6), ("o'hara", 2), ('deh', 1), ('rawood', 1), ('light', 66), ('gun', 37), ('convoy', 145), ('upsurge', 21), ('followed', 102), ('beijing', 319), ('revising', 1), ('limiting', 2), ('rescued', 41), ('engineer', 16), ('chad', 108), ('pilots', 10), ('gunpoint', 6), ('captive', 7), ('160', 24), ('1984', 27), ('sikh', 7), ('sikhs', 6), ('incite', 5), ('indira', 3), ('gandhi', 12), ('compensation', 30), ('politician', 40), ('quotas', 22), ('filled', 21), ('items', 33), ('clothing', 23), ('customs', 48), ('expel', 5), ('popular', 118), ('marwan', 7), ('barghouti', 12), ('withdraws', 5), ('race', 77), ('challenger', 19), ('faruq', 1), ('qaddumi', 1), ('goes', 28), ('45', 58), ('serving', 49), ('filed', 84), ('papers', 14), ('9th', 7), ('powers', 80), ('revive', 25), ('negotiate', 39), ('brushing', 2), ('olmert', 85), ('statements', 41), ('walid', 7), ('moualem', 1), ('suppliers', 10), ('bashar', 34), ('assad', 45), ('impartial', 4), ('arbiter', 3), ('mediate', 18), ('consequences', 21), ('allowing', 72), ('goods', 96), ('retailers', 13), ('doubts', 13), ('play', 86), ('lacks', 18), ('entire', 53), ('golan', 9), ('heights', 11), ('unit', 94), ('coordinated', 34), ('azamiyah', 2), ('amiriyah', 2), ('agitation', 3), ('delaying', 19), ('higher', 118), ('shortages', 44), ('communications', 43), ('jamming', 3), ('broadcasts', 24), ('telecommunication', 4), ('interfering', 17), ('signals', 7), ('blaming', 21), ('indicated', 39), ('source', 76), ('soil', 49), ('itu', 1), ('provider', 4), ('eutelsat', 2), ('disputed', 94), ('means', 56), ('disruptions', 6), ('geneva', 45), ('polio', 36), ('nigeria', 183), ('burkina', 16), ('faso', 16), ('ivory', 81), ('producers', 20), ('harming', 8), ('cases', 231), ('170', 21), ('eradicate', 5), ('hampered', 20), ('vaccine', 38), ('contaminated', 16), ('infertility', 2), ('nervous', 11), ('paralysis', 7), ('add', 15), ('portable', 3), ('devices', 31), ('touch', 13), ('screen', 14), ('macrumors', 1), ('com', 8), ('website', 44), ('watches', 14), ('developments', 50), ('apple', 7), ('patent', 2), ('phones', 16), ('computers', 24), ('beneath', 20), ('display', 17), ('screens', 5), ('saving', 12), ('competitor', 2), ('windows', 18), ('keyboard', 1), ('mouse', 8), ('counterparts', 22), ('regained', 12), ('diwaniyah', 4), ('militiamen', 23), ('pair', 14), ('liberian', 22), ('ellen', 15), ('johnson', 29), ('sirleaf', 20), ('congolese', 47), ('kabila', 20), ('mrs', 27), ('reconstruction', 77), ('debt', 151), ('supportive', 3), ('emerging', 25), ('ruined', 4), ('diyala', 21), ('mohammed', 115), ('ramadan', 37), ('patriotic', 5), ('kurdistan', 40), ('nouri', 43), ('maliki', 65), ('23', 97), ('shaab', 2), ('michel', 10), ('barnier', 5), ('implement', 37), ('discussing', 35), ('stabilizing', 9), ('resigned', 84), ('confidence', 80), ('motion', 28), ('troop', 34), ('withdrawals', 9), ('withdrawn', 32), ('batch', 10), ('parallel', 5), ('handing', 13), ('allowed', 138), ('completes', 3), ('faction', 44), ('ramallah', 35), ('overdue', 2), ('salaries', 15), ('wing', 57), ('televised', 36), ('ended', 192), ('moqtada', 19), ('sadr', 42), ('interior', 149), ('responded', 53), ('ordering', 16), ('redeploy', 2), ('confrontation', 17), ('askar', 14), ('akayev', 18), ('kyrgyzstan', 47), ('accept', 70), ('resignation', 81), ('technically', 5), ('deposed', 31), ('letter', 92), ('75', 47), ('deputies', 10), ('videotaped', 8), ('traveled', 52), ('unstable', 5), ('osh', 5), ('boucher', 9), ('islamabad', 113), ('meetings', 83), ('khurshid', 2), ('kasuri', 12), ('pervez', 82), ('musharraf', 170), ('hardliners', 2), ('adopted', 56), ('brussels', 43), ('embargo', 43), ('import', 24), ('gems', 6), ('metals', 7), ('relatives', 51), ('subject', 32), ('assets', 59), ('freeze', 32), ('meaningful', 3), ('sierra', 18), ('leone', 18), ('unable', 32), ('exact', 16), ('schoolchildren', 3), ('freetown', 1), ('syphon', 1), ('maritime', 24), ('uncommon', 3), ('boats', 33), ('overloaded', 6), ('standards', 51), ('frequently', 40), ('lacking', 8), ('ignored', 20), ('treating', 19), ('viktor', 93), ('yushchenko', 135), ('poisoned', 16), ('tcdd', 2), ('harmful', 14), ('dioxin', 4), ('contaminant', 1), ('orange', 13), ('substance', 10), ('normal', 35), ('yanukovych', 57), ('midst', 11), ('heated', 7), ('interviewed', 11), ('flawed', 26), ('supreme', 163), ('overturned', 15), ('dumped', 20), ('tradition', 27), ('vatican', 87), ('stamp', 18), ('pope', 210), ('vacant', 7), ('see', 100), ('image', 28), ('crossed', 46), ('keys', 14), ('traditional', 50), ('stamps', 11), ('papal', 8), ('symbols', 5), ('valid', 8), ('interregnum', 1), ('conclave', 1), ('cardinals', 5), ('capable', 29), ('detonating', 8), ('radioactive', 5), ('dirty', 11), ('annual', 139), ('proliferation', 43), ('cia', 101), ('stated', 6), ('desire', 24), ('using', 148), ('chemical', 79), ('biological', 7), ('improvised', 11), ('obtainable', 1), ('toxins', 1), ('radiological', 1), ('substances', 7), ('convinced', 9), ('pursuing', 28), ('clandestine', 9), ('factions', 63), ('hebron', 13), ('smaller', 46), ('ninth', 21), ('settle', 16), ('larger', 57), ('divided', 75), ('drove', 54), ('268', 2), ('infection', 28), ('dr', 32), ('lee', 30), ('consultant', 3), ('partly', 14), ('facility', 122), ('paktika', 21), ('sexual', 32), ('needles', 1), ('twelve', 8), ('demining', 1), ('paktia', 7), ('deminers', 1), ('navy', 71), ('iranativu', 1), ('regrouped', 2), ('mount', 26), ('skater', 1), ('michelle', 10), ('kwan', 6), ('pulled', 49), ('dashing', 1), ('superstar', 3), ('eluded', 2), ('prestigious', 7), ('career', 41), ('medal', 22), ('withdrew', 56), ('hip', 7), ('muscle', 7), ('injury', 43), ('recurring', 3), ('groin', 4), ('strain', 203), ('herself', 19), ('ever', 73), ('native', 39), ('bye', 4), ('onto', 21), ('titles', 9), ('silver', 15), ('bronze', 13), ('medals', 15), ('awarded', 25), ('events', 65), ('anticipated', 8), ('alpine', 5), ('skiing', 5), ('downhill', 15), ('whistle', 1), ('blower', 1), ('mordechai', 3), ('vanunu', 7), ('giving', 83), ('unauthorized', 8), ('divulging', 2), ('barred', 36), ('contacts', 18), ('resort', 68), ('hotel', 62), ('ghazala', 1), ('gardens', 6), ('sharm', 16), ('el', 89), ('sheik', 7), ('targeted', 110), ('bazaar', 2), ('naama', 1), ('outdoor', 10), ('cafe', 9), ('hotels', 27), ('packed', 35), ('tourists', 61), ('busy', 32), ('summer', 51), ('vacation', 12), ('34', 54), ('exaggerate', 1), ('mastermind', 14), ('surfaced', 13), ('audiotape', 8), ('internet', 111), ('minute', 45), ('aim', 22), ('ascendant', 1), ('seats', 149), ('tape', 47), ('ayatollah', 41), ('sistani', 9), ('approving', 11), ('crush', 16), ('stronghold', 60), ('innocents', 2), ('fought', 64), ('authenticity', 12), ('completely', 30), ('little', 96), ('ceremonies', 34), ('marked', 50), ('moments', 7), ('silence', 22), ('reading', 16), ('bells', 7), ('tolled', 3), ('bagpipes', 2), ('moment', 27), ('hijacked', 36), ('raged', 8), ('colombo', 26), ('lawn', 2), ('vice', 187), ('biden', 18), ('qaeda', 6), ('crashed', 60), ('wreathlaying', 1), ('shanksville', 2), ('pennsylvania', 10), ('93', 15), ('hijackers', 9), ('presumably', 1), ('plane', 111), ('hitting', 26), ('intended', 59), ('rally', 109), ('king', 188), ('gyanendra', 35), ('pokhara', 2), ('coincided', 7), ('parties', 184), ('deserted', 6), ('businesses', 75), ('accompanied', 18), ('tamils', 5), ('registering', 5), ('nominations', 5), ('legitimize', 2), ('absolute', 21), ('sell', 53), ('submarines', 5), ('kommersant', 4), ('starts', 21), ('contract', 59), ('older', 13), ('636', 1), ('677', 1), ('amur', 6), ('supplied', 15), ('prompted', 53), ('expressions', 3), ('deals', 53), ('kalashnikov', 3), ('rifles', 17), ('weaponry', 9), ('critic', 24), ('complain', 11), ('discrimination', 24), ('sinhalese', 4), ('dick', 36), ('cheney', 70), ('stabilize', 23), ('abdullah', 83), ('strategy', 55), ('brazil', 148), ('shoot', 17), ('smuggling', 56), ('drugs', 64), ('traffickers', 24), ('colombia', 223), ('bogota', 17), ('reaffirming', 1), ('expansion', 46), ('brazilian', 54), ('steps', 60), ('error', 13), ('peru', 70), ('missionary', 4), ('dismantled', 11), ('inside', 95), ('commanders', 41), ('hitch', 3), ('paperwork', 3), ('transfer', 44), ('controls', 48), ('relaxed', 4), ('procedures', 19), ('qalqiliya', 4), ('bethlehem', 17), ('extremists', 61), ('sabotage', 18), ('halt', 68), ('designates', 1), ('albania', 15), ('macedonia', 34), ('eligible', 31), ('kunduz', 6), ('rural', 34), ('nimroz', 4), ('defectors', 12), ('repatriated', 9), ('informants', 5), ('executions', 11), ('example', 15), ('thinking', 6), ('discourage', 7), ('specifies', 2), ('returns', 27), ('koreans', 32), ('precision', 2), ('airstrike', 34), ('ripped', 37), ('elder', 13), ('naqib', 4), ('specify', 15), ('europeanunion', 1), ('agenda', 42), ('manuel', 29), ('barroso', 14), ('bloc', 62), ('satisfied', 9), ('rejecting', 29), ('agreements', 73), ('estonia', 16), ('latvia', 33), ('urge', 23), ('seek', 94), ('chechnya', 31), ('mortars', 27), ('settlements', 41), ('abdel', 28), ('razek', 1), ('majaidie', 1), ('shelling', 14), ('response', 145), ('gunfire', 38), ('jail', 66), ('collaborators', 2), ('masked', 15), ('jailhouse', 2), ('ayman', 28), ('zawahiri', 35), ('lithuania', 23), ('congratulate', 5), ('chancellor', 69), ('schroeder', 50), ('optimism', 8), ('lithuanian', 6), ('adamkus', 4), ('congratulated', 15), ('conversation', 13), ('partners', 32), ('modernization', 8), ('questioned', 63), ('objectivity', 3), ('characterized', 16), ('vastly', 4), ('opponent', 21), ('forecasters', 61), ('ernesto', 9), ('thinks', 15), ('urging', 91), ('posed', 18), ('debby', 2), ('weakened', 21), ('miami', 26), ('cape', 23), ('verde', 8), ('addis', 16), ('ababa', 15), ('explain', 16), ('unity', 63), ('meles', 9), ('zenawi', 10), ('conspiring', 7), ('overthrow', 42), ('suggested', 44), ('129', 5), ('journalists', 159), ('treason', 17), ('commit', 16), ('genocide', 89), ('disciplinary', 8), ('extremely', 30), ('troubling', 3), ('shielded', 1), ('prosecution', 36), ('undermined', 7), ('proceedings', 33), ('stephanides', 2), ('cypriot', 31), ('nationals', 60), ('siphoned', 2), ('away', 133), ('devised', 2), ('impoverished', 29), ('complying', 4), ('pullback', 4), ('millimeters', 3), ('tank', 25), ('stealing', 14), ('happy', 18), ('disarmament', 28), ('huge', 79), ('swaths', 4), ('firmly', 4), ('uzbekistan', 39), ('kyrgyz', 19), ('andijan', 11), ('villages', 70), ('teshiktosh', 1), ('karasu', 1), ('uzbek', 33), ('karimov', 13), ('radicals', 7), ('restraint', 11), ('roman', 54), ('catholic', 73), ('chapel', 1), ('pontiff', 51), ('religions', 5), ('promotion', 15), ('forgiveness', 5), ('catholics', 17), ('evil', 11), ('armies', 6), ('love', 17), ('turkey', 359), ('settling', 2), ('objections', 19), ('luxembourg', 23), ('retain', 16), ('agree', 74), ('negotiating', 19), ('framework', 15), ('officially', 64), ('midnight', 19), ('indirect', 13), ('associate', 15), ('hosni', 42), ('mubarak', 80), ('supporters', 194), ('embarks', 1), ('oath', 15), ('libyan', 29), ('moammar', 11), ('gadhafi', 21), ('nationally', 9), ('77', 21), ('nascent', 2), ('legislative', 57), ('criticisms', 4), ('rife', 3), ('irregularities', 28), ('challengers', 4), ('guarantees', 13), ('fans', 20), ('soccer', 31), ('football', 85), ('travels', 14), ('qualifying', 9), ('hiroyuki', 4), ('hosoda', 4), ('precondition', 3), ('safely', 11), ('spectators', 5), ('protected', 13), ('scenes', 5), ('mob', 11), ('game', 57), ('cameras', 8), ('throwing', 18), ('cans', 2), ('field', 63), ('path', 26), ('victorious', 6), ('rare', 37), ('occurrence', 4), ('flying', 50), ('drones', 13), ('looking', 78), ('programs', 96), ('detect', 11), ('weaknesses', 7), ('defenses', 11), ('editions', 3), ('launching', 40), ('restricting', 11), ('reactor', 41), ('grade', 31), ('dated', 10), ('circumstances', 18), ('samuel', 14), ('jang', 2), ('jae', 1), ('saddened', 4), ('passing', 46), ('condolence', 3), ('repressive', 10), ('regimes', 7), ('extent', 10), ('practice', 39), ('philippine', 46), ('exercises', 29), ('carmen', 1), ('mindanao', 5), ('chanted', 19), ('jolo', 7), ('sayyaf', 15), ('animal', 34), ('dolphins', 9), ('appalling', 2), ('solomon', 9), ('prohibits', 2), ('training', 110), ('wfp', 24), ('feed', 21), ('devastation', 13), ('hurricanes', 25), ('gustav', 10), ('ike', 4), ('rations', 3), ('beans', 12), ('vegetable', 3), ('canned', 2), ('fish', 43), ('supply', 95), ('storage', 13), ('warehouses', 2), ('liquid', 10), ('stoves', 1), ('cooking', 5), ('sweden', 62), ('nobel', 53), ('announcing', 10), ('winners', 13), ('oslo', 10), ('prizes', 3), ('medicine', 26), ('physics', 1), ('chemistry', 3), ('animals', 26), ('cages', 1), ('overcrowded', 12), ('shallow', 2), ('polluted', 6), ('pens', 2), ('gavutu', 1), ('economics', 7), ('instituted', 9), ('1968', 10), ('swedish', 36), ('philanthropist', 2), ('alfred', 3), ('check', 19), ('banquet', 3), ('1896', 2), ('chooses', 4), ('laureate', 20), ('literary', 3), ('choose', 29), ('1901', 2), ('barrage', 7), ('mortar', 42), ('rounds', 35), ('landed', 38), ('fortified', 11), ('houses', 57), ('offices', 88), ('embassies', 32), ('suffer', 22), ('scratches', 1), ('sunburn', 1), ('appear', 56), ('undernourished', 1), ('temporarily', 59), ('38', 46), ('postpone', 16), ('offshore', 45), ('fields', 32), ('outgoing', 33), ('luiz', 19), ('inacio', 20), ('lula', 20), ('da', 31), ('silva', 32), ('signature', 13), ('owned', 107), ('petrobras', 7), ('sole', 13), ('operator', 5), ('unexplored', 2), ('ventures', 8), ('potentially', 22), ('reserves', 55), ('southeastern', 97), ('meters', 69), ('below', 60), ('floor', 14), ('barrels', 81), ('provision', 12), ('opinion', 66), ('americans', 202), ('wrong', 29), ('rehabilitate', 2), ('wild', 39), ('abc', 29), ('indicates', 37), ('democrats', 107), ('point', 98), ('advantage', 27), ('republicans', 38), ('equipped', 9), ('handle', 8), ('hypothetical', 3), ('presumed', 5), ('51', 23), ('nears', 3), ('primaries', 5), ('hillary', 25), ('cite', 5), ('healthcare', 5), ('adults', 16), ('margin', 23), ('plus', 16), ('minus', 6), ('points', 81), ('castro', 135), ('welcomed', 42), ('ailing', 15), ('brother', 85), ('fidel', 60), ('tomb', 12), ('icon', 4), ('simon', 16), ('bolivar', 14), ('variety', 22), ('transported', 10), ('partner', 48), ('latin', 76), ('pact', 48), ('mixed', 28), ('reaction', 20), ('elbaradei', 33), ('praised', 72), ('undermine', 25), ('restricts', 3), ('conducting', 50), ('1998', 77), ('analyst', 7), ('garang', 30), ('federalism', 16), ('lecturer', 2), ('gai', 2), ('yoh', 3), ('unisa', 1), ('pretoria', 6), ('credits', 5), ('advocacy', 13), ('shutdown', 6), ('somali', 151), ('materialized', 3), ('spla', 6), ('democratize', 1), ('franklin', 4), ('delano', 1), ('roosevelt', 4), ('disability', 2), ('award', 55), ('integrate', 2), ('disabled', 6), ('polish', 64), ('lech', 12), ('kaczynski', 18), ('disabilities', 2), ('guaranteed', 13), ('convention', 19), ('legs', 3), ('paralyzed', 4), ('1945', 15), ('alarmed', 5), ('closure', 33), ('shabelle', 4), ('baidoa', 19), ('zemedkun', 1), ('tekle', 1), ('denial', 6), ('ogaden', 5), ('ethiopia', 115), ('wardheer', 1), ('purported', 22), ('hardware', 6), ('somalis', 19), ('bordering', 41), ('kuwaiti', 17), ('threatening', 64), ('demands', 106), ('rai', 2), ('previously', 112), ('certified', 7), ('confirming', 8), ('dominant', 20), ('128', 8), ('275', 16), ('briefly', 54), ('younis', 17), ('roof', 22), ('firing', 52), ('discontent', 6), ('cash', 60), ('strapped', 11), ('renounce', 20), ('certain', 58), ("n't", 66), ('produced', 46), ('quantities', 10), ('virgin', 8), ('possessions', 6), ('petition', 20), ('timex', 3), ('inc', 18), ('changes', 90), ('generalized', 1), ('preferences', 5), ('requested', 47), ('covered', 26), ('58', 26), ('tariff', 6), ('classifications', 1), ('decided', 89), ('categories', 1), ('potential', 78), ('producer', 63), ('seller', 4), ('priced', 10), ('battery', 10), ('operated', 36), ('thailand', 123), ('beneficiaries', 2), ('totaled', 5), ('1988', 29), ('representative', 57), ('malay', 5), ('10th', 18), ('mauritius', 7), ('portuguese', 13), ('dutch', 103), ('prince', 74), ('maurits', 1), ('nassau', 1), ('assumed', 34), ('1715', 1), ('overseeing', 16), ('establishing', 21), ('plantation', 6), ('cane', 3), ('1810', 1), ('napoleonic', 3), ('strategically', 3), ('playing', 40), ('submarine', 7), ('collection', 9), ('stable', 55), ('attracted', 26), ('considerable', 14), ('incomes', 10), ('amiin', 2), ('premises', 4), ('adawe', 1), ('saed', 1), ('apparel', 9), ('creole', 9), ('morocco', 60), ('benefits', 48), ('costs', 76), ('proximity', 8), ('manufacturing', 62), ('remittances', 31), ('exporter', 27), ('phosphate', 12), ('pursued', 11), ('vi', 3), ('performance', 34), ('steady', 19), ('industrial', 78), ('fta', 5), ('illiteracy', 2), ('rates', 94), ('alleviating', 1), ('underdevelopment', 1), ('expanding', 27), ('replacing', 11), ('urban', 22), ('slums', 7), ('subsidized', 6), ('housing', 83), ('deficits', 17), ('widened', 4), ('reducing', 39), ('adapting', 1), ('sluggish', 5), ('improving', 45), ('young', 104), ('moroccans', 9), ('disparity', 2), ('wealth', 36), ('confronting', 5), ('phosphates', 4), ('products', 87), ('centrally', 3), ('explanation', 11), ('averaged', 6), ('curb', 28), ('crime', 80), ('gray', 5), ('attracting', 17), ('catalyst', 4), ('dec', 4), ('albanians', 16), ('residing', 1), ('greece', 41), ('offset', 13), ('towering', 1), ('primarily', 33), ('farming', 24), ('prevalence', 5), ('inefficient', 6), ('plots', 15), ('reliance', 14), ('hydropower', 9), ('antiquated', 1), ('inadequate', 27), ('contribute', 28), ('environment', 58), ('fdi', 3), ('lowest', 24), ('embarked', 6), ('climate', 61), ('completion', 11), ('thermal', 3), ('vlore', 1), ('generation', 13), ('lines', 58), ('montenegro', 25), ('kosovo', 103), ('relieve', 5), ('rail', 14), ('barrier', 20), ('spared', 4), ('plagued', 28), ('deposits', 19), ('exploiting', 11), ('except', 20), ('location', 36), ('adequate', 18), ('connections', 4), ('hinder', 5), ('original', 25), ('compact', 13), ('1986', 18), ('amended', 9), ('federated', 7), ('micronesia', 3), ('fsm', 3), ('2023', 3), ('establishes', 5), ('contributions', 24), ('payouts', 3), ('perpetuity', 1), ('outlook', 7), ('appears', 58), ('fragile', 13), ('reduction', 39), ('discovery', 41), ('exploitation', 9), ('contributed', 36), ('fluctuating', 2), ('swings', 2), ('components', 15), ('dominate', 15), ('livelihood', 11), ('equatorial', 7), ('guinea', 31), ('cocoa', 24), ('neglect', 4), ('successive', 9), ('diminished', 4), ('intention', 25), ('reinvest', 1), ('mismanagement', 11), ('transparency', 17), ('candidacy', 17), ('extractive', 6), ('undeveloped', 2), ('zinc', 6), ('diamonds', 15), ('columbite', 1), ('tantalite', 1), ('peaked', 4), ('boss', 8), ('gone', 36), ('canada', 104), ('taunted', 2), ('montreal', 5), ('parting', 1), ('tears', 3), ('solely', 5), ('attractions', 3), ('pray', 16), ('forgive', 3), ('neck', 8), ('touching', 5), ('rite', 1), ('reservists', 3), ('abusing', 21), ('character', 11), ('george', 81), ('w', 19), ('please', 12), ('drive', 47), ('headlights', 2), ('kerry', 13), ('night', 125), ('topologist', 1), ('coffee', 20), ('doughnut', 1), ('lot', 16), ('unjust', 2), ('remind', 2), ('condemn', 8), ('profession', 5), ('apples', 2), ('very', 97), ('lonely', 2), ('imaginary', 3), ('courts', 37), ('graner', 2), ('sergeant', 14), ('javal', 1), ('sabrina', 2), ('harman', 1), ('hood', 6), ('instead', 76), ('orbits', 2), ('trips', 18), ('quote', 28), ('roskosmos', 1), ('ton', 17), ('capsule', 2), ('launch', 113), ('2018', 2), ('siberia', 9), ('module', 4), ('engine', 15), ('phased', 8), ('replacement', 14), ('soyuz', 4), ('scrutiny', 11), ('rough', 10), ('landings', 2), ('cancellation', 10), ('timidria', 1), ('ates', 1), ('mali', 38), ('intimidated', 7), ('slavery', 11), ('bondage', 1), ('enslaved', 2), ('saharan', 16), ('routes', 21), ('reason', 53), ('venue', 8), ('generations', 9), ('africans', 20), ('embattled', 13), ('laurent', 19), ('gbagbo', 28), ('alassane', 7), ('ouattara', 15), ('refusing', 14), ('yield', 13), ('presidency', 86), ('organizing', 20), ('dispatched', 13), ('thabo', 12), ('mbeki', 16), ('meant', 49), ('restore', 63), ('split', 46), ('motorbike', 3), ('guard', 74), ('preempt', 2), ('leftist', 66), ('marching', 5), ('istanbul', 27), ('taksim', 2), ('limits', 34), ('flowers', 9), ('fear', 79), ('observances', 10), ('1977', 10), ('thirty', 9), ('narrowed', 7), ('consideration', 17), ('adel', 9), ('mahdi', 12), ('contention', 3), ('leaves', 42), ('dawa', 3), ('runner', 12), ('belong', 14), ('48', 26), ('challenging', 15), ('shipping', 23), ('magnate', 5), ('kahraman', 1), ('sadikoglu', 1), ('ransom', 60), ('adequately', 5), ('enforced', 3), ('racism', 12), ('intolerance', 6), ('gaps', 4), ('addressing', 21), ('strasbourg', 13), ('adversity', 1), ('essential', 20), ('46', 32), ('brings', 30), ('continent', 56), ('armenia', 15), ('aerial', 7), ('tramway', 3), ('longest', 14), ('serzh', 1), ('sarkisian', 1), ('mountains', 24), ('engineering', 13), ('feat', 3), ('spans', 2), ('vorotan', 1), ('gorge', 5), ('linking', 31), ('highway', 41), ('tatev', 1), ('monastery', 3), ('monasteries', 2), ('attraction', 3), ('develop', 123), ('borders', 122), ('ricardo', 15), ('alarcon', 6), ('gholam', 4), ('haddad', 4), ('resumed', 60), ('thanked', 25), ('indiana', 10), ('dan', 14), ('burton', 2), ('coordination', 22), ('intervene', 13), ('mexican', 106), ('colombian', 111), ('endanger', 4), ('break', 64), ('deadlock', 13), ('insisted', 30), ('alternative', 29), ('austrians', 2), ('object', 13), ('privileged', 5), ('unacceptable', 26), ('goal', 67), ('proposals', 50), ('soften', 1), ('neighbor', 24), ('delayed', 56), ('cooperate', 55), ('commander', 181), ('slaughtering', 3), ('qasab', 3), ('andres', 23), ('valencia', 6), ('eln', 16), ('francisco', 19), ('galan', 7), ('locals', 10), ('sons', 18), ('successful', 45), ('worries', 17), ('buildup', 9), ('practices', 21), ('tone', 7), ('casts', 2), ('sino', 4), ('hu', 80), ('jintao', 43), ('arrive', 31), ('manipulates', 1), ('unfairly', 10), ('cheap', 6), ('changed', 39), ('gripping', 3), ('bringing', 57), ('disarm', 42), ('authorized', 25), ('idriss', 13), ('deby', 23), ("n'djamena", 5), ('exceptional', 4), ('oust', 22), ('decree', 19), ('censor', 4), ('curtail', 11), ('remove', 38), ('command', 57), ('girija', 2), ('prasad', 5), ('koirala', 3), ('represents', 23), ('feelings', 7), ('curtailing', 3), ('reinstate', 7), ('paramilitary', 36), ('grown', 29), ('rallies', 26), ('curbing', 7), ('representing', 24), ('contact', 98), ('colleague', 8), ('saadoun', 2), ('janabi', 1), ('represented', 17), ('deteriorating', 20), ('impossible', 12), ('lawyer', 66), ('murders', 21), ('143', 3), ('adjourned', 15), ('alert', 37), ('tel', 21), ('aviv', 20), ('barricades', 6), ('entry', 34), ('jams', 4), ('importance', 26), ('opium', 33), ('cultivation', 12), ('patrick', 11), ('fequiere', 1), ('distribute', 7), ('identification', 11), ('cards', 11), ('polling', 47), ('places', 35), ('preparations', 20), ('balloting', 25), ('jean', 59), ('betrand', 1), ('aristide', 59), ('calm', 31), ('shipment', 11), ('shoes', 12), ('traders', 22), ('skirted', 2), ('blockade', 34), ('tunnel', 27), ('suffocating', 2), ('israelis', 71), ('ivorian', 9), ('happened', 69), ('siegouekou', 1), ('smashed', 12), ('doors', 9), ('knives', 5), ('throats', 3), ('precious', 6), ('ingredient', 9), ('chocolate', 10), ('drilling', 17), ('pipelines', 15), ('rita', 23), ('barrel', 107), ('falling', 51), ('unlike', 9), ('spike', 7), ('eased', 14), ('analysts', 132), ('diminishing', 3), ('cutting', 46), ('excess', 7), ('refining', 18), ('veered', 5), ('runway', 11), ('flames', 13), ('aboard', 35), ('conflicting', 19), ('fatalities', 23), ('scene', 74), ('originated', 10), ('stopover', 2), ('amman', 18), ('aviation', 27), ('alternatives', 6), ('takeoff', 2), ('115', 11), ('mykola', 1), ('azarov', 2), ('threaten', 22), ('suck', 1), ('neither', 44), ('possess', 7), ('waits', 2), ('deploy', 22), ('hybrid', 13), ('speeded', 1), ('tougher', 13), ('heroin', 21), ('battling', 52), ('directly', 41), ('nighttime', 3), ('karshi', 3), ('khanabad', 3), ('airfield', 4), ('aftermath', 22), ('criticizing', 24), ('fearing', 6), ('bury', 2), ('mudslides', 33), ('typhoons', 4), ('assessing', 8), ('powerful', 77), ('nanmadol', 2), ('packing', 6), ('gloria', 14), ('arroyo', 21), ('heave', 1), ('rescue', 103), ('hungry', 9), ('shelter', 42), ('homeless', 46), ('bekaa', 7), ('remaining', 72), ('en', 9), ('jumblatt', 5), ('cameroon', 17), ('issa', 3), ('tchiroma', 2), ('respective', 6), ('examinations', 2), ('bakassi', 2), ('splinter', 4), ('marine', 56), ('commando', 7), ('ukrainians', 14), ('vessels', 26), ('circulation', 5), ('brain', 27), ('advancement', 2), ('findings', 28), ('flavinols', 2), ('oxidant', 1), ('flow', 32), ('researchers', 40), ('eating', 28), ('bitter', 16), ('taste', 3), ('massacre', 35), ('testing', 37), ('calorie', 3), ('foods', 5), ('a9', 1), ('motorway', 2), ('gleneagles', 4), ('scotland', 20), ('hooded', 5), ('rocks', 18), ('objects', 12), ('stirling', 1), ('railway', 13), ('edge', 5), ('g', 33), ('canceled', 58), ('outbreaks', 52), ('surrounding', 40), ('kouchner', 6), ('turmoil', 22), ('afp', 33), ('pivotal', 1), ('strengthening', 27), ('exposure', 13), ('hospitalized', 35), ('hypothermia', 1), ('implicates', 4), ('hassan', 62), ('majid', 9), ('execution', 35), ('basra', 45), ('rangoon', 45), ('real', 72), ('supplier', 11), ('gambari', 5), ('spate', 6), ('escape', 49), ('inmate', 3), ('lance', 3), ('edward', 8), ('caraballo', 3), ('running', 120), ('torturing', 6), ('lieutenants', 7), ('irna', 18), ('hosseini', 6), ('lessen', 6), ('pain', 15), ('swiss', 46), ('intermediary', 2), ('supporting', 70), ('date', 96), ('expressing', 19), ('apparent', 39), ('abandons', 2), ('participates', 6), ('reject', 31), ('acknowledge', 11), ('exist', 20), ('stress', 8), ('makeshift', 8), ('shelters', 15), ('offenses', 21), ('roaming', 4), ('tent', 10), ('rapes', 8), ('topic', 3), ('input', 1), ('immediate', 112), ('serve', 58), ('volunteers', 22), ('cable', 21), ('notified', 11), ('postings', 1), ('dates', 11), ('harry', 23), ('thomas', 31), ('dismissal', 13), ('extra', 27), ('precedents', 2), ('directed', 29), ('assignments', 4), ('1969', 8), ('aligned', 13), ('nam', 7), ('sidelines', 25), ('havana', 50), ('m', 33), ('fugitive', 26), ('coal', 45), ('warming', 38), ('marthinus', 1), ('schalkwyk', 2), ('mandatory', 7), ('efficiency', 11), ('carbon', 21), ('dioxide', 9), ('emissions', 34), ('greenhouse', 18), ('gases', 7), ('captures', 1), ('stores', 23), ('2025', 2), ('deif', 2), ('humiliation', 2), ('hands', 41), ('removal', 12), ('nothing', 65), ('shaken', 10), ('iftikhar', 5), ('chaudhry', 15), ('abused', 20), ('interfere', 10), ('judiciary', 18), ('swore', 1), ('rana', 1), ('bhagwandas', 1), ('h5n1', 215), ('flu', 579), ('domestic', 108), ('poultry', 121), ('waterfowl', 3), ('bombmaker', 4), ('video', 126), ('profile', 24), ('dark', 10), ('shadow', 19), ('lab', 18), ('verified', 17), ('farm', 100), ('lyons', 1), ('birds', 191), ('slaughtered', 19), ('illness', 37), ('fears', 39), ('persistent', 13), ('knee', 11), ('champion', 66), ('marat', 3), ('safin', 6), ('hopman', 4), ('perth', 6), ('struggled', 16), ('tendonitis', 1), ('masters', 8), ('shanghai', 22), ('rather', 47), ('replaced', 39), ('teimuraz', 2), ('gabashvili', 2), ('svetlana', 1), ('kuznetsova', 1), ('serbia', 58), ('perceive', 1), ('occupying', 11), ('pull', 42), ('defuse', 15), ('convince', 10), ('impulse', 1), ('normalizing', 3), ('sochi', 5), ('hijacker', 4), ('passenger', 44), ('chadian', 21), ('surrendering', 4), ('103', 10), ('fasher', 6), ('pistol', 6), ('asylum', 42), ('extremist', 40), ('noordin', 6), ('subur', 2), ('sugiarto', 2), ('java', 26), ('inner', 9), ('circle', 5), ('accomplices', 4), ('jemaah', 10), ('islamiah', 1), ('arm', 17), ('bali', 34), ('nightclub', 9), ('202', 12), ('medics', 9), ('avenge', 4), ('jihad', 68), ('netanya', 1), ('shaul', 12), ('mofaz', 15), ('shin', 2), ('bet', 5), ('caught', 60), ('maher', 7), ('uda', 3), ('branch', 20), ('detain', 9), ('commented', 29), ('itar', 13), ('tass', 13), ('intoxicated', 2), ('authentic', 3), ('governmental', 23), ('responding', 25), ('nutrition', 8), ('drought', 39), ('famine', 19), ('ethnically', 8), ('yugoslav', 31), ('slobodan', 13), ('milosevic', 42), ('subpoena', 4), ('believers', 5), ('proudly', 1), ('signs', 51), ('encourage', 41), ('infringe', 2), ('separation', 12), ('requesting', 5), ('testimony', 29), ('responses', 4), ('judges', 42), ('instructed', 7), ('writing', 21), ('witness', 29), ('madeleine', 4), ('albright', 1), ('rudolf', 2), ('scharping', 1), ('appointed', 61), ('counsel', 10), ('failing', 59), ('counts', 27), ('conflicts', 32), ('pardoned', 11), ('765', 1), ('pardon', 9), ('boards', 5), ('pardons', 11), ('eve', 53), ('celebration', 18), ('celebrated', 29), ('rest', 60), ('apostolic', 2), ('eucharist', 2), ('specific', 33), ('follows', 63), ('julian', 5), ('calendar', 12), ('gregorian', 1), ('afar', 16), ('examining', 9), ('applications', 8), ('assadullah', 2), ('azhari', 3), ('laboratory', 42), ('detected', 35), ('samples', 44), ('chickens', 61), ('kapisa', 3), ('logar', 8), ('nangarhar', 15), ('suspicions', 7), ('laghman', 5), ('parwan', 2), ('fao', 11), ('sharp', 39), ('controversy', 18), ('conspicuous', 1), ('headscarves', 3), ('skullcaps', 1), ('crucifixes', 2), ('donald', 54), ('rumsfeld', 77), ('mismanaging', 1), ('carolina', 27), ('supporter', 14), ('remembered', 8), ('worst', 89), ('secretaries', 3), ('farewell', 4), ('devotion', 1), ('finest', 1), ('hasty', 1), ('senses', 3), ('too', 100), ('reverence', 2), ('properly', 20), ('pulling', 16), ('reiterated', 11), ('themselves', 60), ('taped', 7), ('failures', 12), ('levels', 76), ('dangers', 9), ('orleans', 95), ('disproportionately', 1), ('blacks', 5), ('quick', 20), ('strokes', 5), ('dramatically', 20), ('journals', 1), ('lancetand', 1), ('lancet', 1), ('neurology', 1), ('counteracting', 1), ('oxford', 2), ('researcher', 2), ('rothwell', 1), ('vast', 29), ('symptoms', 22), ('care', 104), ('professionals', 16), ('aggressive', 25), ('tissue', 5), ('causes', 38), ('facial', 2), ('numbness', 1), ('slurred', 1), ('partial', 20), ('sudden', 7), ('headaches', 3), ('treatments', 6), ('thinning', 2), ('cholesterol', 4), ('medications', 6), ('crude', 147), ('inventories', 19), ('reflecting', 3), ('decreased', 17), ('35', 73), ('cents', 57), ('68', 14), ('tenths', 15), ('publishing', 17), ('cartoons', 46), ('bayji', 4), ('rubble', 27), ('observed', 23), ('guided', 4), ('munitions', 8), ('emile', 16), ('lahoud', 25), ('slain', 20), ('express', 20), ('condolences', 21), ('mourners', 20), ('drawings', 4), ('project', 100), ('traces', 6), ('05', 13), ('recorded', 34), ('easing', 20), ('weakening', 10), ('tangible', 3), ('attractive', 7), ('investors', 70), ('speculators', 3), ('interpreted', 6), ('ben', 20), ('bernanke', 16), ('pointing', 3), ('reductions', 5), ('weaken', 19), ('exporting', 33), ('opec', 60), ('cartel', 25), ('pump', 8), ('gerard', 12), ('latortue', 14), ('bertrand', 29), ('disagrees', 3), ('publication', 19), ('blasphemous', 9), ('objection', 4), ('exile', 57), ('followers', 22), ('foes', 4), ('ignore', 9), ('prohibiting', 4), ('disturbing', 2), ('trend', 13), ('exceptions', 2), ('prohibitions', 1), ('perceptions', 3), ('tool', 7), ('instrument', 4), ('33', 40), ('alarm', 5), ('circumvent', 2), ('wording', 2), ('inconvenient', 2), ('meteorologists', 5), ('strengthened', 22), ('category', 19), ('missed', 25), ('opportunity', 46), ('120', 44), ('bahamas', 13), ('recruited', 7), ('khaled', 18), ('meshaal', 7), ('viewed', 12), ('assassinated', 20), ('unusually', 7), ('affecting', 9), ('baltic', 14), ('nordic', 9), ('shaukat', 18), ('aziz', 54), ('buses', 22), ('negotiator', 45), ('produce', 75), ('purposes', 40), ('rohani', 2), ('length', 11), ('vigilant', 4), ('fails', 21), ('uphold', 4), ('imposition', 4), ('shrapnel', 5), ('mcdonald', 10), ('st', 35), ('petersburg', 12), ('injuring', 35), ('shattered', 10), ('ceiling', 3), ('environmental', 65), ('disprove', 1), ('secretly', 48), ('investigators', 75), ('table', 14), ('nevsky', 2), ('prospekt', 2), ('tracked', 4), ('drone', 35), ('airspace', 21), ('ten', 25), ('meddling', 3), ('salons', 1), ('beauty', 9), ('parlors', 1), ('cater', 2), ('enforce', 14), ('stricter', 3), ('interpretation', 2), ('reza', 22), ('asefi', 16), ('installation', 8), ('cafes', 7), ('shops', 37), ('unsuitable', 1), ('fundamentalist', 4), ('brand', 10), ('hairdresser', 1), ('impacted', 6), ('ghoul', 2), ('violate', 29), ('makeup', 6), ('nulcear', 1), ('alleges', 13), ('parchin', 10), ('photographer', 12), ('gabriele', 5), ('torsello', 10), ('libya', 38), ('advised', 15), ('kind', 25), ('broadcasters', 6), ('indecent', 3), ('broadcasting', 26), ('supervise', 3), ('content', 13), ('films', 21), ('platform', 19), ('conservative', 72), ('principles', 12), ('performances', 6), ('sharply', 73), ('1979', 28), ('snyder', 3), ('rini', 5), ('appointment', 20), ('honiara', 3), ('downer', 10), ('survival', 6), ('businessmen', 19), ('110', 17), ('retrieved', 5), ('recorder', 4), ('wreckage', 21), ('airliner', 15), ('box', 11), ('blizzard', 5), ('survived', 53), ('asserts', 3), ('addicts', 1), ('rehabilitation', 13), ('lined', 6), ('clients', 12), ('tijuana', 4), ('identify', 42), ('gangs', 35), ('engaged', 33), ('cartels', 14), ('felipe', 21), ('calderon', 20), ('105', 14), ('tons', 62), ('marijuana', 7), ('bust', 2), ('holmes', 2), ('horn', 16), ('outskirts', 24), ('squalid', 1), ('munich', 8), ('brutalized', 1), ('amount', 46), ('shirin', 3), ('ebadi', 5), ('summons', 2), ('blatant', 4), ('voices', 6), ('complaint', 16), ('cast', 50), ('doubt', 21), ('noting', 22), ('handles', 5), ('matters', 14), ('bases', 50), ('restructure', 6), ('installations', 15), ('realignment', 1), ('jersey', 17), ('virginia', 20), ('commissioners', 1), ('deliberations', 1), ('mcpherson', 1), ('closures', 6), ('recommended', 25), ('maine', 5), ('hawaii', 9), ('zawahri', 4), ('trained', 33), ('save', 37), ('certify', 2), ('submit', 17), ('husband', 42), ('instantly', 3), ('transporting', 20), ('planted', 29), ('mistook', 6), ('toy', 8), ('zaman', 2), ('shaped', 10), ('dir', 5), ('placed', 69), ('accidentally', 18), ('deliberately', 11), ('taxi', 11), ('hover', 1), ('degrees', 18), ('celsius', 13), ('hurriyet', 1), ('unlicensed', 3), ('cds', 2), ('laptops', 2), ('pech', 1), ('kunar', 35), ('nationality', 17), ('whom', 47), ('younus', 1), ('khalis', 7), ('thwarted', 6), ('giuseppe', 1), ('pisanu', 3), ('cagliari', 1), ('sardinia', 2), ('milan', 11), ('subway', 41), ('basilica', 11), ('bologna', 2), ('expelled', 19), ('petronio', 1), ('tycoon', 14), ('khodorkovsky', 40), ('conviction', 21), ('features', 16), ('fresco', 1), ('insulting', 11), ('italians', 6), ('strictly', 7), ('regulate', 8), ('suggestions', 11), ('submission', 3), ('amendments', 21), ('presently', 3), ('branches', 8), ('various', 52), ('oversight', 11), ('reflection', 6), ('kremlin', 38), ('misanthropic', 1), ('ideologies', 2), ('crucial', 28), ('afford', 13), ('pretrial', 2), ('businessman', 22), ('complicity', 8), ('selling', 46), ('ingredients', 6), ('frans', 1), ('anraat', 3), ('rotterdam', 1), ('richest', 9), ('retaliation', 27), ('backing', 43), ('62', 17), ('defendant', 20), ('chemicals', 17), ('halabja', 6), ('girl', 75), ('underway', 26), ('motions', 2), ('fatality', 10), ('73', 31), ('171', 5), ('59', 24), ('coinciding', 3), ('drawdown', 2), ('innocence', 5), ('bureaucrats', 1), ('opposed', 59), ('renaming', 1), ('advice', 9), ('arresting', 17), ('adnan', 7), ('dulaimi', 7), ('shocked', 8), ('sectarianism', 1), ('marginalized', 2), ('giant', 69), ('yukos', 56), ('zeng', 5), ('qinghong', 2), ('technological', 6), ('exploration', 28), ('shortfall', 6), ('trinidad', 2), ('tobago', 2), ('balad', 21), ('wrapped', 13), ('blankets', 7), ('arriving', 36), ('tikrit', 28), ('reconcile', 6), ('discrepancy', 1), ('suspending', 18), ('shortage', 27), ('roadway', 1), ('festivities', 11), ('bicycle', 13), ('inquiry', 32), ('benazir', 12), ('bhutto', 23), ('ki', 26), ('creation', 39), ('fact', 21), ('widower', 2), ('asif', 20), ('zardari', 31), ('medalist', 11), ('reasons', 40), ('au', 98), ('evi', 1), ('sachenbacher', 1), ('naturally', 3), ('endurance', 1), ('epo', 1), ('kikkan', 1), ('randall', 1), ('leif', 1), ('zimmermann', 2), ('suspensions', 4), ('punishments', 4), ('retroactive', 3), ('races', 6), ('worse', 26), ('superiors', 4), ('assert', 5), ('refinery', 28), ('repairs', 10), ('optimistic', 17), ('view', 30), ('scattered', 9), ('h', 16), ('grubbs', 2), ('schrock', 2), ('yves', 2), ('chauvin', 2), ('academy', 19), ('sciences', 5), ('stockholm', 10), ('trio', 7), ('environmentally', 7), ('plastics', 1), ('1971', 10), ('metathesis', 1), ('molecules', 2), ('rearranged', 1), ('developed', 71), ('efficient', 18), ('catalysts', 1), ('reproduce', 2), ('scientist', 22), ('centrifuges', 13), ('qadeer', 8), ('hindering', 4), ('impassable', 2), ('irresponsible', 11), ('father', 76), ('virtual', 9), ('secrets', 15), ('ecowas', 5), ('togo', 35), ('togolese', 13), ('faure', 10), ('gnassingbe', 32), ('installed', 31), ('reacting', 4), ('eyadema', 11), ('naming', 6), ('branded', 2), ('seizure', 22), ('violation', 34), ('english', 45), ('beckham', 9), ('premier', 12), ('tottenham', 2), ('hotspur', 1), ('stint', 2), ('club', 30), ('rationing', 3), ('hoped', 26), ('galaxy', 6), ('mls', 3), ('teammates', 2), ('reluctant', 5), ('offseason', 1), ('tore', 17), ('achilles', 2), ('tendon', 1), ('ac', 3), ('hotspurs', 1), ('redknapp', 1), ('unconditional', 4), ('tin', 12), ('76th', 3), ('birthday', 20), ('without', 281), ('write', 14), ('proper', 17), ('chronic', 11), ('ailments', 6), ('propaganda', 21), ('bombed', 27), ('tanker', 24), ('attached', 20), ('khyber', 18), ('regularly', 21), ('pass', 62), ('inspector', 19), ('earmarked', 5), ('stuart', 8), ('bowen', 2), ('documentation', 5), ('incompetence', 3), ('haste', 4), ('account', 64), ('indications', 8), ('militia', 90), ('taxpayer', 3), ('designated', 24), ('difficulties', 18), ('kiev', 39), ('vow', 7), ('repeat', 14), ('presidents', 56), ('aleksander', 2), ('kwasniewski', 3), ('valdas', 3), ('alpha', 13), ('dominican', 20), ('barahona', 1), ('maximum', 24), ('22nd', 4), ('breaking', 21), ('greek', 70), ('alphabet', 3), ('turks', 16), ('caicos', 2), ('consumption', 17), ('switching', 1), ('proponents', 3), ('cleaning', 11), ('clean', 35), ('stiff', 12), ('mistakenly', 13), ('miners', 55), ('henan', 5), ('eilat', 2), ('cocked', 1), ('weapon', 42), ('realized', 8), ('chest', 12), ('withheld', 7), ('guardian', 14), ('armorgroup', 2), ('employee', 23), ('withhold', 4), ('magna', 8), ('mcalpine', 8), ('frank', 19), ('stronach', 5), ('stepping', 19), ('automotive', 7), ('xinhua', 126), ('outburst', 2), ('dengfeng', 1), ('overhead', 7), ('satisfactory', 4), ('profit', 35), ('achieved', 34), ('stephen', 18), ('akerfeldt', 1), ('load', 12), ('enters', 2), ('downturn', 30), ('declines', 12), ('dividend', 8), ('shares', 57), ('wallowing', 1), ('125', 10), ('canadian', 84), ('stock', 85), ('yesterday', 18), ('625', 3), ('controlling', 13), ('shareholder', 8), ('unsuccessfully', 7), ('seat', 72), ('throughout', 83), ('personally', 13), ('restructuring', 23), ('assisted', 10), ('manfred', 3), ('gingl', 1), ('108', 2), ('consulting', 9), ('caribs', 1), ('colonization', 3), ('saint', 35), ('1719', 2), ('18th', 24), ('ceded', 16), ('1783', 4), ('1960', 26), ('1962', 19), ('grenadines', 5), ('indies', 9), ('slovene', 3), ('austro', 9), ('hungarian', 24), ('dissolution', 9), ('1918', 6), ('slovenes', 4), ('serbs', 24), ('croats', 14), ('multinational', 15), ('1929', 7), ('slovenia', 19), ('distanced', 4), ('dissatisfied', 6), ('exercise', 37), ('succeeded', 24), ('1991', 86), ('historical', 8), ('transformation', 7), ('acceded', 3), ('eurozone', 2), ('15th', 19), ('crnojevic', 1), ('dynasty', 9), ('serbian', 60), ('principality', 9), ('zeta', 2), ('subsequent', 28), ('ottoman', 16), ('theocracy', 1), ('bishop', 9), ('princes', 1), ('1852', 1), ('secular', 27), ('absorbed', 4), ('constituent', 7), ('1992', 37), ('looser', 1), ('invoked', 2), ('severing', 3), ('exceeded', 9), ('threshold', 2), ('declare', 21), ('basutoland', 1), ('renamed', 5), ('lesotho', 9), ('1966', 8), ('basuto', 1), ('hegang', 1), ('heilongjiang', 6), ('moshoeshoe', 1), ('exiled', 22), ('1990', 61), ('letsie', 1), ('iii', 7), ('restored', 38), ('mutiny', 2), ('contentious', 10), ('botswana', 12), ('aegis', 1), ('relative', 22), ('hotly', 6), ('contested', 16), ('aggrieved', 2), ('applied', 12), ('proportional', 2), ('mainstays', 4), ('enjoys', 11), ('compared', 54), ('curacao', 5), ('excellent', 10), ('harbor', 14), ('accommodate', 5), ('tankers', 14), ('leases', 2), ('single', 59), ('refined', 6), ('deadliest', 52), ('cave', 8), ('ins', 4), ('attempting', 27), ('soils', 2), ('hamper', 6), ('budgetary', 8), ('complicate', 4), ('pension', 18), ('systems', 47), ('quarrel', 3), ('arisen', 1), ('horse', 15), ('stag', 9), ('hunter', 21), ('revenge', 11), ('conquer', 5), ('iron', 15), ('jaws', 1), ('guide', 6), ('reins', 2), ('saddle', 1), ('saddled', 2), ('bridled', 1), ('overcame', 6), ('mouth', 16), ('friend', 24), ('bit', 18), ('spur', 11), ('prefer', 4), ('theirs', 1), ('violated', 22), ('vietnamese', 22), ('raising', 50), ('foil', 2), ('pomegranate', 1), ('tree', 28), ('beautiful', 6), ('height', 9), ('bramble', 4), ('hedge', 4), ('boastful', 1), ('dear', 2), ('vain', 4), ('disputings', 1), ('flourishing', 2), ('traveller', 3), ('splendid', 3), ('orders', 48), ('fill', 15), ('inquired', 7), ('replied', 35), ('boxing', 2), ('gloves', 1), ('tongues', 1), ('pugilists', 1), ('statistician', 1), ('personality', 4), ('accountant', 2), ('ellsworth', 3), ('dakota', 5), ('generals', 28), ('commanding', 8), ('heard', 43), ('frost', 1), ('noon', 5), ('scrambling', 5), ('curfews', 13), ('musicians', 11), ('o', 9), ('whispered', 1), ('gauge', 5), ('index', 49), ('indicators', 7), ('predict', 18), ('prompting', 23), ('kfm', 1), ('andrew', 17), ('mwenda', 3), ('junk', 4), ('sedition', 1), ('yoweri', 13), ('museveni', 22), ('rory', 1), ('irishman', 1), ('assignment', 2), ('vanished', 6), ('airports', 23), ('iskandariyah', 6), ('counting', 21), ('auditing', 4), ('ecuadorean', 11), ('migrants', 36), ('sank', 9), ('hoping', 31), ('airplane', 10), ('ecuador', 76), ('participating', 20), ('doomed', 2), ('sail', 5), ('manta', 1), ('ivanov', 29), ('evolves', 1), ('broadly', 3), ('participate', 43), ('reverse', 23), ('sterilization', 3), ('surgery', 55), ('12th', 13), ('opt', 1), ('students', 88), ('quell', 16), ('grieving', 7), ('parents', 31), ('govern', 7), ('strategies', 9), ('divisions', 13), ('globalization', 10), ('resolving', 27), ('stockhlom', 1), ('harold', 1), ('pinter', 1), ('era', 31), ('mocked', 1), ('centimeter', 3), ('shahr', 1), ('kord', 1), ('incentives', 43), ('javier', 16), ('solana', 25), ('mitchell', 17), ('holds', 45), ('launches', 12), ('saeb', 11), ('erekat', 11), ('shuttle', 48), ('forth', 5), ('firefight', 19), ('gunfight', 13), ('raz', 1), ('implicated', 19), ('hunting', 28), ('loyalists', 5), ('annulled', 4), ('venezuelans', 7), ('neighbors', 48), ('mistakes', 5), ('corrected', 4), ('amend', 11), ('overseen', 3), ('promotes', 3), ('landmark', 22), ('turnout', 11), ('erben', 2), ('drop', 61), ('intermittent', 3), ('sixto', 3), ('theft', 11), ('kanyabayonga', 2), ('units', 30), ('reinforcements', 7), ('kinshasa', 15), ('rwanda', 61), ('rwandan', 48), ('hutu', 33), ('1994', 62), ('zoellick', 23), ('ninh', 3), ('binh', 1), ('tested', 65), ('intergovernmental', 5), ('learning', 11), ('wen', 17), ('jiabao', 12), ('li', 18), ('zhaoxing', 3), ('chengdu', 3), ('rio', 19), ('janeiro', 15), ('clashing', 3), ('daylight', 5), ('exchanged', 12), ('lobbing', 3), ('downtown', 17), ('providencia', 10), ('shantytown', 3), ('fragments', 2), ('barracks', 7), ('mobilized', 5), ('slum', 11), ('dwellers', 2), ('complained', 32), ('kills', 14), ('apologized', 18), ('nana', 1), ('effah', 1), ('apenteng', 1), ('drafting', 18), ('resolve', 87), ('precede', 1), ('urgency', 2), ('chirac', 49), ('renovation', 2), ('blackouts', 3), ('cubans', 25), ('plants', 39), ('ones', 23), ('generators', 3), ('electrical', 19), ('hottest', 2), ('conditioning', 1), ('surges', 4), ('appliances', 2), ('rot', 1), ('admitted', 53), ('radios', 11), ('resold', 1), ('stray', 3), ('bullets', 12), ('siad', 13), ('barre', 16), ('fence', 23), ('kissufin', 1), ('crossing', 89), ('spotting', 1), ('holed', 6), ('tanks', 28), ('karni', 6), ('terminal', 5), ('bulldozers', 7), ('farmlands', 1), ('yunis', 2), ('incursions', 6), ('bolivian', 25), ('evo', 22), ('morales', 55), ('welcomes', 8), ('forgives', 2), ('humiliations', 2), ('inauguration', 24), ('welcome', 24), ('coca', 17), ('eradication', 9), ('campaigned', 8), ('uses', 20), ('cocaine', 26), ('sure', 30), ('bolivia', 62), ('plotting', 46), ('institution', 12), ('ridiculous', 6), ('regret', 11), ('apart', 22), ('mulford', 4), ('sincere', 7), ('regrets', 9), ('context', 1), ('shyam', 4), ('saran', 5), ('summoned', 14), ('inappropriate', 10), ('conductive', 1), ('inspection', 15), ('searches', 13), ('factories', 21), ('moderate', 53), ('densely', 11), ('populated', 18), ('confined', 7), ('uncovered', 32), ('laboratories', 8), ('spark', 17), ('cycle', 7), ('hub', 13), ('defending', 23), ('74', 12), ('welfare', 18), ('alwi', 1), ('shihab', 3), ('measured', 10), ('magnitude', 50), ('obstructing', 5), ('deliveries', 19), ('deny', 32), ('petersen', 7), ('kilinochchi', 3), ('reviving', 6), ('assess', 19), ('progressive', 17), ('225', 10), ('unification', 24), ('386', 2), ('vied', 1), ('176', 1), ('allocated', 8), ('proportion', 5), ('receives', 6), ('hindu', 19), ('kush', 1), ('hijacking', 10), ('somalians', 2), ('miltzow', 2), ('unloaded', 2), ('afternoon', 38), ('merka', 1), ('boarded', 9), ('maize', 4), ('freighter', 3), ('torgelow', 2), ('semlow', 4), ('sailed', 5), ('kenya', 121), ('donated', 17), ('felt', 12), ('peshawar', 24), ('chitral', 3), ('inspect', 13), ('turnabout', 1), ('brothers', 18), ('ahbash', 3), ('refuses', 12), ('sponsoring', 3), ('draft', 78), ('veto', 28), ('oppose', 55), ('saad', 13), ('vaccination', 12), ('damages', 21), ('tigray', 1), ('amhara', 1), ('oromia', 1), ('kicked', 13), ('immunizations', 5), ('judicial', 28), ('experience', 30), ('alito', 25), ('appeals', 37), ('qualities', 2), ('mastery', 4), ('disappointed', 11), ('retiring', 10), ('sandra', 11), ("o'connor", 12), ('nominated', 31), ('harriet', 4), ('miers', 8), ('lacked', 9), ('humayun', 1), ('hamidzada', 2), ('colleagues', 22), ('persona', 1), ('grata', 1), ('tribes', 12), ('misunderstanding', 2), ('aleem', 2), ('siddique', 2), ('clarify', 3), ('wedding', 9), ('bilge', 1), ('ankara', 40), ('mardin', 1), ('allotment', 1), ('besir', 1), ('atalay', 1), ('ntv', 2), ('clan', 12), ('feud', 7), ('democratization', 4), ('farmaner', 1), ('forget', 2), ('suppression', 7), ('legislators', 22), ('extending', 32), ('roh', 15), ('moo', 11), ('hyun', 11), ('endorsed', 31), ('contingent', 21), ('assigned', 8), ('arbil', 3), ('secondhand', 4), ('smoke', 19), ('sections', 9), ('nonsmokers', 2), ('restaurants', 15), ('surgeon', 5), ('really', 10), ('tobacco', 17), ('infant', 8), ('lung', 7), ('infections', 19), ('asthma', 3), ('prevention', 16), ('430', 5), ('portugal', 32), ('weekend', 27), ('lisbon', 3), ('ticket', 10), ('charities', 8), ('lineup', 5), ('wide', 53), ('incoming', 16), ('implies', 2), ('nominees', 18), ('objected', 9), ('am', 24), ('rugova', 4), ('motorcade', 13), ('window', 15), ('blown', 18), ('schedule', 30), ('proves', 5), ('elements', 16), ('hundred', 34), ('crawford', 10), ('ranch', 28), ('elevated', 3), ('shift', 15), ('hatched', 1), ('capitals', 8), ('candle', 1), ('theater', 14), ('beni', 3), ('suef', 2), ('triggering', 24), ('stampede', 10), ('exit', 16), ('burning', 32), ('storey', 1), ('blaze', 15), ('350', 17), ('farah', 14), ('encountered', 16), ('contrast', 4), ('60th', 19), ('protested', 34), ('calmly', 1), ('baden', 2), ('angela', 31), ('merkel', 59), ('hall', 37), ('cordon', 3), ('detaining', 12), ('cindy', 4), ('sheehan', 6), ('rationale', 2), ('trash', 8), ('bins', 1), ('absence', 13), ('generating', 9), ('shwe', 21), ('maung', 7), ('aye', 4), ('hosted', 18), ('routine', 18), ('suffers', 15), ('hypertension', 2), ('rumors', 6), ('gravely', 3), ('squared', 2), ('motors', 24), ('loss', 78), ('gm', 14), ('posted', 66), ('quarter', 89), ('722', 2), ('buyouts', 1), ('hourly', 1), ('automaker', 11), ('struggling', 51), ('cars', 67), ('dana', 8), ('perino', 7), ('competitive', 11), ('daniele', 4), ('mastrogiacomo', 10), ('repubblica', 4), ('ettore', 1), ('francesco', 1), ('sequi', 1), ('covering', 21), ('rajouri', 2), ('miles', 5), ('responsiblity', 1), ('avian', 58), ('influenza', 20), ('understands', 8), ('sentiments', 4), ('rivals', 32), ('explained', 8), ('purpose', 16), ('mediation', 10), ('battles', 21), ('capabilities', 17), ('historic', 34), ('sydney', 21), ('marketplace', 5), ('forum', 31), ('ore', 7), ('negotiated', 14), ('zealand', 54), ('mired', 9), ('elderly', 15), ('bullet', 11), ('riddled', 6), ('note', 20), ('else', 18), ('remnants', 16), ('reversing', 4), ('57', 29), ('claude', 3), ('juncker', 2), ('staunch', 3), ('denmark', 45), ('worrisome', 1), ('resurgence', 3), ('gears', 1), ('juncture', 1), ('prepares', 19), ('ouster', 23), ('benefit', 24), ('tainted', 9), ('criminals', 31), ('dubious', 1), ('origin', 15), ('pushed', 64), ('militarized', 3), ('crossings', 15), ('directions', 3), ('khalikov', 1), ('approaching', 15), ('needing', 1), ('diarrhea', 10), ('judgments', 2), ('chair', 14), ('ministerial', 10), ('kuwait', 51), ('kingpin', 5), ('orlandez', 3), ('gamboa', 3), ('smuggled', 16), ('boasted', 2), ('lords', 3), ('covertly', 4), ('extradited', 21), ('laundering', 21), ('barranquilla', 1), ('shipped', 11), ('confederation', 11), ('entities', 11), ('inflated', 6), ('losses', 64), ('hurt', 97), ('ultimately', 11), ('counterproductive', 5), ('habits', 6), ('distorting', 3), ('structure', 25), ('salary', 10), ('95', 31), ('employed', 17), ('laid', 23), ('leadership', 79), ('harms', 3), ('plo', 2), ('excuse', 9), ('obstruct', 1), ('reciprocated', 1), ('bp', 20), ('dudley', 3), ('signaling', 4), ('tnk', 4), ('hayward', 2), ('discussions', 43), ('sale', 54), ('spill', 23), ('compelled', 5), ('skeptical', 2), ('replaces', 9), ('monterrey', 3), ('enrique', 4), ('barrios', 1), ('gate', 13), ('reynaldo', 1), ('ramos', 4), ('mayor', 69), ('fernando', 7), ('larrazabal', 1), ('nuevo', 10), ('leon', 2), ('collusion', 1), ('seventh', 38), ('nigerian', 84), ('abuja', 17), ('indefinite', 5), ('postponement', 9), ('nureddin', 1), ('mezni', 2), ('logistical', 19), ('escalating', 20), ('infighting', 14), ('jendayi', 2), ('frazer', 2), ('khalid', 14), ('kidwai', 1), ('alertness', 1), ('conceivable', 1), ('scenario', 5), ('timessays', 1), ('suggests', 13), ('khawaza', 2), ('kehla', 1), ('staving', 1), ('matter', 56), ('abandoned', 29), ('lodged', 3), ('dam', 25), ('memorandum', 8), ('bunji', 1), ('hydroelectric', 8), ('227', 4), ('hailed', 20), ('123', 10), ('dogharoun', 1), ('khatami', 39), ('desires', 2), ('studying', 15), ('salahaddin', 1), ('zafaraniyah', 1), ('reutersnews', 1), ('swapping', 1), ('clothes', 15), ('individuals', 30), ('visitors', 32), ('swapped', 1), ('questioning', 38), ('expires', 26), ('indefinitely', 10), ('warring', 11), ('organize', 12), ('driven', 53), ('nasa', 55), ('phoenix', 14), ('mars', 30), ('ideal', 5), ('planet', 27), ('polar', 15), ('subterranean', 1), ('ice', 49), ('dig', 9), ('martian', 7), ('layer', 2), ('landing', 24), ('glitch', 2), ('ranging', 19), ('negative', 15), ('headline', 1), ('vh1', 1), ('feature', 7), ('composite', 9), ('tumbled', 2), ('nikkei', 11), ('sensex', 1), ('mumbai', 31), ('lingering', 5), ('hang', 11), ('seng', 9), ('indexes', 11), ('frankfurt', 9), ('plunge', 5), ('revitalize', 5), ('rebates', 3), ('reserve', 47), ('meets', 28), ('stave', 2), ('deploying', 10), ('moshe', 6), ('yaalon', 3), ('breakthrough', 11), ('mamadou', 8), ('tandja', 15), ('seyni', 2), ('oumarou', 4), ('returning', 58), ('posts', 26), ('toppled', 21), ('hama', 1), ('amadou', 4), ('keeping', 28), ('aichatou', 1), ('mindaoudou', 1), ('albade', 1), ('abouba', 1), ('mahamane', 1), ('lamine', 1), ('zeine', 1), ('spiritual', 25), ('dalai', 36), ('lama', 41), ('buddhist', 34), ('admired', 1), ('teachings', 6), ('leonid', 15), ('kuchma', 20), ('yanukovich', 2), ('backer', 5), ('farce', 3), ('unforseeable', 1), ('inadmissible', 2), ('tim', 9), ('nardiello', 4), ('skeleton', 6), ('arbitrator', 1), ('sexually', 19), ('bobsled', 1), ('effective', 49), ('rejoin', 4), ('altenberg', 1), ('sliders', 2), ('zach', 1), ('lund', 2), ('publicly', 33), ('whistleblower', 1), ('revealing', 1), ('technician', 6), ('contacting', 5), ('warheads', 12), ('neutron', 1), ('hydrogen', 2), ('convert', 13), ('cameraman', 12), ('pointless', 1), ('stories', 10), ('victim', 54), ('outlet', 3), ('abductions', 16), ('implicitly', 1), ('critically', 13), ('beersheba', 1), ('larijani', 21), ('rush', 11), ('settlers', 54), ('mashaal', 10), ('unrealistic', 2), ('assassinations', 7), ('inform', 12), ('resuming', 18), ('self', 57), ('underestimated', 1), ('richards', 10), ('eye', 17), ('ball', 12), ('vacuum', 8), ('nawzad', 1), ('landmine', 15), ('confiscated', 21), ('hide', 14), ('gunbattle', 29), ('myanmar', 17), ('tachileik', 2), ('mekong', 5), ('drivers', 19), ('tents', 16), ('blocks', 13), ('caffeine', 1), ('methamphetamine', 5), ('tablets', 1), ('restive', 45), ('instill', 4), ('beheadings', 7), ('deliberate', 6), ('756', 2), ('hurting', 11), ('fixed', 17), ('retirees', 3), ('golden', 8), ('grapple', 1), ('retirement', 38), ('mil', 19), ('arcega', 19), ('holiest', 9), ('najaf', 16), ('carnage', 2), ('risen', 49), ('66', 12), ('clearly', 6), ('ignite', 2), ('degraded', 2), ('wetlands', 7), ('pandemic', 36), ('nairobi', 41), ('habitats', 6), ('ponds', 1), ('paddy', 4), ('isolation', 23), ('fixes', 1), ('suit', 18), ('slap', 3), ('dahoun', 1), ('gignor', 1), ('cheering', 7), ('forcefully', 3), ('evict', 5), ('demobilized', 6), ('suburb', 20), ('tabarre', 1), ('whatever', 8), ('ex', 30), ('rebellion', 23), ('identified', 118), ('39', 25), ('spaniard', 9), ('moutaz', 1), ('almallah', 3), ('dabas', 3), ('warrant', 24), ('slough', 1), ('bow', 4), ('magistrate', 3), ('mohannad', 1), ('passport', 11), ('revitalizing', 2), ('jumpstart', 2), ('assure', 4), ('alleging', 17), ('farfetched', 1), ('stops', 17), ('michael', 67), ('montedison', 3), ('v', 20), ('tender', 4), ('outstanding', 21), ('erbamont', 2), ('pharmaceuticals', 8), ('incorporated', 8), ('advertised', 1), ('72', 21), ('pursuant', 3), ('volta', 1), ('coups', 12), ('multiparty', 13), ('blaise', 2), ('compaore', 2), ('1987', 17), ('securely', 1), ('durable', 5), ('density', 3), ('unrest', 60), ('cote', 11), ("d'ivoire", 9), ('ghana', 33), ('seasonal', 8), ('burkinabe', 1), ('wealthiest', 7), ('republics', 16), ('output', 56), ('waves', 34), ('fortunes', 5), ('rebound', 8), ('credit', 56), ('tame', 1), ('kuna', 1), ('nevertheless', 14), ('stubbornly', 2), ('uneven', 5), ('retains', 8), ('privatization', 23), ('stabilization', 12), ('lag', 1), ('accession', 11), ('accelerate', 6), ('anemic', 4), ('annexed', 19), ('thirds', 38), ('mauritania', 30), ('guerrilla', 27), ('polisario', 11), ('contesting', 5), ('algeria', 24), ('genoese', 1), ('fortress', 1), ('monaco', 7), ('1215', 1), ('grimaldi', 1), ('1297', 1), ('1331', 1), ('1419', 1), ('spurred', 15), ('railroad', 4), ('linkup', 1), ('casino', 7), ('mild', 9), ('scenery', 1), ('gambling', 7), ('recreation', 2), ('crab', 3), ('forsaking', 1), ('seashore', 5), ('meadow', 2), ('feeding', 7), ('ate', 9), ('deserve', 7), ('adapted', 1), ('contentment', 1), ('element', 6), ('happiness', 2), ('groom', 2), ('currycombing', 1), ('rubbing', 1), ('stole', 12), ('oats', 1), ('alas', 2), ('wish', 9), ('walking', 10), ('here', 24), ('scoundrel', 2), ('cried', 7), ('answered', 7), ('makers', 10), ('mustard', 3), ('pressed', 10), ('specifics', 4), ('might', 87), ('product', 17), ('rochester', 1), ('ny', 1), ('thing', 12), ('yellow', 10), ('pitching', 2), ('unfortunately', 6), ('mother', 46), ('prayed', 11), ('fervently', 1), ('prayer', 22), ('excessively', 2), ('styling', 1), ('gel', 1), ('dragged', 6), ('independently', 14), ('thwart', 7), ('chidambaram', 3), ('alarmist', 1), ('preparedness', 2), ('westerners', 16), ('destination', 16), ('festivals', 2), ('advisories', 1), ('holidays', 11), ('commemoration', 6), ('colonists', 3), ('kitts', 8), ('montserrat', 2), ('1632', 1), ('flee', 29), ('fulfilled', 5), ('promise', 24), ('froce', 1), ('zimbabwe', 96), ('mugabe', 43), ('chinamasa', 4), ('chairmanship', 9), ('chiweshe', 3), ('zanu', 6), ('pf', 6), ('draw', 39), ('mdc', 10), ('redistricting', 1), ('constituencies', 5), ('possession', 21), ('reflects', 9), ('inclusive', 3), ('consultation', 3), ('incorporating', 1), ('substantial', 35), ('frenzy', 1), ('lawmaking', 1), ('25th', 4), ('1980', 18), ('complied', 6), ('qualifiers', 2), ('surprising', 2), ('reigning', 6), ('champions', 8), ('blowing', 10), ('horns', 7), ('singing', 5), ('praises', 5), ('menas', 1), ('pharaohs', 3), ('stunning', 2), ('faithful', 13), ('converted', 7), ('landholdings', 1), ('fifa', 29), ('ranks', 19), ('154th', 1), ('niamey', 2), ('stade', 2), ('général', 1), ('kountché', 1), ('kick', 8), ('blistering', 1), ('sun', 26), ('striker', 5), ('ouwa', 1), ('moussa', 15), ('maazou', 3), ('scored', 56), ('getting', 47), ('defender', 3), ('abel', 3), ('shafy', 1), ('goalkeeper', 1), ('essam', 2), ('hadary', 1), ('bordeaux', 1), ('net', 38), ('equalizer', 1), ('rarely', 8), ('midfield', 1), ('fathallah', 1), ('offside', 1), ('solid', 19), ('drops', 11), ('bottom', 6), ('01', 12), ('inauspicious', 1), ('continental', 10), ('johannesburg', 17), ('eruption', 9), ('soufriere', 1), ('volcano', 16), ('hosts', 14), ('pushes', 3), ('qualify', 5), ('gabon', 17), ('hometown', 13), ('intensified', 24), ('inconclusive', 4), ('governing', 34), ('sylvester', 1), ('stallone', 2), ('fines', 15), ('importing', 7), ('hormone', 3), ('movie', 36), ('room', 23), ('possessing', 8), ('enhancing', 7), ('endured', 11), ('occurring', 6), ('penalty', 32), ('fine', 25), ('unlikely', 17), ('collided', 9), ('tractor', 7), ('maharashtra', 9), ('bombay', 9), ('nagpur', 2), ('tracks', 8), ('trains', 20), ('collision', 10), ('punjab', 10), ('laloo', 1), ('yadav', 3), ('pages', 8), ('oral', 2), ('histories', 2), ('transmissions', 3), ('robust', 12), ('firefighters', 18), ('twin', 15), ('towers', 5), ('sued', 9), ('privacy', 7), ('jeopardize', 5), ('zacarias', 3), ('moussaoui', 4), ('hazardous', 8), ('waste', 19), ('shores', 1), ('containers', 10), ('illnesses', 6), ('radiation', 2), ('sickness', 1), ('ulcers', 2), ('abdominal', 5), ('hemorrhages', 1), ('unusual', 10), ('skin', 7), ('dumping', 7), ('toxic', 23), ('accelerated', 4), ('dislodged', 1), ('davos', 4), ('switzerland', 49), ('taro', 8), ('aso', 15), ('lend', 5), ('rebounded', 16), ('announce', 33), ('stormy', 4), ('jabella', 1), ('grapes', 3), ('citrus', 7), ('fruits', 7), ('hazelnuts', 1), ('manganese', 3), ('copper', 17), ('alcoholic', 2), ('nonalcoholic', 1), ('beverages', 3), ('machinery', 13), ('lengthy', 12), ('anything', 13), ('consulate', 33), ('jeddah', 17), ('merciful', 1), ('improvement', 14), ('banking', 54), ('availability', 4), ('external', 42), ('risks', 19), ('spokesperson', 6), ('convoys', 13), ('operational', 16), ('wrapping', 8), ('looks', 25), ('feeds', 2), ('connecticut', 9), ('disgruntled', 3), ('beer', 6), ('spree', 5), ('hartford', 2), ('distributors', 5), ('warehouse', 9), ('welcoming', 3), ('equip', 2), ('extension', 28), ('component', 3), ('repatriating', 2), ('havens', 11), ('restrict', 15), ('overcome', 21), ('interruptions', 3), ('renovating', 2), ('relying', 5), ('jaap', 14), ('hoop', 17), ('scheffer', 19), ('sense', 17), ('clamp', 4), ('digging', 8), ('holes', 2), ('solo', 2), ('meter', 48), ('safeguard', 9), ('islamiyah', 9), ('teenager', 25), ('seventeen', 4), ('tufail', 1), ('matoo', 1), ('baku', 9), ("t'bilisi", 2), ('ceyhan', 3), ('erzerum', 1), ('kars', 1), ('akhalkalaki', 2), ('capitalize', 2), ('mattoo', 1), ('teargas', 6), ('shell', 43), ('bag', 13), ('47', 23), ('entirety', 2), ('christians', 53), ('celebrating', 17), ('apprehension', 1), ('collect', 9), ('simplified', 1), ('enforcement', 29), ('cracked', 5), ('petty', 4), ('anxious', 5), ('feels', 8), ('invincible', 1), ('volunteer', 7), ('neediest', 1), ('fellow', 29), ('queen', 13), ('elizabeth', 7), ('pride', 9), ('gratitude', 2), ('highlighted', 8), ('diversity', 4), ('tolerance', 11), ('ordinary', 9), ('orthodox', 30), ('patriarch', 8), ('alexy', 2), ('deeds', 3), ('pool', 13), ('joy', 7), ('cathedral', 7), ('siberian', 4), ('yakutsk', 2), ('eroded', 5), ('surplus', 18), ('borrowing', 9), ('benedict', 71), ('feast', 4), ('epiphany', 2), ('kings', 4), ('wise', 5), ('jesus', 25), ('isi', 1), ('rafael', 18), ('nadal', 5), ('slam', 9), ('melbourne', 21), ('pinning', 1), ('regulation', 3), ('attract', 16), ('domestically', 7), ('joins', 8), ('andre', 9), ('agassi', 14), ('russians', 10), ('maria', 11), ('sharapova', 8), ('fitness', 3), ('seed', 31), ('federer', 14), ('ankle', 5), ('method', 7), ('infiltration', 4), ('nicholas', 13), ('burns', 19), ('infiltrating', 3), ('igniting', 1), ('discarding', 1), ('madagascar', 10), ('liberalization', 5), ('prepared', 47), ('concessions', 12), ('expense', 12), ('compromises', 1), ('subsidies', 39), ('iacovou', 2), ('ambassadors', 15), ('text', 11), ('gul', 18), ('abandon', 36), ('imposes', 4), ('tries', 4), ('normalize', 4), ('wounds', 23), ('mainstay', 8), ('employing', 6), ('sadrist', 2), ('vocal', 8), ('transferring', 10), ('responsibilities', 10), ('basketball', 17), ('yao', 10), ('ming', 3), ('sore', 1), ('toe', 3), ('houston', 19), ('nba', 10), ('boomed', 2), ('266', 2), ('miss', 7), ('getter', 1), ('averaging', 6), ('rebounds', 3), ('shots', 26), ('114', 9), ('expulsion', 11), ('requirements', 19), ('agoa', 2), ('termination', 5), ('apartments', 7), ('pakistanis', 32), ('belgian', 16), ('financing', 16), ('blow', 38), ('bangkok', 19), ('dissolve', 9), ('crippling', 8), ('abhisit', 3), ('vejjajiva', 1), ('thaksin', 30), ('predecessor', 18), ('somchai', 1), ('wongsawat', 1), ('dispersing', 3), ('shirted', 1), ('wracked', 5), ('deforestation', 3), ('erosion', 4), ('aggravated', 4), ('firewood', 2), ('weah', 23), ('sainworla', 1), ('affiliate', 4), ('veritas', 1), ('monrovia', 5), ('talked', 8), ('butty', 1), ('hubble', 13), ('telescope', 10), ('fix', 11), ('camera', 7), ('photo', 11), ('galaxies', 8), ('ring', 28), ('beaming', 1), ('repair', 20), ('ravalomanana', 7), ('aggressively', 8), ('images', 40), ('revolutionized', 1), ('universe', 4), ('aqsa', 41), ('martyrs', 35), ('persuaded', 2), ('30th', 12), ('hakim', 11), ('dealt', 8), ('blows', 7), ('infidels', 3), ('endorses', 3), ('authenticated', 4), ('richardson', 5), ('endorse', 5), ('portland', 3), ('oregon', 7), ('hispanic', 5), ('hispanics', 4), ('tended', 1), ('delegates', 42), ('selected', 24), ('caucuses', 4), ('wary', 5), ('entering', 35), ('uncertain', 13), ('revelers', 4), ('pack', 4), ('celebrates', 10), ('carnival', 10), ('lent', 5), ('repentance', 1), ('parades', 10), ('featuring', 8), ('sensual', 1), ('dance', 13), ('samba', 4), ('culminates', 1), ('sambadrome', 3), ('brazilians', 6), ('outlandish', 2), ('costumes', 4), ('masks', 5), ('drinking', 29), ('celebrations', 38), ('squeeze', 3), ('indulgence', 1), ('fasting', 8), ('hanging', 14), ('beit', 12), ('considers', 35), ('1853', 1), ('collaborating', 3), ('row', 14), ('excerpts', 6), ('aired', 36), ('filmed', 5), ('style', 27), ('signaled', 6), ('anytime', 2), ('faltering', 6), ('positioned', 6), ('fed', 27), ('steadily', 17), ('served', 90), ('penal', 2), ('1864', 1), ('balance', 27), ('hikes', 13), ('slash', 7), ('siege', 15), ('beslan', 13), ('ingushetia', 5), ('seizing', 11), ('ordeal', 1), ('chaotic', 7), ('330', 10), ('teleconference', 1), ('noumea', 1), ('caledonia', 5), ('bagram', 14), ('spoken', 7), ('showdown', 4), ('tactic', 5), ('filibuster', 2), ('frist', 6), ('priscilla', 2), ('owen', 3), ('filibusters', 4), ('changing', 12), ('drastically', 5), ('commits', 3), ('2014', 10), ('2019', 2), ('accordance', 12), ('boycotting', 8), ('asad', 5), ('hashimi', 3), ('arranging', 6), ('jetliners', 4), ('airbus', 17), ('surprised', 12), ('regain', 11), ('ills', 6), ('mankind', 2), ('prevailed', 2), ('1055', 1), ('1002', 1), ('somewhat', 11), ('fleets', 5), ('cope', 17), ('khursheed', 6), ('hatred', 12), ('wafted', 1), ('heaven', 2), ('righteous', 2), ('vengeance', 3), ('persecutors', 1), ('bikindi', 3), ('youth', 26), ('sports', 41), ('tanzania', 29), ('composed', 3), ('encouraged', 31), ('hutus', 7), ('tutsis', 16), ('consulted', 2), ('juvenal', 2), ('habyarimana', 2), ('lyrics', 3), ('privately', 19), ('tutsi', 9), ('stationing', 1), ('tariq', 7), ('youssef', 9), ('baathist', 3), ('sympathizers', 7), ('entreated', 5), ('jupiter', 9), ('unceasing', 1), ('warfare', 12), ('indissoluble', 1), ('proof', 18), ('soured', 3), ('defusing', 3), ('attorney', 66), ('spies', 13), ('vicente', 32), ('rangel', 16), ('attaché', 2), ('correa', 19), ('mentioned', 21), ('filmmaker', 11), ('documentary', 10), ('outrage', 17), ('decreed', 2), ('henceforth', 2), ('habitations', 1), ('edelist', 1), ('incorrect', 6), ('archival', 1), ('footage', 19), ('illustrate', 2), ('copy', 5), ('film', 63), ('transcript', 1), ('eliezer', 2), ('ueberroth', 2), ('baseball', 24), ('permission', 34), ('treasury', 46), ('inaugural', 5), ('classic', 10), ('hence', 3), ('arises', 2), ('abound', 1), ('singly', 2), ('discern', 1), ('puerto', 13), ('rico', 6), ('panama', 40), ('transactions', 10), ('zaraqawi', 1), ('yasin', 2), ('malik', 8), ('adviser', 18), ('sanjaya', 1), ('baru', 1), ('tv', 39), ('channel', 36), ('geo', 4), ('convened', 8), ('invitation', 15), ('warlords', 25), ('paving', 6), ('locked', 16), ('feel', 8), ('unsafe', 5), ('unions', 34), ('sirnak', 4), ('averted', 3), ('stoppages', 1), ('attendants', 2), ('alitalia', 1), ('walkout', 6), ('telecommunications', 32), ('slalom', 12), ('bormio', 1), ('orchid', 1), ('growers', 4), ('grab', 9), ('bigger', 9), ('unique', 14), ('varieties', 2), ('wagner', 2), ('farc', 64), ('alvaro', 33), ('uribe', 46), ('valle', 2), ('cauca', 1), ('swap', 10), ('rightist', 16), ('paramilitaries', 18), ('rewrite', 4), ('cedatos', 1), ('gallup', 4), ('78', 22), ('inefficiency', 1), ('centralize', 1), ('stripping', 2), ('unpopular', 9), ('redefine', 2), ('shelled', 5), ('reaching', 34), ('unveiled', 29), ('epidemic', 16), ('anticipates', 3), ('gatherings', 7), ('stockpiles', 6), ('viral', 6), ('tamiflu', 8), ('beachfront', 4), ('pkk', 65), ('minibus', 16), ('jenin', 13), ('marti', 3), ('viewers', 11), ('granma', 10), ('article', 28), ('wpmf', 1), ('dishes', 4), ('nepali', 7), ('ilam', 2), ('680', 1), ('persuading', 7), ('insult', 7), ('outbursts', 1), ('revolt', 15), ('pushing', 18), ('intense', 33), ('loi', 1), ('sam', 14), ('rashakai', 1), ('tang', 2), ('khata', 1), ('bajaur', 10), ('rehman', 5), ('offensives', 8), ('reserved', 6), ('militancy', 8), ('discouraging', 5), ('undertaking', 2), ('beating', 34), ('assaulting', 7), ('uniformed', 5), ('punching', 4), ('drunkenness', 2), ('pictures', 35), ('manhandling', 1), ('credentials', 11), ('handcuffs', 4), ('beaten', 19), ('deserting', 1), ('looting', 9), ('arrives', 11), ('respects', 13), ('universities', 12), ('offend', 3), ('ekmeleddin', 4), ('ihsanoglu', 4), ('finnish', 7), ('kaliningrad', 2), ('interfax', 19), ('denying', 31), ('matti', 1), ('vanhanen', 1), ('reward', 22), ('paraded', 1), ('harcourt', 10), ('emancipation', 11), ('delta', 62), ('mend', 7), ('refuted', 2), ('hoshyar', 9), ('zebari', 14), ('maysan', 1), ('margaret', 17), ('beckett', 6), ('muthana', 1), ('mean', 21), ('redeployed', 1), ('covert', 9), ('reid', 22), ('lewis', 8), ('libby', 16), ('probing', 12), ('leak', 6), ('karl', 7), ('rove', 4), ('economist', 16), ('konan', 3), ('banny', 11), ('mediators', 21), ('dakar', 9), ('senegal', 42), ('abijan', 1), ('andaman', 6), ('archipelago', 15), ('teenagers', 13), ('wandering', 1), ('nicobari', 1), ('tribespeople', 2), ('hill', 34), ('flooded', 29), ('emaciated', 1), ('lived', 41), ('coconuts', 6), ('esmatullah', 1), ('alizai', 1), ('mian', 3), ('neshin', 1), ('tactical', 3), ('recapture', 4), ('chora', 1), ('casualty', 13), ('deutsche', 2), ('welle', 2), ('baghlan', 6), ('explosive', 35), ('musayyib', 2), ('spiraling', 4), ('topics', 16), ('absolutely', 4), ('unproven', 1), ('alonso', 10), ('complexes', 1), ('desalinization', 1), ('toulouse', 1), ('pedro', 6), ('esquisabel', 1), ('urtuzaga', 1), ('mushir', 1), ('masri', 16), ('steinmeier', 10), ('atrocious', 2), ('senseless', 1), ('successor', 31), ('honduran', 9), ('fatally', 9), ('tegucigalpa', 3), ('timothy', 7), ('markey', 3), ('leg', 28), ('pronounced', 2), ('fatal', 23), ('robbery', 10), ('dea', 1), ('scott', 33), ('mcclellan', 25), ('idol', 8), ('hopefuls', 5), ('implicating', 4), ('mourned', 2), ('legislator', 11), ('publisher', 7), ('gibran', 1), ('tueni', 5), ('coincide', 13), ('axe', 8), ('alaina', 1), ('sang', 8), ('tepid', 1), ('rendition', 2), ('dixie', 1), ('chicks', 1), ('nice', 5), ('nick', 6), ('charisma', 1), ('cowell', 6), ('wto', 26), ('bids', 9), ('goody', 1), ('payable', 1), ('kearny', 2), ('accessories', 3), ('cosmetic', 3), ('92', 11), ('takeover', 17), ('1830', 5), ('prospered', 6), ('technologically', 3), ('flemings', 1), ('walloons', 1), ('turkmenistan', 12), ('intensive', 11), ('irrigated', 2), ('oases', 1), ('tabaldo', 1), ('leslie', 6), ('nina', 2), ('simone', 2), ('feeling', 11), ('cotton', 15), ('consumed', 3), ('employ', 8), ('workforce', 12), ('tribally', 1), ('cautious', 6), ('sustain', 15), ('endemic', 6), ('educational', 8), ('ashgabat', 1), ('statistics', 15), ('margins', 2), ('antonella', 3), ('barba', 2), ('racy', 1), ('sensation', 1), ('particular', 21), ('berdimuhamedow', 2), ('unified', 15), ('dual', 11), ('redenomination', 1), ('manat', 1), ('gasoline', 66), ('caspian', 6), ('bureaucratic', 5), ('impede', 4), ('hungary', 24), ('d', 31), ('1000', 9), ('bulwark', 1), ('polyglot', 2), ('1956', 7), ('warsaw', 19), ('janos', 1), ('kadar', 1), ('liberalizing', 3), ('introducing', 4), ('goulash', 1), ('confinement', 8), ('rotating', 14), ('frog', 2), ('marsh', 1), ('beasts', 9), ('physician', 10), ('heal', 4), ('pretend', 1), ('prescribe', 4), ('lame', 2), ('gait', 1), ('wrinkled', 2), ('swallow', 8), ('reared', 3), ('snake', 2), ('chink', 1), ('eat', 18), ('injunction', 8), ('pitcher', 8), ('filberts', 2), ('grasped', 2), ('unwilling', 3), ('bitterly', 4), ('lamented', 4), ('disappointment', 6), ('bystander', 6), ('quantity', 6), ('readily', 2), ('unspecified', 12), ('federally', 3), ('administered', 18), ('fatas', 1), ('livelihoods', 6), ('governed', 6), ('pashtun', 3), ('elders', 22), ('ravine', 5), ('malakand', 2), ('poorly', 15), ('disregard', 3), ('dmitri', 16), ('medvedev', 31), ('ratification', 12), ('prague', 7), ('submitted', 29), ('synchronize', 1), ('arsenals', 5), ('milestone', 8), ('nadhem', 1), ('skirmish', 4), ('cricket', 32), ('337', 5), ('stumps', 5), ('nld', 13), ('landslide', 24), ('matthew', 5), ('hayden', 11), ('28th', 2), ('124', 3), ('sourav', 3), ('ganguly', 6), ('surpassing', 3), ('countrymen', 3), ('bradman', 1), ('ricky', 1), ('ponting', 1), ('bowlers', 6), ('damper', 2), ('anil', 5), ('kumble', 6), ('84', 19), ('zaheer', 1), ('ejected', 4), ('grounds', 25), ('tips', 6), ('interviews', 19), ('entice', 2), ('prisons', 46), ('nabih', 5), ('berri', 5), ('odds', 13), ('amr', 8), ('simple', 15), ('outlining', 8), ('swift', 9), ('hanged', 7), ('juma', 3), ('himat', 2), ('describing', 10), ('mutilated', 5), ('trees', 22), ('sayed', 6), ('agha', 7), ('saqeb', 1), ('ahmadi', 7), ('section', 31), ('cordons', 1), ('rock', 42), ('segment', 3), ('fencing', 6), ('barbed', 1), ('tunceli', 6), ('outlawed', 24), ('roadblock', 6), ('unilateral', 17), ('ignoring', 8), ('complaining', 6), ('pains', 5), ('lagging', 4), ('050', 1), ('calories', 1), ('requirement', 4), ('cheerful', 2), ('experiencing', 11), ('discomfort', 3), ('exhaustion', 4), ('spends', 4), ('ghanaian', 4), ('accra', 6), ('entirely', 13), ('argue', 16), ('gradual', 8), ('consolidation', 5), ('conceived', 4), ('kwame', 2), ('nkrumah', 2), ('fresh', 41), ('pacifism', 1), ('rating', 21), ('slashed', 10), ('ratings', 11), ('bonds', 12), ('speculative', 1), ('athens', 15), ('downgraded', 7), ('sovereign', 22), ('notches', 1), ('debts', 8), ('lenders', 9), ('downgrades', 1), ('stocks', 24), ('quan', 1), ('favorable', 11), ('investigative', 12), ('communists', 6), ('caller', 1), ('coverage', 24), ('occupy', 4), ('contained', 26), ('lynndie', 5), ('arraignment', 1), ('georgy', 1), ('gongadze', 2), ('maltreatment', 2), ('maltreat', 1), ('mistrial', 2), ('presiding', 9), ('mistreating', 4), ('tunisia', 10), ('bias', 5), ('dominique', 17), ('villepin', 19), ('reactors', 23), ('escalate', 5), ('decapitated', 4), ('dennis', 6), ('hastert', 1), ('inspiring', 3), ('contest', 13), ('captain', 31), ('bowman', 2), ('shifting', 8), ('entrenched', 5), ('pockets', 7), ('centraql', 1), ('bodyguard', 17), ('conversations', 10), ('bahraini', 2), ('likelihood', 4), ('emirate', 3), ('mani', 1), ('shankar', 1), ('aiyar', 2), ('amanullah', 4), ('jadoon', 1), ('pressured', 11), ('57th', 1), ('588', 1), ('differs', 4), ('releases', 9), ('khin', 17), ('nyunt', 11), ('accusations', 53), ('dissidents', 31), ('threatens', 22), ('defenders', 3), ('kravchenko', 1), ('drc', 31), ('intimidation', 8), ('afraid', 8), ('legitimate', 16), ('coastline', 10), ('sulawesi', 4), ('generated', 15), ('tomini', 1), ('gorontalo', 1), ('unfounded', 7), ('crushing', 7), ('barreled', 1), ('pangandaran', 2), ('668', 1), ('volunteered', 3), ('skip', 3), ('lady', 17), ('laura', 8), ('reunite', 8), ('skipped', 2), ('meal', 5), ('rainfall', 11), ('rainy', 5), ('depletion', 5), ('livestock', 25), ('herds', 2), ('kenyans', 17), ('nominate', 7), ('closest', 9), ('karen', 21), ('hughes', 8), ('reputation', 9), ('requires', 35), ('advisors', 13), ('tutwiler', 1), ('greeted', 13), ('exploited', 3), ('rangers', 1), ('villagers', 55), ('5000', 2), ('wildfires', 6), ('scorched', 2), ('canary', 11), ('fires', 32), ('tenerife', 4), ('gran', 6), ('canaria', 3), ('stabilized', 5), ('moderated', 3), ('inspected', 5), ('forests', 5), ('incinerated', 1), ('blazes', 7), ('coastal', 45), ('resorts', 11), ('caregivers', 1), ('environmentalists', 5), ('arsonists', 3), ('wildfire', 4), ('confessed', 15), ('vaclav', 3), ('havel', 7), ('autocracy', 4), ('authors', 3), ('mary', 9), ('robinson', 2), ('retired', 48), ('archbishop', 20), ('desmond', 7), ('tutu', 7), ('soros', 3), ('mlada', 1), ('fronta', 1), ('dnes', 1), ('subsided', 4), ('1997', 52), ('chart', 4), ('speculation', 34), ('nasal', 2), ('tube', 7), ('intake', 1), ('throat', 8), ('armchair', 1), ('works', 43), ('aides', 28), ('overlooking', 3), ('bless', 2), ('cheers', 3), ('applause', 4), ('microphone', 4), ('discharged', 8), ('tracheotomy', 1), ('bethelehem', 1), ('manger', 7), ('nativity', 5), ('infiltrate', 3), ('mardan', 1), ('registry', 5), ('boris', 13), ('tadic', 13), ('considerations', 1), ('albanian', 20), ('backs', 8), ('supervised', 4), ('hinted', 2), ('supervision', 8), ('shrine', 33), ('cancel', 18), ('timely', 2), ('yasukuni', 4), ('audience', 20), ('atlanta', 19), ('referring', 21), ('register', 20), ('parlimentary', 1), ('appoint', 20), ('canceling', 2), ('liners', 4), ('flare', 7), ('elaborate', 24), ('why', 47), ('democrat', 23), ('duluiyah', 1), ('triangle', 6), ('extinguished', 1), ('saboteurs', 2), ('guardsman', 1), ('gerry', 3), ('kate', 4), ('mccann', 4), ('blessed', 2), ('photograph', 12), ('siblings', 7), ('devout', 2), ('emotions', 2), ('publicize', 2), ('disappearance', 9), ('outcry', 6), ('celebrities', 4), ('duties', 33), ('hrw', 6), ('condemns', 15), ('lists', 14), ('absurd', 3), ('contends', 9), ('revised', 17), ('bench', 3), ('declaring', 10), ('sawers', 1), ('ditch', 2), ('javad', 3), ('vaeedi', 2), ('consensus', 16), ('unexpectedly', 5), ('strongest', 14), ('jobless', 9), ('weak', 29), ('787', 2), ('stimulus', 25), ('cultural', 22), ('secessionist', 9), ('haqqani', 3), ('facilitator', 2), ('paktiya', 1), ('honorary', 3), ('lien', 1), ('chan', 8), ('mainland', 43), ('guiding', 2), ('greenspan', 21), ('retire', 8), ('regarded', 4), ('speculate', 5), ('bullhorn', 1), ('occupants', 4), ('ak', 7), ('academic', 8), ('glenn', 2), ('hubbard', 1), ('feldstein', 1), ('danilo', 6), ('anderson', 20), ('charred', 5), ('positively', 3), ('prosecuting', 4), ('ibero', 4), ('119', 10), ('jump', 15), ('seem', 7), ('rises', 6), ('assessed', 5), ('2020', 12), ('sufficient', 16), ('egyptians', 16), ('resulting', 22), ('regulators', 21), ('fined', 13), ('357', 1), ('antitrust', 2), ('neelie', 1), ('kroes', 1), ('double', 60), ('633', 2), ('technical', 30), ('smoothly', 4), ('unjustified', 5), ('icy', 12), ('shutting', 7), ('snarling', 1), ('domodedovo', 2), ('sheremetyevo', 1), ('snapped', 4), ('slicked', 1), ('encased', 1), ('ria', 2), ('novosti', 3), ('taxis', 1), ('charging', 14), ('sex', 35), ('42', 28), ('guess', 2), ('physical', 24), ('appearance', 34), ('karachi', 51), ('condom', 3), ('stolen', 18), ('espionage', 12), ('goodwill', 13), ('identities', 14), ('bears', 15), ('ken', 4), ('salazar', 3), ('applying', 8), ('endangered', 13), ('species', 24), ('originate', 1), ('habitat', 6), ('application', 9), ('farther', 9), ('arctic', 15), ('mechanism', 7), ('millimeter', 2), ('bear', 15), ('touchstone', 1), ('melting', 9), ('atmosphere', 23), ('warms', 1), ('balochistan', 2), ('raziq', 1), ('bugti', 6), ('quetta', 24), ('wiped', 13), ('profits', 31), ('unfair', 17), ('graft', 9), ('homemade', 16), ('entrance', 18), ('faulty', 4), ('outdated', 6), ('146', 2), ('perceived', 10), ('focuses', 8), ('bribes', 15), ('ignores', 2), ('payments', 45), ('angola', 36), ('alasay', 1), ('patrols', 20), ('janica', 3), ('kostelic', 11), ('spindleruv', 1), ('mlyn', 1), ('kathrin', 1), ('zettel', 1), ('08', 8), ('marlies', 1), ('schild', 1), ('circuit', 9), ('steel', 18), ('mill', 7), ('kryvorizhstal', 2), ('consortium', 9), ('yulia', 12), ('tymoshenko', 31), ('privatizations', 5), ('reviewed', 7), ('fairly', 11), ('zvarych', 1), ('quit', 43), ('canal', 24), ('schemes', 4), ('wa', 9), ('shan', 22), ('mae', 1), ('spilling', 3), ('manufacture', 10), ('frontier', 23), ('javed', 5), ('cheema', 4), ('jirga', 4), ('aborted', 1), ('abduct', 1), ('tribesmen', 21), ('passage', 22), ('freeing', 10), ('sanctuaries', 3), ('swine', 25), ('woefully', 2), ('everyone', 21), ('susceptible', 1), ('h1n1', 8), ('wealthy', 19), ('biased', 12), ('affluence', 1), ('medicines', 8), ('gets', 10), ('providers', 2), ('inoculated', 1), ('atheist', 1), ('confirms', 11), ('429', 2), ('absentee', 3), ('provisional', 20), ('registered', 32), ('precinct', 4), ('gubernatorial', 3), ('christine', 3), ('gregoire', 1), ('barreling', 1), ('mayhem', 1), ('ruin', 3), ('stretches', 3), ('minnesota', 9), ('explicitly', 3), ('predicting', 6), ('locales', 2), ('discourages', 1), ('outages', 6), ('airline', 30), ('servicing', 10), ('reagan', 7), ('bloomberg', 19), ('indoors', 2), ('sidewalks', 1), ('guidance', 8), ('trades', 2), ('otherwise', 7), ('productivity', 13), ('hike', 19), ('minimum', 14), ('wage', 22), ('pensions', 4), ('steep', 13), ('utility', 6), ('users', 20), ('kilowatt', 1), ('heaviest', 8), ('conservation', 12), ('rasul', 1), ('aadham', 1), ('khurmatu', 3), ('laborers', 15), ('180', 15), ('ramadi', 42), ('anbar', 50), ('max', 5), ('baucus', 1), ('montana', 1), ('exemption', 1), ('basis', 37), ('rebuked', 1), ('harassment', 10), ('bastion', 3), ('encouraging', 16), ('infringement', 1), ('arizona', 25), ('concealing', 2), ('sanctioned', 4), ('cracks', 4), ('conscripted', 2), ('residency', 4), ('disclose', 10), ('slaughter', 15), ('bypass', 4), ('arteries', 3), ('recovers', 4), ('collective', 4), ('chaired', 8), ('pranab', 4), ('mukherjee', 6), ('tripled', 3), ('morris', 9), ('greatly', 12), ('deteriorated', 10), ('severely', 22), ('flowing', 14), ('starving', 1), ('nahr', 9), ('bared', 10), ('tripoli', 13), ('surveyed', 19), ('disapprove', 7), ('flags', 17), ('bombarded', 4), ('firefights', 3), ('sweet', 1), ('disruption', 7), ('minsk', 16), ('soaring', 28), ('skepticism', 4), ('hailing', 8), ('announcements', 3), ('sheikh', 47), ('shallah', 2), ('brotherhood', 42), ('intensifying', 4), ('rejection', 9), ('barring', 4), ('politics', 40), ('independents', 6), ('bargaining', 4), ('chip', 4), ('avi', 1), ('dichter', 1), ('mhawesh', 1), ('qadi', 1), ('gilad', 2), ('shalit', 3), ('commandos', 13), ('dressed', 19), ('randomly', 2), ('naseem', 1), ('haji', 1), ('akhtar', 10), ('salerno', 2), ('retaliated', 11), ('pairs', 4), ('freeskate', 1), ('highlights', 6), ('duel', 2), ('maxim', 2), ('marinin', 3), ('tatiana', 6), ('totmianina', 3), ('zhang', 10), ('hao', 1), ('thieves', 8), ('sarah', 7), ('grandmother', 5), ('grabs', 4), ('combines', 7), ('liv', 1), ('grete', 1), ('poiree', 3), ('pipe', 4), ('snowboarding', 2), ('kelly', 2), ('skating', 10), ('arson', 8), ('suburbs', 13), ('rioters', 16), ('racial', 9), ('treats', 5), ('kogelo', 1), ('hid', 12), ('electrocuted', 5), ('plata', 7), ('americas', 12), ('opens', 9), ('democratically', 14), ('honduras', 22), ('delegations', 6), ('encompass', 2), ('nestor', 8), ('kirchner', 12), ('pirates', 84), ('destroyer', 5), ('uss', 7), ('winston', 1), ('churchill', 1), ('piracy', 22), ('kitchen', 6), ('door', 16), ('maneuvering', 1), ('sixth', 39), ('compete', 30), ('sean', 24), ('maroney', 1), ('usa', 11), ('featured', 6), ('mohamad', 7), ('miracle', 3), ('resulted', 55), ('azahari', 8), ('husin', 7), ('tapes', 1), ('rented', 3), ("l'equipe", 3), ('676', 2), ('valentino', 1), ('rossi', 2), ('387', 2), ('formula', 7), ('distance', 15), ('kenenisa', 1), ('bekele', 1), ('compiling', 2), ('81', 19), ('wimbledon', 12), ('965', 1), ('bridge', 26), ('450', 12), ('rumor', 1), ('shoved', 2), ('tigris', 1), ('unverifiable', 4), ('wildly', 1), ('clad', 5), ('eleven', 9), ('lashkar', 23), ('jhangvi', 5), ('multan', 5), ('yemeni', 21), ('abyan', 5), ('secessionists', 1), ('placing', 14), ('asset', 8), ('zimbabwean', 10), ('throw', 9), ('lavish', 6), ('poisoning', 10), ('111', 5), ('concentrations', 1), ('zamfara', 1), ('epidemiologists', 1), ('pediatricians', 2), ('concentration', 6), ('kidneys', 1), ('reproductive', 1), ('herald', 22), ('85th', 2), ('chinhoyi', 1), ('harare', 10), ('tigers', 13), ('mullaittivu', 2), ('sprayed', 4), ('disinfectant', 1), ('poles', 5), ('erected', 3), ('correspondent', 24), ('1500', 3), ('sheltered', 3), ('rangin', 3), ('dadfar', 4), ('spanta', 5), ('masahiko', 1), ('komuri', 3), ('komura', 1), ('morgan', 5), ('tsvangirai', 3), ('sheltering', 5), ('yousuf', 19), ('raza', 16), ('gilani', 18), ('mehmood', 3), ('qureshi', 4), ('syed', 10), ('geelani', 1), ('hurriyat', 9), ('penitentiary', 6), ('upheaval', 2), ('quashed', 1), ('saga', 1), ('chaos', 29), ('massively', 1), ('airing', 6), ('duncan', 2), ('chairs', 11), ('publicist', 8), ('unvarnished', 1), ('truth', 14), ('denounces', 2), ('snuff', 1), ('integra', 3), ('mailing', 1), ('entitles', 1), ('nov', 9), ('exercised', 3), ('hammer', 3), ('operates', 13), ('hallwood', 2), ('cleveland', 16), ('merchant', 6), ('integration', 27), ('mere', 4), ('eliminated', 17), ('advantages', 3), ('downsized', 1), ('lagged', 3), ('overstated', 1), ('lowered', 8), ('sound', 30), ('fuad', 7), ('masoum', 2), ('prudent', 7), ('pegged', 4), ('euro', 54), ('modest', 10), ('timor', 12), ('leste', 3), ('westward', 5), ('supplemented', 1), ('piped', 2), ('convenes', 9), ('repository', 2), ('preserve', 12), ('resettled', 6), ('idps', 2), ('markedly', 3), ('procurement', 4), ('underlying', 5), ('duchy', 3), ('1809', 1), ('invasions', 5), ('albeit', 1), ('finns', 1), ('remarkable', 4), ('diversified', 8), ('initiation', 1), ('equality', 19), ('challenged', 20), ('fluctuations', 3), ('clipperton', 1), ('tuna', 5), ('raven', 3), ('swan', 13), ('desired', 3), ('plumage', 2), ('supposing', 3), ('color', 7), ('arose', 5), ('washing', 3), ('swam', 5), ('altars', 1), ('lakes', 6), ('pools', 2), ('cleansing', 4), ('feathers', 8), ('perished', 4), ('habit', 2), ('alter', 5), ('nettle', 2), ('hurts', 4), ('touched', 9), ('gently', 1), ('grasp', 1), ('boldly', 2), ('soft', 11), ('silk', 3), ('dust', 6), ('philosophy', 2), ('objectives', 9), ('mathematics', 1), ('kids', 11), ('financier', 3), ('bible', 3), ('pharaoh', 1), ('nile', 4), ('helium', 1), ('stationary', 3), ('pencils', 1), ('hiking', 2), ('trailing', 3), ('elevators', 1), ('escalators', 1), ('switches', 1), ('diapers', 1), ('keel', 1), ('balloon', 5), ('batteries', 5), ('recharge', 1), ('draining', 2), ('fifty', 7), ('ninety', 2), ('ethiopians', 12), ('malnutrition', 5), ('eliminate', 18), ('sanitation', 14), ('malnourished', 1), ('aerospace', 2), ('norad', 3), ('maneuvers', 10), ('elmendorf', 1), ('alaska', 8), ('khabarovsk', 7), ('drik', 1), ('divert', 5), ('extorting', 2), ('ransoms', 2), ('trainers', 2), ('peterson', 3), ('squads', 9), ('circulated', 6), ('rallied', 27), ('shouting', 11), ('denounce', 8), ('watched', 22), ('outcome', 14), ('gassing', 1), ('glyn', 1), ('davies', 2), ('frame', 4), ('drafted', 9), ('parliamentarian', 5), ('alaeddin', 1), ('boroujerdi', 4), ('kilograms', 19), ('substantially', 18), ('debating', 5), ('binding', 12), ('benchmarks', 6), ('securing', 10), ('desk', 4), ('override', 2), ('udi', 1), ('adam', 17), ('votel', 1), ('henry', 5), ('paulson', 6), ('lending', 10), ('reemergence', 1), ('distress', 5), ('creditors', 7), ('loans', 37), ('forgiven', 4), ('derailment', 2), ('mangled', 2), ('preston', 3), ('griffal', 2), ('joye', 2), ('luge', 4), ('doubles', 16), ('berth', 1), ('niccum', 3), ('quinn', 2), ('placid', 1), ('medalists', 2), ('grimmette', 1), ('martinin', 1), ('singles', 16), ('jonathan', 13), ('myles', 2), ('compatriot', 7), ('mazdzer', 1), ('161', 2), ('benshoofin', 1), ('samantha', 3), ('retrosi', 1), ('erin', 2), ('hamlin', 1), ('courtney', 2), ('zablocki', 1), ('lantos', 3), ('presidium', 1), ('yang', 2), ('hyong', 1), ('sop', 1), ('paek', 1), ('veligonda', 1), ('andhra', 8), ('resumption', 19), ('participated', 15), ('boycotted', 32), ('shattering', 4), ('lull', 9), ('beheaded', 11), ('derailed', 9), ('swollen', 4), ('shrank', 4), ('faster', 15), ('consumers', 33), ('pessimistic', 5), ('clues', 10), ('drives', 15), ('midwest', 8), ('flood', 33), ('inayatullah', 1), ('bhat', 2), ('acted', 21), ('stroll', 2), ('predominantly', 19), ('tal', 15), ('baath', 9), ('barham', 3), ('salih', 4), ('marshy', 1), ('brightly', 1), ('colored', 2), ('sodden', 1), ('valuable', 7), ('marlins', 2), ('josh', 4), ('avoided', 5), ('arbitration', 11), ('pitchers', 1), ('stints', 1), ('sep', 1), ('postseason', 1), ('championship', 17), ('02', 23), ('yankees', 4), ('sirens', 3), ('wailed', 1), ('remembrance', 6), ('motorists', 5), ('commemorations', 7), ('yad', 1), ('vashem', 1), ('auschwitz', 9), ('rescuers', 40), ('ropes', 4), ('wade', 6), ('carriages', 1), ('shofar', 2), ('ram', 6), ('sounded', 4), ('trek', 3), ('birkenau', 2), ('roma', 1), ('gypsies', 1), ('takes', 65), ('extermination', 5), ('promising', 19), ('malawai', 1), ('cassim', 2), ('chilumpha', 8), ('assassin', 3), ('malawi', 33), ('bingu', 6), ('mutharika', 18), ('mates', 3), ('feuding', 4), ('lessons', 4), ('luc', 1), ('chatel', 1), ('objecting', 1), ('google', 33), ('maps', 7), ('mislead', 3), ('impression', 4), ('update', 7), ('listing', 3), ('views', 27), ('530', 2), ('foreigner', 6), ('icrc', 6), ('spirits', 4), ('gauthier', 1), ('lefevre', 3), ('germans', 17), ('sister', 19), ('sibling', 1), ('geneina', 4), ('staffer', 3), ('communities', 71), ('captors', 10), ('hostility', 5), ('bashir', 26), ('mathieu', 4), ('kerekou', 9), ('olusegun', 14), ('obasanjo', 27), ('babies', 4), ('mouths', 1), ('sterility', 2), ('crimea', 7), ('unmarried', 1), ('palin', 1), ('teen', 11), ('spotlight', 2), ('padden', 2), ('teens', 3), ('conveyed', 6), ('management', 53), ('lapses', 2), ('kojo', 3), ('correct', 11), ('flaws', 5), ('cotecna', 1), ('lucrative', 7), ('contracts', 21), ('siyam', 1), ('upbeat', 3), ('assessment', 15), ('steve', 13), ('centanni', 3), ('olaf', 3), ('wiig', 3), ('solovtsov', 1), ('intermediate', 1), ('uncalled', 1), ('jaroslaw', 4), ('mirek', 1), ('topolanek', 1), ('syrians', 5), ('caption', 3), ('apology', 15), ('mix', 6), ('redskins', 1), ('taylor', 25), ('gunshot', 6), ('wound', 8), ('doubted', 1), ('cholera', 16), ('177', 2), ('sickened', 4), ('bissau', 6), ('instigate', 3), ('assassinate', 12), ('notched', 1), ('delray', 3), ('beach', 19), ('ramon', 6), ('delgado', 2), ('paraguay', 21), ('cruised', 1), ('xavier', 3), ('malisse', 4), ('justin', 5), ('gimelstob', 1), ('oliver', 2), ('marach', 1), ('guillermo', 4), ('todd', 1), ('widom', 1), ('decrease', 8), ('plaguing', 4), ('ministries', 10), ('chile', 60), ('tariffs', 16), ('panamanian', 11), ('intestinal', 15), ('raad', 2), ('juhyi', 1), ('cousin', 10), ('sultan', 8), ('hashim', 2), ('purports', 1), ('ansar', 11), ('sunna', 8), ('embraces', 1), ('dining', 8), ('dehydration', 7), ('darren', 2), ('boisvert', 1), ('distributing', 14), ('winterized', 1), ('plastic', 16), ('sheets', 4), ('essentially', 1), ('revived', 8), ('justices', 16), ('reconsider', 10), ('lawsuit', 23), ('tortured', 15), ('freely', 6), ('practicing', 4), ('imprisonment', 13), ('restoration', 11), ('humanely', 3), ('eritrean', 17), ('jemua', 3), ('ruphael', 1), ('amen', 1), ('benishangul', 1), ('gumuz', 1), ('latrines', 1), ('contaminate', 1), ('eritrea', 39), ('arnold', 8), ('schwarzenegger', 13), ('capping', 2), ('course', 29), ('treasure', 4), ('bold', 4), ('pollution', 23), ('chicago', 13), ('tribune', 1), ('print', 4), ('electronic', 28), ('protections', 4), ('random', 10), ('profiling', 5), ('dictatorship', 15), ('laith', 2), ('kuba', 1), ('rats', 1), ('badr', 4), ('brigade', 15), ('rockslide', 2), ('limestone', 1), ('cliff', 6), ('manshiyet', 2), ('nasr', 2), ('debris', 25), ('rockslides', 2), ('farmers', 49), ('cristina', 4), ('fernandez', 11), ('rebate', 4), ('soy', 3), ('sunflower', 1), ('seeds', 8), ('grains', 2), ('subsidize', 1), ('grain', 14), ('roadblocks', 7), ('constructed', 12), ('revise', 5), ('redistribute', 5), ('william', 36), ('brownfield', 9), ('trafficking', 52), ('accusation', 14), ('sana', 4), ('sidnaya', 2), ('extremism', 20), ('stems', 5), ('student', 41), ('rhine', 1), ('westphalia', 1), ('scanned', 2), ('profiled', 1), ('observatory', 6), ('magazine', 49), ('unauthenticated', 1), ('interrogated', 8), ('accompanying', 5), ('behead', 3), ('minas', 5), ('yousifi', 4), ('spear', 2), ('kilogram', 6), ('qaim', 7), ('karabila', 2), ('pharmaceutical', 11), ('merck', 10), ('experimental', 2), ('viruses', 8), ('cervical', 6), ('bavarian', 1), ('guenther', 1), ('beckstein', 1), ('gardasil', 2), ('papillovirus', 1), ('lesions', 1), ('cancerous', 2), ('transmitted', 15), ('hpvs', 2), ('immune', 3), ('glaxosmithkline', 3), ('eric', 5), ('kiraithe', 2), ('andrej', 1), ('hermlin', 1), ('gerd', 1), ('uwe', 1), ('fleur', 1), ('dissel', 1), ('gush', 1), ('katif', 1), ('hanif', 2), ('atmar', 3), ('hai', 2), ('muthmahien', 1), ('puppets', 2), ('183', 1), ('watchlist', 1), ('milliyet', 2), ('book', 34), ('updated', 6), ('fundamentalism', 6), ('separatism', 3), ('buys', 6), ('marburg', 19), ('244', 1), ('luanda', 10), ('stricken', 17), ('uige', 13), ('contagious', 6), ('incurable', 4), ('ebola', 16), ('bodily', 7), ('fluids', 9), ('hygienic', 3), ('restraints', 4), ('affiliated', 14), ('conceded', 4), ('confident', 15), ('expelling', 6), ('enemies', 13), ('willingly', 2), ('aggressors', 1), ('accusing', 53), ('mughani', 3), ('aiming', 7), ('minimize', 5), ('abdulatif', 1), ('sener', 1), ('crane', 4), ('guinean', 1), ('lansana', 3), ('conte', 5), ('humans', 65), ('everglades', 2), ('stretch', 12), ('wildlife', 17), ('glades', 1), ('shrink', 6), ('wading', 1), ('1930s', 8), ("'ll", 9), ('plenty', 3), ('alligators', 1), ('lane', 1), ('awesome', 1), ('sight', 10), ('anywhere', 10), ('kibo', 7), ('installing', 5), ('toilet', 8), ('fujimori', 33), ('ancestry', 3), ('petitioned', 7), ('extradite', 15), ('embezzlement', 9), ('sanctioning', 1), ('chronicles', 1), ('feminist', 1), ('1965', 7), ('sculptures', 3), ('behnam', 2), ('nateghi', 2), ('jim', 19), ('bertel', 9), ('communication', 20), ('clementina', 6), ('cantoni', 10), ('file', 20), ('widows', 4), ('tearful', 2), ('frode', 1), ('andresen', 3), ('sprint', 4), ('ruhpolding', 1), ('raphael', 2), ('rösch', 1), ('penalties', 6), ('greis', 1), ('standings', 9), ('298', 2), ('laps', 2), ('misses', 1), ('thai', 50), ('nahdlatul', 1), ('ulama', 1), ('hasyim', 1), ('muzadi', 3), ('shinawatra', 12), ('bhumibol', 3), ('adulyadej', 3), ('650', 10), ('der', 4), ('spiegel', 3), ('landmines', 5), ('formation', 24), ('abakar', 1), ('itno', 2), ('adre', 3), ('janjaweed', 15), ('mulino', 2), ('whoever', 5), ('something', 20), ('nephew', 10), ('liberty', 9), ('canyon', 4), ('ecosystem', 4), ('dirk', 1), ('kempthorne', 2), ('lever', 1), ('releasing', 17), ('glen', 1), ('regulates', 2), ('sediment', 3), ('beaches', 7), ('downstream', 4), ('tallest', 3), ('skyscraper', 1), ('experiments', 12), ('darien', 1), ('superintendent', 1), ('motives', 1), ('suggesting', 13), ('timed', 3), ('bulldozer', 1), ('discovering', 4), ('fog', 5), ('disagreements', 12), ('halutz', 8), ('occasional', 6), ('stance', 16), ('102', 7), ('166', 5), ('popularity', 15), ('rightwing', 2), ('congressional', 57), ('philippe', 4), ('douste', 5), ('blazy', 5), ('ingrid', 4), ('betancourt', 11), ('gabriel', 2), ('unthinkable', 2), ('usmani', 1), ('turban', 8), ('shabab', 23), ('daynunay', 1), ('publicity', 4), ('stunt', 2), ('overrun', 7), ('acquire', 5), ('konstantin', 4), ('kosachyov', 2), ('telegraph', 9), ('betweens', 1), ('teheran', 1), ('delivering', 14), ('falls', 8), ('bargain', 9), ('bisengimina', 3), ('pled', 1), ('gikoro', 1), ('arlete', 1), ('ramaroson', 1), ('confess', 3), ('quarantine', 9), ('aware', 20), ('organisation', 1), ('luzon', 4), ('logging', 5), ('assist', 24), ('dubai', 28), ('doncasters', 1), ('broader', 12), ('angered', 30), ('lima', 8), ('authorizing', 16), ('faxed', 2), ('santiago', 17), ('monteiro', 1), ('tasked', 7), ('ensuring', 3), ('contempt', 3), ('thein', 8), ('shein', 2), ('sentences', 37), ('permitted', 7), ('nearing', 11), ('enshrine', 1), ('bankruptcy', 14), ('jurisdiction', 14), ('yuganskneftgaz', 1), ('anyway', 3), ('suing', 3), ('firms', 34), ('gazprom', 43), ('rosneft', 12), ('weightlifting', 4), ('competitions', 2), ('owes', 8), ('rogers', 5), ('175', 3), ('148', 11), ('153', 2), ('placement', 3), ('perpetual', 6), ('preferred', 13), ('retractable', 1), ('holders', 5), ('convertible', 4), ('redeem', 1), ('conversion', 21), ('coupon', 1), ('liechtenstein', 16), ('industrialized', 34), ('enterprise', 8), ('easy', 8), ('incorporation', 2), ('induced', 6), ('nominal', 4), ('plutonium', 12), ('sport', 18), ('disrepute', 1), ('franc', 3), ('efta', 4), ('harmonize', 2), ('oecd', 12), ('grey', 4), ('model', 14), ('uruguay', 30), ('educated', 5), ('2000s', 3), ('readmitted', 2), ('brake', 1), ('vigorous', 4), ('decelerated', 1), ('managed', 31), ('expenditure', 1), ('float', 2), ('equals', 7), ('pounds', 4), ('rapid', 27), ('significantly', 26), ('utilities', 7), ('contributes', 9), ('secession', 19), ('depreciate', 2), ('considerably', 3), ('hoard', 1), ('restrain', 5), ('uncertainty', 10), ('iwf', 1), ('sanction', 2), ('coaches', 5), ('1291', 1), ('defensive', 6), ('cantons', 1), ('succeeding', 6), ('localities', 2), ('1499', 1), ('1848', 2), ('modified', 13), ('1874', 2), ('centralized', 4), ('neutrality', 9), ('honored', 10), ('crow', 14), ('jealous', 2), ('omen', 2), ('perching', 1), ('cawed', 1), ('loudly', 2), ('wondered', 2), ('foreboded', 1), ('companion', 9), ('journey', 12), ('caw', 2), ('cry', 1), ('tanner', 2), ('unpleasant', 1), ('smell', 2), ('tan', 3), ('yard', 11), ('accustomed', 3), ('inconvenience', 2), ('farmer', 25), ('implacable', 1), ('tow', 4), ('tail', 12), ('centre', 2), ('insured', 4), ('dissemble', 2), ('pond', 2), ('frogs', 7), ('pelt', 1), ('pleasure', 5), ('shantytowns', 2), ('softball', 4), ('inning', 1), ('math', 1), ('complicated', 5), ("'m", 9), ('customer', 6), ('clerk', 5), ('leaned', 2), ('priest', 19), ('blackboard', 3), ('dry', 21), ('chalk', 3), ('doctor', 27), ('cure', 4), ('looked', 12), ('improves', 4), ('pathogenic', 5), ('jaji', 1), ('kaduna', 5), ('disinfected', 4), ('ratifying', 1), ('armenians', 11), ('hanan', 1), ('raufi', 1), ('armor', 10), ('piercing', 1), ('applies', 4), ('anders', 5), ('fogh', 4), ('rasmussen', 5), ('sewage', 8), ('dmitry', 7), ('ophelia', 8), ('northward', 3), ('battering', 4), ('massachusetts', 17), ('nova', 4), ('scotia', 2), ('occupation', 34), ('bystanders', 11), ('sari', 1), ('pul', 5), ('robbed', 7), ('nicotine', 7), ('cigarettes', 10), ('inhale', 1), ('yielded', 5), ('smoker', 1), ('varied', 2), ('116', 2), ('brands', 5), ('addictive', 1), ('grows', 4), ('incursion', 19), ('recep', 31), ('tayyip', 31), ('erdogan', 47), ('patience', 5), ('mounting', 25), ('classify', 8), ('khushab', 3), ('kim', 78), ('sook', 2), ('cautiously', 3), ('chung', 12), ('dong', 7), ('concerning', 7), ('nobutaka', 4), ('machimura', 6), ('nimeiri', 4), ('lslamic', 1), ('79', 14), ('omdurman', 2), ('1985', 16), ('chapters', 2), ('sharia', 8), ('alienated', 2), ('barges', 2), ('gunship', 4), ('warri', 3), ('ijaw', 3), ('airstrip', 4), ('carolyn', 9), ('presutti', 6), ('accomplished', 7), ('resettlement', 6), ('hmong', 5), ('score', 12), ('handful', 11), ('midwestern', 11), ('wisconsin', 2), ('tham', 1), ('krabok', 1), ('enhanced', 6), ('screenings', 3), ('joyce', 3), ('mujuru', 3), ('msika', 1), ('filling', 5), ('vacancy', 3), ('muzenda', 1), ('chosen', 26), ('emmerson', 1), ('mnangawa', 1), ('ariane', 3), ('quentier', 1), ('derailing', 2), ('educating', 2), ('transatlantic', 2), ('trends', 7), ('marshall', 7), ('polled', 6), ('bryan', 4), ('whitman', 5), ('cyrus', 1), ('kar', 2), ('laywers', 1), ('prayerful', 1), ('attendance', 6), ('adrian', 3), ('fenty', 1), ('gift', 6), ('yunnan', 7), ('shook', 14), ('yanjin', 3), ('county', 24), ('zhaotong', 1), ('seismological', 2), ('hillsides', 2), ('guizhou', 2), ('plateau', 5), ('uri', 7), ('bu', 1), ('scrap', 10), ('repealing', 2), ('impasse', 10), ('pick', 22), ('regressing', 2), ('staffers', 16), ('compounds', 8), ('sub', 24), ('finals', 19), ('daniel', 23), ('bennett', 6), ('khairul', 1), ('amri', 1), ('agu', 1), ('casmir', 1), ('substitute', 2), ('mahyadi', 1), ('panggabean', 1), ('deflected', 2), ('shaky', 7), ('shaktoi', 2), ('friction', 4), ('sees', 14), ('audio', 16), ('recording', 19), ('hakimullah', 2), ('mehsud', 6), ('journalism', 9), ('yahoo', 7), ('relating', 9), ('osako', 1), ('subpoenas', 1), ('australians', 12), ('smuggle', 9), ('schapelle', 2), ('corby', 2), ('fitzgerald', 4), ('operative', 16), ('identity', 27), ('toughest', 2), ('rossendorf', 1), ('dresden', 4), ('reunification', 19), ('observe', 17), ('loading', 5), ('echoing', 3), ('yorker', 4), ('psychological', 5), ('momir', 1), ('savic', 2), ('visegrad', 2), ('dayton', 16), ('oilrig', 1), ('valerie', 3), ('plame', 4), ('flipped', 4), ('switch', 7), ('drenched', 4), ('recreated', 2), ('getulio', 1), ('vargas', 2), ('1953', 8), ('ironically', 1), ('rig', 12), ('unresolved', 10), ('kitgum', 1), ('mediator', 13), ('betty', 3), ('bigombe', 1), ('displacing', 5), ('knowingly', 3), ('lander', 5), ('garden', 15), ('drinkable', 1), ('owners', 20), ('marlon', 3), ('defillo', 1), ('shelves', 3), ('garmser', 4), ('importer', 2), ('readiness', 9), ('bracing', 9), ('tightening', 3), ('kamchatka', 2), ('remorse', 2), ('hanoi', 18), ('resident', 5), ('vaccinations', 9), ('nonbinding', 1), ('meaning', 11), ('opinions', 7), ('unfit', 2), ('soe', 3), ('tangerang', 4), ('83rd', 1), ('becoming', 43), ('190', 7), ('mutate', 17), ('transmissible', 4), ('zhari', 4), ('panjwayi', 5), ('credible', 13), ('expands', 4), ('wanting', 3), ('hashish', 1), ('fueling', 12), ('genital', 1), ('mutilation', 2), ('cruel', 10), ('unicef', 14), ('girls', 41), ('subjected', 8), ('circumcision', 2), ('genitalia', 1), ('hemorrhaging', 1), ('processing', 30), ('totally', 7), ('chechen', 33), ('chechens', 3), ('jails', 11), ('abusers', 2), ('surayud', 6), ('chulanont', 3), ('consultations', 14), ('unite', 8), ('fueled', 21), ('executives', 21), ('awaiting', 18), ('sighting', 6), ('crescent', 6), ('abstaining', 3), ('sunset', 3), ('depending', 7), ('scorching', 3), ('warmest', 4), ('koran', 16), ('invitations', 1), ('iftars', 1), ('meals', 6), ('conclude', 9), ('fitr', 12), ('nyala', 2), ('arming', 5), ('olli', 4), ('heinonen', 4), ('dubbed', 13), ('newspapers', 41), ('naturalized', 2), ('legally', 12), ('outpost', 14), ('reputed', 5), ('cache', 19), ('containing', 12), ('morphine', 1), ('enacted', 18), ('ineffectiveness', 3), ('marseille', 2), ('kibar', 1), ('housed', 12), ('filing', 10), ('ages', 3), ('delinquency', 1), ('baume', 1), ('immigrant', 16), ('rioted', 5), ('bayelsa', 5), ('bilfinger', 3), ('berger', 5), ('subsidiary', 13), ('extracted', 6), ('warplanes', 34), ('mau', 4), ('1950s', 5), ('colonial', 15), ('argues', 5), ('1952', 4), ('1959', 12), ('drill', 13), ('teikoku', 1), ('explore', 8), ('exclusive', 13), ('demarcation', 6), ('analyzing', 2), ('authorization', 8), ('undersea', 4), ('desecration', 6), ('ceased', 7), ('disclosed', 12), ('uproar', 2), ('newsweek', 7), ('interrogators', 21), ('flushed', 3), ('rattle', 4), ('retracted', 4), ('interceptor', 6), ('alexei', 5), ('kuznetsov', 2), ('characteristics', 1), ('fabricating', 2), ('massacring', 2), ('argument', 11), ('plundering', 1), ('resource', 15), ('reparations', 1), ('camilla', 5), ('onlookers', 4), ('glimpse', 5), ('duchess', 3), ('cornwall', 3), ('married', 24), ('dedicate', 1), ('tasnim', 3), ('aslam', 5), ('scrapped', 6), ('princess', 2), ('diana', 1), ('sarajevo', 11), ('dragomir', 2), ('relation', 4), ('corps', 27), ('radovan', 12), ('karadzic', 18), ('ratko', 11), ('mladic', 14), ('triple', 15), ('182', 1), ('amarah', 3), ('gedi', 19), ('airstrips', 2), ('yusef', 2), ('abdullahi', 13), ('parliamentarians', 6), ('relocate', 12), ('relocated', 3), ('manchester', 2), ('refinement', 1), ('originally', 37), ('initiate', 5), ('imprisoned', 32), ('royalist', 6), ('supposed', 15), ('turki', 5), ('fuheid', 1), ('muteiry', 2), ('fawaz', 2), ('nashmi', 1), ('timing', 11), ('khobar', 1), ('nineteen', 2), ('mara', 2), ('salvatrucha', 2), ('racketeering', 1), ('indictments', 9), ('suburban', 1), ('traditionally', 18), ('salvador', 37), ('await', 6), ('fists', 1), ('salute', 1), ('rachel', 1), ('chandler', 2), ('seychelles', 15), ('signal', 10), ('yacht', 2), ('lynn', 3), ('seas', 9), ('hijackings', 9), ('armada', 1), ('warships', 10), ('blindfolded', 8), ('ihab', 3), ('sherif', 4), ('mashruh', 1), ('krishna', 3), ('mahara', 1), ('dialysis', 1), ('coma', 13), ('anat', 1), ('dolev', 1), ('accumulated', 4), ('accumulation', 2), ('ningxia', 2), ('zhongwei', 1), ('sitaula', 1), ('prachanda', 7), ('130', 29), ('reappearing', 1), ('gotten', 5), ('graphic', 5), ('hassem', 1), ('knew', 11), ('exchanges', 9), ('barzan', 2), ('yelled', 3), ('ramsey', 1), ('clampdown', 5), ('kid', 8), ('wo', 4), ('headfirst', 1), ('snowbank', 1), ('bob', 18), ('ritchie', 3), ('sue', 6), ('false', 34), ('roughed', 1), ('michigan', 8), ('inviting', 4), ('insufficient', 10), ('existed', 8), ('headlines', 4), ('marrying', 1), ('divorcing', 2), ('actress', 21), ('pamela', 2), ('span', 5), ('provoking', 4), ('garissa', 1), ('disrupt', 34), ('bananas', 7), ('yes', 9), ('oranges', 1), ('exempts', 2), ('commodies', 1), ('197', 2), ('inconsistencies', 2), ('discrepancies', 1), ('dili', 1), ('martinho', 1), ('gusmao', 1), ('recount', 4), ('horta', 1), ('lu', 7), ('olo', 1), ('brawl', 5), ('blindness', 2), ('deregulation', 1), ('uncontrolled', 1), ('sticks', 6), ('musa', 13), ('sudi', 1), ('yalahow', 1), ('presumptive', 3), ('edgware', 1), ('hafs', 3), ('congratulating', 1), ('expert', 18), ('clarke', 11), ('babar', 3), ('presenting', 5), ('labeled', 8), ('combatant', 3), ('saqiz', 2), ('concides', 1), ('sped', 5), ('notifying', 1), ('tracking', 9), ('chiefs', 19), ('radioed', 2), ('confrontations', 5), ('protocol', 19), ('greenpeace', 4), ('displayed', 9), ('whales', 19), ('famed', 8), ('brandenburg', 1), ('whale', 6), ('moratorium', 12), ('mammals', 2), ('fisherman', 15), ('nets', 8), ('collisions', 1), ('biologist', 3), ('stefanie', 1), ('werner', 1), ('drown', 2), ('whaling', 15), ('anchorage', 1), ('teenage', 12), ('jackson', 29), ('molested', 2), ('bedroom', 1), ('arjun', 1), ('narsingh', 2), ('recalled', 20), ('reformists', 3), ('280', 4), ('disqualify', 2), ('loyalty', 4), ('accuser', 3), ('testified', 6), ('patient', 14), ('conservatives', 11), ('disqualifications', 1), ('defended', 44), ('monthly', 18), ('compelling', 1), ('license', 22), ('concession', 3), ('lara', 5), ('tolerate', 12), ('outlets', 13), ('rctv', 12), ('overly', 2), ('metal', 16), ('exposition', 2), ('katowice', 4), ('climb', 5), ('silesia', 1), ('homing', 2), ('pigeon', 3), ('entertainer', 6), ('drink', 14), ('wine', 4), ('jet', 36), ('liquor', 6), ('hanoun', 7), ('nearest', 4), ('mustafa', 12), ('productive', 7), ('carat', 4), ('gemstone', 1), ('auctioned', 1), ('blue', 7), ('bested', 1), ('hancock', 1), ('fetched', 2), ('moussaieff', 1), ('jewelers', 2), ('jeweler', 3), ('collects', 7), ('gemstones', 1), ('vivid', 1), ('boron', 1), ('stone', 16), ('crystal', 1), ('tareq', 3), ('hashemi', 8), ('benchmark', 8), ('dna', 14), ('revoke', 1), ('identifications', 1), ('sophisticated', 8), ('cyclone', 22), ('moriarty', 2), ('sidr', 4), ('bangladeshi', 22), ('plentiful', 3), ('anticipating', 1), ('bermuda', 10), ('horbach', 1), ('luxury', 20), ('reinsurance', 3), ('derives', 4), ('arable', 2), ('ubangi', 1), ('shari', 1), ('tumultuous', 1), ('misrule', 1), ('lasted', 18), ('ange', 1), ('felix', 4), ('patasse', 1), ('bozize', 2), ('tacit', 1), ('affirmed', 5), ('countryside', 11), ('lawlessness', 7), ('persist', 2), ('gilbert', 5), ('1892', 1), ('1915', 1), ('1941', 6), ('makin', 1), ('tarawa', 1), ('amphibious', 3), ('victories', 5), ('garrisons', 1), ('1943', 1), ('kiribati', 7), ('relinquished', 5), ('sparsely', 2), ('capitalist', 3), ('reversal', 5), ('reflected', 13), ('oversupply', 1), ('actually', 20), ('jalil', 2), ('jilani', 1), ('contagion', 1), ('indebted', 11), ('privatize', 4), ('competitiveness', 5), ('estate', 20), ('oversaw', 9), ('savings', 10), ('pressuring', 8), ('serpent', 12), ('asleep', 10), ('nook', 1), ('greedily', 2), ('turning', 29), ('mortal', 2), ('agony', 2), ('exclaimed', 7), ('unhappy', 4), ('windfall', 2), ('sacrifices', 5), ('intruded', 1), ('domain', 3), ('pasture', 4), ('desiring', 2), ('stranger', 1), ('punishing', 4), ('consented', 6), ('obtaining', 7), ('madagonia', 3), ('antipathy', 1), ('novakatka', 2), ('novakatkan', 2), ('apologise', 1), ('novakatkans', 2), ('ensued', 3), ('madagonians', 1), ('chagrined', 1), ('thereafter', 4), ('innings', 14), ('halted', 23), ('printed', 13), ('garnered', 3), ('rigged', 25), ('molestation', 8), ('jurors', 7), ('santa', 15), ('barbara', 4), ('fergana', 1), ('leaks', 5), ('malicious', 3), ('disgusting', 4), ('leaked', 7), ('nonexistent', 1), ('card', 18), ('uncontained', 1), ('disappointing', 9), ('charki', 2), ('overpowered', 3), ('heidar', 2), ('moslehi', 2), ('mahabad', 3), ('petra', 3), ('akbar', 17), ('rafsanjani', 14), ('expediency', 2), ('arch', 3), ('accepts', 6), ('observing', 14), ('cooling', 5), ('plunging', 5), ('mahinda', 5), ('rajapakse', 5), ('briefed', 6), ('burden', 22), ('christiane', 1), ('berthiaume', 1), ('litani', 3), ('404', 2), ('approaches', 8), ('navi', 1), ('pillay', 1), ('intimidate', 5), ('taint', 1), ('secede', 4), ('amputation', 1), ('prevail', 3), ('thanksgiving', 21), ('depart', 9), ('credited', 6), ('azimi', 5), ('belonged', 11), ('strains', 7), ('isaf', 16), ('inciting', 11), ('izzadeen', 3), ('heckling', 1), ('anjem', 1), ('choudary', 1), ('ghurabaa', 1), ('electrician', 2), ('aged', 6), ('bibles', 2), ('slit', 2), ('zirve', 1), ('malatya', 2), ('jumped', 21), ('printing', 7), ('wrestling', 1), ('trabzon', 1), ('blabague', 1), ('marboulaye', 1), ('demonstrating', 7), ('disruptive', 3), ('infiltrated', 7), ('demonstrator', 2), ('tram', 1), ('waged', 13), ('intensity', 2), ('seeks', 22), ('diluting', 1), ('appeasing', 2), ('taunt', 2), ('affront', 2), ('behavior', 20), ('punched', 2), ('shoulders', 2), ('stomach', 9), ('cremation', 2), ('uncertainties', 2), ('inhibit', 1), ('constructive', 9), ('stakeholder', 2), ('raises', 10), ('contracting', 9), ('ducks', 20), ('checking', 3), ('crater', 5), ('roving', 1), ('panorama', 1), ('rover', 6), ('formations', 2), ('layers', 3), ('exposed', 20), ('mystery', 2), ('reconnaissance', 12), ('wheeled', 2), ('descend', 1), ('pregnancy', 5), ('adolescent', 1), ('leta', 5), ('fincher', 5), ('yu', 4), ('woo', 5), ('ik', 1), ('obudu', 1), ('90th', 3), ('leveled', 3), ('passports', 9), ('hidden', 14), ('lagos', 26), ('stoppage', 8), ('adams', 4), ('oshiomole', 1), ('costly', 6), ('arabian', 12), ('riyadh', 17), ('fagih', 3), ('organizer', 5), ('learn', 16), ('manage', 10), ('healthier', 1), ('stabbing', 4), ('rahman', 13), ('aref', 8), ('overthrown', 4), ('91', 8), ('1958', 5), ('prop', 3), ('cardiovascular', 1), ('overweight', 2), ('obese', 2), ('smith', 29), ('deterrent', 3), ('yong', 4), ('isolate', 5), ('stifle', 3), ('destroy', 36), ('aggressor', 1), ('breaks', 15), ('jong', 27), ('il', 27), ('pending', 18), ('beef', 22), ('auto', 14), ('insurance', 47), ('estimating', 1), ('adjusters', 1), ('clearer', 2), ('becomes', 11), ('burn', 12), ('looters', 5), ('ravage', 1), ('herbert', 4), ('walker', 3), ('fundraising', 6), ('sarkozy', 44), ('moody', 3), ('underestimating', 1), ('mortgage', 13), ('defaults', 3), ('spy', 28), ('specified', 4), ('sensitivities', 3), ('dhafra', 1), ('emirates', 20), ('2s', 1), ('380th', 1), ('expeditionary', 1), ('enduring', 5), ('altitude', 6), ('unnamed', 28), ('oman', 18), ('haditha', 11), ('mizhir', 1), ('yousi', 1), ('bongo', 5), ('meantime', 15), ('staging', 13), ('install', 14), ('mukhtaran', 1), ('mai', 3), ('raped', 10), ('punishment', 13), ('marriage', 22), ('oppressed', 4), ('strides', 4), ('terminals', 3), ('bonny', 2), ('contractual', 1), ('lifts', 3), ('flagged', 9), ('biscaglia', 1), ('bangladeshis', 5), ('overboard', 2), ('overtook', 1), ('indoor', 8), ('ashia', 1), ('hansen', 8), ('contender', 8), ('birmingham', 2), ('breathing', 6), ('circulatory', 2), ('joaquin', 4), ('navarro', 5), ('valls', 4), ('scriptures', 1), ('cardinal', 12), ('camillo', 1), ('ruini', 1), ('bishops', 4), ('ryan', 8), ('crocker', 6), ('cardio', 1), ('fever', 23), ('urinary', 1), ('tract', 3), ('gravity', 4), ('underwent', 29), ('serviceman', 3), ('soh', 1), ('letting', 5), ('antonov', 1), ('pronk', 6), ('antonovs', 2), ('insist', 8), ('sok', 1), ('chol', 1), ('naypyidaw', 2), ('severed', 12), ('chun', 8), ('doo', 1), ('hwan', 2), ("ya'akov", 1), ('alperon', 2), ('careers', 2), ('physicians', 7), ('knocking', 6), ('lamp', 1), ('wayward', 1), ('macy', 3), ('1924', 4), ('balloons', 2), ('resemble', 2), ('characters', 3), ('cat', 11), ('hat', 2), ('knocked', 12), ('lamppost', 2), ('endorsing', 3), ('nicolas', 27), ('endorsement', 7), ('segolene', 4), ('centrist', 9), ('bayrou', 2), ('fulfill', 6), ('almanza', 1), ('patron', 2), ('carlos', 35), ('logarda', 1), ('leyva', 2), ('putumayo', 3), ('dror', 1), ('extortion', 3), ('compensate', 10), ('evacuation', 21), ('hardline', 13), ('nationalists', 14), ('encounter', 4), ('soku', 1), ('briton', 9), ('females', 1), ('prosecuted', 7), ('unreported', 5), ('bodman', 5), ('interviewers', 1), ('loaned', 4), ('refiners', 5), ('caverns', 2), ('surged', 23), ('heating', 13), ('converting', 6), ('yoram', 1), ('haham', 1), ('mobsters', 1), ('zahir', 5), ('scheme', 6), ('hapoalim', 2), ('customers', 15), ('mozambique', 16), ('vibrant', 1), ('impressed', 6), ('disasters', 28), ('susan', 4), ('schwab', 2), ('roemer', 1), ('bipartisan', 7), ('singled', 9), ('crises', 7), ('lender', 2), ('overhaul', 4), ('184', 3), ('finishing', 5), ('nicaragua', 40), ('kazem', 1), ('vaziri', 1), ('hamaneh', 1), ('investor', 16), ('artist', 16), ('lars', 2), ('vilks', 7), ('telling', 16), ('baghdadi', 5), ('momammad', 1), ('amy', 1), ('katz', 1), ('dog', 23), ('barbaric', 6), ('shocking', 5), ('8th', 6), ('14th', 11), ('narcotics', 10), ('devoted', 5), ('poppies', 4), ('reopen', 19), ('bountiful', 2), ('harvest', 10), ('pleased', 6), ('kidnap', 20), ('modifications', 3), ('conform', 5), ('fabian', 1), ('osuji', 2), ('paying', 28), ('adolphus', 1), ('wabara', 1), ('yediot', 6), ('ahronot', 3), ('slated', 10), ('fahd', 5), ('comprising', 2), ('boosted', 25), ('41', 37), ('mercantile', 8), ('answer', 18), ('unwelcome', 4), ('dismay', 3), ('273', 1), ('welsh', 1), ('murdering', 12), ('comrades', 4), ('bragg', 2), ('hasan', 5), ('101st', 5), ('airborne', 9), ('ambushing', 3), ('slept', 5), ('hlinethaya', 1), ('constant', 5), ('ridicule', 4), ('snap', 7), ('sentencing', 6), ('madonna', 11), ('orphans', 6), ('blantyre', 3), ('roses', 1), ('translated', 3), ('orphan', 1), ('lilongwe', 3), ('liz', 1), ('rosenberg', 1), ('plight', 5), ('farms', 42), ('bolivarian', 2), ('412', 1), ('athanase', 1), ('seromba', 1), ('lenient', 3), ('orchestrate', 2), ('parish', 3), ('demolish', 4), ('moderates', 3), ('h5', 7), ('jalgaon', 4), ('nandurbar', 1), ('navapur', 1), ('culled', 20), ('heihe', 1), ('blagoveshchensk', 1), ('65th', 3), ('liberated', 2), ('exterminate', 2), ('undesirable', 1), ('taipei', 23), ('declares', 8), ('opposing', 16), ('annexation', 3), ('fail', 23), ('guidelines', 17), ('renegade', 10), ('kfar', 3), ('darom', 2), ('committees', 11), ('consular', 12), ('pardoning', 1), ('elite', 8), ('seals', 4), ('sailor', 3), ('evaded', 2), ('scouring', 1), ('villaraigosa', 3), ('xvi', 17), ('studio', 11), ('angelus', 1), ('hearts', 4), ('loves', 1), ('blessing', 5), ('le', 9), ('figaro', 3), ('reasonable', 6), ('understand', 10), ('kevin', 20), ('costner', 6), ('breached', 5), ('fledgling', 5), ('actor', 20), ('mahee', 5), ('breach', 9), ('songwriter', 8), ('llc', 1), ('concerts', 6), ('marketing', 5), ('reneged', 5), ('qualifies', 1), ('takatoshi', 1), ('kato', 2), ('unsustainable', 3), ('therefore', 4), ('sustaining', 2), ('toufik', 1), ('hanouichi', 1), ('mohcine', 1), ('bouarfa', 1), ('jew', 1), ('acquitted', 14), ('meknes', 1), ('fez', 1), ('speaks', 6), ('trilateral', 1), ('gadahn', 1), ('fbi', 26), ('urgent', 12), ('demolition', 5), ('popularly', 6), ('ann', 5), ('tibaijuka', 1), ('evicted', 4), ('remainder', 8), ('semi', 27), ('zimbabweans', 1), ('charting', 1), ('scenarios', 4), ('qiyue', 3), ('mentality', 1), ('possibilities', 2), ('daioyu', 1), ('senkaku', 1), ('japanto', 1), ('advises', 4), ('ap', 11), ('kaka', 3), ('44th', 2), ('scoreless', 1), ('stuttgart', 4), ('abalo', 1), ('53rd', 1), ('soo', 2), ('ahn', 1), ('jung', 3), ('sputtered', 1), ('canadians', 15), ('wonderful', 2), ('professionalism', 2), ("'ve", 3), ('norman', 2), ('kember', 1), ('loney', 1), ('harmeet', 1), ('sooden', 1), ('swords', 2), ('righteousness', 2), ('sao', 15), ('tome', 5), ('damiao', 1), ('vaz', 4), ('almeida', 4), ('fradique', 1), ('menezes', 12), ('awarding', 1), ('doubtful', 1), ('credibility', 13), ('servants', 12), ('stockpile', 6), ('rebellious', 2), ('painful', 6), ('31st', 7), ('administrator', 16), ('ntawukulilyayo', 3), ('gisagara', 1), ('arusha', 2), ('prosecute', 13), ('braved', 1), ('consulates', 7), ('torrijos', 4), ('lage', 7), ('splitting', 4), ('shield', 18), ('rogue', 5), ('complemented', 1), ('mobile', 41), ('bernardo', 3), ('alvarez', 5), ('posada', 18), ('carriles', 19), ('cubana', 1), ('shannon', 8), ('demagogue', 2), ('walbrecher', 1), ('jr', 8), ('1st', 3), ('citadel', 3), ('principal', 8), ('fidelity', 2), ('succeeds', 5), ('l', 5), ('kane', 2), ('tunisians', 2), ('versus', 4), ('terminated', 2), ('sept', 3), ('hobbled', 3), ('instability', 32), ('overdependence', 3), ('ineligible', 1), ('amounts', 19), ('modernizing', 2), ('emphasis', 2), ('improvements', 14), ('impediment', 3), ('blueprint', 3), ('partnerships', 6), ('receipts', 8), ('aluminum', 5), ('competes', 2), ('feedstock', 1), ('petrochemical', 6), ('struggles', 6), ('sponsorship', 3), ('expatriate', 5), ('slower', 7), ('subsidy', 3), ('bailout', 8), ('marino', 14), ('relies', 15), ('ceramics', 1), ('fabrics', 1), ('furniture', 2), ('paints', 2), ('tiles', 2), ('comparable', 5), ('repatriate', 4), ('untaxed', 1), ('outflows', 2), ('harmonizing', 1), ('influenced', 8), ('fundamental', 7), ('necessities', 3), ('occupier', 1), ('exploit', 1), ('exhausted', 10), ('deeper', 7), ('secondary', 8), ('mined', 3), ('anticipation', 6), ('nauru', 5), ('cushion', 2), ('frozen', 18), ('wages', 9), ('overstaffed', 1), ('departments', 6), ('arkansas', 4), ('afloat', 1), ('varying', 2), ('colonies', 8), ('merged', 7), ('gambia', 7), ('senegambia', 1), ('envisaged', 1), ('casamance', 3), ('mfdc', 1), ('democracies', 10), ('abdoulaye', 2), ('reelected', 9), ('autocratic', 5), ('undeclared', 3), ('snared', 1), ('hare', 19), ('homewards', 1), ('horseback', 4), ('begged', 4), ('pretense', 3), ('purchasing', 6), ('horseman', 2), ('sorely', 3), ('physicist', 4), ('mathematician', 3), ('faculty', 2), ('catches', 6), ('bucket', 4), ('leap', 1), ('sink', 2), ('puts', 17), ('sit', 9), ('melissa', 2), ('relevant', 3), ('thus', 14), ('solved', 4), ('picking', 9), ('retreat', 10), ('ashcroft', 3), ('ridge', 6), ('counterterrorism', 7), ('cofer', 1), ('schoomaker', 2), ('ultimate', 6), ('triumph', 2), ('cleland', 2), ('insisting', 8), ('isfahan', 9), ('installs', 1), ('restarting', 3), ('intentions', 18), ('appointments', 9), ('unnecessary', 7), ('steer', 2), ('leumi', 2), ('discounted', 5), ('builder', 1), ('raping', 6), ('modeled', 4), ('stockpiling', 2), ('enticed', 2), ('tossed', 3), ('peacekeeper', 10), ('bribing', 1), ('slovenian', 2), ('janez', 1), ('jansa', 1), ('roused', 1), ('notably', 8), ('obeid', 2), ('zambia', 18), ('verify', 10), ('sutanto', 2), ('batu', 2), ('rocked', 13), ('booby', 1), ('retrieving', 1), ('masterminding', 2), ('69th', 2), ('tech', 24), ('hewlett', 1), ('packard', 1), ('carly', 1), ('fiorina', 2), ('helm', 3), ('directors', 13), ('hp', 2), ('profitable', 6), ('printer', 1), ('merge', 12), ('compaq', 1), ('wayman', 1), ('ceo', 3), ('resisting', 2), ('syarhei', 1), ('antonchyk', 1), ('vintsuk', 2), ('vyachorka', 2), ('unsanctioned', 1), ('milinkevich', 8), ('quashing', 2), ('genuine', 3), ('planche', 3), ('illegitimate', 5), ('principle', 14), ('wana', 3), ('insincere', 1), ('preconditions', 6), ('embarrassment', 3), ('admiration', 3), ('occasions', 12), ('keen', 2), ('harmony', 4), ('traditions', 6), ('harboring', 8), ('preceded', 2), ('guangdong', 19), ('migrant', 10), ('shanwei', 1), ('sichuan', 27), ('mireya', 2), ('moscoso', 2), ('coping', 3), ('guatemala', 33), ('belize', 8), ('farid', 6), ('soleiman', 1), ('upheld', 16), ('instance', 5), ('competitors', 9), ('servers', 2), ('angel', 6), ('mejia', 2), ('tolima', 1), ('typical', 5), ('victor', 13), ('antioquia', 1), ('billingslea', 4), ('commissioned', 5), ('financially', 6), ('feasible', 3), ('reaffirmed', 18), ('natwar', 8), ('silent', 7), ('simba', 1), ('banadir', 1), ('inflammatory', 3), ('landscape', 1), ('via', 16), ('zulima', 6), ('palacio', 6), ('shatt', 1), ('waterway', 2), ('yuganskneftegas', 5), ('bulba', 1), ('baikal', 2), ('payback', 1), ('specialists', 6), ('twins', 2), ('sulaymaniya', 3), ('quarantined', 8), ('obstacle', 8), ('creates', 4), ('slate', 8), ('maale', 5), ('adumim', 6), ('brad', 9), ('delp', 5), ('roadmap', 4), ('ultranationalist', 4), ('sacred', 8), ('sanctuary', 11), ('khogyani', 1), ('boston', 9), ('fiancee', 2), ('hampshire', 3), ('afterward', 9), ('espino', 1), ('rounded', 13), ('regrettable', 3), ('thapa', 1), ('protective', 5), ('toxicology', 3), ('examiner', 1), ('sealing', 3), ('bathroom', 7), ('charcoal', 4), ('grills', 1), ('castelgandolfo', 1), ('twenty', 11), ('monoxide', 2), ('semifinals', 13), ('confederations', 4), ('nuremberg', 1), ('adriano', 2), ('ronaldinho', 2), ('frankenstadion', 1), ('lukas', 1), ('podolski', 1), ('23rd', 6), ('ballack', 1), ('48th', 1), ('semifinal', 15), ('loser', 1), ('leipzig', 1), ('venture', 17), ('ukrgazenergo', 1), ('oversee', 15), ('naftogaz', 2), ('rosukrenergo', 1), ('monopoly', 15), ('sullivan', 2), ('cubic', 16), ('anabel', 5), ('medina', 11), ('garrigues', 8), ('canberra', 7), ('ekaterina', 2), ('bychkova', 1), ('shahar', 1), ('peer', 2), ('bounced', 3), ('aiko', 2), ('nakamura', 2), ('catalonia', 3), ('cassation', 2), ('julia', 4), ('scruff', 1), ('cho', 5), ('yoon', 2), ('jeong', 2), ('czink', 1), ('classical', 3), ('pianist', 2), ('berkofsky', 1), ('firebrand', 1), ('virtuosity', 1), ('irina', 2), ('robertson', 4), ('berkovsky', 1), ('fame', 14), ('charitable', 8), ('scot', 1), ('riddlesberger', 1), ('contents', 9), ('solicit', 1), ('captives', 10), ('leverage', 2), ('anatolia', 7), ('colonels', 1), ('belonging', 29), ('topple', 14), ('rooted', 9), ('launchers', 12), ('eighty', 3), ('solidify', 1), ('nationalistic', 1), ('tendencies', 1), ('populations', 14), ('afl', 1), ('cio', 1), ('unionists', 1), ('calmed', 2), ('encourages', 7), ('priests', 17), ('embrace', 5), ('digital', 7), ('forms', 17), ('distances', 5), ('clergy', 5), ('notable', 2), ('vocation', 1), ('languages', 3), ('youtube', 6), ('facebook', 14), ('pope2you', 1), ('cricketers', 2), ('fixing', 5), ('salman', 8), ('butt', 5), ('tabloid', 3), ('intentionally', 2), ('bowl', 6), ('balls', 6), ('icc', 3), ('resistant', 11), ('pascal', 4), ('ringwald', 1), ('correctly', 5), ('derived', 3), ('artemisia', 1), ('ineffective', 3), ('artemisian', 1), ('medication', 12), ('mefloquine', 1), ('borne', 6), ('firat', 1), ('hakurk', 1), ('strongholds', 17), ('warmongering', 1), ('waheed', 5), ('arshad', 5), ('grass', 9), ('oklahoma', 11), ('plymouth', 1), ('imitation', 2), ('ideology', 7), ('aleppo', 2), ('sofyan', 2), ('djalil', 1), ('edited', 2), ('ambiguous', 2), ('grabbed', 3), ('anna', 5), ('politkovskaya', 5), ('fireworks', 7), ('careless', 1), ('discarded', 1), ('novaya', 1), ('gazeta', 1), ('elevator', 3), ('trincomalee', 6), ('lakshman', 4), ('kadirgamar', 4), ('rick', 2), ('perry', 8), ('ramirez', 20), ('tunnels', 20), ('fred', 5), ('eckhard', 3), ('misunderstood', 2), ('mechanical', 5), ('martina', 7), ('hingis', 15), ('professional', 21), ('volvo', 1), ('pattaya', 2), ('homelessness', 2), ('crowns', 2), ('allocating', 1), ('stocking', 3), ('amendment', 29), ('unrelated', 7), ('paya', 1), ('lebar', 1), ('refuel', 1), ('occurs', 7), ('grozny', 5), ('caucasus', 12), ('starye', 1), ('atagi', 2), ('novye', 1), ('disinformation', 1), ('discredit', 5), ('aslan', 4), ('maskhadov', 10), ('consistently', 4), ('favored', 15), ('timeline', 3), ('adamantly', 2), ('timelines', 1), ('desecrated', 7), ('faizabad', 2), ('badakhshan', 3), ('unconfirmed', 4), ('avalanche', 8), ('kohistan', 2), ('exploring', 3), ('romanian', 21), ('bucharest', 6), ('marie', 14), ('jeanne', 2), ('ion', 3), ('sorin', 2), ('miscoci', 2), ('eduard', 3), ('ovidiu', 2), ('ohanesian', 2), ('translator', 11), ('monaf', 1), ('traian', 6), ('basescu', 9), ('collecting', 10), ('launcher', 8), ('dragan', 7), ('crnogorac', 2), ('banja', 2), ('luka', 2), ('atrocity', 3), ('purnomo', 1), ('yusgiantoro', 1), ('tapped', 3), ('rudd', 5), ('770', 1), ('minustah', 2), ('marc', 6), ('plum', 1), ('257', 3), ('nasir', 1), ('dhakla', 1), ('shaikh', 4), ('undergoing', 13), ('mansoor', 2), ('facilitating', 4), ('guam', 5), ('ayodhya', 2), ('mammoth', 3), ('verdicts', 3), ('convictions', 6), ('undersecretary', 19), ('juster', 1), ('biotechnology', 8), ('nano', 4), ('micro', 1), ('miniature', 1), ('atoms', 2), ('christina', 1), ('rocca', 1), ('jannati', 6), ('sermon', 4), ('instigating', 3), ('anatolian', 2), ('schelling', 3), ('aumann', 3), ('theory', 6), ('mathematically', 2), ('mundane', 1), ('choices', 6), ('runoffs', 2), ('federico', 4), ('lombardi', 4), ('poitou', 1), ('charentes', 1), ('salehi', 5), ('respublika', 1), ('disparaging', 1), ('kazakhs', 1), ('editor', 34), ('galina', 2), ('dyrdina', 1), ('dogged', 2), ('lawsuits', 17), ('firebombing', 2), ('incumbent', 15), ('nursultan', 5), ('nazarbayev', 9), ('spin', 18), ('boldak', 14), ('riding', 15), ('samir', 4), ('sumaidaie', 3), ('rabbi', 4), ('desecrating', 1), ('sabbath', 3), ('yosef', 2), ('shalom', 8), ('eliashiv', 1), ('violates', 9), ('refraining', 1), ('electronics', 12), ('ultra', 10), ('judaism', 4), ('noble', 3), ('uniforms', 14), ('aiding', 13), ('miranshah', 5), ('liter', 11), ('hearted', 4), ('poppy', 13), ('expensive', 14), ('lightly', 5), ('spokesmen', 8), ('kyaw', 3), ('hsann', 2), ('subsidizing', 2), ('varies', 3), ('muhammed', 3), ('sunrise', 3), ('sundown', 4), ('iftaar', 1), ('kabal', 1), ('everyday', 3), ('greedy', 2), ('hainan', 5), ('campus', 3), ('danzhou', 1), ('ripe', 1), ('reed', 4), ('plc', 3), ('oct', 4), ('89', 10), ('141', 5), ('pence', 9), ('94', 12), ('149', 3), ('packaging', 2), ('118', 1), ('discontinued', 2), ('pretax', 2), ('133', 2), ('expectations', 13), ('135', 11), ('388', 1), ('disposal', 6), ('ppp', 6), ('household', 10), ('demographic', 3), ('delphi', 4), ('reorganize', 2), ('fertility', 2), ('necessitate', 1), ('exceed', 10), ('municipalities', 2), ('transfers', 11), ('amounting', 1), ('chronically', 2), ('advances', 5), ('deepest', 2), ('projection', 1), ('upswing', 6), ('attributable', 3), ('rebounding', 4), ('bundesbank', 1), ('parent', 6), ('likewise', 3), ('annum', 1), ('2016', 3), ('viking', 3), ('tapered', 1), ('adoption', 11), ('christianity', 11), ('olav', 1), ('tryggvason', 1), ('994', 1), ('1397', 1), ('1814', 4), ('norwegians', 3), ('cession', 1), ('nationalism', 3), ('1905', 4), ('neutral', 9), ('outset', 1), ('nonetheless', 5), ('1940', 6), ('miller', 17), ('adjacent', 8), ('referenda', 4), ('preserving', 9), ('exiles', 12), ('rpf', 4), ('upheavals', 2), ('exacerbated', 8), ('culminating', 2), ('orchestrated', 6), ('rwandans', 1), ('approximately', 23), ('retribution', 2), ('zaire', 2), ('bent', 4), ('retaking', 1), ('rout', 2), ('kigali', 4), ('andorra', 7), ('comparative', 1), ('sheep', 12), ('consist', 3), ('perfumes', 2), ('monkeys', 3), ('mimics', 1), ('apt', 2), ('pupils', 1), ('arrayed', 1), ('danced', 3), ('courtiers', 1), ('spectacle', 3), ('till', 2), ('courtier', 1), ('mischief', 1), ('pocket', 5), ('nuts', 3), ('forgot', 2), ('dancing', 6), ('actors', 7), ('tearing', 5), ('robes', 2), ('amidst', 1), ('laughter', 3), ('huntsman', 2), ('dogs', 9), ('basket', 2), ('wished', 5), ('owner', 22), ('longing', 1), ('abstain', 2), ('enjoy', 18), ('thief', 5), ('plunder', 1), ('honest', 13), ('merely', 6), ('wiled', 1), ('dragging', 2), ('inaction', 2), ('edwards', 4), ('frontrunner', 4), ('lashed', 11), ('raffaele', 5), ('checked', 10), ('fluid', 2), ('scar', 1), ('cavity', 1), ('tension', 22), ('flared', 8), ('claudia', 2), ('annyaso', 1), ('complication', 2), ('populous', 11), ('ted', 2), ('onulak', 1), ('passionate', 1), ('blues', 12), ('jazz', 7), ('greats', 2), ('charlie', 1), ('parker', 2), ('hooker', 2), ('influences', 1), ('eyesight', 2), ('inspiration', 4), ('unleashed', 3), ('monsoon', 22), ('chittagong', 4), ('hillside', 1), ('mud', 11), ('thunderstorms', 1), ('lightning', 9), ('surgeons', 2), ('drain', 4), ('quadruple', 4), ('lasts', 2), ('taji', 3), ('qada', 1), ('flashpoint', 2), ('golf', 2), ('clijsters', 8), ('vera', 2), ('douchevina', 3), ('eastbourne', 1), ('tournaments', 4), ('wells', 10), ('junior', 15), ('amelie', 2), ('mauresmo', 3), ('strapping', 1), ('shortness', 1), ('breath', 4), ('impunity', 5), ('nowak', 6), ('tennessee', 6), ('highlight', 11), ('accomplishments', 1), ('smoky', 1), ('recommit', 1), ('stewardship', 2), ('cleaner', 5), ('ozone', 1), ('engines', 9), ('acres', 1), ('chide', 1), ('sidestep', 1), ('migrating', 8), ('flamingo', 3), ('milder', 3), ('h5n2', 5), ('variant', 4), ('admiral', 20), ('hector', 1), ('persecution', 12), ('carmona', 5), ('vault', 6), ('robberies', 1), ('belo', 1), ('horizonte', 1), ('dealership', 2), ('fraction', 7), ('daring', 2), ('fortaleza', 1), ('phony', 2), ('landscaping', 1), ('thick', 6), ('virulent', 3), ('readings', 6), ('ituri', 9), ('stationed', 19), ('sein', 6), ('ward', 8), ('strengthens', 3), ('grip', 8), ('bunker', 6), ('dump', 14), ('flock', 14), ('liaoning', 8), ('reformers', 4), ('societies', 6), ('ambition', 3), ('944', 1), ('bamboo', 1), ('pipes', 3), ('unlawful', 7), ('kyu', 1), ('hyung', 2), ('araujo', 2), ('interfered', 1), ('maltreating', 2), ('13th', 15), ('rampaging', 2), ('aflame', 1), ('204', 1), ('permitting', 1), ('amiens', 1), ('savigny', 1), ('sur', 3), ('orge', 1), ('lyon', 8), ('firebomb', 1), ('younger', 18), ('erupt', 3), ('turns', 8), ('appearances', 9), ('seclusion', 2), ('zamoanga', 1), ('opposite', 3), ('hauling', 3), ('compressed', 1), ('liquified', 1), ('g8', 18), ('completing', 11), ('obligation', 4), ('bernie', 4), ('mac', 3), ('comedy', 9), ('comic', 5), ('mccullogh', 1), ('letterman', 1), ('drama', 3), ('grinning', 2), ('leash', 3), ('ibn', 1), ('disagree', 4), ('64th', 1), ('pearl', 10), ('battleship', 1), ('sunk', 2), ('guests', 8), ('fleet', 23), ('waver', 1), ('argued', 23), ('compliant', 2), ('ringleader', 5), ('karrada', 3), ('mess', 1), ('greed', 3), ('renewable', 10), ('hazem', 3), ('shaalan', 3), ('appealing', 19), ('diverse', 11), ('antiviral', 1), ('respirator', 2), ('broad', 30), ('fourteen', 5), ('inhumane', 2), ('afterwards', 12), ('killers', 4), ('bayaman', 2), ('erkinbayev', 6), ('kara', 2), ('belongs', 8), ('rift', 6), ('bounty', 11), ('hunters', 15), ('rodrigo', 9), ('granda', 6), ('froze', 6), ('colombians', 3), ('fossils', 1), ('dinosaurs', 3), ('fossilized', 1), ('bones', 3), ('titanosaurs', 4), ('queensland', 2), ('brisbane', 5), ('nicknamed', 2), ('cooper', 2), ('ranchers', 2), ('eromanga', 1), ('discoveries', 7), ('weighs', 1), ('prehistoric', 1), ('roam', 1), ('necks', 2), ('tails', 3), ('sauropods', 1), ('sajida', 1), ('rishawi', 2), ('belt', 13), ('detonate', 10), ('absentia', 10), ('jordanians', 4), ('bolton', 22), ('appearing', 11), ('fielding', 3), ('bluntly', 2), ('assertion', 8), ('possessed', 2), ('dodd', 4), ('dreadfully', 1), ('mozambican', 2), ('departed', 4), ('receding', 4), ('rajasthan', 3), ('barmer', 1), ('rubber', 12), ('euphrates', 10), ('anchorwoman', 1), ('carries', 12), ('unearthed', 6), ('anjar', 2), ('uniform', 13), ('guerrero', 3), ('amritsar', 3), ('lahore', 22), ('wagah', 2), ('problematic', 3), ('chilpancingo', 1), ('instructions', 4), ('ira', 12), ('kurzban', 2), ('ploy', 3), ('desperately', 3), ('depleting', 2), ('submerged', 8), ('airlifted', 4), ('pleading', 4), ('bags', 6), ('overwhelmed', 5), ('sick', 23), ('desperate', 10), ('sos', 1), ('envoys', 15), ('rid', 9), ('podium', 3), ('dissatisified', 1), ('contend', 4), ('roddick', 17), ('berdych', 2), ('ferrer', 3), ('spots', 10), ('atp', 3), ('novak', 2), ('djokovic', 2), ('swede', 4), ('robin', 4), ('soderling', 1), ('finale', 1), ('finalist', 1), ('gael', 1), ('monfils', 1), ('verdasco', 2), ('nikolay', 2), ('davydenko', 2), ('argentine', 25), ('potro', 1), ('edition', 15), ('photography', 1), ('pale', 3), ('branislav', 2), ('jovicevic', 1), ('gaining', 18), ('assisting', 10), ('disrupting', 19), ('warplane', 2), ('cartosat', 2), ('hamsat', 2), ('sriharokota', 2), ('indigenous', 28), ('pslav', 2), ('blasted', 5), ('spaceport', 1), ('bengal', 18), ('heavier', 1), ('precise', 1), ('frequencies', 4), ('5400', 1), ('homicides', 1), ('physically', 7), ('heightened', 14), ('87', 14), ('dealers', 6), ('escalation', 8), ('badri', 1), ('servicemen', 9), ('askari', 2), ('priorities', 11), ('silencers', 1), ('izmir', 7), ('neftaly', 1), ('platero', 2), ('synagogues', 2), ('deceptive', 1), ('tactics', 13), ('advertise', 2), ('tar', 1), ('manufacturers', 6), ('labeling', 1), ('aspect', 1), ('advertising', 8), ('altria', 1), ('philip', 6), ('195', 3), ('louis', 13), ('tsunamis', 4), ('droughts', 5), ('weary', 2), ('comoros', 5), ('barry', 8), ('verbal', 5), ('altercation', 2), ('funneled', 1), ('slight', 14), ('courthouse', 5), ('wali', 13), ('counterterrorist', 1), ('mansur', 2), ('heather', 6), ('mills', 6), ('vows', 3), ('mccartney', 5), ('stars', 13), ('contestant', 1), ('artificial', 4), ('limb', 1), ('sleeve', 1), ('prosthesis', 1), ('slipping', 4), ('fee', 10), ('viva', 1), ('rated', 4), ('candles', 5), ('performers', 6), ('curtains', 1), ('panicking', 2), ('underfoot', 1), ('mainstream', 10), ('kennedy', 35), ('roll', 10), ('akwa', 1), ('ibom', 1), ('indonesians', 5), ('afren', 1), ('steal', 8), ('fairer', 2), ('3600', 1), ('gradually', 19), ('postwar', 3), ('publish', 10), ('examination', 6), ('deepening', 2), ('miran', 5), ('mukhtar', 1), ('resorted', 2), ('stood', 14), ('wiping', 4), ('adversely', 3), ('swaziland', 11), ('kadhimiya', 3), ('ambush', 45), ('mehmet', 9), ('yilmaz', 1), ('resit', 1), ('isik', 1), ('hawija', 1), ('turkmens', 2), ('knowledge', 16), ('confidential', 10), ('discussion', 16), ('pumping', 7), ('aquifer', 3), ('scarcity', 1), ('moral', 10), ('drawing', 12), ('condolezza', 1), ('depress', 2), ('steepest', 2), ('partisan', 4), ('cbo', 1), ('threefold', 1), ('ryazanov', 2), ('pays', 4), ('230', 4), ('periodic', 5), ('airlift', 4), ('presents', 13), ('accurate', 8), ('nabil', 4), ('jolted', 4), ('negar', 1), ('kerman', 1), ('torbat', 1), ('heydariyeh', 1), ('seismic', 3), ('fault', 7), ('ancient', 22), ('bam', 4), ('lindsay', 11), ('lohan', 9), ('utah', 6), ('cirque', 5), ('lodge', 8), ('alcohol', 12), ('sundance', 2), ('rehab', 1), ('wonderland', 1), ('chrysler', 9), ('570', 1), ('statehood', 7), ('monica', 2), ('ca', 5), ('dui', 1), ('clinic', 14), ('coretta', 3), ('baja', 5), ('diego', 8), ('surgeries', 2), ('rays', 2), ('unconventional', 1), ('levy', 8), ('mwanawasa', 14), ('undergone', 5), ('ovarian', 3), ('baseless', 8), ('starvation', 9), ('pyinmana', 2), ('zambian', 9), ('rupiah', 5), ('banda', 9), ('bunkers', 3), ('rugged', 12), ('defensible', 1), ('fallback', 1), ('belgrade', 24), ('soren', 2), ('jessen', 3), ('vuk', 2), ('draskovic', 2), ('vojislav', 5), ('kostunica', 3), ('administering', 4), ('nawaz', 12), ('excluded', 4), ('detail', 7), ('khair', 1), ('shuja', 1), ('gereshk', 2), ('malian', 1), ('mauritanian', 8), ('sid', 2), ('ould', 12), ('hamma', 3), ('maghreb', 6), ('nouakchott', 4), ('nouadhibou', 1), ('dsm', 3), ('235', 1), ('guilders', 9), ('113', 6), ('144', 4), ('outlay', 2), ('barthelemy', 3), ('villas', 1), ('inhibits', 1), ('attracts', 3), ('hadj', 1), ('ondimba', 1), ('structures', 7), ('abundant', 3), ('nonpermanent', 6), ('prix', 3), ('stonemason', 1), ('marinus', 1), ('301', 1), ('1975', 18), ('emigration', 5), ('dependence', 20), ('prolonged', 11), ('frelimo', 3), ('marxism', 1), ('renamo', 1), ('delicate', 3), ('joaquim', 1), ('chissano', 1), ('armando', 3), ('emilio', 1), ('guebuza', 2), ('silverstone', 1), ('questionable', 3), ('disqualification', 2), ('defaulted', 4), ('dollarization', 1), ('terminate', 2), ('discouraged', 2), ('ecuadorian', 5), ('remittance', 3), ('flows', 9), ('lethal', 18), ('domenech', 3), ('uphill', 1), ('ceremonial', 8), ('startup', 1), ('saltillo', 1), ('respecting', 3), ('navigation', 4), ('tire', 5), ('maintenance', 16), ('cameroonian', 1), ('kilted', 1), ('protester', 6), ('staple', 5), ('playlists', 1), ('scandals', 18), ('awori', 2), ('mwiraria', 1), ('prevalent', 3), ('manifesto', 3), ('giuliana', 2), ('sgrena', 4), ('susilo', 8), ('bambang', 9), ('yudhoyono', 12), ('blockaded', 2), ('conserve', 2), ('necessarily', 2), ('unveiling', 1), ('bellamy', 1), ('desperation', 3), ('laments', 2), ('initiatives', 16), ('engulfed', 4), ('baixinglou', 1), ('chaoyang', 1), ('diners', 1), ('waiters', 1), ('improper', 5), ('stove', 2), ('flexible', 3), ('nath', 4), ('backtrack', 1), ('poorer', 5), ('mosques', 14), ('onitsha', 2), ('anambra', 2), ('scuffle', 2), ('hausas', 1), ('ibos', 1), ('sparks', 2), ('reprisals', 3), ('asadabad', 5), ('overshadowed', 2), ('truckers', 4), ('dc', 3), ('repercussions', 2), ('anibal', 1), ('cavaco', 3), ('cap', 3), ('repression', 16), ('correo', 1), ('caroni', 1), ('persecuting', 2), ('styled', 1), ('openness', 6), ('protectionism', 3), ('balanced', 5), ('hamza', 7), ('muhajer', 3), ('landmarks', 3), ('neighborhoods', 11), ('lendu', 5), ('deploring', 1), ('socialists', 3), ('ride', 4), ('calmer', 2), ('jela', 1), ('franceschi', 1), ('transforming', 4), ('balkans', 10), ('succumb', 2), ('hygiene', 3), ('hampton', 1), ('grouping', 8), ('informal', 16), ('stalemate', 13), ('alexi', 1), ('barinov', 5), ('nenets', 1), ('lukoil', 4), ('hussain', 9), ('shahristani', 2), ('perhaps', 9), ('timeframe', 2), ('cautioned', 12), ('premature', 3), ('recruit', 8), ('extends', 9), ('attorneys', 13), ('whites', 2), ('selection', 13), ('molesting', 2), ('neverland', 4), ('acquittal', 1), ('mannar', 2), ('67', 16), ('ljubicic', 6), ('moya', 8), ('chennai', 3), ('squandered', 2), ('tiebreaker', 2), ('kristof', 1), ('vliegen', 2), ('seymour', 2), ('hersh', 5), ('scare', 7), ('rallying', 3), ('memo', 10), ('individual', 21), ('drowning', 2), ('electrocution', 2), ('collapsing', 3), ('downed', 8), ('downpour', 1), ('drainage', 3), ('sprinters', 2), ('konstantinos', 1), ('kenteris', 3), ('thanou', 3), ('lausanne', 4), ('render', 2), ('provisionally', 2), ('athletics', 5), ('iaaf', 1), ('accorded', 4), ('citizenship', 35), ('pentastar', 1), ('dodge', 1), ('jeep', 4), ('governance', 14), ('multimillion', 2), ('systemic', 3), ('arap', 3), ('moi', 5), ('taha', 6), ('amiri', 1), ('shiite', 1), ('clogged', 4), ('retract', 1), ('elias', 4), ('napoleon', 2), ('gomez', 3), ('mena', 4), ('safwat', 1), ('gallbladder', 3), ('grooming', 2), ('gamal', 7), ('succession', 17), ('hamstring', 1), ('olsson', 4), ('dn', 2), ('athletic', 1), ('marian', 1), ('oprea', 1), ('dmitrij', 1), ('valukevic', 1), ('chandrika', 6), ('kumaratunga', 9), ('flocked', 1), ('televisions', 1), ('regard', 9), ('ethnicity', 2), ('staggering', 1), ('deadlocked', 10), ('overture', 1), ('difficulty', 10), ('stall', 5), ('wreck', 4), ('likud', 30), ('scuttle', 1), ('shinui', 3), ('defied', 10), ('knesset', 5), ('karami', 9), ('derail', 17), ('brazzaville', 4), ('grounded', 10), ('unimaginable', 3), ('dimension', 1), ('pedophiles', 2), ('priesthood', 1), ('halls', 2), ('powered', 7), ('accountability', 6), ('hierarchy', 1), ('swiftly', 1), ('errant', 3), ('fastest', 14), ('kyoto', 17), ('sells', 8), ('fisheries', 5), ('busan', 3), ('assurances', 12), ('koreas', 23), ('lucio', 5), ('gutierrez', 13), ('examined', 10), ('infiltrators', 2), ('950', 2), ('cement', 5), ('pilings', 1), ('sandy', 3), ('floating', 6), ('swimming', 3), ('pulls', 6), ('ninewa', 1), ('2900', 1), ('deployments', 4), ('straining', 2), ('truckloads', 2), ('jeered', 1), ('folk', 4), ('joan', 1), ('baez', 1), ('casey', 4), ('vigils', 1), ('pave', 6), ('incumbents', 1), ('suleiman', 7), ('forging', 6), ('motorist', 3), ('damiri', 1), ('tayyeb', 1), ('posing', 9), ('greenback', 3), ('upward', 8), ('ayoub', 1), ('qawasmi', 3), ('sweeps', 4), ('fewest', 1), ('gordon', 16), ('outlined', 9), ('accountable', 9), ('shopping', 24), ('fashion', 13), ('retail', 21), ('weakest', 2), ('lure', 3), ('shoppers', 4), ('erez', 4), ('infiltrations', 3), ('cfco', 1), ('pointe', 1), ('noire', 1), ('aab', 1), ('ghum', 1), ('baluchistan', 46), ('baluchis', 2), ('waving', 9), ('fnj', 1), ('subdue', 4), ('worsens', 1), ('mendez', 2), ('pits', 6), ('jacob', 6), ('zuma', 20), ('verdict', 19), ('soweto', 2), ('apartheid', 19), ('consensual', 4), ('drawn', 23), ('incidence', 3), ('sweeping', 9), ('423', 2), ('defines', 4), ('constitutes', 2), ('outlines', 5), ('invite', 4), ('uae', 6), ('dhabi', 1), ('attiya', 2), ('trusts', 1), ('liable', 3), ('painkiller', 1), ('vioxx', 6), ('diploma', 1), ('debates', 4), ('migrated', 2), ('haggling', 1), ('immigrate', 1), ('tentatively', 3), ('builds', 2), ('ford', 21), ('unveils', 1), ('carmakers', 1), ('toyota', 10), ('sinai', 16), ('badran', 1), ('wheel', 1), ('explorer', 3), ('suv', 1), ('lutfullah', 2), ('mashal', 2), ('silvio', 7), ('berlusconi', 16), ('fatwa', 4), ('zoo', 10), ('panda', 10), ('crushed', 9), ('newborn', 2), ('cub', 8), ('yinghua', 2), ('zookeepers', 1), ('cubs', 2), ('incubator', 1), ('jingguo', 1), ('nurse', 1), ('rolled', 6), ('pandas', 8), ('captivity', 16), ('gomoa', 1), ('buduburam', 1), ('resettle', 5), ('rabiah', 1), ('authenticate', 2), ('cousins', 1), ('taba', 4), ('core', 13), ('catalina', 1), ('1928', 4), ('lie', 10), ('perpetuate', 1), ('beatings', 6), ('shocks', 7), ('ingestion', 1), ('urine', 4), ('forcibly', 8), ('algerians', 2), ('abdelaziz', 7), ('bouteflika', 5), ('enable', 4), ('khalaf', 6), ('facilitation', 2), ('levey', 3), ('cornerstone', 3), ('excludes', 1), ('fallout', 3), ('undercut', 4), ('bedouin', 4), ('daytime', 2), ('umm', 3), ('haiman', 1), ('fugitives', 7), ('evacuating', 6), ('netzarim', 1), ('morag', 1), ('stir', 4), ('ateret', 1), ('unamid', 1), ('frightened', 4), ('neglecting', 2), ('jakob', 4), ('kellenberger', 3), ('listens', 1), ('jurists', 1), ('dadis', 2), ('camara', 5), ('vowing', 8), ('arbitrarily', 1), ('fayssal', 1), ('mekdad', 1), ('erecting', 2), ('rauf', 7), ('shields', 8), ('knock', 2), ('throwers', 3), ('thrown', 12), ('veracity', 1), ('tikriti', 1), ('perjury', 3), ('disc', 1), ('contradictory', 3), ('celebratory', 2), ('xinjiang', 12), ('turpan', 2), ('distributed', 10), ('690', 1), ('catastrophic', 8), ('551', 1), ('fema', 12), ('hadley', 8), ('moustapha', 7), ('balkh', 1), ('atta', 4), ('teamed', 4), ('mihtarlam', 1), ('openings', 1), ('offshoot', 2), ('mistaken', 7), ('translation', 4), ('stringers', 1), ('notice', 17), ('occasionally', 7), ('elco', 2), ('rockford', 1), ('fasteners', 1), ('155', 4), ('pressures', 6), ('outweighs', 1), ('denominated', 3), ('creditor', 1), ('onset', 3), ('dwindled', 1), ('dried', 3), ('appreciation', 5), ('dilma', 1), ('rousseff', 1), ('1829', 2), ('protracted', 2), ('abolished', 6), ('1981', 15), ('ec', 3), ('prospect', 5), ('default', 3), ('emu', 3), ('voluntarily', 11), ('fishermen', 18), ('tokelau', 4), ('confine', 1), ('recurrent', 6), ('copra', 5), ('postage', 7), ('souvenir', 1), ('coins', 5), ('handicrafts', 7), ('remitted', 1), ('khurasan', 1), ('medieval', 5), ('merv', 1), ('1865', 5), ('1885', 3), ('ussr', 12), ('hydrocarbon', 2), ('boon', 1), ('underdeveloped', 4), ('extraction', 7), ('plaintiff', 1), ('actively', 7), ('saparmurat', 2), ('nyyazow', 2), ('gurbanguly', 1), ('cook', 10), ('fruit', 6), ('pearls', 2), ('emigrants', 5), ('bloated', 3), ('accumulating', 1), ('encouragement', 4), ('rekindled', 2), ('heathens', 1), ('peking', 1), ('tongue', 2), ('translate', 1), ('editorial', 7), ('pang', 1), ('devils', 1), ('dwellings', 4), ('mongolian', 3), ('barbarity', 1), ('incensed', 1), ('reader', 5), ('wager', 1), ('dug', 3), ('sown', 3), ('thistles', 2), ('janitor', 1), ('organist', 1), ('pews', 1), ('kari', 2), ('barber', 3), ('conakry', 1), ('squeezed', 1), ("o'keefe", 1), ('reveals', 2), ('incredibly', 1), ('celestial', 1), ('astronomical', 1), ('grounding', 5), ('columbia', 10), ('overrule', 1), ('dumarsais', 2), ('simeus', 3), ('arlington', 4), ('cemetery', 8), ('mulgueta', 1), ('debalk', 1), ('newcastle', 1), ('fowl', 6), ('harmless', 3), ('pigeons', 5), ('watching', 10), ('migratory', 12), ('aden', 21), ('drownings', 1), ('137', 4), ('134', 2), ('wreath', 6), ('unknowns', 1), ('escaping', 4), ('homelands', 4), ('ruthless', 4), ('doves', 1), ('narathiwat', 9), ('origami', 1), ('folded', 1), ('symbolizing', 2), ('thais', 2), ('andean', 12), ('occassion', 1), ('picnics', 1), ('cemeteries', 1), ('pacts', 2), ('disadvantage', 2), ('cheaper', 12), ('looms', 2), ('banning', 12), ('xian', 1), ('han', 1), ('archaeologists', 9), ('wuhan', 1), ('peugeot', 3), ('citroen', 2), ('dongfeng', 2), ('presided', 6), ('yakaghund', 1), ('mohmand', 10), ('flattened', 5), ('wheelchairs', 1), ('lawless', 8), ('extricate', 1), ('bilingual', 1), ('ashti', 1), ('adjourn', 3), ('recess', 8), ('stab', 5), ('protestant', 9), ('missionaries', 8), ('ahmet', 5), ('necdet', 4), ('sezer', 4), ('justification', 5), ('anhui', 3), ('dangtu', 1), ('produces', 19), ('uniting', 2), ('perpetrators', 10), ('promptly', 6), ('cowardly', 3), ('curling', 3), ('swedes', 4), ('identical', 1), ('landslides', 16), ('farmland', 5), ('strips', 2), ('photojournalist', 5), ('stampa', 1), ('arturo', 3), ('parisi', 1), ('pelosi', 9), ('trajectory', 1), ('setbacks', 3), ('crumpton', 2), ('miliant', 1), ('324', 2), ('iskandariya', 1), ('recruits', 15), ('graduated', 1), ('courses', 8), ('sulaymaniyeh', 1), ('emerli', 1), ('maref', 1), ('consuming', 4), ('mirpur', 1), ('sacramento', 1), ('scurrying', 1), ('cool', 7), ('grids', 3), ('conditioners', 1), ('mahmood', 1), ('holbrooke', 3), ('reviews', 5), ('paired', 2), ('developmentaly', 1), ('ganji', 6), ('massoud', 8), ('moghaddasi', 1), ('articles', 16), ('negroponte', 19), ('640', 1), ('projections', 4), ('stockpiled', 1), ('relocating', 2), ('relocation', 3), ('donor', 36), ('michaelle', 1), ('youngest', 10), ('adrienne', 1), ('clarkson', 8), ('immigrated', 2), ('quebec', 3), ('dictatorial', 2), ('dominion', 3), ('1867', 4), ('beliefs', 5), ('turnover', 1), ('respondents', 11), ('referendums', 3), ('rejections', 2), ('unanimous', 6), ('commonly', 5), ('awacs', 1), ('acquiring', 5), ('latvian', 5), ('riga', 2), ('flies', 4), ('margraten', 1), ('kathleen', 4), ('blanco', 6), ('cameron', 7), ('erased', 1), ('wrap', 3), ('vermilion', 1), ('tidal', 6), ('nagin', 13), ('algiers', 10), ('kohat', 1), ('tirah', 2), ('raymond', 5), ('azar', 2), ('marathon', 6), ('mizuki', 1), ('noguchi', 4), ('thigh', 5), ('tomiaki', 1), ('fukuda', 6), ('marathoner', 1), ('holder', 6), ('paula', 6), ('radcliffe', 1), ('fracture', 2), ('liaison', 3), ('lancaster', 1), ('surveys', 9), ('tzachi', 1), ('hanegbi', 1), ('tragic', 3), ('parcel', 3), ('explode', 7), ('defused', 4), ('packages', 9), ('anarchist', 1), ('stranding', 3), ('kaesong', 1), ('bride', 2), ('sting', 2), ('switched', 2), ('routinely', 13), ('drills', 9), ('vitaly', 2), ('churkin', 2), ('identifying', 5), ('roberto', 7), ('tarongoy', 3), ('roy', 7), ('hallums', 5), ('tattoo', 2), ('dating', 9), ('drum', 1), ('bugle', 1), ('pageant', 1), ('twilight', 1), ('jeff', 3), ('feuer', 1), ('storied', 2), ('bordered', 2), ('entrapment', 1), ('gdansk', 3), ('shipyard', 5), ('streamline', 4), ('hefty', 2), ('sustainability', 5), ('walesa', 2), ('27th', 6), ('speedy', 2), ('affluent', 4), ('mansour', 3), ('undercover', 11), ('fake', 13), ('khamenei', 23), ('nida', 2), ('tribe', 8), ('rampant', 6), ('fairness', 4), ('destabilized', 3), ('adventure', 3), ('plaza', 1), ('encircled', 1), ('revava', 1), ('hilltop', 3), ('expecting', 3), ('brent', 9), ('demarcating', 1), ('tarasyuk', 5), ('stagnant', 3), ('abyei', 1), ('kashmirs', 1), ('ravi', 6), ('khanna', 6), ('upgraded', 7), ('saffir', 1), ('simpson', 2), ('sometime', 7), ('sits', 9), ('quitting', 4), ('monarch', 12), ('moldova', 13), ('disney', 8), ('premiere', 4), ('doubling', 5), ('viewership', 1), ('musicalphenomenon', 1), ('zac', 1), ('efron', 1), ('ashley', 2), ('tisdale', 1), ('spawning', 1), ('patriot', 11), ('defeatism', 1), ('justified', 11), ('facts', 5), ('kenichiro', 3), ('sasae', 3), ('80s', 3), ('stalinist', 2), ('convincing', 4), ('kharazzi', 2), ('nasser', 12), ('defiance', 6), ('mockery', 1), ('kocharian', 2), ('characterizing', 2), ('chased', 12), ('agreeing', 4), ('sacrificed', 3), ('detecting', 3), ('santo', 6), ('domingo', 4), ('145', 5), ('higüey', 1), ('faxas', 1), ('216', 2), ('quand', 1), ('nguyen', 5), ('quoc', 1), ('thanh', 2), ('hoa', 2), ('248', 3), ('resurfaced', 4), ('shamil', 7), ('basayev', 15), ('nalchik', 2), ('kabardino', 1), ('balkaria', 1), ('exploiters', 1), ('laos', 16), ('reliable', 2), ('exponential', 1), ('pornography', 4), ('tehrik', 1), ('maulvi', 2), ('appropriately', 4), ('hadithah', 1), ('blitz', 1), ('natonski', 1), ('accords', 32), ('adamantios', 1), ('vassilakis', 1), ('recommitted', 1), ('decentralized', 2), ('compensated', 6), ('menatep', 4), ('incurred', 3), ('plummeted', 3), ('skeptics', 1), ('kajaki', 3), ('illicit', 5), ('harvests', 3), ('overshot', 4), ('nbc', 6), ('bremer', 5), ('hatta', 1), ('radjasa', 1), ('correspondents', 3), ('microsystems', 1), ('dominance', 8), ('228', 3), ('beitar', 1), ('illit', 1), ('efrat', 1), ('resilience', 2), ('courage', 10), ('shoulder', 12), ('eduardo', 8), ('marcelo', 1), ('antezana', 1), ('gonzalo', 2), ('disposing', 1), ('dimming', 2), ('nonessential', 2), ('lights', 10), ('cheated', 1), ('riches', 2), ('pumped', 4), ('widow', 11), ('user', 1), ('antarctic', 24), ('pyramids', 2), ('smithsonian', 5), ('castle', 1), ('fist', 3), ('cube', 2), ('bachelet', 16), ('candlelight', 2), ('saved', 9), ('sleet', 1), ('bout', 1), ('decimate', 1), ('wholesale', 5), ('dhusamareb', 1), ('marergur', 1), ('ahlu', 3), ('waljama', 1), ('strewn', 1), ('hizbul', 4), ('promoted', 12), ('jeny', 1), ('figueredo', 4), ('frias', 1), ('mccormack', 18), ('attachés', 1), ('tribesman', 3), ('maarib', 2), ('sabotaged', 4), ('operatives', 9), ('proceeds', 9), ('evangelicals', 1), ('apprehend', 1), ('aqili', 2), ('presentation', 4), ('actual', 12), ('referral', 14), ('intend', 8), ('chamber', 14), ('mainz', 1), ('oshkosh', 3), ('wis', 1), ('352', 3), ('chassis', 3), ('softer', 4), ('motor', 10), ('phasing', 2), ('deere', 1), ('midsized', 2), ('inhabitants', 11), ('sultanate', 5), ('muscat', 2), ('1970', 8), ('qaboos', 2), ('restrictive', 7), ('hockey', 4), ('uprisings', 5), ('omanis', 1), ('marches', 11), ('reshuffled', 2), ('acquired', 12), ('1946', 4), ('reestablished', 4), ('hafiz', 1), ("ba'th", 1), ('alawite', 1), ('sect', 7), ('jarome', 1), ('iginla', 1), ('dany', 1), ('heatley', 1), ('shane', 4), ('doan', 1), ('ostensible', 2), ('hizballah', 3), ('antigovernment', 2), ("da'ra", 1), ('repeal', 11), ('legalization', 1), ('quelling', 1), ('tjarnqvist', 1), ('henrik', 1), ('sedin', 1), ('remarkably', 3), ('trafficked', 4), ('hemispheres', 1), ('dredging', 2), ('aragonite', 1), ('sands', 1), ('warden', 5), ('locks', 2), ('mechanic', 1), ('imprudent', 1), ('imprudence', 1), ('wonder', 2), ('thoughtful', 1), ('vicissitudes', 1), ('fortune', 4), ('nap', 1), ('ox', 8), ('cosily', 1), ('rage', 5), ('awakened', 3), ('slumber', 1), ('barked', 1), ('bite', 3), ('muttering', 1), ('ah', 4), ('grudge', 2), ('distant', 10), ('astronomers', 7), ('quite', 13), ('elementary', 5), ('betsy', 1), ('ross', 3), ('qingdao', 4), ('taught', 10), ('techniques', 10), ('gallop', 1), ('sirte', 3), ('shandong', 5), ('jinan', 1), ('trillion', 18), ('pillar', 1), ('liberal', 19), ('metin', 2), ('kaplan', 2), ('caliph', 1), ('cologne', 3), ('mausoleum', 3), ('kemal', 4), ('ataturk', 2), ('caliphate', 1), ('lobbies', 1), ('alireza', 1), ('jamshidi', 1), ('soheil', 1), ('farshad', 1), ('ghorbanpour', 3), ('lies', 12), ('scout', 1), ('transmit', 5), ('masood', 3), ('bastani', 1), ('cyber', 7), ('guarded', 10), ('basilan', 2), ('disguised', 8), ('ghalawiya', 1), ('sleeper', 1), ('isselmou', 1), ('violators', 6), ('liberate', 1), ('cancellations', 4), ("o'hare", 1), ('precipitation', 2), ('visible', 4), ('exception', 5), ('fared', 4), ('reinforce', 3), ('quito', 10), ('amazon', 5), ('sucumbios', 3), ('petroecuador', 4), ('zionist', 2), ('lago', 1), ('agrio', 1), ('lucky', 2), ('turkeys', 7), ('alternate', 10), ('disneyland', 6), ('marshals', 2), ('roasted', 2), ('valiant', 1), ('true', 18), ('nicole', 2), ('orinoco', 3), ('basin', 4), ('recommendation', 15), ('mitofsky', 2), ('obrador', 16), ('prd', 1), ('pan', 9), ('madrazo', 5), ('institutional', 4), ('pri', 4), ('goodluck', 3), ('umaru', 2), ("yar'adua", 7), ('ailment', 4), ('notify', 3), ('notification', 3), ('electing', 4), ('98', 10), ('edged', 2), ('598', 1), ('gores', 1), ('conclusions', 4), ('airbase', 7), ('downing', 6), ('medellin', 2), ('merida', 3), ('780', 3), ('673', 1), ('unpunished', 3), ('assassinating', 2), ('melchior', 1), ('ndadaye', 1), ('kalenga', 1), ('ramadhani', 1), ('masterminds', 4), ('collected', 15), ('analyzed', 1), ('precursor', 3), ('punk', 2), ('anthony', 9), ('lovato', 4), ('defunct', 5), ('mest', 2), ('telephoning', 1), ('wayne', 5), ('matt', 2), ('albums', 6), ('maverick', 1), ('label', 2), ('disbanding', 2), ('needy', 5), ('blumenthal', 2), ('citgo', 4), ('discount', 6), ('embarrass', 1), ('nabaie', 1), ('shepherd', 13), ('unused', 5), ('soar', 5), ('inspects', 1), ('169', 4), ('hafun', 3), ('desolation', 1), ('swimmers', 2), ('currents', 1), ('yuriy', 5), ('yekhanurov', 14), ('plachkov', 6), ('interviewer', 5), ('dianne', 1), ('sawyer', 1), ('shorter', 5), ('barcelona', 10), ('956', 2), ('sand', 3), ('dune', 1), ('granada', 3), ('rider', 3), ('wear', 8), ('sainct', 1), ('swans', 12), ('danube', 3), ('veterinary', 18), ('thessaloniki', 1), ('tenth', 3), ('publishes', 4), ('duck', 6), ('markos', 2), ('kyprianou', 1), ('kongsak', 2), ('wanthana', 1), ('interested', 11), ('pattani', 10), ('yala', 10), ('gibraltar', 9), ('wu', 6), ('bangguo', 2), ('buying', 21), ('noriega', 4), ('populist', 3), ('banners', 11), ('mebki', 2), ('bouake', 2), ('revision', 6), ('ivorians', 2), ('contestants', 2), ('messages', 31), ('eliminating', 13), ('jared', 1), ('cotter', 1), ('jason', 5), ('sloan', 1), ('vie', 1), ('jumper', 1), ('roar', 3), ('ljoekelsoey', 2), ('mitterndorf', 1), ('faultless', 1), ('jumps', 2), ('207', 1), ('788', 2), ('widhoelzl', 2), ('762', 1), ('morgenstern', 1), ('752', 1), ('planica', 1), ('overpayments', 1), ('gaming', 10), ('fees', 17), ('unlocked', 1), ('footlocker', 1), ('gambled', 1), ('neutralized', 4), ('respectively', 5), ('parked', 15), ('lt', 7), ('gen', 8), ('barno', 5), ('kagame', 14), ('evaluating', 3), ('gunboats', 2), ('baylesa', 1), ('boyfriend', 3), ('joel', 4), ('madden', 2), ('charlotte', 2), ('ruz', 7), ('predominately', 6), ('halfway', 2), ('contrary', 6), ('starbucks', 6), ('pricey', 1), ('4th', 1), ('seattle', 8), ('drinkers', 2), ('avoiding', 1), ('hamburgers', 2), ('briskly', 1), ('hey', 2), ('ballad', 1), ('pharrell', 1), ('downloads', 1), ('download', 2), ('themed', 1), ('hamburg', 2), ('yazidi', 2), ('sinjar', 2), ('dakhil', 1), ('qasim', 2), ('reference', 15), ('yazidis', 1), ('saeedi', 2), ('gentry', 1), ('disorders', 2), ('1772', 1), ('1795', 3), ('prussia', 2), ('partitioned', 3), ('279', 1), ('levies', 2), ('unlawfully', 3), ('kivu', 11), ('nioka', 2), ('hillah', 5), ('beheading', 5), ('spied', 2), ('comparatively', 1), ('tolerant', 3), ('850', 8), ('burundian', 1), ('amisom', 2), ('logistics', 14), ('onyango', 1), ('omollo', 1), ('ochami', 1), ('entitled', 6), ('bahonar', 2), ('vahidi', 2), ('marzieh', 1), ('vahid', 2), ('dastjerdi', 1), ('shock', 7), ('transform', 5), ('dilapidated', 2), ('underclass', 1), ('caretaker', 12), ('indulge', 1), ('sajjad', 2), ('seeduzzaman', 1), ('siddiqui', 3), ('elahi', 1), ('bakhsh', 1), ('soomro', 1), ('fakhar', 1), ('personalities', 4), ('ubaydi', 3), ('curtain', 6), ('caches', 4), ('sharki', 2), ('shadid', 2), ('eldest', 5), ('yonhap', 14), ('dignitary', 1), ('undated', 1), ('krzanich', 1), ('ho', 4), ('chi', 1), ('minh', 1), ('hayat', 5), ('thoroughly', 2), ('depots', 2), ('quality', 12), ('pneumonia', 7), ('evaluates', 1), ('culmination', 2), ('belatedly', 2), ('80th', 4), ('presenter', 1), ('gala', 2), ('nicaraguan', 10), ('ortega', 12), ('culminate', 3), ('danes', 2), ('equitable', 4), ('solutions', 5), ('raw', 15), ('ohn', 1), ('bo', 1), ('zin', 1), ('khun', 1), ('sai', 1), ('937', 2), ('wrongfully', 2), ('disbanded', 11), ('purged', 2), ('nib', 1), ('yucatan', 8), ('cancun', 12), ('emptied', 3), ('bused', 1), ('rafts', 2), ('communiqué', 1), ('banjul', 2), ('undermining', 10), ('privatizing', 2), ('simplify', 2), ('innovative', 5), ('boom', 19), ('autopsy', 2), ('halemi', 1), ('pathology', 1), ('autopsies', 1), ('fukusho', 1), ('shinobu', 1), ('hasegawa', 1), ('chaman', 3), ('frustration', 6), ('disillusioned', 1), ('torch', 34), ('flame', 12), ('everest', 9), ('relay', 28), ('ron', 5), ('zonen', 1), ('survivor', 5), ('rodney', 1), ('melville', 1), ('extort', 3), ('cyclical', 2), ('nights', 4), ('djibouti', 25), ('ganey', 1), ('firimbi', 1), ('thune', 2), ('daschle', 3), ('employer', 2), ('111th', 1), ('ed', 2), ('rendell', 1), ('survive', 7), ('daxing', 1), ('xingning', 2), ('roles', 10), ('mayors', 6), ('meizhou', 1), ('apologize', 8), ('loved', 11), ('drunk', 5), ('harmonized', 2), ('mutahida', 2), ('majlis', 4), ('amal', 3), ('qazi', 6), ('betterment', 2), ('masses', 6), ('enriching', 16), ('breaches', 6), ('npt', 3), ('arguing', 12), ('petroleos', 4), ('perez', 11), ('retaining', 2), ('portfolio', 4), ('consolidated', 2), ('forever', 5), ('domineering', 1), ('minded', 5), ('impending', 4), ('ratio', 7), ('hugh', 4), ('tub', 2), ('baked', 2), ('whittaker', 1), ('weddings', 2), ('notting', 1), ('swung', 3), ('insurer', 3), ('306', 1), ('415', 1), ('underperforming', 1), ('basotho', 1), ('sacu', 8), ('dependency', 9), ('royalties', 8), ('mineworkers', 1), ('milling', 2), ('canning', 1), ('leather', 2), ('jute', 2), ('herding', 1), ('drawback', 1), ('362', 1), ('precipitously', 1), ('contributor', 4), ('bhutan', 10), ('sinchulu', 1), ('ceding', 1), ('1907', 2), ('whereby', 1), ('bhutanese', 3), ('criteria', 5), ('krone', 1), ('1947', 14), ('indo', 3), ('formalized', 2), ('defined', 3), ('unhcr', 2), ('jigme', 2), ('singye', 1), ('wangchuck', 2), ('introduce', 5), ('abdicated', 2), ('throne', 16), ('khesar', 1), ('namgyel', 1), ('renegotiated', 2), ('thimphu', 1), ('loosening', 1), ('socialism', 11), ('alleviate', 4), ('inefficiencies', 3), ('preferential', 2), ('vegetables', 7), ('pigs', 9), ('licensing', 6), ('jay', 5), ('venturing', 1), ('peacocks', 4), ('walk', 12), ('moulting', 1), ('1902', 3), ('administer', 3), ('strutted', 1), ('cheat', 1), ('striding', 1), ('pecked', 1), ('plucked', 1), ('borrowed', 2), ('plumes', 1), ('jays', 1), ('behaviour', 1), ('equally', 6), ('annoyed', 4), ('seagull', 1), ('bolted', 3), ('gullet', 1), ('kite', 7), ('richly', 2), ('spendthrift', 1), ('pawned', 1), ('cloak', 1), ('sour', 2), ('inch', 5), ('nose', 12), ('admit', 9), ('solemnly', 1), ('bougainville', 1), ('teeth', 5), ('claws', 4), ('cleanup', 6), ('skimming', 2), ('vents', 2), ('separating', 11), ('cleaned', 5), ('liters', 6), ('oily', 1), ('skimmers', 1), ('halting', 6), ('hookup', 1), ('containment', 2), ('1890s', 1), ('lisa', 3), ('pensacola', 1), ('leased', 1), ('gushing', 1), ('322', 1), ('auditors', 4), ('intensify', 9), ('dues', 1), ('guesthouse', 1), ('vanuatu', 8), ('examples', 3), ('tegua', 1), ('inland', 3), ('accelerating', 7), ('waterborne', 4), ('manifestation', 1), ('executing', 5), ('executes', 1), ('minors', 2), ('juveniles', 3), ('gallows', 2), ('stamped', 1), ('headscarf', 3), ('crying', 5), ('newscaster', 1), ('malfeasance', 1), ('sir', 4), ('allan', 1), ('kemakeza', 1), ('reestablishing', 1), ('unreasonable', 4), ('tiananmen', 10), ('spearheaded', 4), ('ramsi', 1), ('pet', 7), ('cats', 4), ('asians', 4), ('spacewalk', 13), ('fossum', 1), ('garan', 1), ('nitrogen', 3), ('panels', 7), ('analysis', 9), ('olive', 8), ('ridiculed', 3), ('fig', 3), ('seasons', 4), ('yasuo', 3), ('astronaut', 9), ('akihiko', 1), ('hoshide', 2), ('robotic', 5), ('plaintiffs', 2), ('shower', 1), ('foliage', 1), ('despoiling', 1), ('salva', 5), ('kiir', 6), ('mayardit', 2), ('denuded', 1), ('injure', 4), ('radicalism', 3), ('deceive', 1), ('wider', 5), ('democratizing', 1), ('bossaso', 1), ('vaunting', 1), ('purity', 2), ('fearlessness', 1), ('pained', 1), ('subscribers', 2), ('infectious', 6), ('manila', 22), ('silvestre', 1), ('afable', 1), ('moro', 4), ('milf', 3), ('kuala', 8), ('lumpur', 8), ('renounced', 7), ('pure', 3), ('enterprising', 1), ('fearless', 1), ('modernizes', 1), ('arts', 8), ('disappearing', 2), ('schearf', 1), ('airs', 3), ('reasoned', 1), ('folly', 2), ('slumping', 4), ('expenses', 7), ('swicord', 1), ('expression', 15), ('supplement', 6), ('signatories', 1), ('mao', 5), ('rui', 1), ('zhu', 1), ('houze', 1), ('pu', 1), ('endeavoured', 1), ('discover', 5), ('prosperity', 11), ('191', 6), ('199', 2), ('keeps', 10), ('lundestad', 1), ('168', 1), ('spaceship', 3), ('aliens', 5), ('cattle', 22), ('roswell', 1), ('selections', 2), ('u2', 4), ('bono', 2), ('geldof', 3), ('surviving', 8), ('anh', 1), ('ha', 2), ('tay', 1), ('duong', 1), ('vinh', 2), ('1948', 12), ('gore', 13), ('167', 5), ('kalam', 4), ('grim', 3), ('nicobar', 6), ('nadu', 2), ('pondicherry', 1), ('kerala', 5), ('refers', 3), ('clears', 4), ('stakes', 5), ('rails', 1), ('mehrabpur', 1), ('sindh', 6), ('welded', 1), ('contraction', 14), ('darkness', 1), ('meterologists', 1), ('tammy', 3), ('canaveral', 6), ('stan', 10), ('dissipating', 2), ('hollywood', 12), ('wizard', 3), ('oz', 2), ('mgm', 2), ('distinctive', 4), ('roaring', 1), ('restructured', 3), ('movies', 8), ('spyglass', 1), ('entertainment', 10), ('outdo', 1), ('triumphs', 1), ('carl', 4), ('icahn', 1), ('tickets', 11), ('dvds', 2), ('rosales', 3), ('addresses', 9), ('plavia', 1), ('pennetta', 3), ('streak', 4), ('lucie', 5), ('safarova', 6), ('dinara', 5), ('safina', 6), ('gregory', 4), ('patterson', 2), ('firearm', 2), ('recruiting', 23), ('conspirator', 2), ('levar', 1), ("jam'iyyat", 1), ('ul', 7), ('saheeh', 1), ('hammad', 2), ('samana', 1), ('psychiatric', 4), ('latina', 1), ('scapegoating', 1), ('zulia', 4), ('hilda', 2), ('solis', 3), ('criminalizes', 1), ('alienating', 1), ('compassionate', 3), ('flocking', 1), ('charm', 6), ('floral', 3), ('facelift', 1), ('makeover', 2), ('warner', 4), ('modernity', 1), ('cubanization', 1), ('bloodiest', 9), ('dhahran', 2), ('urges', 8), ('vigilance', 3), ('frequented', 2), ('amhed', 1), ('mounted', 10), ('gustavo', 2), ('dominguez', 4), ('osbek', 1), ('castillo', 1), ('diamondbacks', 1), ('feeder', 2), ('alabama', 7), ('francisely', 1), ('bueno', 1), ('pitches', 2), ('braves', 1), ('ilyas', 1), ('shurpayev', 2), ('reestablish', 2), ('repressed', 1), ('hernan', 1), ('arboleda', 2), ('khetaguri', 1), ('ruptured', 3), ('inguri', 1), ('tbilisi', 14), ('malfunctioned', 2), ('strangled', 2), ('raced', 4), ('wta', 6), ('jaw', 1), ('airplanes', 11), ('dagestan', 7), ('extensively', 1), ('ridden', 5), ('signings', 1), ('nickel', 7), ('untapped', 2), ('repayment', 3), ('economically', 5), ('dismiss', 18), ('astana', 3), ('unconstitutional', 8), ('serhiy', 3), ('holovaty', 3), ('betrayal', 1), ('exceeding', 6), ('pretty', 4), ('definite', 1), ('suitable', 3), ('datta', 3), ('khel', 4), ('shana', 1), ('garhi', 1), ('natanz', 6), ('observation', 2), ('ransacking', 1), ('crowds', 23), ('airlifting', 4), ('familiar', 8), ('piled', 2), ('bula', 1), ('hawo', 1), ('bertie', 3), ('ahern', 3), ('belfast', 3), ('protestants', 2), ('photographic', 1), ('overturning', 3), ('vandalized', 2), ('baran', 1), ('formality', 3), ('solemn', 3), ('illusion', 1), ('helmet', 1), ('hoisting', 1), ('confront', 13), ('sergio', 2), ('viera', 2), ('mello', 2), ('aftab', 1), ('sherpao', 1), ('yasir', 3), ('adil', 3), ('deported', 13), ('arrival', 25), ('lodi', 1), ('wirayuda', 2), ('albar', 2), ('solve', 13), ('diplomatically', 2), ('background', 8), ('accidental', 10), ('metropolitan', 3), ('disturbances', 2), ('zazai', 1), ('manhattan', 8), ('morgenthau', 3), ('jennifer', 9), ('marco', 4), ('muniz', 1), ('unaware', 2), ('errors', 8), ('maywand', 1), ('glorifies', 3), ('households', 10), ('tolls', 2), ('mishandling', 3), ('hosada', 1), ('misunderstandings', 2), ('mobbed', 1), ('guangzhou', 10), ('supermarket', 2), ('shenzhen', 3), ('textbooks', 3), ('eviction', 6), ('butts', 1), ('canes', 1), ('openly', 10), ('tabare', 2), ('vazquez', 5), ('uruguayan', 3), ('montevideo', 5), ('jorge', 8), ('oncologist', 1), ('meddles', 1), ('interferes', 1), ('meddle', 2), ('suppresses', 1), ('heydari', 2), ('inevitable', 2), ('shaping', 2), ('nevada', 5), ('checks', 13), ('balances', 2), ('fathers', 1), ('retaliate', 7), ('suggest', 12), ('voicing', 2), ('tours', 4), ('vested', 2), ('factual', 2), ('electronically', 2), ('email', 2), ('transcripts', 6), ('acceptance', 3), ('kashmiris', 3), ('uighurs', 5), ('turkic', 2), ('uighur', 5), ('guise', 3), ('judith', 1), ('latham', 1), ('explores', 2), ('dateline', 1), ('minerals', 11), ('griffin', 3), ('isro', 1), ('madhavan', 1), ('nair', 1), ('tighter', 6), ('applicants', 4), ('chandrayaan', 2), ('array', 1), ('sensors', 2), ('resting', 7), ('salvation', 1), ('sayidat', 1), ('nejat', 1), ('netted', 3), ('bruce', 4), ('golding', 4), ('borrowers', 6), ('structuring', 2), ('iscuande', 2), ('chartered', 8), ('mcguffin', 1), ('maan', 6), ('metric', 14), ('ocampo', 2), ('harun', 1), ('kushayb', 1), ('uzair', 1), ('paracha', 3), ('slip', 3), ('plotted', 3), ('duped', 2), ('nathan', 4), ('solicited', 1), ('reassure', 2), ('mashhadani', 3), ('sulaimaniya', 2), ('irbil', 3), ('foe', 2), ('disagreed', 3), ('aspects', 3), ('seekers', 12), ('dublin', 3), ('frustrated', 6), ('ecolog', 1), ('summon', 1), ('mustaqbal', 1), ('fruitful', 5), ('sessions', 7), ('liuguantun', 1), ('tangshan', 1), ('hebei', 7), ('helland', 1), ('quarterfinals', 11), ('flushing', 3), ('meadows', 1), ('stanilas', 1), ('warwrinka', 1), ('ernest', 3), ('gulbis', 1), ('jul', 10), ('justine', 4), ('henin', 8), ('serena', 3), ('database', 8), ('quadrupled', 2), ('spellings', 1), ('aliases', 2), ('compilation', 1), ('banco', 1), ('championed', 1), ('conditional', 2), ('zabi', 1), ('taifi', 2), ('commuters', 4), ('automobile', 8), ('carmaker', 5), ("gm's", 1), ('conventional', 8), ('combine', 3), ('automakers', 7), ('slump', 9), ('54', 20), ('nanhai', 1), ('baotou', 2), ('mongolia', 15), ('miner', 4), ('yachts', 3), ('bombardier', 1), ('crj', 3), ('commuter', 9), ('robot', 5), ('backpack', 3), ('bakr', 2), ('client', 16), ('intercepting', 2), ('wiretapping', 4), ('wiretaps', 4), ('das', 4), ('wiretapped', 1), ('intercepted', 18), ('semana', 1), ('wrongful', 2), ('pilar', 1), ('hurtado', 2), ('imprisoning', 3), ('assured', 21), ('thugs', 1), ('clubs', 7), ('seizures', 8), ('shengyou', 1), ('roth', 2), ('feigning', 1), ('mandated', 6), ('murderous', 2), ('builders', 2), ('aquatic', 3), ('oda', 1), ('976', 2), ('hok', 1), ('buro', 1), ('happold', 1), ('aquatics', 1), ('gateway', 4), ('476', 1), ('balfour', 1), ('beatty', 1), ('reconfigured', 1), ('chattisgarh', 1), ('peasants', 4), ('landless', 8), ('roots', 4), ('heritage', 10), ('developers', 2), ('malls', 2), ('rocco', 1), ('buttiglione', 1), ('description', 3), ('homosexuality', 4), ('sin', 1), ('primerica', 4), ('valued', 9), ('472', 1), ('delisted', 1), ('duluth', 1), ('ga', 1), ('subsidiaries', 2), ('marketed', 3), ('liabilities', 2), ('fiji', 9), ('endowed', 4), ('fijians', 2), ('harmed', 5), ('author', 10), ('ruler', 16), ('smarter', 2), ('realize', 2), ('adept', 1), ('arrivals', 9), ('dipped', 3), ('landholders', 1), ('jubilee', 1), ('unmasking', 1), ('relied', 4), ('secretive', 4), ('mcc', 2), ('opted', 4), ('hipc', 9), ('benefiting', 3), ('multilateral', 14), ('civic', 3), ('macro', 1), ('violently', 2), ('unrwa', 1), ('regulated', 2), ('flourishes', 2), ('astrology', 1), ('outsiders', 5), ('underestimate', 1), ('handicapped', 1), ('km', 7), ('stream', 10), ('gum', 2), ('astute', 1), ('flesh', 2), ('meat', 24), ('fiercely', 7), ('esteem', 1), ('mortals', 1), ('disguise', 4), ('sculptor', 3), ('statues', 2), ('juno', 3), ('sum', 4), ('certainly', 3), ('statue', 4), ('messenger', 1), ('gods', 2), ('fling', 1), ('journeying', 1), ('tall', 7), ('waited', 8), ('nearer', 2), ('loosen', 1), ('faggot', 1), ('companions', 6), ('wood', 15), ('anticipations', 1), ('outrun', 2), ('realities', 2), ('hot', 13), ('strand', 1), ('pursuer', 1), ('recollecting', 1), ('tumultous', 1), ('hollow', 3), ('heartless', 1), ('rang', 6), ('skipper', 1), ("'t", 1), ('ai', 3), ('convergent', 1), ('sat', 8), ('murmured', 1), ('sadly', 2), ('soul', 8), ('marooned', 1), ('shareman', 1), ('ample', 2), ('hauls', 1), ('pudong', 1), ('screened', 3), ('temperature', 5), ('mistreated', 7), ('deportees', 1), ('deportation', 13), ('qatada', 1), ('retrieve', 2), ('projectiles', 3), ('lerner', 1), ('sufa', 1), ('nahal', 1), ('alzheimer', 3), ('illinois', 16), ('antihistamine', 1), ('spray', 2), ('lifestyle', 2), ('heredity', 1), ('determining', 8), ('batter', 1), ('vero', 1), ('southward', 1), ('ticketing', 1), ('rong', 1), ('diving', 5), ('sellers', 3), ('utilized', 1), ('oversold', 1), ('skidded', 3), ('165', 6), ('divides', 5), ('lobbed', 2), ('blackhawk', 1), ('causalities', 2), ('registration', 15), ('etienne', 1), ('tshisekedi', 1), ('militarily', 2), ('mingled', 2), ('pemex', 7), ('veracruz', 8), ('weakness', 1), ('fertilizer', 14), ('fireball', 2), ('coahuila', 1), ('charai', 1), ('persists', 1), ('bills', 16), ('item', 5), ('wasteful', 2), ('tacked', 1), ('additions', 1), ('earmarks', 1), ('xdr', 4), ('tb', 9), ('gauteng', 1), ('surprises', 1), ('clarification', 4), ('reacted', 10), ('angrily', 4), ('quo', 2), ('manama', 1), ('attitude', 3), ('dialog', 1), ('namibian', 8), ('namibians', 2), ('grimes', 2), ('frederic', 1), ('piry', 1), ('telegram', 3), ('angelo', 2), ('sodano', 2), ('spinal', 4), ('mad', 10), ('cow', 15), ('shinzo', 5), ('abe', 9), ('reimposed', 4), ('veal', 3), ('vladivostok', 2), ('navies', 2), ('kwazulu', 1), ('natal', 2), ('select', 11), ('hajim', 1), ('hassani', 2), ('mento', 1), ('tshabalala', 4), ('msimang', 1), ('uncensored', 3), ('blacked', 1), ('underscoring', 1), ('stoned', 2), ('ghassan', 1), ('daglas', 1), ('torched', 9), ('blazing', 1), ('vary', 4), ('settler', 10), ('retaliating', 1), ('caravan', 1), ('belaroussi', 2), ('wakil', 2), ('muttawakil', 2), ('rank', 7), ('hardcore', 1), ('admitting', 3), ('stanislaw', 2), ('wielgus', 5), ('conscious', 1), ('hayabullah', 1), ('rafiqi', 1), ('collaborated', 3), ('qabail', 1), ('izarra', 2), ('telesur', 5), ('mouthpiece', 3), ('rhetoric', 4), ('scared', 7), ('solders', 1), ('vinci', 3), ('theaters', 7), ('attach', 5), ('disclaimer', 3), ('fiction', 4), ('520', 2), ('repsol', 1), ('britney', 9), ('spears', 8), ('federline', 8), ('lapd', 2), ('norma', 1), ('probed', 2), ('nonspecific', 1), ('uncorroborated', 1), ('eimiller', 2), ('jayden', 2), ('bode', 5), ('countryman', 7), ('daron', 2), ('rahlves', 5), ('hermann', 1), ('maier', 3), ('walchhofer', 6), ('fritz', 3), ('strobl', 2), ('apollo', 1), ('anton', 5), ('ohno', 1), ('skates', 1), ('pechstein', 1), ('anni', 1), ('friesinger', 1), ('stressing', 2), ('armin', 1), ('zoeggeler', 1), ('forensics', 3), ('daud', 2), ('cooperative', 2), ('harmonious', 3), ('resolves', 1), ('strive', 2), ('forgo', 2), ('propose', 9), ('forbidding', 3), ('qala', 11), ('overran', 3), ('appointing', 5), ('super', 13), ('sciri', 1), ('sway', 4), ('echoes', 1), ('supplying', 14), ('norinco', 1), ('zibo', 1), ('chemet', 1), ('aero', 2), ('hongdu', 1), ('ounion', 1), ('limmt', 2), ('metallurgy', 1), ('walt', 1), ('miramax', 2), ('660', 1), ('filmyard', 3), ('holdings', 8), ('nonrefundable', 1), ('deposit', 3), ('tutor', 1), ('pulp', 3), ('shakespeare', 2), ('iger', 1), ('pixar', 1), ('marvel', 1), ('bidders', 2), ('harvey', 1), ('weinstein', 2), ('bloodthirsty', 1), ('beast', 4), ('stanizai', 2), ('hated', 1), ('monster', 1), ('mccellan', 1), ('provocative', 6), ('ronnie', 2), ('ultimatum', 5), ('andry', 2), ('rajoelina', 4), ('najibullah', 2), ('mujahedin', 6), ('mwangura', 2), ('mombasa', 3), ('seafarers', 2), ('container', 5), ('740', 1), ('kismayo', 2), ('receving', 1), ('inconceivable', 2), ('advocated', 4), ('ecuadoreans', 1), ('proven', 9), ('outraged', 6), ('fradkov', 2), ('pills', 3), ('tachilek', 1), ('760', 2), ('proposing', 2), ('textiles', 9), ('byrd', 2), ('sebastien', 2), ('loeb', 7), ('stretching', 4), ('noel', 1), ('choong', 2), ('imb', 1), ('hijack', 6), ('alerted', 7), ('warship', 6), ('melted', 4), ('subaru', 4), ('petter', 3), ('solberg', 3), ('auc', 3), ('plagues', 3), ('demobilization', 4), ('hurriyah', 2), ('ambushes', 8), ('marcus', 2), ('gronholm', 1), ('thirteen', 6), ('lahiya', 3), ('immunizing', 1), ('duaik', 1), ('zahar', 4), ('mitsubishi', 1), ('gigi', 1), ('galli', 1), ('turbo', 1), ('charger', 1), ('malfunction', 2), ('valves', 1), ('guajira', 2), ('connects', 2), ('maracaibo', 1), ('pdvsa', 8), ('haaretz', 6), ('gazan', 1), ('inquest', 2), ('constituted', 1), ('admission', 7), ('guilt', 1), ('stripped', 6), ('bickering', 1), ('monoply', 1), ('plays', 13), ('xp', 1), ('routing', 2), ('mikheil', 5), ('saakashvili', 30), ('reformist', 9), ('lado', 1), ('gurgenidze', 3), ('grigol', 1), ('mgalobishvili', 1), ('markko', 1), ('technocrat', 1), ('banker', 4), ('newhouse', 1), ('barricade', 3), ('bernama', 2), ('asean', 20), ('jabaliya', 3), ('gearing', 4), ('patricia', 2), ('swing', 7), ('advising', 6), ('sezibera', 1), ('massing', 2), ('atenco', 2), ('qassim', 1), ('nuristan', 11), ('tamin', 2), ('nuristani', 2), ('sleeping', 4), ('branco', 1), ('scathing', 1), ('charsadda', 4), ('giliani', 1), ('heinous', 5), ('commerical', 1), ('veneman', 3), ('traumatized', 1), ('untreated', 1), ('adwa', 1), ('underscores', 2), ('wlodzimierz', 1), ('cimoszewicz', 3), ('odzimierz', 1), ('untouched', 2), ('tarnished', 2), ('searchers', 2), ('leftists', 5), ('leopoldo', 1), ('bravo', 5), ('taiana', 1), ('buenos', 8), ('aires', 8), ('parliaments', 2), ('dabaa', 2), ('kam', 4), ('dhiren', 1), ('barot', 2), ('qaisar', 1), ('shaffi', 1), ('nadeem', 1), ('tarmohammed', 1), ('esa', 1), ('hindi', 7), ('citicorp', 1), ('prudential', 6), ('newark', 2), ('subcommittee', 7), ('trucking', 3), ('littered', 3), ('unexploded', 4), ('vigil', 3), ('julie', 2), ('myers', 9), ('oaxaca', 7), ('painted', 4), ('graffiti', 1), ('ulises', 2), ('ruiz', 3), ('resigns', 2), ('elephant', 7), ('elephants', 11), ('culling', 7), ('thin', 5), ('turner', 1), ('durand', 1), ('strikers', 1), ('noses', 1), ('stomachs', 1), ('contacted', 13), ('lauder', 1), ('schneider', 1), ('anxiety', 2), ('instances', 9), ('semitism', 2), ('semitic', 3), ('interfaith', 2), ('inforadio', 1), ('daylam', 2), ('blasting', 5), ('bushehr', 13), ('camilo', 2), ('reyes', 7), ('premeditated', 3), ('strayed', 6), ('oswaldo', 1), ('jarrin', 1), ('razali', 2), ('nyan', 3), ('intelogic', 5), ('trace', 4), ('unaffiliated', 1), ('asher', 2), ('edelman', 4), ('ackerman', 4), ('datapoint', 1), ('maximize', 2), ('marty', 3), ('harvesting', 4), ('finfish', 1), ('krill', 3), ('licenses', 9), ('specialized', 2), ('cruise', 9), ('manpower', 4), ('instabilities', 1), ('purely', 4), ('administrations', 3), ('guiana', 4), ('guadeloupe', 3), ('martinique', 1), ('mayotte', 1), ('reunion', 3), ('overpopulated', 1), ('inefficiently', 1), ('resilient', 6), ('garment', 7), ('totaling', 6), ('fy09', 1), ('fy10', 1), ('fourths', 3), ('pulses', 1), ('sugarcane', 4), ('scope', 9), ('mw', 1), ('hampers', 2), ('susceptibility', 2), ('plank', 2), ('brook', 2), ('beware', 3), ('lest', 2), ('grasping', 1), ('stating', 5), ('idf', 1), ('teach', 11), ('mossad', 3), ('dynamics', 2), ('strelets', 1), ('anatoliy', 1), ('hrytsenko', 2), ('theoretically', 1), ('catering', 2), ('debated', 4), ('signatures', 6), ('symbolically', 2), ('ch', 2), ('chinook', 9), ('tamara', 3), ('lawrence', 7), ('tishrin', 1), ('torrential', 11), ('sincerity', 1), ('254', 4), ('zhouqu', 1), ('gansu', 3), ('nasrallah', 9), ('worsening', 8), ('watchers', 1), ('climbing', 4), ('emotional', 4), ('jammed', 1), ('think', 26), ('disgraced', 10), ('lobbyist', 4), ('abramoff', 11), ('knowing', 4), ('ethics', 9), ('resurgent', 11), ('wrongly', 8), ('harper', 10), ('reprinting', 4), ('depiction', 4), ('haider', 7), ('muttahida', 6), ('quami', 3), ('mqm', 11), ('reprinted', 4), ('foment', 2), ('tashkent', 9), ('revolutions', 2), ('dow', 14), ('jones', 22), ('urdu', 5), ('awami', 8), ('pashtuns', 4), ('termed', 9), ('gymnasium', 1), ('teammate', 4), ('yi', 6), ('jianlian', 1), ('fouled', 1), ('rican', 10), ('narvaez', 1), ('spilled', 7), ('insults', 3), ('locker', 1), ('shielding', 2), ('deplored', 4), ('marchers', 7), ('wore', 1), ('t', 7), ('shirts', 8), ('waved', 8), ("dvd's", 1), ('denis', 6), ('sassou', 5), ('nguesso', 4), ('palma', 3), ('sola', 1), ('chilitepic', 1), ('270', 6), ('baby', 14), ('medan', 1), ('cluster', 8), ('147', 7), ('bekasi', 2), ('dispatch', 6), ('okinawa', 2), ('galle', 4), ('flash', 17), ('lankans', 4), ('tahseen', 1), ('abhorrent', 2), ('poul', 1), ('nielsen', 1), ('caring', 2), ('undecided', 7), ('farabaugh', 1), ('tong', 5), ('reebok', 1), ('vines', 3), ('don', 5), ('alston', 5), ('nord', 1), ('eclair', 1), ('gerais', 2), ('casket', 2), ('gobernador', 1), ('valadares', 1), ('movements', 14), ('lori', 2), ('berenson', 7), ('alejandro', 5), ('toledo', 5), ('tupac', 2), ('amaru', 2), ('airmen', 3), ('emergencies', 3), ('keesler', 2), ('biloxi', 1), ('rotate', 3), ('expletive', 1), ('laughed', 4), ('bashkortostan', 1), ('oskar', 1), ('kaibyshev', 3), ('patented', 2), ('alekseyeva', 1), ('grateful', 5), ('outrageous', 2), ('framed', 2), ('bannu', 4), ('rewriting', 1), ('andrade', 1), ('diaz', 7), ('gereida', 1), ('dishonest', 2), ('lauderdale', 3), ('formalizing', 1), ('reservations', 3), ('cursed', 4), ('odd', 2), ('unlimited', 2), ('totalitarian', 1), ('resembles', 3), ('pinchuk', 1), ('mei', 6), ('guilin', 1), ('wolong', 1), ('andrei', 5), ('nesterenko', 2), ('nestrenko', 1), ('proceeding', 2), ('ashura', 11), ('ritual', 7), ('farsi', 3), ('mir', 15), ('hossein', 14), ('mousavi', 15), ('mehdi', 9), ('karroubi', 4), ('mourino', 2), ('tellez', 2), ('recordings', 4), ('boxes', 6), ('avenue', 3), ('paseo', 1), ('reforma', 1), ('vasconcelos', 1), ('ghulam', 4), ('nadi', 1), ('tahab', 1), ('hizb', 2), ('mujahedeen', 3), ('snarled', 1), ('inception', 1), ('choking', 4), ('congestion', 1), ('briefing', 12), ('steven', 4), ('whitcomb', 2), ('underneath', 3), ('servicemembers', 2), ('hunan', 8), ('conjunction', 1), ('simulated', 1), ('tarin', 1), ('kot', 6), ('neumann', 3), ('phoned', 3), ('speeding', 5), ('purim', 1), ('galloway', 1), ('luther', 8), ('rejects', 14), ('reverend', 7), ('barter', 1), ('disrepair', 1), ('portrays', 3), ('galleries', 2), ('showcasing', 3), ('showcase', 3), ('mort', 3), ('reelection', 4), ('denpasar', 1), ('myuran', 2), ('sukumaran', 2), ('czugaj', 1), ('stephens', 1), ('jalawla', 2), ('renae', 1), ('earliest', 3), ('burial', 9), ('crypt', 3), ('lambasting', 1), ('adoring', 1), ('harshly', 3), ('divisiveness', 1), ('transparent', 10), ('embraced', 2), ('antalya', 2), ('emily', 2), ('henochowicz', 2), ('canister', 1), ('flotilla', 9), ('canisters', 4), ('sparingly', 1), ('bartering', 2), ('catching', 4), ('besides', 6), ('conspirators', 3), ('236', 1), ('quezon', 2), ('emptying', 2), ('domenici', 1), ('reap', 1), ('inspections', 15), ('empowered', 2), ('pulwama', 3), ('katsav', 8), ('vetoed', 1), ('secularists', 5), ('telerate', 6), ('est', 1), ('576', 1), ('tendered', 5), ('barron', 1), ('alluvial', 2), ('namibia', 16), ('gem', 4), ('backyard', 4), ('cereal', 3), ('hides', 2), ('distributions', 1), ('gini', 1), ('coefficient', 1), ('rand', 4), ('allotments', 1), ('1806', 2), ('germanic', 1), ('1815', 4), ('1866', 1), ('shortcomings', 3), ('colonized', 4), ('macau', 18), ('sar', 3), ('practiced', 1), ('evolution', 6), ('1951', 4), ('supranational', 1), ('phenomenon', 3), ('annals', 1), ('dynastic', 3), ('norm', 2), ('cede', 3), ('overarching', 1), ('entity', 6), ('orientation', 3), ('exodus', 2), ('yemenis', 1), ('subdued', 4), ('kooyong', 3), ('delimitation', 1), ('huthi', 1), ('zaydi', 1), ('tentative', 3), ('revitalized', 1), ('socioeconomic', 2), ("sana'a", 1), ('hardened', 2), ('unifying', 2), ('strangle', 2), ('sprain', 1), ('racquetball', 1), ('loosed', 1), ('coil', 1), ('irritated', 1), ('prey', 9), ('poison', 11), ('rustic', 1), ('ignorant', 1), ('aloft', 5), ('goose', 15), ('thereupon', 2), ('induce', 1), ('mused', 1), ('bother', 2), ('noticed', 6), ('ilyushin', 1), ('rosoboronexport', 1), ('izvestia', 3), ('sukhoi', 2), ('ranked', 23), ('nightline', 1), ('balboa', 1), ('detailing', 2), ('carriers', 9), ('embarking', 1), ('232nd', 1), ('reminded', 5), ('risking', 1), ('proud', 6), ('roche', 14), ('juste', 1), ('moradi', 1), ('narrated', 5), ('prolong', 3), ('geoffrey', 2), ('allotted', 2), ('inked', 2), ('avoidance', 1), ('taxation', 7), ('yoshimasa', 1), ('hayashi', 1), ('johndroe', 4), ('praising', 6), ('pacifist', 2), ('hangzhou', 2), ('zhejiang', 7), ('xi', 4), ('jinping', 1), ('goldman', 8), ('sachs', 6), ('yuan', 10), ('undervalued', 1), ('ninevah', 3), ('wipe', 3), ('reinforcement', 2), ('2nd', 1), ('battalion', 4), ('502nd', 1), ('infantry', 6), ('scholarships', 1), ('specter', 1), ('contradicted', 1), ('tolerated', 4), ('nsanje', 1), ('depot', 7), ('expired', 14), ('gah', 7), ('pepfar', 1), ('maroua', 1), ('laborer', 2), ('lobbying', 8), ('modify', 2), ('mutation', 3), ('rania', 1), ('joyous', 1), ('somber', 2), ('ruins', 10), ('eni', 1), ('wintry', 1), ('dictates', 1), ('roza', 6), ('otunbayeva', 7), ('bishkek', 13), ('tenure', 5), ('kurmanbek', 9), ('bakiyev', 17), ('uzbeks', 6), ('intercontinental', 6), ('topol', 8), ('ivanovo', 1), ('teikovo', 1), ('payload', 2), ('countering', 1), ('interceptors', 3), ('radar', 13), ('macarthur', 1), ('bidding', 10), ('parcels', 1), ('fragmented', 2), ('undemocratic', 2), ('fatma', 2), ('zahraa', 1), ('etman', 1), ('camped', 4), ('incentive', 2), ('compel', 1), ('choreographer', 4), ('kidd', 3), ('exuberant', 1), ('broadway', 2), ('guys', 1), ('dolls', 2), ('1954', 5), ('brides', 1), ('choreography', 2), ('chanthalangsy', 1), ('sawang', 1), ('vientiane', 3), ('112', 5), ('hafez', 2), ('cracking', 7), ('frigid', 1), ('dikweneh', 1), ('ablaze', 3), ('ambulances', 1), ('parisian', 2), ('connect', 7), ('paddick', 1), ('russell', 1), ('kerik', 9), ('abruptly', 7), ('nanny', 2), ('madaen', 1), ('marjah', 2), ('thefts', 2), ('complicating', 3), ('intermediaries', 1), ('kuwaitis', 1), ('mutairi', 1), ('representation', 15), ('nobody', 4), ('traced', 4), ('degrading', 4), ('bloemfontein', 1), ('720', 3), ('lieu', 1), ('soup', 5), ('conjured', 1), ('bratislava', 5), ('slovak', 4), ('gasparovic', 1), ('czechoslovakia', 7), ('shed', 2), ('slashing', 9), ('wholesalers', 1), ('swindler', 2), ('madoff', 11), ('162', 6), ('legendary', 6), ('koufax', 1), ('mets', 2), ('wilpon', 1), ('citigroup', 7), ('pyramid', 4), ('causality', 1), ('ghad', 7), ('nour', 13), ('chanting', 15), ('aboul', 5), ('gheit', 7), ('grandchild', 2), ('baladiyat', 1), ('medically', 2), ('shlomo', 1), ('mor', 1), ('hadassah', 5), ('anesthesia', 1), ('dosage', 1), ('sedated', 1), ('unconsciousness', 1), ('hemorrhage', 5), ('function', 5), ('chances', 7), ('481', 2), ('elimination', 3), ('painting', 4), ('farouk', 4), ('vase', 1), ('sutham', 1), ('saengprathum', 1), ('kelantan', 1), ('weighing', 4), ('polytechnic', 1), ('adb', 3), ('backcountry', 1), ('hindus', 4), ('babri', 1), ('birthplace', 4), ('rama', 1), ('shiv', 1), ('sena', 1), ('safer', 6), ('historian', 5), ('irving', 8), ('hitler', 6), ('784', 1), ('atrophy', 4), ('heishan', 1), ('seyoum', 1), ('mesfin', 1), ('guangxi', 2), ('naeem', 2), ('noor', 2), ('awan', 1), ('tanzanian', 3), ('cayenne', 1), ('brace', 2), ('damrey', 5), ('khanun', 1), ('talim', 1), ('dheere', 3), ('disfigured', 1), ('wins', 15), ('guarding', 8), ('mats', 1), ('talal', 1), ('professor', 15), ('sattar', 1), ('qassem', 2), ('suha', 1), ('kidwa', 6), ('asks', 6), ('dordain', 1), ('updates', 5), ('payloads', 2), ('exomars', 2), ('capped', 4), ('260', 5), ('slick', 13), ('shoigu', 1), ('poured', 9), ('benzene', 11), ('poisons', 3), ('songhua', 9), ('flowed', 2), ('harbin', 10), ('meteorological', 9), ('foul', 1), ('diluted', 3), ('interruption', 1), ('outed', 1), ('wilson', 15), ('sad', 1), ('revelation', 1), ('underscored', 3), ('defiled', 1), ('commended', 4), ('diligence', 1), ('advisory', 10), ('adopting', 2), ('resolutely', 3), ('consultative', 2), ('scrapping', 2), ('choco', 1), ('exploratory', 3), ('euros', 5), ('wines', 1), ('00e', 1), ('dreamliner', 3), ('globovision', 2), ('zuloaga', 4), ('nippon', 3), ('airasia', 1), ('hamdania', 3), ('pendleton', 2), ('assaults', 12), ('trigger', 7), ('gomhouria', 1), ('trumped', 2), ('ralia', 1), ('thrived', 1), ('mhz', 2), ('frequency', 5), ('1030', 1), ('khz', 5), ('cranks', 1), ('helpful', 6), ('thayer', 1), ('kadhim', 1), ('abid', 2), ('suraiwi', 2), ('chained', 2), ('defacate', 1), ('allege', 14), ('finely', 1), ('orbiter', 3), ('armchairs', 1), ('punitive', 1), ('orbit', 8), ('theorize', 1), ('harassing', 3), ('petro', 3), ('verbytsky', 1), ('crimean', 5), ('succumbed', 3), ('embankment', 2), ('sandbag', 1), ('reinforced', 5), ('levee', 7), ('winfield', 1), ('peyton', 4), ('inundate', 1), ('embankments', 1), ('ruining', 1), ('soybeans', 1), ('skyrocketed', 1), ('royalists', 1), ('sahafi', 1), ('onboard', 4), ('stadiums', 9), ('abundance', 3), ('mentions', 2), ('nfl', 6), ('oakland', 3), ('involve', 10), ('equus', 4), ('liquidation', 1), ('preference', 3), ('paso', 5), ('boots', 2), ('accrue', 2), ('dividends', 1), ('redeemed', 1), ('1652', 1), ('spice', 1), ('boers', 3), ('trekked', 1), ('1886', 2), ('subjugation', 1), ('encroachments', 1), ('boer', 1), ('1899', 2), ('afrikaners', 1), ('1910', 6), ('anc', 10), ('nelson', 9), ('mandela', 15), ('boycotts', 2), ('ushered', 12), ('imbalances', 5), ('decent', 5), ('kgalema', 1), ('motlanthe', 1), ('santos', 8), ('locomotives', 3), ('promarket', 1), ('underemployment', 5), ('narcotrafficking', 1), ('exporters', 8), ('oceans', 8), ('delimited', 1), ('waterways', 4), ('thinned', 2), ('dukedom', 2), ('constitutionally', 3), ('1603', 2), ('tokugawa', 1), ('shogunate', 1), ('flowering', 1), ('kanagawa', 1), ('1854', 2), ('intensively', 3), ('modernize', 2), ('industrialize', 1), ('formosa', 1), ('sakhalin', 2), ('1931', 1), ('manchuria', 1), ('1937', 6), ('honshu', 1), ('saibou', 1), ('standstill', 3), ('col', 6), ('bare', 4), ('madhuri', 1), ('gupta', 2), ('duration', 1), ('minimal', 4), ('agrarian', 4), ('sahel', 1), ('tuareg', 5), ('nigerien', 1), ('growling', 1), ('snapping', 1), ('oxen', 4), ('hay', 9), ('selfish', 3), ('wrathful', 1), ('tyrannical', 1), ('gentle', 1), ('reign', 3), ('wolf', 23), ('lamb', 4), ('panther', 1), ('amity', 1), ('oh', 4), ('longed', 2), ('shall', 6), ('swooped', 3), ('devouring', 2), ('coils', 1), ('enabling', 4), ('spat', 1), ('exertions', 2), ('slake', 1), ('thirst', 4), ('draught', 1), ('deserves', 3), ('juror', 1), ('certificate', 4), ('afflicted', 3), ('softening', 4), ('gentleman', 2), ('excused', 2), ('housecat', 1), ('enlist', 2), ('furred', 1), ('aswan', 2), ('setback', 9), ('abul', 2), ('appreciates', 2), ('pretext', 4), ('unimaginably', 1), ('armistice', 6), ('denunciations', 2), ('lurking', 1), ('yitzhak', 1), ('rabin', 2), ('leah', 1), ('paint', 6), ('theodore', 2), ('herzl', 1), ('defaced', 1), ('neo', 2), ('hail', 5), ('beilin', 1), ('gurion', 1), ('graveyard', 1), ('vandalism', 4), ('rooting', 7), ('sadah', 1), ('newlands', 2), ('shivnarine', 1), ('chanderpaul', 2), ('samuels', 3), ('crease', 3), ('297', 2), ('214', 1), ('makhaya', 1), ('ntini', 2), ('dale', 4), ('steyn', 3), ('wickets', 14), ('durban', 5), ('sung', 8), ('www', 2), ('gov', 2), ('cn', 1), ('bucca', 1), ('biographies', 1), ('buyers', 7), ('reassured', 4), ('aig', 7), ('mv', 9), ('towed', 3), ('miscommunication', 1), ('offloaded', 1), ('tighten', 14), ('crewmembers', 3), ('cetacean', 1), ('acid', 6), ('boarding', 6), ('antarctica', 12), ('forbid', 4), ('gonzales', 22), ('cabeza', 1), ('vaca', 1), ('prompt', 6), ('photographed', 6), ('publications', 7), ('livingstone', 2), ('shoko', 1), ('lusaka', 2), ('cooked', 7), ('improperly', 5), ('unconditionally', 3), ('mediated', 7), ('muallem', 1), ('sheiria', 1), ('retake', 3), ('effigies', 2), ('qualified', 11), ('experiences', 3), ('baluch', 9), ('warmer', 5), ('humidity', 2), ('transmitting', 2), ('interrupt', 3), ('jailing', 3), ('riad', 1), ('seif', 2), ('faulting', 2), ('energetic', 2), ('tommy', 8), ('franks', 3), ('menem', 6), ('thales', 2), ('spectrum', 5), ('dovonou', 1), ('cotonou', 2), ('adjarra', 1), ('porto', 1), ('novo', 2), ('7th', 2), ('precautionary', 2), ('infecting', 4), ('wiesenthal', 7), ('scheussel', 1), ('tributes', 2), ('tireless', 3), ('immunity', 8), ('adolf', 3), ('eichmann', 2), ('bombardment', 5), ('pelting', 1), ('eggs', 17), ('incapacitating', 1), ('azhar', 6), ('haifa', 5), ('poors', 1), ('shiller', 2), ('subprime', 2), ('homeowners', 13), ('shrinking', 5), ('detroit', 9), ('solving', 2), ('paused', 1), ('usaid', 4), ('oxfam', 10), ('grenoble', 2), ('streetcar', 1), ('monde', 5), ('robber', 2), ('longwang', 4), ('swirling', 2), ('pounding', 5), ('valenzuela', 3), ('departs', 1), ('tecnica', 1), ('loja', 1), ('universidad', 1), ('andes', 1), ('cartagena', 4), ('kleinkirchheim', 2), ('franz', 3), ('klammer', 2), ('068472222', 1), ('nike', 1), ('michaela', 2), ('dorfmeister', 3), ('downhills', 1), ('finishes', 2), ('882', 1), ('anja', 2), ('paerson', 4), ('differ', 4), ('592', 1), ('khristenko', 2), ('versions', 3), ('panetta', 2), ('obeidi', 3), ('catch', 16), ('bribe', 3), ('installment', 4), ('evacuees', 9), ('presses', 1), ('lembe', 2), ('bukavu', 2), ('kenyon', 2), ('jensen', 1), ('methods', 13), ('phuket', 1), ('downplaying', 3), ('tornadoes', 2), ('tornado', 3), ('yazoo', 1), ('haley', 3), ('barbour', 3), ('counties', 3), ('incomplete', 1), ('reburied', 2), ('anup', 1), ('raj', 1), ('sharma', 4), ('requiring', 8), ('flush', 9), ('abdulkadir', 5), ('ngos', 6), ('jabril', 1), ('abdulle', 1), ('dar', 6), ('es', 5), ('salaam', 5), ('malindi', 3), ('mauritian', 1), ('rodrigues', 2), ('scares', 2), ('grouper', 1), ('malachite', 2), ('determines', 2), ('eels', 1), ('chaka', 1), ('fattah', 1), ('fills', 1), ('rhode', 2), ('vermont', 3), ('gunfights', 1), ('copacabana', 1), ('ipanema', 1), ('legalize', 1), ('tai', 2), ('shen', 1), ('kuo', 3), ('gregg', 1), ('bergersen', 3), ('jawid', 1), ('qiang', 2), ('wei', 6), ('unexpected', 7), ('reshuffle', 8), ('tichaona', 1), ('jokonya', 5), ('moyo', 7), ('traitors', 1), ('katzenellenbogen', 2), ('withered', 1), ('dongzhou', 3), ('escorting', 4), ('reckless', 4), ('helpe', 1), ('monument', 14), ('savannah', 3), ('erect', 2), ('chasseurs', 1), ('volontaires', 1), ('domingue', 1), ('regiment', 5), ('1779', 1), ('tremendous', 5), ('debut', 7), ('georgetown', 3), ('beta', 10), ('churns', 3), ('sofia', 4), ('turb', 1), ('ulent', 1), ('osce', 5), ('passy', 1), ('surpass', 2), ('2035', 1), ('albert', 4), ('keidel', 2), ('regardless', 5), ('carnegie', 2), ('endowment', 2), ('negligence', 8), ('tampering', 6), ('sahrawi', 1), ('coordinating', 7), ('casablanca', 5), ('bart', 1), ('stupak', 1), ('gauging', 2), ('pricing', 6), ('camila', 2), ('guerra', 2), ('grammyawards', 1), ('las', 7), ('vegas', 3), ('comprised', 4), ('mario', 6), ('domm', 1), ('samo', 1), ('parra', 1), ('mientes', 1), ('dejarte', 1), ('amar', 1), ('grammys', 4), ('guerraas', 1), ('bachata', 1), ('fukuoko', 1), ('univision', 1), ('hilary', 7), ('duff', 3), ('judgmental', 1), ('incessant', 2), ('starlet', 1), ('realizes', 3), ('dignity', 4), ('girlfriend', 9), ('richie', 1), ('gypsy', 2), ('deter', 11), ('logs', 2), ('mindful', 3), ('councilor', 1), ('jiaxuan', 1), ('orderly', 4), ('lehman', 1), ('ubs', 1), ('issuing', 5), ('stearns', 1), ('renowed', 1), ('farka', 1), ('toure', 9), ('guitar', 4), ('genre', 1), ('sounds', 3), ('praise', 11), ('legend', 8), ('ry', 2), ('cooder', 2), ('timbuktu', 1), ('grammy', 7), ('beloved', 2), ('belet', 1), ('weyn', 1), ('clans', 5), ('anarchy', 3), ('brick', 2), ('rooms', 7), ('walls', 14), ('tasks', 3), ('dextre', 3), ('destiny', 2), ('endeavour', 4), ('compartment', 1), ('latifiya', 1), ('hafidh', 1), ('closes', 3), ('salvadoran', 4), ('deferring', 1), ('disband', 2), ('amani', 1), ('wreaking', 1), ('havoc', 3), ('lanes', 2), ('laissez', 1), ('faire', 1), ('archaic', 1), ('intellectual', 4), ('entrepot', 1), ('rebuilt', 4), ('ballooning', 1), ('rafiq', 4), ('reining', 3), ('expenditures', 8), ('enterprises', 11), ('receipt', 7), ('conditioned', 1), ('mayen', 1), ('exploitable', 4), ('bbl', 2), ('manigat', 3), ('jonas', 2), ('savimbi', 3), ('accrued', 1), ('arrears', 2), ('peg', 4), ('angolan', 5), ('kwanza', 1), ('depreciated', 1), ('possesses', 3), ('fishery', 1), ('imbalance', 8), ('revival', 3), ('rutile', 1), ('protectorates', 1), ('1942', 3), ('baker', 6), ('malaya', 1), ('1957', 4), ('sarawak', 1), ('borneo', 2), ('mahathir', 9), ('najib', 9), ('razak', 3), ('wanderings', 2), ('armourer', 1), ('glided', 2), ('pricked', 2), ('dart', 1), ('fangs', 1), ('wrath', 4), ('useless', 2), ('insensible', 1), ('salesmen', 1), ('dina', 1), ('cody', 1), ('slumps', 1), ('troubles', 7), ('bamako', 3), ('hammered', 4), ('min', 2), ('oceania', 1), ('ashr', 2), ('jem', 3), ('khalil', 3), ('incidences', 1), ('forcible', 1), ('funerals', 6), ('interpol', 12), ('notices', 3), ('mabhouh', 1), ('circulate', 1), ('deyda', 1), ('hydara', 2), ('indirectly', 5), ('berezovsky', 1), ('akhmed', 1), ('zakayev', 1), ('greenest', 1), ('soothe', 1), ('zelaya', 12), ('tsang', 5), ('h9n2', 1), ('subtypes', 1), ('hoax', 6), ('martinez', 2), ('wardak', 8), ('warren', 3), ('buffett', 3), ('micheletti', 4), ('intent', 5), ('mansehra', 1), ('balakot', 2), ('reclusive', 4), ('frees', 2), ('sort', 6), ('emerges', 1), ('jade', 2), ('lots', 1), ('nargis', 6), ('rubies', 1), ('auctions', 1), ('earner', 4), ('jewelry', 6), ('paulo', 10), ('nishin', 1), ('seemed', 7), ('poised', 6), ('ma', 10), ('ying', 2), ('jeou', 2), ('hsieh', 3), ('jeopardy', 2), ('impeachment', 12), ('legality', 6), ('andras', 3), ('batiz', 3), ('ruegen', 5), ('012', 2), ('maharastra', 2), ('guirassy', 2), ('asphyxiated', 1), ('salvatore', 1), ('sagues', 1), ('senegalese', 9), ('prosecutions', 1), ('thorough', 6), ('rattled', 3), ('kandill', 1), ('karliova', 2), ('bingol', 3), ('atop', 9), ('tectonic', 2), ('faultline', 1), ('mercenaries', 2), ('chenembiri', 1), ('bhunu', 1), ('ranged', 1), ('teodoro', 2), ('obiang', 1), ('nguema', 1), ('unspoiled', 1), ('maciej', 1), ('nowicki', 1), ('baltica', 2), ('pillars', 2), ('rospuda', 1), ('pristine', 1), ('peat', 2), ('bog', 1), ('eagles', 3), ('wolves', 2), ('lynx', 1), ('augustow', 1), ('abductees', 5), ('nadia', 3), ('fayoum', 1), ('michalak', 1), ('thuan', 1), ('clouded', 1), ('harass', 1), ('venues', 3), ('theo', 4), ('bouyeri', 4), ('hofstad', 1), ('nansan', 1), ('kokang', 3), ('brader', 1), ('levin', 3), ('sates', 2), ('soothing', 1), ('normalization', 4), ('teng', 1), ('hui', 2), ('sighted', 4), ('medecins', 4), ('sans', 3), ('frontieres', 3), ('perpetrating', 1), ('cohesion', 2), ('labado', 3), ('bihar', 4), ('erkki', 1), ('tuomioja', 1), ('influx', 3), ('insulza', 3), ('derbez', 4), ('eighteen', 4), ('oas', 9), ('recessed', 3), ('stored', 9), ('haisori', 1), ('destabilization', 1), ('rugigana', 1), ('ngabo', 3), ('faustin', 1), ('kayumba', 1), ('nyamwasa', 4), ('ottawa', 5), ('forbes', 7), ('benn', 4), ('develops', 1), ('izzat', 1), ('douri', 1), ('hasina', 3), ('inhuman', 3), ('dock', 4), ('ration', 1), ('cosmonaut', 1), ('salizhan', 2), ('sharipov', 2), ('leroy', 2), ('chiao', 2), ('professors', 3), ('scorpions', 4), ('males', 6), ('branko', 2), ('grujic', 1), ('octopus', 3), ('decadence', 1), ('decay', 2), ('psychic', 2), ('aspire', 1), ('perfection', 1), ('oberhausen', 1), ('legged', 1), ('creature', 3), ('mussels', 1), ('archaeologist', 6), ('susanne', 5), ('osthoff', 6), ('peacemaker', 1), ('reconstruct', 4), ('parks', 4), ('ideas', 9), ('reconstructing', 1), ('prone', 9), ('counternarcotics', 3), ('shaanxi', 6), ('wayaobao', 1), ('zichang', 1), ('expeditions', 3), ('howells', 1), ('277', 1), ('automatically', 8), ('kantathi', 1), ('supamongkhon', 1), ('kimchi', 2), ('spicy', 1), ('garlicky', 1), ('cabbage', 2), ('dish', 2), ('epitomizes', 1), ('zero', 11), ('achin', 2), ('alzouma', 1), ('yada', 1), ('adamou', 1), ('cuvette', 1), ('hemorrhagic', 6), ('bleeding', 7), ('heath', 1), ('anura', 1), ('bandaranaike', 1), ('sarath', 2), ('amunugama', 1), ('portfolios', 2), ('mediocre', 1), ('peril', 1), ('essay', 2), ('isaiah', 1), ('planner', 4), ('toppling', 3), ('unpublished', 1), ('memoir', 4), ('authored', 1), ('294', 1), ('surpasses', 1), ('2927', 1), ('firecrackers', 3), ('rear', 2), ('downward', 11), ('polarize', 1), ('symbolic', 4), ('renault', 6), ('interlagos', 1), ('060092593', 1), ('clocked', 2), ('mclaren', 2), ('mercedes', 2), ('wurz', 2), ('050474537', 1), ('kimi', 1), ('raikkonen', 2), ('193', 4), ('trails', 2), ('realistically', 1), ('finish', 21), ('ferrari', 6), ('schumacher', 1), ('falcon', 2), ('quails', 1), ('intrusion', 6), ('heels', 7), ('drifted', 2), ('prolific', 1), ('hispaniola', 4), ('bore', 4), ('frayed', 2), ('shunned', 2), ('summits', 4), ('jovic', 3), ('functional', 1), ('joao', 1), ('vieira', 3), ('billboard', 1), ('vocals', 1), ('larry', 4), ('lamm', 1), ('trumpet', 2), ('loughnane', 1), ('daya', 2), ('sandagiri', 1), ('nino', 2), ('malam', 1), ('bacai', 1), ('sanha', 2), ('bandung', 2), ('toddler', 1), ('698', 2), ('qinghai', 9), ('yushu', 1), ('guangrong', 1), ('thoughts', 5), ('kamau', 1), ('pistols', 3), ('luggage', 3), ('artur', 1), ('trafficker', 3), ('salinas', 2), ('edgar', 2), ('marina', 1), ('otalora', 1), ('finances', 9), ('norte', 2), ('contrasted', 2), ('condoleeza', 1), ('ludicrous', 1), ('campo', 2), ('raiding', 2), ('barricaded', 1), ('shangla', 1), ('sarfraz', 1), ('naeemi', 1), ('dera', 12), ('dd', 4), ('unicorp', 2), ('kingsbridge', 1), ('cara', 2), ("dunkin'", 4), ('donuts', 1), ('pill', 8), ('delaware', 6), ('dunkin', 1), ('468', 1), ('randolph', 1), ('relax', 3), ('wishing', 11), ('defection', 2), ('virtually', 11), ('fiber', 3), ('casinos', 1), ('cepa', 1), ('pataca', 1), ('palm', 4), ('profited', 2), ('petronas', 2), ('riskier', 1), ('decreasing', 4), ('revisions', 1), ('malays', 2), ('polynesian', 5), ('1889', 1), ('1925', 2), ('586', 1), ('euphausia', 1), ('superba', 1), ('027', 1), ('patagonian', 3), ('toothfish', 3), ('dissostichus', 1), ('eleginoides', 1), ('bass', 1), ('910', 1), ('591', 1), ('396', 1), ('ccamlr', 1), ('unregulated', 1), ('376', 2), ('213', 3), ('552', 2), ('799', 1), ('operators', 8), ('iaato', 1), ('overflights', 3), ('guarantor', 2), ('sideline', 1), ('uniosil', 1), ('furthering', 1), ('stamping', 2), ('1936', 4), ('franco', 4), ('dynamic', 5), ('fatherland', 1), ('wintertime', 1), ('curled', 1), ('tee', 1), ('kites', 3), ('olden', 1), ('neigh', 2), ('enchanted', 1), ('imitate', 1), ('writers', 5), ('brilliant', 2), ('indolent', 2), ('dull', 1), ('industrious', 1), ('seventy', 4), ('poetry', 1), ('honoured', 1), ('compiler', 1), ('sixteen', 4), ('volumes', 1), ('tabulated', 1), ('hog', 2), ('cocks', 2), ('skulked', 2), ('crowed', 2), ('lustily', 1), ('hawk', 10), ('behold', 1), ('goeth', 1), ('boasting', 2), ('vanquished', 3), ('cock', 3), ('calamitously', 1), ('prowl', 1), ('dwell', 1), ('whichever', 1), ('quarrelling', 1), ('corner', 4), ('138', 3), ('pep', 1), ('marjayoun', 2), ('tzipi', 4), ('livni', 10), ('redirect', 1), ('groomed', 1), ('spam', 5), ('sim', 1), ('unicom', 1), ('telecom', 3), ('somewhere', 9), ('gallon', 4), ('declarations', 2), ('628', 1), ('westerly', 1), ('kewell', 3), ('animated', 4), ('referee', 4), ('aussies', 3), ('markus', 2), ('siegler', 1), ('liverpool', 6), ('inconsistent', 3), ('merk', 3), ('insulted', 2), ('fouls', 1), ('stuff', 3), ('nrc', 1), ('handelsblad', 1), ('henk', 1), ('morsink', 2), ('thank', 5), ('forested', 1), ('hyderabad', 5), ('zedong', 3), ('akihito', 5), ('empress', 3), ('michiko', 2), ('lays', 1), ('saipan', 2), ('1944', 5), ('mudslide', 8), ('fading', 2), ('slide', 8), ('reservoir', 5), ('shanxi', 6), ('torrent', 2), ('plowed', 3), ('bassist', 2), ('orlando', 1), ('cachaito', 1), ('buena', 1), ('vista', 2), ('prostate', 3), ('glory', 5), ('acclaim', 2), ('singers', 4), ('compay', 1), ('segundo', 1), ('ruben', 2), ('gonzalez', 2), ('bright', 3), ('dai', 3), ('chopan', 3), ('manhunt', 6), ('nurja', 1), ('exaggerated', 3), ('memin', 2), ('pinguin', 2), ('depicts', 3), ('lips', 4), ('jesse', 3), ('racist', 3), ('contribution', 12), ('purchases', 8), ('sa', 4), ('sooner', 6), ('armitage', 7), ('exxonmobil', 8), ('conocophillips', 5), ('nationalize', 12), ('unwanted', 4), ('reality', 9), ('euthanized', 1), ('pets', 1), ('immensely', 2), ('expertise', 4), ('clinics', 5), ('dwyer', 6), ('ruth', 3), ('turk', 5), ('cares', 1), ('grief', 5), ('offended', 2), ('knows', 8), ('demeanor', 2), ('hindawi', 1), ('duluiya', 1), ('conservationists', 2), ('machinea', 1), ('projected', 10), ('guji', 2), ('borena', 2), ('shakiso', 2), ('arero', 2), ('yabello', 2), ('jaatanni', 1), ('taadhii', 1), ('sided', 5), ('virunga', 2), ('scooter', 3), ('segway', 2), ('upright', 1), ('gyroscopes', 1), ('tricky', 1), ('scooters', 1), ('eavesdropping', 5), ('warrantless', 1), ('boundaries', 7), ('minster', 8), ('lowers', 2), ('mascots', 1), ('jing', 4), ('poaching', 3), ('bunia', 3), ('floribert', 1), ('ndjabu', 2), ('integrationist', 1), ('precedence', 1), ('guatemalan', 4), ('rigoberta', 1), ('menchu', 1), ('rulings', 5), ('miroslav', 4), ('bralo', 3), ('jokers', 1), ('forcers', 1), ('nastase', 4), ('contradicting', 1), ('dwain', 2), ('lifetime', 7), ('cheats', 1), ('dhia', 1), ('najim', 3), ('freelance', 2), ('mironov', 3), ('rodina', 2), ('pensioners', 4), ('maneuver', 4), ('assimilating', 1), ('visually', 1), ('impaired', 2), ('blind', 6), ('ancic', 5), ('ordina', 1), ('den', 1), ('bosch', 4), ('taping', 1), ('llodra', 2), ('klara', 1), ('koukalova', 2), ('countrywoman', 2), ('macedonian', 10), ('ljube', 1), ('boskovski', 4), ('ljubotno', 1), ('skopje', 1), ('johan', 2), ('tarulovski', 2), ('foodstuffs', 2), ('malcolm', 2), ('reception', 2), ('radisson', 1), ('confession', 8), ('tung', 17), ('chee', 4), ('hwa', 4), ('cppcc', 3), ('useful', 3), ('retaliatory', 3), ('deploys', 2), ('categorically', 3), ('militarization', 1), ('telecast', 1), ('gulbuddin', 3), ('hekmatyar', 5), ('islami', 4), ('weaponization', 1), ('achievement', 7), ('conferring', 2), ('singnaghi', 1), ('striving', 2), ('aspirations', 6), ('tugboats', 1), ('mavi', 1), ('marmaraout', 1), ('commandeered', 3), ('ashdod', 2), ('emmanuel', 5), ('akitani', 5), ('neuilly', 1), ('gilchrist', 2), ('olympio', 2), ('andiwal', 2), ('marja', 1), ('jalani', 1), ('apologizes', 2), ('levinson', 2), ('kish', 1), ('locating', 2), ('duffle', 1), ('218', 4), ('243', 2), ('ashwell', 1), ('131', 3), ('overs', 9), ('bowler', 9), ('dwayne', 1), ('jerome', 1), ('centurion', 2), ('germ', 1), ('anthrax', 2), ('rihab', 1), ('huda', 1), ('saleh', 12), ('amash', 1), ('marwa', 1), ('schultz', 3), ('demonstrates', 3), ('conventions', 4), ('brookings', 1), ('impacts', 2), ('camaraderie', 1), ('outdoors', 1), ('monaliza', 1), ('noormohammadi', 1), ('endangers', 3), ('porous', 1), ('husaybah', 5), ('320', 5), ('thunderous', 1), ('arish', 3), ('dynamite', 2), ('ashkelon', 4), ('sderot', 2), ('uthmani', 1), ('funnel', 1), ('southerners', 2), ('opera', 8), ('luciano', 1), ('pavarotti', 3), ('modena', 1), ('polyclinic', 1), ('pancreatic', 1), ('aficionados', 1), ('tenor', 1), ('celebrity', 6), ('cutoff', 2), ('beattie', 4), ('boiled', 2), ('reassuring', 2), ('wang', 5), ('minghe', 1), ('upstream', 3), ('mohamud', 5), ('salad', 1), ('nur', 4), ('adaado', 3), ('posting', 5), ('sixty', 5), ('crooner', 1), ('talent', 2), ('shrugged', 1), ('suggestion', 8), ('suichuan', 1), ('jiangxi', 3), ('mala', 1), ('shorish', 1), ('akre', 1), ('bijeel', 1), ('shaikan', 1), ('rovi', 1), ('sarta', 1), ('dihok', 1), ('measuring', 5), ('aegean', 4), ('quakes', 2), ('chimneys', 1), ('embarrased', 1), ('halftime', 1), ('performer', 1), ('jacksonville', 1), ('janet', 6), ('timberlake', 4), ('mccarthy', 1), ('facets', 1), ('costume', 3), ('sporting', 6), ('contenders', 1), ('baya', 1), ('beiji', 2), ('paulos', 2), ('faraj', 2), ('rahho', 1), ('nineveh', 2), ('blackmailed', 2), ('archeologist', 1), ('energize', 1), ('verifiably', 1), ('catherine', 7), ('baber', 2), ('mike', 16), ('mcdaniel', 2), ('floodwaters', 13), ('spills', 6), ('ecosystems', 2), ('pontchartrain', 1), ('megumi', 1), ('yokota', 2), ('faking', 1), ('mocks', 1), ('convene', 3), ('alfonso', 2), ('duarte', 3), ('340', 2), ('logos', 2), ('223', 3), ('ripping', 4), ('mobilize', 7), ('hamesh', 1), ('koreb', 1), ('trawler', 1), ('detonators', 2), ('hmawbi', 1), ('township', 4), ('joyful', 5), ('gracious', 1), ('faiths', 7), ('bijbehera', 1), ('amor', 1), ('almagro', 1), ('newmont', 3), ('buyat', 1), ('arsenic', 2), ('ghazi', 5), ('aridi', 1), ('bugojno', 1), ('diver', 2), ('hamadi', 4), ('parole', 5), ('focal', 3), ('twa', 1), ('stethem', 1), ('lovers', 1), ('410', 1), ('guinness', 1), ('yerevan', 1), ('candy', 3), ('587', 1), ('elah', 1), ('dufour', 1), ('novi', 1), ('alessandria', 1), ('piemonte', 1), ('savin', 3), ('cent', 3), ('stamford', 1), ('conn', 2), ('magnified', 1), ('nonrecurring', 1), ('valuation', 1), ('adjustments', 2), ('segments', 4), ('principally', 4), ('geodynamic', 1), ('kos', 1), ('astypaleia', 1), ('excels', 1), ('sufficiency', 1), ('contractions', 1), ('stimulated', 2), ('surpluses', 3), ('adjustment', 4), ('cambodians', 6), ('khmers', 1), ('descendants', 7), ('angkor', 1), ('cham', 1), ('ushering', 2), ('1863', 6), ('indochina', 2), ('1887', 1), ('phnom', 5), ('penh', 5), ('hardships', 2), ('pol', 3), ('pot', 1), ('semblance', 2), ('normalcy', 1), ('contending', 1), ('norodom', 2), ('sihanouk', 1), ('sihamoni', 1), ('aboriginal', 2), ('1770', 2), ('capt', 2), ('alam', 4), ('qom', 2), ('ageing', 1), ('amounted', 3), ('fy06', 1), ('earns', 1), ('ascension', 2), ('falklands', 3), ('dhekelia', 4), ('penning', 1), ('fold', 4), ('perceiving', 2), ('tortoise', 10), ('antagonist', 1), ('leisurely', 1), ('sauntering', 1), ('wayside', 3), ('fatigue', 4), ('cheer', 1), ('shame', 3), ('schemed', 1), ('foxes', 3), ('tailless', 1), ('deprivation', 2), ('brush', 1), ('interrupting', 4), ('spider', 3), ('spun', 1), ('thriller', 2), ('grossing', 2), ('shahab', 1), ('tallies', 3), ('entries', 3), ('greg', 3), ('schulte', 3), ('pedophile', 4), ('ideological', 2), ('easter', 12), ('gossip', 2), ('colom', 6), ('hamilton', 2), ('salma', 1), ('hayek', 2), ('valentina', 1), ('paloma', 1), ('pinault', 3), ('henri', 4), ('ugly', 3), ('ventanazul', 1), ('metro', 6), ('goldwyn', 1), ('ppr', 1), ('labels', 1), ('gucci', 1), ('balenciaga', 1), ('puma', 1), ('divorce', 6), ('dismissing', 3), ('159th', 1), ('paynesville', 1), ('congotown', 1), ('compatible', 2), ('volt', 2), ('needlessly', 1), ('antagonizes', 1), ('myth', 5), ('uncalculated', 1), ('melih', 1), ('gokcek', 1), ('nuns', 5), ('drapchi', 1), ('16s', 2), ('oscar', 5), ('arias', 4), ('manual', 2), ('otton', 1), ('cafta', 10), ('comprises', 8), ('statute', 3), ('backdrop', 4), ('351', 2), ('187', 1), ('breeding', 3), ('heraldo', 1), ('munoz', 1), ('manipur', 2), ('concealed', 4), ('imphal', 1), ('okram', 1), ('ibobi', 1), ('282', 1), ('hussey', 1), ('symonds', 5), ('lbw', 1), ('yousef', 3), ('phrase', 3), ('typically', 4), ('defenseless', 2), ('wrestler', 4), ('luo', 3), ('meng', 1), ('diuretic', 1), ('purge', 4), ('hua', 1), ('offenders', 7), ('michele', 5), ('alliot', 6), ('athlete', 2), ('swimmer', 1), ('ouyang', 1), ('kunpeng', 1), ('steroid', 4), ('281', 1), ('gayle', 2), ('paced', 1), ('pitch', 3), ('batsman', 5), ('fours', 6), ('267', 1), ('internationals', 2), ('authorites', 1), ('hilla', 8), ('occured', 2), ('outlaws', 1), ('bulava', 1), ('mutates', 3), ('penetrate', 2), ('stavropol', 1), ('rogge', 5), ('delanoe', 1), ('sebastian', 5), ('coe', 1), ('balkenende', 2), ('suite', 1), ('audiences', 12), ('yimou', 1), ('colorful', 6), ('dragons', 2), ('extravagant', 3), ('theme', 6), ('civilization', 3), ('torchbearer', 2), ('cauldron', 4), ('constructively', 2), ('jowhar', 2), ('perina', 1), ('slim', 6), ('simultaneously', 4), ('mohsen', 8), ('lowering', 5), ('seems', 7), ('spaniards', 7), ('artibonite', 1), ('medusa', 2), ('refocus', 1), ('azimbek', 1), ('beknazarov', 1), ('nepotism', 1), ('maksim', 2), ('rewards', 7), ('awakening', 2), ('adolescence', 1), ('sexuality', 2), ('examines', 2), ('adolescents', 1), ('zheng', 2), ('mok', 3), ('elaine', 7), ('advertisements', 5), ('ads', 2), ('reciprocate', 1), ('ad', 5), ('lipsky', 3), ('tower', 6), ('verifiable', 1), ('denuclearization', 2), ('donkey', 2), ('unanswered', 2), ('pouring', 4), ('pumps', 3), ('locate', 10), ('yichang', 1), ('hubei', 6), ('wanzhou', 1), ('downpours', 1), ('abkhaz', 6), ('mujhava', 1), ('outspoken', 8), ('chlorine', 4), ('commonplace', 1), ('reshape', 1), ('broadening', 1), ('ojedokun', 1), ('screeners', 1), ('baggage', 1), ('glut', 1), ('unsold', 1), ('ulf', 2), ('henricsson', 2), ('giovanni', 1), ('claudio', 2), ('fava', 1), ('lawmakes', 1), ('porter', 6), ('goss', 12), ('tenet', 2), ('voluntary', 3), ('overdrafts', 1), ('unwarranted', 2), ('kallenberger', 1), ('undisclosed', 11), ('bellinger', 1), ('deactivated', 2), ('suitcase', 4), ('ammonium', 1), ('nitrate', 2), ('anfo', 1), ('deactivate', 1), ('backups', 1), ('nikiforov', 2), ('allowance', 4), ('staffing', 2), ('uefa', 5), ('maccabi', 1), ('betar', 1), ('dinamo', 1), ('sums', 4), ('postponing', 5), ('nikolas', 1), ('clichy', 1), ('sous', 2), ('bois', 2), ('napolitano', 4), ('radicalization', 2), ('businesswomen', 1), ('flatly', 4), ('sinking', 3), ('madhav', 2), ('kumar', 4), ('memento', 1), ('sherpa', 1), ('unfurled', 1), ('banner', 6), ('rededicate', 1), ('undertaken', 3), ('commemorated', 1), ('fundraisers', 1), ('commemorate', 12), ('acquisitions', 3), ('yadana', 2), ('dharmeratnam', 3), ('sivaram', 7), ('tamilnet', 3), ('columnist', 3), ('mirror', 6), ('realtors', 2), ('chevron', 11), ('itinerary', 1), ('acknowledges', 7), ('thamilselvan', 1), ('vidar', 1), ('helgesen', 2), ('staying', 3), ('mackay', 2), ('contrasts', 2), ('vacationing', 7), ('rests', 4), ('gagra', 1), ('sukhumi', 2), ('snowstorm', 4), ('subzero', 1), ('slippery', 1), ('nebraska', 6), ('kansas', 5), ('motels', 1), ('disbursing', 3), ('ndc', 4), ('exclusion', 1), ('millenium', 2), ('haruna', 1), ('idrissu', 1), ('abidjan', 7), ('qin', 3), ('protectionist', 2), ('trousers', 2), ('knit', 2), ('underwear', 2), ('safeguards', 4), ('softened', 1), ('adopts', 1), ('jaua', 1), ('vedomosti', 2), ('speculating', 1), ('muifa', 2), ('paralyzing', 1), ('quang', 4), ('ngai', 2), ('thua', 2), ('thien', 2), ('hue', 2), ('tri', 1), ('daklak', 1), ('bonfoh', 2), ('boko', 6), ('suicidal', 1), ('detection', 3), ('allots', 1), ('nonprofit', 3), ('immorality', 1), ('repealed', 1), ('offending', 2), ('shoichi', 1), ('nakagawa', 1), ('penn', 1), ('speculated', 3), ('fights', 3), ('roundly', 1), ('chertoff', 12), ('testifies', 1), ('oversees', 8), ('kozulin', 8), ('hooliganism', 3), ('incitement', 4), ('hadson', 2), ('reimbursed', 1), ('amortization', 1), ('slave', 5), ('lingered', 1), ('melnichenko', 2), ('precipitated', 1), ('1623', 1), ('anguilla', 5), ('rebelled', 1), ('nevis', 6), ('sekou', 1), ('unwillingness', 2), ('culminated', 4), ('sekouba', 1), ('konate', 1), ('conde', 1), ('pattern', 7), ('impressive', 8), ('nafta', 1), ('absorbs', 1), ('buffeted', 1), ('capitalization', 1), ('stuffing', 1), ('couch', 2), ('quills', 1), ('unabated', 4), ('enthusiasm', 2), ('aux', 1), ('dames', 1), ('echo', 2), ('wearily', 1), ('damn', 3), ('robbers', 3), ('tellers', 2), ('wallets', 1), ('etc', 1), ('brutally', 5), ('gagged', 3), ('whispers', 2), ('replies', 2), ('owe', 4), ('teacher', 13), ('plaster', 1), ('shirt', 6), ('noticeable', 1), ('confidently', 1), ('rowdy', 1), ('classroom', 3), ('busied', 1), ('breeze', 2), ('flap', 1), ('stapler', 1), ('stapled', 1), ('parkinson', 5), ('debilitating', 1), ('duelfer', 5), ('addendum', 1), ('policymakers', 4), ('126', 3), ('debriefing', 1), ('villager', 3), ('discriminatory', 2), ("b'tselem", 3), ('mekorot', 1), ('peoples', 14), ('rabat', 3), ('concerted', 4), ('bursa', 1), ('compatriots', 1), ('modifies', 1), ('worry', 13), ('enhances', 1), ('enshrines', 2), ('detriment', 1), ('375', 3), ('ishac', 1), ('diwan', 1), ('qusai', 1), ('wahab', 1), ('bahrainian', 1), ('adds', 9), ('satisfies', 1), ('guy', 2), ('verhofstadt', 1), ('sol', 1), ('preaching', 7), ('tuzla', 1), ('gazans', 2), ('streamed', 2), ('gijs', 1), ('vries', 1), ('fostering', 2), ('muriel', 1), ('degauque', 1), ('yousaf', 2), ('anyama', 1), ('gendarmes', 1), ('connie', 3), ('newman', 1), ('flagrantly', 1), ('semester', 1), ('sizably', 2), ('tulane', 1), ('trailers', 1), ('seminar', 4), ('alerts', 2), ('moinuddin', 1), ('karnataka', 5), ('puri', 1), ('nationalization', 10), ('affirming', 1), ('fayyum', 1), ('giza', 1), ('eradicated', 3), ('lobbied', 2), ('filip', 1), ('velach', 2), ("d'affaires", 2), ('tkachev', 1), ('krasnodar', 1), ('beatrice', 1), ('mtetwa', 2), ('norton', 1), ('dismantle', 20), ('gulu', 2), ('toby', 1), ('harnden', 1), ('simmonds', 1), ('accreditation', 5), ('budapest', 5), ('workings', 1), ('vetoes', 1), ('cooperated', 2), ('sorting', 1), ('ghalib', 2), ('kubba', 2), ('sabotaging', 1), ('adhere', 5), ('investigates', 2), ('schmit', 1), ('gestures', 5), ('zabayda', 1), ('chitchai', 1), ('wannasathit', 1), ('haiphong', 2), ('durango', 1), ('breakdown', 3), ('resembled', 1), ('benedetti', 2), ('incendiary', 1), ('pardo', 1), ('bab', 3), ('sharjee', 1), ('frangiskos', 1), ('ragoussis', 1), ('blige', 2), ('jamie', 4), ('foxx', 3), ('hudson', 4), ('pasadena', 2), ('unpredictable', 3), ('castoff', 1), ('cinderella', 1), ('dreamgirls', 1), ('naacp', 2), ('miro', 1), ('ashdown', 3), ('yousifiyah', 1), ('simplifying', 1), ('ohio', 14), ('ler', 1), ('csw', 2), ('minesweepers', 1), ('scaling', 3), ('airdrops', 1), ('karkh', 1), ('cropper', 3), ('arrange', 8), ('patrolled', 6), ('ogero', 1), ('automobiles', 3), ('hardenne', 7), ('compromised', 1), ('regaining', 1), ('chiang', 2), ('ping', 1), ('kun', 1), ('ghangzhou', 1), ('homage', 3), ('yat', 1), ('sen', 4), ('nangjing', 1), ('slideshow', 1), ('sgt', 1), ('whitney', 2), ('mounts', 1), ('newest', 6), ('surprisingly', 2), ('hate', 10), ('biennial', 2), ('74th', 1), ('weaver', 6), ('jumbo', 2), ('researching', 1), ('overturn', 5), ('evaluation', 3), ('malwiya', 1), ('jagged', 1), ('architecture', 2), ('spiral', 4), ('minarets', 2), ('abbassid', 1), ('hatem', 2), ('edict', 3), ('effectiveness', 5), ('budgets', 2), ('recipient', 3), ('relieved', 5), ('baton', 3), ('thad', 3), ('hovered', 2), ('recalling', 4), ('alps', 1), ('closeness', 1), ('unjustly', 2), ('inducted', 3), ('studded', 1), ('multitudes', 1), ('traumatic', 2), ('transfusion', 1), ('explains', 4), ('655', 2), ('understandable', 2), ('vincennes', 1), ('bandar', 6), ('inductees', 3), ('rockers', 1), ('lynyrd', 1), ('skynyrd', 1), ('butch', 1), ('kievenaar', 1), ('blondie', 1), ('opener', 5), ('warmup', 4), ('competent', 3), ('trumpeter', 1), ('herb', 1), ('alpert', 1), ('moss', 1), ('founders', 1), ('reside', 2), ('arghandab', 2), ('prematurely', 7), ('mitrovica', 3), ('seceded', 3), ('feith', 2), ('websites', 6), ('reunited', 5), ('lafayette', 1), ('novosibirsk', 1), ('disadvantaged', 3), ('stymied', 1), ('geese', 9), ('revoked', 7), ('scheuer', 2), ('inspires', 1), ('hubris', 1), ('anonymously', 1), ('inflamed', 2), ('flocks', 5), ('processed', 5), ('christ', 9), ('scouts', 2), ('kicking', 4), ('drums', 4), ('festive', 1), ('decked', 1), ('zhanjun', 1), ('blacklist', 2), ('accredited', 1), ('liu', 10), ('binjie', 1), ('celso', 3), ('amorim', 5), ('embraer', 1), ('ramping', 1), ("'re", 4), ('sulphur', 2), ("sh'ite", 1), ('sheng', 2), ('huaren', 1), ('booming', 10), ('simmons', 1), ('manas', 3), ('decisive', 4), ('fayyaz', 1), ('baqubah', 2), ('gillard', 2), ('cobbled', 1), ('foreclosures', 7), ('mortgages', 1), ('refinancing', 1), ('properties', 6), ('customized', 1), ('simulates', 1), ('sint', 7), ('maarten', 5), ('fifths', 5), ('juliana', 1), ('weisgan', 1), ('shiloh', 2), ('harbors', 2), ('antilles', 5), ('widening', 6), ('constitute', 5), ('bahamian', 1), ('enactment', 3), ('1891', 2), ('nyasaland', 1), ('1964', 5), ('hastings', 1), ('kamuzu', 1), ('dpp', 2), ('1888', 2), ('1900', 2), ('sprat', 1), ('umpire', 1), ('strolling', 2), ('orchard', 1), ('bunch', 3), ('ripening', 1), ('vine', 4), ('lofty', 2), ('quench', 1), ('quoth', 2), ('paces', 1), ('tempting', 1), ('morsel', 2), ('despise', 1), ('seldom', 2), ('butlers', 1), ('chauffeurs', 1), ('k', 7), ('drawer', 2), ('fifteen', 7), ('clone', 6), ('backfired', 1), ("'d", 2), ('obscene', 1), ('mood', 4), ('tourniquet', 1), ('noticing', 1), ('muentefering', 3), ('recruiter', 2), ('smiled', 3), ('slyly', 1), ('mules', 2), ('dividing', 8), ('fractions', 1), ('uncle', 6), ('hitched', 2), ('mule', 4), ('andrea', 1), ('nahles', 1), ('kajo', 1), ('wasserhoevel', 1), ('trawlers', 2), ('solbes', 1), ('natsios', 6), ('constructing', 1), ('firehouses', 1), ('sonia', 5), ('handgun', 4), ('elvis', 2), ('presley', 3), ('titan', 7), ('memphis', 3), ('mm', 1), ('wesson', 1), ('graceland', 3), ('visitor', 1), ('unfastened', 1), ('beside', 5), ('journeyed', 1), ('dancer', 2), ('sergey', 1), ('magerovskiy', 2), ('tiffany', 1), ('stiegler', 1), ("ba'ath", 2), ('rebecca', 1), ('naturalization', 1), ('tanith', 1), ('belbin', 1), ('shariat', 3), ('shagh', 1), ('transmitter', 2), ('hymns', 3), ('rages', 2), ('listeners', 4), ('flurry', 5), ('initialed', 1), ('valdes', 2), ('nico', 2), ('colombant', 3), ('seydou', 1), ('diarra', 1), ('thundershowers', 1), ('rounder', 1), ('nel', 1), ('boeta', 1), ('dippenaar', 1), ('bowled', 3), ('cannes', 6), ('wong', 3), ('wai', 1), ('stylized', 1), ('sci', 1), ('fi', 1), ('2047', 1), ('bernando', 1), ('vavuniya', 1), ('beatle', 2), ('yuganskneftegaz', 6), ('bogdanchikov', 1), ('donatella', 2), ('versace', 2), ('allegra', 1), ('anorexia', 1), ('zimmerman', 1), ('estanged', 1), ('beck', 4), ('syndicated', 2), ('insider', 2), ('gaunt', 2), ('correspond', 1), ('ferrero', 4), ('ortiz', 4), ('mishandled', 2), ('batumi', 2), ('reforming', 3), ('inject', 1), ('facilitate', 7), ('kazemi', 4), ('qomi', 3), ('naji', 3), ('otri', 1), ('additionally', 7), ('pramoni', 1), ('louise', 2), ('frechette', 1), ('plaque', 1), ('courageous', 7), ('araque', 2), ('kiryenko', 2), ('shurja', 2), ('616', 1), ('eden', 3), ('kolkata', 2), ('wasim', 2), ('jaffer', 4), ('laxman', 4), ('delighted', 1), ('scoring', 8), ('holing', 1), ('sohail', 1), ('tanvir', 1), ('wicket', 4), ('keeper', 2), ('mahendra', 2), ('dhoni', 1), ('waw', 1), ('vomiting', 6), ('kutesa', 3), ('barge', 1), ('looted', 2), ('yorkers', 2), ('trudging', 1), ('jogged', 1), ('biked', 1), ('defying', 4), ('museums', 6), ('costing', 3), ('earn', 5), ('nathalie', 2), ('dechy', 2), ('golovin', 3), ('marta', 3), ('marrero', 2), ('marion', 3), ('bartoli', 2), ('emilie', 1), ('loit', 1), ('ruano', 1), ('pascual', 2), ('panicked', 4), ('bleach', 1), ('restated', 4), ('cereals', 1), ('smerdon', 1), ('brink', 3), ('vukovar', 6), ('awaited', 4), ('mile', 4), ('mrksic', 1), ('radic', 1), ('veselin', 1), ('sljivancanin', 1), ('eprdf', 4), ('534', 1), ('537', 2), ('delegate', 3), ('fossil', 4), ('fuels', 6), ('reforestation', 1), ('cheating', 2), ('robby', 1), ('ginepri', 2), ('gilles', 3), ('muller', 2), ('yeu', 1), ('tzuoo', 1), ('hero', 9), ('paradorn', 2), ('srichaphan', 3), ('neurosurgeon', 1), ('fuji', 4), ('nihon', 1), ('keizai', 1), ('pioneered', 1), ('economical', 1), ('daimlerchrysler', 4), ('gwangju', 1), ('precaution', 6), ('h7', 2), ('206', 3), ('congratulatory', 2), ('tomislav', 1), ('nikolic', 1), ('quagga', 2), ('zebra', 4), ('qua', 1), ('hunted', 2), ('extinction', 3), ('subspecies', 1), ('reinhold', 1), ('rau', 1), ('endeavor', 2), ('impeach', 2), ('yearlong', 2), ('pakitka', 1), ('thrust', 1), ('zabol', 2), ('observance', 8), ('submitting', 1), ('diocese', 1), ('oils', 2), ('rituals', 3), ('commemorating', 6), ('biblical', 3), ('supper', 2), ('apostles', 2), ('wash', 1), ('feet', 14), ('liturgy', 1), ('crucifixion', 3), ('resurrection', 2), ('frances', 5), ('copei', 4), ('booth', 2), ('liberians', 3), ('unchallenged', 3), ('almaty', 2), ('disapproved', 1), ('bolstering', 3), ('clamping', 1), ('peretz', 16), ('rightists', 1), ('coaltion', 1), ('ceramic', 1), ('enlisted', 3), ('couples', 4), ('murugupillai', 1), ('jenita', 1), ('jeyarajah', 1), ('crested', 1), ('myna', 2), ('galam', 1), ('obvious', 4), ('playground', 1), ('aviaries', 1), ('fabricated', 3), ('antoine', 2), ('ghanem', 1), ('deflecting', 1), ('nuria', 1), ('llagostera', 1), ('vives', 1), ('flavia', 2), ('patty', 2), ('schnyder', 2), ('draws', 6), ('pinera', 5), ('aryanto', 1), ('boedihardjo', 1), ('aip', 1), ('hidayat', 1), ('ciamis', 1), ('regency', 1), ('comparing', 6), ('salik', 1), ('firdaus', 1), ('misno', 1), ('arraigned', 2), ('lauro', 1), ('channels', 9), ('fictional', 3), ('savors', 1), ('stirred', 6), ('martinis', 1), ('gary', 2), ('chef', 1), ('clearance', 3), ('rep', 1), ('freer', 1), ('558', 1), ('files', 4), ('clotting', 1), ('defendents', 1), ('zubeidi', 1), ('rms', 3), ('hasbrouk', 1), ('waivers', 2), ('debenture', 1), ('distributes', 2), ('158', 1), ('666', 1), ('austin', 4), ('zalkin', 1), ('608', 1), ('413', 1), ('967', 1), ('809', 1), ('muscovy', 1), ('mongol', 3), ('absorb', 1), ('principalities', 2), ('romanov', 1), ('1682', 1), ('1725', 1), ('hegemony', 1), ('russo', 3), ('1904', 3), ('defeats', 1), ('lenin', 1), ('iosif', 1), ('stalin', 4), ('stagnated', 4), ('gorbachev', 2), ('glasnost', 1), ('perestroika', 1), ('inadvertently', 2), ('splintered', 2), ('shifted', 3), ('legitimacy', 3), ('buttressed', 1), ('carefully', 10), ('seaports', 1), ('dislocation', 1), ('122', 4), ('uninhabitable', 3), ('antigua', 2), ('barbuda', 1), ('constrained', 7), ('bedding', 2), ('spencer', 1), ('topped', 3), ('antiguan', 1), ('niue', 4), ('yawning', 1), ('disadvantages', 1), ('abject', 1), ('engagement', 7), ('hemispheric', 2), ('equaling', 1), ('gardening', 1), ('reengagement', 1), ('mis', 1), ('outlays', 1), ('ant', 4), ('passion', 3), ('lime', 3), ('honey', 4), ('coconut', 1), ('cream', 4), ('dove', 4), ('pitied', 1), ('bough', 1), ('fowling', 1), ('whelp', 2), ('lambs', 1), ('pupil', 1), ('lookout', 1), ('famishing', 1), ('cottage', 3), ('babe', 1), ('sneered', 1), ('collectors', 2), ("i'd", 1), ('compliment', 1), ('durbin', 3), ('battlefield', 4), ('riek', 1), ('machar', 3), ('homestead', 1), ('nyongwa', 1), ('ankunda', 1), ('proxy', 2), ('remarked', 1), ('weakly', 1), ('jolt', 1), ('aftershocks', 4), ('hudur', 2), ('bakool', 4), ('fy08', 1), ('masorin', 2), ('sevastopol', 4), ('tartus', 1), ('decimated', 1), ('doodipora', 1), ('nabi', 4), ('azad', 1), ('rezaei', 3), ('seated', 5), ('registers', 2), ('mostafa', 3), ('moin', 2), ('baqer', 1), ('qalibaf', 2), ('stun', 3), ('constitutions', 1), ('relics', 5), ('saints', 5), ('ecumenical', 1), ('bartholomew', 2), ('constantinople', 3), ('chrysostom', 1), ('nazianzen', 1), ('insurmountable', 2), ('1054', 1), ('crusaders', 2), ('sacking', 1), ('1204', 1), ('salome', 1), ('zurabishvili', 1), ('brunei', 9), ('inforamtion', 1), ('daunting', 3), ('institutionalizing', 1), ('najam', 2), ('weeklong', 5), ('clause', 3), ('briston', 2), ('squibb', 1), ('gilead', 2), ('collaborate', 2), ('dose', 2), ('doses', 6), ('sustiva', 1), ('viread', 1), ('emtriva', 1), ('replication', 1), ('apec', 18), ('malaki', 1), ('hiker', 1), ('shourd', 7), ('untied', 1), ('hikers', 8), ('bittersweet', 1), ('bauer', 5), ('fattal', 4), ('concertacion', 1), ('foxley', 1), ('harvard', 2), ('velasco', 3), ('conquered', 5), ('1824', 1), ('yaqoob', 2), ('bot', 3), ('notari', 1), ('ne', 2), ('involuntarily', 1), ('tubes', 1), ('tombs', 7), ('artifacts', 2), ('zahi', 3), ('hawass', 5), ('servant', 2), ('saqqara', 3), ('murals', 1), ('excavation', 1), ('coffins', 2), ('mummies', 1), ('scribe', 1), ('motorized', 1), ('rickshaw', 1), ('enormous', 5), ('jurist', 2), ('abortion', 21), ('asfandyar', 2), ('marriott', 3), ('idle', 2), ('hello', 1), ('milk', 3), ('collectivization', 1), ('nationalizing', 2), ('russert', 3), ('prodemocracy', 1), ('chronicled', 1), ('eager', 5), ('suppressed', 5), ('rankings', 7), ('maternity', 2), ('skated', 1), ('undefeated', 3), ('moallem', 1), ('appalled', 1), ('barbarism', 1), ('elmar', 3), ('husseinov', 5), ('procession', 12), ('oppressive', 3), ('ilham', 4), ('aliyev', 3), ('provocation', 5), ('garnering', 2), ('inherit', 3), ('huygens', 5), ('saturn', 7), ('composition', 1), ('relaying', 3), ('cassini', 3), ('evolved', 7), ('docks', 1), ('rooftops', 3), ('armstrong', 4), ('banbury', 1), ('appointees', 2), ('roque', 5), ('lenghty', 1), ('hayam', 1), ('zahri', 1), ('borroto', 1), ('genetic', 5), ('feasibility', 3), ('expresses', 6), ('caldwell', 2), ('contemplating', 1), ('egg', 5), ('glittering', 1), ('electorate', 2), ('trick', 4), ('gemelli', 1), ('inflammation', 1), ('engagements', 3), ('muscles', 2), ('minya', 1), ('delight', 2), ('unsanitary', 1), ('simulation', 1), ('helmets', 2), ('followup', 1), ('chelyabinsk', 2), ('nonproliferation', 4), ('solidifies', 1), ('overpass', 3), ('oft', 1), ("o'er", 1), ('reaches', 7), ('earning', 4), ('rounding', 2), ('descending', 1), ('mick', 1), ('jagger', 1), ('hostess', 3), ('oprah', 1), ('winfrey', 1), ('golfer', 1), ('woods', 3), ('longevity', 3), ('consitutional', 1), ('spearker', 1), ('doe', 2), ('misfortune', 2), ('breakthroughs', 3), ('abandoning', 5), ('reversible', 1), ('ghor', 3), ('minimally', 1), ('invasive', 2), ('uterine', 2), ('fibroid', 2), ('embolization', 1), ('hysterectomy', 1), ('shrinks', 1), ('tumors', 4), ('rowed', 1), ('fibroids', 1), ('dave', 2), ('heineman', 2), ('alimport', 1), ('madrassa', 3), ('qilla', 1), ('saifullah', 2), ('belongings', 2), ('uproot', 2), ('arrogance', 1), ('sponsor', 9), ('foxy', 3), ('rapper', 3), ('inga', 1), ('marchand', 1), ('spitting', 1), ('probation', 2), ('counseling', 1), ('manicurists', 1), ('schoolgirl', 3), ('slightest', 1), ('plotter', 1), ('indianapolis', 3), ('reassessed', 1), ('384', 1), ('maligned', 1), ('supremacy', 1), ('malta', 15), ('unify', 2), ('horizons', 4), ('bereavement', 1), ('2a', 2), ('haswa', 1), ('chalabi', 8), ('sii', 4), ('rightly', 5), ('flexibility', 6), ('jib', 1), ('dial', 1), ('stefan', 1), ('mellar', 1), ('deniers', 1), ('profane', 2), ('hikmet', 1), ('cetin', 2), ('rotation', 2), ('pen', 2), ('writes', 3), ('upside', 3), ('°c', 1), ('chiyangwa', 3), ('mashonaland', 1), ('godfrey', 1), ('dzvairo', 1), ('itai', 1), ('marchi', 1), ('kenny', 3), ('karidza', 1), ('tendai', 1), ('matambanadzo', 1), ('inspecting', 2), ('naad', 1), ('mangwana', 1), ('disapproval', 3), ('krygyz', 1), ('sampling', 5), ('decorated', 2), ('dubrovka', 1), ('meridian', 7), ('haden', 1), ('maclellan', 1), ('surrey', 1), ('feniger', 1), ('1912', 2), ('1939', 5), ('partisans', 2), ('xenophobic', 1), ('combative', 1), ('deficiencies', 2), ('judged', 3), ('lsi', 1), ('dp', 5), ('sp', 1), ('wallachia', 1), ('moldavia', 1), ('suzerainty', 1), ('1856', 2), ('1859', 1), ('1862', 1), ('1878', 2), ('transylvania', 1), ('axis', 1), ('soviets', 1), ('abdication', 1), ('nicolae', 1), ('ceausescu', 2), ('securitate', 1), ('draconian', 2), ('principe', 3), ('concessional', 1), ('rescheduling', 1), ('prgf', 2), ('bonuses', 4), ('incredible', 3), ('nomadic', 2), ('1876', 1), ('tsarist', 1), ('1916', 2), ('akaev', 1), ('bakiev', 5), ('manipulated', 3), ('otunbaeva', 1), ('interethnic', 1), ('lark', 1), ('uninterred', 1), ('crest', 1), ('hillock', 1), ('monstrous', 1), ('stuck', 4), ('refrained', 1), ('answering', 4), ('lasting', 16), ('spraying', 2), ('mosquitoes', 2), ('ziemer', 2), ('comedian', 3), ('pryor', 3), ('posthumous', 1), ('deft', 1), ('degenerative', 1), ('nerve', 4), ('1938', 2), ('bowie', 1), ('merle', 1), ('haggard', 1), ('jessye', 1), ('rent', 4), ('yonts', 2), ('latif', 6), ('hakimi', 10), ('chinooks', 1), ('rotor', 1), ('sandstorm', 4), ('wadajir', 1), ('hodan', 1), ('florence', 3), ('reconciling', 1), ('seacoast', 1), ('ambulance', 2), ('kusadasi', 2), ('tak', 2), ('ezzedin', 1), ('qassam', 2), ('conducts', 3), ('ravaging', 2), ('spawned', 2), ('smoldering', 1), ('parched', 1), ('smog', 1), ('brasilia', 5), ('proposes', 6), ('sonthi', 1), ('boonyaratglin', 1), ('extraditing', 3), ('influencing', 2), ('shaima', 1), ('rezayee', 2), ('tolo', 3), ('char', 1), ('bowing', 1), ('shaer', 3), ('360', 3), ('bombard', 1), ('sheriff', 7), ('booked', 1), ('fingerprinted', 1), ('booking', 2), ('shrunk', 1), ('layoff', 1), ('citi', 1), ('replenish', 3), ('merrill', 2), ('lynch', 2), ('brokerage', 2), ('walks', 1), ('ferencevych', 3), ('waning', 1), ('memories', 2), ('turbulent', 5), ('naha', 1), ('mint', 1), ('mouknass', 1), ('definitive', 3), ('brig', 1), ('hartmann', 2), ('sexy', 1), ('pertains', 1), ('salim', 7), ('hamdan', 2), ('mental', 5), ('nasty', 1), ('fsg', 1), ('spessart', 1), ('frigate', 4), ('rheinland', 1), ('pfalz', 1), ('pak', 6), ('ui', 2), ('models', 4), ('strutting', 1), ('catwalk', 1), ('mellon', 1), ('pittsburgh', 4), ('imran', 3), ('siddiqi', 1), ('retained', 8), ('usual', 8), ('kyiv', 3), ('finalizing', 4), ('beechcraft', 1), ('turboprop', 2), ('zhulyany', 1), ('wooden', 6), ('phyu', 3), ('jerzy', 4), ('szmajdzinski', 3), ('chant', 2), ('zionism', 3), ('confronted', 5), ('intoning', 1), ('ruhollah', 3), ('khomeini', 3), ('mica', 1), ('stanisic', 3), ('outs', 3), ('adde', 1), ('slovakian', 1), ('zsolt', 1), ('grebe', 1), ('peregrine', 1), ('gabcikovo', 1), ('upsets', 1), ('robredo', 4), ('philipp', 2), ('kohlschreiber', 1), ('transformer', 1), ('kayettuli', 1), ('ayad', 4), ('gloomier', 1), ('crafting', 3), ('unsealed', 5), ('haris', 1), ('ehsanul', 1), ('sadequee', 1), ('coerced', 2), ('blackmail', 3), ('positioning', 1), ('iraqiya', 4), ('bheri', 1), ('surkhet', 1), ('cables', 3), ('takirambudde', 1), ('totals', 2), ('zalmay', 8), ('khalilzad', 12), ('firefighting', 1), ('arid', 3), ('razed', 2), ('workshop', 3), ('carpentry', 1), ('provoke', 6), ('kuomintang', 1), ('hsiao', 1), ('bi', 2), ('khim', 1), ('safehouse', 2), ('bombmaking', 1), ('blasphemy', 3), ('perwiz', 1), ('kambakhsh', 2), ('defaming', 3), ('reexamine', 1), ('stipulated', 1), ('punishable', 3), ('saarc', 3), ('dissolving', 2), ('142', 3), ('baribari', 1), ('shoreline', 2), ('campaigns', 9), ('alhurra', 1), ('confusion', 3), ('repel', 1), ('foreclosure', 5), ('simkins', 3), ('barretto', 3), ('percussionist', 1), ('conga', 1), ('spanned', 2), ('dizzy', 1), ('gillespie', 1), ('tito', 2), ('puente', 1), ('salsa', 1), ('celia', 1), ('cruz', 5), ('rhythm', 3), ('maduro', 9), ('incomprehensible', 3), ('beached', 2), ('forty', 4), ('spit', 3), ('hopeless', 1), ('refloating', 1), ('refloat', 1), ('settings', 1), ('anticipate', 3), ('claus', 4), ('mythical', 1), ('martti', 2), ('ahtisaari', 3), ('winding', 1), ('trillions', 2), ('borg', 1), ('bonus', 4), ('risky', 1), ('bets', 2), ('farris', 1), ('redrawing', 1), ('separates', 1), ('bathrooms', 2), ('pierced', 1), ('ging', 3), ('cultivated', 3), ('fend', 5), ('attributes', 1), ('sahab', 1), ('rivalries', 3), ('alerting', 2), ('chase', 8), ('europrean', 1), ('greens', 2), ('renate', 1), ('kuenast', 1), ('kuhn', 1), ('batasuna', 1), ('du', 3), ('qinglin', 1), ('lambert', 3), ('nigerians', 2), ('kano', 6), ('mersin', 1), ('counterkidnapping', 1), ('leonel', 3), ('momcilo', 2), ('perisic', 4), ('cinema', 2), ('thatcher', 1), ('pathe', 1), ('productions', 1), ('dj', 1), ('frears', 1), ('helen', 3), ('mirren', 1), ('ratners', 7), ('weisfield', 3), ('outbid', 1), ('qalqilya', 3), ('gerald', 3), ('ratner', 1), ('sweetened', 1), ('acceptances', 1), ('aug', 2), ('rupee', 1), ('overvalued', 1), ('amortizing', 1), ('downgrading', 2), ('eff', 1), ('erratic', 4), ('profitability', 5), ('fluctuates', 3), ('erm2', 1), ('preceding', 4), ('peers', 2), ('tipped', 2), ('bounce', 1), ('payroll', 3), ('revamp', 2), ('mixture', 3), ('supplanted', 1), ('overstaffing', 1), ('mortgaged', 1), ('shortfalls', 6), ('renewing', 5), ('presides', 4), ('uneasy', 2), ('stimulating', 2), ('specializes', 1), ('conformity', 1), ('enhance', 11), ('245', 1), ('qamdo', 1), ('prefecture', 5), ('bern', 1), ('prized', 1), ('sizable', 9), ('secrecy', 4), ('consequently', 2), ('incorporate', 2), ('awaits', 2), ('behest', 1), ('aftershock', 6), ('mururoa', 1), ('atoll', 4), ('polynesia', 2), ('distinguished', 2), ('microenterprises', 1), ('vendors', 3), ('deficient', 2), ('shipwrecked', 2), ('buffetings', 1), ('awoke', 2), ('reproaches', 1), ('calmness', 1), ('plow', 2), ('assuming', 6), ('lash', 4), ('fury', 1), ('sorry', 5), ('cd', 2), ('demolished', 5), ('issawiya', 1), ('daoud', 6), ('criminalizing', 1), ('tainting', 1), ('erich', 1), ('scrambled', 2), ('intercept', 4), ('interrogating', 3), ('circumventing', 1), ('rumbek', 1), ('phil', 8), ('spector', 11), ('superior', 2), ('fidler', 1), ('cutler', 1), ('reconvene', 2), ('technique', 4), ('lana', 3), ('daughters', 5), ('siti', 4), ('fadillah', 2), ('supari', 4), ('probability', 2), ('tynychbek', 1), ('akmatbayev', 2), ('transferable', 1), ('blackout', 9), ('subways', 4), ('outage', 5), ('substation', 2), ('anatoly', 5), ('chubais', 2), ('tula', 1), ('escravos', 1), ('repelled', 7), ('entitlements', 1), ('chookiat', 1), ('ophaswongse', 1), ('wesley', 2), ('moodie', 6), ('legg', 2), ('mason', 2), ('86th', 2), ('querrey', 2), ('aces', 3), ('grosjean', 1), ('danai', 1), ('udomchoke', 1), ('43rd', 2), ('oudsema', 1), ('nightfall', 2), ('scan', 1), ('proclaim', 1), ('accordingly', 1), ('purification', 1), ('thirteenth', 1), ('jailings', 1), ('batted', 1), ('bat', 2), ('shaharyar', 1), ('preside', 3), ('perkins', 3), ('moveon', 1), ('org', 2), ('funneling', 4), ('muqdadiyah', 2), ('inzamam', 1), ('haq', 5), ('hajj', 3), ('stoning', 4), ('mina', 1), ('jamarat', 1), ('devil', 4), ('grueling', 1), ('bodied', 1), ('converge', 2), ('communion', 1), ('kazakh', 2), ('wasit', 1), ('assassins', 2), ('ugandans', 4), ('\x92s', 9), ('milton', 3), ('obote', 3), ('kampala', 5), ('mwesige', 1), ('shaka', 1), ('ssali', 1), ('murat', 3), ('sutalinov', 1), ('objectionable', 1), ('ancestral', 2), ('cancelation', 1), ('herman', 1), ('rompuy', 1), ('refrain', 7), ('insolent', 2), ('capitalized', 4), ('edinburgh', 1), ('undetermined', 5), ('descended', 3), ('talat', 12), ('dervis', 2), ('eroglu', 2), ('favors', 8), ('reunify', 3), ('cypriots', 16), ('hinge', 2), ('chhattisgarh', 1), ('raipur', 1), ('jharkhand', 1), ('schumer', 3), ('fratto', 1), ('indices', 1), ('donating', 7), ('jilin', 3), ('dehui', 1), ('tulsi', 1), ('giri', 1), ('kadima', 16), ('accuracy', 4), ('inaccuracies', 4), ('pat', 5), ('tillman', 2), ('mostafavi', 1), ('jude', 1), ('culprit', 2), ('protein', 5), ('genes', 1), ('proteins', 1), ('sampled', 2), ('demolitions', 2), ('chepas', 2), ('josefa', 1), ('chihuahua', 7), ('baeza', 1), ('aston', 1), ('mulally', 1), ('khamis', 1), ('mallahi', 2), ('accomplice', 7), ('tawhid', 2), ('wal', 15), ('117', 4), ('dahab', 1), ('compiled', 3), ('192', 3), ('255', 2), ('rahul', 3), ('dravid', 6), ('sachin', 1), ('tendulkar', 2), ('dinesh', 2), ('kartik', 1), ('anchored', 3), ('136', 3), ('tallied', 1), ('brar', 1), ('shamsbari', 1), ('doda', 2), ('mart', 14), ('wreaked', 1), ('blockage', 1), ('sidakan', 1), ('132', 5), ('atlantis', 6), ('rex', 1), ('walheim', 1), ('hans', 3), ('schlegel', 2), ('orbital', 1), ('outing', 1), ('crewmates', 1), ('cermak', 3), ('mladen', 3), ('markac', 3), ('krajina', 2), ('leopold', 1), ('eyharts', 1), ('floated', 3), ('deadlines', 4), ('sony', 10), ('ericsson', 3), ('plundered', 1), ('stabbed', 4), ('handsets', 1), ('umpires', 1), ('smear', 2), ('din', 4), ('circulating', 6), ('leaflets', 3), ('restarted', 7), ('charkhi', 4), ('hashimzai', 1), ('sketchy', 4), ('obeyed', 1), ('raging', 2), ('mourns', 2), ('nsc', 1), ('selecting', 1), ('adversaries', 3), ('explicit', 1), ('theatergoers', 1), ('suspense', 1), ('disturbiaunseated', 1), ('blades', 3), ('peeping', 1), ('starring', 3), ('shia', 2), ('labeouf', 1), ('morse', 1), ('takers', 5), ('dreamworks', 1), ('distributor', 1), ('disturbia', 2), ('lebeouf', 1), ('penguin', 2), ('surf', 2), ('transformers', 1), ('hualan', 1), ('mutating', 3), ('ursula', 4), ('plassnik', 2), ('pluralistic', 2), ('nad', 3), ('heartland', 2), ('satan', 1), ('interwoven', 1), ('sud', 2), ('salif', 1), ('sadio', 3), ('ousamane', 1), ('ngom', 1), ('taraba', 1), ('grappling', 3), ('orellana', 2), ('suspends', 1), ('encampment', 1), ('disturbed', 5), ('equity', 3), ('afflicting', 1), ('imperative', 1), ('prohibit', 4), ('defamation', 3), ('oblivious', 1), ('hospitality', 4), ('destitute', 1), ('huts', 1), ('inspire', 5), ('condoms', 2), ('firestorm', 2), ('homily', 2), ('clouds', 3), ('wolfowitz', 10), ('supplemental', 1), ('recounted', 1), ('fats', 4), ('domino', 4), ('nickname', 3), ('fat', 6), ('hits', 3), ('blueberry', 1), ('uncover', 3), ('portrait', 3), ('handsome', 3), ('erasmus', 1), ('avenging', 1), ('dangerously', 2), ('246', 1), ('oleksiy', 1), ('ivchenko', 1), ('ukrainy', 1), ('apprehended', 3), ('predrag', 1), ('kujundzic', 3), ('doboj', 2), ('verifying', 4), ('widen', 5), ("shi'te", 1), ('dulles', 1), ('ahram', 3), ('omission', 1), ('rostock', 1), ('heiligendamm', 1), ('rostok', 1), ('pig', 4), ('streptococcus', 2), ('suis', 2), ('bacteria', 5), ('174', 2), ('pork', 7), ('provocations', 4), ('anwar', 12), ('gargash', 1), ('sift', 1), ('displaying', 1), ('passages', 1), ('bara', 1), ('kazimierz', 3), ('marcinkiewicz', 3), ('caps', 5), ('nuys', 1), ('calif', 1), ('realestate', 1), ('fluctuation', 1), ('361', 3), ('millennia', 2), ('rok', 3), ('dprk', 5), ('1950', 5), ('demilitarized', 3), ('38th', 1), ('myung', 5), ('bak', 5), ('punctuated', 1), ('cheonan', 2), ('1493', 3), ('bartolomeo', 1), ('1648', 3), ('1784', 1), ('gustavia', 1), ('repurchased', 1), ('appellations', 1), ('coat', 2), ('populace', 1), ('collectivity', 1), ('hereditary', 1), ('premiers', 1), ('ensuing', 4), ('assumption', 3), ('promulgation', 1), ('plurality', 1), ('overruled', 1), ('leninist', 2), ('gridlock', 1), ('jhala', 1), ('khanal', 1), ('guyana', 5), ('abolition', 5), ('importation', 3), ('indentured', 1), ('plantations', 3), ('ethnocultural', 1), ('persisted', 3), ('cheddi', 1), ('jagan', 2), ('bharrat', 1), ('jagdeo', 1), ('rhodesia', 1), ('1923', 1), ('1920s', 4), ('unequivocally', 2), ('anticorruption', 3), ('chiluba', 2), ('usd', 2), ('abrupt', 3), ('spratly', 1), ('reefs', 3), ('overlaps', 1), ('reef', 1), ('placard', 2), ('shopkeeper', 2), ('salesman', 2), ('draped', 4), ('pozarevac', 2), ('milorad', 2), ('vucelic', 1), ('mira', 1), ('markovic', 1), ('remembrances', 1), ('surgical', 6), ('mash', 2), ('trauma', 1), ('beja', 3), ('vandalizing', 2), ('shebaa', 2), ('blockbuster', 1), ('thermopylae', 1), ('480', 2), ('grossed', 2), ('copied', 1), ('disciplined', 4), ('amnesties', 1), ('absolve', 1), ('guerillas', 7), ('watchful', 1), ('zhao', 6), ('ziyang', 1), ('magnolia', 1), ('downplayed', 5), ('auspices', 5), ('themes', 2), ('bajram', 4), ('kosumi', 6), ('fatmir', 1), ('sejdiu', 1), ('agim', 2), ('ceku', 4), ('waseem', 1), ('sohrab', 1), ('goth', 1), ('cartographers', 1), ('currencies', 12), ('lutfi', 2), ('haziri', 1), ('commands', 3), ('ethic', 1), ('foster', 4), ('angering', 2), ('bargal', 2), ('puntland', 1), ('dams', 2), ('elyor', 1), ('ganiyev', 1), ('173', 3), ('elsa', 3), ('alvarezes', 1), ('shortwave', 3), ('encryption', 2), ('261', 1), ('543', 2), ('bharatiya', 2), ('janata', 4), ('159', 3), ('dissent', 6), ('thanking', 2), ('wishes', 8), ('kwanzaa', 1), ('wintery', 1), ('valleys', 3), ('jawad', 3), ('bolani', 2), ('qader', 1), ('jassim', 1), ('sherwan', 1), ('waili', 2), ('aigle', 2), ('azur', 2), ('gaulle', 1), ('qarabaugh', 1), ('stifling', 2), ('klerk', 1), ('robben', 2), ('denktash', 3), ('jafaari', 1), ('commend', 1), ('torshin', 1), ('shepel', 1), ('klebnikov', 3), ('writings', 2), ('ereli', 11), ('hanover', 3), ('centrifuge', 2), ('disapproves', 1), ('evangelist', 2), ('wholly', 2), ('childhood', 7), ('cdc', 5), ('declassified', 3), ('gimble', 1), ('assertions', 2), ('telephoned', 6), ('godfather', 2), ('papa', 1), ('autobiography', 3), ('mariann', 1), ('boel', 1), ('sensible', 2), ('zhenchuan', 1), ('breakers', 1), ('hurling', 1), ("n'guesso", 1), ('statutes', 2), ('norms', 3), ('permissible', 1), ('mistreatment', 10), ('definition', 3), ('inflicted', 6), ('gvero', 1), ('trans', 5), ('corridor', 4), ('interstate', 2), ('fingerprint', 2), ('mocny', 1), ('fingerprinting', 2), ('apply', 18), ('accosted', 1), ('trunk', 2), ('anne', 2), ('idrac', 1), ('sustains', 1), ('overuse', 3), ('contamination', 7), ('landfills', 1), ('infants', 4), ('isaias', 3), ('afeworki', 1), ('backward', 4), ('sufficiently', 2), ('bakery', 5), ('rethink', 1), ('susy', 2), ('tekunan', 2), ('quest', 2), ('recycled', 2), ('organic', 1), ('baking', 2), ('cookies', 1), ('unfriendly', 2), ('deserters', 2), ('nasariyah', 1), ('lattes', 1), ('untitled', 1), ('retailer', 8), ('julio', 4), ('cobos', 1), ('thriving', 2), ('lublin', 3), ('synagogue', 3), ('schudrich', 1), ('scrolls', 1), ('rabinnical', 1), ('videos', 8), ('papua', 6), ('merbau', 1), ('undisturbed', 1), ('hifikepunye', 2), ('pohamba', 6), ('windhoek', 2), ('nujoma', 5), ('swapo', 2), ('admhaiyah', 1), ('designation', 4), ('momen', 1), ('dawei', 5), ('ye', 1), ('heliodoro', 1), ('legislatures', 3), ('construct', 5), ('reclassify', 1), ('bloating', 1), ('dot', 1), ('canals', 2), ('riverbanks', 1), ('irrawaddy', 2), ('scarce', 5), ('profiteers', 1), ('exorbitant', 2), ('essentials', 1), ('lecturers', 1), ('knu', 1), ('ghazaliyah', 1), ('policing', 1), ('hovers', 1), ('kph', 2), ('chequers', 1), ('outer', 5), ('peoria', 2), ('kalamazoo', 1), ('mich', 1), ('managers', 12), ('severance', 3), ('maumoon', 1), ('gayoom', 2), ('embark', 1), ('legalized', 4), ('nasheed', 2), ('elevation', 2), ('defeating', 3), ('tsz', 2), ('remotely', 2), ('demarcated', 2), ('coordinates', 2), ('tracts', 2), ('badme', 1), ('eebc', 1), ('immersed', 1), ('advent', 1), ('frg', 2), ('gdr', 2), ('expended', 1), ('heel', 1), ('corporations', 4), ('geographical', 3), ('intervening', 2), ('mitigating', 1), ('radicova', 1), ('boar', 3), ('agonies', 1), ('fiercer', 1), ('renewal', 5), ('vultures', 2), ('crows', 2), ('unto', 1), ('gasping', 1), ('subjects', 4), ('helpless', 3), ('grudges', 1), ('tusks', 1), ('bull', 5), ('gored', 2), ('growled', 1), ('cowards', 1), ('majesty', 1), ('marry', 1), ('proliferating', 1), ('belts', 3), ('vanallen', 2), ('fan', 3), ('ichiro', 1), ('ozawa', 3), ('audiotapes', 1), ('doug', 1), ('wead', 2), ('discusses', 4), ('pathologically', 1), ('liar', 1), ('179', 1), ('343', 1), ('csongrad', 1), ('brett', 3), ('tea', 8), ('196', 1), ('triangular', 2), ('3rd', 2), ('mall', 8), ('abnormally', 1), ('mortality', 4), ('ratnayke', 1), ('velupillai', 2), ('prabhakaran', 3), ('whittington', 10), ('bruised', 1), ('wyoming', 3), ('renowned', 4), ('chahine', 3), ('cosmopolitan', 1), ('multiculturalism', 1), ('imperialism', 3), ('lakhdaria', 2), ('etihad', 1), ('fomenting', 2), ('bastille', 1), ('inalienable', 1), ('instructor', 1), ('basij', 1), ('vigilantes', 1), ('azov', 1), ('kerch', 2), ('volganeft', 1), ('139', 4), ('sulfur', 1), ('cary', 1), ('porpoises', 1), ('arena', 4), ('tuneup', 1), ('leaning', 4), ('nashville', 2), ('fallouj', 1), ('petrodar', 1), ('conglomerate', 1), ('rawalpindi', 7), ('quarterfinal', 6), ('yekiti', 2), ('maashouq', 2), ('khaznawi', 4), ('kameshli', 2), ('arbab', 1), ('basir', 1), ('encountering', 2), ('converts', 2), ('rajah', 1), ('solaiman', 3), ('zamboanga', 1), ('ferry', 12), ('cnooc', 3), ('unocal', 6), ('forceful', 3), ('bidder', 2), ('inlets', 1), ('fumes', 1), ('petrechemical', 1), ('pollutants', 3), ('bearing', 4), ('interpreting', 1), ('sepat', 3), ('taitung', 1), ('gusts', 4), ('plowing', 1), ('kurd', 7), ('guardedly', 1), ('defining', 2), ('grips', 1), ('regrettably', 1), ('channeled', 1), ('culls', 1), ('555', 1), ('mentioning', 5), ('wisely', 1), ('krugman', 1), ('trichet', 1), ('weathered', 3), ('boao', 1), ('rigging', 9), ('mowaffaq', 1), ('rubaie', 1), ('anfal', 5), ('formulas', 1), ('fade', 2), ('urgently', 7), ('phillips', 1), ('comfort', 2), ('ciudad', 6), ('juarez', 10), ('battleground', 1), ('goers', 2), ('sinaloa', 1), ('lags', 2), ('emitted', 1), ('disqualified', 6), ('nosedive', 1), ('shortened', 5), ('646', 2), ('creators', 1), ('890', 1), ('calin', 2), ('tariceanu', 2), ('popov', 1), ('siirt', 2), ('diyarbakir', 4), ('perspective', 4), ('voyage', 3), ('mariam', 3), ('suffocation', 5), ('poncet', 1), ('buffer', 6), ('barcode', 1), ('barcodes', 5), ('clerks', 1), ('inventory', 3), ('scanners', 2), ('exactly', 9), ('barcoded', 1), ('chewing', 1), ('invalidated', 1), ('massed', 2), ('redoing', 1), ('glasgow', 7), ('trainees', 2), ('cylinders', 2), ('nails', 1), ('mack', 3), ('sabeel', 3), ('kafeel', 3), ('haneef', 3), ('macapagal', 4), ('jam', 1), ('refiling', 1), ('soliciting', 3), ('everybody', 2), ('sacrilegious', 1), ('valdivia', 1), ('clot', 4), ('ultrasound', 1), ('unicameral', 1), ('verkhovna', 1), ('rada', 1), ('clots', 2), ('immobility', 1), ('destined', 3), ('suits', 4), ('informer', 3), ('nycz', 1), ('dreaded', 1), ('apparatus', 4), ('uthai', 1), ('burying', 1), ('petrovka', 1), ('smashing', 3), ('bakyt', 1), ('seitov', 1), ('secretariat', 2), ('ramechhap', 1), ('trailed', 1), ('francesca', 2), ('schiavone', 1), ('chess', 2), ('kasparov', 1), ('kasyanov', 1), ('liberals', 2), ('magnet', 1), ('disparate', 1), ('transcends', 1), ('superseded', 1), ('trump', 6), ('cohen', 3), ('blond', 1), ('haired', 1), ('telephones', 1), ('emomali', 2), ('rakhmon', 2), ('hazards', 3), ('tajik', 4), ('jokhang', 1), ('igor', 6), ('andreev', 3), ('gongga', 1), ('rabdhure', 1), ('inflate', 1), ('appease', 3), ('amin', 6), ('finalists', 1), ('finisher', 2), ('mexicans', 2), ('underscore', 4), ('coleco', 3), ('chapter', 6), ('reorganization', 2), ('stockholders', 1), ('unsecured', 2), ('owed', 6), ('reorganized', 2), ('ranger', 1), ('avon', 1), ('glitches', 1), ('patch', 1), ('craze', 1), ('anjouan', 3), ('moheli', 1), ('azali', 3), ('fomboni', 1), ('rotates', 1), ('sambi', 1), ('bacar', 1), ('effected', 2), ('anjouanais', 1), ('comoran', 1), ('dioceses', 2), ('curia', 1), ('mementos', 1), ('moreover', 3), ('1825', 2), ('consisted', 2), ('countercoups', 1), ('bolivians', 5), ('widest', 1), ('empower', 1), ('amerindian', 2), ('lowlands', 1), ('entrant', 1), ('wavered', 1), ('tallinn', 1), ('bursting', 3), ('bubble', 2), ('1861', 3), ('sicily', 2), ('benito', 3), ('mussolini', 3), ('fascist', 3), ('azerbaijani', 3), ('eec', 2), ('forefront', 1), ('outperformed', 1), ('encamped', 3), ('notch', 1), ('boediono', 1), ('continuity', 1), ('impediments', 3), ('conserving', 2), ('peatlands', 1), ('trailblazing', 1), ('redd', 1), ('choked', 2), ('ostrich', 4), ('suppose', 1), ('err', 1), ('testament', 1), ('resigning', 2), ('interred', 1), ('instituting', 2), ('kheir', 2), ('terrorizing', 1), ('negate', 2), ('morally', 1), ('reprehensible', 4), ('silvan', 5), ('chodo', 3), ('firings', 2), ('commute', 1), ('basing', 1), ('grigory', 1), ('karasin', 2), ('lighthouses', 1), ('bachelor', 1), ('toussaint', 2), ('rewarded', 1), ('93rd', 1), ('sfeir', 2), ('maronite', 3), ('redirected', 1), ('unfiltered', 2), ('click', 2), ('redirecting', 1), ('hack', 1), ('blog', 3), ('drummond', 1), ('firecracker', 1), ('diwali', 4), ('ignited', 2), ('pallipat', 1), ('sweets', 1), ('snacks', 1), ('lamps', 1), ('ilo', 3), ('bilis', 2), ('518', 1), ('hun', 4), ('346', 1), ('unverified', 1), ('24th', 3), ('rizkar', 1), ('pawn', 1), ('steadfast', 1), ('covenant', 1), ('deposited', 1), ('byelection', 1), ('disbarred', 1), ('amsterdam', 2), ('rollout', 1), ('hennes', 1), ('mauritz', 1), ('ab', 1), ('purses', 1), ('risque', 1), ('conical', 1), ('bras', 1), ('fellowships', 1), ('orphanages', 1), ('discounts', 4), ('recalls', 1), ('reliability', 1), ('honda', 1), ('hyundai', 2), ('kifaya', 2), ('tomorrow', 3), ('forgery', 2), ('sniffing', 1), ('sherry', 1), ('fining', 1), ('misquoting', 2), ('informing', 4), ('padilla', 5), ('classification', 1), ('goddess', 1), ('beijings', 1), ('capitol', 11), ('memorials', 3), ('postsays', 2), ('hacksaws', 1), ('transports', 1), ('muse', 1), ('lone', 5), ('merging', 2), ('nizar', 2), ('trabelsi', 2), ('bakara', 3), ('620', 1), ('777', 1), ('kochi', 1), ('vasil', 2), ('baziv', 1), ('slauta', 1), ('kharkiv', 1), ('yevhen', 1), ('kushnaryov', 1), ('yuschenko', 3), ('instructors', 2), ('rousing', 1), ('nasiriyah', 3), ('ansa', 3), ('ivashov', 2), ('ryzhkov', 1), ('yevgeny', 1), ('primakov', 1), ('phan', 1), ('khai', 1), ('tran', 1), ('duc', 2), ('luong', 2), ('burundians', 4), ('sized', 3), ('giustra', 1), ('bellerive', 3), ('osterholm', 1), ('spreads', 3), ('hiroshima', 9), ('nagasaki', 4), ('atom', 4), ('katsuya', 1), ('okada', 1), ('naoto', 1), ('kan', 1), ('processes', 6), ('chipmaker', 2), ('shaft', 5), ('laurence', 1), ('golborne', 2), ('analyze', 3), ('boring', 1), ('shafts', 2), ('mardi', 5), ('gras', 5), ('literally', 1), ('precedes', 2), ('purple', 2), ('beads', 2), ('feather', 1), ('boas', 1), ('connick', 1), ('batlle', 2), ('chao', 1), ('congratuatlions', 1), ('posht', 1), ('rud', 1), ('artemisinin', 1), ('bala', 1), ('boluk', 1), ('vx', 3), ('midestern', 1), ('drained', 2), ('newport', 1), ('sodium', 2), ('hydroxide', 1), ('escorts', 2), ('reverses', 1), ('definitely', 1), ('falciparum', 1), ('posters', 8), ('plastered', 1), ('simmering', 2), ("ba'athists", 1), ('blueprints', 2), ('sui', 1), ('nawab', 1), ('maliky', 1), ('risked', 2), ('kandani', 2), ('ngwira', 5), ('miyazaki', 2), ('emergence', 3), ('jaghato', 1), ('nahim', 1), ('pajhwok', 2), ('blechschmidt', 2), ('malawian', 1), ('consolidating', 2), ('yulija', 2), ('plenary', 3), ('willy', 1), ('mwaluka', 1), ('artisans', 1), ('zones', 6), ('kirk', 3), ('tops', 3), ('hotspots', 1), ('consequence', 4), ('kosachev', 2), ('adijon', 1), ('luncheon', 3), ('awake', 2), ('warrior', 1), ('malignant', 2), ('tumor', 4), ('tendering', 1), ('99', 10), ('jon', 2), ('peters', 3), ('guber', 3), ('litigation', 2), ('iles', 5), ('eparses', 2), ('integral', 2), ('taaf', 1), ('archipelagos', 1), ('crozet', 1), ('kerguelen', 2), ('ile', 2), ('fauna', 1), ('adelie', 1), ('slice', 1), ('1840', 3), ('eligibility', 3), ('satisfying', 2), ("mutharika's", 1), ('exhibited', 1), ('goodall', 1), ('gondwe', 1), ('unreliable', 3), ('kiel', 3), ('oresund', 1), ('bosporus', 1), ('seaway', 1), ('hydrographic', 3), ('delimit', 4), ('latitude', 3), ('jomo', 1), ('kenyatta', 2), ('toroitich', 1), ('kanu', 4), ('fractured', 2), ('dislodge', 2), ('multiethnic', 1), ('rainbow', 1), ('narc', 2), ('uhuru', 1), ('conciliatory', 1), ('odm', 1), ('powersharing', 1), ('eliminates', 1), ('coral', 7), ('uninhabited', 6), ('willis', 1), ('islets', 1), ('automated', 3), ('beacons', 1), ('lighthouse', 2), ('dolphin', 5), ('ought', 3), ('gladly', 1), ('traitor', 1), ('nay', 1), ('drunken', 3), ('wallow', 1), ('converged', 1), ('disparities', 4), ('owning', 1), ('jeungsan', 1), ('quoting', 3), ('dhi', 1), ('qar', 2), ('nautical', 1), ('listen', 1), ('7115', 1), ('9885', 1), ('11705', 1), ('11725', 1), ('voanews', 1), ('solvent', 2), ('depressing', 1), ('argentinean', 1), ('angie', 1), ('sanclemente', 1), ('valenica', 1), ('airat', 1), ('vakhitov', 2), ('korans', 2), ('drifting', 2), ('hae', 1), ('panmunjom', 1), ('ashraf', 6), ('sara', 2), ('meadowbrook', 1), ('ozlam', 1), ('sanabel', 1), ('obsessed', 1), ('chorus', 3), ('alexeyenko', 1), ('mathew', 2), ('stewart', 5), ('surveying', 2), ('ordnance', 1), ('swiergosz', 1), ('chelsea', 2), ('goats', 5), ('cows', 2), ('filtering', 3), ('regulating', 3), ('vaguely', 1), ('worded', 3), ('lawbreakers', 2), ('hotmail', 2), ('fortinet', 1), ('irawaddy', 1), ('valery', 1), ('sitnikov', 1), ('bio', 2), ('336', 2), ('enacts', 1), ('fitzpatrick', 1), ('habbaniya', 1), ('yields', 3), ('mandates', 1), ('donation', 5), ('airlifts', 1), ('benghazi', 1), ('heightening', 1), ('choe', 3), ('thae', 1), ('bok', 1), ('su', 4), ('hon', 2), ('okah', 2), ('deceased', 4), ('mandarin', 1), ('tuo', 1), ('bisphenol', 2), ('bpa', 1), ('liver', 2), ('rachid', 2), ('ilhami', 1), ('gafir', 1), ('abdelkader', 1), ('oxygen', 2), ('preacher', 5), ('loyalties', 1), ('jamal', 8), ('nimnim', 1), ('kyodo', 4), ('grieves', 1), ('talangama', 1), ('peng', 3), ('shuai', 1), ('36th', 2), ('michaella', 2), ('krajicek', 7), ('wessels', 2), ('lena', 4), ('groenefeld', 4), ('kiefer', 2), ('stosur', 1), ('arthurs', 1), ('dent', 2), ('preservation', 2), ('1850s', 1), ('clemency', 4), ('disrespectful', 1), ('kai', 4), ('dissipated', 1), ('inundated', 4), ('stanley', 3), ('tookie', 1), ('infamous', 5), ('zinedine', 3), ('zidane', 11), ('inspirational', 1), ('playmaker', 1), ('juventus', 4), ('grouped', 2), ('unnerved', 2), ('westinghouse', 2), ('competed', 6), ('wrecking', 1), ('clunkers', 4), ('fulayfill', 1), ('suez', 4), ('geyer', 1), ('cavalry', 1), ('157', 3), ('jeb', 1), ('rené', 1), ('préval', 2), ('sanderson', 1), ('rony', 1), ('comprise', 4), ('petraeus', 9), ('saves', 3), ('trooper', 1), ('embezzling', 8), ('mobutu', 2), ('sese', 1), ('seko', 1), ('shajoy', 1), ('jesper', 1), ('helsoe', 1), ('feyzabad', 1), ('yazdi', 1), ('tirin', 3), ('mahendranagar', 1), ('sham', 4), ('legitimizing', 2), ('bager', 1), ('uspi', 1), ('asadullah', 2), ('seismologists', 5), ('guardians', 1), ('farraj', 3), ('libbi', 3), ('shiekh', 2), ('insein', 3), ('lntelligence', 1), ('alcantara', 2), ('altcantara', 1), ('technicians', 2), ('pad', 3), ('vsv', 1), ('equator', 3), ('voto', 1), ('bernales', 1), ('convey', 3), ('likening', 1), ('gear', 1), ('pervasive', 2), ('practitioners', 1), ('asserted', 3), ('stalls', 3), ('tarnish', 1), ('depict', 2), ('disastrous', 7), ('detractors', 1), ('wishers', 1), ('iskander', 2), ('paralympic', 3), ('paralympics', 1), ('chongqing', 3), ('urumqi', 2), ('tianjin', 5), ('statisticians', 1), ('gymnasiums', 1), ('sleek', 2), ('chrome', 1), ('oversized', 1), ('wheels', 3), ('velocity', 1), ('575', 1), ('streaked', 1), ('515', 1), ('581', 1), ('levitates', 1), ('magnetic', 2), ('alstom', 1), ('horsepower', 1), ('decker', 3), ('prototype', 3), ('rental', 2), ('786', 1), ('boosters', 3), ('alleviation', 1), ('borrower', 2), ('citibank', 1), ('295', 2), ('revelations', 1), ('britons', 3), ('laying', 9), ('mayo', 1), ('70s', 3), ('environments', 1), ('\x91', 1), ('\x92', 1), ('kickbacks', 6), ('paige', 2), ('kollock', 2), ('chester', 2), ('gunsmoke', 2), ('qualifications', 2), ('decathlon', 1), ('emmy', 2), ('limping', 1), ('starred', 3), ('mccloud', 1), ('taher', 2), ('ani', 1), ('busta', 2), ('rhymes', 3), ('lectures', 1), ('trevor', 1), ('uncooperative', 2), ('jianchao', 1), ('recreational', 2), ('amateur', 2), ('lounderma', 1), ('unnerve', 1), ('550', 5), ('hanun', 1), ('jumping', 4), ('spoiled', 1), ('explanations', 1), ('inaccurate', 2), ('cristobal', 1), ('casas', 1), ('uribana', 1), ('panzhihua', 1), ('tit', 1), ('tat', 1), ('recall', 9), ('gaspard', 1), ('kanyarukiga', 3), ('bulldozing', 1), ('nyange', 1), ('xerox', 3), ('crum', 3), ('forster', 3), ('schooled', 1), ('comparison', 3), ('492', 1), ('cutthroat', 1), ('staunchly', 2), ('freight', 2), ('transshipment', 3), ('hooper', 3), ('overfishing', 2), ('pitcairn', 4), ('1767', 1), ('1790', 1), ('mutineers', 2), ('tahitian', 2), ('vestige', 2), ('outmigration', 1), ('233', 1), ('akrotiri', 4), ('southernmost', 3), ('cemented', 1), ('melanesian', 1), ('ensured', 3), ('melanesians', 1), ('fijian', 1), ('laisenia', 1), ('qarase', 2), ('commodore', 1), ('voreqe', 1), ('bainimarama', 2), ('favoring', 6), ('embroiled', 4), ('cpa', 2), ('influxes', 1), ('obstructed', 3), ('undertook', 4), ('sprang', 1), ('snatch', 1), ('huntsmen', 3), ('hounds', 2), ('brawny', 1), ('refrigerator', 1), ('doorbell', 1), ('revolutionaries', 3), ('pin', 2), ('kung', 2), ('1911', 2), ('hani', 2), ('sibaie', 1), ('moustafa', 1), ('yafei', 1), ('condone', 1), ('unveil', 3), ('amil', 1), ('adamiya', 1), ('stick', 3), ('villa', 1), ('calabar', 2), ('andré', 1), ('nesnera', 1), ('korea\x92s', 1), ('degradation', 4), ('kirilenko', 2), ('slot', 6), ('pectoral', 1), ('booed', 1), ('domachowska', 1), ('davenport', 4), ('venus', 10), ('seemingly', 2), ('happen', 12), ('beachside', 2), ('berms', 1), ('surfboard', 1), ('exciting', 2), ('happens', 3), ('surfers', 2), ('emphasize', 1), ('swells', 1), ('removes', 3), ('posses', 1), ('gholamhossein', 1), ('elham', 2), ('mutually', 4), ('convenient', 1), ('tribunals', 3), ('protects', 5), ('fundamentally', 2), ('540', 3), ('referees', 5), ('theoretical', 1), ('practical', 4), ('sepp', 2), ('blatter', 2), ('6000', 1), ('trim', 2), ('daimler', 1), ('desi', 1), ('bouterse', 4), ('dunem', 3), ('discharges', 3), ('gere', 7), ('shilpa', 4), ('shetty', 8), ('kissed', 4), ('cheeks', 3), ('kissing', 2), ('varanasi', 1), ('meerut', 1), ('endeavourhas', 1), ('undocked', 1), ('looped', 2), ('exterior', 1), ('goodbyes', 1), ('hatches', 1), ('recycling', 1), ('yen', 13), ('expectant', 1), ('umbilical', 1), ('cords', 4), ('cord', 4), ('troedsson', 2), ('tends', 2), ('colder', 5), ('suna', 1), ('afewerki', 1), ('ontario', 1), ('belief', 5), ('worn', 4), ('bayan', 4), ('jabr', 4), ('coastguard', 1), ('alloceans', 2), ('ariana', 4), ('spyros', 1), ('kristina', 1), ('smigun', 2), ('marit', 1), ('bjoergen', 1), ('hilde', 1), ('pedersen', 1), ('snowboard', 2), ('temples', 4), ('genders', 1), ('speedskating', 1), ('ebrahim', 1), ('sheibani', 2), ('saderat', 2), ('jabalya', 2), ('collins', 4), ('frail', 3), ('arthritis', 2), ('akash', 2), ('warhead', 4), ('orissa', 4), ('chandipur', 2), ('bhubaneshwar', 2), ('drinan', 2), ('congestive', 2), ('directive', 3), ('supersedes', 1), ('defer', 1), ('textbook', 1), ('overlooks', 1), ('calculation', 1), ('characterization', 1), ('cynical', 1), ('880', 1), ('azzedine', 1), ('belkadi', 1), ('kerimli', 1), ('pflp', 1), ('saadat', 1), ('rehavam', 1), ('zeevi', 1), ('splattered', 1), ('pierre', 9), ('pettigrew', 1), ('handover', 10), ('gibbs', 6), ('unsure', 2), ('bowling', 1), ('rolando', 1), ('otoniel', 1), ('guevara', 1), ('aharonot', 3), ('samahdna', 1), ('runup', 1), ('chileans', 3), ('libertador', 1), ("o'higgins", 1), ('shaking', 2), ('valparaiso', 1), ('auckland', 5), ('stratfor', 2), ('intelcenter', 1), ('turkestan', 2), ('adumin', 2), ('westernmost', 2), ('heeding', 1), ('rocking', 2), ('energized', 1), ('githongo', 2), ('kiraitu', 1), ('murungi', 3), ('anglo', 7), ('leasing', 5), ('asghar', 2), ('payday', 1), ('michoacan', 3), ('shihri', 3), ('divine', 1), ('everywhere', 2), ('umar', 3), ('abdulmutallab', 2), ('summed', 1), ('barbecues', 1), ('bulandshahr', 1), ('leil', 1), ('commuted', 3), ('nottingham', 3), ('atheists', 1), ('lambeth', 1), ('cloning', 2), ('hwang', 10), ('suk', 2), ('misappropriation', 1), ('veterinarian', 1), ('deceiving', 2), ('ningbo', 4), ('flour', 5), ('veils', 1), ('wives', 6), ('scuffles', 2), ('khori', 1), ('skier', 6), ('wengen', 2), ('lauberhorn', 1), ('alleys', 1), ('bib', 1), ('raich', 3), ('706', 2), ('106', 5), ('589', 1), ('practically', 2), ('plates', 2), ('soonthorn', 3), ('rittipakdee', 1), ('ganei', 2), ('botched', 3), ('unvetted', 1), ('gorges', 1), ('yuanmu', 1), ('overpopulation', 1), ('oic', 2), ('socio', 3), ('mecca', 6), ('caved', 2), ('racing', 4), ('delegitimize', 1), ('drastic', 2), ('newer', 4), ('amrullah', 1), ('tyre', 2), ('militaristic', 1), ('lanterns', 2), ('souls', 2), ('motoyasu', 1), ('candlelit', 1), ('horrific', 2), ('lantern', 1), ('bowed', 3), ('janakpur', 2), ('itahari', 1), ('fonseka', 3), ('rajapaksa', 2), ('astonished', 1), ('hongyao', 1), ('arafura', 1), ('visual', 2), ('merauke', 1), ('upjohn', 9), ('headcount', 1), ('gelles', 1), ('wertheim', 1), ('schroder', 1), ('875', 1), ('apiece', 2), ('suitors', 1), ('igad', 1), ('trimmed', 1), ('kubilius', 1), ('cutback', 1), ('eskom', 1), ('necessitating', 1), ('shedding', 2), ('reaped', 2), ('empowerment', 1), ('fiscally', 2), ('attaining', 2), ('1857', 2), ('guano', 1), ('1898', 6), ('navassa', 1), ('expedition', 3), ('biodiversity', 2), ('1903', 2), ('1914', 3), ('rested', 1), ('panamanians', 1), ('1811', 1), ('chaco', 5), ('1932', 5), ('lowland', 1), ('alfredo', 2), ('stroessner', 1), ('celtic', 2), ('pilgrimage', 4), ('omra', 1), ('norsemen', 1), ('boru', 1), ('1014', 1), ('rebellions', 2), ('repressions', 1), ('1921', 4), ('ulster', 2), ('andrews', 1), ('hart', 9), ('trotted', 1), ('noise', 5), ('scudded', 1), ('optimist', 1), ('pessimist', 1), ('857', 1), ('772', 1), ('wellington', 3), ('107', 4), ('loder', 1), ('crusader', 1), ('sutherland', 4), ('misdemeanor', 1), ('sobriety', 1), ('maiberger', 2), ('misdmeanor', 1), ('keifer', 1), ('obscenity', 3), ('metropolis', 1), ('bei', 5), ('baishiyao', 1), ('cadmium', 6), ('dilute', 3), ('smelter', 2), ('administers', 3), ('politicizing', 2), ('friedan', 4), ('groundwork', 2), ('feminism', 1), ('feminine', 2), ('mystique', 2), ('psychology', 1), ('housewife', 1), ('groundbreaking', 2), ('husbands', 2), ('unfulfilled', 1), ('caucus', 5), ('abdouramane', 1), ('goushmane', 2), ('conviasa', 1), ('controllers', 3), ('sidor', 1), ('margarita', 1), ('ordaz', 3), ('heroes', 3), ('defections', 1), ('portraits', 1), ('privileges', 5), ('yvon', 1), ('neptune', 1), ('jocelerme', 1), ('privert', 1), ('protestors', 1), ('naryn', 1), ('pour', 1), ('onshore', 1), ('changbei', 1), ('petrochina', 1), ('unreleased', 1), ('pneumonic', 1), ('2500', 1), ('infect', 4), ('tawilla', 1), ('cannon', 4), ('widens', 1), ('trimester', 1), ('abortions', 4), ('incest', 1), ('obstruction', 3), ('addington', 1), ('hannah', 1), ('canaries', 1), ('utor', 1), ('whipping', 1), ('huddled', 1), ('mindoro', 2), ('albay', 2), ('mayon', 2), ('recommend', 8), ('sludge', 1), ('walayo', 1), ('loaning', 2), ('hepatitis', 1), ('engineered', 4), ('paves', 2), ('cerda', 1), ('disappearances', 1), ('blockades', 2), ('belkhadem', 1), ('destabilizing', 4), ('ugalde', 1), ('sprinter', 3), ('gatlin', 5), ('eugene', 2), ('magistrates', 1), ('prescribed', 2), ('milky', 1), ('magellanic', 1), ('cloud', 6), ('fragmentary', 1), ('parnaz', 1), ('azima', 3), ('haleh', 1), ('esfandiari', 1), ('woodrow', 1), ('kian', 1), ('tajbakhsh', 1), ('shakeri', 3), ('malibu', 1), ('aided', 5), ('concentrating', 1), ('hyde', 2), ('ohlmert', 1), ('qasr', 1), ('irrigation', 2), ('uncomplicated', 1), ('samawa', 3), ('patu', 1), ('cull', 1), ('extolling', 1), ('diyar', 1), ('altitudes', 2), ('nourished', 1), ('rainstorms', 2), ('napa', 2), ('sonoma', 1), ('swelling', 2), ('indict', 2), ('receded', 1), ('rained', 1), ('1955', 5), ('backlash', 5), ('shieh', 1), ('jhy', 1), ('wey', 1), ('tu', 2), ('cheng', 1), ('shu', 1), ('ezzedine', 1), ('elusive', 2), ('contemplate', 1), ('dairy', 4), ('prithvi', 2), ('aggravate', 1), ('ramush', 4), ('haradinaj', 11), ('guerilla', 3), ('idriz', 1), ('balaj', 1), ('lahi', 1), ('brahimaj', 1), ('subordinates', 3), ('persecuted', 2), ('pune', 1), ('503', 1), ('816', 1), ('scarcely', 3), ('misquoted', 1), ('careful', 4), ('dyes', 2), ('conceal', 2), ('kuril', 2), ('tremors', 3), ('magnitudes', 1), ('destructive', 3), ('coastlines', 4), ('disagreement', 9), ('561', 2), ('exceeds', 4), ('regev', 3), ('taxpayers', 2), ('aia', 2), ('hakkari', 2), ('tidjane', 1), ('thiam', 1), ('hoon', 4), ('falkland', 6), ('landfill', 3), ('wheeler', 5), ('wilmington', 2), ('exiting', 1), ('backers', 7), ('recife', 1), ('gabgbo', 1), ('sculpture', 1), ('gadgets', 1), ('interactive', 3), ('matteo', 1), ('duran', 1), ('graduation', 2), ('benita', 2), ('walder', 1), ('mercosur', 13), ('breaststroke', 2), ('brendan', 1), ('kosuke', 1), ('kitajima', 1), ('omaha', 1), ('medley', 1), ('katie', 1), ('hoff', 1), ('freestyle', 1), ('regents', 1), ('verez', 3), ('bencomo', 3), ('innovations', 1), ('meningitis', 2), ('themba', 1), ('nyathi', 3), ('agca', 5), ('demirbag', 1), ('forgave', 1), ('bette', 1), ('midler', 2), ('celine', 2), ('dion', 2), ('headliner', 2), ('caesar', 1), ('bawdy', 1), ('inaugurating', 2), ('colosseum', 2), ('elton', 5), ('meglen', 1), ('probable', 1), ('insiders', 1), ('cher', 1), ('vaile', 2), ('filter', 3), ('vuvuzelas', 2), ('545', 1), ('trumpets', 1), ('reduces', 1), ('ambient', 1), ('commentary', 4), ('camel', 4), ('racers', 1), ('experimenting', 1), ('robots', 2), ('jockeys', 3), ('lighter', 2), ('underage', 1), ('underfed', 1), ('jockey', 1), ('johnny', 1), ('horne', 3), ('unpremeditated', 1), ('garbage', 1), ('misery', 4), ('chikunova', 2), ('akhrorkhodzha', 1), ('tolipkhodzhayev', 1), ('offline', 1), ('danforth', 2), ('statesmen', 1), ('tearfully', 1), ('chesnot', 2), ('georges', 3), ('malbrunot', 2), ('doaba', 1), ('hangu', 1), ('klaus', 1), ('toepfer', 1), ('poisonous', 5), ('riverside', 1), ('wazirstan', 2), ('inaccessible', 4), ('accessing', 2), ('profiles', 1), ('377', 1), ('rak', 2), ('mushtaq', 1), ('garrison', 4), ('bechuanaland', 1), ('uninterrupted', 2), ('dominates', 3), ('preserves', 1), ('1895', 1), ('reverted', 1), ('democratized', 1), ('watermelons', 1), ('yams', 3), ('bind', 3), ('unload', 2), ('occupies', 3), ('earners', 2), ('quarry', 1), ('subsuming', 1), ('legislated', 1), ('reasoning', 1), ('overgrazing', 1), ('linen', 1), ('travelling', 1), ('tramp', 3), ('superb', 1), ('unconcern', 1), ('characteristic', 1), ('genius', 2), ('contemptuously', 1), ('carved', 3), ('smooth', 5), ('bark', 1), ('birch', 1), ('gump', 1), ('correctness', 2), ('overheard', 1), ('pie', 1), ('disbursed', 1), ('verification', 3), ('akitaka', 1), ('saiki', 2), ('hammering', 1), ('creatively', 1), ('bwakira', 2), ('wet', 4), ('tankbuster', 1), ('kheyal', 1), ('baaz', 1), ('sherzai', 2), ('gardez', 1), ('weed', 2), ('cantarell', 1), ('administrators', 1), ('ourselves', 4), ('narcotic', 2), ('ilayan', 1), ('bribed', 2), ('duda', 1), ('mendonca', 1), ('beds', 2), ('floors', 1), ('crumbled', 1), ('inferior', 1), ('correcting', 1), ('dengue', 1), ('fanning', 2), ('larvae', 1), ('breed', 1), ('284', 2), ('chul', 1), ('woon', 1), ('626', 1), ("n'zerekore", 1), ('yentai', 1), ('kapilvastu', 2), ('bhaikaji', 1), ('ghimire', 1), ('directing', 2), ('prostitution', 2), ('prostitutes', 1), ('handicaps', 1), ('pioneering', 1), ('lagham', 1), ('mengistu', 3), ('haile', 2), ('politicize', 2), ('chaudry', 1), ('156', 3), ('sebastiao', 1), ('veloso', 1), ('mutilating', 2), ('archives', 3), ('preview', 3), ('abdali', 1), ('arak', 2), ('powder', 1), ('keg', 1), ('servicmen', 1), ('jennings', 1), ('securities', 5), ('trader', 3), ('borrows', 1), ('cuomo', 1), ('brokers', 1), ('deporting', 3), ('wemple', 1), ('stoffel', 1), ('clarified', 1), ('kerem', 1), ('chacon', 3), ('yaracuy', 1), ('lapi', 1), ('carabobo', 1), ('henrique', 1), ('salas', 1), ('akerson', 3), ('turnaround', 3), ('jailbreak', 1), ('banghazi', 1), ('ensures', 2), ('unbeatable', 1), ('abdi', 1), ('dagoberto', 1), ('swirled', 1), ('torah', 2), ('enclaves', 8), ('narrowing', 1), ('redistributed', 1), ('overcrowding', 2), ('mutua', 4), ('witch', 1), ('discriminate', 1), ('shoddy', 3), ('resignations', 6), ('francis', 5), ('muthaura', 1), ('kospi', 1), ('tine', 2), ('noureddine', 1), ('deshu', 2), ('kakar', 2), ('marshmallow', 1), ('yam', 1), ('sibghatullah', 1), ('mujaddedi', 2), ('marshmallows', 1), ('cpp', 2), ('bel', 3), ('intersection', 3), ('benigno', 6), ('aquino', 13), ('broadband', 3), ('hilario', 1), ('davide', 1), ('alluded', 1), ('psychiatrist', 1), ('carreno', 1), ('backyards', 1), ('talhi', 1), ('abdallah', 4), ('sughayr', 1), ('khashiban', 1), ('freezes', 1), ('forbids', 7), ('qari', 5), ('frantic', 1), ('chosun', 1), ('ilbo', 2), ('outline', 4), ('saud', 11), ('continuation', 3), ('kuduna', 1), ('abdulhamid', 1), ('abubakar', 5), ('cough', 1), ('lubroth', 1), ('borrow', 5), ('collateral', 1), ('sinbad', 3), ('wikipedia', 3), ('demise', 1), ('erroneous', 2), ('forwarded', 2), ('adkins', 4), ('rosh', 2), ('hashanah', 2), ('awe', 1), ('strangling', 2), ('wolde', 1), ('meshesha', 1), ('ozcan', 1), ('ash', 7), ('icelandic', 2), ('disrupts', 1), ('aena', 1), ('belching', 1), ('abrasive', 1), ('cordoned', 5), ('minar', 1), ('kye', 3), ('lecture', 1), ('stanford', 2), ('haas', 5), ('yongbyong', 1), ('dinners', 3), ('temptations', 1), ('chesney', 2), ('parachute', 1), ('chill', 2), ('clarence', 2), ('gatemouth', 2), ('zydeco', 1), ('cajun', 1), ('okie', 1), ('dokie', 1), ('stomp', 1), ('dandy', 1), ('supervisor', 3), ('zaw', 1), ('shmatko', 2), ('masud', 1), ('malda', 2), ('murshidabad', 1), ('tyranny', 2), ('greatness', 1), ('bullring', 1), ('levan', 2), ('gachechiladze', 2), ('theofilos', 1), ('malenchenko', 1), ('flowstations', 2), ('kula', 1), ('chevrontexaco', 2), ('babel', 1), ('psv', 1), ('eindhoven', 2), ('afellay', 1), ('sota', 1), ('hirayama', 1), ('68th', 1), ('omotoyossi', 1), ('32nd', 1), ('fargo', 2), ('59th', 1), ('5th', 1), ('squatters', 2), ('outposts', 3), ('hilltops', 1), ('evictions', 1), ('thuggish', 1), ('fitch', 1), ('aa', 1), ('bbb', 1), ('intact', 3), ('shidiac', 1), ('lbc', 1), ('judging', 1), ('configuring', 1), ('plainclothes', 2), ('homecoming', 4), ('brining', 1), ('eloy', 1), ('820', 1), ('dushanbe', 2), ('talbak', 1), ('nazarov', 1), ('rakhmonov', 1), ('ralston', 6), ('purina', 1), ('422', 1), ('seafood', 2), ('greenville', 1), ('cake', 2), ('cincinnati', 1), ('rechargeable', 2), ('volume', 2), ('bread', 3), ('eveready', 1), ('1494', 1), ('taino', 3), ('exterminated', 2), ('1655', 1), ('1834', 2), ('geographically', 2), ('mohmmed', 1), ('dahlan', 3), ('bolstered', 4), ('ethanol', 2), ('expiration', 2), ('461', 1), ('statist', 2), ('distortions', 1), ('workshops', 2), ('rigidities', 1), ('weigh', 4), ('mahmud', 3), ('nejad', 1), ('digit', 6), ('excluding', 3), ('41st', 2), ('fended', 1), ('ace', 1), ('hydrocarbons', 5), ('limitations', 3), ('decaying', 1), ('neglected', 1), ('telecoms', 1), ('kazakhstani', 1), ('crunch', 3), ('tenge', 1), ('overreliance', 1), ('petrochemicals', 1), ('lucayan', 1), ('1492', 4), ('1647', 1), ('geography', 2), ('inca', 1), ('conquest', 5), ('1533', 1), ('viceroyalty', 2), ('1717', 1), ('1822', 2), ('offspring', 2), ('handsomest', 2), ('monkey', 3), ('tenderness', 2), ('nosed', 1), ('hairless', 1), ('laugh', 1), ('saluted', 3), ('allot', 2), ('dearest', 1), ('additives', 1), ('scattering', 2), ('paths', 4), ('recede', 1), ('828', 1), ('jixi', 1), ('nehe', 1), ('floruan', 1), ('barbey', 1), ('fataki', 1), ('154', 3), ('demobilize', 2), ('aiff', 2), ('pradip', 1), ('choudhury', 4), ('houghton', 3), ('carmo', 1), ('fernandes', 1), ('negatively', 4), ('morale', 2), ('deandre', 1), ('soulja', 3), ('crank', 1), ('itunes', 1), ('z', 2), ('globalized', 1), ('pinpoint', 1), ('futures', 4), ('refine', 2), ('abductee', 1), ('admited', 1), ('reparation', 2), ('militarism', 2), ('contrite', 1), ('curtailed', 6), ('stumbling', 2), ('solider', 4), ('sidelined', 3), ('funk', 1), ('divers', 2), ('recorders', 3), ('khayrat', 1), ('shater', 1), ('linguists', 1), ('theoneste', 1), ('bagosora', 3), ('codefendants', 1), ('200s', 1), ('bancoro', 2), ('giordani', 1), ('liquidity', 2), ('nationalized', 7), ('recoverable', 1), ('halliburton', 5), ('538', 2), ('carlo', 4), ('azeglio', 1), ('ciampi', 1), ('prestige', 1), ('fadilah', 2), ('immunized', 3), ('264', 1), ('inhumanely', 1), ('corporal', 4), ('payne', 2), ('receptionist', 2), ('manslaughter', 6), ('routed', 3), ('occupiers', 2), ('aaron', 1), ('galindo', 1), ('nandrolone', 1), ('confrontational', 2), ('somebody', 1), ('rebirth', 1), ('advocating', 5), ('unwavering', 1), ('arinze', 1), ('favorite', 6), ('easterly', 1), ('1800', 1), ('converging', 4), ('aktham', 1), ('naisse', 2), ('ennals', 1), ('somalian', 1), ('hatching', 1), ('naise', 1), ('embodied', 1), ('sparrows', 3), ('bussereau', 1), ('perfectly', 1), ('plummets', 1), ('giants', 4), ('finalizes', 1), ('modification', 1), ('barney', 1), ('danielle', 5), ('leeward', 3), ('disseminating', 1), ('usher', 4), ('platinum', 1), ('stylist', 1), ('tameka', 1), ('smalls', 1), ('mtv', 7), ('associations', 4), ('311', 2), ('carme', 1), ('yoadimadji', 1), ('yoadimnadji', 3), ('wilds', 1), ('dell', 3), ('silicon', 1), ('outsourcing', 1), ('numbering', 1), ('\x96', 8), ('veerman', 3), ('capua', 1), ('shih', 1), ('chien', 1), ('emphasizing', 3), ('wahid', 1), ('golo', 1), ('jebel', 3), ('marra', 3), ('moun', 1), ('omari', 1), ('276', 1), ('farooq', 3), ('tap', 2), ('lashing', 2), ('sociology', 1), ('razumkov', 1), ('manning', 5), ('croatians', 1), ('zadar', 1), ('dense', 3), ('zabihullah', 1), ('mujahed', 1), ('canyons', 1), ('naxalites', 1), ('exemplifies', 1), ('massacres', 2), ('welcometousa', 1), ('interagency', 1), ('zahedan', 2), ('guides', 1), ('leaner', 1), ('trimming', 1), ('underreporting', 1), ('moratinos', 1), ('melilla', 8), ('ceuta', 7), ('scaled', 3), ('razor', 6), ('fences', 5), ('novelist', 2), ('kiran', 1), ('desai', 4), ('booker', 2), ('novel', 3), ('inheritance', 1), ('magnificent', 1), ('humane', 3), ('breadth', 1), ('wisdom', 4), ('acuteness', 1), ('crumbling', 1), ('orphaned', 2), ('granddaughter', 2), ('anita', 1), ('vidoje', 2), ('blagojevic', 3), ('genocidal', 1), ('embera', 1), ('jundollah', 2), ('jihadists', 1), ('logical', 3), ('adapt', 2), ('blend', 1), ('norfolk', 1), ('receptive', 1), ('dictators', 1), ('multiply', 1), ('auditorium', 1), ('ogun', 2), ('decontaminating', 1), ('torun', 2), ('boone', 1), ('pickens', 6), ('oilman', 1), ('reno', 1), ('betraying', 3), ('jafarzadeh', 3), ('laser', 1), ('revisit', 2), ('sadrists', 1), ('loach', 1), ('shakes', 1), ('barley', 2), ('palme', 1), ("d'or", 1), ('flanders', 1), ('inarritu', 1), ('volver', 1), ('collectively', 1), ('cauvin', 2), ('narino', 1), ('cao', 1), ('gangchuan', 1), ('ojea', 2), ('quintana', 2), ('rakhine', 3), ('butheetaung', 1), ('oo', 4), ('hulk', 1), ('hogan', 2), ('clearwater', 2), ('bollea', 4), ('supra', 1), ('median', 3), ('shelor', 2), ('graziano', 2), ('extricated', 1), ('bayfront', 1), ('jassar', 3), ('rawi', 1), ('jibouri', 1), ('speedskater', 3), ('joey', 2), ('cheek', 8), ('champ', 1), ('johann', 1), ('koss', 1), ('emphasizes', 2), ('zetas', 4), ('cardenas', 5), ('hidalgo', 1), ('heriberto', 1), ('lazcano', 1), ('endure', 3), ('samoa', 2), ('retaken', 1), ('primitive', 1), ('caroline', 1), ('nkurunziza', 2), ('bulls', 1), ('pastured', 1), ('guileful', 1), ('feasted', 1), ('237', 1), ('lease', 4), ('overland', 2), ('bentegeat', 1), ('refusals', 1), ('granville', 1), ('abdelrahman', 1), ('rahama', 1), ('confessing', 1), ('disgrace', 2), ('ireju', 1), ('bares', 1), ('furious', 1), ('teaches', 3), ('messaging', 1), ('dalailama', 1), ('evan', 1), ('floundering', 1), ('reasonably', 3), ('kizza', 4), ('besigye', 16), ('gwan', 2), ('commandoes', 2), ('script', 1), ('mauled', 2), ('xijing', 1), ("xi'an", 1), ('lip', 5), ('eyebrow', 1), ('recluse', 1), ('disfigurement', 1), ('loose', 6), ('sadness', 4), ('tirelessly', 1), ('beings', 2), ('denuclearize', 1), ('papacy', 6), ('gianfranco', 1), ('fini', 2), ('bezoti', 1), ('marshburn', 1), ('40th', 1), ('kopra', 3), ('koichi', 2), ('wakata', 2), ('tuning', 1), ('macchiavello', 1), ('overthrowing', 1), ('patterns', 2), ('crossroads', 2), ('guaranteeing', 3), ('narrows', 1), ('rohmer', 5), ('relationships', 8), ('philosophical', 2), ('maud', 1), ('claire', 2), ('unionist', 3), ('paisley', 4), ('sinn', 4), ('fein', 4), ('chastelain', 1), ('unitary', 1), ('pristina', 6), ('borys', 1), ('247', 2), ('lutsenko', 1), ('tsushko', 1), ('staunchest', 1), ('despises', 1), ('tabloids', 1), ('fodder', 3), ('soap', 1), ('operas', 1), ('alien', 1), ('confer', 3), ('ruggiero', 3), ('fulfills', 2), ('982', 1), ('straits', 2), ('hafeez', 2), ('heir', 2), ('recommending', 4), ('ascend', 2), ('chrysanthemum', 2), ('vovk', 1), ('rs', 1), ('12m', 1), ('kapustin', 1), ('yar', 1), ('teaching', 6), ('rosa', 2), ('floats', 2), ('strings', 1), ('lesser', 7), ('climatic', 2), ('lenten', 1), ('packs', 1), ('grassland', 1), ('cannons', 3), ('demostrators', 1), ('slutskaya', 2), ('skate', 1), ('evgeny', 1), ('plushenko', 1), ('skaters', 2), ('entrants', 2), ('elena', 2), ('sokolova', 1), ('viktoria', 1), ('volchkova', 1), ('navka', 1), ('kostomarov', 1), ('dancers', 1), ('technocrats', 2), ('creativity', 2), ('greener', 1), ('equalize', 1), ('shota', 1), ('utiashvili', 1), ('turbine', 1), ('poti', 1), ('jane', 3), ('fonda', 3), ('scolded', 3), ('antics', 1), ('publicized', 4), ('capp', 1), ('wooten', 1), ('massey', 3), ('raleigh', 1), ('charleston', 3), ('manchin', 1), ('gazette', 1), ('sago', 1), ('fractious', 1), ('define', 1), ('distinguish', 2), ('absent', 5), ('marker', 1), ('estonian', 1), ('ruutel', 1), ('andrus', 1), ('ansip', 3), ('juhan', 1), ('andriy', 1), ('shevchenko', 1), ('ronaldo', 2), ('balking', 1), ('qeshm', 1), ('unhitches', 1), ('1600', 1), ('unintentional', 1), ('demonized', 1), ('molded', 1), ('explaining', 3), ('roseneft', 2), ('yugansk', 3), ('managerial', 1), ('asses', 3), ('mate', 4), ('plouffe', 1), ('erik', 5), ('solheim', 5), ('misallocation', 1), ('renegotiate', 1), ('wmd', 1), ('fiercest', 2), ('blanketed', 3), ('adhamiyah', 1), ('waziriyah', 1), ('muscular', 1), ('shafi', 1), ('vahiuddin', 1), ('calcutta', 2), ('toiba', 6), ('ghettos', 2), ('inward', 1), ('cartoonists', 1), ('depictions', 4), ('civilizations', 1), ('bandage', 1), ('kulov', 4), ('runners', 5), ('diddy', 2), ('combs', 6), ('mogul', 2), ('rechnitzer', 3), ('salvage', 2), ('retreating', 1), ('bosco', 2), ('katutsi', 2), ('iowa', 17), ('gamma', 3), ('dissipate', 1), ('coasts', 1), ('coppola', 1), ('limon', 1), ('elgon', 3), ('sirisia', 1), ('sabaot', 1), ('exempt', 3), ('avigdor', 3), ('yisrael', 1), ('beitenu', 1), ('goverment', 1), ('calf', 1), ('kulyab', 2), ('displeased', 1), ('ordinance', 2), ('newfoundland', 1), ('348', 2), ('sq', 1), ('subsidizes', 2), ('cashew', 1), ('shepherds', 3), ('hut', 1), ('haunch', 1), ('mutton', 1), ('peanuts', 2), ('kernels', 1), ('undp', 1), ('dahomey', 1), ('1872', 4), ('clamor', 1), ('yayi', 3), ('boni', 2), ('outsider', 1), ('cumulative', 1), ('dampened', 2), ('nigh', 1), ('squid', 2), ('furnish', 1), ('wool', 2), ('dane', 2), ('dampen', 1), ('abated', 1), ('commercially', 4), ('eco', 2), ('perch', 1), ('jackdaw', 2), ('envy', 2), ('emulate', 3), ('whir', 2), ('wings', 7), ('entangled', 1), ('fleece', 1), ('fluttered', 1), ('clipped', 1), ('daw', 2), ('woodcutter', 1), ('expedient', 1), ('importunities', 1), ('suitor', 1), ('extract', 3), ('fearfully', 4), ('cheerfully', 1), ('assented', 2), ('toothless', 1), ('clawless', 1), ('woodman', 1), ('sowing', 2), ('hemp', 4), ('hopping', 2), ('repent', 1), ('heed', 3), ('despised', 2), ('aisle', 1), ('messed', 1), ('crapping', 1), ('tayr', 1), ('filsi', 1), ('recommends', 3), ('rehnquist', 7), ('pausing', 2), ('nixon', 2), ('roberts', 9), ('panhandle', 2), ('solemly', 1), ('hallandale', 2), ('boating', 2), ('obsolete', 1), ('shalikashvili', 1), ('inscribed', 2), ('romano', 3), ('prodi', 6), ('tacitly', 1), ('cardboard', 1), ('deliberating', 1), ('reinstatement', 3), ('savo', 1), ('todovic', 3), ('comprehend', 1), ('timoshenko', 2), ('distracted', 1), ('balikesir', 1), ('antoin', 1), ('rezko', 5), ('dom', 1), ('foca', 4), ('interrogations', 5), ('afrik', 1), ('chariot', 6), ('antiquities', 7), ('tutankhamun', 1), ('tut', 2), ('1922', 4), ('mussa', 6), ('taif', 2), ('skyline', 1), ('stephanie', 2), ('demerits', 1), ('misused', 1), ('breaching', 2), ('blanked', 1), ('emmen', 1), ('argentines', 2), ('harrison', 2), ('otalvaro', 1), ('52nd', 1), ('lionel', 1), ('messi', 1), ('doetinchem', 1), ('taye', 1), ('taiwo', 1), ('g7', 4), ('kolesnikov', 1), ('331', 1), ('wirajuda', 1), ('bahlul', 2), ('medic', 4), ('sassi', 1), ('347', 1), ('oviedo', 1), ('norbert', 1), ('lammert', 1), ('diminish', 2), ('girona', 1), ('bigots', 1), ('horror', 4), ('apure', 1), ('catalonians', 1), ('backpacks', 2), ('luton', 2), ('rocio', 1), ('esteban', 1), ('cooperativa', 1), ('boniface', 1), ('alexandre', 3), ('ramazan', 2), ('localized', 1), ('wikileaks', 3), ('aftenposten', 1), ('sami', 2), ('arian', 3), ('noneducational', 1), ('understood', 2), ('reschedule', 3), ('proliferate', 1), ('novin', 2), ('mesbah', 2), ('judgment', 8), ('regina', 1), ('reichenm', 1), ('reassurance', 1), ('douglas', 2), ('infomation', 1), ('569', 1), ('badakshan', 2), ('mohean', 1), ('depth', 3), ('vladislav', 3), ('ardzinba', 4), ('khadjimba', 4), ('nodar', 1), ('khashba', 1), ('bagapsh', 9), ('voided', 1), ('186', 2), ('gali', 1), ('kharj', 2), ('majmaah', 1), ('assorted', 1), ('rodon', 1), ('sinmun', 2), ('vicious', 3), ('somaliland', 2), ('hargeisa', 1), ('maigao', 1), ('paksitan', 1), ('pollsters', 3), ('1718', 1), ('safta', 1), ('2013', 3), ('forge', 3), ('naysayers', 1), ('cynics', 1), ('strenuously', 1), ('disappear', 3), ('renovations', 2), ('balancing', 1), ('reauthorize', 2), ('redeployment', 2), ('assumptions', 1), ('disturbingly', 1), ('hezb', 2), ('olympia', 2), ('relays', 1), ('merajudeen', 1), ('patan', 2), ('stanishev', 2), ('reprocessing', 3), ('odom', 2), ('squander', 1), ('invading', 3), ('compounded', 3), ('181', 2), ('hospitalizations', 1), ('diverting', 2), ('gripped', 1), ('hurry', 1), ('50th', 1), ('ysidro', 1), ('embolden', 2), ('adjust', 5), ('diameter', 1), ('fushun', 2), ('sinuiju', 1), ('rob', 2), ('portman', 1), ('iiss', 1), ('hails', 1), ('tipping', 1), ('badaun', 1), ('organiation', 1), ('pedestrians', 4), ('shahawar', 1), ('matin', 1), ('siraj', 3), ('brooklyn', 1), ('stoked', 1), ('impress', 1), ('eldawoody', 3), ('flagship', 2), ('frazier', 2), ('observes', 3), ("qur'an", 1), ('scud', 1), ('showering', 1), ('machines', 8), ('heist', 2), ('thankful', 1), ('agendas', 1), ('discretion', 2), ('bradford', 1), ('ernst', 1), ('disburses', 1), ('kasami', 1), ('malegaon', 2), ('shab', 1), ('barat', 1), ('1621', 1), ('sophistication', 1), ('cpsc', 1), ('toys', 4), ('dallas', 4), ('minivan', 2), ('trailer', 2), ('undocumented', 2), ('chieftain', 1), ('darfuri', 1), ('rizeigat', 2), ('eissa', 1), ('aliu', 2), ('mas', 3), ('bahr', 4), ('ghazal', 1), ('hinges', 2), ('tandem', 1), ('jebaliya', 1), ('podemos', 1), ('akylbek', 1), ('sariyev', 2), ('ricans', 1), ('plebiscites', 1), ('guei', 1), ('blatantly', 1), ('disaffected', 1), ('linas', 1), ('marcoussis', 1), ('guillaume', 1), ('soro', 2), ('ouagadougou', 1), ('reintegration', 1), ('dramane', 1), ('pleasant', 1), ('nonpolluting', 1), ('thrives', 1), ('jurisdictions', 1), ('monopolies', 1), ('computerized', 1), ('tamper', 1), ('biometric', 1), ('reassert', 1), ('annapolis', 2), ('optician', 1), ('telescopes', 1), ('munificent', 1), ('patronage', 1), ('languishing', 2), ('kangaroo', 1), ('awkwardly', 1), ('bulky', 2), ('pouch', 1), ('desirous', 1), ('deceitful', 1), ('smiling', 2), ('consciousness', 5), ('insupportable', 1), ('wit', 3), ('cheerless', 1), ('unappreciated', 1), ('implored', 1), ('creator', 2), ('endow', 1), ('wag', 2), ('resentment', 1), ('affection', 4), ('fulness', 1), ('thereof', 1), ('incaudate', 1), ('conferred', 5), ('chin', 1), ('wags', 1), ('gratification', 1), ('pluto', 5), ('charon', 1), ('geology', 1), ('kuiper', 1), ('payenda', 1), ('rocketed', 1), ('caves', 1), ('subverting', 1), ('mahamadou', 1), ('issoufou', 1), ('sarturday', 1), ('shahidi', 2), ('hassas', 1), ('checkpost', 1), ('khela', 1), ('barbershops', 1), ('jabber', 1), ('alias', 3), ('belgaum', 1), ('mohamuud', 1), ('musse', 1), ('gurage', 1), ('dastardly', 1), ('responsibilty', 1), ('ossetian', 1), ('katsina', 1), ('annulment', 2), ('acquittals', 1), ('convict', 1), ('hostilities', 6), ('dormant', 2), ('mazda', 1), ('outsold', 1), ('volkswagen', 1), ('agadez', 1), ('uninsured', 2), ('initials', 3), ('mnj', 1), ('bales', 2), ('crusted', 1), ('pastures', 2), ('immobilized', 1), ('drifts', 1), ('injection', 4), ('siding', 1), ('antonin', 1), ('scalia', 1), ('hermogenes', 1), ('esperon', 1), ('dulmatin', 1), ('patek', 1), ('suspiciously', 1), ('behaving', 1), ('insistence', 4), ('stopper', 1), ('roxana', 2), ('saberi', 7), ('evin', 1), ('genetically', 4), ('hats', 1), ('cobs', 1), ('fillon', 5), ('recognizable', 1), ('pests', 1), ('monsanto', 1), ('frattini', 1), ('oumar', 4), ('konare', 6), ('deterioration', 4), ('detached', 2), ('chairwoman', 2), ('colllins', 1), ('strangely', 1), ('ineffectively', 1), ('galveston', 1), ('aurangabad', 1), ('laredo', 9), ('berrones', 1), ('casings', 2), ('lao', 3), ('apache', 2), ('abusive', 5), ('yankee', 2), ('handkerchiefs', 1), ('colors', 1), ('dazzled', 1), ('filmmakers', 3), ('documentaries', 1), ('restless', 1), ('frontline', 2), ('pbs', 2), ('suli', 3), ('katyusha', 3), ('controller', 1), ('nevzlin', 4), ('sunda', 1), ('arc', 2), ('volcanos', 1), ('encircling', 1), ('val', 4), ('grace', 2), ('gaule', 1), ('dabaan', 1), ('nuri', 2), ('naqoura', 3), ('baalbek', 1), ('swearing', 4), ('ural', 1), ('distorted', 1), ('potent', 2), ('unbiased', 1), ('inquiries', 2), ('migrate', 1), ('roshan', 1), ('interpretations', 2), ('pepsico', 6), ('pepsi', 1), ('indra', 1), ('nooyi', 3), ('madras', 1), ('undergraduate', 1), ('graduate', 1), ('yale', 1), ('skillfully', 1), ('sightings', 1), ('congregate', 1), ('surroundings', 2), ('lunchtime', 2), ('grower', 1), ('masjid', 1), ('eyewitness', 1), ('excrement', 2), ('preachers', 2), ('forgiving', 1), ('motivation', 2), ('geologist', 1), ('piotr', 1), ('stanczak', 1), ('geofizyka', 1), ('krakow', 1), ('contributors', 1), ('rehearsing', 1), ('revelry', 2), ('penitence', 1), ('unami', 1), ('rancher', 2), ('muga', 1), ('apondi', 2), ('cholmondeley', 3), ('delamere', 1), ('harbored', 1), ('sympathetic', 3), ('nonassociated', 1), ('2022', 1), ('causeway', 1), ('maastricht', 2), ('amerindians', 1), ('annihilated', 1), ('1697', 2), ('revolted', 1), ("l'ouverture", 1), ('1804', 2), ('postponements', 2), ('inaugurate', 1), ('neighbours', 1), ('avarice', 1), ('neighbour', 3), ('avaricious', 1), ('envious', 1), ('vices', 1), ('farmyard', 3), ('grievously', 2), ('spectator', 1), ('shake', 3), ('devoured', 2), ('surfeited', 2), ('scratched', 1), ('jog', 1), ('enables', 1), ('nursing', 2), ('ivaylo', 1), ('kalfin', 1), ('calculated', 1), ('preparatory', 1), ('convenience', 1), ('seibu', 3), ('sogo', 3), ('afghani', 1), ('consult', 5), ('magloire', 1), ('retailing', 1), ('chains', 2), ('diary', 3), ('davit', 1), ('kezerashvili', 2), ('nomura', 1), ('parachinar', 4), ('sahibzada', 2), ('anis', 2), ('kasai', 1), ('occidental', 1), ('constatin', 1), ('kanow', 2), ('217', 1), ('irin', 1), ('burials', 1), ('ears', 6), ('dominating', 1), ('ilya', 1), ('kovalchuk', 1), ('goaltender', 1), ('evgeni', 1), ('nabokov', 1), ('shutout', 1), ('surging', 6), ('clinched', 2), ('suspecting', 3), ('suleimaniya', 1), ('abating', 3), ('abolishes', 1), ('blacklisted', 3), ('tier', 1), ('482', 1), ('203', 4), ('salami', 2), ('tor', 2), ('m1', 1), ('belfort', 1), ('whistler', 1), ('skied', 2), ('ivica', 2), ('100ths', 3), ('zurbriggen', 1), ('nkosazana', 1), ('dlamini', 1), ('transitioning', 1), ('extraditions', 1), ('revert', 1), ('mamoun', 1), ('darkazanli', 2), ('sphere', 4), ('enlargement', 3), ('unstoppable', 1), ('paule', 1), ('kieny', 1), ('obesity', 7), ('irreversible', 1), ('zuckerberg', 1), ('entrepreneur', 2), ('billionaires', 1), ('unfolding', 2), ('bacterium', 1), ('ubiquitous', 1), ('frenk', 1), ('guni', 1), ('rusere', 1), ('revoking', 2), ('otto', 1), ('molina', 5), ('finishers', 1), ('cpj', 1), ('090', 1), ('prosper', 1), ('alcoa', 1), ('santuario', 1), ('guatica', 1), ('restrepo', 1), ('reintegrate', 1), ('downbeat', 1), ('hausa', 1), ('impressions', 1), ('showered', 1), ('unprotected', 1), ('undermines', 2), ('intercourse', 1), ('ibaraki', 2), ('contingency', 1), ('sabawi', 2), ('jvp', 2), ('sars', 3), ('verge', 1), ('clinching', 1), ('huckabee', 1), ('sayyed', 1), ('tantawi', 1), ('bioterrorism', 1), ('purifying', 1), ('accommodating', 1), ('emptive', 3), ('bleak', 2), ('unhelpful', 2), ('navtej', 1), ('sarna', 1), ('trapping', 4), ('billboards', 3), ('decorations', 6), ('swastika', 2), ('fascists', 1), ('neon', 1), ('sizes', 1), ('underfunding', 1), ('baltasar', 1), ('garzon', 1), ('ramzi', 1), ('binalshibh', 1), ('tahar', 1), ('ezirouali', 1), ('heptathlon', 1), ('blonska', 3), ('natalia', 1), ('dobrynska', 1), ('alwan', 1), ('tamimi', 2), ('baquoba', 1), ('motorcycles', 6), ('rowsch', 2), ('shaways', 3), ('hourmadji', 1), ('doumgor', 1), ('chadians', 1), ('duekoue', 4), ('khaleda', 1), ('zia', 5), ('tarique', 1), ('taka', 1), ('zhuang', 1), ('collaborative', 1), ('cabezas', 1), ('unsatisfactory', 1), ('inflationary', 2), ('zeros', 1), ('dzhennet', 1), ('abdurakhmanova', 1), ('umalat', 1), ('magomedov', 1), ('markha', 1), ('ustarkhanova', 2), ('ramzan', 4), ('kadyrov', 7), ('videotapes', 4), ('inflame', 2), ('leashes', 1), ('blogger', 6), ('kareem', 3), ('illustrates', 1), ('relented', 1), ('reappointing', 1), ('hamed', 3), ('aberrahman', 1), ('unprofessional', 2), ('epsilon', 4), ('catastrophes', 1), ('happening', 1), ('doctrine', 4), ('envisions', 2), ('penetrating', 2), ('busters', 1), ('tauran', 2), ('zaragoza', 4), ('gmt', 1), ('andrey', 1), ('denisov', 1), ('underline', 1), ('373', 3), ('erode', 3), ("madai'ni", 1), ('overrunning', 1), ('249', 1), ('particulate', 1), ('cavic', 1), ('pluralism', 2), ('azizullah', 1), ('lodin', 1), ('evangelical', 1), ('nita', 2), ('zelenak', 1), ('nipayia', 1), ('asir', 1), ('raouf', 3), ('handwriting', 1), ('shorja', 1), ('inflaming', 1), ('cud', 3), ('uedf', 1), ('vaccinating', 1), ('jeanet', 1), ('goot', 1), ('infectiousness', 1), ('thereby', 1), ('inoculation', 1), ('hebrides', 3), ('isle', 5), ('1765', 1), ('extinct', 2), ('manx', 1), ('gaelic', 1), ('cultures', 3), ('idi', 1), ('promulgated', 1), ('amending', 1), ('loosened', 2), ('respectable', 1), ('didier', 3), ('ratsiraka', 2), ('antananarivo', 1), ('lessened', 2), ('simplification', 1), ('mitigated', 1), ('graduates', 1), ('bedside', 3), ('vineyards', 1), ('spades', 1), ('mattocks', 1), ('repaid', 1), ('superabundant', 1), ('mechanisms', 1), ('preserved', 4), ('melody', 2), ('surety', 1), ('pleases', 1), ('begging', 1), ('coward', 1), ('cookstown', 1), ('tyrone', 1), ('paralysed', 1), ('waist', 1), ('wheelchair', 4), ('stool', 2), ('newell', 2), ('negligent', 1), ('letten', 2), ('bernazzani', 1), ('misconduct', 3), ('levees', 10), ('nacion', 1), ('mladjen', 1), ('kenjic', 2), ('vojkovici', 1), ('fraught', 1), ('detachment', 3), ('petitioning', 2), ('kiwayu', 1), ('pitted', 2), ('ta', 1), ('tuol', 1), ('sleng', 1), ('duch', 1), ('amezcua', 2), ('adan', 1), ('stimulant', 6), ('minds', 2), ('mirrored', 1), ('despondent', 1), ('hobart', 3), ('boulevard', 1), ('vasilily', 1), ('filipchuk', 1), ('introduces', 3), ('thespians', 1), ('shakespeareans', 1), ('zaher', 1), ('sardar', 2), ('barometer', 2), ('lifeline', 1), ('georgians', 2), ('guideline', 1), ('vaccinated', 1), ('gregorio', 2), ('rosal', 1), ('deserts', 1), ('greenery', 1), ('sonntags', 1), ('blick', 1), ('reassigned', 1), ('millerwise', 1), ('dyck', 1), ('hierarchical', 1), ('alec', 2), ('staffed', 1), ('pleshkov', 1), ('krasnokamensk', 1), ('chita', 2), ('reprisal', 3), ('forays', 1), ('harcharik', 1), ('\x93', 2), ('faisal', 8), ('angioplasty', 1), ('pakhtunkhwa', 2), ('impeding', 1), ('schedules', 1), ('hadjiya', 1), ('handcuffed', 2), ('skirmishes', 3), ('bands', 2), ('terrorize', 1), ('fldr', 1), ('handler', 1), ('canine', 1), ('soiling', 1), ('ketzer', 1), ('discharge', 2), ('emptively', 1), ('halfun', 1), ('bartlett', 2), ('weaker', 4), ('spiked', 2), ('haroon', 2), ('aswat', 5), ('30s', 1), ('niyazov', 1), ('schulz', 1), ('corroborate', 1), ('chevallier', 1), ('shek', 1), ('jarrah', 1), ('danny', 1), ('ayalon', 1), ('coldplay', 1), ('rude', 1), ('gwyneth', 3), ('paltrow', 2), ('folha', 2), ('paolo', 1), ('opined', 1), ('nicest', 1), ('testy', 1), ('ringtone', 1), ('edging', 1), ('068171296', 1), ('068217593', 1), ('fraenzi', 2), ('aufdenblatten', 1), ('068263889', 1), ('068275463', 1), ('782', 1), ('685', 1), ('redesigned', 1), ('slope', 1), ('sangju', 1), ('4000', 1), ('paz', 6), ('mexicali', 1), ('marghzar', 1), ('underfunded', 1), ('unprepared', 1), ('comrade', 1), ('infromation', 2), ('nigerla', 1), ('shoppertrak', 1), ('rct', 1), ('wielding', 5), ('nutritional', 2), ('blanket', 2), ('quietly', 1), ('elaborating', 1), ('chaparhar', 1), ('radioactivity', 1), ('sprawling', 2), ('expatriates', 2), ('narim', 1), ('alcolac', 1), ('vwr', 1), ('thermo', 1), ('fisher', 3), ('fujian', 7), ('tributary', 2), ('yangtze', 2), ('avert', 4), ('maruf', 1), ('bakhit', 1), ('fayez', 1), ('vest', 2), ('ncri', 2), ('630', 2), ('55th', 2), ('minia', 1), ('sights', 1), ('exxon', 1), ('reaping', 1), ('sanchez', 4), ('hers', 1), ('baloyi', 2), ('hop', 2), ('cheadle', 1), ('portray', 3), ('remixed', 1), ('benham', 1), ('natagehi', 1), ('paratroopers', 1), ('excursion', 1), ('retreated', 3), ('landale', 1), ('mirko', 1), ('norac', 1), ('ademi', 1), ('medak', 1), ('raffarin', 5), ('gall', 1), ('bladder', 1), ('reims', 1), ('penjwin', 1), ('mukasey', 5), ('durham', 1), ('taxiways', 1), ('hornafrik', 1), ('abdirahman', 1), ('dinari', 1), ('sreten', 1), ('lukic', 3), ('vascular', 1), ('1726', 1), ('1828', 1), ('tupamaros', 1), ('yearend', 2), ('frente', 1), ('amplio', 1), ('freest', 1), ('pa', 4), ('intifada', 2), ('salam', 1), ('fayyad', 1), ('uptick', 1), ('mandeb', 1), ('hormuz', 1), ('malacca', 1), ('cranes', 1), ('plowlands', 1), ('brandishing', 1), ('sling', 3), ('forsook', 1), ('liliput', 1), ('earnest', 1), ('suffice', 1), ('oak', 2), ('crept', 4), ('hearty', 2), ('groan', 1), ('lament', 3), ('cries', 3), ('orator', 3), ('organ', 2), ('unblotted', 3), ('escutcheon', 3), ('finger', 1), ('scorn', 2), ('misdeeds', 1), ('whitewash', 1), ('mortification', 1), ('tired', 2), ('partitioning', 2), ('unsettling', 1), ('buyout', 1), ('breakout', 1), ('machetes', 2), ('kuru', 1), ('wareng', 1), ('barakin', 1), ('ladi', 1), ('jos', 5), ('haram', 2), ('assumes', 4), ('anwarul', 1), ('foy', 3), ('usman', 1), ('ghani', 2), ('phases', 2), ('unseated', 1), ('shoving', 4), ('railed', 1), ('undetected', 2), ('wadia', 1), ('jaish', 1), ('hafsa', 1), ('misusing', 3), ('hiked', 1), ('busier', 1), ('quelled', 1), ('harbi', 4), ('yaounde', 1), ('debriefed', 2), ('cancels', 1), ('fasted', 1), ('bed', 4), ('nidal', 1), ('saada', 1), ('amona', 1), ('overstaying', 1), ('zenani', 2), ('culpable', 1), ('homicide', 5), ('grandchildren', 1), ('computing', 2), ('browsing', 2), ('conferencing', 2), ('hrd', 1), ('sibal', 3), ('laptop', 9), ('kapil', 1), ('ipad', 4), ('gadget', 2), ('linux', 1), ('campuses', 3), ('perito', 1), ('glacier', 7), ('glacieres', 1), ('spectacular', 1), ('breakup', 6), ('abdolsamad', 1), ('khorramshahi', 1), ('shatters', 1), ('dharamsala', 3), ('martic', 3), ('interception', 1), ('arjangi', 1), ('conson', 2), ('elliott', 1), ('abrams', 1), ('welch', 1), ('catandunes', 1), ('floodwalls', 2), ('berkeley', 2), ('minke', 2), ('crusade', 1), ('satirical', 1), ('eternal', 4), ('anesthetic', 2), ('bharti', 2), ('duke', 3), ('shopkeepers', 2), ('rockefeller', 1), ('zaldivar', 1), ('detainment', 3), ('gusting', 1), ('treacherous', 1), ('pile', 1), ('trnava', 1), ('unaffected', 2), ('compensating', 1), ('ethem', 2), ('erdagi', 2), ('tuition', 1), ('horses', 2), ('biak', 1), ('yearly', 4), ('tremendously', 1), ('amazed', 2), ('deception', 2), ('substantiate', 1), ('supposition', 1), ('involuntary', 1), ('callup', 1), ('chimango', 1), ('188', 1), ('asgiriya', 1), ('kandy', 1), ('toss', 3), ('seam', 1), ('hoggard', 3), ('spinner', 1), ('monty', 1), ('panesar', 3), ('sangakkara', 2), ('collingwood', 1), ('prasanna', 1), ('jayawardene', 1), ('alastair', 1), ('baba', 3), ('gana', 1), ('kingibe', 1), ('divisive', 2), ('intrude', 1), ('unintended', 1), ('pregnancies', 2), ('roe', 2), ('legalizing', 2), ('conception', 1), ('liken', 1), ('latifullah', 1), ('banditry', 3), ('sha', 2), ('zukang', 1), ('dictate', 3), ('hailu', 2), ('shawel', 1), ('abqaiq', 3), ('mosaic', 1), ('farthest', 1), ('snapshot', 1), ('bang', 1), ('turbans', 1), ('mature', 1), ('galactic', 1), ('sorts', 1), ('clarity', 1), ('ammar', 2), ('nom', 1), ('guerre', 1), ('masons', 1), ('muqataa', 1), ('royalty', 2), ('morality', 1), ('headdress', 1), ('wearers', 1), ('detector', 3), ('irreparable', 2), ('escalates', 1), ('grievances', 6), ('shaaban', 1), ('muntadar', 2), ('nhk', 2), ('arbor', 3), ('tsa', 2), ('grassroots', 2), ('plantings', 1), ('overshadows', 2), ('headgear', 1), ('medicare', 6), ('seniors', 4), ('prescription', 8), ('preventative', 1), ('enroll', 2), ('pronounces', 1), ('subjecting', 2), ('commons', 2), ('protégé', 1), ('suited', 1), ('jusuf', 1), ('kalla', 1), ('mukmin', 1), ('mammy', 1), ('maiduguri', 4), ('fare', 1), ('mohtarem', 1), ('manzur', 1), ('births', 2), ('disengage', 1), ('rowhani', 7), ('adamant', 1), ('muntazer', 2), ('zaidi', 5), ('ducked', 1), ('masterminded', 1), ('murderers', 3), ('hangings', 1), ('khazaee', 1), ('tightens', 1), ('invalid', 2), ('reluctantly', 1), ('1713', 1), ('utrecht', 1), ('gibraltarians', 1), ('tripartite', 1), ('cooperatively', 1), ('noncolonial', 1), ('sporadically', 1), ('durrani', 1), ('1747', 1), ('empires', 3), ('notional', 1), ('1919', 2), ('experiment', 1), ('tottering', 1), ('relentless', 1), ('ladin', 1), ('bonn', 1), ('manipulation', 3), ('sandinista', 5), ('contra', 3), ('saavedra', 1), ('mitch', 1), ('depressed', 6), ('polarized', 2), ('buoyant', 1), ('rope', 3), ('soaked', 2), ('strange', 1), ('lacerated', 1), ('faint', 2), ('scampered', 2), ('woe', 2), ('belabored', 1), ('tonight', 1), ('toil', 1), ('waggon', 1), ('rut', 1), ('hercules', 5), ('exertion', 1), ('tuba', 1), ('firmn', 1), ('hotbeds', 1), ('mitsumi', 1), ('overtime', 2), ('pastor', 3), ('quran', 2), ('puli', 1), ('obscure', 1), ('terry', 2), ('mizhar', 1), ('618', 1), ('varema', 1), ('divorced', 3), ('pummeling', 1), ('northwesterly', 1), ('pitting', 3), ('lobby', 4), ('vitamin', 1), ('therapies', 1), ('retroviral', 3), ('tac', 3), ('matthias', 1), ('rath', 2), ('defamatory', 2), ('nutrients', 3), ('bounnyang', 1), ('vorachit', 1), ('ome', 1), ('taj', 1), ('nenbutsushu', 2), ('macedonians', 1), ('463', 1), ('counterattack', 1), ('109', 4), ('hogg', 4), ('yuganskeneftegaz', 1), ('ramin', 1), ('mahmanparast', 1), ('mobs', 2), ('dimona', 3), ('adhaim', 1), ('waxman', 2), ('emissary', 1), ('censors', 1), ('sudharmono', 4), ('suharto', 2), ('golkar', 1), ('jonah', 1), ('brass', 1), ('viewing', 2), ('unopposed', 2), ('nasdaq', 3), ('cac', 3), ('dax', 3), ('532', 1), ('864', 1), ('406', 1), ('ounce', 3), ('electricidad', 1), ('edc', 1), ('aes', 2), ('nationalizations', 1), ('mouthing', 1), ('articulated', 1), ('iadb', 1), ('ramda', 5), ('crimping', 1), ('breakdowns', 2), ('tomohito', 3), ('mikasa', 1), ('emperors', 2), ('heirs', 2), ('concubines', 1), ('trusted', 2), ('counterfeit', 3), ('rsf', 5), ('photographers', 4), ('saidi', 1), ('tohid', 1), ('bighi', 1), ('henghameh', 1), ('somaieh', 1), ('nosrati', 1), ('matinpour', 1), ('concede', 5), ('239', 1), ('sinopec', 2), ('vitoria', 2), ('espirito', 1), ('catu', 1), ('bahia', 1), ('gasene', 1), ('datafolha', 2), ('zoran', 2), ('djindjic', 5), ('dejan', 1), ('bugsy', 1), ('milenkovic', 2), ('nebojsa', 1), ('covic', 2), ('seselj', 4), ('instrumental', 1), ('baluyevsky', 2), ('hajime', 1), ('massaki', 1), ('mutalib', 1), ('convening', 1), ('procedural', 1), ('clyburn', 2), ('gamble', 1), ('affordable', 3), ('baki', 3), ('andar', 1), ('rejoining', 1), ('madelyn', 1), ('dunham', 3), ('impregnating', 1), ('dusan', 2), ('tesic', 2), ('matabeleland', 1), ('counterfeiting', 1), ('hargreaves', 2), ('geologists', 1), ('confessions', 1), ('ranges', 2), ('censored', 1), ('piot', 1), ('pears', 1), ('shoe', 1), ('baitullah', 1), ('perpetuating', 1), ('ericq', 1), ('edouard', 2), ('alexis', 3), ('les', 1), ('cayes', 1), ('attainable', 1), ('augustin', 1), ('haro', 3), ('welt', 1), ('saroki', 1), ('overheating', 3), ('predictions', 3), ('bolder', 1), ('cautions', 2), ('cutbacks', 3), ('curve', 4), ('mountainside', 2), ('herve', 1), ('morin', 1), ('areva', 1), ('stupid', 2), ('gay', 12), ('silbert', 1), ('californian', 1), ('marriages', 2), ('gujarat', 2), ('chuck', 1), ('hamel', 1), ('corrosion', 1), ('prudhoe', 1), ('diseased', 2), ('implanted', 1), ('manufactures', 1), ('syncardia', 1), ('tucson', 2), ('nabbed', 2), ('eskisehir', 1), ('kiliclar', 1), ('nazer', 1), ('osprey', 1), ('reacts', 1), ('garner', 2), ('concluding', 3), ('obey', 2), ('sticking', 5), ('unfold', 1), ('weblogs', 1), ('blogs', 1), ('aleim', 1), ('saadi', 3), ('gothenburg', 1), ('scandinavia', 1), ('gungoren', 1), ('savage', 1), ('olusegan', 1), ('yedioth', 3), ('ahronoth', 3), ('trouncing', 1), ('prefers', 1), ('taker', 1), ('denials', 2), ('444', 4), ('slaying', 4), ('younes', 1), ('megawatt', 1), ('deutchmark', 1), ('dinar', 1), ('privatized', 3), ('infusion', 1), ('criminality', 1), ('insecurity', 5), ('fundamentals', 3), ('chernobyl', 1), ('expansionary', 5), ('lows', 3), ('riverine', 1), ('semidesert', 1), ('adherence', 2), ('devaluation', 1), ('cfa', 2), ('jeopardized', 2), ('predominate', 1), ('ranches', 1), ('breadfruit', 2), ('tomatoes', 4), ('melons', 1), ('exemptions', 1), ('booty', 1), ('spoil', 2), ('modestly', 2), ('heap', 2), ('witnessing', 1), ('learns', 1), ('misfortunes', 2), ('beg', 1), ('requite', 1), ('kindness', 1), ('flattered', 1), ('yours', 2), ('messes', 1), ('eats', 1), ('behaves', 1), ('cubapetroleo', 1), ('cnbc', 1), ('bell', 2), ('coercive', 1), ('conniving', 1), ('dismisses', 1), ('protocols', 2), ('doubting', 1), ('chests', 1), ('beltran', 1), ('shyloh', 1), ('giddens', 2), ('barman', 2), ('naqba', 2), ('entangle', 1), ('405', 1), ('ve', 1), ('plummeting', 3), ('counterinsurgency', 2), ('falluja', 1), ('conduit', 1), ('remissaninthe', 1), ('ravix', 2), ('roared', 1), ('formulations', 1), ('differing', 1), ('2050', 1), ('polluting', 1), ('mortally', 3), ('bitar', 1), ('bitarov', 1), ('makhachkala', 2), ('adilgerei', 1), ('magomedtagirov', 1), ('xianghe', 1), ('salang', 1), ('avalanches', 3), ('belgians', 1), ('returnees', 2), ('consisting', 3), ('statoil', 1), ('krasnoyarsk', 1), ('assortment', 1), ('firearms', 4), ('subtracted', 1), ('mawlawi', 1), ('masah', 1), ('ulema', 1), ('cambridge', 1), ('incapacitated', 2), ('azocar', 1), ('moviemaking', 2), ('reinforces', 2), ('bollywood', 1), ('kiteswere', 1), ('tarnishing', 2), ('silverman', 1), ('misguided', 3), ('deifies', 1), ('militarist', 1), ('gao', 3), ('shara', 3), ('demonstrably', 1), ('antony', 1), ('ambareesh', 1), ('jayprakash', 1), ('narain', 1), ('rashtriya', 1), ('dal', 1), ('jabel', 1), ('wasting', 2), ('20s', 3), ('creutzfeldt', 1), ('bovine', 1), ('spongiform', 1), ('encephalopathy', 1), ('alienation', 1), ('victimize', 1), ('loosely', 1), ('smash', 1), ('delivers', 1), ('samho', 1), ('dream', 3), ('filipinos', 5), ('qater', 1), ('frontal', 1), ('aboutorabi', 1), ('fard', 1), ('pretending', 3), ('seeker', 1), ('joongang', 1), ('esfandiar', 1), ('mashaie', 5), ('heater', 2), ('arg', 2), ('exits', 1), ('fang', 1), ('llam', 1), ('impassioned', 1), ('vandemoortele', 1), ('defies', 1), ('constituency', 5), ('kakdwip', 2), ('1004', 1), ('101', 5), ('bricks', 6), ('maghazi', 1), ('thun', 1), ('statistical', 1), ('lindy', 1), ('makubalo', 1), ('variation', 3), ('methodology', 2), ('attache', 2), ('olesgun', 1), ('miserable', 1), ('turkana', 1), ('rongai', 1), ('marigat', 1), ('mogotio', 1), ('corresponding', 1), ('hawks', 3), ('mesa', 8), ('sledge', 1), ('hammers', 1), ('structurally', 1), ('unsound', 1), ('seabees', 1), ('shujaat', 1), ('zamir', 1), ('exploding', 2), ('asef', 1), ('shawkat', 1), ('escapees', 2), ('bumpy', 1), ('firebase', 1), ('ripley', 1), ('baskin', 1), ('bypassed', 1), ('resorting', 2), ('inroads', 1), ('wefaq', 1), ('botanic', 1), ('partnered', 1), ('orchids', 1), ('splash', 1), ('brilliance', 1), ('kohlu', 2), ('shahid', 1), ('rivalry', 4), ('durjoy', 1), ('bangla', 1), ('bogra', 1), ('demographer', 1), ('spouse', 1), ('mu', 1), ('guangzhong', 1), ('outlaw', 3), ('fetus', 1), ('passover', 2), ('heartened', 1), ('motivating', 2), ('shies', 1), ('kanan', 1), ('trent', 4), ('duffy', 1), ('deir', 2), ('balah', 1), ('caterpillar', 2), ('swerve', 1), ('aweil', 1), ('hanifa', 1), ('733', 1), ('g20', 1), ('azizi', 1), ('jalali', 1), ('benoit', 1), ('maimed', 1), ('ceel', 1), ('waaq', 1), ('vacationers', 2), ('campsites', 1), ('schoolgirls', 1), ('motorbikes', 1), ('bundled', 1), ('iapa', 1), ('overhauling', 1), ('entitlement', 1), ('exceedingly', 1), ('explorers', 3), ('samoan', 1), ('pago', 2), ('togoland', 1), ('facade', 1), ('rpt', 1), ('continually', 1), ('capitalism', 3), ('gardener', 2), ('tile', 1), ('prospering', 1), ('watered', 2), ('tilemaker', 1), ('shine', 1), ('bolting', 1), ('breakfast', 5), ('abstraction', 1), ('pickle', 1), ('fork', 2), ('spectacles', 1), ('needless', 1), ('lens', 1), ('wharf', 1), ('swamp', 2), ('stumped', 1), ('predator', 1), ('alarming', 2), ('chemist', 2), ('coupled', 2), ('brewed', 1), ('adhesive', 1), ('togetherness', 1), ('monosodium', 1), ('glue', 1), ('uhm', 4), ('shimbun', 1), ('valero', 1), ('prejudice', 1), ('tarcisio', 1), ('bertone', 2), ('furor', 1), ('priestly', 1), ('celibacy', 1), ('galo', 1), ('chiriboga', 1), ('rejoined', 3), ('dries', 1), ('buehring', 1), ('mudasir', 2), ('gujri', 1), ('gopal', 1), ('slams', 1), ('tolerating', 1), ('animists', 1), ('heineken', 1), ('pingdingshan', 1), ('notoriously', 2), ('sarin', 2), ('informant', 2), ('coded', 1), ('correspondence', 1), ('deciphering', 1), ('reaffirm', 3), ('endeavouris', 1), ('endeavourundocked', 1), ('spacewalks', 4), ('educate', 1), ('26th', 2), ('stern', 5), ('invaders', 2), ('hell', 1), ('wook', 1), ('gainers', 1), ('performace', 1), ('concacaf', 1), ('frisked', 2), ('slots', 4), ('hamarjajab', 1), ('schuessel', 3), ('bridged', 1), ('khatibi', 1), ('communicate', 2), ('conscience', 2), ('flourish', 1), ('curbs', 2), ('abilities', 1), ('literacy', 2), ('rangeen', 1), ('refueling', 2), ('milomir', 1), ('stakic', 2), ('sample', 3), ('h7n3', 1), ('droppings', 1), ('thyroid', 1), ('47s', 1), ('slayings', 1), ('warranted', 2), ('kharazi', 2), ('zvyagintsev', 1), ('parulski', 1), ('tusk', 6), ('smolensk', 1), ('djinnit', 1), ('unacceptably', 1), ('civility', 2), ('reconstructive', 2), ('aisha', 3), ('grossman', 1), ('sliced', 1), ('hacked', 1), ('canons', 1), ('glad', 1), ('assailed', 3), ('unravel', 1), ('fabric', 1), ('betting', 4), ('calculators', 1), ('bookmaking', 1), ('khurul', 1), ('kalmykia', 1), ('expectation', 1), ('ipsos', 2), ('midterm', 2), ('exams', 5), ('answers', 2), ('khouna', 1), ('haidallah', 1), ('maaouiya', 1), ('taya', 3), ('advertisement', 1), ('expansive', 1), ('communicated', 1), ('fares', 1), ('firmest', 1), ('mediating', 1), ('pepper', 1), ('truncheons', 1), ('beyazit', 1), ('activated', 2), ('bluefin', 4), ('wwf', 2), ('sushi', 1), ('leigh', 3), ('liang', 3), ('eighties', 2), ('sever', 2), ('jinling', 1), ('identifies', 3), ('shadi', 3), ('spiraled', 1), ('hagino', 1), ('uji', 1), ('unaccounted', 3), ('deserter', 2), ('townspeople', 2), ('shfaram', 1), ('renen', 1), ('schorr', 1), ('shooter', 1), ('kach', 1), ('belhadj', 1), ('sibneft', 2), ('measles', 4), ('inoculate', 1), ('nomads', 2), ('ziyad', 1), ('karbouli', 4), ('desouki', 1), ('vaccinators', 1), ('macheke', 1), ('aeneas', 1), ('chigwedere', 1), ('comatose', 2), ('unconscious', 2), ('lci', 1), ('initiating', 2), ('react', 1), ('relate', 1), ('incur', 1), ('directives', 1), ('jameson', 5), ('grill', 5), ('maybe', 2), ('raiser', 1), ('picnic', 1), ('kickball', 1), ('volleyball', 1), ('archery', 2), ('crafts', 1), ('creek', 1), ('roast', 1), ('fixings', 1), ('drinks', 4), ('raffle', 3), ('dreams', 2), ('enclosed', 2), ('bray', 2), ('241', 1), ('2661', 1), ('jcfundrzr', 1), ('aol', 3), ('colonizers', 1), ('distinct', 3), ('linguistic', 2), ('1906', 1), ('condominium', 1), ('963', 1), ('1839', 2), ('knife', 2), ('benelux', 1), ('bolshevik', 2), ('agrochemicals', 1), ('aral', 1), ('stagnation', 1), ('curtailment', 1), ('1935', 1), ('ferdinand', 2), ('edsa', 2), ('corazon', 2), ('estrada', 3), ('insurgencies', 1), ('conqueror', 1), ('flapped', 1), ('exultingly', 1), ('pounced', 1), ('undisputed', 1), ('comission', 1), ('sportsmen', 1), ('coho', 1), ('walleye', 1), ('kowal', 2), ('reproduced', 1), ('muskie', 1), ('kowalski', 1), ('franken', 2), ('laotian', 1), ('harkin', 1), ('dispose', 1), ('waiver', 1), ('suicides', 1), ('malpractice', 4), ('premiums', 3), ('standby', 6), ('baiji', 7), ('uday', 1), ('numaniya', 1), ('domed', 1), ('cykla', 1), ('assailant', 1), ('fathi', 1), ('nuaimi', 1), ('void', 1), ('firmer', 1), ('fiery', 5), ('jiang', 1), ('jufeng', 1), ('skull', 7), ('bildnewspaper', 1), ('josef', 4), ('fastening', 1), ('ornament', 1), ('exposing', 3), ('align', 1), ('propped', 1), ('robustly', 1), ('getters', 1), ('mizarkhel', 1), ('layoffs', 2), ('737s', 1), ('747', 5), ('rashad', 1), ('356', 1), ('tareen', 3), ('defaulting', 1), ('disbursements', 1), ('pontificate', 1), ('inserted', 3), ('delegated', 1), ('urbi', 1), ('et', 2), ('orbi', 1), ('supremacist', 1), ('mahlangu', 1), ('afrikaner', 1), ("terre'blanche", 2), ('bludgeoned', 1), ('stemmed', 2), ('seal', 10), ('fenced', 1), ('abbott', 3), ('259', 1), ('labs', 1), ('kaletra', 1), ('generic', 1), ('overpricing', 1), ('muted', 1), ('malaysians', 1), ('vacations', 1), ('caucasian', 1), ('teresa', 1), ('borcz', 1), ('mailed', 2), ('harkat', 1), ('zihad', 1), ('communal', 2), ('ranbir', 1), ('dayers', 1), ('poroshenko', 2), ('acrimonious', 1), ('chrysostomos', 1), ('heretic', 1), ('poachers', 1), ('skins', 1), ('imperialist', 1), ('crucified', 1), ('relaxing', 2), ('bakri', 5), ('overflowed', 4), ('neiva', 1), ('jebii', 1), ('kilimo', 2), ('xii', 3), ('1582', 1), ('noncompliant', 1), ('yunlin', 1), ('platon', 3), ('lebedev', 4), ('mariane', 1), ('habib', 2), ('odierno', 1), ('zctu', 2), ('bobbie', 1), ('vihemina', 1), ('prout', 1), ('wrought', 1), ('czar', 2), ('singling', 1), ('chibebe', 1), ('seige', 1), ('tobie', 1), ('okala', 2), ('brownback', 2), ('hicks', 9), ('cosatu', 5), ('homosexual', 1), ('massouda', 2), ('enthusiastic', 2), ('alexeyev', 3), ('discriminated', 1), ('insights', 1), ('kappes', 3), ('sulik', 1), ('rebut', 1), ('touchdown', 6), ('rod', 2), ('broncos', 1), ('yards', 2), ('undrafted', 1), ('quarterback', 3), ('jake', 1), ('plummer', 1), ('kicker', 1), ('elam', 1), ('receiver', 1), ('samie', 1), ('corpse', 1), ('kits', 1), ('repaired', 4), ('reopening', 6), ('kaman', 2), ('hasten', 2), ('relaunch', 2), ('monk', 6), ('yury', 2), ('luzhkov', 1), ('satanic', 1), ('thu', 3), ('htay', 1), ('ashin', 1), ('gambira', 2), ('mandalay', 2), ('hkamti', 1), ('sagaing', 1), ('willian', 1), ('orchestrating', 2), ('sadiq', 1), ('defy', 4), ('bandundu', 2), ('mende', 3), ('merchandise', 3), ('navigate', 2), ('slimy', 1), ('nickelodeon', 2), ('messiest', 1), ('dispenser', 1), ('goo', 1), ('sandler', 1), ('stiller', 1), ('wannabe', 1), ('signifies', 2), ('youthful', 1), ('lighthearted', 1), ('imprisioned', 1), ('trivial', 2), ('meltdown', 2), ('1745', 1), ('cozumel', 3), ('theodor', 2), ('zu', 2), ('guttenberg', 3), ('schneiderhan', 3), ('ou', 2), ('xinqian', 1), ('adjusted', 1), ('asadollah', 1), ('sabouri', 2), ('obligates', 1), ('disposition', 2), ('leftwing', 1), ('relates', 1), ('buraydah', 1), ('tata', 9), ('cheapest', 3), ('singur', 1), ('uttarakhand', 1), ('chapfika', 1), ('zvakwana', 1), ('tuvalu', 7), ('atolls', 3), ('seamen', 2), ('ttf', 2), ('nz', 2), ('vulnerability', 2), ('remoteness', 2), ('lobster', 2), ('anguillan', 1), ('intercommunal', 1), ('trnc', 1), ('impetus', 1), ('reuniting', 2), ('acquis', 1), ('forbade', 1), ('altered', 3), ('flute', 2), ('projecting', 1), ('tunes', 1), ('haul', 4), ('leaping', 1), ('perverse', 1), ('creatures', 4), ('merrily', 1), ('goblet', 1), ('signboard', 2), ('unwittingly', 2), ('dashed', 2), ('jarring', 1), ('terribly', 2), ('zeal', 1), ('naturedly', 1), ('benefactor', 1), ('gnawed', 1), ('orioles', 1), ('baltimore', 1), ('playoffs', 4), ('outfield', 1), ('reindeer', 2), ('deserved', 2), ('rudolph', 2), ('glowing', 1), ('proboscis', 1), ('pinna', 1), ('cylinder', 2), ('origins', 3), ('jumpers', 2), ('beltrame', 2), ('stefano', 1), ('chiapolino', 2), ('predazzo', 1), ('belluno', 1), ('spleen', 1), ('cavalese', 1), ('fractures', 2), ('concussion', 2), ('roofs', 4), ('montas', 2), ('someday', 1), ('zwelinzima', 1), ('vavi', 1), ('skewed', 2), ('uz', 1), ('mushahid', 1), ('squadron', 1), ('gymnast', 3), ('shawn', 1), ('brightest', 1), ('enming', 1), ('aspiring', 1), ('olympian', 1), ('sagging', 1), ('backgrounds', 3), ('gdps', 1), ('arawak', 2), ('1672', 1), ('inevitably', 1), ('slows', 2), ('simmers', 1), ('policharki', 1), ('khaisor', 1), ('siphiwe', 2), ('safa', 2), ('sedibe', 2), ('memorable', 2), ('symbolism', 1), ('heighten', 1), ('enviable', 1), ('curator', 3), ('xinmiao', 1), ('qing', 1), ('1644', 1), ('spanning', 2), ('shula', 3), ('zaken', 2), ('andijon', 4), ('cascade', 2), ('adwar', 1), ('rabiyaa', 1), ('faisalabad', 2), ('sidique', 2), ('shehzad', 1), ('tanweer', 1), ('hasib', 1), ('sidon', 2), ('slid', 1), ('shehade', 2), ('uncovering', 1), ('housekeeper', 2), ('taser', 1), ('giuliani', 1), ('patriots', 2), ('lubanga', 7), ('hema', 1), ('serge', 1), ('brammertz', 2), ('acutely', 1), ('utmost', 2), ('laughing', 3), ('ilna', 1), ('sajedinia', 1), ('karoubi', 1), ('rightful', 2), ('geagea', 2), ('liquids', 2), ('believing', 3), ('liner', 1), ("ma'ariv", 1), ('nazif', 2), ('heidelberg', 1), ('intestine', 1), ('mullen', 5), ('hoekstra', 1), ('diane', 1), ('feinstein', 1), ('saxby', 2), ('chambliss', 3), ('waking', 2), ('comfortably', 2), ('dozing', 1), ('bailey', 1), ('videolink', 1), ('rohrabacher', 3), ('neil', 1), ('abercrombie', 2), ('silenced', 2), ('horrifying', 1), ('owl', 4), ('counseled', 1), ('acorn', 1), ('sprout', 2), ('heroism', 1), ('ignace', 1), ('schops', 1), ("voa's", 1), ('ivanic', 2), ('acorns', 1), ('mistletoe', 1), ('irremediable', 1), ('insure', 1), ('mikerevic', 1), ('dismissals', 1), ('pluck', 2), ('flax', 1), ('boded', 1), ("pe'at", 1), ('sadeh', 1), ('lastly', 1), ('darts', 1), ('dormitories', 1), ('disobey', 1), ('credence', 1), ('321', 2), ('stubb', 1), ('wisest', 1), ('odde', 1), ('leaflet', 1), ('solitude', 1), ('mixing', 1), ('nagano', 2), ('ayyub', 1), ('khakrez', 1), ('touts', 1), ('haleem', 1), ('traded', 5), ('121', 3), ('329', 1), ('913', 1), ('781', 1), ('abdulaziz', 2), ('intending', 2), ('colts', 2), ('lions', 3), ('touchdowns', 3), ('cowboys', 1), ('julius', 1), ('phillip', 2), ('madhya', 1), ('buzzard', 1), ('hotline', 2), ('outstrip', 1), ('205', 1), ('9942', 1), ('vaeidi', 1), ('soltanieh', 1), ('provincal', 1), ('zinjibar', 2), ('crazy', 1), ('pest', 1), ('fouling', 1), ('insect', 1), ('extracting', 1), ('cesar', 1), ('abderrahaman', 1), ('infidel', 1), ('noaman', 2), ('gomaa', 2), ('swear', 2), ('confiscate', 1), ('affiliates', 5), ('aqaba', 2), ('ashland', 1), ('scam', 4), ('gyeong', 1), ('nuptials', 1), ('bryant', 1), ('sita', 1), ('di', 4), ('miliatry', 1), ('garikoitz', 1), ('aspiazu', 2), ('rubina', 2), ('pyrenees', 1), ('tablet', 3), ('wireless', 1), ('txeroki', 1), ('myricks', 1), ('demotion', 1), ('samsun', 1), ('seaside', 3), ('dimensional', 2), ('3d', 3), ('vertigo', 1), ('modell', 2), ('heralds', 1), ('filmmaking', 1), ('replicating', 1), ('physiology', 1), ('nausea', 2), ('715', 1), ('1278', 1), ('andorrans', 1), ('1607', 1), ('onward', 1), ('seu', 1), ("d'urgell", 1), ('feudal', 2), ('titular', 1), ('benefitted', 1), ('hellenic', 1), ('inequities', 1), ('ohrid', 2), ('fronts', 3), ('keeling', 1), ('1609', 2), ('1820s', 2), ('clunie', 1), ('cocos', 1), ('1841', 1), ('skillful', 1), ('meshes', 1), ('exacted', 1), ('promissory', 1), ('raft', 2), ('747s', 1), ('chopper', 1), ('locator', 1), ('renouncing', 1), ('unknowingly', 2), ('crisp', 1), ('overpaid', 1), ('dilemma', 1), ('athar', 1), ('chalit', 1), ('phukphasuk', 1), ('thirapat', 1), ('serirangsan', 1), ('paulino', 1), ('matip', 1), ('splm', 2), ('django', 2), ('reinhardt', 2), ('bossa', 2), ('aneurysm', 2), ('ventura', 1), ('dans', 1), ('mon', 1), ('jobim', 1), ('acronym', 1), ('expending', 1), ('deviated', 1), ('cerberus', 2), ('fragility', 1), ('waive', 3), ('enrollment', 1), ('seminars', 1), ('confuse', 1), ('straightened', 2), ('nissan', 6), ('mckiernan', 1), ('unites', 1), ('solitary', 4), ('buhriz', 2), ('taza', 1), ('dutton', 1), ('buchan', 1), ('gasses', 2), ('unscheduled', 2), ('curbed', 1), ('shrines', 3), ('babil', 2), ('victors', 1), ('qurans', 2), ('mcadams', 2), ('peacekeeers', 1), ('demented', 1), ('storming', 3), ('invoke', 1), ('emission', 1), ('brokering', 2), ('fledged', 1), ('kien', 1), ('premiering', 1), ('dramatizes', 1), ('integrating', 1), ('comeback', 4), ('indifferent', 1), ('dishonor', 1), ('pararajasingham', 1), ('undertake', 1), ('yuli', 1), ('tamir', 2), ('soleil', 2), ('ronit', 1), ('tirosh', 1), ('reconstructed', 1), ('pieced', 1), ('presidencies', 1), ('goran', 1), ('persson', 2), ('laila', 2), ('freivalds', 2), ('tempered', 1), ('sharpest', 2), ('gag', 1), ('colby', 1), ('vokey', 1), ('cerveny', 2), ('mcnuggets', 1), ('abdurrahman', 1), ('yalcinkaya', 1), ('dtp', 2), ('fda', 5), ('salmonella', 2), ('unger', 1), ('budny', 1), ('marxists', 1), ('rash', 2), ('miliband', 6), ('jacqui', 1), ('headless', 1), ('jamaat', 2), ('fitna', 1), ('geert', 1), ('wilders', 1), ('quotations', 1), ('summoning', 2), ('stein', 4), ('bloom', 1), ('narthiwat', 1), ('sungai', 1), ('kolok', 1), ('carrasquero', 1), ('kumgang', 1), ('reunions', 1), ('defected', 12), ('mclaughlin', 1), ('disrespectfully', 1), ('tabulation', 1), ('des', 2), ('moines', 1), ('intuitive', 1), ('talker', 1), ('dismal', 1), ('saudis', 3), ('shuttered', 1), ('humphreys', 1), ('kozloduy', 1), ('reinsurer', 1), ('zurich', 3), ('reinsurers', 1), ('jetliner', 1), ('cargolux', 1), ('utilize', 1), ('quieter', 1), ('380', 3), ('superjumbo', 3), ('alu', 3), ('alkhanov', 6), ('danilovich', 2), ('justly', 1), ('militaries', 1), ('dahoud', 1), ('withstood', 1), ('idled', 2), ('mpla', 2), ('dos', 2), ('unita', 2), ('helena', 2), ('tristan', 1), ('cunha', 1), ('agriculturally', 1), ('tasalouti', 1), ('expectancy', 1), ('vertical', 1), ('horizontal', 1), ('clusters', 1), ('mitigate', 1), ('outreach', 1), ('1667', 1), ('nominally', 1), ('1650', 1), ('saeedlou', 1), ('precipice', 1), ('endeavoring', 1), ('willful', 1), ('funny', 1), ('mane', 1), ('wig', 2), ('windy', 1), ('charming', 1), ('sisters', 1), ('blandly', 1), ('gust', 1), ('foolish', 4), ('bald', 2), ('glistening', 1), ('billiard', 1), ('embarrassed', 1), ('woodchopper', 1), ('besought', 1), ('sadeq', 3), ('mahsouli', 1), ('thoughtless', 1), ('deity', 1), ('salivated', 1), ('thorn', 4), ('amphitheatre', 1), ('devour', 1), ('honourably', 1), ('abstained', 2), ('claimant', 1), ('yogi', 2), ('berra', 1), ('stocky', 1), ('catcher', 1), ('horrified', 3), ('miraculous', 1), ('bathtub', 2), ('invented', 2), ('1850', 2), ('1875', 2), ('bothered', 1), ('miscreants', 1), ('toad', 1), ('evicting', 1), ('reply', 4), ('query', 1), ("ha'aretz", 1), ('utilization', 1), ('interaction', 1), ('unsolicited', 1), ('kramer', 3), ('spammers', 2), ('server', 1), ('disabling', 1), ('upgrading', 2), ('faraa', 1), ('yamoun', 1), ('mohammadi', 2), ('parental', 1), ('jeffery', 2), ('jeffrey', 4), ('hamstrung', 1), ('orion', 2), ('workhorse', 1), ('dhanush', 1), ('subhadra', 1), ('moiseyev', 5), ('balletic', 1), ('acrobatic', 1), ('dances', 2), ('souq', 1), ('ghazl', 1), ('inoculations', 1), ('surfaces', 2), ('rye', 1), ('bluegrass', 1), ('turf', 2), ('mown', 1), ('fertilized', 1), ('billed', 1), ('successors', 2), ('flares', 1), ('abizaid', 3), ('reyna', 5), ('sprained', 1), ('ligament', 2), ('saddamists', 1), ('gianni', 1), ('magazzeni', 2), ('piles', 1), ('echoupal', 4), ('kiosks', 1), ('expensively', 1), ('rafidain', 1), ('finsbury', 1), ('stirring', 4), ('notoriety', 1), ('sermons', 2), ('earl', 1), ('christa', 2), ('mcauliffe', 2), ('liftoff', 2), ('booster', 3), ('columbiadisintegred', 1), ('showings', 1), ('cycling', 2), ('rowing', 3), ('disciplines', 1), ('steele', 5), ('adolfo', 3), ('scilingo', 2), ('drugged', 1), ('recanted', 2), ('cult', 2), ('embracing', 2), ('headlining', 1), ('differed', 1), ('erian', 1), ('acceptable', 5), ('complains', 1), ('poster', 1), ('ezzat', 1), ('itv1', 1), ('suleimaniyah', 1), ('salah', 1), ('reservist', 1), ('genitals', 1), ('kerkorian', 1), ('ghosn', 1), ('patzi', 2), ('contry', 1), ('transporation', 1), ('263', 1), ('299', 1), ('diagnostic', 1), ('utterly', 1), ('irrelevant', 1), ('interahamwe', 2), ('dean', 4), ('orjiako', 1), ('hassanpour', 1), ('abdolvahed', 1), ('hiva', 1), ('botimar', 1), ('asou', 1), ('zhou', 2), ('guocong', 1), ('grossly', 1), ('rings', 2), ('jamuna', 1), ('witty', 5), ('051655093', 1), ('hilton', 2), ('camille', 1), ('karolina', 1), ('sprem', 1), ('580', 2), ('harkleroad', 1), ('smyr', 3), ('petrova', 2), ('mujahadeen', 1), ('adjourns', 1), ('gaston', 1), ('gaudio', 1), ('kanaan', 1), ('postcard', 2), ('qaumi', 2), ('parlemannews', 1), ('crackdowns', 2), ('alyaty', 1), ('melange', 1), ('edison', 1), ('confidentiality', 3), ('baptiste', 2), ('natama', 1), ('unavoidable', 1), ('wannian', 1), ('whereas', 1), ('hanyuan', 1), ('zhengyu', 1), ('dung', 1), ('halo', 1), ('skulls', 3), ('trenches', 1), ('lining', 1), ('specialize', 1), ('validated', 1), ('si', 1), ('cumple', 1), ('rumbo', 2), ('propio', 2), ('mvr', 1), ('depressions', 1), ('1851', 1), ('courier', 2), ('rustlers', 1), ('pokot', 1), ('samburu', 2), ('letimalo', 1), ('combing', 1), ('bend', 1), ('oceanic', 3), ('wilderness', 1), ('archipelagoes', 1), ('boasts', 2), ('aquarium', 1), ('turtles', 3), ('vlado', 1), ('buckovski', 4), ('cathy', 1), ('majtenyi', 1), ('uwezo', 1), ('theatre', 1), ('colonize', 1), ('equatoria', 3), ('1870s', 2), ('mahdist', 2), ('facilitated', 3), ('rum', 1), ('distilling', 1), ('croix', 1), ('mindaugas', 1), ('1236', 2), ('1386', 1), ('1569', 1), ('abortive', 1), ('variations', 1), ('banana', 3), ('gonsalves', 1), ('flea', 5), ('dare', 2), ('limbs', 1), ('notarized', 1), ('hint', 1), ('pinions', 1), ('biceps', 1), ('pugiliste', 1), ('ladders', 1), ('stepstools', 1), ('demontrators', 1), ('unregistered', 2), ('shaath', 2), ('qidwa', 1), ('pall', 1), ('sugars', 1), ('clinical', 4), ('diet', 1), ('shelley', 1), ('schlender', 1), ('staggered', 2), ('hammond', 1), ('obamas', 1), ('tayeb', 1), ('musbah', 1), ('abstentions', 2), ('pilgrimages', 1), ('inflicting', 1), ('khar', 1), ('hacari', 1), ('santander', 1), ('honked', 1), ('circled', 1), ('unleaded', 1), ('spite', 2), ('kalameh', 1), ('sabz', 1), ('refiner', 1), ('sk', 2), ('bazian', 1), ('dahuk', 1), ('uloum', 3), ('injustices', 1), ('impulses', 1), ('disloyalty', 1), ('adulthood', 2), ('olds', 1), ('juristictions', 1), ('camouflage', 2), ('kimonos', 1), ('ud', 1), ('gabla', 1), ('concocted', 1), ('animosity', 1), ('berman', 3), ('lowey', 2), ('appropriations', 1), ('jalalzadeh', 1), ('cai', 1), ('guo', 1), ('quiang', 1), ('gunpowder', 1), ('designing', 1), ('firework', 1), ('guggenheim', 1), ('yiru', 1), ('7000', 1), ('aka', 3), ('tanoh', 1), ('bouna', 1), ('abderahmane', 1), ('vezzaz', 1), ('tintane', 1), ('acapulco', 3), ('otay', 1), ('yunesi', 2), ('interrogate', 1), ('moken', 1), ('banged', 1), ('offerings', 1), ('loftis', 1), ('schilling', 1), ('rood', 3), ('jamaicans', 2), ('asafa', 1), ('usain', 1), ('bolt', 4), ('haniyah', 1), ('farhat', 1), ('cautioning', 1), ('rematch', 1), ('gels', 1), ('cabins', 1), ('100th', 2), ('bermet', 2), ('akayeva', 3), ('aidar', 1), ('reactions', 3), ('sequence', 1), ('tyson', 1), ('sonora', 1), ('anxiously', 1), ('steam', 2), ('approves', 3), ('shenzhou', 4), ('liwei', 1), ('naser', 1), ('earthen', 1), ('raba', 1), ('borderline', 1), ('spearheading', 1), ('genoino', 3), ('tarso', 1), ('genro', 1), ('treasurer', 1), ('dirceu', 1), ('reshuffling', 1), ('nestle', 3), ('trickle', 2), ('worshipers', 4), ('timer', 1), ('coexist', 2), ('gaudy', 1), ('props', 1), ('muqudadiyah', 1), ('silo', 1), ('kicks', 1), ('schoch', 3), ('bruhin', 3), ('relais', 1), ('prommegger', 1), ('heinz', 1), ('inniger', 1), ('jaquet', 1), ('460', 1), ('kohli', 1), ('daniela', 1), ('meuli', 1), ('pomagalski', 1), ('isabelle', 1), ('blanc', 1), ('kamel', 2), ('ibb', 1), ('baptist', 3), ('jibla', 1), ('bruno', 1), ('gollnisch', 2), ('disputing', 2), ('nullify', 2), ('acholi', 1), ('aphaluck', 1), ('bhatiasevi', 1), ('292', 1), ('vomit', 1), ('saliva', 1), ('dhess', 1), ('shalabi', 1), ('dagma', 1), ('djamel', 1), ('discotheques', 2), ('dusseldorf', 1), ('abdalla', 1), ('trademark', 2), ('trademarks', 1), ('vuitton', 3), ('counterfeiters', 1), ('bernd', 2), ('alois', 1), ('soldaten', 1), ('cavernous', 1), ('armory', 1), ('lekki', 1), ('decommission', 1), ('acehnese', 1), ('resent', 1), ('nogaideli', 2), ('burdzhanadze', 1), ('42nd', 1), ('murphy', 2), ('translates', 1), ('shaleyste', 1), ('cart', 1), ('reconcilation', 1), ('alimov', 1), ('berkin', 1), ('chaika', 1), ('emigre', 1), ('pavel', 1), ('ryaguzov', 1), ('anglican', 2), ('canterbury', 3), ('rowan', 2), ('mostar', 2), ('krzyzewski', 2), ('grocery', 2), ('annualized', 1), ('coalitions', 2), ('jalbire', 1), ('retires', 1), ('265', 2), ('shearer', 1), ('tremor', 2), ('pius', 5), ('archive', 3), ('initiators', 1), ('middleman', 1), ('lupolianski', 1), ('marshal', 1), ('slavonia', 1), ('interlarded', 1), ('downturns', 1), ('vagaries', 1), ('statutory', 1), ('gateways', 1), ('ltte', 5), ('vulnerabilities', 1), ('alleviated', 1), ('eater', 1), ('halter', 2), ('courted', 2), ('ashamed', 1), ('admirer', 1), ('hairs', 1), ('zealous', 1), ('dazzling', 1), ('boisterous', 1), ('tilt', 1), ('kalachen', 1), ('rapporteur', 1), ('557', 1), ('522', 1), ('439', 2), ('enroute', 1), ('whatsoever', 1), ('xiamen', 1), ('kaohsiung', 1), ('lambasted', 1), ('unsavory', 1), ('dunking', 1), ('filthy', 1), ('workable', 1), ('jocic', 1), ('cimpl', 2), ('flashing', 3), ('floodwater', 1), ('getty', 2), ('marble', 1), ('bc', 2), ('tombstone', 3), ('sculpted', 1), ('voulgarakis', 1), ('popovkin', 1), ('jabir', 1), ('jubran', 1), ('fayfi', 2), ('barzani', 11), ('veiled', 2), ('manar', 3), ('iqbal', 3), ('machinists', 1), ('gene', 3), ('destroys', 2), ('insulin', 1), ('glucose', 1), ('snipers', 1), ('carrizales', 1), ('atal', 1), ('bihari', 1), ('vajpayee', 4), ('bhartiya', 1), ('bjp', 2), ('earle', 1), ('prosecutorial', 1), ('pornographic', 1), ('passwords', 1), ('proliferators', 1), ('axum', 5), ('obelisk', 7), ('teshome', 1), ('toga', 1), ('granite', 2), ('structur', 1), ('unsaturated', 1), ('clog', 1), ('applauding', 1), ('hubs', 1), ('artistic', 1), ('sahar', 1), ('sepehri', 1), ('evangelina', 1), ('elizondo', 1), ('phantom', 1), ('915', 1), ('konarak', 1), ('prediction', 2), ('furnished', 1), ('spaces', 1), ('wmo', 1), ('factored', 1), ('salaheddin', 1), ('talib', 1), ('nader', 1), ('shaban', 1), ('seaport', 1), ('1750', 1), ('lithuanians', 1), ('slovaks', 6), ('conducive', 3), ('chipaque', 1), ('policymaking', 1), ('sabirjon', 1), ('yakubov', 3), ('kwaito', 1), ('derivative', 1), ('mbalax', 1), ('youssou', 1), ("n'dour", 1), ('ovation', 2), ('stiffly', 1), ('stumbled', 2), ('fracturing', 1), ('inequalities', 1), ('batna', 1), ('likened', 2), ('roulette', 1), ('irresponsibly', 1), ('sittwe', 1), ('birdflu', 1), ('sparrowhawk', 1), ('jubilation', 1), ('unfunded', 1), ('specializing', 1), ('warriors', 2), ("jama'at", 1), ("qu'ran", 1), ('nahda', 1), ('rusafa', 1), ('decisively', 2), ('latifiyah', 1), ('aps', 1), ('intercede', 1), ('151', 1), ('austere', 1), ('pachyderm', 1), ('foments', 1), ('perception', 1), ('favoritism', 1), ('crimp', 1), ('pastrana', 5), ('arbour', 1), ('indiscriminate', 2), ('pastoral', 1), ('nomadism', 1), ('sedentary', 1), ('quarreled', 1), ('literate', 1), ('indebtedness', 1), ('saa', 1), ('duhalde', 1), ('peso', 3), ('bottomed', 1), ('audacious', 1), ('understating', 1), ('exacerbating', 1), ('dominica', 1), ('ecotourism', 2), ('geothermal', 1), ('lightening', 1), ('loads', 2), ('luxuries', 1), ('overburdened', 1), ('traveler', 4), ('intensely', 1), ('shining', 1), ('afforded', 3), ('galloped', 2), ('quarreling', 1), ('hopped', 1), ('groaned', 3), ('antagonists', 1), ('comb', 1), ('fahim', 1), ('kohdamani', 2), ('emroz', 1), ('ajmal', 2), ('alamzai', 2), ('didadgah', 1), ('rodong', 1), ('wenesday', 1), ('organizational', 1), ('faulted', 1), ('responders', 1), ('toilets', 2), ('gijira', 1), ('fdic', 1), ('tartous', 1), ('rainstorm', 1), ('nirvana', 2), ('cobain', 4), ('wed', 2), ('bean', 1), ('flannel', 1), ('sweaters', 1), ('smells', 1), ('patat', 1), ('jabor', 1), ('dalian', 1), ('tian', 1), ('neng', 1), ('meishan', 1), ('vent', 1), ('reformer', 1), ('lukewarm', 1), ('realistic', 2), ('puebla', 2), ('mosleshi', 1), ('637', 1), ('texmelucan', 1), ('rehn', 3), ('herceptin', 2), ('drugmaker', 2), ('ayar', 1), ('postpones', 1), ('lazio', 1), ('fiorentina', 1), ('manipulate', 3), ('serie', 1), ('handpicking', 1), ('relegation', 1), ('demotions', 1), ('164', 1), ('fulani', 1), ('herdsmen', 1), ('martyrdom', 4), ('hamm', 6), ('creationism', 1), ('intelligent', 2), ('gymnastics', 1), ('healed', 1), ('molucca', 1), ('ternate', 1), ('vivanews', 1), ('pm', 2), ('radius', 2), ('rotator', 1), ('cuff', 1), ('keywords', 1), ('chat', 1), ('alluding', 2), ('nsa', 6), ('accessed', 1), ('storing', 1), ('wiretap', 1), ('pbsnewshour', 1), ('alternates', 1), ('mighty', 1), ('najjar', 1), ('badawi', 2), ('demonizing', 3), ('meza', 1), ('oleksander', 1), ('horobets', 1), ('despicable', 1), ('wallet', 1), ('rfid', 1), ('biographical', 1), ('bezsmertniy', 1), ('terrence', 1), ('craybas', 4), ('corina', 1), ('morariu', 1), ('mashona', 1), ('obliged', 2), ('qutbi', 1), ('dome', 5), ('tahhar', 2), ('toluca', 1), ('alistair', 1), ('pietersen', 2), ('367', 1), ('323', 1), ('aristolbulo', 1), ('isturiz', 1), ('timergarah', 1), ('sibbi', 1), ('streamlines', 1), ('siphon', 1), ('pullouts', 1), ('rouen', 1), ('haze', 3), ('islamophobia', 2), ('xenophobia', 2), ('manifestations', 1), ('disproportionally', 1), ('overrepresented', 1), ('pekanbara', 1), ('islamophobic', 1), ('underdocumented', 1), ('underreported', 1), ('hexaflouride', 1), ('tackling', 1), ('tindouf', 1), ('lugar', 3), ('yudhyono', 1), ('peipah', 2), ('lekima', 1), ('guanglie', 1), ('mesopotamia', 1), ('abnormalities', 1), ('tassos', 2), ('papadopoulos', 5), ('haves', 1), ('nots', 1), ('satisfy', 3), ('keith', 1), ('snorted', 1), ('ashes', 2), ('nme', 2), ('ingesting', 1), ('cremated', 1), ('dad', 3), ('cared', 1), ('bert', 1), ('famously', 1), ('luck', 7), ('petitions', 1), ('dissenting', 1), ('edmund', 1), ('vacated', 1), ('strikingly', 1), ('sews', 1), ('devra', 1), ('robitaille', 1), ('fliers', 1), ('washingtonians', 1), ('designs', 5), ('mousa', 1), ("'80s", 1), ('irrelevent', 1), ('confiscation', 2), ('valuables', 1), ('khagrachhari', 2), ('bengali', 4), ('statments', 1), ('baghaichhari', 1), ('karradah', 2), ('gravesite', 1), ('zelenovic', 2), ('khanty', 1), ('mansiisk', 1), ('padded', 1), ('burdensome', 1), ('po', 1), ('psl', 1), ('straddling', 1), ('overexploitation', 1), ('deepwater', 1), ('kjetil', 1), ('aamodt', 1), ('110474537', 1), ('concessionary', 1), ('globally', 2), ('ladder', 1), ('broadened', 3), ('deepened', 1), ('parity', 1), ('aggravating', 2), ('110625', 1), ('maaouya', 1), ('sidi', 2), ('cheikh', 2), ('abdallahi', 2), ('afro', 2), ('mauritanians', 1), ('moor', 1), ('berber', 1), ("qa'ida", 1), ('aqim', 1), ('combi', 1), ('jar', 1), ('smeared', 1), ('suffocated', 1), ('expiring', 1), ('fondles', 1), ('nurtures', 1), ('hates', 1), ('neglects', 1), ('caressed', 1), ('smothered', 1), ('nurtured', 1), ('banjo', 1), ('halifax', 2), ('passaro', 7), ('plotters', 1), ('simulating', 2), ('goldschmidt', 2), ('alishar', 1), ('rizgar', 2), ('arsala', 3), ('mirajuddin', 1), ('giro', 1), ('melt', 4), ('sheet', 3), ('pole', 4), ('rignot', 1), ('glaciers', 3), ('corpus', 3), ('christi', 3), ('kenedy', 1), ('neal', 1), ('redraw', 3), ('unmonitored', 1), ('josslyn', 1), ('aberle', 1), ('incarceration', 1), ('unpaid', 2), ('readied', 1), ('homayun', 1), ('interpret', 2), ('combining', 3), ('khamail', 1), ('reassess', 1), ('wnba', 1), ('playoff', 1), ('hawi', 1), ('parma', 4), ('peeling', 1), ('rides', 1), ('khao', 1), ('lak', 1), ('agitated', 3), ('trumpeting', 1), ('trunks', 1), ('calamities', 1), ('perishable', 1), ('dodger', 1), ('millionaire', 2), ('hopkins', 2), ('walikale', 3), ('alison', 1), ('forges', 1), ('karate', 2), ('eddie', 2), ('enzo', 2), ('sportscar', 1), ('irwindale', 1), ('speedway', 1), ('redline', 2), ('deuce', 1), ('bigalow', 1), ('sadek', 1), ('exotic', 3), ('enzos', 1), ('anheuser', 4), ('busch', 4), ('brewing', 1), ('bottler', 1), ('budweiser', 2), ('immense', 2), ('brewer', 1), ('caldera', 4), ('coltan', 1), ('cassiterite', 1), ('diaoyu', 1), ('unguarded', 1), ('perimeter', 1), ('leftover', 1), ('maaleh', 3), ('nullified', 3), ('retroactively', 1), ('shifts', 2), ('hib', 2), ('shuffle', 1), ('541', 1), ('proportions', 1), ('dharmendra', 1), ('rajaratnam', 1), ('placards', 2), ('sasa', 1), ('radak', 1), ('ovcara', 1), ('saidati', 1), ('mukakibibi', 2), ('umurabyo', 2), ('publishers', 1), ('hatf', 2), ('agnes', 1), ('uwimana', 1), ('disobedience', 1), ('224', 3), ('462', 1), ('745', 1), ('arenas', 1), ('uladi', 1), ('mazlan', 1), ('jusoh', 1), ('resonated', 1), ('deepens', 1), ('katarina', 1), ('srebotnik', 5), ('asb', 2), ('shinboue', 1), ('asagoe', 2), ('leanne', 1), ('lubiani', 1), ('87th', 1), ('qantas', 4), ('a380', 1), ('a380s', 2), ('midair', 1), ('rolls', 1), ('royce', 1), ('turbines', 1), ('volleys', 1), ('bani', 1), ('navarre', 1), ('corpsman', 1), ('kor', 1), ('affirmation', 1), ('yankham', 1), ('kengtung', 1), ('silently', 2), ('countdown', 1), ('manan', 1), ('452', 1), ('edible', 1), ('kavkazcenter', 1), ('khalim', 1), ('sadulayev', 1), ('rivaling', 1), ('telethons', 1), ('kalma', 2), ('acropolis', 2), ('unesco', 1), ('parthenon', 1), ('392', 1), ('485', 1), ('clamped', 1), ('drywall', 1), ('helipad', 1), ('kruif', 1), ('bedfordshire', 1), ('khayam', 3), ('bedford', 1), ('skyjackers', 1), ('mourn', 5), ('straying', 2), ('expedite', 2), ('726', 1), ('atwood', 2), ('artificially', 2), ('chang', 4), ('bestseller', 1), ('nanking', 1), ('cola', 3), ('pesticides', 1), ('residue', 1), ('cse', 3), ('statistically', 1), ('stringent', 3), ('kennedys', 1), ('jacqueline', 1), ('onassis', 1), ('stillborn', 1), ('repose', 1), ('hkd', 1), ('konstanz', 1), ('hajdib', 2), ('suitcases', 1), ('dortmund', 1), ('koblenz', 1), ('kazmi', 1), ('outnumbering', 1), ('8000', 1), ('hycamtin', 2), ('cisplatin', 1), ('cervix', 1), ('organs', 3), ('prolonging', 1), ('hina', 1), ('miljus', 3), ('jutarnji', 1), ('bats', 1), ('loj', 2), ('diligently', 1), ('cowboy', 2), ('shy', 1), ('casual', 1), ('bankrupt', 3), ('grinstein', 1), ('destinations', 2), ('rafat', 1), ('ecc', 2), ('costliest', 1), ('burdens', 1), ('rmi', 2), ('downsizing', 1), ('cooled', 1), ('lackluster', 2), ('psa', 1), ('nagorno', 1), ('karabakh', 1), ('chiefdoms', 1), ('springboard', 1), ('1844', 1), ('dominicans', 1), ('unsettled', 2), ('leonidas', 1), ('trujillo', 1), ('1930', 2), ('balaguer', 3), ('popes', 2), ('1870', 1), ('circumscribed', 1), ('lateran', 1), ('catholicism', 3), ('concordat', 1), ('primacy', 1), ('interreligious', 2), ('profess', 1), ('ungrudgingly', 1), ('commiserated', 1), ('surely', 1), ('vasp', 3), ('celma', 1), ('slater', 2), ('illustrious', 1), ('inhabitant', 1), ('mcqueen', 2), ('mc', 1), ("'70s", 1), ('bullitt', 1), ('papillon', 1), ('memorabilia', 1), ('boyhood', 1), ('yung', 1), ('meaningless', 1), ('graz', 1), ('saturated', 2), ('nozari', 1), ('dormitory', 2), ('hamshahri', 1), ('shargh', 1), ('unforgivable', 2), ('chow', 1), ('panyu', 2), ('chilled', 1), ('fuzhou', 1), ('antibiotics', 4), ('jessica', 1), ('fiance', 1), ('raviglione', 3), ('xtr', 1), ('ludford', 1), ('hanjiang', 1), ('mashjid', 1), ('jalna', 1), ('osaid', 1), ('falluji', 1), ('najdi', 1), ('newsletter', 1), ('sawt', 1), ('disseminate', 1), ('jihadist', 1), ('khaleeq', 1), ('tumbling', 1), ('kel', 1), ('seun', 1), ('kuti', 2), ('fela', 1), ('nnamdi', 1), ('moweta', 1), ('leo', 1), ('cheaply', 1), ('undercutting', 1), ('bomblets', 1), ('hyper', 1), ('eavesdrop', 3), ('clearances', 1), ('assistants', 1), ('dubs', 1), ('vivanco', 2), ('discriminating', 1), ('disregarding', 1), ('laguna', 1), ('aynin', 1), ('haaretzthat', 1), ('zigana', 1), ('gumushane', 1), ('surround', 1), ('intersect', 1), ('semiarid', 1), ('arla', 1), ('loses', 1), ('chretien', 1), ('secularist', 1), ('koksal', 1), ('toptan', 1), ('rim', 1), ('disowning', 1), ('lustiger', 2), ('notre', 1), ('dame', 1), ('patriarchal', 1), ('khalayleh', 1), ('doomsday', 1), ('gobe', 1), ('squadrons', 1), ('uprooting', 1), ('admits', 2), ('conclusive', 1), ('waded', 1), ('unprovoked', 1), ('faults', 2), ('habsadeh', 2), ('shargudud', 2), ('hamidreza', 1), ('estefan', 1), ('feliciano', 2), ('thalia', 1), ('jamrud', 1), ('venevision', 1), ('ovidio', 1), ('cuesta', 1), ('baramullah', 1), ('srinigar', 1), ('assembling', 1), ('bikaner', 1), ('sketch', 1), ('amos', 1), ('kimunya', 2), ('esmat', 1), ('klain', 1), ('599', 1), ('emanate', 1), ('dahl', 1), ('froeshaug', 1), ('4x5', 1), ('atiku', 1), ('lai', 1), ('immoral', 1), ('yambio', 1), ('seyi', 1), ('memene', 1), ('kerosene', 1), ('veil', 1), ('ramdi', 1), ('deepak', 1), ('gurung', 1), ('256', 3), ('voyages', 1), ('bago', 1), ('kyauk', 1), ('ein', 2), ('kanyutkwin', 1), ('allert', 1), ('behave', 2), ('428', 1), ('sounding', 1), ('kleiner', 1), ('caufield', 1), ('byers', 1), ('blossomed', 1), ('lockerbie', 2), ('nurses', 5), ('machakos', 1), ('drank', 1), ('brew', 2), ('makutano', 1), ('methanol', 1), ("chang'aa", 1), ('akhmad', 2), ('palpa', 1), ('bitlis', 1), ('sulaimaniyah', 2), ('pejak', 1), ('hyperinflation', 2), ('cessation', 1), ('midway', 3), ('nwr', 3), ('hawaiian', 1), ('papahanaumokuakea', 1), ('refuges', 2), ('terrestrial', 1), ('corals', 1), ('shellfish', 1), ('seabirds', 1), ('insects', 1), ('vegetation', 2), ('colon', 1), ('traverse', 1), ('firsts', 1), ('aruban', 1), ('aruba', 2), ('dip', 1), ('footing', 2), ('frequenting', 1), ('lamentation', 1), ('fuss', 1), ('batsmen', 1), ('jaques', 2), ('harbhajan', 2), ('493', 1), ('332', 2), ('shbak', 3), ('intimidating', 1), ('intra', 1), ('sucre', 1), ('gasquet', 3), ('mirnyi', 3), ('olivier', 1), ('rochus', 1), ('ramstein', 1), ('pontifical', 1), ('poupard', 1), ('castel', 1), ('gandolfo', 1), ('byzantine', 3), ('nun', 5), ('theorized', 1), ('othman', 1), ('swank', 1), ('guild', 3), ('cate', 3), ('blanchett', 3), ('portrayal', 1), ('katherine', 1), ('hepburn', 1), ('aviator', 2), ('freeman', 1), ('shaloub', 1), ('orbach', 1), ('ensemble', 1), ('quirky', 1), ('sideways', 2), ('duress', 2), ('jpmorgan', 1), ('materazzi', 4), ('midfielder', 1), ('ramming', 1), ('verbally', 2), ('gunning', 2), ('wael', 1), ('rubaei', 1), ('arafa', 2), ('izzariya', 1), ('schatten', 2), ('embryos', 1), ('embryonic', 2), ('preclude', 1), ('underlings', 1), ('coercion', 1), ('impropriety', 1), ('127', 1), ('fadela', 1), ('chaib', 1), ('sufferer', 1), ('315', 1), ('beleaguered', 2), ('kandili', 1), ('anatolianews', 1), ('erzurum', 1), ('governorates', 1), ('qods', 1), ('explosively', 2), ('efps', 2), ('shorten', 1), ('healing', 2), ('adhamiya', 2), ('significance', 2), ('editorials', 1), ('gye', 1), ('wafd', 1), ('europol', 3), ('baleno', 1), ('newseum', 4), ('fatigues', 1), ('revote', 1), ('picnicking', 1), ('aleg', 1), ('qadis', 1), ('badghis', 5), ('iveta', 2), ('benesova', 4), ('na', 1), ('jarmila', 1), ('gajdosova', 1), ('ting', 1), ('tiantian', 1), ('bild', 4), ('rtl', 2), ('scandalized', 1), ('anymore', 1), ('downriver', 2), ('mains', 1), ('mechanics', 1), ('ana', 1), ('rauchenstein', 1), ('sabaa', 1), ('inflexible', 2), ('spelled', 1), ('guzzling', 1), ('drel', 2), ('46th', 2), ('contradict', 2), ('lobes', 1), ('sedatives', 1), ("l'aquila", 4), ('caricatures', 2), ('soir', 1), ('acquaint', 1), ('acosta', 4), ('inflammable', 1), ('consumes', 1), ('philanthropy', 1), ('mikati', 5), ('nhs', 3), ('loopholes', 1), ('hutton', 1), ('paktiawal', 3), ('disgust', 2), ('forgivable', 1), ('faras', 1), ('jabouri', 2), ('aqidi', 1), ('163', 3), ('joanne', 1), ('moore', 6), ('fahad', 1), ('cured', 1), ('melanne', 1), ('verveer', 3), ('epidemics', 1), ('invests', 2), ('yomiuri', 2), ('nanjing', 1), ('haerbaling', 1), ('divulge', 1), ('crafted', 1), ('naimi', 3), ('pennies', 1), ('counselor', 2), ('tsholotsho', 1), ('chinotimba', 1), ('inbalance', 1), ('madero', 1), ('otis', 1), ('powerlines', 1), ('whiskey', 1), ('gunshots', 2), ('banderas', 4), ('710', 2), ('abolishing', 1), ('commutes', 1), ('convicts', 2), ('footprints', 1), ('khuzestan', 1), ('saudia', 1), ('jules', 1), ('guere', 1), ('influnce', 1), ('majzoub', 1), ('samkelo', 1), ('mokhine', 1), ('jehangir', 1), ('mirza', 1), ('enthusiastically', 1), ('acknowledgment', 1), ('muqrin', 2), ('thrifty', 1), ('handmade', 1), ('ellice', 3), ('polynesians', 1), ('micronesians', 1), ('tonga', 3), ('squash', 2), ('vanilla', 1), ('tongan', 1), ('upturn', 1), ('tanganyika', 1), ('zanzibar', 3), ('minimizing', 1), ('negation', 1), ('1010', 1), ('tifa', 1), ('fashioning', 1), ('fowler', 3), ('twigs', 2), ('thrush', 1), ('fitting', 5), ('intently', 1), ('upwards', 1), ('trod', 2), ('viper', 4), ('swoon', 1), ('purposed', 1), ('myself', 3), ('unawares', 1), ('snares', 1), ('ahmedou', 1), ('ashayier', 1), ('kouk', 1), ("sa'dun", 1), ('hamduni', 1), ('foreman', 4), ('talha', 2), ('hmas', 1), ('fitted', 1), ('turnbull', 1), ('awantipora', 1), ('saldanha', 1), ('lateef', 1), ('adegbite', 1), ('tenets', 1), ('ulemas', 2), ('betrayed', 2), ('darkest', 1), ('zarqwai', 1), ('scrubbed', 1), ('hangar', 1), ('dovish', 2), ('annex', 1), ('blocs', 2), ('unilaterally', 2), ('sabri', 1), ('annexing', 1), ('disengagement', 3), ('shas', 3), ('abolish', 3), ('335', 1), ('treetops', 1), ('compliments', 1), ('obaidullah', 1), ('akhund', 2), ('lal', 1), ('advani', 4), ('jinnah', 2), ('subcontinent', 1), ('patched', 1), ('vassily', 1), ('kononov', 3), ('slandered', 1), ('reese', 2), ('yoshie', 1), ('sato', 1), ('yokosuka', 1), ('kitty', 1), ('assessors', 1), ('vimpelcom', 1), ('jaunpur', 1), ('patna', 1), ('rdx', 2), ('hexogen', 1), ('unclaimed', 1), ('bavaria', 1), ('allay', 1), ('aggressions', 1), ('58th', 1), ('wrecked', 1), ('shadows', 1), ('shines', 1), ('aijalon', 1), ('gomes', 2), ('renunciation', 2), ('unhindered', 1), ('kasab', 1), ('taiba', 2), ('faizullah', 1), ('akhbar', 1), ('youm', 1), ('sects', 1), ('solecki', 3), ('jamia', 1), ('americanism', 1), ('049', 1), ('verizon', 2), ('kgb', 2), ('stepan', 1), ('sukhorenko', 2), ('proclaiming', 2), ('georgi', 1), ('parvanov', 1), ('prolongs', 1), ('normalized', 1), ('hennadiy', 1), ('vasylyev', 1), ('epa', 1), ('sandwich', 2), ('ecweru', 1), ('swahili', 1), ('mulongoti', 1), ('bududa', 1), ('header', 1), ('khedira', 1), ('82nd', 1), ('mesut', 1), ('oezil', 1), ('mueller', 6), ('edinson', 1), ('cavani', 1), ('equalized', 1), ('forlan', 1), ('marcell', 1), ('jansen', 1), ('montagnards', 3), ('resettling', 1), ('unsubstantiated', 1), ('smearing', 2), ('categorized', 1), ('shegag', 1), ('karo', 1), ('hudaidah', 1), ('411', 1), ('luca', 2), ('badoer', 1), ('maranello', 1), ('reggio', 1), ('emilia', 1), ('venice', 1), ('ignites', 1), ('scoop', 1), ('footprint', 1), ('subsurface', 1), ('679', 1), ('cantv', 2), ('447', 1), ('yasar', 2), ('buyukanit', 1), ('tokage', 3), ('tolling', 2), ('wadowice', 1), ('knees', 1), ('wept', 2), ('worshipped', 1), ('readies', 1), ('gurirab', 1), ('redistribution', 1), ('sensing', 1), ('kindergarten', 3), ('petting', 1), ('binyamina', 1), ('symptom', 1), ('chilling', 1), ('echoed', 2), ('bullying', 1), ('deprive', 1), ('mastering', 1), ('wabho', 1), ('wajama', 2), ('electricial', 1), ('gojko', 2), ('jankovic', 5), ('flourished', 2), ('trusting', 1), ('triumf', 1), ('riza', 2), ('greenland', 6), ('vikings', 1), ('easternmost', 1), ('kwajalein', 1), ('usaka', 1), ('1881', 1), ('bourguiba', 2), ('paroled', 1), ('offender', 1), ('joachim', 1), ('ruecker', 1), ('repressing', 1), ('unmatched', 1), ('zine', 1), ('abidine', 1), ('tunis', 1), ('ghannouchi', 1), ("m'bazaa", 1), ('viable', 2), ('fluctuated', 1), ('terrible', 1), ('alderman', 2), ('raccoon', 2), ('zoological', 1), ('tales', 1), ('graze', 1), ('tidings', 1), ('hound', 3), ('whence', 1), ('entertained', 1), ('boatmen', 1), ('outpouring', 2), ('emotion', 1), ('yielding', 1), ('gasped', 1), ('wretched', 1), ('perilous', 1), ('clauses', 3), ('renoun', 1), ('elves', 1), ('subordinate', 1), ('leonardo', 1), ('dicaprio', 2), ('overaggressive', 1), ('sparing', 2), ('falun', 5), ('gong', 5), ('ching', 1), ('hsi', 1), ('mistreats', 1), ('baltim', 1), ('kafr', 1), ('zigazag', 1), ('kakooza', 2), ('entebbe', 1), ('jovica', 1), ('simatovic', 1), ('insidious', 1), ('nihilism', 1), ('fanatic', 1), ('copts', 1), ('nightly', 1), ('renegotiating', 1), ('karabilah', 4), ('cone', 1), ('dye', 2), ('foam', 1), ('insulation', 2), ('disintegration', 1), ('goldsmith', 1), ("u'zayra", 1), ('larkin', 1), ('khayber', 1), ('hyuck', 1), ('format', 1), ('worthless', 1), ('satterfield', 4), ('617', 2), ('gulag', 2), ('dae', 1), ('ri', 2), ('jo', 3), ('hamash', 1), ('levied', 1), ('bextra', 2), ('prescriptions', 1), ('somavia', 1), ('spelling', 1), ('passersby', 2), ('sayings', 1), ('donetsk', 2), ('borjomi', 1), ('jaca', 1), ('pyeongchang', 1), ('salzburg', 1), ('questionnaire', 1), ('accommodations', 1), ('6th', 1), ('belligerence', 2), ('negotiation', 3), ('buddhism', 1), ('anuradhapura', 1), ('circa', 2), ('polonnaruwa', 1), ('1070', 1), ('1200', 1), ('mcchrystal', 1), ('pinerolo', 1), ('1500s', 1), ('sliding', 4), ('glides', 1), ('circular', 1), ('matesi', 3), ('briquettes', 1), ('norpro', 1), ('tenaris', 2), ('xingguang', 1), ('1796', 1), ('1802', 1), ('alain', 1), ('pellegrini', 1), ('ceylon', 1), ('lubero', 1), ('guehenno', 1), ('eelam', 1), ('overshadow', 1), ('natasha', 2), ('kofoworola', 1), ('quist', 3), ('petkoff', 3), ('anguish', 1), ('cual', 1), ('ojeda', 1), ('borges', 1), ('proficiency', 1), ('indoctrinate', 1), ('bounds', 1), ('weekends', 1), ('gravel', 1), ('kihonge', 1), ('supervising', 1), ('wreaths', 1), ('yugoslavian', 1), ('dacic', 1), ('colleen', 1), ('larose', 3), ('drillings', 1), ('arising', 3), ('pedraz', 1), ('couso', 2), ('taras', 1), ('portsyuk', 1), ('maori', 2), ('buenaventura', 1), ('chieftains', 1), ('waitangi', 1), ('npa', 1), ('1843', 1), ('tsotne', 1), ('gamsakhurdia', 5), ('zviad', 1), ('hajan', 1), ('coleman', 2), ('lapsed', 1), ('printemps', 1), ('464', 1), ('dispatching', 1), ('brandished', 2), ('vampire', 1), ('rigorous', 2), ('refill', 1), ('usage', 2), ('wraps', 2), ('trespassing', 1), ('botanical', 2), ('seini', 1), ('sperling', 4), ('leniency', 1), ('rebuke', 1), ('khamas', 1), ('hajem', 1), ('depended', 3), ('bole', 1), ('nihal', 1), ('denigrates', 1), ('ruble', 1), ('steward', 1), ('codes', 1), ('göteborg', 1), ('krisztina', 1), ('nagy', 1), ('anp', 3), ('tiechui', 1), ('paramedics', 1), ('irrawady', 1), ('fazul', 1), ('stanezai', 1), ('impervious', 1), ('buster', 1), ('lioness', 1), ('harden', 1), ('drift', 1), ('easley', 1), ('laszlo', 1), ('solyom', 1), ('hares', 3), ('mufti', 1), ('shuttles', 1), ('jalula', 1), ('yourselves', 1), ('amerli', 1), ('petrol', 1), ('invariably', 1), ('passers', 1), ('leveraged', 1), ('maltese', 1), ('faroe', 2), ('faroese', 1), ('moorish', 1), ('dynasties', 1), ('258', 3), ("sa'adi", 1), ('1578', 1), ('alaouite', 1), ('1860', 1), ('internationalized', 1), ('tangier', 1), ('bicameral', 1), ('moderately', 1), ('taboo', 2), ('hobbles', 1), ('overspending', 1), ('overborrowing', 1), ('realizing', 3), ('stables', 1), ('favourite', 2), ('lapdog', 4), ('licked', 1), ('dainty', 1), ('lap', 2), ('blinking', 1), ('stroked', 1), ('commenced', 1), ('prancing', 1), ('pitchforks', 1), ('clumsy', 1), ('jesting', 1), ('joke', 1), ('summers', 1), ('natured', 1), ('shibis', 1), ('dhimbil', 2), ('hawiye', 2), ('llasa', 1), ('radhia', 1), ('achouri', 1), ('trily', 1), ('showdowns', 1), ('reprimands', 1), ('sanaa', 1), ('coleand', 1), ('supertanker', 1), ('limburg', 1), ('fawzi', 2), ('supervises', 1), ('dmitrivev', 1), ('prestwick', 1), ('geithner', 2), ('jagdish', 1), ('tytler', 2), ('yongbyon', 1), ('renaissance', 1), ('kedallah', 1), ('younous', 1), ('purging', 1), ('demeans', 1), ('gratuitous', 1), ('instructional', 1), ('kuta', 1), ('eide', 6), ('taormina', 1), ('suggestive', 1), ('taboos', 1), ('menstrual', 1), ('unidentifed', 1), ('provocateurs', 1), ('enticing', 2), ('385', 1), ('gration', 3), ('falsifying', 1), ('saqib', 3), ('shootouts', 1), ('eastward', 1), ('alternately', 1), ('hendrik', 1), ('taatgen', 1), ('ambrosio', 1), ('intakes', 1), ('wastewater', 1), ('yingde', 1), ('reservoirs', 1), ('neurological', 1), ('knockout', 1), ('strongmen', 1), ('nazareth', 3), ('yardenna', 1), ('dwelling', 1), ('courtyard', 1), ('annunciation', 1), ('copenhagen', 1), ('enacting', 1), ('snub', 1), ('siachen', 1), ('equate', 2), ('kalikot', 1), ('seiken', 1), ('sugiura', 2), ('spouses', 1), ('torres', 1), ('yake', 1), ('867', 1), ('headbands', 1), ('453', 2), ('577', 1), ('969', 1), ('729', 1), ('lewthwaite', 2), ('jamaican', 3), ('germaine', 1), ('fanatics', 1), ('twisted', 1), ('holdouts', 1), ('aurobindo', 1), ('pharma', 1), ('lamivudine', 1), ('zidovudine', 1), ('nevirapine', 6), ('regimen', 1), ('patents', 1), ('sharpened', 1), ('daley', 1), ('rahm', 1), ('lid', 2), ('repays', 1), ('liempde', 1), ('nuriye', 1), ('kesbir', 1), ('climbs', 1), ('cot', 1), ('greeks', 2), ('breasts', 1), ('exam', 1), ('malakhel', 1), ('osmani', 1), ('aubenas', 1), ('reopens', 1), ('xinjuan', 1), ('bruises', 1), ('georg', 1), ('trondheim', 1), ('vague', 1), ('bernt', 1), ('eidsvig', 2), ('choir', 2), ('pedophilia', 1), ('jac', 2), ('takeifa', 1), ('keita', 1), ('ricci', 2), ('shyrock', 1), ('hulya', 2), ('kocyigit', 2), ('675', 1), ('architectural', 1), ('profound', 2), ('burgeoning', 1), ('hinting', 1), ('managua', 1), ('disavowed', 1), ('murr', 1), ('shameful', 2), ('thuggery', 1), ('michuki', 1), ('bitten', 1), ('doghmush', 2), ('neskovic', 1), ('bijeljina', 1), ('gebran', 2), ('amagasaki', 1), ('osaka', 1), ('milososki', 1), ('beneficial', 1), ('mia', 1), ('crvenkovski', 1), ('nikola', 2), ('gruevski', 1), ('swerved', 1), ('althing', 1), ('930', 1), ('askja', 1), ('emigrated', 3), ('paracel', 2), ('pattle', 1), ('woody', 1), ('1788', 1), ('islanders', 1), ('mechanized', 1), ('bailouts', 2), ('rutte', 1), ('dwindling', 2), ('industrialization', 1), ('soles', 1), ('fasten', 2), ('idols', 1), ('unlucky', 1), ('pedestal', 1), ('hardly', 1), ('sultans', 1), ('splendour', 1), ('dukeness', 1), ('liege', 1), ('gorgeous', 1), ('jewel', 1), ('gleaming', 1), ('badgesty', 1), ('catarrh', 1), ('overtaken', 2), ('rabbit', 1), ('obstructs', 1), ('amusement', 2), ('cheyenne', 1), ('showers', 1), ('wednesdays', 1), ('upholding', 1), ('abbasali', 1), ('kadkhodai', 1), ('tahreer', 1), ('compare', 2), ('peshmerga', 1), ('ludlam', 2), ('christman', 1), ('starved', 1), ('menoufia', 1), ('43nd', 1), ('unasur', 1), ('clarifies', 3), ('basile', 1), ('casmoussa', 1), ('guenael', 1), ('rodier', 1), ('glacial', 2), ('attributing', 1), ('colonia', 1), ('cachet', 1), ('burrow', 1), ('gino', 1), ('casassa', 1), ('repeals', 1), ('segun', 1), ('odegbami', 1), ('nfa', 1), ('salisu', 1), ('hendarso', 1), ('danuri', 2), ('sumatran', 1), ('religiously', 1), ('269', 1), ('alienate', 1), ('hazel', 1), ('blears', 1), ('khalidiyah', 1), ('bakwa', 1), ('asghari', 7), ('ziba', 1), ('sharq', 1), ('awsat', 1), ('tempers', 1), ('designating', 1), ('excited', 1), ('spontaneous', 2), ('motel', 1), ('dietary', 1), ('hauled', 1), ('pragmatists', 1), ('copes', 1), ('revisited', 1), ('asteroid', 5), ('stargazers', 1), ('wd5', 1), ('1908', 2), ('megaton', 1), ('exhumation', 1), ('glorious', 1), ('auwaerter', 2), ('johns', 1), ('fabrizio', 1), ('meoni', 3), ('kiffa', 1), ('motorcyclists', 1), ('charter97', 1), ('cyril', 2), ('despres', 1), ('ktm', 1), ('8956', 1), ('ceremon', 1), ('flabbergasted', 1), ('asparagus', 1), ('dirt', 2), ('acidic', 1), ('vapor', 1), ('cassettes', 1), ('augment', 1), ('mcbride', 1), ('vocalist', 1), ('nichols', 1), ('craig', 1), ('wiseman', 1), ('peformed', 1), ('mcgraw', 1), ('gretchen', 1), ('horizon', 1), ('kris', 1), ('kristofferson', 1), ('zamani', 1), ('benisede', 3), ('speedboats', 1), ('coherent', 1), ('nyein', 1), ('ko', 1), ('naing', 1), ('appropriated', 1), ('fadhila', 1), ('perverted', 1), ('cctv', 1), ('nozzles', 1), ('salomi', 1), ('reads', 1), ('blackwater', 1), ('incited', 2), ('ire', 1), ('benedicto', 1), ('jimenez', 3), ('commercio', 1), ('zevallos', 1), ('dee', 1), ('boersma', 1), ('serfdom', 1), ('dumitru', 1), ('newsroom', 1), ('formalities', 1), ('dens', 1), ('airdrop', 1), ('ingmar', 1), ('bergman', 3), ('actresses', 1), ('ullman', 1), ('bibi', 1), ('andersson', 1), ('faro', 1), ('strawberries', 1), ('madness', 1), ('tinged', 1), ('melancholy', 1), ('humor', 1), ('iconic', 1), ('knight', 1), ('bylaw', 1), ('thg', 1), ('tetrahydrogestrinone', 1), ('boa', 1), ('mailbox', 1), ('measurer', 1), ('nist', 4), ('machining', 1), ('invent', 1), ('braille', 1), ('nanotechnology', 1), ('cybersecurity', 1), ('grid', 1), ('standardized', 1), ('hoses', 1), ('mummified', 1), ('mummy', 2), ('earthenware', 1), ('sarcophagus', 2), ('teir', 1), ('atoun', 1), ('theyab', 2), ('beatified', 2), ('habsburg', 1), ('sainthood', 2), ('emmerick', 1), ('visions', 1), ('mel', 1), ('gibson', 1), ('ludovica', 1), ('angelis', 1), ('beatification', 1), ('directorate', 1), ('touted', 1), ('prizren', 2), ('handovers', 1), ('vigilante', 1), ('edwin', 1), ('idema', 3), ('hornets', 3), ('lakers', 2), ('workload', 1), ('rezai', 3), ('principlists', 1), ('reem', 1), ('zeid', 1), ('khazaal', 1), ('gheorghe', 1), ('flutur', 1), ('caraorman', 1), ('leavitt', 2), ('preventive', 3), ('huy', 1), ('nga', 1), ('locus', 1), ('interwar', 2), ('moldovan', 1), ('dniester', 1), ('slavic', 1), ('transnistria', 1), ('voronin', 2), ('pcrm', 1), ('aie', 2), ('reconstituted', 1), ('barren', 1), ('heyday', 1), ('1755', 1), ('crocodile', 1), ('murderer', 1), ('bosom', 1), ('coldness', 1), ('grin', 1), ('coals', 1), ('pleasures', 1), ('thawed', 1), ('civilly', 1), ('foreshadows', 1), ('budgeted', 2), ('940', 1), ('tedder', 2), ('onerepublic', 2), ('bedingfield', 1), ('dreaming', 1), ('beatboxing', 2), ('beatboxers', 1), ('adverse', 4), ('dispenses', 1), ('anybody', 1), ('kapondi', 1), ('joshua', 1), ('kutuny', 1), ('wilfred', 1), ('machage', 1), ('mistaking', 2), ('dragmulla', 1), ('hurl', 1), ('marts', 1), ('pierce', 6), ('29th', 3), ('elvira', 1), ('nabiullina', 1), ('karel', 2), ('gucht', 1), ('shoaib', 1), ('festus', 1), ('okonkwo', 1), ('attends', 1), ('amed', 1), ('snag', 1), ('olpc', 3), ('exclusively', 1), ('loophole', 1), ('kordofan', 1), ('sla', 1), ('ghubaysh', 1), ('federations', 1), ('edel', 1), ('checkup', 1), ('poet', 1), ('rivero', 1), ('osvaldo', 2), ('espinoso', 1), ('chepe', 1), ('conspired', 1), ('gulzar', 1), ('nabeel', 1), ('shamin', 1), ('uddin', 1), ('skill', 2), ('treasures', 1), ('dakhlallah', 1), ('ideals', 1), ('brice', 1), ('hortefeux', 2), ('flashlight', 1), ('interrogator', 1), ('azmi', 1), ('bishara', 5), ('enkhbayar', 1), ('samper', 3), ('cali', 1), ('barco', 1), ('bogata', 1), ('headache', 1), ('tagging', 1), ('mastered', 1), ('doused', 1), ('methane', 3), ('unfolded', 2), ('blizzards', 1), ('herders', 1), ('afder', 1), ('liban', 1), ('gode', 1), ('deteriorate', 1), ('atomstroyexport', 1), ('blowtorch', 1), ('deteriorates', 1), ('kisangani', 1), ('kidnapper', 1), ('temur', 1), ('pasted', 1), ('krivtsov', 1), ('iraqna', 1), ('nicanor', 2), ('faeldon', 2), ('posh', 1), ('makati', 1), ('deluxe', 1), ('ghorian', 1), ('navfor', 1), ('harardhere', 1), ('sacrificial', 1), ('altar', 2), ('ubt', 1), ('sardenberg', 2), ('validity', 2), ('trampling', 1), ('keck', 1), ('infrared', 2), ('vortex', 3), ('vortices', 1), ('tf', 2), ('teenaged', 1), ('jungles', 2), ('mastung', 1), ('voltage', 2), ('naushki', 1), ('wellhead', 1), ('cawthorne', 1), ('shams', 2), ('dignified', 1), ('emails', 1), ('flakus', 1), ('weird', 1), ('mingora', 1), ('neolithic', 1), ('republished', 1), ('alok', 1), ('tomar', 2), ('lauded', 1), ('palocci', 1), ('rato', 1), ('ringing', 1), ('tet', 1), ('hermosa', 1), ('myitsone', 1), ('northernmost', 2), ('kachin', 1), ('humam', 1), ('hammoudi', 1), ('narayan', 1), ('pokhrel', 3), ('mora', 2), ('huerta', 1), ('announces', 2), ('knut', 1), ('ahnlund', 3), ('elfriede', 1), ('jelinek', 1), ('descriptions', 2), ('irreparably', 1), ('horace', 1), ('engdahl', 1), ('madison', 1), ('tinted', 1), ('glasses', 1), ('lennon', 1), ('lyricist', 1), ('taupin', 1), ('serenaded', 1), ('comedians', 2), ('whoopie', 1), ('goldberg', 1), ('portraying', 1), ('waldron', 2), ('shaheen', 1), ('nexus', 1), ('cheecha', 1), ('watni', 1), ('corvettes', 1), ('overruns', 1), ('sipah', 1), ('sahaba', 1), ('husbandry', 1), ('terrain', 4), ('upscale', 5), ('conscientious', 1), ('encompasses', 2), ('entrepreneurship', 1), ('supplements', 1), ('pretended', 1), ('lameness', 1), ('butcher', 2), ('pulse', 1), ('mouthful', 1), ('vexation', 1), ('sup', 1), ('flagon', 1), ('insert', 1), ('requital', 1), ('pager', 1), ('anesthetist', 1), ('stethoscope', 1), ('dangling', 1), ('ellison', 4), ('hardfought', 1), ('mcneill', 2), ('286', 1), ('252', 1), ('tuncay', 1), ('seyranlioglu', 2), ('heroic', 1), ('tabinda', 1), ('kamiya', 1), ('astros', 2), ('clemens', 3), ('outfielders', 1), ('sammy', 1), ('sosa', 1), ('leagues', 1), ('hander', 1), ('strikeouts', 1), ('cy', 1), ('rippled', 1), ('deauville', 1), ('kouguell', 1), ('comerio', 2), ('compares', 2), ('padang', 1), ('daraga', 1), ('durian', 1), ('catanduanes', 1), ('enezi', 1), ('kut', 1), ('rescind', 1), ('ryon', 1), ('dawoud', 1), ('rasheed', 1), ('thawing', 1), ('predictor', 1), ('groundhog', 4), ('punxsutawney', 2), ('stump', 1), ('carryover', 1), ('candlemas', 1), ('copa', 2), ('wwii', 1), ('blared', 1), ('dabbagh', 1), ('keynote', 1), ('complementary', 1), ('genome', 1), ('dripped', 1), ('notion', 1), ('atttacks', 1), ('hurriya', 1), ('kufa', 1), ('boodai', 1), ('swamped', 1), ('tearfund', 1), ('andrhra', 1), ('ar', 1), ('rutbah', 1), ('khurram', 1), ('mehran', 1), ('msn', 1), ('bing', 1), ('sufi', 1), ('sufis', 1), ('heretics', 1), ('turkman', 1), ('qaratun', 1), ('khairuddin', 1), ('nassif', 2), ('590', 1), ('yair', 1), ('klein', 2), ('landlords', 1), ('mykolaiv', 1), ('maariv', 2), ('rohingya', 2), ('chasing', 1), ('loyalist', 1), ('lvf', 1), ('tyrants', 1), ('oppress', 1), ('rocker', 1), ('springsteen', 1), ('repertoire', 1), ('bmg', 2), ('idolwinner', 1), ('refrigerated', 1), ('kabylie', 2), ('abass', 1), ('barbero', 1), ('ieds', 2), ('neutralizing', 1), ('tessa', 1), ('jowell', 1), ('expose', 1), ('baojing', 1), ('jianhao', 1), ('blackmailing', 1), ('blackmailer', 2), ('maurice', 1), ('floquet', 2), ('88th', 1), ('somme', 1), ('triomphe', 1), ('lek', 2), ('pongpa', 1), ('alimony', 2), ('wasser', 1), ('backup', 1), ('irreconcilable', 1), ('tracy', 1), ('mcgrady', 2), ('cavaliers', 2), ('lebron', 1), ('assists', 2), ('pacers', 1), ('croshere', 1), ('steals', 1), ('dunleavy', 1), ('faridullah', 1), ('kani', 1), ('wam', 1), ('interivew', 1), ('jianzhong', 1), ('surkh', 1), ('nangahar', 1), ('empowers', 2), ('penalize', 1), ('baptismal', 1), ('arranges', 1), ('shutters', 1), ('portico', 1), ('rites', 1), ('asserting', 1), ('sarabjit', 1), ('moods', 1), ('retirements', 1), ('politicized', 1), ('derg', 1), ('selassie', 1), ('mayan', 2), ('kingdoms', 1), ('colchis', 1), ('kartli', 1), ('iberia', 1), ('330s', 1), ('persians', 2), ('shevardnadze', 2), ('oceanographic', 1), ('circumpolar', 1), ('acc', 2), ('mingle', 1), ('convergence', 4), ('discrete', 1), ('ecologic', 1), ('concentrates', 1), ('approximates', 1), ('imply', 2), ('1631', 1), ('retook', 1), ('1633', 1), ('amongst', 1), ('roamed', 1), ('amused', 1), ('frightening', 3), ('frighten', 1), ('fool', 2), ('perched', 1), ('beak', 1), ('wily', 1), ('stratagem', 1), ('complexion', 1), ('deservedly', 1), ('deceitfully', 1), ('refute', 1), ('housedog', 3), ('reproached', 1), ('luxuriate', 1), ('depicted', 1), ('zuhair', 1), ('reapprove', 1), ('abiya', 1), ('rizvan', 1), ('chitigov', 1), ('shali', 1), ('rescinded', 1), ('judo', 1), ('repatriation', 1), ('ticona', 2), ('esperanza', 1), ('copiapo', 1), ('chute', 2), ('libel', 2), ('shadowed', 1), ('nazran', 1), ('zyazikov', 1), ('jafri', 1), ('sodomy', 2), ('venomous', 1), ('vindicated', 1), ('samara', 1), ('arsamokov', 1), ('ingush', 1), ('condominiums', 1), ('equipping', 1), ('interacted', 1), ('ahvaz', 2), ('kayhan', 1), ('pinas', 1), ('schoof', 1), ('ayala', 1), ('alabang', 1), ('baikonur', 1), ('cosmodrome', 1), ('pythons', 1), ('kandal', 1), ('wedded', 1), ('python', 5), ('chamrouen', 2), ('kroung', 1), ('pich', 1), ('cage', 1), ('subscribe', 1), ('animism', 1), ('inhabit', 1), ('inanimate', 1), ('snakes', 1), ('neth', 1), ('vy', 1), ('dharmsala', 1), ('acknowledging', 1), ('worthwhile', 1), ('rosaries', 1), ('colleges', 2), ('scorpion', 1), ('venom', 1), ('selectively', 1), ('516', 1), ('collier', 3), ('howell', 1), ('enlarged', 1), ('franchises', 1), ('echocardiograms', 1), ('knicks', 1), ('eddy', 1), ('curry', 1), ('irregular', 1), ('heartbeat', 1), ('hoiberg', 1), ('guenter', 1), ('verheugen', 1), ('sonntag', 2), ('sheeting', 1), ('coveted', 1), ('lithium', 4), ('entitle', 1), ('salar', 1), ('uyuni', 1), ('sics', 2), ('dahir', 1), ('aweys', 1), ('ousting', 3), ('powerless', 1), ('tvn', 1), ('stainmeier', 1), ('butmir', 1), ('eufor', 1), ('karameh', 1), ('sapporo', 2), ('dwarfed', 1), ('jeremic', 2), ('pressewednesday', 1), ('bud', 1), ('selig', 1), ('fehr', 1), ('sox', 1), ('sama', 1), ('murillo', 1), ('hokkaido', 1), ('stiffer', 1), ('cyclist', 2), ('hondo', 4), ('carphedon', 1), ('murcia', 1), ('gerolsteiner', 1), ('issori', 1), ('duggal', 2), ('scourge', 1), ('atmospheric', 2), ('buoys', 1), ('thammararoj', 1), ('killer', 1), ('cerkez', 5), ('dario', 1), ('kordic', 1), ('ganiyu', 1), ('adewale', 1), ('badoosh', 1), ('babaker', 1), ('zibari', 1), ('dodik', 2), ('lajcak', 1), ('clamps', 1), ('anand', 1), ('sterilized', 1), ('balasingham', 2), ('nimal', 1), ('siripala', 1), ('salvaging', 1), ('cabs', 1), ('greeting', 3), ('fortunate', 1), ('feasts', 1), ('pacheco', 2), ('costan', 1), ('merchants', 2), ('emphatically', 1), ('sakineh', 1), ('ashtiani', 2), ('lashes', 2), ('adultery', 2), ('starve', 1), ('inexplicable', 1), ('5\xa0storm', 1), ('measurement', 1), ('measurements', 1), ('outcries', 1), ('zim', 3), ('shinsei', 1), ('maru', 1), ('compensatiion', 1), ('bayu', 1), ('krisnamurthi', 1), ('parviz', 1), ('davoodi', 1), ('vollsmose', 1), ('odense', 1), ('conversions', 1), ('evangelization', 1), ('merapi', 3), ('volcanologist', 1), ('surano', 2), ('eruptions', 2), ('lene', 1), ('espersen', 2), ('baghran', 2), ('merimee', 3), ('understates', 1), ('thankot', 1), ('bhairahawa', 1), ('geophysical', 1), ('sparse', 1), ('guernsey', 4), ('congratulation', 1), ('summarizes', 1), ('flaring', 2), ('pollutes', 1), ('afghanis', 1), ('waistcoat', 1), ('stained', 1), ('fayaz', 4), ('biakand', 1), ('sunnah', 1), ('215', 1), ('aulnay', 1), ('eker', 1), ('mellot', 1), ('korangal', 1), ('commissions', 2), ('shon', 1), ('meckfessel', 1), ('fadlallah', 1), ('dispel', 1), ('kahar', 2), ('koyair', 1), ('drunkedness', 1), ('screaming', 2), ('documenting', 2), ('crosses', 1), ('hossam', 1), ('zaki', 1), ('sadat', 1), ('outstripped', 1), ('legia', 1), ('elijah', 1), ('cummings', 2), ('missteps', 1), ('maas', 1), ('muhamed', 1), ('sacirbey', 2), ('garza', 1), ('superdome', 1), ('workouts', 1), ('trinity', 1), ('alamodome', 1), ('herbal', 1), ('palnoo', 1), ('kulgam', 1), ('detonator', 2), ('resembling', 2), ('siasi', 1), ('unprincipled', 1), ('coldest', 1), ('mikulski', 1), ('shyster', 1), ('uhstad', 1), ('thura', 2), ('mahn', 2), ('hardliner', 1), ('indicative', 1), ('3700', 1), ('bermel', 1), ('ghormley', 2), ('slumped', 1), ('bargains', 1), ('resales', 1), ('janice', 2), ('karpinski', 4), ('kabungulu', 2), ('kibembi', 1), ('shryock', 1), ('carcasses', 1), ('manifest', 1), ('portoroz', 1), ('obita', 1), ('rostov', 1), ('flagrant', 2), ('checkups', 1), ('dhangadi', 1), ('reclaimed', 1), ('interesting', 2), ('fragmentation', 1), ('nesterenk', 1), ('cruiser', 1), ('chabanenko', 1), ('rearming', 1), ('harith', 1), ('tayseer', 1), ('endeavouron', 1), ('spacewalking', 1), ('reaffirms', 1), ('bergner', 1), ('rish', 1), ('ashfaq', 1), ('parvez', 1), ('kayani', 1), ('fatou', 1), ('bensouda', 1), ('hemingway', 1), ('herrington', 2), ('brighton', 1), ('everado', 1), ('tobasco', 1), ('montiel', 1), ('handpicked', 1), ('ofakim', 1), ('unbeaten', 2), ('resurrected', 1), ('tajouri', 1), ('campaigner', 1), ('polska', 1), ('diminishes', 1), ('junoon', 2), ('obsession', 1), ('falu', 2), ('ethnomusicologist', 1), ('q', 1), ('nabarro', 1), ('mirwaiz', 1), ('vilsack', 1), ('kucinich', 1), ('rodham', 2), ('dulaymi', 2), ('nationalizes', 1), ('poleo', 2), ('mezerhane', 2), ('eugenio', 1), ('anez', 1), ('nunez', 1), ('romani', 1), ('lately', 1), ('distract', 1), ('morshed', 1), ('ldp', 1), ('responsive', 1), ('jawed', 1), ('ludin', 1), ('sordid', 1), ('horticulture', 1), ('evolving', 1), ('derive', 1), ('onerous', 1), ('jdx', 1), ('multilaterals', 1), ('exacerbates', 1), ('tarmiyah', 1), ('judaidah', 1), ('ratios', 1), ('mingling', 1), ('lott', 2), ('witt', 2), ('zalinge', 1), ('minimized', 1), ('demoralized', 1), ('disinvest', 1), ('digits', 2), ('suleyman', 1), ('demirel', 3), ('aysegul', 1), ('esenler', 1), ('bourgas', 1), ('egebank', 1), ('joblessness', 1), ('haridy', 1), ('redmond', 2), ('mirdamadi', 1), ('pakhtoonkhaw', 1), ('iteere', 1), ('tracing', 1), ('almazbek', 1), ('atambayev', 2), ('absalon', 2), ('appointee', 1), ('hashem', 1), ('ashibli', 1), ('manie', 1), ('clerq', 1), ('torching', 1), ('jumbled', 1), ('acclaimed', 1), ('avant', 1), ('garde', 1), ('yakov', 1), ('chernikhov', 1), ('274', 1), ('antiques', 1), ('hermitage', 1), ('gilded', 1), ('ladle', 1), ('gastrostomy', 1), ('zoba', 1), ('yass', 1), ('aalam', 3), ('saloumi', 1), ('nkunda', 1), ('rutshuru', 1), ('disproportionate', 1), ('fiat', 3), ('opel', 4), ('cordero', 1), ('montezemolo', 1), ('corriere', 1), ('della', 1), ('sera', 1), ('kamin', 3), ('disregards', 1), ('kotkai', 2), ('bajur', 1), ('resealed', 1), ('squeezing', 1), ('workforces', 1), ('democratia', 1), ('datanalisis', 2), ('channu', 1), ('torchbearers', 1), ('piazza', 2), ('massaua', 1), ('livio', 1), ('berruti', 1), ('palazzo', 1), ('citta', 1), ('kissem', 1), ('tchangai', 1), ('walla', 1), ('lome', 1), ('emmanual', 1), ('townsend', 1), ('jackets', 1), ('schwarzenburg', 2), ('frenchmen', 1), ('choi', 1), ('youn', 1), ('jin', 1), ('spilt', 1), ('unjustifiable', 1), ('beruit', 1), ('odyssey', 1), ('quds', 2), ('indignation', 1), ('boulder', 1), ('zwally', 1), ('bioethics', 1), ('sperm', 1), ('kompas', 1), ('iwan', 1), ('darmawan', 2), ('rois', 1), ('underlined', 1), ('ridding', 1), ('untrue', 1), ('1452', 1), ('459', 1), ('magazines', 2), ('pamphlets', 3), ('decried', 1), ('vadym', 1), ('chuprun', 1), ('preach', 1), ('namik', 1), ('infuriated', 1), ('bumper', 2), ('abdulla', 1), ('shahin', 1), ('pegs', 1), ('pagonis', 1), ('habila', 1), ('bluefields', 1), ('steelers', 1), ('chargers', 1), ('falcons', 2), ('rams', 2), ('seahawks', 1), ('invalidate', 2), ('kubis', 2), ('tarija', 1), ('308', 1), ('afari', 1), ('djan', 1), ('tain', 2), ('brong', 1), ('ahafo', 1), ('npp', 1), ('duping', 1), ('marinellis', 2), ('fleeced', 1), ('ziauddin', 1), ('gilgit', 1), ('conoco', 1), ('gela', 1), ('bezhuashvili', 1), ('abiding', 1), ('rebuffing', 1), ('ghost', 3), ('602', 1), ('universally', 1), ('soundly', 2), ('originates', 1), ('latgalians', 1), ('panabaj', 1), ('moviegoers', 1), ('irate', 1), ('propriety', 1), ('bhagwagar', 1), ('sensationalizing', 1), ('revalue', 1), ('churn', 1), ('345', 1), ('anatol', 1), ('liabedzka', 1), ('krasovsky', 1), ('1776', 1), ('confederacy', 1), ('buoyed', 1), ('desolate', 1), ('indisputably', 1), ('1614', 1), ('trappers', 1), ('beerenberg', 1), ('winters', 1), ('victorian', 1), ('awkward', 1), ('bengalis', 2), ('wajed', 1), ('harangued', 1), ('nineva', 1), ('pushpa', 1), ('dahal', 1), ('dasain', 1), ('predetermined', 1), ('stunned', 1), ('reverberated', 1), ('downfall', 1), ('prabtibha', 1), ('patil', 1), ('fallujans', 1), ('swazis', 1), ('mswati', 3), ('grudgingly', 1), ('backslid', 1), ('mattel', 4), ('gagging', 1), ('cesme', 1), ('ksusadasi', 1), ('bassel', 1), ('fleihan', 4), ('sanitary', 1), ('typhoid', 1), ('aguilar', 4), ('zinser', 4), ('morelos', 1), ('disdain', 1), ('lugansville', 1), ('espiritu', 1), ('bankroll', 1), ('fundraiser', 2), ('1845', 1), ('chandpur', 1), ('apologies', 1), ('giorgio', 1), ('exhort', 1), ('darwin', 1), ('resurrect', 1), ('adana', 1), ('disparage', 1), ('enraged', 1), ('idolatry', 1), ('goatherd', 2), ('eventide', 1), ('pulikovsky', 2), ('lively', 2), ('selective', 1), ('snowed', 1), ('herd', 2), ('strangers', 1), ('abundantly', 1), ('qubad', 1), ('sonata', 2), ('follower', 2), ('ingratitude', 1), ('restriction', 1), ('hindrance', 1), ('iwc', 1), ('hunts', 1), ('puteh', 2), ('nahrawan', 1), ('becher', 1), ("sa'eed", 1), ('comptroller', 1), ('feeble', 1), ('compassionately', 1), ('advertisers', 1), ('nursery', 2), ('abayi', 1), ('abia', 2), ('kourou', 1), ('moans', 1), ('tonic', 1), ('nutrient', 1), ('helios', 1), ('optical', 1), ('parasol', 1), ('halts', 1), ('ge', 2), ('hints', 2), ('mistrust', 1), ('fixation', 1), ('reminder', 1), ('tunisian', 2), ('hedi', 1), ('yousseff', 1), ('boudhiba', 4), ('planners', 1), ('mentally', 2), ('trench', 5), ('fanned', 1), ('andreu', 1), ('hearse', 1), ('volodymyr', 2), ('lytvyn', 1), ('ulrich', 1), ('wilhelm', 1), ('juventud', 1), ('rebelde', 1), ('conjwayo', 1), ('tiempo', 1), ('mauricio', 1), ('zapata', 1), ('arauca', 4), ('bernal', 2), ('sununu', 1), ('tripura', 5), ('dangabari', 1), ('svalbard', 1), ('wainganga', 1), ('jens', 1), ('stoltenberg', 1), ('environmentalist', 1), ('wangari', 1), ('maathai', 1), ('capsize', 1), ('bhandara', 1), ('925', 1), ('janan', 1), ('souray', 1), ('gloom', 1), ('lashkargah', 1), ('tables', 1), ('pham', 1), ('thrive', 1), ('painstakingly', 1), ('anchor', 1), ('ulsan', 1), ('sopranos', 2), ('mobster', 1), ('soprano', 3), ('hangout', 1), ('satriale', 1), ('manny', 1), ('costeira', 3), ('condominum', 1), ('serial', 1), ('authentication', 1), ('demolishing', 1), ('pals', 1), ('episode', 1), ('reprocess', 2), ('gref', 1), ('pai', 1), ('f15', 1), ('dohuk', 1), ('okay', 1), ('insurers', 1), ('caters', 1), ('caymanians', 1), ('czechs', 4), ('preoccupied', 1), ('sudeten', 1), ('ruthenians', 1), ('reich', 1), ('truncated', 1), ('ruthenia', 1), ('velvet', 2), ('siam', 1), ('chinnawat', 1), ('shahdi', 1), ('mohanna', 1), ('wetchachiwa', 1), ('udd', 2), ('confiscating', 1), ('cleavages', 1), ('politic', 1), ('marginal', 1), ('underinvestment', 1), ('vitality', 1), ('dombrovskis', 1), ('meekness', 1), ('gentleness', 1), ('temper', 1), ('altogether', 1), ('boldness', 1), ('bridle', 1), ('dread', 1), ('valynkin', 1), ('mainichi', 1), ('amelia', 1), ('rowers', 3), ('falash', 2), ('mura', 2), ('rabbis', 4), ('renting', 1), ('lehava', 1), ('rentals', 1), ('lifestyles', 1), ('muttur', 1), ('hanssen', 1), ('indescribable', 1), ('horrors', 1), ('miracles', 1), ('e2', 1), ('pingtung', 1), ('azizuddin', 3), ('flattening', 1), ('shanties', 1), ('browne', 1), ('inductee', 1), ('songwriters', 2), ('masser', 1), ('burgie', 1), ('bobby', 1), ('teddy', 1), ('randazzo', 1), ('dylan', 1), ('nominating', 2), ('catalog', 1), ('induction', 1), ('abac', 7), ('vindicates', 1), ('septum', 1), ('cerebral', 1), ('balart', 2), ('piling', 1), ('orenburg', 1), ('beiring', 4), ('2017', 1), ('polo', 1), ('rugby', 1), ('roller', 1), ('equestrian', 1), ('equine', 1), ('hallams', 1), ('muammar', 1), ('divas', 1), ('antwerp', 2), ('potts', 1), ('angelina', 1), ('jolie', 5), ('pitt', 3), ('naankuse', 1), ('dedication', 1), ('baboons', 1), ('leopards', 1), ('dalia', 1), ('itzik', 1), ('diouf', 3), ('seasonally', 1), ('tropics', 1), ('climates', 1), ('modifying', 1), ('acidity', 1), ('salinity', 1), ('henman', 2), ('idaho', 1), ('taepodong', 1), ('geoscience', 1), ('spewing', 1), ('plume', 1), ('gulfport', 1), ('sancha', 1), ('waterworks', 1), ('changqi', 1), ('jubilant', 1), ('neve', 1), ('dekalim', 1), ('commissioning', 1), ('mo', 1), ('mowlam', 5), ('layered', 1), ('hospice', 1), ('radiotherapy', 1), ('shelve', 1), ('iskan', 1), ('harithiya', 1), ('brazen', 1), ('mashhad', 2), ('nourollah', 1), ('niaraki', 1), ('dousing', 1), ('fuselage', 1), ('tupolev', 1), ('airtour', 1), ('silos', 2), ('logjams', 1), ('ballenas', 1), ('implicate', 2), ('defrauding', 1), ('congressmen', 2), ('psychologically', 1), ('unfurling', 1), ('ayham', 1), ('sammarei', 2), ('mujaheddin', 1), ('distinction', 1), ('radhika', 1), ('coomaraswamy', 1), ('westminster', 2), ('cormac', 1), ('delmas', 1), ('challengerspace', 1), ('minuteman', 3), ('donovan', 1), ('watan', 1), ('blackburn', 1), ('basically', 1), ('sneaking', 1), ('torkham', 2), ('burqa', 1), ('yoshinori', 1), ('ono', 1), ('yvelines', 1), ('hauts', 1), ('seine', 2), ("d'", 1), ('oise', 1), ('zheijiang', 1), ('forklift', 2), ('inscriptions', 1), ('phoenixes', 1), ('etched', 1), ('porcelain', 1), ('mirrors', 3), ('avril', 2), ('lavigne', 2), ('derek', 1), ('whibley', 1), ('bedrooms', 1), ('sauna', 1), ('disarms', 1), ('noranit', 1), ('setabutr', 1), ('amass', 1), ('sigou', 1), ('solidaria', 1), ('salvadorans', 1), ('vulgar', 1), ('gniljane', 1), ('selim', 1), ('krasniqi', 2), ('transocean', 1), ('trident', 1), ('channeling', 1), ('indus', 1), ('fused', 1), ('aryan', 1), ('scythians', 1), ('satisfactorily', 1), ('marginalization', 1), ('rocky', 1), ('brightens', 1), ('microchips', 1), ('nicaraguans', 2), ('idiot', 1), ('drunkard', 1), ('clinging', 1), ('chinchilla', 1), ('mongols', 3), ('chinggis', 1), ('khaan', 1), ('eurasian', 1), ('steppe', 1), ('mongolians', 1), ('mprp', 4), ('mpp', 2), ('waemu', 1), ('disbursement', 2), ('bakers', 1), ('concealment', 1), ('nibble', 1), ('tendrils', 1), ('rustling', 1), ('arrow', 1), ('maltreated', 1), ('partridge', 2), ('earnestly', 2), ('partridges', 1), ('recompense', 1), ('scruple', 1), ('hen', 3), ('pondered', 1), ('matched', 1), ('425', 1), ('touches', 1), ('closings', 1), ('mohaqiq', 2), ('nasab', 1), ('haqooq', 1), ('zan', 1), ('vujadin', 1), ('popovic', 1), ('milutinovic', 1), ('sainovic', 1), ('dragoljub', 1), ('ojdanic', 1), ('lazarevic', 2), ('moses', 1), ('bittok', 3), ('lottery', 1), ('refinances', 1), ('cyanide', 1), ('cashed', 1), ('echeverria', 4), ('tavis', 1), ('smiley', 1), ('imagine', 1), ('evading', 1), ('conscription', 1), ('adi', 2), ('abeto', 2), ('asmara', 1), ('qadir', 1), ('sinak', 1), ('muadham', 1), ('brillantes', 1), ('kilju', 1), ('cabin', 1), ('spinetta', 1), ('cricketer', 1), ('mauro', 1), ('vechchio', 1), ('sharks', 1), ('stalking', 1), ('crittercam', 1), ('saola', 1), ('hachijo', 1), ('habibur', 1), ('gaddafi', 1), ('thilan', 1), ('samaraweera', 1), ('tharanga', 1), ('paranavithana', 1), ('purposely', 2), ('misleading', 1), ('culprits', 2), ('devise', 1), ('sisli', 1), ('netting', 2), ('compounding', 1), ('agence', 1), ('presse', 1), ('jaime', 2), ('razuri', 6), ('riskiest', 1), ('womb', 1), ('veterinarians', 1), ('farhatullah', 1), ('kypriano', 1), ('kristin', 1), ('halvorsen', 1), ('crave', 1), ('leong', 1), ('lazy', 1), ('mandera', 1), ('prep', 1), ('skelleftea', 1), ('carli', 1), ('lloyd', 2), ('dribbled', 1), ('footed', 1), ('ricocheted', 1), ('pia', 1), ('sundhage', 1), ('cups', 1), ('olmo', 2), ('severity', 1), ('kazemeini', 1), ('disapora', 1), ('speeds', 1), ('parallels', 1), ('lagunas', 1), ('chacahua', 1), ('lazaro', 2), ('cabo', 1), ('corrientes', 1), ('guatemalans', 1), ('tabasco', 1), ('chanet', 2), ('mengal', 1), ('salem', 1), ('ushakov', 2), ('respectfully', 1), ('355', 1), ('overtake', 1), ('latvians', 1), ('erase', 1), ('clinch', 1), ('certificates', 4), ('kyat', 1), ('siddiq', 2), ('ronco', 1), ('doled', 1), ('highness', 1), ('surkhanpha', 2), ('kiriyenko', 2), ('megawatts', 2), ('royals', 1), ('scimitar', 1), ('graduating', 1), ('sandhurst', 1), ('accompany', 1), ('isna', 1), ('zolfaghari', 2), ('nie', 1), ('frailty', 1), ('decriminalizing', 1), ('sweltering', 1), ('tursunov', 1), ('edgardo', 1), ('massa', 1), ('gremelmayr', 1), ('bjorn', 1), ('phau', 1), ('teesta', 1), ('ecology', 1), ('ganges', 1), ('damadola', 1), ('scold', 1), ('cuzco', 1), ('reckon', 1), ('incwala', 1), ('swazi', 1), ('nhlanhla', 1), ('nhlabatsi', 1), ('mbabane', 1), ('hms', 1), ('seawater', 1), ('yunus', 1), ('qanuni', 4), ('tadjoura', 1), ('loudspeakers', 1), ('ophir', 1), ('pines', 1), ('ney', 3), ('relinquish', 1), ('rosemond', 1), ('pradel', 1), ('certainty', 2), ('kostiw', 2), ('indiscretion', 1), ('distraction', 2), ('shoplifting', 1), ('tetanus', 2), ('husseindoust', 1), ('destablize', 1), ('dabous', 2), ('yanbu', 2), ('howlwadaag', 2), ('streamlined', 1), ('weiner', 1), ('lobbyists', 1), ('tenzin', 1), ('taklha', 1), ('attribute', 1), ('vials', 1), ('profiting', 1), ('ciampino', 1), ('martha', 1), ('karua', 2), ('goldenberg', 1), ('murungaru', 2), ('kendrick', 1), ('meek', 2), ('cong', 1), ('superhighway', 1), ('shady', 1), ('loitering', 1), ('johanns', 3), ('boyle', 1), ('1860s', 1), ('sughd', 1), ('ssr', 2), ('deluge', 1), ('khujand', 1), ('rasht', 1), ('sedimentary', 1), ('basins', 1), ('garments', 1), ('overflowing', 1), ('damsel', 1), ('reclining', 1), ('forgetting', 1), ('nurture', 1), ('truss', 2), ('curious', 1), ('sandbags', 1), ('learnt', 1), ('escapes', 1), ('chevy', 1), ('gallegos', 1), ('sheldon', 1), ('quail', 3), ('katharine', 2), ('shotgun', 4), ('pellets', 2), ('avid', 2), ('232', 1), ('honesty', 1), ('728', 1), ('arnoldo', 1), ('aleman', 1), ('discredited', 1), ('sandanista', 1), ('54th', 1), ('dikes', 1), ('vicinity', 1), ('flashpoints', 1), ('osnabrueck', 1), ('montiglio', 2), ('processor', 1), ('westland', 1), ('hallmark', 1), ('slaughterhouse', 2), ('usda', 1), ('vani', 1), ('contreras', 1), ('averting', 1), ('jeopardizes', 1), ('34th', 1), ('socially', 1), ('hatim', 1), ('1657', 1), ('basset', 1), ('megrahi', 1), ('mans', 1), ('saydiyah', 1), ('neighbourhood', 1), ('lightened', 1), ('lateral', 1), ('limped', 1), ('2100', 1), ('edt', 1), ('doubly', 1), ('aoun', 1), ('sulieman', 1), ('dushevina', 2), ('ivana', 1), ('lisjak', 1), ('gaz', 1), ('byes', 1), ('virginie', 1), ('razzano', 2), ('kveta', 1), ('peschke', 1), ('tsvetana', 1), ('pironkova', 1), ('rae', 1), ('bareli', 1), ('70th', 1), ('rajiv', 1), ('tarmac', 2), ('safeguarding', 1), ('refurbished', 1), ('bike', 1), ('walkways', 1), ('sorted', 1), ('crawl', 1), ('unidentifiied', 1), ('gruesome', 1), ("'n", 1), ('caliber', 1), ('chairperson', 1), ('jaoko', 1), ('recounting', 1), ('episodes', 1), ('pheasants', 1), ('townships', 1), ('reinvigorate', 1), ('doi', 1), ('talaeng', 1), ('quadriplegic', 1), ('clips', 1), ('streaming', 1), ('outward', 1), ('sunspots', 1), ('disembark', 2), ('489', 1), ('forgotten', 1), ('jaber', 1), ('wafa', 1), ('saboor', 1), ('hadhar', 1), ('leatherback', 1), ('fascinate', 1), ('roams', 1), ('nesting', 1), ('leatherbacks', 1), ('phrases', 3), ('tubigan', 1), ('joko', 1), ('suyono', 1), ('intensifies', 1), ('apostates', 1), ('qais', 1), ('shameri', 1), ('sabotages', 1), ('rekindle', 1), ('lawfully', 1), ('empt', 1), ('seche', 1), ('otari', 1), ('unleash', 1), ('miscalculated', 1), ('zap', 1), ('ararat', 1), ('mazlum', 1), ('mattei', 1), ('nuimei', 1), ('listened', 1), ('\x97', 1), ('monuc', 1), ('anyplace', 1), ('catapulted', 1), ('showcased', 1), ('madhoun', 1), ('leveling', 1), ('kumtor', 1), ('cis', 1), ('recapitalization', 1), ('cycles', 1), ('grytviken', 2), ('shackleton', 1), ('fated', 1), ('nm', 2), ('aviary', 1), ('nightingale', 3), ('wont', 1), ('peacock', 1), ('bajura', 1), ('accham', 1), ('kohistani', 1), ('ahadi', 1), ('locusts', 1), ('zinder', 1), ('caste', 2), ('castes', 1), ('fortis', 1), ('stella', 1), ('artois', 1), ('radek', 1), ('stepanek', 2), ('johansson', 1), ('tulkarm', 1), ('maclang', 1), ('batad', 1), ('banaue', 1), ('ifugao', 1), ('legaspi', 1), ('starmagazine', 1), ('distorts', 1), ('muasher', 1), ('safehaven', 1), ('wachira', 1), ('waruru', 1), ('73rd', 1), ('holodomor', 1), ('kiwis', 1), ('scorers', 1), ('201', 1), ('ashraful', 1), ('napier', 1), ('kindhearts', 3), ('detective', 1), ('lilienfeld', 2), ('colt', 1), ('cobra', 1), ('revolver', 1), ('definitively', 1), ('holster', 1), ('aussie', 1), ('flyway', 1), ('yuanshi', 1), ('xianliang', 1), ('sprees', 1), ('gedo', 1), ('udf', 2), ('bakili', 1), ('muluzi', 1), ('365', 1), ('yuvraj', 1), ('araft', 1), ('goodbye', 1), ('saca', 1), ('shaowu', 1), ('chairmen', 1), ('mumps', 1), ('rubella', 1), ('autism', 1), ('upali', 1), ('ealier', 1), ('affiliations', 1), ('hypocrisy', 1), ('buechel', 4), ('gardena', 1), ('guay', 1), ('442', 1), ('420', 1), ('aksel', 1), ('svindal', 1), ('417', 1), ('configured', 1), ('nahum', 1), ('repeating', 1), ('costello', 2), ('aleksandar', 2), ('pera', 1), ('petrasevic', 1), ('vukov', 1), ('csatia', 1), ('metullah', 1), ('teeple', 1), ('unspent', 1), ('helmund', 1), ('hushiar', 1), ('facilitates', 1), ('loveland', 1), ('butchers', 1), ('unionize', 1), ('yevpatoria', 2), ('acetylene', 1), ('basement', 1), ('monsoons', 1), ('rhee', 3), ('bong', 1), ('rumbling', 2), ('saqlawiyah', 1), ('rappers', 2), ('nampo', 1), ('samad', 1), ('achakzai', 2), ('kojak', 1), ('sulaiman', 1), ('pashtoonkhwa', 1), ('milli', 1), ('cholily', 1), ('221', 1), ('chakul', 1), ('consent', 1), ('abune', 1), ('sbs', 3), ('squares', 1), ('sicko', 1), ('humaneness', 1), ('harshest', 1), ('wealthier', 1), ('heeds', 1), ('https', 1), ('celebritiesforcharity', 1), ('raffles', 1), ('netraffle', 1), ('cfm', 1), ('airfare', 1), ('costumed', 1), ('urchins', 1), ('porch', 1), ('treaters', 1), ('gravelly', 1), ('beggars', 1), ('decorating', 1), ('halloween', 2), ('upshot', 1), ('dusk', 1), ('ghouls', 1), ('starlets', 1), ('distasteful', 1), ('nisr', 1), ('khantumani', 1), ('178', 1), ('moravia', 1), ('magyarization', 1), ('afars', 2), ('issas', 1), ('gouled', 1), ('aptidon', 1), ('guelleh', 1), ('socializing', 1), ('terrified', 1), ('slew', 1), ('pounce', 1), ('remedy', 1), ('doosnoswair', 1), ('oliveira', 1), ('567', 1), ('flavors', 2), ('onion', 1), ('chili', 1), ('eggplant', 1), ('smoked', 1), ('trout', 1), ('spaghetti', 1), ('parmesan', 1), ('spinach', 1), ('avocado', 1), ('lipstick', 2), ('prints', 3), ('custodian', 1), ('squeegee', 1), ('educators', 1), ('believer', 1), ('séances', 1), ('ear', 2), ('merriment', 1), ('fooling', 1), ('gullible', 1), ('poke', 1), ('mansions', 1), ('scenic', 1), ('314', 1), ('inoperable', 3), ('amharic', 1), ('murtha', 3), ('stinging', 1), ('misled', 1), ('rotfeld', 1), ('surrenders', 1), ('onal', 1), ('ulus', 1), ('cabello', 1), ('youssifiyeh', 1), ('kamdesh', 1), ('abbreviations', 1), ('translations', 1), ('transplanting', 1), ('casualities', 1), ('rawhi', 1), ('fattouh', 1), ('corners', 1), ('407', 1), ('francs', 1), ('abetting', 2), ('jokic', 1), ('murghab', 1), ('cellist', 2), ('conductor', 1), ('mstislav', 1), ('rostropovich', 3), ('constrain', 2), ('moderating', 1), ('39th', 1), ('adrien', 1), ('houngbedji', 1), ('vishnevskaya', 1), ('laughable', 1), ('laiyan', 1), ('jiaotong', 1), ('tp', 1), ('rovnag', 1), ('abdullayev', 1), ('filya', 1), ('implications', 1), ('nabucco', 1), ('bypassing', 1), ('colchester', 1), ('shabat', 1), ('jabbar', 1), ('jeremy', 1), ('hobbs', 1), ('khasavyurt', 1), ('longyan', 1), ('shawal', 2), ('mensehra', 1), ('dialed', 1), ('gibb', 1), ('guadalupe', 1), ('escamilla', 1), ('mota', 1), ('syvkovych', 2), ('rajapakshe', 1), ('kentung', 1), ('leonella', 1), ('sgorbati', 1), ('disable', 1), ('amerzeb', 1), ('beats', 1), ('slinging', 1), ('irresistable', 1), ('shrek', 1), ('grosses', 1), ('bourj', 1), ('abi', 1), ('haidar', 1), ('puccio', 1), ('vince', 1), ('spadea', 1), ('taik', 1), ('melli', 1), ('proliferator', 1), ('agartala', 1), ('sébastien', 1), ('monte', 3), ('citroën', 1), ('xsara', 1), ('wrc', 1), ('duval', 1), ('grönholm', 1), ('auriol', 1), ('submachine', 1), ('eminem', 2), ('mathers', 2), ('estranged', 1), ('hailie', 1), ('remarried', 1), ('recouped', 1), ('continents', 1), ('waldner', 1), ('tycze', 1), ('substantiated', 1), ('establishments', 1), ('ventilation', 1), ('passive', 1), ('petropavlosk', 1), ('kamchatskii', 1), ('fedora', 1), ('adventurer', 1), ('spielberg', 1), ('paramount', 1), ('indy', 1), ('mangos', 1), ('avocados', 1), ('1634', 1), ('bonaire', 1), ('isla', 1), ('refineria', 1), ('lied', 1), ('burner', 1), ('fuller', 2), ('housekeeping', 1), ('whiten', 1), ('blacken', 1), ('heifer', 3), ('harnessed', 1), ('tormented', 1), ('yoke', 1), ('smile', 1), ('idleness', 1), ('grasshoppers', 2), ('lighten', 1), ('labors', 1), ('hive', 1), ('tasted', 1), ('honeycomb', 1), ('brute', 1), ('brayed', 1), ('cudgelling', 1), ('fright', 1), ('irrationality', 1), ('lakeside', 1), ('vijay', 1), ('nambiar', 2), ('silly', 1), ('equations', 2), ('approximation', 2), ('clapping', 1), ('luay', 1), ('zaid', 1), ('sander', 1), ('maddalena', 1), ('sardinian', 1), ('humberto', 1), ('valbuena', 1), ('caqueta', 1), ('gospel', 2), ('pilgrim', 2), ('1890', 1), ('dorsey', 1), ('mahalia', 1), ('13010', 1), ('druze', 2), ('majdal', 1), ('matara', 1), ('muhsin', 1), ('matwali', 1), ('atwah', 1), ('intoxicating', 1), ('maysoon', 1), ('damaluji', 1), ('massum', 1), ('homeowner', 1), ('micky', 1), ('rosenfeld', 1), ('talansky', 1), ('congratulations', 1), ('barka', 1), ('tokar', 2), ('fay', 2), ('kousalyan', 1), ('shipyards', 1), ('disillusionment', 1), ('pictured', 1), ('kira', 1), ('plastinina', 1), ('mikhailova', 1), ('emma', 1), ('sarrata', 1), ('chronicle', 1), ('jamahiriyah', 1), ('pariah', 1), ("l'aquilla", 2), ('walled', 1), ('sevilla', 1), ('middlesbrough', 2), ('maresca', 1), ('78th', 1), ('84th', 1), ('fabiano', 1), ('frédéric', 1), ('kanouté', 1), ('collemaggio', 1), ('tarsyuk', 1), ('adriatic', 1), ('slovenians', 1), ('dagger', 1), ('stashes', 1), ('unleashing', 1), ('worm', 3), ('essebar', 2), ('atilla', 1), ('ekici', 2), ('onna', 1), ('shkin', 1), ('abruzzo', 1), ('cruelty', 1), ('amicable', 1), ('remnant', 1), ('fades', 1), ('animist', 1), ('gil', 1), ('hoffman', 1), ('helmut', 1), ('kohl', 2), ('reunifying', 1), ('238', 1), ('verbeke', 1), ('intercepts', 1), ('annul', 2), ('symbolize', 1), ('ishaq', 1), ('alako', 1), ('rahmatullah', 1), ('nazari', 1), ('robinsons', 1), ('quentin', 1), ('tarantino', 1), ('grindhouse', 1), ('debuted', 1), ('tracker', 1), ('dergarabedian', 1), ('takhar', 1), ('icelanders', 1), ('bootleg', 1), ('reykjavik', 1), ('marginalize', 1)])
47959
{'the': 1, 'in': 2, 'of': 3, 'to': 4, 'a': 5, 'and': 6, "'s": 7, 'for': 8, 'has': 9, 'on': 10, 'is': 11, 'that': 12, 'have': 13, 'u': 14, 'with': 15, 'said': 16, 'was': 17, 'at': 18, 'says': 19, 's': 20, 'from': 21, 'by': 22, 'he': 23, 'an': 24, 'as': 25, 'say': 26, 'it': 27, 'are': 28, 'were': 29, 'his': 30, 'president': 31, 'will': 32, 'officials': 33, 'government': 34, 'mr': 35, 'two': 36, 'been': 37, 'killed': 38, 'people': 39, 'after': 40, 'not': 41, 'its': 42, 'be': 43, 'but': 44, 'they': 45, 'more': 46, 'also': 47, 'year': 48, 'new': 49, 'united': 50, 'military': 51, 'last': 52, 'who': 53, 'country': 54, 'than': 55, 'minister': 56, 'police': 57, 'one': 58, 'their': 59, 'iraq': 60, 'which': 61, 'security': 62, 'this': 63, 'about': 64, 'other': 65, 'states': 66, 'had': 67, 'least': 68, 'state': 69, 'three': 70, 'tuesday': 71, 'week': 72, 'since': 73, 'world': 74, 'forces': 75, 'thursday': 76, 'group': 77, 'iran': 78, 'over': 79, 'friday': 80, 'monday': 81, 'wednesday': 82, 'against': 83, 'during': 84, 'when': 85, 'al': 86, 'sunday': 87, '000': 88, 'troops': 89, 'oil': 90, 'authorities': 91, 'would': 92, 'into': 93, 'iraqi': 94, 'saturday': 95, 'first': 96, 'month': 97, 'prime': 98, 'foreign': 99, 'city': 100, 'some': 101, 'up': 102, 'nuclear': 103, 'out': 104, 'international': 105, 'attacks': 106, 'militants': 107, 'palestinian': 108, 'between': 109, 'israeli': 110, 'afghanistan': 111, 'nations': 112, 'china': 113, 'south': 114, 'called': 115, 'years': 116, 'former': 117, 'no': 118, 'talks': 119, 'attack': 120, 'war': 121, 'bush': 122, 'israel': 123, 'province': 124, 'day': 125, 'or': 126, 'million': 127, 'several': 128, 'near': 129, 'north': 130, 'n': 131, 'leader': 132, 'pakistan': 133, 'party': 134, 'southern': 135, 'region': 136, 'four': 137, 'afghan': 138, 'earlier': 139, 'there': 140, 'countries': 141, 'including': 142, 'agency': 143, 'report': 144, 'all': 145, 'could': 146, 'saying': 147, 'soldiers': 148, 'elections': 149, 'news': 150, 'baghdad': 151, 'violence': 152, 'national': 153, 'under': 154, '1': 155, 'official': 156, 'economic': 157, 'european': 158, 'reports': 159, 'political': 160, 'election': 161, 'general': 162, 'spokesman': 163, 'capital': 164, 'american': 165, 'court': 166, 'bomb': 167, 'before': 168, 'if': 169, 'statement': 170, 'leaders': 171, 'wounded': 172, 'five': 173, 'rebels': 174, 'made': 175, 'told': 176, 'percent': 177, 'him': 178, 'union': 179, 'where': 180, 'led': 181, 'members': 182, 'may': 183, 'british': 184, 'peace': 185, 'border': 186, 'most': 187, 'part': 188, 'her': 189, 'while': 190, 'others': 191, 'next': 192, 'because': 193, 'them': 194, 'rights': 195, 'human': 196, 'program': 197, 'russia': 198, 'many': 199, 'house': 200, 'expected': 201, 'died': 202, 'six': 203, 'meeting': 204, 'fighting': 205, 'held': 206, 'health': 207, 'economy': 208, 'opposition': 209, 'killing': 210, 'time': 211, 'flu': 212, 'taleban': 213, 'being': 214, 'another': 215, 'those': 216, 'late': 217, 'what': 218, 'northern': 219, 'off': 220, 'recent': 221, 'ministry': 222, 'gaza': 223, 'she': 224, '10': 225, 'russian': 226, 'washington': 227, 'high': 228, 'insurgents': 229, 'secretary': 230, 'power': 231, 'help': 232, 'down': 233, 'western': 234, 'suspected': 235, 'top': 236, 'released': 237, 'bird': 238, 'india': 239, 'days': 240, 'nato': 241, 'weapons': 242, 'old': 243, 'town': 244, 'through': 245, 'korea': 246, 'army': 247, 'later': 248, 'groups': 249, 'can': 250, 'any': 251, 'parliament': 252, 'vote': 253, 'death': 254, 'men': 255, 'nearly': 256, 'area': 257, 'announced': 258, 'coalition': 259, 'thousands': 260, 'defense': 261, 'accused': 262, 'pakistani': 263, 'aid': 264, 'reported': 265, 'only': 266, 'second': 267, 'early': 268, 'central': 269, '2': 270, 'west': 271, 'bank': 272, 'set': 273, 'qaida': 274, 'following': 275, 'number': 276, 'found': 277, 'council': 278, 'support': 279, 'arrested': 280, 'chief': 281, 'end': 282, 'chinese': 283, 'militant': 284, 'organization': 285, 'meanwhile': 286, 'african': 287, 'charges': 288, 'both': 289, 'plan': 290, 'visit': 291, 'took': 292, 'months': 293, 'began': 294, 'hamas': 295, 'major': 296, 'among': 297, 'force': 298, 'take': 299, 'agreement': 300, 'trade': 301, 'fire': 302, 'french': 303, 'such': 304, 'home': 305, 'energy': 306, 'efforts': 307, 'suicide': 308, 'company': 309, 'local': 310, 'islamic': 311, 'prices': 312, "'": 313, '20': 314, 'did': 315, 'man': 316, 'nation': 317, 'workers': 318, 'car': 319, 'eastern': 320, 'iranian': 321, 'long': 322, 'media': 323, 'presidential': 324, '5': 325, 'came': 326, 'now': 327, 'january': 328, 'based': 329, 'food': 330, 'still': 331, 'billion': 332, 'should': 333, 'place': 334, 'so': 335, 'east': 336, 'department': 337, '30': 338, 'plans': 339, 'public': 340, 'sudan': 341, 'muslim': 342, 'eu': 343, 'japan': 344, 'chavez': 345, 'hit': 346, 'venezuela': 347, 'democratic': 348, 'deal': 349, 'terrorist': 350, 'work': 351, 'anti': 352, 'used': 353, 'dead': 354, 'turkey': 355, '3': 356, 'until': 357, 'africa': 358, 'member': 359, 'control': 360, 'seven': 361, '15': 362, 'indian': 363, 'virus': 364, 'left': 365, 'run': 366, 'meet': 367, 'growth': 368, 'past': 369, 'well': 370, 'ago': 371, '2003': 372, 'march': 373, 'tehran': 374, 'blast': 375, 'weeks': 376, "shi'ite": 377, 'newspaper': 378, 'met': 379, 'known': 380, 'britain': 381, 'make': 382, 'office': 383, 'darfur': 384, 'detained': 385, 'reporters': 386, 'confirmed': 387, 'head': 388, 'global': 389, 'rebel': 390, 'france': 391, 'september': 392, 'outside': 393, 'burma': 394, 'third': 395, 'white': 396, 'across': 397, 'beijing': 398, 'eight': 399, 'civilians': 400, 'agreed': 401, 'gas': 402, 'december': 403, 'continue': 404, 'gunmen': 405, 'information': 406, 'just': 407, 'prison': 408, 'areas': 409, 'around': 410, 'release': 411, 'along': 412, 'scheduled': 413, 'island': 414, '11': 415, 'financial': 416, 'lebanon': 417, 'development': 418, 'children': 419, 'coast': 420, 'terrorism': 421, 'stop': 422, 'signed': 423, 'issued': 424, 'lawmakers': 425, '12': 426, 'hundreds': 427, 'largest': 428, 'syria': 429, 'administration': 430, 'suspects': 431, 'large': 432, 'taliban': 433, '50': 434, 'protests': 435, 'york': 436, 'television': 437, 'law': 438, 'independence': 439, 'kilometers': 440, 'must': 441, 'women': 442, 'july': 443, 'use': 444, 'separate': 445, 'free': 446, 'claimed': 447, 'trying': 448, 'back': 449, 'do': 450, 'without': 451, 'london': 452, 'germany': 453, '7': 454, 'constitution': 455, 'fired': 456, 'air': 457, 'intelligence': 458, 'europe': 459, 'operations': 460, 'however': 461, 'victims': 462, 'decision': 463, 'korean': 464, 'incident': 465, 'officers': 466, 'parliamentary': 467, 'egypt': 468, 'somalia': 469, 'include': 470, 'term': 471, 'discuss': 472, 'november': 473, 'center': 474, 'pro': 475, 'system': 476, 'conference': 477, 'main': 478, '8': 479, 'series': 480, 'aimed': 481, 'february': 482, 'republic': 483, 'return': 484, 'key': 485, 'hurricane': 486, 'operation': 487, '25': 488, 'responsibility': 489, 'shot': 490, 'post': 491, 'august': 492, 'then': 493, 'crisis': 494, 'commission': 495, 'ruling': 496, 'investigation': 497, 'small': 498, 'ahead': 499, '4': 500, 'despite': 501, 'civil': 502, 'possible': 503, 'june': 504, 'strip': 505, '2008': 506, 'service': 507, 'calls': 508, 'urged': 509, 'case': 510, 'soldier': 511, 'hold': 512, 'move': 513, 'mission': 514, 'team': 515, 'october': 516, 'alleged': 517, 'market': 518, 'deadly': 519, 'fuel': 520, 'taken': 521, 'won': 522, 'conflict': 523, 'today': 524, 'crimes': 525, 'drug': 526, 'abbas': 527, 'summit': 528, 'trial': 529, 'process': 530, 'obama': 531, 'venezuelan': 532, 'hospital': 533, 'bombings': 534, 'arab': 535, 'asia': 536, '2004': 537, 'final': 538, 'syrian': 539, 'how': 540, 'relations': 541, 'injured': 542, 'close': 543, 'base': 544, 'protest': 545, 'prisoners': 546, 'sanctions': 547, 'congress': 548, 'voa': 549, 'cuba': 550, 'much': 551, 'way': 552, 'roadside': 553, 'remain': 554, 'democracy': 555, 'each': 556, 'armed': 557, 'increase': 558, 'same': 559, 'mahmoud': 560, 'comes': 561, 'district': 562, '2001': 563, '40': 564, 'approved': 565, 'building': 566, 'latest': 567, 'senior': 568, 'water': 569, 'warned': 570, 'rice': 571, 'kurdish': 572, 'cases': 573, 'storm': 574, 'record': 575, 'press': 576, 'palestinians': 577, 'explosion': 578, 'effort': 579, 'face': 580, 'turkish': 581, 'campaign': 582, '00': 583, 'john': 584, '100': 585, 'companies': 586, 'majority': 587, 'working': 588, 'production': 589, 'colombia': 590, 'show': 591, 'denied': 592, 'carried': 593, 'recently': 594, 'demand': 595, 'indonesia': 596, 'money': 597, 'deaths': 598, 'ordered': 599, 'federal': 600, 'whether': 601, 'strike': 602, 'bombing': 603, 'hours': 604, 'japanese': 605, 'protesters': 606, 'already': 607, 'allow': 608, '2010': 609, 'lebanese': 610, 'caused': 611, 'half': 612, 'remains': 613, 'cut': 614, 'opened': 615, 'emergency': 616, 'calling': 617, 'attacked': 618, 'moscow': 619, 'results': 620, 'bomber': 621, 'h5n1': 622, 'failed': 623, 'does': 624, 'middle': 625, 'site': 626, '6': 627, 'clear': 628, 'sector': 629, 'ukraine': 630, 'corruption': 631, 'kidnapped': 632, 'committee': 633, 'rejected': 634, 'nine': 635, 'us': 636, 'saudi': 637, '2006': 638, 'sunni': 639, 'pope': 640, 'mexico': 641, '2009': 642, 'due': 643, 'issues': 644, '13': 645, 'sent': 646, 'station': 647, 'bill': 648, 'within': 649, 'expressed': 650, 'residents': 651, 'action': 652, 'witnesses': 653, 'give': 654, 'embassy': 655, 'arrest': 656, 'you': 657, 'ban': 658, 'relief': 659, 'german': 660, 'strain': 661, 'americans': 662, 'disease': 663, 'further': 664, 'policy': 665, 'kashmir': 666, 'i': 667, '14': 668, 'taking': 669, 'planned': 670, 'occurred': 671, 'carrying': 672, 'islands': 673, 'future': 674, 'seized': 675, 'ethnic': 676, 'ministers': 677, 'strong': 678, 'launched': 679, 'concerns': 680, 'family': 681, '18': 682, 'increased': 683, 'neighboring': 684, 'come': 685, 'fighters': 686, 'exports': 687, 'asked': 688, 'april': 689, 'making': 690, 'territory': 691, 'supporters': 692, 'vehicle': 693, '17': 694, 'saddam': 695, 'justice': 696, 'radio': 697, 'ended': 698, 'call': 699, 'criticized': 700, '9': 701, 'captured': 702, 'taiwan': 703, 'birds': 704, 'heavy': 705, 'movement': 706, 'civilian': 707, 'leading': 708, 'cabinet': 709, 'involved': 710, 'speaking': 711, 'role': 712, 'woman': 713, 'king': 714, 'homes': 715, 'cooperation': 716, 'vice': 717, 'camp': 718, '2007': 719, '2002': 720, 'independent': 721, 'situation': 722, 'charged': 723, 'go': 724, 'backed': 725, 'judge': 726, 'parties': 727, 'put': 728, 'begin': 729, 'trip': 730, 'nigeria': 731, 'candidate': 732, 'gdp': 733, 'haiti': 734, 'business': 735, 'ties': 736, 'open': 737, 'land': 738, 'commander': 739, 'space': 740, '200': 741, 'deputy': 742, 'spain': 743, 'became': 744, 'own': 745, 'regional': 746, 'ms': 747, 'poor': 748, 'far': 749, 'experts': 750, 'problems': 751, '2005': 752, 'outbreak': 753, 'egyptian': 754, '16': 755, 'labor': 756, 'uranium': 757, 'blamed': 758, 'non': 759, 'special': 760, 'went': 761, 'services': 762, 'comments': 763, 'believed': 764, 'forced': 765, 'ambassador': 766, 'lead': 767, 'aircraft': 768, 'per': 769, 'issue': 770, 'continued': 771, 'missing': 772, 'provide': 773, 'details': 774, 'joint': 775, 'america': 776, 'current': 777, 'activities': 778, 'exploded': 779, 'medical': 780, 'life': 781, 'negotiations': 782, 'detainees': 783, 'times': 784, 'missile': 785, 'price': 786, 'become': 787, 'senate': 788, 'get': 789, 'tribal': 790, 'order': 791, 'musharraf': 792, 'parts': 793, 'rule': 794, 'community': 795, 'industry': 796, 'fight': 797, 'threat': 798, 'muslims': 799, 'bodies': 800, 'want': 801, 'change': 802, 'century': 803, 'seeking': 804, 'illegal': 805, 'natural': 806, 'quotes': 807, 'terrorists': 808, 'reached': 809, 'received': 810, 'annan': 811, 'measures': 812, '60': 813, 'allegations': 814, 'arrived': 815, 'linked': 816, 'supplies': 817, 'address': 818, 'referendum': 819, 'supreme': 820, 'alliance': 821, 'investment': 822, 'gulf': 823, 'round': 824, 'form': 825, 'wants': 826, 'declared': 827, 're': 828, 'travel': 829, 'win': 830, 'village': 831, '500': 832, 'earthquake': 833, 'sharon': 834, 'decades': 835, 'ruled': 836, 'level': 837, 'officer': 838, 'asian': 839, 'journalists': 840, 'rocket': 841, 'again': 842, 'dollars': 843, 'threatened': 844, 'olympic': 845, 'right': 846, 'tsunami': 847, 'toward': 848, 'sides': 849, 'mostly': 850, 'ali': 851, 'exchange': 852, 'wanted': 853, 'private': 854, 'citizens': 855, 'activists': 856, 'religious': 857, 'published': 858, 'kandahar': 859, 'body': 860, 'withdrawal': 861, 'fled': 862, 'offensive': 863, 'interview': 864, 'immediately': 865, 'tried': 866, 'hugo': 867, 'abu': 868, 'seen': 869, 'dispute': 870, 'reforms': 871, 'low': 872, 'fell': 873, 'communist': 874, 'elected': 875, 'atomic': 876, 'explosives': 877, 'kabul': 878, 'similar': 879, 'build': 880, 'cuban': 881, 'concern': 882, 'toll': 883, 'terror': 884, 'construction': 885, 'fatah': 886, 'debt': 887, 'somali': 888, 'attempt': 889, 'karzai': 890, 'growing': 891, 'network': 892, 'few': 893, 'authority': 894, 'cities': 895, 'candidates': 896, 'interim': 897, 'interior': 898, 'seats': 899, 'refused': 900, 'sea': 901, 'continues': 902, 'almost': 903, 'bring': 904, 'sudanese': 905, 'holding': 906, 'using': 907, 'brazil': 908, 'need': 909, 'tax': 910, '80': 911, 'suspect': 912, 'broke': 913, 'crude': 914, 'announcement': 915, 'putin': 916, 'yet': 917, 'ousted': 918, 'mass': 919, 'leave': 920, 'pressure': 921, 'convoy': 922, 'response': 923, 'sri': 924, 'massive': 925, 'millions': 926, 'reform': 927, 'wounding': 928, 'congo': 929, 'passed': 930, 'like': 931, 'full': 932, 'warning': 933, 'lost': 934, 'rate': 935, 'port': 936, 'arms': 937, 'governor': 938, '26': 939, 'assembly': 940, 'added': 941, 'himself': 942, 'proposed': 943, 'prevent': 944, 'assistance': 945, 'person': 946, 'population': 947, 'currently': 948, 'clashes': 949, 'insurgent': 950, 'test': 951, 'spread': 952, 'likely': 953, 'cross': 954, 'ahmadinejad': 955, 'soon': 956, 'named': 957, 'total': 958, 'good': 959, 'australia': 960, 'katrina': 961, 'raid': 962, 'peaceful': 963, 'annual': 964, 'returned': 965, 'voted': 966, 'jewish': 967, 'allowed': 968, 'needed': 969, 'charge': 970, 'hezbollah': 971, 'policemen': 972, 'evidence': 973, 'legal': 974, '70': 975, 'hussein': 976, 'although': 977, '06': 978, 'share': 979, 'violent': 980, 'fraud': 981, 'conditions': 982, 'associated': 983, 'nepal': 984, 'yushchenko': 985, 'castro': 986, 'director': 987, 'progress': 988, 'casualties': 989, 'spanish': 990, 'de': 991, 'front': 992, 'italy': 993, 'probe': 994, 'school': 995, 'away': 996, 'demonstrators': 997, 'less': 998, 'spoke': 999, 'detention': 1000, 'analysts': 1001, 'assassination': 1002, 'paul': 1003, 'destroyed': 1004, 'prosecutors': 1005, 'road': 1006, 'patrol': 1007, 'elsewhere': 1008, 'pyongyang': 1009, 'activity': 1010, 'related': 1011, 'enough': 1012, 'spending': 1013, 'important': 1014, 'sales': 1015, 'diplomatic': 1016, 'believe': 1017, 'hour': 1018, 'even': 1019, 'given': 1020, 'blair': 1021, 'agriculture': 1022, 'keep': 1023, 'struck': 1024, 'tests': 1025, 'proposal': 1026, 'living': 1027, 'georgia': 1028, 'facilities': 1029, 'access': 1030, 'freed': 1031, 'estimated': 1032, 'governments': 1033, 'tens': 1034, 'resolution': 1035, 'bombs': 1036, 'iraqis': 1037, 'insurgency': 1038, 'constitutional': 1039, 'closed': 1040, 'video': 1041, 'xinhua': 1042, 'representatives': 1043, 'poll': 1044, 'tribunal': 1045, 'voters': 1046, 'night': 1047, 'reach': 1048, 'paris': 1049, 'turned': 1050, 'resume': 1051, 'dozens': 1052, 'offer': 1053, '21': 1054, 'start': 1055, 'senator': 1056, 'peacekeeping': 1057, 'tourism': 1058, 'social': 1059, 'custody': 1060, 'hopes': 1061, 'policies': 1062, 'board': 1063, 'weather': 1064, 'pay': 1065, 'thailand': 1066, 'develop': 1067, 'name': 1068, 'clinton': 1069, 'kill': 1070, 'promised': 1071, 'fourth': 1072, 'enrichment': 1073, 'southeast': 1074, 'diplomats': 1075, 'soviet': 1076, 'facility': 1077, 'borders': 1078, 'australian': 1079, 'fund': 1080, 'san': 1081, 'behind': 1082, '24': 1083, 'includes': 1084, 'planning': 1085, 'rose': 1086, 'interest': 1087, 'line': 1088, 'brought': 1089, 'speech': 1090, 'measure': 1091, 'peacekeepers': 1092, 'poultry': 1093, 'kenya': 1094, 'damage': 1095, '19': 1096, 'bus': 1097, 'cup': 1098, 'running': 1099, 'journalist': 1100, 'italian': 1101, 'gave': 1102, 'allegedly': 1103, 'mosque': 1104, 'highest': 1105, 'humanitarian': 1106, 'short': 1107, 'lower': 1108, 'responsible': 1109, 'games': 1110, 'visited': 1111, 'popular': 1112, 'higher': 1113, 'identified': 1114, '22': 1115, 'rival': 1116, 'investigating': 1117, '2000': 1118, 'agencies': 1119, 'involvement': 1120, 'discovered': 1121, 'sources': 1122, 'pledged': 1123, 'joined': 1124, 'claim': 1125, 'suspended': 1126, 'join': 1127, 'denies': 1128, 'hamid': 1129, 'condemned': 1130, 'coming': 1131, 'season': 1132, 'abducted': 1133, 'list': 1134, 'tensions': 1135, 'mohammed': 1136, 'ethiopia': 1137, 'indonesian': 1138, 'rising': 1139, 'leaving': 1140, 'faces': 1141, 'lives': 1142, 'aids': 1143, 'trading': 1144, 'provincial': 1145, 'jobs': 1146, 'shows': 1147, 'refugees': 1148, 'connection': 1149, 'budget': 1150, 'ceremony': 1151, 'cause': 1152, 'airport': 1153, 'developing': 1154, 'once': 1155, 'gathered': 1156, 'strikes': 1157, 'northwest': 1158, 'niger': 1159, 'helped': 1160, 'chairman': 1161, 'ii': 1162, 'secret': 1163, 'islamabad': 1164, 'launch': 1165, 'begun': 1166, 'staff': 1167, 'request': 1168, 'freedom': 1169, 'comment': 1170, 'ship': 1171, 'able': 1172, 'polls': 1173, 'imposed': 1174, 'helmand': 1175, 'step': 1176, 'often': 1177, 'previously': 1178, 'immediate': 1179, 'plant': 1180, 'ocean': 1181, 'victory': 1182, 'islam': 1183, 'education': 1184, '300': 1185, 'ready': 1186, 'showed': 1187, 'internet': 1188, 'plane': 1189, 'colombian': 1190, 'helicopter': 1191, 'appeared': 1192, 'convicted': 1193, 'combat': 1194, 'arabia': 1195, 'worldwide': 1196, 'try': 1197, 'demanding': 1198, 'families': 1199, 'islamist': 1200, 'technology': 1201, 'church': 1202, 'targeted': 1203, 'training': 1204, 'unemployment': 1205, 'dollar': 1206, 'poverty': 1207, 'every': 1208, 'anniversary': 1209, 'controversial': 1210, 'previous': 1211, 'rally': 1212, 'together': 1213, 'cost': 1214, 'never': 1215, 'claims': 1216, 'hard': 1217, 'condition': 1218, 'infected': 1219, 'mosul': 1220, 'criticism': 1221, 'serious': 1222, 'chad': 1223, 'domestic': 1224, 'nearby': 1225, '27': 1226, 'lack': 1227, 'included': 1228, 'better': 1229, 'bosnian': 1230, 'offered': 1231, 'river': 1232, 'owned': 1233, 'democrats': 1234, 'barrel': 1235, 'treatment': 1236, 'having': 1237, 'resources': 1238, 'export': 1239, 'missiles': 1240, 'approval': 1241, 'demands': 1242, 'mexican': 1243, 'hariri': 1244, 'tour': 1245, 'amid': 1246, 'daily': 1247, 'hong': 1248, 'electoral': 1249, 'focus': 1250, 'personnel': 1251, 'affairs': 1252, 'clash': 1253, 'separately': 1254, 'demonstrations': 1255, 'guilty': 1256, 'provided': 1257, 'match': 1258, 'young': 1259, 'canada': 1260, 'care': 1261, 'injuries': 1262, 'protect': 1263, 'burmese': 1264, '31': 1265, 'largely': 1266, 'entered': 1267, 'broadcast': 1268, 'northwestern': 1269, 'event': 1270, 'poland': 1271, 'dutch': 1272, 'kosovo': 1273, 'rescue': 1274, 'suffered': 1275, 'doctors': 1276, 'period': 1277, 'described': 1278, 'act': 1279, 'dropped': 1280, 'red': 1281, 'laden': 1282, 'followed': 1283, 'infrastructure': 1284, 'kofi': 1285, 'opening': 1286, 'bin': 1287, 'battle': 1288, 'murder': 1289, 'cia': 1290, 'quake': 1291, 'legislation': 1292, 'hope': 1293, 'delegation': 1294, 'abuse': 1295, 'crackdown': 1296, 'guards': 1297, 'guantanamo': 1298, 'republican': 1299, 'create': 1300, 'boost': 1301, 'mine': 1302, 'see': 1303, 'farm': 1304, 'too': 1305, 'project': 1306, 'remote': 1307, 'whose': 1308, 'sign': 1309, 'jerusalem': 1310, 'coup': 1311, 'find': 1312, 'hand': 1313, 'condoleezza': 1314, 'safety': 1315, 'holiday': 1316, 'traveling': 1317, 'kong': 1318, 'treaty': 1319, 'income': 1320, 'ukrainian': 1321, 'barack': 1322, 'attend': 1323, 'association': 1324, 'serb': 1325, 'cease': 1326, 'point': 1327, 'contact': 1328, 'au': 1329, 'christian': 1330, 'voting': 1331, 'declined': 1332, 'controlled': 1333, 'hostages': 1334, 'decade': 1335, 'vehicles': 1336, 'scientists': 1337, 'common': 1338, 'ethiopian': 1339, 'electricity': 1340, 'reduce': 1341, '23': 1342, 'southeastern': 1343, 'very': 1344, 'hurt': 1345, 'beirut': 1346, 'significant': 1347, '90': 1348, 'sentenced': 1349, 'fox': 1350, 'quoted': 1351, 'criminal': 1352, 'cairo': 1353, 'ending': 1354, 'targets': 1355, 'mogadishu': 1356, 'goods': 1357, 'little': 1358, 'programs': 1359, 'date': 1360, 'zimbabwe': 1361, 'california': 1362, 'displaced': 1363, 'mid': 1364, 'helping': 1365, 'agricultural': 1366, 'critics': 1367, 'allies': 1368, 'spokeswoman': 1369, 'provinces': 1370, 'demanded': 1371, 'raids': 1372, 'raised': 1373, 'funds': 1374, 'inside': 1375, 'supply': 1376, 'orleans': 1377, 'morning': 1378, 'concerned': 1379, 'jordan': 1380, 'robert': 1381, 'heavily': 1382, 'texas': 1383, 'appeal': 1384, 'projects': 1385, 'unit': 1386, 'disputed': 1387, 'seek': 1388, 'rates': 1389, 'lanka': 1390, 'send': 1391, 'great': 1392, 'vietnam': 1393, 'refugee': 1394, 'markets': 1395, 'crash': 1396, 'viktor': 1397, 'affected': 1398, 'buildings': 1399, 'tony': 1400, 'rise': 1401, 'needs': 1402, 'improve': 1403, 'rockets': 1404, 'facing': 1405, 'separatist': 1406, 'shooting': 1407, 'stability': 1408, 'envoy': 1409, 'additional': 1410, 'withdraw': 1411, 'train': 1412, 'search': 1413, 'message': 1414, 'letter': 1415, 'these': 1416, 'banned': 1417, 'membership': 1418, 'crew': 1419, 'presence': 1420, 'urging': 1421, 'review': 1422, '1995': 1423, 'upon': 1424, '0': 1425, 'watch': 1426, 'disaster': 1427, 'vladimir': 1428, 'bombers': 1429, 'settlement': 1430, 'changes': 1431, 'militia': 1432, 'served': 1433, '28': 1434, 'votes': 1435, 'mainly': 1436, 'fall': 1437, 'regime': 1438, 'tokyo': 1439, 'stepped': 1440, 'holy': 1441, 'raise': 1442, 'el': 1443, 'genocide': 1444, 'decided': 1445, 'worst': 1446, 'crossing': 1447, 'quarter': 1448, 'streets': 1449, 'live': 1450, 'regions': 1451, 'study': 1452, 'consumer': 1453, 'amnesty': 1454, 'organizations': 1455, 'paid': 1456, 'fishing': 1457, 'offices': 1458, 'students': 1459, 'permanent': 1460, 'son': 1461, 'remarks': 1462, 'links': 1463, 'started': 1464, 'range': 1465, 'replace': 1466, 'history': 1467, 'firm': 1468, 'formally': 1469, 'sparked': 1470, 'stations': 1471, 'vatican': 1472, 'products': 1473, 'resolve': 1474, 'might': 1475, 'rains': 1476, 'black': 1477, 'killings': 1478, 'shortly': 1479, 'invasion': 1480, 'delhi': 1481, 'limited': 1482, 'pacific': 1483, 'session': 1484, 'repeatedly': 1485, 'result': 1486, 'play': 1487, 'presidency': 1488, '1991': 1489, 'street': 1490, 'dismissed': 1491, 'delay': 1492, 'cleric': 1493, 'positive': 1494, 'laws': 1495, 'continuing': 1496, 'truck': 1497, 'prosecutor': 1498, 'tournament': 1499, 'considered': 1500, 'olmert': 1501, 'football': 1502, 'brother': 1503, 'stock': 1504, 'carry': 1505, 'counter': 1506, 'kidnappers': 1507, 'unless': 1508, 'greater': 1509, 'tamil': 1510, 'accident': 1511, 'direct': 1512, 'threats': 1513, 'christmas': 1514, 'target': 1515, 'filed': 1516, 'resigned': 1517, 'canadian': 1518, 'nigerian': 1519, 'pirates': 1520, 'born': 1521, 'schools': 1522, 'executive': 1523, 'erupted': 1524, 'employees': 1525, 'beginning': 1526, 'militias': 1527, 'best': 1528, 'consider': 1529, 'meetings': 1530, 'giving': 1531, 'abdullah': 1532, 'housing': 1533, 'throughout': 1534, 'avoid': 1535, 'waziristan': 1536, 'ground': 1537, 'widespread': 1538, 'incidents': 1539, '1990s': 1540, 'mark': 1541, 'florida': 1542, 'accord': 1543, 'deficit': 1544, 'northeastern': 1545, 'pervez': 1546, 'recovery': 1547, 'damaged': 1548, 'located': 1549, 'lawyers': 1550, 'e': 1551, 'spent': 1552, 'increasing': 1553, 'shut': 1554, 'headquarters': 1555, 'bilateral': 1556, 'ivory': 1557, 'resignation': 1558, 'barrels': 1559, 'points': 1560, 'george': 1561, 'flights': 1562, 'risk': 1563, 'child': 1564, 'towns': 1565, 'upcoming': 1566, 'finance': 1567, 'driver': 1568, 'league': 1569, 'mohammad': 1570, 'torture': 1571, '1999': 1572, 'gold': 1573, 'powers': 1574, 'confidence': 1575, 'mubarak': 1576, 'crime': 1577, 'hu': 1578, 'politicians': 1579, 'ways': 1580, 'wife': 1581, 'accounts': 1582, 'diplomat': 1583, 'singh': 1584, 'research': 1585, 'sending': 1586, 'checkpoint': 1587, 'job': 1588, 'blew': 1589, 'side': 1590, 'rich': 1591, 'chemical': 1592, 'huge': 1593, 'fear': 1594, 'leadership': 1595, 'established': 1596, 'stopped': 1597, 'equipment': 1598, 'deployed': 1599, 'buy': 1600, 'looking': 1601, 'potential': 1602, 'industrial': 1603, 'draft': 1604, 'loss': 1605, 'kim': 1606, 'targeting': 1607, 'fair': 1608, 'visiting': 1609, 'panel': 1610, 'recession': 1611, 'bosnia': 1612, 'marines': 1613, 'effect': 1614, 'liberation': 1615, 'khan': 1616, 'reuters': 1617, 'netherlands': 1618, 'race': 1619, 'reconstruction': 1620, '1998': 1621, 'powerful': 1622, 'rumsfeld': 1623, 'southwestern': 1624, 'scale': 1625, '2011': 1626, 'apparently': 1627, 'norway': 1628, 'providing': 1629, 'present': 1630, 'maoist': 1631, 'moving': 1632, 'conducted': 1633, 'winds': 1634, 'enter': 1635, 'critical': 1636, 'stay': 1637, 'host': 1638, 'jose': 1639, 'ahmed': 1640, 'source': 1641, 'latin': 1642, 'costs': 1643, 'instead': 1644, 'levels': 1645, 'father': 1646, 'ecuador': 1647, 'triggered': 1648, 'ariel': 1649, 'created': 1650, 'aung': 1651, 'abroad': 1652, 'arrests': 1653, 'olympics': 1654, 'push': 1655, 'worth': 1656, 'hague': 1657, 'inflation': 1658, 'committed': 1659, 'zone': 1660, 'divided': 1661, 'businesses': 1662, 'produce': 1663, 'investigators': 1664, 'girl': 1665, 'fifth': 1666, 'necessary': 1667, 'petroleum': 1668, 'protection': 1669, 'worked': 1670, 'considering': 1671, 'moved': 1672, 'detonated': 1673, 'agree': 1674, 'prince': 1675, 'guard': 1676, 'scene': 1677, 'iaea': 1678, 'banks': 1679, 'survey': 1680, 'doing': 1681, 'thousand': 1682, 'haitian': 1683, 'ongoing': 1684, 'dominated': 1685, 'argentina': 1686, 'waters': 1687, 'kirkuk': 1688, 'age': 1689, 'imports': 1690, 'pipeline': 1691, 'vowed': 1692, 'plot': 1693, 'attackers': 1694, 'operating': 1695, 'winner': 1696, 'unidentified': 1697, 'ever': 1698, 'agreements': 1699, 'catholic': 1700, '35': 1701, 'sharply': 1702, 'figures': 1703, 'passengers': 1704, '150': 1705, 'weekly': 1706, 'zarqawi': 1707, 'hostage': 1708, 'hiv': 1709, 'document': 1710, 'minority': 1711, 'survivors': 1712, 'bangladesh': 1713, 'allowing': 1714, 'praised': 1715, 'remaining': 1716, 'real': 1717, 'conservative': 1718, 'seat': 1719, 'heart': 1720, 'dictator': 1721, 'stand': 1722, 'bay': 1723, 'imf': 1724, 'position': 1725, 'peninsula': 1726, 'caracas': 1727, 'explosions': 1728, 'occupied': 1729, 'tropical': 1730, 'unclear': 1731, 'navy': 1732, 'israelis': 1733, 'developed': 1734, 'benedict': 1735, 'communities': 1736, 'co': 1737, 'documents': 1738, 'saw': 1739, 'opponents': 1740, 'discussed': 1741, 'slow': 1742, '400': 1743, 'sentence': 1744, 'worker': 1745, 'formed': 1746, 'status': 1747, 'restrictions': 1748, 'currency': 1749, 'strengthen': 1750, 'accept': 1751, 'cheney': 1752, 'peru': 1753, 'villages': 1754, 'supporting': 1755, 'investors': 1756, 'greek': 1757, 'helicopters': 1758, 'damascus': 1759, 'earth': 1760, 'severe': 1761, 'un': 1762, 'ships': 1763, 'naval': 1764, 'highly': 1765, 'funeral': 1766, 'dialogue': 1767, 'failure': 1768, 'escaped': 1769, 'czech': 1770, 'fiscal': 1771, 'biggest': 1772, 'funding': 1773, 'according': 1774, 'chancellor': 1775, 'meters': 1776, 'happened': 1777, 'placed': 1778, 'giant': 1779, 'mayor': 1780, 'dangerous': 1781, 'kyi': 1782, 'built': 1783, 'designed': 1784, 'your': 1785, 'blasts': 1786, 'cuts': 1787, 'handed': 1788, 'university': 1789, 'completed': 1790, 'rain': 1791, 'abdul': 1792, 'troubled': 1793, 'crowd': 1794, 'resort': 1795, 'halt': 1796, 'jihad': 1797, 'safe': 1798, 'counterpart': 1799, 'overall': 1800, 'elect': 1801, 'actions': 1802, 'truce': 1803, 'music': 1804, 'mining': 1805, 'winter': 1806, 'goal': 1807, 'cars': 1808, 'michael': 1809, 'expect': 1810, 'jan': 1811, '600': 1812, 'nationwide': 1813, 'destruction': 1814, 'terms': 1815, 'sites': 1816, 'average': 1817, 'involving': 1818, 'prominent': 1819, 'david': 1820, '29': 1821, 'positions': 1822, 'web': 1823, 'figure': 1824, 'resistance': 1825, 'valley': 1826, 'quickly': 1827, 'light': 1828, 'jail': 1829, 'opinion': 1830, "n't": 1831, 'leftist': 1832, 'lawyer': 1833, 'champion': 1834, 'posted': 1835, 'attorney': 1836, 'gasoline': 1837, 'marched': 1838, 'widely': 1839, 'flooding': 1840, 'floods': 1841, 'suu': 1842, 'ensure': 1843, 'sectors': 1844, '1996': 1845, 'kidnapping': 1846, 'deadline': 1847, 'sought': 1848, 'tennis': 1849, 'extended': 1850, 'omar': 1851, 'illegally': 1852, 'though': 1853, 'osama': 1854, 'decline': 1855, 'kingdom': 1856, 'autonomy': 1857, 'receive': 1858, 'revolutionary': 1859, 'maliki': 1860, 'events': 1861, 'nothing': 1862, 'environmental': 1863, 'tested': 1864, 'pkk': 1865, 'takes': 1866, 'humans': 1867, 'kenyan': 1868, 'indicted': 1869, 'problem': 1870, 'my': 1871, 'immigrants': 1872, 'croatia': 1873, 'rules': 1874, 'fully': 1875, 'maintain': 1876, 'played': 1877, 'suffering': 1878, 'ceasefire': 1879, 'boat': 1880, 'compound': 1881, 'fought': 1882, 'drugs': 1883, 'officially': 1884, 'polish': 1885, 'threatening': 1886, 'break': 1887, 'account': 1888, 'pushed': 1889, 'losses': 1890, 'farc': 1891, 'attempts': 1892, 'cancer': 1893, 'preparing': 1894, 'foreigners': 1895, 'revenue': 1896, 'challenges': 1897, 'boy': 1898, 'internal': 1899, 'believes': 1900, 'approve': 1901, 'longer': 1902, 'conduct': 1903, 'immigration': 1904, 'accuses': 1905, 'formal': 1906, 'ugandan': 1907, 'square': 1908, 'complete': 1909, 'causing': 1910, 'we': 1911, 'factions': 1912, 'questioned': 1913, 'unity': 1914, 'field': 1915, 'producer': 1916, 'restore': 1917, 'follows': 1918, 'film': 1919, 'producing': 1920, 'estimates': 1921, 'itself': 1922, 'wall': 1923, 'reportedly': 1924, 'strongly': 1925, 'talabani': 1926, 'defeated': 1927, 'sovereignty': 1928, 'delivery': 1929, 'uganda': 1930, 'accuse': 1931, 'showing': 1932, 'hotel': 1933, 'bloc': 1934, 'sweden': 1935, 'manufacturing': 1936, 'hassan': 1937, 'tons': 1938, 'pass': 1939, '1994': 1940, 'bolivia': 1941, 'delta': 1942, 'indicate': 1943, 'planes': 1944, 'words': 1945, 'either': 1946, 'difficult': 1947, 'heads': 1948, 'centers': 1949, 'negotiators': 1950, 'tibet': 1951, 'especially': 1952, '32': 1953, 'addition': 1954, 'hearing': 1955, 'determine': 1956, 'particularly': 1957, 'uk': 1958, 'going': 1959, 'tourists': 1960, 'extremists': 1961, 'forecasters': 1962, 'climate': 1963, 'appointed': 1964, 'chickens': 1965, '1990': 1966, 'drop': 1967, 'rwanda': 1968, 'neighborhood': 1969, 'prize': 1970, 'value': 1971, 'star': 1972, 'commercial': 1973, 'bid': 1974, 'pentagon': 1975, 'boycott': 1976, 'territories': 1977, 'jailed': 1978, 'raided': 1979, 'makes': 1980, '1989': 1981, 'defeat': 1982, 'stronghold': 1983, 'crashed': 1984, 'steps': 1985, 'nationals': 1986, 'below': 1987, 'fidel': 1988, 'cash': 1989, 'morocco': 1990, 'ransom': 1991, 'resumed': 1992, 'caught': 1993, 'rest': 1994, 'themselves': 1995, 'opec': 1996, 'serbian': 1997, 'unrest': 1998, 'double': 1999, 'chile': 2000, 'sectarian': 2001, 'promote': 2002, 'secure': 2003, 'impact': 2004, 'donors': 2005, 'headed': 2006, 'revenues': 2007, 'minutes': 2008, 'block': 2009, 'gain': 2010, 'snow': 2011, 'forward': 2012, 'expand': 2013, 'sold': 2014, 'postponed': 2015, 'initial': 2016, 'assets': 2017, 'intended': 2018, 'contract': 2019, 'temporarily': 2020, 'jean': 2021, 'aristide': 2022, 'failing': 2023, 'opposed': 2024, 'single': 2025, 'merkel': 2026, 'blood': 2027, 'newly': 2028, 'teams': 2029, 'struggle': 2030, 'ballots': 2031, 'towards': 2032, 'turn': 2033, 'runs': 2034, 'reduced': 2035, 'overnight': 2036, 'pleaded': 2037, 'granted': 2038, 'vienna': 2039, 'prophet': 2040, 'material': 2041, 'taxes': 2042, '45': 2043, 'certain': 2044, 'environment': 2045, 'lines': 2046, 'appears': 2047, 'canceled': 2048, 'serve': 2049, 'serbia': 2050, 'avian': 2051, 'returning': 2052, 'homeland': 2053, 'unknown': 2054, 'thought': 2055, 'package': 2056, 'collapse': 2057, 'follow': 2058, 'scandal': 2059, 'devastated': 2060, '700': 2061, 'favor': 2062, 'assault': 2063, 'wing': 2064, 'yanukovych': 2065, 'larger': 2066, 'legislative': 2067, 'game': 2068, 'houses': 2069, 'representative': 2070, 'bringing': 2071, 'command': 2072, 'cents': 2073, 'exile': 2074, 'shares': 2075, 'self': 2076, 'congressional': 2077, 'aceh': 2078, 'reporting': 2079, 'let': 2080, 'voice': 2081, 'investigate': 2082, 'violations': 2083, 'caribbean': 2084, 'finding': 2085, 'available': 2086, 'feb': 2087, 'danish': 2088, 'pre': 2089, 'recognize': 2090, 'debate': 2091, 'data': 2092, 'mohamed': 2093, 'expects': 2094, 'wars': 2095, 'moon': 2096, 'wave': 2097, 'england': 2098, 'means': 2099, 'adopted': 2100, 'withdrew': 2101, 'smuggling': 2102, 'appear': 2103, 'continent': 2104, 'delayed': 2105, 'marine': 2106, 'yukos': 2107, 'presidents': 2108, 'matter': 2109, 'output': 2110, 'credit': 2111, 'scored': 2112, 'roads': 2113, 'flight': 2114, 'anyone': 2115, 'monetary': 2116, 'attending': 2117, 'cell': 2118, 'bad': 2119, 'lion': 2120, 'attention': 2121, 'ambushed': 2122, 'increasingly': 2123, 'tibetan': 2124, 'mar': 2125, 'renewed': 2126, 'radical': 2127, 'differences': 2128, 'khartoum': 2129, 'removed': 2130, 'institutions': 2131, 'agents': 2132, 'attended': 2133, 'remained': 2134, 'speaker': 2135, 'repeated': 2136, 'recovered': 2137, 'strategy': 2138, 'reserves': 2139, 'award': 2140, 'stable': 2141, 'cooperate': 2142, 'miners': 2143, 'surgery': 2144, 'morales': 2145, 'oppose': 2146, 'nasa': 2147, 'villagers': 2148, 'resulted': 2149, 'challenge': 2150, 'torn': 2151, 'female': 2152, 'offering': 2153, 'camps': 2154, 'uprising': 2155, 'winning': 2156, 'improved': 2157, 'los': 2158, 'abuses': 2159, 'presented': 2160, 'capacity': 2161, 'receiving': 2162, 'drove': 2163, '34': 2164, 'brazilian': 2165, 'roman': 2166, 'briefly': 2167, 'donald': 2168, 'aziz': 2169, 'compared': 2170, 'zealand': 2171, 'sale': 2172, 'victim': 2173, 'settlers': 2174, 'banking': 2175, 'euro': 2176, 'vessel': 2177, 'above': 2178, 'sharing': 2179, 'organized': 2180, 'monitor': 2181, 'monitors': 2182, '1980s': 2183, 'evening': 2184, 'nomination': 2185, 'separatists': 2186, 'slightly': 2187, 'jazeera': 2188, 'kurds': 2189, 'contain': 2190, 'finished': 2191, 'ally': 2192, 'seriously': 2193, 'entire': 2194, 'responded': 2195, 'sell': 2196, 'prompted': 2197, 'deals': 2198, 'nobel': 2199, 'reason': 2200, 'eve': 2201, 'survived': 2202, 'admitted': 2203, 'moderate': 2204, 'wide': 2205, 'driven': 2206, 'christians': 2207, 'accusations': 2208, 'management': 2209, 'accusing': 2210, 'rafik': 2211, 'collapsed': 2212, 'observers': 2213, 'federation': 2214, 'georgian': 2215, 'extend': 2216, 'tiger': 2217, 'ehud': 2218, 'grew': 2219, 'story': 2220, 'deployment': 2221, 'blocked': 2222, 'kilometer': 2223, 'willing': 2224, 'visits': 2225, 'centuries': 2226, 'transport': 2227, 'angeles': 2228, 'traveled': 2229, 'firing': 2230, 'battling': 2231, 'outbreaks': 2232, 'various': 2233, 'deadliest': 2234, '1997': 2235, 'trafficking': 2236, 'surveillance': 2237, 'begins': 2238, 'initiative': 2239, 'different': 2240, 'fighter': 2241, 'defendants': 2242, 'earnings': 2243, 'deep': 2244, 'know': 2245, 'burned': 2246, 'treated': 2247, 'gathering': 2248, 'tourist': 2249, 'dozen': 2250, 'forcing': 2251, 'jakarta': 2252, 'northeast': 2253, 'fallujah': 2254, 'relatives': 2255, 'standards': 2256, 'summer': 2257, 'pontiff': 2258, 'falling': 2259, 'signs': 2260, 'struggling': 2261, 'kuwait': 2262, 'karachi': 2263, 'stressed': 2264, 'threw': 2265, 'establish': 2266, 'influence': 2267, 'word': 2268, 'patients': 2269, '800': 2270, 'revolution': 2271, 'grand': 2272, 'suspend': 2273, 'decide': 2274, 'policeman': 2275, 'ibrahim': 2276, '36': 2277, 'beat': 2278, 'jun': 2279, 'player': 2280, 'chance': 2281, 'protesting': 2282, 'developments': 2283, 'traditional': 2284, 'marked': 2285, 'schroeder': 2286, 'flying': 2287, 'conducting': 2288, 'proposals': 2289, 'havana': 2290, 'cast': 2291, 'bases': 2292, 'raising': 2293, 'magnitude': 2294, 'anbar': 2295, 'thai': 2296, 'singapore': 2297, 'straight': 2298, 'riots': 2299, 'bureau': 2300, 'materials': 2301, 'austria': 2302, 'pilgrims': 2303, 'clashed': 2304, 'prisoner': 2305, 'staged': 2306, 'ease': 2307, '55': 2308, 'searching': 2309, 'serving': 2310, 'soil': 2311, 'pulled': 2312, 'meant': 2313, 'escape': 2314, 'index': 2315, 'chirac': 2316, 'ice': 2317, 'effective': 2318, 'risen': 2319, 'switzerland': 2320, 'farmers': 2321, 'magazine': 2322, 'multi': 2323, 'sets': 2324, 'kidnappings': 2325, 'commitment': 2326, 'citing': 2327, 'transferred': 2328, 'question': 2329, 'lawmaker': 2330, 'stage': 2331, 'musab': 2332, "shi'ites": 2333, 'driving': 2334, 'singer': 2335, 'seeded': 2336, 'atlantic': 2337, 'malaysia': 2338, 'defend': 2339, 'phone': 2340, 'mccain': 2341, 'peter': 2342, 'grenade': 2343, 'marking': 2344, 'customs': 2345, 'controls': 2346, 'partner': 2347, 'pact': 2348, 'benefits': 2349, 'secretly': 2350, 'orders': 2351, 'shuttle': 2352, 'neighbors': 2353, 'rwandan': 2354, 'meter': 2355, 'retired': 2356, 'typhoon': 2357, 'shown': 2358, 'signing': 2359, 'reconciliation': 2360, 'starting': 2361, 'gates': 2362, 'asking': 2363, 'track': 2364, 'yemen': 2365, 'capture': 2366, 'lankan': 2367, 'stormed': 2368, 'jets': 2369, 'respond': 2370, 'jordanian': 2371, 'apr': 2372, 'muhammad': 2373, 'interests': 2374, 'airlines': 2375, 'strategic': 2376, 'allawi': 2377, 'done': 2378, 'gained': 2379, 'satellite': 2380, 'provides': 2381, 'congolese': 2382, 'kyrgyzstan': 2383, '75': 2384, 'tape': 2385, 'requested': 2386, 'drive': 2387, 'polling': 2388, 'rather': 2389, 'whom': 2390, 'systems': 2391, 'reserve': 2392, 'getting': 2393, 'prepared': 2394, 'why': 2395, 'sheikh': 2396, 'erdogan': 2397, 'insurance': 2398, 'riot': 2399, 'daughter': 2400, 'hiding': 2401, 'appealed': 2402, 'seoul': 2403, 'monarchy': 2404, 'economists': 2405, 'closely': 2406, 'jalal': 2407, 'memorial': 2408, '07': 2409, 'devastating': 2410, 'frequent': 2411, '1993': 2412, 'kazakhstan': 2413, 'afghans': 2414, 'disappeared': 2415, 'ghraib': 2416, 'citizen': 2417, 'insists': 2418, 'blame': 2419, 'luis': 2420, 'brown': 2421, 'cyprus': 2422, 'crossed': 2423, 'smaller': 2424, 'expansion': 2425, 'passing': 2426, 'philippine': 2427, '38': 2428, 'produced': 2429, 'split': 2430, 'cutting': 2431, 'homeless': 2432, 'swiss': 2433, 'cartoons': 2434, 'opportunity': 2435, 'amount': 2436, 'selling': 2437, 'plotting': 2438, 'mother': 2439, 'treasury': 2440, 'uribe': 2441, 'prisons': 2442, 'baluchistan': 2443, 'twice': 2444, 'corporation': 2445, 'stronger': 2446, 'pledge': 2447, 'our': 2448, 'noted': 2449, 'numerous': 2450, 'belarus': 2451, '250': 2452, 'commerce': 2453, 'philippines': 2454, 'swept': 2455, 'stalled': 2456, 'buried': 2457, 'players': 2458, 'james': 2459, 'allows': 2460, 'van': 2461, 'paper': 2462, 'confirm': 2463, 'royal': 2464, '19th': 2465, 'empire': 2466, 'active': 2467, 'industries': 2468, 'arafat': 2469, 'c': 2470, 'supported': 2471, 'guerrillas': 2472, 'assad': 2473, 'geneva': 2474, 'minute': 2475, 'offshore': 2476, 'improving': 2477, 'successful': 2478, 'basra': 2479, 'rangoon': 2480, 'coal': 2481, 'negotiator': 2482, 'english': 2483, 'holds': 2484, 'denmark': 2485, 'restive': 2486, 'coastal': 2487, 'payments': 2488, 'ambush': 2489, 'ask': 2490, 'reporter': 2491, 'fate': 2492, 'videotape': 2493, 'basic': 2494, 'got': 2495, 'colonel': 2496, 'numbers': 2497, 'marks': 2498, 'hospitals': 2499, 'heading': 2500, 'impose': 2501, 'acting': 2502, 'humanity': 2503, 'rodriguez': 2504, 'brief': 2505, 'hunger': 2506, 'shortages': 2507, 'website': 2508, 'faction': 2509, 'transfer': 2510, 'suggested': 2511, 'neither': 2512, 'passenger': 2513, 'samples': 2514, '120': 2515, 'defended': 2516, 'sarkozy': 2517, 'accepted': 2518, 'resign': 2519, 'spying': 2520, 'cargo': 2521, 'kept': 2522, 'runoff': 2523, 'distribution': 2524, 'traffic': 2525, 'alexander': 2526, 'powell': 2527, 'koizumi': 2528, 'primary': 2529, 'kathmandu': 2530, 'rome': 2531, 'lifted': 2532, 'closing': 2533, 'scores': 2534, 'temporary': 2535, 'grave': 2536, 'delivered': 2537, 'computer': 2538, 'chen': 2539, 'moves': 2540, 'lieutenant': 2541, 'setting': 2542, 'rebuild': 2543, 'acknowledged': 2544, 'commissioner': 2545, 'communications': 2546, 'nouri': 2547, 'brussels': 2548, 'embargo': 2549, 'proliferation': 2550, 'injury': 2551, 'fish': 2552, 'jintao': 2553, 'backing': 2554, 'heard': 2555, 'participate': 2556, 'incentives': 2557, 'discussions': 2558, 'mugabe': 2559, 'shell': 2560, 'works': 2561, 'mainland': 2562, 'quit': 2563, 'gazprom': 2564, 'becoming': 2565, 'required': 2566, 'determined': 2567, 'declining': 2568, 'advance': 2569, 'trapped': 2570, 'seconds': 2571, '64': 2572, 'arabs': 2573, 'recover': 2574, 'preval': 2575, 'inspectors': 2576, 'temperatures': 2577, 'southwest': 2578, 'closer': 2579, 'festival': 2580, 'probably': 2581, 'economies': 2582, 'language': 2583, 'jews': 2584, 'monitoring': 2585, 'sadr': 2586, 'agenda': 2587, 'overthrow': 2588, 'hosni': 2589, 'mortar': 2590, 'welcomed': 2591, 'leaves': 2592, 'disarm': 2593, 'shelter': 2594, 'pull': 2595, 'asylum': 2596, 'milosevic': 2597, 'judges': 2598, 'laboratory': 2599, 'identify': 2600, 'husband': 2601, 'weapon': 2602, 'external': 2603, 'delegates': 2604, 'senegal': 2605, 'rock': 2606, 'ramadi': 2607, 'brotherhood': 2608, 'farms': 2609, 'preliminary': 2610, 'gang': 2611, 'ranking': 2612, '65': 2613, 'poorest': 2614, 'easily': 2615, 'cited': 2616, 'supports': 2617, 'me': 2618, 'aide': 2619, 'society': 2620, 'socialist': 2621, 'possibility': 2622, 'grenades': 2623, 'suspension': 2624, 'trucks': 2625, 'speed': 2626, 'finland': 2627, 'competition': 2628, 'faced': 2629, 'broken': 2630, 'prayers': 2631, 'limit': 2632, 'costa': 2633, 'attempted': 2634, 'standing': 2635, 'sustained': 2636, 'rescued': 2637, 'statements': 2638, 'career': 2639, 'ayatollah': 2640, 'commanders': 2641, 'settlements': 2642, 'reactor': 2643, 'bordering': 2644, 'greece': 2645, 'discovery': 2646, 'highway': 2647, 'directly': 2648, 'hands': 2649, 'encourage': 2650, 'subway': 2651, 'lama': 2652, 'nairobi': 2653, 'aqsa': 2654, 'sports': 2655, 'lived': 2656, 'fresh': 2657, 'student': 2658, 'girls': 2659, 'newspapers': 2660, 'mobile': 2661, 'upper': 2662, 'device': 2663, 'telephone': 2664, 'organizers': 2665, 'bail': 2666, 'respect': 2667, 'die': 2668, 'referred': 2669, 'dependent': 2670, 'friends': 2671, 'transportation': 2672, 'seeing': 2673, 'artillery': 2674, 'fast': 2675, 'demonstration': 2676, 'look': 2677, 'prepare': 2678, 'route': 2679, 'sugar': 2680, 'herzegovina': 2681, 'advanced': 2682, 'speak': 2683, 'accidents': 2684, 'ahmad': 2685, 'astronauts': 2686, 'sponsored': 2687, 'personal': 2688, 'museum': 2689, 'politician': 2690, 'kurdistan': 2691, 'frequently': 2692, 'launching': 2693, 'playing': 2694, 'researchers': 2695, 'surrounding': 2696, 'extremist': 2697, '33': 2698, 'purposes': 2699, 'khodorkovsky': 2700, 'reasons': 2701, 'ankara': 2702, 'images': 2703, 'panama': 2704, 'politics': 2705, 'rescuers': 2706, 'nicaragua': 2707, 'strength': 2708, 'enemy': 2709, 'denounced': 2710, 'prevented': 2711, 'liberia': 2712, 'lukashenko': 2713, 'confirmation': 2714, 'manmohan': 2715, 'contracted': 2716, 'gives': 2717, 'pullout': 2718, 'factory': 2719, 'relatively': 2720, 'swat': 2721, 'mullah': 2722, 'teachers': 2723, 'rape': 2724, 'mines': 2725, '52': 2726, 'underground': 2727, 'jacques': 2728, '37': 2729, 'inmates': 2730, 'cold': 2731, 'negotiate': 2732, 'indicated': 2733, 'native': 2734, 'uzbekistan': 2735, 'practice': 2736, 'wild': 2737, 'reducing': 2738, 'reduction': 2739, 'changed': 2740, 'apparent': 2741, 'fears': 2742, 'replaced': 2743, 'drought': 2744, 'sharp': 2745, 'creation': 2746, 'kiev': 2747, 'plants': 2748, 'khatami': 2749, 'draw': 2750, 'subsidies': 2751, 'tv': 2752, 'sixth': 2753, 'eritrea': 2754, 'experienced': 2755, 'declaration': 2756, 'fallen': 2757, 'eventually': 2758, 'richard': 2759, 'focused': 2760, 'nominee': 2761, 'big': 2762, 'suspicion': 2763, 'records': 2764, 'initially': 2765, 'croatian': 2766, 'imported': 2767, 'overseas': 2768, 'grow': 2769, 'honor': 2770, 'regulations': 2771, 'vaccine': 2772, 'gunfire': 2773, 'landed': 2774, 'republicans': 2775, 'mali': 2776, 'remove': 2777, 'warming': 2778, 'causes': 2779, 'libya': 2780, 'kremlin': 2781, 'restored': 2782, 'afternoon': 2783, 'seventh': 2784, 'questioning': 2785, 'retirement': 2786, 'thirds': 2787, 'net': 2788, 'blow': 2789, 'celebrations': 2790, 'nor': 2791, 'transitional': 2792, 'bound': 2793, 'unharmed': 2794, 'foundation': 2795, 'combined': 2796, 'roughly': 2797, 'disputes': 2798, '53': 2799, 'ismail': 2800, 'withdrawing': 2801, 'wake': 2802, 'handling': 2803, 'questions': 2804, 'spreading': 2805, 'investments': 2806, 'zabul': 2807, 'cnn': 2808, 'violating': 2809, 'sergei': 2810, 'complex': 2811, 'hostile': 2812, 'usually': 2813, 'mountainous': 2814, 'joseph': 2815, 'angry': 2816, 'photographs': 2817, 'charles': 2818, 'relationship': 2819, 'deliver': 2820, '85': 2821, 'uruzgan': 2822, 'gun': 2823, 'ramadan': 2824, 'implement': 2825, 'ripped': 2826, 'rare': 2827, 'indicates': 2828, 'courts': 2829, 'alert': 2830, 'testing': 2831, 'illness': 2832, 'shops': 2833, 'save': 2834, 'exercise': 2835, '1992': 2836, 'appeals': 2837, 'hall': 2838, 'basis': 2839, 'loans': 2840, 'sentences': 2841, 'originally': 2842, 'salvador': 2843, '41': 2844, 'stroke': 2845, 'plunged': 2846, 'stands': 2847, 'sworn': 2848, 'kibaki': 2849, 'require': 2850, 'grant': 2851, 'chilean': 2852, 'clerics': 2853, 'complaints': 2854, 'qatar': 2855, 'resolved': 2856, 'partnership': 2857, 'districts': 2858, 'raul': 2859, 'assistant': 2860, 'green': 2861, 'pursue': 2862, 'slogans': 2863, 'attacking': 2864, 'extradition': 2865, 'ability': 2866, 'charter': 2867, 'brigades': 2868, 'expanded': 2869, 'title': 2870, 'cover': 2871, 'executed': 2872, 'centered': 2873, 'dealing': 2874, 'celebrate': 2875, 'polio': 2876, 'televised': 2877, 'barred': 2878, 'hijacked': 2879, 'dick': 2880, 'prosecution': 2881, 'swedish': 2882, 'operated': 2883, 'wealth': 2884, 'location': 2885, 'contributed': 2886, 'gone': 2887, 'paramilitary': 2888, 'arriving': 2889, 'migrants': 2890, 'dalai': 2891, 'pandemic': 2892, 'movie': 2893, 'abandon': 2894, 'aired': 2895, 'channel': 2896, 'angola': 2897, 'william': 2898, 'jet': 2899, 'destroy': 2900, 'donor': 2901, 'duty': 2902, 'transition': 2903, 'surge': 2904, 'airstrikes': 2905, 'territorial': 2906, 'surrounded': 2907, 'allied': 2908, 'crowded': 2909, 'breakaway': 2910, 'stem': 2911, 'forming': 2912, 'success': 2913, 'couple': 2914, 'invited': 2915, 'tight': 2916, 'lay': 2917, 'mikhail': 2918, 'agent': 2919, 'promoting': 2920, 'attacker': 2921, 'surface': 2922, '20th': 2923, 'settled': 2924, 'introduced': 2925, 'size': 2926, 'norwegian': 2927, 'politically': 2928, 'volatile': 2929, 'discussing': 2930, 'ramallah': 2931, 'normal': 2932, 'packed': 2933, 'gyanendra': 2934, 'zawahiri': 2935, 'rounds': 2936, 'places': 2937, 'aboard': 2938, 'massacre': 2939, 'hospitalized': 2940, 'execution': 2941, 'detected': 2942, 'st': 2943, 'injuring': 2944, 'drone': 2945, 'gangs': 2946, 'kunar': 2947, 'togo': 2948, 'clean': 2949, 'profit': 2950, 'saint': 2951, 'replied': 2952, 'substantial': 2953, 'martyrs': 2954, 'entering': 2955, 'considers': 2956, 'pictures': 2957, 'explosive': 2958, 'requires': 2959, 'sex': 2960, 'carlos': 2961, 'kennedy': 2962, 'citizenship': 2963, 'crops': 2964, 'extensive': 2965, 'capita': 2966, 'flew': 2967, 'ass': 2968, 'lift': 2969, 'putting': 2970, 'advisor': 2971, 'bloody': 2972, 'successfully': 2973, 'martin': 2974, '17th': 2975, 'evacuated': 2976, 'junta': 2977, 'loyal': 2978, 'khost': 2979, 'affect': 2980, 'boeing': 2981, 'prior': 2982, 'eta': 2983, 'participation': 2984, 'creating': 2985, 'bashar': 2986, 'coordinated': 2987, 'troop': 2988, 'ceremonies': 2989, 'macedonia': 2990, 'rural': 2991, 'airstrike': 2992, 'animal': 2993, 'assumed': 2994, 'performance': 2995, 'limits': 2996, 'entry': 2997, 'blockade': 2998, 'emissions': 2999, 'bali': 3000, 'recorded': 3001, 'violation': 3002, 'achieved': 3003, 'hundred': 3004, 'protested': 3005, 'historic': 3006, 'buddhist': 3007, 'waves': 3008, 'governing': 3009, 'permission': 3010, 'unions': 3011, 'reaching': 3012, 'beating': 3013, 'hill': 3014, 'speculation': 3015, 'appearance': 3016, 'book': 3017, 'firms': 3018, 'industrialized': 3019, 'occupation': 3020, 'warplanes': 3021, 'false': 3022, 'disrupt': 3023, 'editor': 3024, 'torch': 3025, 'transit': 3026, 'wearing': 3027, 'institute': 3028, 'colony': 3029, 'finally': 3030, 'opposes': 3031, 'bodyguards': 3032, 'pressing': 3033, 'straw': 3034, 'informed': 3035, 'engineers': 3036, 'slowly': 3037, 'autonomous': 3038, 'cambodia': 3039, 'activist': 3040, 'baquba': 3041, 'photos': 3042, 'belgium': 3043, 'boosting': 3044, 'names': 3045, 'alive': 3046, 'legislature': 3047, 'items': 3048, 'boats': 3049, 'latvia': 3050, 'proceedings': 3051, 'uzbek': 3052, 'outgoing': 3053, 'elbaradei': 3054, 'closure': 3055, 'primarily': 3056, 'opium': 3057, 'mudslides': 3058, 'g': 3059, 'afp': 3060, 'm': 3061, 'specific': 3062, 'exporting': 3063, 'engaged': 3064, 'trained': 3065, 'hutu': 3066, 'publicly': 3067, 'ghana': 3068, 'announce': 3069, 'consulate': 3070, 'alvaro': 3071, 'intense': 3072, 'scott': 3073, 'shrine': 3074, 'duties': 3075, 'consumers': 3076, 'flood': 3077, 'malawi': 3078, 'google': 3079, 'fujimori': 3080, 'chechen': 3081, 'guatemala': 3082, 'minor': 3083, 'birth': 3084, 'blames': 3085, 'talk': 3086, 'pinochet': 3087, 'influential': 3088, 'madrid': 3089, 'regular': 3090, 'obtained': 3091, 'algerian': 3092, 'cleared': 3093, 'contractors': 3094, 'strained': 3095, 'goals': 3096, 'netanyahu': 3097, 'recognized': 3098, 'surrender': 3099, 'shootout': 3100, 'gains': 3101, '16th': 3102, 'written': 3103, 'apartment': 3104, 'generally': 3105, 'merger': 3106, 'claiming': 3107, 'ambitions': 3108, 'withdrawn': 3109, 'subject': 3110, 'freeze': 3111, 'unable': 3112, 'dr': 3113, 'sexual': 3114, 'busy': 3115, 'koreans': 3116, 'partners': 3117, 'embassies': 3118, 'fields': 3119, 'silva': 3120, '46': 3121, 'flow': 3122, 'conflicts': 3123, 'inquiry': 3124, 'gnassingbe': 3125, 'complained': 3126, 'uncovered': 3127, 'deny': 3128, 'extending': 3129, 'portugal': 3130, 'burning': 3131, 'rivals': 3132, 'visitors': 3133, 'penalty': 3134, 'pakistanis': 3135, 'vicente': 3136, 'telecommunications': 3137, 'cricket': 3138, 'fires': 3139, 'registered': 3140, 'boycotted': 3141, 'imprisoned': 3142, 'instability': 3143, 'accords': 3144, 'standoff': 3145, 'string': 3146, 'feared': 3147, 'investigated': 3148, 'mandate': 3149, 'mountain': 3150, 'founded': 3151, 'subsistence': 3152, 'eagle': 3153, 'ran': 3154, 'billions': 3155, 'succeed': 3156, 'jury': 3157, 'fierce': 3158, 'employment': 3159, 'charity': 3160, 'turin': 3161, 'depends': 3162, 'rapidly': 3163, 'solidarity': 3164, 'demonstrated': 3165, 'ghazni': 3166, 'azerbaijan': 3167, 'solution': 3168, 'protecting': 3169, 'rebuilding': 3170, 'rica': 3171, 'senators': 3172, 'berlin': 3173, 'motivated': 3174, 'property': 3175, 'devices': 3176, 'deposed': 3177, 'eligible': 3178, 'chechnya': 3179, 'cypriot': 3180, 'soccer': 3181, 'grade': 3182, 'da': 3183, 'remittances': 3184, 'guinea': 3185, 'linking': 3186, 'arrive': 3187, 'calm': 3188, 'reject': 3189, 'thomas': 3190, 'yugoslav': 3191, '73': 3192, 'zardari': 3193, 'installed': 3194, 'hoping': 3195, 'parents': 3196, 'era': 3197, 'nominated': 3198, 'endorsed': 3199, 'angela': 3200, 'forum': 3201, 'criminals': 3202, '95': 3203, 'mumbai': 3204, 'seed': 3205, 'encouraged': 3206, 'denying': 3207, 'successor': 3208, 'd': 3209, 'medvedev': 3210, 'section': 3211, 'captain': 3212, 'dissidents': 3213, 'drc': 3214, 'profits': 3215, 'tymoshenko': 3216, 'managed': 3217, 'recep': 3218, 'tayyip': 3219, 'messages': 3220, 'sensitive': 3221, 'preventing': 3222, 'destroying': 3223, 'gap': 3224, 'hideout': 3225, 'predicted': 3226, 'trials': 3227, 'adding': 3228, 'trust': 3229, 'tell': 3230, 'annually': 3231, 'possibly': 3232, 'park': 3233, 'congressman': 3234, 'conspiracy': 3235, 'surrendered': 3236, 'count': 3237, 'purchase': 3238, 'funded': 3239, 'boys': 3240, 'louisiana': 3241, 'existing': 3242, 'college': 3243, 'centimeters': 3244, 'burundi': 3245, 'map': 3246, 'increases': 3247, '140': 3248, 'howard': 3249, 'stadium': 3250, '2012': 3251, 'ireland': 3252, 'assailants': 3253, 'persian': 3254, 'concluded': 3255, 'joining': 3256, 'textile': 3257, 'compensation': 3258, 'lee': 3259, 'completely': 3260, 'extremely': 3261, 'garang': 3262, 'brings': 3263, 'insisted': 3264, 'view': 3265, 'club': 3266, 'downturn': 3267, 'units': 3268, 'sure': 3269, 'experience': 3270, 'schedule': 3271, 'individuals': 3272, 'mars': 3273, 'ex': 3274, 'mauritania': 3275, 'orthodox': 3276, 'thaksin': 3277, 'technical': 3278, 'airline': 3279, 'compete': 3280, 'sound': 3281, 'angered': 3282, 'uruguay': 3283, 'processing': 3284, 'broad': 3285, 'likud': 3286, 'saakashvili': 3287, 'estimate': 3288, 'waiting': 3289, 'class': 3290, 'store': 3291, 'loan': 3292, 'addressed': 3293, 'peres': 3294, 'alone': 3295, '1970s': 3296, 'hunt': 3297, 'link': 3298, 'acts': 3299, 'diseases': 3300, 'malaria': 3301, 'voter': 3302, 'stopping': 3303, 'maintained': 3304, 'kashmiri': 3305, 'division': 3306, 'christopher': 3307, 'operate': 3308, 'blocking': 3309, 'enriched': 3310, 'lopez': 3311, 'williams': 3312, 'comprehensive': 3313, 'science': 3314, 'irish': 3315, 'ill': 3316, 'picked': 3317, 'art': 3318, 'johnson': 3319, 'capable': 3320, 'manuel': 3321, 'rejecting': 3322, 'impoverished': 3323, 'libyan': 3324, 'exercises': 3325, 'choose': 3326, 'wrong': 3327, 'abc': 3328, '1988': 3329, 'alternative': 3330, 'grown': 3331, 'directed': 3332, 'commented': 3333, 'testimony': 3334, 'witness': 3335, 'celebrated': 3336, 'vast': 3337, 'bertrand': 3338, 'violate': 3339, 'planted': 3340, 'ivanov': 3341, '57': 3342, 'abandoned': 3343, 'gunbattle': 3344, 'flee': 3345, 'fifa': 3346, 'enforcement': 3347, 'fellow': 3348, 'drinking': 3349, 'tanzania': 3350, 'unveiled': 3351, 'flooded': 3352, 'submitted': 3353, 'weak': 3354, 'chaos': 3355, 'course': 3356, '130': 3357, 'jackson': 3358, 'turning': 3359, 'smith': 3360, 'underwent': 3361, 'belonging': 3362, 'amendment': 3363, 'pilot': 3364, '76': 3365, 'carroll': 3366, 'moroccan': 3367, 'effects': 3368, 'danger': 3369, 'enclave': 3370, 'hearings': 3371, 'tough': 3372, 'timetable': 3373, 'disrupted': 3374, 'arabic': 3375, 'drew': 3376, 'journal': 3377, 'always': 3378, 'worried': 3379, 'none': 3380, 'walked': 3381, 'p': 3382, 'refineries': 3383, 'wrote': 3384, 'standard': 3385, 'vulnerable': 3386, 'earned': 3387, 'foot': 3388, 'internationally': 3389, 'harsh': 3390, 'ammunition': 3391, 'goes': 3392, 'motion': 3393, 'image': 3394, 'pursuing': 3395, 'infection': 3396, 'abdel': 3397, 'ayman': 3398, 'disarmament': 3399, 'irregularities': 3400, 'mixed': 3401, 'curb': 3402, 'contribute': 3403, 'plagued': 3404, 'quote': 3405, 'gbagbo': 3406, 'findings': 3407, 'eating': 3408, '1979': 3409, 'crucial': 3410, 'exploration': 3411, 'tikrit': 3412, 'refinery': 3413, 'subsequent': 3414, 'tree': 3415, 'generals': 3416, 'hunting': 3417, 'tanks': 3418, 'veto': 3419, 'judicial': 3420, 'ranch': 3421, 'meets': 3422, 'keeping': 3423, 'extension': 3424, 'ring': 3425, 'article': 3426, 'leg': 3427, 'aides': 3428, '42': 3429, 'soaring': 3430, 'electronic': 3431, 'dubai': 3432, 'spy': 3433, 'unnamed': 3434, 'disasters': 3435, 'paying': 3436, 'indigenous': 3437, 'relay': 3438, 'warns': 3439, 'counted': 3440, 'nature': 3441, 'culture': 3442, 'multiple': 3443, 'pace': 3444, 'ends': 3445, 'tom': 3446, 'indictment': 3447, 'beyond': 3448, 'highs': 3449, 'jaafari': 3450, 'srebrenica': 3451, 'pop': 3452, 'b': 3453, 'slowed': 3454, 'eid': 3455, 'overwhelmingly': 3456, 'bulgaria': 3457, 'bought': 3458, 'del': 3459, 'modern': 3460, 'approach': 3461, 'prospects': 3462, '1967': 3463, 'band': 3464, 'churches': 3465, 'romania': 3466, 'gerhard': 3467, 'missions': 3468, 'clearing': 3469, 'idea': 3470, '1984': 3471, 'mrs': 3472, 'tradition': 3473, 'hotels': 3474, 'moment': 3475, 'returns': 3476, 'mortars': 3477, 'advantage': 3478, 'exporter': 3479, 'expanding': 3480, 'inadequate': 3481, 'istanbul': 3482, 'tunnel': 3483, 'aviation': 3484, 'brain': 3485, 'strengthening': 3486, 'extra': 3487, 'counts': 3488, 'carolina': 3489, 'rubble': 3490, 'retaliation': 3491, 'shortage': 3492, 'bombed': 3493, 'secular': 3494, 'attempting': 3495, 'resolving': 3496, 'weekend': 3497, 'planet': 3498, 'guerrilla': 3499, 'style': 3500, 'fed': 3501, 'balance': 3502, 'integration': 3503, 'rallied': 3504, 'views': 3505, 'obasanjo': 3506, 'rapid': 3507, 'doctor': 3508, 'identity': 3509, 'corps': 3510, 'jong': 3511, 'il': 3512, 'nicolas': 3513, 'semi': 3514, 'sichuan': 3515, 'formerly': 3516, 'microsoft': 3517, 'peruvian': 3518, 'leads': 3519, 'innocent': 3520, 'refusal': 3521, 'refer': 3522, 'interrogation': 3523, 'administrative': 3524, 'represent': 3525, 'abkhazia': 3526, 'mistake': 3527, 'junichiro': 3528, 'residence': 3529, 'municipal': 3530, 'f': 3531, 'trouble': 3532, 'options': 3533, 'deeply': 3534, 'jack': 3535, 'europeans': 3536, 'slovakia': 3537, 'surprise': 3538, 'nepalese': 3539, 'iyad': 3540, 'friendly': 3541, 'read': 3542, '1982': 3543, 'warn': 3544, 'climbed': 3545, 'portion': 3546, 'holocaust': 3547, 'brokered': 3548, 'himalayan': 3549, 'flawed': 3550, 'mount': 3551, 'colombo': 3552, 'hitting': 3553, 'miami': 3554, 'path': 3555, 'medicine': 3556, 'animals': 3557, 'covered': 3558, '58': 3559, 'attracted': 3560, '48': 3561, 'unacceptable': 3562, 'rallies': 3563, 'importance': 3564, 'vessels': 3565, 'fugitive': 3566, 'java': 3567, 'broadcasting': 3568, 'underway': 3569, 'ki': 3570, 'worse': 3571, 'hoped': 3572, '1960': 3573, 'cocaine': 3574, 'expires': 3575, 'posts': 3576, 'sun': 3577, 'shots': 3578, 'youth': 3579, 'wto': 3580, 'contained': 3581, 'bridge': 3582, 'bashir': 3583, 'significantly': 3584, 'chosen': 3585, 'lagos': 3586, 'fbi': 3587, 'think': 3588, 'fly': 3589, 'founder': 3590, 'reflect': 3591, 'longtime': 3592, 'religion': 3593, 'subsequently': 3594, 'bahrain': 3595, 'recognition': 3596, 'someone': 3597, 'belarusian': 3598, 'consecutive': 3599, 'famous': 3600, '43': 3601, 'guns': 3602, 'sometimes': 3603, 'donations': 3604, 'tied': 3605, 'srinagar': 3606, 'dropping': 3607, 'phase': 3608, 'spend': 3609, '44': 3610, 'payment': 3611, 'parade': 3612, 'alberto': 3613, 'upset': 3614, 'austrian': 3615, 'visa': 3616, 'compromise': 3617, 'karbala': 3618, 'revealed': 3619, 'generate': 3620, 'decisions': 3621, 'shared': 3622, 'slowdown': 3623, 'yugoslavia': 3624, 'squad': 3625, 'notes': 3626, 'revive': 3627, 'emerging': 3628, 'awarded': 3629, 'tank': 3630, 'hurricanes': 3631, 'hillary': 3632, 'undermine': 3633, 'montenegro': 3634, 'original': 3635, 'intention': 3636, 'thanked': 3637, 'authorized': 3638, 'balloting': 3639, 'sidelines': 3640, 'responding': 3641, 'aggressive': 3642, 'lahoud': 3643, 'cartel': 3644, 'missed': 3645, 'ten': 3646, 'kind': 3647, 'recommended': 3648, 'solana': 3649, 'cubans': 3650, 'bolivian': 3651, 'alito': 3652, 'structure': 3653, 'dam': 3654, 'spiritual': 3655, '39': 3656, 'fine': 3657, 'looks': 3658, 'teenager': 3659, 'jesus': 3660, 'warlords': 3661, 'mcclellan': 3662, 'grounds': 3663, 'livestock': 3664, 'stimulus': 3665, 'swine': 3666, 'arizona': 3667, 'taylor': 3668, 'debris': 3669, 'farmer': 3670, 'mounting': 3671, 'rigged': 3672, 'boosted': 3673, 'argentine': 3674, 'djibouti': 3675, 'arrival': 3676, 'anger': 3677, 'involves': 3678, 'soared': 3679, 'evasion': 3680, 'emerged': 3681, 'brigadier': 3682, 'carter': 3683, 'choice': 3684, 'priority': 3685, 'colin': 3686, 'picture': 3687, 'focusing': 3688, 'maoists': 3689, 'atrocities': 3690, 'gunned': 3691, 'armored': 3692, 'type': 3693, 'motorcycle': 3694, 'easier': 3695, 'palace': 3696, 'shah': 3697, 'sunnis': 3698, 'unprecedented': 3699, 'freezing': 3700, 'mourning': 3701, 'ports': 3702, 'gunman': 3703, 'strict': 3704, 'aside': 3705, 'plea': 3706, 'sharif': 3707, 'opportunities': 3708, 'regarding': 3709, 'flag': 3710, 'refuge': 3711, 'qureia': 3712, 'tells': 3713, 'maker': 3714, 'iranians': 3715, 'damaging': 3716, 'mottaki': 3717, 'option': 3718, 'antonio': 3719, '160': 3720, 'broadcasts': 3721, 'computers': 3722, 'import': 3723, 'maritime': 3724, 'desire': 3725, 'critic': 3726, 'discrimination': 3727, 'traffickers': 3728, 'wfp': 3729, 'farming': 3730, 'lowest': 3731, 'contributions': 3732, 'cocoa': 3733, 'mountains': 3734, 'neighbor': 3735, 'representing': 3736, 'criticizing': 3737, 'profile': 3738, 'outskirts': 3739, '59': 3740, 'tanker': 3741, 'designated': 3742, 'maximum': 3743, '18th': 3744, 'hungarian': 3745, 'serbs': 3746, 'succeeded': 3747, 'friend': 3748, 'inauguration': 3749, 'welcome': 3750, 'unification': 3751, 'peshawar': 3752, 'am': 3753, 'triggering': 3754, 'motors': 3755, 'benefit': 3756, 'landing': 3757, 'warrant': 3758, 'algeria': 3759, 'here': 3760, 'promise': 3761, 'intensified': 3762, 'selected': 3763, 'baseball': 3764, 'hungary': 3765, 'landslide': 3766, 'outlawed': 3767, 'stocks': 3768, 'coverage': 3769, 'elaborate': 3770, 'physical': 3771, 'species': 3772, 'quetta': 3773, 'canal': 3774, 'sean': 3775, 'correspondent': 3776, 'formation': 3777, 'assist': 3778, 'county': 3779, 'sub': 3780, 'married': 3781, 'tech': 3782, 'belgrade': 3783, 'shopping': 3784, 'antarctic': 3785, 'meat': 3786, 'rushed': 3787, 'procedure': 3788, 'tragedy': 3789, 'concert': 3790, 'networks': 3791, 'narrow': 3792, 'piece': 3793, 'liberties': 3794, 'detainee': 3795, 'comply': 3796, 'harm': 3797, 'stranded': 3798, 'bbc': 3799, 'abduction': 3800, '88': 3801, 'spring': 3802, 'unmanned': 3803, 'promises': 3804, 'fleeing': 3805, 'wait': 3806, 'code': 3807, 'badly': 3808, 'nablus': 3809, 'dawn': 3810, 'warnings': 3811, 'percentage': 3812, 'hardest': 3813, 'storms': 3814, '56': 3815, 'rashid': 3816, 'flown': 3817, 'vital': 3818, 'marred': 3819, 'dissolved': 3820, 'whole': 3821, 'things': 3822, 'nazi': 3823, 'male': 3824, 'hopeful': 3825, 'mediterranean': 3826, '49': 3827, 'clothing': 3828, 'militiamen': 3829, 'stabilize': 3830, 'urge': 3831, 'lithuania': 3832, 'cape': 3833, 'luxembourg': 3834, '51': 3835, 'margin': 3836, 'shipping': 3837, 'andres': 3838, 'deby': 3839, 'represents': 3840, 'rita': 3841, 'fatalities': 3842, 'harry': 3843, 'stores': 3844, 'governmental': 3845, 'observed': 3846, 'bhutto': 3847, 'employee': 3848, 'restructuring': 3849, 'prompting': 3850, 'airports': 3851, 'reverse': 3852, 'zoellick': 3853, 'ones': 3854, 'fence': 3855, 'defending': 3856, 'ouster': 3857, 'laid': 3858, 'spill': 3859, 'isolation': 3860, 'rebellion': 3861, 'privatization': 3862, 'room': 3863, 'toxic': 3864, '47': 3865, 'wounds': 3866, 'weah': 3867, 'fatal': 3868, 'reactors': 3869, 'democrat': 3870, 'atmosphere': 3871, 'frontier': 3872, 'lashkar': 3873, '02': 3874, 'lawsuit': 3875, 'pollution': 3876, 'shabab': 3877, 'daniel': 3878, 'halted': 3879, 'fever': 3880, 'surged': 3881, 'dog': 3882, 'taipei': 3883, 'fail': 3884, 'approximately': 3885, 'fleet': 3886, 'argued': 3887, 'sick': 3888, 'koreas': 3889, 'drawn': 3890, 'khamenei': 3891, 'recruiting': 3892, 'crowds': 3893, 'ranked': 3894, 'wolf': 3895, 'voiced': 3896, 'task': 3897, 'everything': 3898, 'overwhelming': 3899, 'millennium': 3900, 'pledges': 3901, 'attributed': 3902, 'nationalist': 3903, 'campaigning': 3904, 'aims': 3905, 'lose': 3906, 'haniyeh': 3907, 'chicken': 3908, 'alongside': 3909, 'benjamin': 3910, 'machine': 3911, 'propelled': 3912, 'renew': 3913, 'maintaining': 3914, 'lavrov': 3915, 'rafah': 3916, 'motive': 3917, 'scientific': 3918, 'juan': 3919, '03': 3920, 'quarters': 3921, 'mississippi': 3922, 'lying': 3923, 'contractor': 3924, 'isolated': 3925, 'sparking': 3926, 'delays': 3927, 'mail': 3928, 'hear': 3929, 'values': 3930, 'punish': 3931, '1949': 3932, 'song': 3933, 'implemented': 3934, 'suspicious': 3935, 'shipments': 3936, 'quotas': 3937, 'counterparts': 3938, 'liberian': 3939, 'medal': 3940, 'aim': 3941, 'silence': 3942, 'suffer': 3943, 'potentially': 3944, 'variety': 3945, 'purported': 3946, 'roof': 3947, 'urban': 3948, 'coordination': 3949, 'oust': 3950, 'traders': 3951, 'threaten': 3952, 'deploy': 3953, 'aftermath': 3954, 'turmoil': 3955, 'volunteers': 3956, 'shanghai': 3957, 'symptoms': 3958, 'followers': 3959, 'strengthened': 3960, 'buses': 3961, 'reza': 3962, 'noting': 3963, 'businessman': 3964, 'scientist': 3965, 'seizure': 3966, 'exiled': 3967, 'relative': 3968, 'violated': 3969, 'vietnamese': 3970, 'museveni': 3971, 'landmark': 3972, 'evo': 3973, 'apart': 3974, 'colleagues': 3975, 'legislators': 3976, 'prayer': 3977, '78': 3978, 'reward': 3979, 'reid': 3980, 'elders': 3981, 'trees': 3982, 'sovereign': 3983, 'threatens': 3984, 'cultural': 3985, 'resulting': 3986, 'shan': 3987, 'passage': 3988, 'wage': 3989, 'severely': 3990, 'honduras': 3991, 'piracy': 3992, 'herald': 3993, 'watched': 3994, 'pick': 3995, 'license': 3996, 'cyclone': 3997, 'bangladeshi': 3998, 'burden': 3999, 'seeks': 4000, 'beef': 4001, 'marriage': 4002, 'owner': 4003, 'tension': 4004, 'monsoon': 4005, 'bolton': 4006, 'lahore': 4007, 'ancient': 4008, 'manila': 4009, 'cattle': 4010, 'jones': 4011, 'gonzales': 4012, 'behalf': 4013, 'awards': 4014, 'achieve': 4015, 'spot': 4016, 'smoking': 4017, 'benin': 4018, 'eighth': 4019, 'equal': 4020, 'wrongdoing': 4021, 'youths': 4022, 'restaurant': 4023, '11th': 4024, 'faith': 4025, 'rene': 4026, 'bian': 4027, 'travelers': 4028, 'sahara': 4029, 'islamists': 4030, 'dinner': 4031, 'diplomacy': 4032, 'carrier': 4033, 'version': 4034, 'classified': 4035, 'disperse': 4036, 'competing': 4037, 'zapatero': 4038, 'corp': 4039, 'commonwealth': 4040, 'brutal': 4041, '63': 4042, '1974': 4043, 'hemisphere': 4044, 'yasser': 4045, 'root': 4046, 'committing': 4047, 'solar': 4048, 'intends': 4049, 'rivers': 4050, 'advocates': 4051, 'upsurge': 4052, 'filled': 4053, 'consequences': 4054, 'blaming': 4055, '170': 4056, 'diyala': 4057, 'ninth': 4058, 'paktika': 4059, 'onto': 4060, 'absolute': 4061, 'starts': 4062, 'opponent': 4063, 'weakened': 4064, 'gadhafi': 4065, '77': 4066, 'feed': 4067, 'establishing': 4068, 'abusing': 4069, 'routes': 4070, 'addressing': 4071, 'practices': 4072, 'murders': 4073, 'tel': 4074, 'heroin': 4075, 'arroyo': 4076, 'offenses': 4077, 'cable': 4078, 'carbon': 4079, 'chadian': 4080, 'writing': 4081, 'condolences': 4082, 'fails': 4083, 'airspace': 4084, 'films': 4085, 'wreckage': 4086, 'felipe': 4087, 'doubt': 4088, 'conviction': 4089, 'amendments': 4090, 'balad': 4091, 'fact': 4092, 'propaganda': 4093, 'regularly': 4094, 'breaking': 4095, 'declare': 4096, 'hunter': 4097, 'counting': 4098, 'factories': 4099, 'damages': 4100, 'contingent': 4101, 'shwe': 4102, 'covering': 4103, 'battles': 4104, 'sydney': 4105, 'extradited': 4106, 'laundering': 4107, 'toppled': 4108, 'larijani': 4109, 'confiscated': 4110, 'outstanding': 4111, '72': 4112, 'possession': 4113, 'overcome': 4114, 'melbourne': 4115, 'mentioned': 4116, 'mean': 4117, 'mediators': 4118, 'particular': 4119, 'rating': 4120, 'karen': 4121, 'referring': 4122, 'greenspan': 4123, 'regulators': 4124, 'tribesmen': 4125, 'everyone': 4126, 'yemeni': 4127, 'acted': 4128, 'contracts': 4129, 'paraguay': 4130, 'conversion': 4131, 'dry': 4132, 'fueled': 4133, 'executives': 4134, 'interrogators': 4135, 'actress': 4136, 'thanksgiving': 4137, 'jumped': 4138, 'evacuation': 4139, 'professional': 4140, 'romanian': 4141, 'individual': 4142, 'retail': 4143, 'ford': 4144, 'aden': 4145, 'buying': 4146, 'assured': 4147, 'finish': 4148, 'abortion': 4149, 'lifting': 4150, '900': 4151, 'recommendations': 4152, 'engage': 4153, 'participants': 4154, 'ballot': 4155, 'healthy': 4156, 'diagnosed': 4157, 'understanding': 4158, 'offers': 4159, 'malaysian': 4160, 'prosperous': 4161, 'handled': 4162, 'awareness': 4163, 'ballistic': 4164, 'ossetia': 4165, 'pose': 4166, 'studies': 4167, 'specifically': 4168, 'championships': 4169, 'matches': 4170, 'coordinate': 4171, 'doping': 4172, 'restoring': 4173, 'seize': 4174, 'forecast': 4175, 'gather': 4176, 'wind': 4177, 'notorious': 4178, 'founding': 4179, 'peacefully': 4180, 'checkpoints': 4181, 'herat': 4182, 'worshippers': 4183, 'investigations': 4184, 'provisions': 4185, 'inter': 4186, 'crack': 4187, 'curfew': 4188, 'basque': 4189, 'granting': 4190, '1976': 4191, 'heat': 4192, 'album': 4193, 'establishment': 4194, 'shootings': 4195, 'davis': 4196, 'god': 4197, 'lands': 4198, 'letters': 4199, 'producers': 4200, 'hampered': 4201, 'beneath': 4202, 'sirleaf': 4203, 'kabila': 4204, 'ignored': 4205, 'dumped': 4206, 'fans': 4207, 'laureate': 4208, 'inacio': 4209, 'lula': 4210, 'reaction': 4211, 'dominant': 4212, 'renounce': 4213, 'petition': 4214, 'barrier': 4215, 'except': 4216, 'coffee': 4217, 'africans': 4218, 'organizing': 4219, 'essential': 4220, 'deteriorating': 4221, 'aviv': 4222, 'preparations': 4223, 'scotland': 4224, 'exist': 4225, 'abused': 4226, 'branch': 4227, 'properly': 4228, 'quick': 4229, 'dramatically': 4230, 'partial': 4231, 'slain': 4232, 'express': 4233, 'mourners': 4234, 'easing': 4235, 'ben': 4236, 'assassinated': 4237, 'appointment': 4238, 'calderon': 4239, 'virginia': 4240, 'transporting': 4241, 'defendant': 4242, 'asif': 4243, 'birthday': 4244, 'attached': 4245, 'dominican': 4246, 'participating': 4247, 'uses': 4248, 'influenza': 4249, 'note': 4250, 'bp': 4251, 'escalating': 4252, 'hailed': 4253, 'kuchma': 4254, 'suburb': 4255, 'v': 4256, 'trains': 4257, 'repair': 4258, 'archbishop': 4259, 'albanian': 4260, 'audience': 4261, 'register': 4262, 'appoint': 4263, 'anderson': 4264, 'patrols': 4265, 'provisional': 4266, 'users': 4267, 'challenged': 4268, 'extremism': 4269, 'communication': 4270, 'file': 4271, 'something': 4272, 'aware': 4273, 'owners': 4274, 'recalled': 4275, 'luxury': 4276, 'actually': 4277, 'estate': 4278, 'behavior': 4279, 'ducks': 4280, 'exposed': 4281, 'emirates': 4282, 'kidnap': 4283, 'culled': 4284, 'kevin': 4285, 'actor': 4286, 'ramirez': 4287, 'tunnels': 4288, 'admiral': 4289, 'dependence': 4290, 'zuma': 4291, '54': 4292, 'asean': 4293, 'dismantle': 4294, 'sealed': 4295, 'orakzai': 4296, 'greatest': 4297, 'mehlis': 4298, 'tiny': 4299, 'weight': 4300, 'listed': 4301, 'stake': 4302, 'alan': 4303, 'permit': 4304, 'prove': 4305, 'evacuate': 4306, 'recognizes': 4307, 'locations': 4308, 'stones': 4309, 'ratified': 4310, 'smugglers': 4311, 'losing': 4312, 'searched': 4313, 'lake': 4314, 'fort': 4315, 'expire': 4316, 'requests': 4317, 'shui': 4318, 'imposing': 4319, 'samarra': 4320, 'enrich': 4321, 'nationalities': 4322, 'implementation': 4323, 'fewer': 4324, 'purchased': 4325, 'dujail': 4326, 'tear': 4327, 'crop': 4328, 'shore': 4329, 'electric': 4330, 'murdered': 4331, 'interference': 4332, 'wilma': 4333, 'ravaged': 4334, 'challenger': 4335, 'delaying': 4336, 'moqtada': 4337, 'treating': 4338, 'herself': 4339, 'procedures': 4340, 'kyrgyz': 4341, 'objections': 4342, 'negotiating': 4343, 'midnight': 4344, 'chanted': 4345, 'check': 4346, 'luiz': 4347, 'convention': 4348, 'baidoa': 4349, 'somalis': 4350, 'steady': 4351, 'deposits': 4352, 'w': 4353, 'laurent': 4354, 'francisco': 4355, 'decree': 4356, 'conflicting': 4357, 'expressing': 4358, 'shadow': 4359, 'slaughtered': 4360, 'famine': 4361, 'inventories': 4362, 'weaken': 4363, 'publication': 4364, 'category': 4365, 'platform': 4366, 'businessmen': 4367, 'expelled': 4368, 'academy': 4369, 'inspector': 4370, 'frank': 4371, 'stepping': 4372, '1962': 4373, 'slovenia': 4374, '15th': 4375, 'firefight': 4376, 'implicated': 4377, 'rio': 4378, 'electrical': 4379, 'deliveries': 4380, 'assess': 4381, 'hindu': 4382, 'smoke': 4383, 'infections': 4384, '60th': 4385, 'prepares': 4386, 'logistical': 4387, 'ranging': 4388, 'sexually': 4389, 'drivers': 4390, 'mil': 4391, 'arcega': 4392, 'annexed': 4393, 'ranks': 4394, 'solid': 4395, 'waste': 4396, 'risks': 4397, 'scheffer': 4398, 'burns': 4399, 'houston': 4400, 'requirements': 4401, 'bangkok': 4402, 'privately': 4403, 'correa': 4404, 'footage': 4405, 'beaten': 4406, 'interfax': 4407, 'warsaw': 4408, '84': 4409, 'interviews': 4410, 'villepin': 4411, 'atlanta': 4412, 'wealthy': 4413, 'bloomberg': 4414, 'hike': 4415, 'surveyed': 4416, 'dressed': 4417, '81': 4418, 'yousuf': 4419, 'equality': 4420, 'kilograms': 4421, 'resumption': 4422, 'predominantly': 4423, 'promising': 4424, 'sister': 4425, 'beach': 4426, 'marburg': 4427, 'jim': 4428, 'secession': 4429, 'priest': 4430, 'incursion': 4431, 'finals': 4432, 'recording': 4433, 'reunification': 4434, 'cache': 4435, 'gedi': 4436, 'chiefs': 4437, 'protocol': 4438, 'whales': 4439, 'reopen': 4440, 'carriles': 4441, 'amounts': 4442, 'hare': 4443, 'guangdong': 4444, 'undersecretary': 4445, 'stationed': 4446, 'appealing': 4447, 'disrupting': 4448, 'gradually': 4449, 'verdict': 4450, 'apartheid': 4451, 'produces': 4452, 'negroponte': 4453, 'liberal': 4454, 'boom': 4455, 'gunships': 4456, 'crews': 4457, 'condemning': 4458, 'geological': 4459, 'earthquakes': 4460, 'deemed': 4461, 'simply': 4462, 'bolster': 4463, 'overthrew': 4464, 'approached': 4465, 'fraudulent': 4466, 'bans': 4467, 'chose': 4468, 'unanimously': 4469, 'rammed': 4470, 'ioc': 4471, 'implementing': 4472, 'quota': 4473, 'permits': 4474, '61': 4475, '1973': 4476, 'lord': 4477, 'software': 4478, 'diversify': 4479, 'page': 4480, 'restricted': 4481, 'rioting': 4482, 'la': 4483, 'ownership': 4484, 'depend': 4485, 'structural': 4486, 'quiet': 4487, 'recovering': 4488, 'cambodian': 4489, 'dedicated': 4490, 'bernard': 4491, 'manouchehr': 4492, 'thanks': 4493, 'mediate': 4494, 'lacks': 4495, 'windows': 4496, 'akayev': 4497, 'sierra': 4498, 'leone': 4499, 'stamp': 4500, 'contacts': 4501, 'biden': 4502, 'accompanied': 4503, 'sabotage': 4504, 'posed': 4505, 'happy': 4506, 'throwing': 4507, 'circumstances': 4508, 'kaczynski': 4509, 'inc': 4510, '10th': 4511, 'adequate': 4512, '1986': 4513, 'trips': 4514, 'sons': 4515, 'refining': 4516, 'amman': 4517, 'rocks': 4518, 'irna': 4519, 'greenhouse': 4520, 'judiciary': 4521, 'lab': 4522, 'celebration': 4523, 'controversy': 4524, 'khaled': 4525, 'shaukat': 4526, 'accidentally': 4527, 'degrees': 4528, 'suspending': 4529, 'efficient': 4530, 'khyber': 4531, 'difficulties': 4532, 'stephen': 4533, 'yesterday': 4534, 'pension': 4535, 'bit': 4536, 'predict': 4537, 'li': 4538, 'apologized': 4539, 'drafting': 4540, 'populated': 4541, 'welfare': 4542, 'brothers': 4543, 'nominees': 4544, 'blown': 4545, 'hosted': 4546, 'routine': 4547, 'else': 4548, 'resuming': 4549, 'suit': 4550, '1980': 4551, 'firefighters': 4552, 'surplus': 4553, 'rafael': 4554, 'gul': 4555, 'predecessor': 4556, 'proof': 4557, 'adviser': 4558, 'paramilitaries': 4559, 'pushing': 4560, 'eat': 4561, 'administered': 4562, 'cancel': 4563, 'stolen': 4564, 'entrance': 4565, 'steel': 4566, 'gilani': 4567, 'eliminate': 4568, 'substantially': 4569, 'mutharika': 4570, 'sport': 4571, 'hanoi': 4572, 'awaiting': 4573, 'enacted': 4574, 'karadzic': 4575, 'traditionally': 4576, 'bob': 4577, 'expert': 4578, 'monthly': 4579, 'lasted': 4580, 'card': 4581, 'pending': 4582, 'oman': 4583, 'answer': 4584, 'shield': 4585, 'posada': 4586, 'frozen': 4587, 'intentions': 4588, 'zambia': 4589, 'reaffirmed': 4590, 'spin': 4591, 'enjoy': 4592, 'younger': 4593, 'g8': 4594, 'gaining': 4595, 'bengal': 4596, '1975': 4597, 'lethal': 4598, 'scandals': 4599, 'fishermen': 4600, 'mccormack': 4601, 'trillion': 4602, 'true': 4603, 'veterinary': 4604, 'dismiss': 4605, 'intercepted': 4606, 'macau': 4607, 'apec': 4608, 'apply': 4609, 'tense': 4610, 'spacecraft': 4611, 'mwai': 4612, 'detailed': 4613, 'rulers': 4614, 'factor': 4615, 'jill': 4616, 'cooperating': 4617, 'grants': 4618, 'pieces': 4619, 'haven': 4620, 'direction': 4621, 'battled': 4622, 'indication': 4623, 'manufactured': 4624, 'councils': 4625, 'duma': 4626, 'spotted': 4627, 'gesture': 4628, 'carol': 4629, 'landfall': 4630, 'learned': 4631, 'connected': 4632, 'effectively': 4633, 'transmission': 4634, 'imam': 4635, 'reopened': 4636, 'sisco': 4637, 'dissident': 4638, 'shells': 4639, 'destabilize': 4640, 'narrowly': 4641, 'interpreter': 4642, 'auction': 4643, 'maintains': 4644, 'gotovina': 4645, '1960s': 4646, 'slaves': 4647, 'rely': 4648, 'describes': 4649, 'online': 4650, 'freedoms': 4651, 'depression': 4652, 'intervention': 4653, 'exhibition': 4654, 'interfering': 4655, 'display': 4656, 'confrontation': 4657, 'rifles': 4658, 'shoot': 4659, 'bogota': 4660, 'bethlehem': 4661, 'treason': 4662, 'catholics': 4663, 'love': 4664, 'kuwaiti': 4665, 'younis': 4666, 'deficits': 4667, 'attracting': 4668, 'transparency': 4669, 'candidacy': 4670, 'ton': 4671, 'consideration': 4672, 'worries': 4673, 'represented': 4674, 'drilling': 4675, 'cameroon': 4676, 'verified': 4677, 'arm': 4678, 'decreased': 4679, 'publishing': 4680, '110': 4681, 'jersey': 4682, 'submit': 4683, 'nationality': 4684, '62': 4685, 'chemicals': 4686, 'arresting': 4687, 'optimistic': 4688, 'tore': 4689, 'proper': 4690, 'consumption': 4691, 'andrew': 4692, 'mitchell': 4693, 'wen': 4694, 'downtown': 4695, 'coca': 4696, 'spark': 4697, 'progressive': 4698, 'donated': 4699, 'tobacco': 4700, '350': 4701, 'capabilities': 4702, 'employed': 4703, 'abuja': 4704, 'eye': 4705, 'myanmar': 4706, 'fixed': 4707, 'alleging': 4708, 'stops': 4709, '1987': 4710, 'product': 4711, 'johannesburg': 4712, 'gabon': 4713, 'unlikely': 4714, 'copper': 4715, 'jeddah': 4716, 'hoop': 4717, 'sense': 4718, 'celebrating': 4719, 'basketball': 4720, 'steadily': 4721, 'airbus': 4722, 'cope': 4723, 'outrage': 4724, 'margaret': 4725, 'takeover': 4726, 'unilateral': 4727, 'dominique': 4728, 'bodyguard': 4729, 'khin': 4730, 'lady': 4731, 'kenyans': 4732, 'revised': 4733, 'unfair': 4734, 'flags': 4735, 'eliminated': 4736, 'adam': 4737, 'championship': 4738, 'germans': 4739, 'eritrean': 4740, 'stricken': 4741, 'wildlife': 4742, 'releasing': 4743, 'santiago': 4744, 'massachusetts': 4745, 'observe': 4746, 'mutate': 4747, 'akbar': 4748, 'riyadh': 4749, 'guidelines': 4750, 'xvi': 4751, 'puts': 4752, 'venture': 4753, 'priests': 4754, 'forms': 4755, 'strongholds': 4756, 'lawsuits': 4757, 'miller': 4758, 'roddick': 4759, 'succession': 4760, 'derail': 4761, 'kyoto': 4762, 'notice': 4763, 'fees': 4764, 'flash': 4765, 'bakiyev': 4766, 'eggs': 4767, 'tung': 4768, 'iowa': 4769, 'hideouts': 4770, 'investigator': 4771, 'washed': 4772, 'mention': 4773, 'musician': 4774, 'abductors': 4775, 'pradesh': 4776, 'invest': 4777, 'monitored': 4778, 'ambitious': 4779, 'mails': 4780, 'diamond': 4781, 'factional': 4782, 'integrity': 4783, 'chain': 4784, 'commodities': 4785, 'stages': 4786, 'aging': 4787, 'kamal': 4788, 'veteran': 4789, 'monks': 4790, 'tibetans': 4791, 'diabetes': 4792, 'pearson': 4793, 'balkan': 4794, '04': 4795, 'sweep': 4796, 'treat': 4797, 'coach': 4798, 'existence': 4799, 'bar': 4800, 'jericho': 4801, '83': 4802, '82': 4803, 'descent': 4804, 'tajikistan': 4805, 'hair': 4806, 'cites': 4807, 'commitments': 4808, 'obtain': 4809, 'columbus': 4810, 'marxist': 4811, 'persons': 4812, 'extreme': 4813, 'employs': 4814, 'examine': 4815, 'yusuf': 4816, 'minorities': 4817, 'consists': 4818, 'ashore': 4819, '69': 4820, 'engineer': 4821, 'burkina': 4822, 'faso': 4823, 'contaminated': 4824, 'phones': 4825, 'ordering': 4826, 'exact': 4827, 'poisoned': 4828, 'settle': 4829, 'sharm': 4830, 'crush': 4831, 'reading': 4832, 'estonia': 4833, 'characterized': 4834, 'addis': 4835, 'explain': 4836, 'commit': 4837, 'retain': 4838, 'postpone': 4839, 'adults': 4840, 'plus': 4841, 'simon': 4842, 'federalism': 4843, '275': 4844, 'overseeing': 4845, 'albanians': 4846, 'pray': 4847, 'lot': 4848, 'saharan': 4849, 'mbeki': 4850, 'eln': 4851, 'bitter': 4852, 'struggled': 4853, 'afar': 4854, 'pulling': 4855, 'professionals': 4856, 'emile': 4857, 'bernanke': 4858, 'asefi': 4859, 'horn': 4860, 'complaint': 4861, 'features': 4862, 'h': 4863, 'turks': 4864, 'ceded': 4865, 'ottoman': 4866, 'contested': 4867, 'mouth': 4868, 'quell': 4869, 'javier': 4870, 'barre': 4871, 'prevention': 4872, 'incoming': 4873, 'elements': 4874, 'exit': 4875, 'encountered': 4876, 'purpose': 4877, 'remnants': 4878, 'smuggled': 4879, 'abductions': 4880, 'tents': 4881, 'najaf': 4882, 'republics': 4883, 'westerners': 4884, 'destination': 4885, 'volcano': 4886, 'rebounded': 4887, 'operational': 4888, 'attract': 4889, 'belgian': 4890, 'financing': 4891, 'tutsis': 4892, 'rangel': 4893, 'locked': 4894, 'rightist': 4895, 'epidemic': 4896, 'minibus': 4897, 'libby': 4898, 'economist': 4899, 'topics': 4900, 'masri': 4901, 'dmitri': 4902, 'argue': 4903, 'legitimate': 4904, 'consensus': 4905, 'sufficient': 4906, 'egyptians': 4907, 'homemade': 4908, 'encouraging': 4909, 'minsk': 4910, 'rioters': 4911, 'door': 4912, 'raza': 4913, 'cleveland': 4914, 'doubles': 4915, 'singles': 4916, 'cholera': 4917, 'tariffs': 4918, 'plastic': 4919, 'justices': 4920, 'ebola': 4921, 'stance': 4922, 'authorizing': 4923, 'liechtenstein': 4924, '1985': 4925, 'staffers': 4926, 'audio': 4927, 'operative': 4928, 'dayton': 4929, 'koran': 4930, 'immigrant': 4931, 'metal': 4932, 'stone': 4933, 'isaf': 4934, 'learn': 4935, 'mozambique': 4936, 'investor': 4937, 'artist': 4938, 'telling': 4939, 'opposing': 4940, 'administrator': 4941, 'upheld': 4942, 'via': 4943, 'cubic': 4944, 'knowledge': 4945, 'discussion': 4946, 'maintenance': 4947, 'initiatives': 4948, 'repression': 4949, 'informal': 4950, '67': 4951, 'gordon': 4952, 'sinai': 4953, 'berlusconi': 4954, 'captivity': 4955, 'landslides': 4956, 'articles': 4957, 'laos': 4958, 'bachelet': 4959, 'obrador': 4960, 'enriching': 4961, 'throne': 4962, 'client': 4963, 'ruler': 4964, 'illinois': 4965, 'bills': 4966, 'namibia': 4967, 'catch': 4968, 'mike': 4969, 'peretz': 4970, 'lasting': 4971, 'cypriots': 4972, 'kadima': 4973, 'besigye': 4974, 'pounded': 4975, 'strife': 4976, 'residential': 4977, 'desert': 4978, 'hectares': 4979, 'concrete': 4980, 'kidney': 4981, 'timber': 4982, 'macroeconomic': 4983, 'secured': 4984, 'pound': 4985, 'portions': 4986, 'corrupt': 4987, 'conclusion': 4988, 'brian': 4989, 'narrates': 4990, 'slowing': 4991, 'books': 4992, 'barriers': 4993, 'owns': 4994, 'sporadic': 4995, 'assume': 4996, 'cells': 4997, 'suddenly': 4998, 'bulk': 4999, 'veterans': 5000, 'musical': 5001, 'performing': 5002, 'emperor': 5003, 'warm': 5004, 'governors': 5005, 'j': 5006, 'facto': 5007, 'chris': 5008, 'universal': 5009, 'peak': 5010, 'martial': 5011, 'maryland': 5012, 'tip': 5013, 'patrolling': 5014, 'osman': 5015, 'watchdog': 5016, 'adopt': 5017, 'commodity': 5018, 'acquisition': 5019, '1983': 5020, 'drowned': 5021, 'tulkarem': 5022, 'restart': 5023, 'aggression': 5024, 'planting': 5025, 'manager': 5026, 'orbiting': 5027, 'joe': 5028, 'removing': 5029, 'jamaica': 5030, 'croat': 5031, 'willingness': 5032, 'remark': 5033, 'add': 5034, 'ellen': 5035, 'salaries': 5036, 'overturned': 5037, 'silver': 5038, 'medals': 5039, 'downhill': 5040, '93': 5041, 'supplied': 5042, 'albania': 5043, 'example': 5044, 'specify': 5045, 'masked': 5046, 'congratulated': 5047, 'thinks': 5048, 'ababa': 5049, 'promotion': 5050, 'framework': 5051, 'associate': 5052, 'oath': 5053, 'sayyaf': 5054, 'ailing': 5055, '1945': 5056, 'plots': 5057, 'components': 5058, 'dominate': 5059, 'diamonds': 5060, 'engine': 5061, 'ouattara': 5062, 'challenging': 5063, 'armenia': 5064, 'ricardo': 5065, 'adjourned': 5066, 'pipelines': 5067, 'pain': 5068, 'shelters': 5069, 'chaudhry': 5070, 'defuse': 5071, 'mofaz': 5072, 'nangarhar': 5073, 'tenths': 5074, 'advised': 5075, 'airliner': 5076, 'installations': 5077, 'secrets': 5078, 'multinational': 5079, 'horse': 5080, 'iron': 5081, 'fill': 5082, 'kinshasa': 5083, 'janeiro': 5084, 'inspection': 5085, 'roh': 5086, 'restaurants': 5087, 'window': 5088, 'shift': 5089, 'blaze': 5090, 'suffers': 5091, 'elderly': 5092, 'origin': 5093, 'crossings': 5094, 'approaching': 5095, 'studying': 5096, 'clothes': 5097, 'polar': 5098, 'negative': 5099, 'tandja': 5100, 'leonid': 5101, 'landmine': 5102, 'spurred': 5103, 'moussa': 5104, 'fines': 5105, 'twin': 5106, 'aso': 5107, 'restrict': 5108, 'ambassadors': 5109, 'siege': 5110, 'invitation': 5111, 'revolt': 5112, 'archipelago': 5113, 'cotton': 5114, 'sustain': 5115, 'statistics': 5116, 'unified': 5117, 'poorly': 5118, 'simple': 5119, 'athens': 5120, 'generated': 5121, 'confessed': 5122, 'condemns': 5123, 'jump': 5124, 'bears': 5125, 'arctic': 5126, 'bear': 5127, 'bribes': 5128, 'laborers': 5129, '180': 5130, 'slaughter': 5131, 'distance': 5132, 'participated': 5133, 'faster': 5134, 'drives': 5135, 'tal': 5136, 'assessment': 5137, 'apology': 5138, 'intestinal': 5139, 'tortured': 5140, 'dictatorship': 5141, 'brigade': 5142, 'transmitted': 5143, 'confident': 5144, 'extradite': 5145, 'janjaweed': 5146, 'popularity': 5147, 'garden': 5148, 'colonial': 5149, 'resource': 5150, 'triple': 5151, 'supposed': 5152, 'seychelles': 5153, 'fisherman': 5154, 'whaling': 5155, 'santa': 5156, 'breaks': 5157, 'meantime': 5158, 'customers': 5159, 'canadians': 5160, 'sao': 5161, 'relies': 5162, 'semifinal': 5163, 'oversee': 5164, 'monopoly': 5165, 'hingis': 5166, 'favored': 5167, 'incumbent': 5168, 'riding': 5169, 'junior': 5170, '13th': 5171, 'hunters': 5172, 'envoys': 5173, 'edition': 5174, '1981': 5175, 'recruits': 5176, 'basayev': 5177, 'recommendation': 5178, 'collected': 5179, 'parked': 5180, 'reference': 5181, 'raw': 5182, 'expression': 5183, 'mongolia': 5184, 'wood': 5185, 'registration': 5186, 'cow': 5187, 'mir': 5188, 'mousavi': 5189, 'inspections': 5190, 'goose': 5191, 'representation': 5192, 'chanting': 5193, 'wins': 5194, 'professor': 5195, 'wilson': 5196, 'mandela': 5197, 'malta': 5198, 'wal': 5199, 'egeland': 5200, 'describe': 5201, 'sumatra': 5202, 'spare': 5203, 'punished': 5204, 'waging': 5205, 'shimon': 5206, 'tally': 5207, 'ski': 5208, 'dying': 5209, 'separated': 5210, 'protectorate': 5211, 'landlocked': 5212, 'sitting': 5213, 'shop': 5214, 'criticize': 5215, 'reveal': 5216, 'empty': 5217, 'wartime': 5218, 'doubled': 5219, 'wheat': 5220, 'loaded': 5221, 'detentions': 5222, 'managing': 5223, 'advancing': 5224, 'appropriate': 5225, 'athletes': 5226, 'preparation': 5227, 'skills': 5228, 'taiwanese': 5229, 'slammed': 5230, 'battered': 5231, 'eventual': 5232, 'warrants': 5233, 'oldest': 5234, 'stemming': 5235, 'pointed': 5236, 'bulgarian': 5237, 'palestine': 5238, 'cartoon': 5239, 'invaded': 5240, 'roger': 5241, 'accounting': 5242, 'ronald': 5243, 'medium': 5244, 'indians': 5245, 'oriented': 5246, 'hurled': 5247, 'lunar': 5248, 'excessive': 5249, 'lieberman': 5250, 'broadcaster': 5251, 'temple': 5252, 'departure': 5253, 'divide': 5254, 'mideast': 5255, 'capsized': 5256, 'dhaka': 5257, 'sailors': 5258, '220': 5259, 'remember': 5260, 'ratify': 5261, 'designate': 5262, 'reviewing': 5263, 'contains': 5264, 'pledging': 5265, 'papers': 5266, 'screen': 5267, 'watches': 5268, 'pair': 5269, 'askar': 5270, 'harmful': 5271, 'keys': 5272, 'partly': 5273, 'mastermind': 5274, 'barroso': 5275, 'shelling': 5276, 'stealing': 5277, 'travels': 5278, 'samuel': 5279, 'floor': 5280, 'bolivar': 5281, 'considerable': 5282, 'reliance': 5283, 'rail': 5284, 'sergeant': 5285, 'replacement': 5286, 'refusing': 5287, 'belong': 5288, 'longest': 5289, 'dan': 5290, 'eased': 5291, 'gloria': 5292, 'ukrainians': 5293, 'supporter': 5294, '68': 5295, 'latortue': 5296, 'baltic': 5297, 'table': 5298, 'enforce': 5299, 'cartels': 5300, '105': 5301, 'matters': 5302, 'tycoon': 5303, 'write': 5304, 'repeat': 5305, 'guardian': 5306, 'croats': 5307, 'harbor': 5308, 'tankers': 5309, 'kills': 5310, 'summoned': 5311, 'theater': 5312, 'farah': 5313, 'gm': 5314, 'negotiated': 5315, 'chair': 5316, 'infighting': 5317, 'khalid': 5318, 'phoenix': 5319, 'hide': 5320, 'nevertheless': 5321, 'independently': 5322, 'hosts': 5323, 'improvement': 5324, 'jaap': 5325, 'agassi': 5326, 'federer': 5327, 'hanging': 5328, 'row': 5329, 'bagram': 5330, 'unique': 5331, 'sam': 5332, 'zebari': 5333, 'rotating': 5334, 'lists': 5335, 'strongest': 5336, 'charging': 5337, 'identities': 5338, 'minimum': 5339, 'flowing': 5340, 'democratically': 5341, 'placing': 5342, 'truth': 5343, 'sanitation': 5344, 'outcome': 5345, 'olusegun': 5346, 'distributing': 5347, 'grain': 5348, 'accusation': 5349, 'affiliated': 5350, 'delivering': 5351, 'bankruptcy': 5352, 'jurisdiction': 5353, 'model': 5354, 'crow': 5355, '79': 5356, 'shook': 5357, 'sees': 5358, 'unicef': 5359, 'consultations': 5360, 'outpost': 5361, 'mladic': 5362, 'patient': 5363, 'drink': 5364, 'dna': 5365, 'innings': 5366, 'rafsanjani': 5367, 'observing': 5368, 'hidden': 5369, 'auto': 5370, 'install': 5371, 'nationalists': 5372, 'acquitted': 5373, 'improvements': 5374, 'marino': 5375, 'thus': 5376, 'principle': 5377, 'fame': 5378, 'topple': 5379, 'populations': 5380, 'facebook': 5381, 'marie': 5382, 'boldak': 5383, 'uniforms': 5384, 'expensive': 5385, 'dump': 5386, 'flock': 5387, 'heightened': 5388, '87': 5389, 'slight': 5390, 'stood': 5391, 'clinic': 5392, 'mwanawasa': 5393, 'mosques': 5394, 'governance': 5395, 'fastest': 5396, 'referral': 5397, 'chamber': 5398, 'yekhanurov': 5399, 'kagame': 5400, 'logistics': 5401, 'yonhap': 5402, '1947': 5403, 'contraction': 5404, 'tbilisi': 5405, 'metric': 5406, 'multilateral': 5407, 'fertilizer': 5408, 'supplying': 5409, 'dow': 5410, 'baby': 5411, 'movements': 5412, 'hossein': 5413, 'rejects': 5414, 'roche': 5415, 'expired': 5416, 'allege': 5417, 'wickets': 5418, 'tighten': 5419, 'monument': 5420, 'walls': 5421, 'peoples': 5422, 'ohio': 5423, 'feet': 5424, 'mart': 5425, 'coordinator': 5426, 'chambers': 5427, 'burst': 5428, 'associates': 5429, 'demonstrate': 5430, 'fit': 5431, 'bars': 5432, 'courtroom': 5433, 'casting': 5434, 'ray': 5435, 'amir': 5436, 'jaffna': 5437, 'breast': 5438, 'twitter': 5439, 'coffin': 5440, 'inspired': 5441, 'satellites': 5442, 'reinstated': 5443, 'obstacles': 5444, 'finds': 5445, '71': 5446, 'stayed': 5447, 'diesel': 5448, 'lhasa': 5449, 'helps': 5450, 'walter': 5451, 'disarming': 5452, 'speeches': 5453, 'normally': 5454, 'strait': 5455, 'persuade': 5456, 'bloodshed': 5457, 'highways': 5458, 'lra': 5459, 'billionaire': 5460, 'guest': 5461, 'airliners': 5462, 'foiled': 5463, 'shareholders': 5464, 'dismantling': 5465, 'proved': 5466, 'unsuccessful': 5467, 'combination': 5468, 'jointly': 5469, 'mineral': 5470, 'hampering': 5471, 'simultaneous': 5472, 'talking': 5473, 'mind': 5474, 'intel': 5475, 'crown': 5476, 'jammu': 5477, 'instruments': 5478, 'catastrophe': 5479, 'rouge': 5480, 'situations': 5481, 'cbs': 5482, 'occasion': 5483, 'sacrifice': 5484, 'muzaffarabad': 5485, 'hosting': 5486, 'retailers': 5487, 'doubts': 5488, 'touch': 5489, 'handing': 5490, 'orange': 5491, 'hebron': 5492, 'bronze': 5493, 'surfaced': 5494, 'older': 5495, 'error': 5496, 'elder': 5497, 'conversation': 5498, 'karimov': 5499, 'indirect': 5500, 'guarantees': 5501, 'protected': 5502, 'drones': 5503, 'devastation': 5504, 'storage': 5505, 'winners': 5506, 'signature': 5507, 'sole': 5508, 'advocacy': 5509, 'guaranteed': 5510, 'portuguese': 5511, 'offset': 5512, 'generation': 5513, 'compact': 5514, 'fragile': 5515, 'kerry': 5516, 'embattled': 5517, 'yield': 5518, 'dispatched': 5519, 'strasbourg': 5520, 'engineering': 5521, 'intervene': 5522, 'deadlock': 5523, 'object': 5524, 'idriss': 5525, 'flames': 5526, 'hybrid': 5527, 'tougher': 5528, 'railway': 5529, 'exposure': 5530, 'dismissal': 5531, 'aligned': 5532, 'persistent': 5533, 'itar': 5534, 'tass': 5535, 'slobodan': 5536, '05': 5537, 'trend': 5538, 'bahamas': 5539, 'alleges': 5540, 'content': 5541, 'rehabilitation': 5542, 'celsius': 5543, 'afford': 5544, 'wrapped': 5545, 'bicycle': 5546, 'centrifuges': 5547, 'togolese': 5548, 'alpha': 5549, 'mistakenly': 5550, 'controlling': 5551, 'personally': 5552, 'curfews': 5553, 'yoweri': 5554, '12th': 5555, 'divisions': 5556, 'gunfight': 5557, 'siad': 5558, 'searches': 5559, 'hub': 5560, 'inspect': 5561, 'saad': 5562, 'kicked': 5563, 'motorcade': 5564, 'absence': 5565, 'gate': 5566, 'suggests': 5567, 'convert': 5568, 'critically': 5569, 'blocks': 5570, 'multiparty': 5571, 'faithful': 5572, 'hometown': 5573, 'machinery': 5574, 'anything': 5575, 'convoys': 5576, 'bag': 5577, 'queen': 5578, 'pool': 5579, 'nicholas': 5580, 'hubble': 5581, 'uncertain': 5582, 'dance': 5583, 'hikes': 5584, 'beslan': 5585, 'spies': 5586, 'puerto': 5587, 'jenin': 5588, 'respects': 5589, 'teenagers': 5590, 'casualty': 5591, 'coincide': 5592, 'nld': 5593, 'odds': 5594, 'entirely': 5595, 'contest': 5596, 'advisors': 5597, 'greeted': 5598, 'boris': 5599, 'tadic': 5600, 'fined': 5601, 'goodwill': 5602, 'endangered': 5603, 'wiped': 5604, 'productivity': 5605, 'steep': 5606, 'tripoli': 5607, 'commandos': 5608, 'suburbs': 5609, 'tigers': 5610, 'operates': 5611, 'swan': 5612, 'jonathan': 5613, 'steve': 5614, 'imprisonment': 5615, 'schwarzenegger': 5616, 'chicago': 5617, 'uige': 5618, 'enemies': 5619, 'suggesting': 5620, 'preferred': 5621, 'modified': 5622, 'mediator': 5623, 'credible': 5624, 'dubbed': 5625, 'subsidiary': 5626, 'drill': 5627, 'exclusive': 5628, 'abdullahi': 5629, 'coma': 5630, 'musa': 5631, 'outlets': 5632, 'reflected': 5633, 'printed': 5634, 'waged': 5635, 'rahman': 5636, 'mortgage': 5637, 'staging': 5638, 'punishment': 5639, 'hardline': 5640, 'heating': 5641, 'credibility': 5642, 'prosecute': 5643, 'rocked': 5644, 'directors': 5645, 'victor': 5646, 'rounded': 5647, 'semifinals': 5648, 'undergoing': 5649, 'aiding': 5650, 'poppy': 5651, 'expectations': 5652, 'honest': 5653, 'belt': 5654, 'uniform': 5655, 'tactics': 5656, 'louis': 5657, 'wali': 5658, 'stars': 5659, 'presents': 5660, 'stalemate': 5661, 'attorneys': 5662, 'selection': 5663, 'gutierrez': 5664, 'fashion': 5665, 'core': 5666, 'nagin': 5667, 'routinely': 5668, 'fake': 5669, 'moldova': 5670, 'quite': 5671, 'shepherd': 5672, 'eliminating': 5673, 'spacewalk': 5674, 'gore': 5675, 'confront': 5676, 'deported': 5677, 'solve': 5678, 'checks': 5679, 'hot': 5680, 'deportation': 5681, 'super': 5682, 'plays': 5683, 'contacted': 5684, 'bushehr': 5685, 'bishkek': 5686, 'radar': 5687, 'nour': 5688, 'slick': 5689, 'homeowners': 5690, 'methods': 5691, 'floodwaters': 5692, 'teacher': 5693, 'worry': 5694, 'yen': 5695, 'mercosur': 5696, 'aquino': 5697, 'spirit': 5698, 'undergo': 5699, 'panic': 5700, 'lunch': 5701, 'broker': 5702, 'arrangement': 5703, 'garcia': 5704, 'flores': 5705, 'adult': 5706, 'augusto': 5707, 'melinda': 5708, 'tuberculosis': 5709, 'finalized': 5710, 'types': 5711, 'initiated': 5712, '1972': 5713, 'accounted': 5714, 'pursuit': 5715, 'mutual': 5716, 'forest': 5717, '97': 5718, 'escalated': 5719, 'harder': 5720, 'treaties': 5721, 'rifle': 5722, 'donate': 5723, 'graves': 5724, 'honoring': 5725, 'andy': 5726, 'mothers': 5727, 'symbol': 5728, 'skilled': 5729, 'anonymity': 5730, 'enjoyed': 5731, 'interrupted': 5732, 'extraordinary': 5733, 'condemnation': 5734, 'passes': 5735, 'classes': 5736, 'depicting': 5737, 'vision': 5738, 'performed': 5739, 'compliance': 5740, '1978': 5741, 'regulatory': 5742, 'proclaimed': 5743, 'armenian': 5744, 'invested': 5745, 'tie': 5746, 'design': 5747, 'unarmed': 5748, 'repay': 5749, 'gandhi': 5750, 'barghouti': 5751, 'saving': 5752, 'regained': 5753, 'kasuri': 5754, 'vacation': 5755, 'authenticity': 5756, 'defectors': 5757, 'beans': 5758, 'overcrowded': 5759, 'provision': 5760, 'tomb': 5761, 'lech': 5762, 'phosphate': 5763, 'please': 5764, 'thabo': 5765, 'mahdi': 5766, 'runner': 5767, 'racism': 5768, 'impossible': 5769, 'cultivation': 5770, 'shoes': 5771, 'smashed': 5772, 'objects': 5773, 'removal': 5774, '202': 5775, 'shaul': 5776, 'separation': 5777, 'calendar': 5778, 'failures': 5779, 'gerard': 5780, 'viewed': 5781, 'petersburg': 5782, 'photographer': 5783, 'principles': 5784, 'clients': 5785, 'benazir': 5786, 'premier': 5787, 'tin': 5788, 'stiff': 5789, 'chest': 5790, 'load': 5791, 'declines': 5792, 'botswana': 5793, 'applied': 5794, 'launches': 5795, 'jiabao': 5796, 'exchanged': 5797, 'bullets': 5798, 'institution': 5799, '74': 5800, 'felt': 5801, 'refuses': 5802, 'vaccination': 5803, "o'connor": 5804, 'tribes': 5805, 'clan': 5806, 'detaining': 5807, 'organize': 5808, 'warheads': 5809, 'cameraman': 5810, 'inform': 5811, 'ball': 5812, '66': 5813, 'coups': 5814, 'stabilization': 5815, 'stole': 5816, 'thing': 5817, '01': 5818, 'robust': 5819, 'lengthy': 5820, 'concessions': 5821, 'expense': 5822, '30th': 5823, 'beit': 5824, 'changing': 5825, 'accordance': 5826, 'surprised': 5827, 'hatred': 5828, 'warfare': 5829, 'slalom': 5830, 'universities': 5831, 'probing': 5832, 'turkmenistan': 5833, 'workforce': 5834, 'unspecified': 5835, 'ratification': 5836, 'bonds': 5837, 'investigative': 5838, 'photograph': 5839, '2020': 5840, 'icy': 5841, 'espionage': 5842, 'yulia': 5843, 'biased': 5844, 'conservation': 5845, 'greatly': 5846, 'hid': 5847, 'americas': 5848, 'kirchner': 5849, 'wimbledon': 5850, '450': 5851, 'timor': 5852, 'preserve': 5853, 'ethiopians': 5854, 'binding': 5855, 'assassinate': 5856, 'constructed': 5857, 'stretch': 5858, 'shinawatra': 5859, 'experiments': 5860, 'disagreements': 5861, 'broader': 5862, 'rosneft': 5863, 'plutonium': 5864, 'oecd': 5865, 'journey': 5866, 'tail': 5867, 'looked': 5868, 'chung': 5869, 'score': 5870, 'australians': 5871, 'rig': 5872, 'fueling': 5873, 'fitr': 5874, 'legally': 5875, 'containing': 5876, 'housed': 5877, '1959': 5878, 'disclosed': 5879, 'radovan': 5880, 'relocate': 5881, 'moratorium': 5882, 'teenage': 5883, 'tolerate': 5884, 'rctv': 5885, 'mustafa': 5886, 'serpent': 5887, 'reconnaissance': 5888, 'arabian': 5889, 'burn': 5890, 'cardinal': 5891, 'severed': 5892, 'knocked': 5893, 'murdering': 5894, 'consular': 5895, 'urgent': 5896, 'menezes': 5897, 'servants': 5898, 'merge': 5899, 'occasions': 5900, 'launchers': 5901, 'medication': 5902, 'caucasus': 5903, 'electronics': 5904, '94': 5905, 'exiles': 5906, 'sheep': 5907, 'blues': 5908, 'persecution': 5909, 'afterwards': 5910, 'rubber': 5911, 'carries': 5912, 'ira': 5913, 'drawing': 5914, 'alcohol': 5915, 'rugged': 5916, 'nawaz': 5917, 'ould': 5918, 'yudhoyono': 5919, 'cautioned': 5920, 'assurances': 5921, 'thrown': 5922, 'xinjiang': 5923, 'fema': 5924, 'ussr': 5925, 'migratory': 5926, 'andean': 5927, 'cheaper': 5928, 'banning': 5929, 'monarch': 5930, 'nasser': 5931, 'chased': 5932, 'shoulder': 5933, 'promoted': 5934, 'actual': 5935, 'acquired': 5936, 'lies': 5937, 'swans': 5938, 'caretaker': 5939, 'quality': 5940, 'ortega': 5941, 'cancun': 5942, 'flame': 5943, 'arguing': 5944, 'walk': 5945, 'nose': 5946, '1948': 5947, 'hollywood': 5948, 'suggest': 5949, 'seekers': 5950, 'briefing': 5951, 'assaults': 5952, 'ushered': 5953, 'antarctica': 5954, 'interpol': 5955, 'zelaya': 5956, 'impeachment': 5957, 'dera': 5958, 'contribution': 5959, 'nationalize': 5960, 'saleh': 5961, 'easter': 5962, 'audiences': 5963, 'goss': 5964, 'commemorate': 5965, 'chertoff': 5966, 'procession': 5967, 'khalilzad': 5968, 'talat': 5969, 'anwar': 5970, 'currencies': 5971, 'managers': 5972, 'ferry': 5973, 'happen': 5974, 'gay': 5975, 'defected': 5976, 'functioning': 5977, 'detlev': 5978, 'bridges': 5979, 'songs': 5980, 'francois': 5981, 'tackle': 5982, 'upgrade': 5983, 'speakers': 5984, 'tightened': 5985, '96': 5986, '750': 5987, 'vaccines': 5988, 'glass': 5989, 'observer': 5990, 'audit': 5991, 'permanently': 5992, 'regards': 5993, 'proceed': 5994, 'globe': 5995, 'gunbattles': 5996, 'serves': 5997, 'capability': 5998, 'inflows': 5999, 'sentiment': 6000, 'corporate': 6001, 'kharrazi': 6002, 'employers': 6003, 'hired': 6004, 'inaugurated': 6005, 'batons': 6006, 'choosing': 6007, 'factors': 6008, 'affects': 6009, 'clark': 6010, 'roed': 6011, 'larsen': 6012, 'master': 6013, 'guarantee': 6014, 'obligations': 6015, '104': 6016, 'colorado': 6017, 'kony': 6018, 'reversed': 6019, 'fm': 6020, 'arsenal': 6021, 'yuri': 6022, 'emerge': 6023, 'gross': 6024, 'scottish': 6025, 'latter': 6026, 'inhabited': 6027, 'attained': 6028, 'ivan': 6029, 'sailing': 6030, 'manner': 6031, 'sends': 6032, 'utc': 6033, 'programming': 6034, 'dignitaries': 6035, 'discipline': 6036, 'denouncing': 6037, 'gifts': 6038, 'imperial': 6039, 'writer': 6040, 'eyes': 6041, 'heights': 6042, 'nervous': 6043, 'midst': 6044, 'interviewed': 6045, 'stamps': 6046, 'dirty': 6047, 'improvised': 6048, 'approving': 6049, 'complain': 6050, 'dismantled': 6051, 'executions': 6052, 'andijan': 6053, 'restraint': 6054, 'evil': 6055, 'moammar': 6056, 'safely': 6057, 'mob': 6058, 'detect': 6059, 'defenses': 6060, 'restricting': 6061, 'fortified': 6062, 'strapped': 6063, 'pursued': 6064, 'replacing': 6065, 'explanation': 6066, 'completion': 6067, 'exploiting': 6068, 'livelihood': 6069, 'mismanagement': 6070, 'character': 6071, 'scrutiny': 6072, 'slavery': 6073, 'curtail': 6074, 'patrick': 6075, 'identification': 6076, 'cards': 6077, 'shipment': 6078, 'runway': 6079, '115': 6080, 'supplier': 6081, 'acknowledge': 6082, 'notified': 6083, 'dates': 6084, 'efficiency': 6085, 'knee': 6086, 'occupying': 6087, 'pardoned': 6088, 'pardons': 6089, 'fao': 6090, 'reiterated': 6091, 'length': 6092, 'box': 6093, 'deliberately': 6094, 'taxi': 6095, 'milan': 6096, 'basilica': 6097, 'insulting': 6098, 'suggestions': 6099, 'oversight': 6100, 'festivities': 6101, 'medalist': 6102, 'irresponsible': 6103, 'eyadema': 6104, 'chronic': 6105, 'cleaning': 6106, 'enjoys': 6107, 'revenge': 6108, 'spur': 6109, 'musicians': 6110, 'ecuadorean': 6111, 'saeb': 6112, 'erekat': 6113, 'amend': 6114, 'turnout': 6115, 'theft': 6116, 'learning': 6117, 'slum': 6118, 'radios': 6119, 'regret': 6120, 'densely': 6121, 'disappointed': 6122, 'sandra': 6123, 'moo': 6124, 'hyun': 6125, 'automaker': 6126, 'competitive': 6127, 'bullet': 6128, 'shipped': 6129, 'confederation': 6130, 'entities': 6131, 'ultimately': 6132, 'warring': 6133, 'nikkei': 6134, 'hang': 6135, 'indexes': 6136, 'breakthrough': 6137, 'rush': 6138, 'hurting': 6139, 'passport': 6140, 'cote': 6141, 'accession': 6142, 'polisario': 6143, 'prayed': 6144, 'holidays': 6145, 'drops': 6146, 'endured': 6147, 'havens': 6148, 'tolerance': 6149, 'maria': 6150, 'text': 6151, 'expulsion': 6152, 'fix': 6153, 'photo': 6154, 'hakim': 6155, 'seizing': 6156, 'regain': 6157, 'somewhat': 6158, 'filmmaker': 6159, 'viewers': 6160, 'credentials': 6161, 'arrives': 6162, 'emancipation': 6163, 'banny': 6164, 'legislator': 6165, '92': 6166, 'intensive': 6167, 'feeling': 6168, 'dual': 6169, 'hayden': 6170, 'experiencing': 6171, 'ratings': 6172, 'favorable': 6173, 'pressured': 6174, 'nyunt': 6175, 'rainfall': 6176, 'canary': 6177, 'resorts': 6178, 'kostelic': 6179, 'fairly': 6180, 'confirms': 6181, 'retaliated': 6182, 'usa': 6183, 'chairs': 6184, 'soft': 6185, 'kids': 6186, 'shouting': 6187, 'beheaded': 6188, 'arbitration': 6189, 'teen': 6190, 'correct': 6191, 'panamanian': 6192, 'ansar': 6193, 'restoration': 6194, 'fernandez': 6195, 'pharmaceutical': 6196, 'betancourt': 6197, 'nearing': 6198, '148': 6199, 'yard': 6200, 'armenians': 6201, 'bystanders': 6202, 'handful': 6203, 'midwestern': 6204, 'meaning': 6205, 'jails': 6206, 'argument': 6207, 'sarajevo': 6208, 'ratko': 6209, 'timing': 6210, 'knew': 6211, 'clarke': 6212, 'conservatives': 6213, 'countryside': 6214, 'indebted': 6215, 'belonged': 6216, 'inciting': 6217, 'becomes': 6218, 'haditha': 6219, 'cat': 6220, '14th': 6221, 'madonna': 6222, 'committees': 6223, 'studio': 6224, 'ap': 6225, 'sanctuary': 6226, 'twenty': 6227, 'medina': 6228, 'resistant': 6229, 'oklahoma': 6230, 'translator': 6231, 'liter': 6232, '135': 6233, 'transfers': 6234, 'adoption': 6235, 'christianity': 6236, 'lashed': 6237, 'populous': 6238, 'mud': 6239, 'highlight': 6240, 'completing': 6241, 'diverse': 6242, 'bounty': 6243, 'appearing': 6244, 'priorities': 6245, 'swaziland': 6246, 'lindsay': 6247, 'prolonged': 6248, 'neighborhoods': 6249, 'voluntarily': 6250, 'respondents': 6251, 'undercover': 6252, 'patriot': 6253, 'justified': 6254, 'widow': 6255, 'inhabitants': 6256, 'marches': 6257, 'repeal': 6258, 'interested': 6259, 'banners': 6260, 'kivu': 6261, 'disbanded': 6262, 'loved': 6263, 'perez': 6264, 'socialism': 6265, 'separating': 6266, 'prosperity': 6267, 'tickets': 6268, 'airplanes': 6269, 'minerals': 6270, 'quarterfinals': 6271, 'select': 6272, 'qala': 6273, 'nuristan': 6274, 'elephants': 6275, 'teach': 6276, 'torrential': 6277, 'abramoff': 6278, 'resurgent': 6279, 'mqm': 6280, 'ashura': 6281, 'poison': 6282, 'madoff': 6283, 'benzene': 6284, 'qualified': 6285, 'deter': 6286, 'praise': 6287, 'enterprises': 6288, 'zero': 6289, 'downward': 6290, 'wishing': 6291, 'virtually': 6292, 'undisclosed': 6293, 'chevron': 6294, 'enhance': 6295, 'spector': 6296, 'ereli': 6297, 'capitol': 6298, 'zidane': 6299, 'haradinaj': 6300, 'saud': 6301, 'barzani': 6302, 'iceland': 6303, 'hole': 6304, 'allen': 6305, '09': 6306, 'perform': 6307, 'bottles': 6308, 'concentrated': 6309, 'whereabouts': 6310, 'jungle': 6311, 'predicts': 6312, 'precautions': 6313, 'dramatic': 6314, 'witnessed': 6315, 'exported': 6316, 'transformed': 6317, 'bloodless': 6318, 'forestry': 6319, 'arranged': 6320, 'authoritarian': 6321, 'locally': 6322, 'acute': 6323, 'respiratory': 6324, 'misuse': 6325, 'doha': 6326, 'determination': 6327, 'rampage': 6328, 'haitians': 6329, 'tribute': 6330, 'highlighting': 6331, 'poses': 6332, 'accepting': 6333, 'degree': 6334, 'agrees': 6335, 'postal': 6336, 'jalalabad': 6337, 'intervened': 6338, 'forged': 6339, 'manufacturer': 6340, 'flat': 6341, 'resisted': 6342, 'sustainable': 6343, 'ponte': 6344, 'suriname': 6345, 'striking': 6346, 'artists': 6347, 'technologies': 6348, 'authorize': 6349, 'censorship': 6350, 'khmer': 6351, 'outright': 6352, 'cayman': 6353, 'shape': 6354, '21st': 6355, 'rahim': 6356, 'greetings': 6357, 'engaging': 6358, 'toured': 6359, 'pilots': 6360, 'suppliers': 6361, 'michel': 6362, 'batch': 6363, 'deputies': 6364, 'substance': 6365, 'michelle': 6366, 'outdoor': 6367, 'pennsylvania': 6368, 'zenawi': 6369, 'dated': 6370, 'repressive': 6371, 'extent': 6372, 'gustav': 6373, 'liquid': 6374, 'announcing': 6375, 'oslo': 6376, '1968': 6377, 'transported': 6378, 'quantities': 6379, 'priced': 6380, 'battery': 6381, 'incomes': 6382, 'rough': 6383, 'cancellation': 6384, 'observances': 6385, '1977': 6386, 'indiana': 6387, 'locals': 6388, 'unfairly': 6389, 'chocolate': 6390, 'originated': 6391, 'tent': 6392, 'shaken': 6393, 'interfere': 6394, 'dark': 6395, 'convince': 6396, '103': 6397, 'jemaah': 6398, 'counsel': 6399, 'weakening': 6400, 'mcdonald': 6401, 'shattered': 6402, 'brand': 6403, 'parchin': 6404, 'torsello': 6405, 'downer': 6406, 'shaped': 6407, 'fatality': 6408, 'repairs': 6409, 'stockholm': 6410, '1971': 6411, 'faure': 6412, '125': 6413, 'assisted': 6414, 'contentious': 6415, 'excellent': 6416, 'airplane': 6417, 'globalization': 6418, 'providencia': 6419, 'inappropriate': 6420, 'measured': 6421, '225': 6422, 'hijacking': 6423, 'retiring': 6424, 'really': 6425, 'ticket': 6426, 'crawford': 6427, 'stampede': 6428, 'mastrogiacomo': 6429, 'mediation': 6430, 'diarrhea': 6431, 'ministerial': 6432, 'salary': 6433, 'nuevo': 6434, '123': 6435, 'indefinitely': 6436, 'deploying': 6437, 'stories': 6438, 'mashaal': 6439, 'richards': 6440, 'walking': 6441, 'makers': 6442, 'pressed': 6443, 'yellow': 6444, 'mdc': 6445, 'blowing': 6446, 'continental': 6447, 'collision': 6448, 'punjab': 6449, 'containers': 6450, 'unusual': 6451, 'russians': 6452, 'madagascar': 6453, 'transferring': 6454, 'responsibilities': 6455, 'yao': 6456, 'nba': 6457, 'telescope': 6458, 'celebrates': 6459, 'carnival': 6460, 'parades': 6461, '330': 6462, '2014': 6463, 'documentary': 6464, 'classic': 6465, 'transactions': 6466, 'swap': 6467, 'granma': 6468, 'bajaur': 6469, 'harcourt': 6470, 'alonso': 6471, 'steinmeier': 6472, 'robbery': 6473, 'physician': 6474, 'describing': 6475, 'slashed': 6476, 'tunisia': 6477, 'conversations': 6478, 'coastline': 6479, 'declaring': 6480, '119': 6481, 'perceived': 6482, 'manufacture': 6483, 'freeing': 6484, 'gets': 6485, 'servicing': 6486, 'harassment': 6487, 'disclose': 6488, 'deteriorated': 6489, 'bared': 6490, 'akhtar': 6491, 'zhang': 6492, 'skating': 6493, 'zimbabwean': 6494, 'poisoning': 6495, 'harare': 6496, 'syed': 6497, 'modest': 6498, 'maneuvers': 6499, 'securing': 6500, 'lending': 6501, 'clues': 6502, 'captors': 6503, 'ministries': 6504, 'cousin': 6505, 'reconsider': 6506, 'random': 6507, 'merck': 6508, 'luanda': 6509, 'sight': 6510, 'anywhere': 6511, 'cantoni': 6512, '650': 6513, 'nephew': 6514, 'uncertainty': 6515, 'honored': 6516, 'armor': 6517, 'cigarettes': 6518, 'scrap': 6519, 'impasse': 6520, 'unresolved': 6521, 'cruel': 6522, 'filing': 6523, 'signal': 6524, 'warships': 6525, 'insufficient': 6526, 'bermuda': 6527, 'savings': 6528, 'asleep': 6529, 'raises': 6530, 'manage': 6531, 'raped': 6532, 'compensate': 6533, 'narcotics': 6534, 'harvest': 6535, 'slated': 6536, 'renegade': 6537, 'understand': 6538, 'exhausted': 6539, 'democracies': 6540, 'retreat': 6541, 'peacekeeper': 6542, 'verify': 6543, 'migrant': 6544, 'captives': 6545, 'maskhadov': 6546, 'collecting': 6547, 'ultra': 6548, '89': 6549, 'household': 6550, 'exceed': 6551, 'checked': 6552, 'wells': 6553, 'pearl': 6554, 'renewable': 6555, 'detonate': 6556, 'absentia': 6557, 'euphrates': 6558, 'desperate': 6559, 'spots': 6560, 'assisting': 6561, 'fee': 6562, 'mainstream': 6563, 'roll': 6564, 'publish': 6565, 'confidential': 6566, 'moral': 6567, 'balkans': 6568, 'memo': 6569, 'deadlocked': 6570, 'difficulty': 6571, 'defied': 6572, 'grounded': 6573, 'examined': 6574, 'toyota': 6575, 'zoo': 6576, 'panda': 6577, 'lie': 6578, 'distributed': 6579, 'cook': 6580, 'columbia': 6581, 'watching': 6582, 'mohmand': 6583, 'perpetrators': 6584, 'youngest': 6585, 'algiers': 6586, 'courage': 6587, 'lights': 6588, 'motor': 6589, 'distant': 6590, 'taught': 6591, 'techniques': 6592, 'guarded': 6593, 'quito': 6594, 'alternate': 6595, '98': 6596, 'barcelona': 6597, 'pattani': 6598, 'yala': 6599, 'gaming': 6600, 'nicaraguan': 6601, 'undermining': 6602, 'roles': 6603, 'bhutan': 6604, 'tiananmen': 6605, 'keeps': 6606, 'stan': 6607, 'entertainment': 6608, 'mounted': 6609, 'accidental': 6610, 'households': 6611, 'guangzhou': 6612, 'openly': 6613, 'jul': 6614, 'heritage': 6615, 'author': 6616, 'stream': 6617, 'reacted': 6618, 'mad': 6619, 'settler': 6620, 'disgraced': 6621, 'harper': 6622, 'rican': 6623, 'transparent': 6624, 'yuan': 6625, 'ruins': 6626, 'bidding': 6627, 'harbin': 6628, 'advisory': 6629, 'involve': 6630, 'anc': 6631, 'oxfam': 6632, 'beta': 6633, 'paulo': 6634, 'ma': 6635, 'hawk': 6636, 'livni': 6637, 'projected': 6638, 'macedonian': 6639, 'tortoise': 6640, 'cafta': 6641, 'locate': 6642, 'nationalization': 6643, 'hate': 6644, 'liu': 6645, 'booming': 6646, 'carefully': 6647, 'hakimi': 6648, 'sony': 6649, 'wolfowitz': 6650, 'mistreatment': 6651, 'whittington': 6652, 'juarez': 6653, '99': 6654, 'venus': 6655, 'handover': 6656, 'hwang': 6657, 'levees': 6658, 'seal': 6659, 'siniora': 6660, 'missouri': 6661, 'epicenter': 6662, 'disorder': 6663, 'screening': 6664, 'advocate': 6665, 'zapatista': 6666, 'objective': 6667, 'arabiya': 6668, 'allegiance': 6669, 'combatants': 6670, '1963': 6671, 'partially': 6672, 'imminent': 6673, 'copies': 6674, 'deciding': 6675, 'capturing': 6676, 'slipped': 6677, 'sniper': 6678, 'visas': 6679, 'friendship': 6680, 'unchanged': 6681, 'unhurt': 6682, 'forensic': 6683, 'achievements': 6684, 'honors': 6685, 'contributing': 6686, 'consistent': 6687, 'inability': 6688, '185': 6689, 'unofficial': 6690, 'sky': 6691, '86': 6692, 'guardsmen': 6693, 'dostum': 6694, 'resolutions': 6695, 'disarmed': 6696, 'miguel': 6697, 'deport': 6698, 'emphasized': 6699, 'airways': 6700, 'testify': 6701, 'covers': 6702, 'arrangements': 6703, 'zagreb': 6704, 'literature': 6705, 'pirate': 6706, 'occur': 6707, 'austerity': 6708, 'recruitment': 6709, 'worsened': 6710, 'investing': 6711, 'respected': 6712, 'justify': 6713, 'nazis': 6714, 'arguments': 6715, 'recognizing': 6716, 'batticaloa': 6717, 'ian': 6718, 'golan': 6719, 'stabilizing': 6720, 'withdrawals': 6721, 'boucher': 6722, 'convinced': 6723, 'clandestine': 6724, 'titles': 6725, 'cafe': 6726, 'sistani': 6727, 'hijackers': 6728, 'weaponry': 6729, 'repatriated': 6730, 'satisfied': 6731, 'ernesto': 6732, 'meles': 6733, 'nationally': 6734, 'qualifying': 6735, 'dolphins': 6736, 'solomon': 6737, 'instituted': 6738, 'equipped': 6739, 'collection': 6740, 'apparel': 6741, 'creole': 6742, 'moroccans': 6743, 'hydropower': 6744, 'amended': 6745, 'exploitation': 6746, 'successive': 6747, 'siberia': 6748, 'generations': 6749, 'flowers': 6750, 'thirty': 6751, 'adel': 6752, 'buildup': 6753, 'ivorian': 6754, 'doors': 6755, 'ingredient': 6756, 'unlike': 6757, 'hungry': 6758, 'en': 6759, 'majid': 6760, 'dioxide': 6761, 'inner': 6762, 'nightclub': 6763, 'medics': 6764, 'detain': 6765, 'pardon': 6766, 'examining': 6767, 'dangers': 6768, 'blasphemous': 6769, 'ignore': 6770, 'affecting': 6771, 'nordic': 6772, 'beauty': 6773, 'hawaii': 6774, 'richest': 6775, 'advice': 6776, 'scattered': 6777, 'virtual': 6778, 'beckham': 6779, 'consulting': 6780, 'indies': 6781, 'austro': 6782, 'dissolution': 6783, 'dynasty': 6784, 'principality': 6785, 'bishop': 6786, 'exceeded': 6787, 'lesotho': 6788, 'stag': 6789, 'height': 6790, 'o': 6791, 'sank': 6792, 'strategies': 6793, 'eradication': 6794, 'regrets': 6795, 'boarded': 6796, 'lacked': 6797, 'wedding': 6798, 'sections': 6799, 'objected': 6800, 'generating': 6801, 'mired': 6802, 'tainted': 6803, 'excuse': 6804, 'replaces': 6805, 'postponement': 6806, 'dig': 6807, 'composite': 6808, 'seng': 6809, 'frankfurt': 6810, 'tim': 6811, 'holiest': 6812, 'spaniard': 6813, "d'ivoire": 6814, 'mild': 6815, 'ate': 6816, 'wish': 6817, 'chairmanship': 6818, 'reflects': 6819, 'eruption': 6820, 'collided': 6821, 'maharashtra': 6822, 'bombay': 6823, 'sued': 6824, 'connecticut': 6825, 'warehouse': 6826, 'safeguard': 6827, 'islamiyah': 6828, 'baku': 6829, 'collect': 6830, 'pride': 6831, 'ordinary': 6832, 'borrowing': 6833, 'slam': 6834, 'andre': 6835, '114': 6836, 'dissolve': 6837, 'tutsi': 6838, 'youssef': 6839, 'jupiter': 6840, 'grab': 6841, 'bigger': 6842, 'unpopular': 6843, 'looting': 6844, 'hoshyar': 6845, 'covert': 6846, 'dakar': 6847, 'honduran': 6848, 'fatally': 6849, 'bids': 6850, '1000': 6851, 'beasts': 6852, 'swift': 6853, 'lenders': 6854, 'presiding': 6855, 'releases': 6856, 'closest': 6857, 'reputation': 6858, 'mary': 6859, 'disappearance': 6860, 'contends': 6861, 'jobless': 6862, 'secessionist': 6863, 'application': 6864, 'farther': 6865, 'melting': 6866, 'graft': 6867, 'circuit': 6868, 'consortium': 6869, 'wa': 6870, 'minnesota': 6871, 'morris': 6872, 'nahr': 6873, 'rejection': 6874, 'racial': 6875, 'opens': 6876, 'eleven': 6877, 'throw': 6878, 'hurriyat': 6879, 'nov': 6880, 'convenes': 6881, 'touched': 6882, 'objectives': 6883, 'squads': 6884, 'drafted': 6885, 'lull': 6886, 'derailed': 6887, 'baath': 6888, 'auschwitz': 6889, 'kerekou': 6890, 'brownfield': 6891, 'fluids': 6892, "'ll": 6893, 'embezzlement': 6894, 'bertel': 6895, 'standings': 6896, 'liberty': 6897, 'telegraph': 6898, 'bargain': 6899, 'quarantine': 6900, 'contributes': 6901, 'neutrality': 6902, 'companion': 6903, "'m": 6904, 'carolyn': 6905, 'journalism': 6906, 'relating': 6907, 'smuggle': 6908, 'readiness': 6909, 'bracing': 6910, 'vaccinations': 6911, 'conclude': 6912, 'indictments': 6913, 'seas': 6914, 'hijackings': 6915, 'exchanges': 6916, 'yes': 6917, 'tracking': 6918, 'displayed': 6919, 'oversaw': 6920, 'disappointing': 6921, 'depart': 6922, 'stomach': 6923, 'constructive': 6924, 'contracting': 6925, 'passports': 6926, 'flagged': 6927, 'centrist': 6928, 'briton': 6929, 'singled': 6930, 'airborne': 6931, 'le': 6932, 'breach': 6933, 'wages': 6934, 'reelected': 6935, 'sit': 6936, 'picking': 6937, 'isfahan': 6938, 'appointments': 6939, 'competitors': 6940, 'brad': 6941, 'boston': 6942, 'afterward': 6943, 'contents': 6944, 'rooted': 6945, 'grass': 6946, 'basescu': 6947, 'nazarbayev': 6948, 'violates': 6949, 'pence': 6950, 'neutral': 6951, 'preserving': 6952, 'dogs': 6953, 'lightning': 6954, 'engines': 6955, 'ituri': 6956, 'appearances': 6957, 'comedy': 6958, 'rodrigo': 6959, 'rid': 6960, 'servicemen': 6961, 'mehmet': 6962, 'lohan': 6963, 'chrysler': 6964, 'starvation': 6965, 'zambian': 6966, 'banda': 6967, 'guilders': 6968, 'flows': 6969, 'bambang': 6970, 'hussain': 6971, 'perhaps': 6972, 'extends': 6973, 'kumaratunga': 6974, 'regard': 6975, 'karami': 6976, 'posing': 6977, 'outlined': 6978, 'accountable': 6979, 'waving': 6980, 'sweeping': 6981, 'crushed': 6982, 'narathiwat': 6983, 'archaeologists': 6984, 'protestant': 6985, 'pelosi': 6986, 'surveys': 6987, 'packages': 6988, 'drills': 6989, 'dating': 6990, 'brent': 6991, 'sits': 6992, 'saved': 6993, 'operatives': 6994, 'proceeds': 6995, 'pan': 6996, 'anthony': 6997, 'gibraltar': 6998, 'everest': 6999, 'dependency': 7000, 'pigs': 7001, 'admit': 7002, 'intensify': 7003, 'analysis': 7004, 'astronaut': 7005, 'addresses': 7006, 'bloodiest': 7007, 'jennifer': 7008, 'slump': 7009, 'commuter': 7010, 'valued': 7011, 'fiji': 7012, 'arrivals': 7013, 'hipc': 7014, 'tb': 7015, 'abe': 7016, 'torched': 7017, 'britney': 7018, 'propose': 7019, 'proven': 7020, 'textiles': 7021, 'reformist': 7022, 'myers': 7023, 'instances': 7024, 'licenses': 7025, 'cruise': 7026, 'scope': 7027, 'chinook': 7028, 'nasrallah': 7029, 'ethics': 7030, 'tashkent': 7031, 'termed': 7032, 'mehdi': 7033, 'burial': 7034, 'flotilla': 7035, 'prey': 7036, 'carriers': 7037, 'kurmanbek': 7038, 'kerik': 7039, 'slashing': 7040, 'poured': 7041, 'songhua': 7042, 'meteorological': 7043, 'stadiums': 7044, 'nelson': 7045, 'hay': 7046, 'setback': 7047, 'mv': 7048, 'baluch': 7049, 'detroit': 7050, 'evacuees': 7051, 'flush': 7052, 'girlfriend': 7053, 'toure': 7054, 'mahathir': 7055, 'najib': 7056, 'senegalese': 7057, 'atop': 7058, 'oas': 7059, 'stored': 7060, 'ideas': 7061, 'prone': 7062, 'qinghai': 7063, 'finances': 7064, 'somewhere': 7065, 'reality': 7066, 'overs': 7067, 'bowler': 7068, 'adds': 7069, 'geese': 7070, 'christ': 7071, 'hero': 7072, 'channels': 7073, 'brunei': 7074, 'sponsor': 7075, 'campaigns': 7076, 'maduro': 7077, 'sizable': 7078, 'blackout': 7079, '\x92s': 7080, 'rigging': 7081, 'exactly': 7082, 'hiroshima': 7083, 'petraeus': 7084, 'laying': 7085, 'recall': 7086, 'pierre': 7087, 'upjohn': 7088, 'hart': 7089, 'disagreement': 7090, 'roberts': 7091, 'bagapsh': 7092, 'laredo': 7093, 'laptop': 7094, 'hicks': 7095, 'tata': 7096, 'kcna': 7097, 'finalize': 7098, 'marcos': 7099, 'sabah': 7100, 'scholars': 7101, 'harassed': 7102, 'whenever': 7103, 'vaccinate': 7104, 'assaulted': 7105, 'crippled': 7106, 'enabled': 7107, 'trap': 7108, 'sevan': 7109, 'diverted': 7110, 'proclamation': 7111, 'johnston': 7112, 'invade': 7113, 'collaboration': 7114, 'sher': 7115, 'ethical': 7116, 'quarterly': 7117, 'indicating': 7118, 'dispersed': 7119, 'affair': 7120, 'corpses': 7121, 'abducting': 7122, 'nancy': 7123, 'blake': 7124, 'mayer': 7125, 'inequality': 7126, 'authorizes': 7127, 'unannounced': 7128, 'offense': 7129, 'campbell': 7130, 'enjoying': 7131, 'victoria': 7132, '1917': 7133, 'revered': 7134, 'communism': 7135, 'geographic': 7136, 'commanded': 7137, 'juba': 7138, 'shouted': 7139, 'docked': 7140, 'purportedly': 7141, 'midday': 7142, 'moreno': 7143, 'hindered': 7144, 'benefited': 7145, '737': 7146, 'refuse': 7147, 'momentum': 7148, 'transaction': 7149, 'historically': 7150, 'wales': 7151, 'mosquito': 7152, 'rescheduled': 7153, 'grenada': 7154, 'wire': 7155, 'fischer': 7156, 'vincent': 7157, 'criticizes': 7158, 'volcanic': 7159, 'memory': 7160, 'manipulating': 7161, '240': 7162, 'parking': 7163, 'bond': 7164, 'harming': 7165, 'com': 7166, 'mouse': 7167, 'videotaped': 7168, 'lacking': 7169, 'papal': 7170, 'valid': 7171, 'detonating': 7172, 'twelve': 7173, 'anticipated': 7174, 'unauthorized': 7175, 'audiotape': 7176, 'raged': 7177, 'optimism': 7178, 'modernization': 7179, 'verde': 7180, 'disciplinary': 7181, 'cameras': 7182, 'ventures': 7183, 'handle': 7184, 'confirming': 7185, '128': 7186, 'virgin': 7187, 'proximity': 7188, 'boss': 7189, 'neck': 7190, 'condemn': 7191, 'phased': 7192, 'venue': 7193, 'colleague': 7194, 'assessing': 7195, 'edward': 7196, 'stress': 7197, 'makeshift': 7198, 'rapes': 7199, '1969': 7200, 'masters': 7201, 'nutrition': 7202, 'ethnically': 7203, 'applications': 7204, 'logar': 7205, 'remembered': 7206, 'munitions': 7207, 'pump': 7208, 'installation': 7209, 'munich': 7210, 'toy': 7211, 'regulate': 7212, 'branches': 7213, 'complicity': 7214, 'shocked': 7215, 'qadeer': 7216, 'stuart': 7217, 'indications': 7218, 'realized': 7219, 'magna': 7220, 'mcalpine': 7221, 'dividend': 7222, 'shareholder': 7223, 'historical': 7224, '1966': 7225, 'cave': 7226, 'budgetary': 7227, 'commanding': 7228, 'welcomes': 7229, 'campaigned': 7230, 'laboratories': 7231, 'allocated': 7232, 'miers': 7233, 'assigned': 7234, 'infant': 7235, 'charities': 7236, 'capitals': 7237, 'trash': 7238, 'dana': 7239, 'understands': 7240, 'explained': 7241, 'memorandum': 7242, 'hydroelectric': 7243, 'mamadou': 7244, 'vacuum': 7245, 'golden': 7246, 'whatever': 7247, 'pharmaceuticals': 7248, 'incorporated': 7249, 'seasonal': 7250, 'rebound': 7251, 'retains': 7252, 'kitts': 7253, 'champions': 7254, 'kick': 7255, 'rarely': 7256, 'possessing': 7257, 'tracks': 7258, 'pages': 7259, 'hazardous': 7260, 'taro': 7261, 'wrapping': 7262, 'digging': 7263, 'feels': 7264, 'highlighted': 7265, 'patriarch': 7266, 'joins': 7267, 'sharapova': 7268, 'mainstay': 7269, 'vocal': 7270, 'crippling': 7271, 'talked': 7272, 'galaxies': 7273, 'aggressively': 7274, 'dealt': 7275, 'featuring': 7276, 'fasting': 7277, 'boycotting': 7278, 'malik': 7279, 'convened': 7280, 'feel': 7281, 'tamiflu': 7282, 'offensives': 7283, 'militancy': 7284, 'lewis': 7285, 'idol': 7286, 'axe': 7287, 'sang': 7288, 'employ': 7289, 'educational': 7290, 'confinement': 7291, 'swallow': 7292, 'injunction': 7293, 'pitcher': 7294, 'milestone': 7295, 'amr': 7296, 'outlining': 7297, 'ignoring': 7298, 'gradual': 7299, 'debts': 7300, 'shifting': 7301, 'intimidation': 7302, 'afraid': 7303, 'laura': 7304, 'reunite': 7305, 'hughes': 7306, 'throat': 7307, 'discharged': 7308, 'backs': 7309, 'supervision': 7310, 'chan': 7311, 'retire': 7312, 'academic': 7313, 'applying': 7314, 'focuses': 7315, '08': 7316, 'h1n1': 7317, 'medicines': 7318, 'guidance': 7319, 'heaviest': 7320, 'chaired': 7321, 'hailing': 7322, 'thieves': 7323, 'arson': 7324, 'nestor': 7325, 'azahari': 7326, 'asset': 7327, 'publicist': 7328, 'lowered': 7329, 'diversified': 7330, 'feathers': 7331, 'alaska': 7332, 'denounce': 7333, 'andhra': 7334, 'midwest': 7335, 'chilumpha': 7336, 'wound': 7337, 'decrease': 7338, 'sultan': 7339, 'sunna': 7340, 'dining': 7341, 'revived': 7342, 'arnold': 7343, 'seeds': 7344, 'interrogated': 7345, 'viruses': 7346, '1930s': 7347, 'toilet': 7348, 'halutz': 7349, 'turban': 7350, 'falls': 7351, 'lima': 7352, 'thein': 7353, 'owes': 7354, 'enterprise': 7355, 'easy': 7356, 'sewage': 7357, 'ophelia': 7358, 'classify': 7359, 'sharia': 7360, 'compounds': 7361, '1953': 7362, 'subjected': 7363, 'unite': 7364, 'explore': 7365, 'authorization': 7366, 'blindfolded': 7367, 'kid': 7368, 'michigan': 7369, 'existed': 7370, 'labeled': 7371, 'famed': 7372, 'nets': 7373, 'hashemi': 7374, 'benchmark': 7375, 'sophisticated': 7376, 'pressuring': 7377, 'molestation': 7378, 'approaches': 7379, 'stoppage': 7380, 'aref': 7381, '91': 7382, 'indoor': 7383, 'hansen': 7384, 'contender': 7385, 'ryan': 7386, 'insist': 7387, 'chun': 7388, 'mercantile': 7389, 'declares': 7390, 'elite': 7391, 'songwriter': 7392, 'remainder': 7393, 'shannon': 7394, 'jr': 7395, 'principal': 7396, 'receipts': 7397, 'bailout': 7398, 'influenced': 7399, 'secondary': 7400, 'colonies': 7401, 'insisting': 7402, 'notably': 7403, 'milinkevich': 7404, 'harboring': 7405, 'belize': 7406, 'natwar': 7407, 'quarantined': 7408, 'obstacle': 7409, 'slate': 7410, 'sacred': 7411, 'garrigues': 7412, 'charitable': 7413, 'salman': 7414, 'perry': 7415, 'avalanche': 7416, 'launcher': 7417, 'biotechnology': 7418, 'shalom': 7419, 'spokesmen': 7420, 'adjacent': 7421, 'exacerbated': 7422, 'flared': 7423, 'clijsters': 7424, 'migrating': 7425, 'ward': 7426, 'grip': 7427, 'liaoning': 7428, 'lyon': 7429, 'turns': 7430, 'guests': 7431, 'belongs': 7432, 'assertion': 7433, 'submerged': 7434, 'escalation': 7435, 'advertising': 7436, 'barry': 7437, 'steal': 7438, 'accurate': 7439, 'lodge': 7440, 'diego': 7441, 'levy': 7442, 'baseless': 7443, 'mauritanian': 7444, 'ceremonial': 7445, 'susilo': 7446, 'grouping': 7447, 'recruit': 7448, 'moya': 7449, 'downed': 7450, 'sells': 7451, 'upward': 7452, 'cub': 7453, 'pandas': 7454, 'forcibly': 7455, 'vowing': 7456, 'shields': 7457, 'catastrophic': 7458, 'hadley': 7459, 'cemetery': 7460, 'lawless': 7461, 'recess': 7462, 'missionaries': 7463, 'courses': 7464, 'massoud': 7465, 'clarkson': 7466, 'tribe': 7467, 'disney': 7468, 'dominance': 7469, 'eduardo': 7470, 'intend': 7471, '1970': 7472, 'ox': 7473, 'disguised': 7474, 'wear': 7475, 'gen': 7476, 'seattle': 7477, '850': 7478, 'yucatan': 7479, 'apologize': 7480, 'sacu': 7481, 'royalties': 7482, 'vanuatu': 7483, 'olive': 7484, 'kuala': 7485, 'lumpur': 7486, 'arts': 7487, 'surviving': 7488, 'movies': 7489, 'urges': 7490, 'unconstitutional': 7491, 'familiar': 7492, 'background': 7493, 'manhattan': 7494, 'errors': 7495, 'jorge': 7496, 'chartered': 7497, 'henin': 7498, 'database': 7499, 'automobile': 7500, 'conventional': 7501, 'seizures': 7502, 'landless': 7503, 'waited': 7504, 'sat': 7505, 'soul': 7506, 'determining': 7507, 'veracruz': 7508, 'namibian': 7509, 'spears': 7510, 'federline': 7511, 'holdings': 7512, 'ambushes': 7513, 'pdvsa': 7514, 'buenos': 7515, 'aires': 7516, 'worsening': 7517, 'wrongly': 7518, 'awami': 7519, 'shirts': 7520, 'waved': 7521, 'cluster': 7522, 'hunan': 7523, 'luther': 7524, 'katsav': 7525, 'goldman': 7526, 'lobbying': 7527, 'topol': 7528, 'irving': 7529, 'guarding': 7530, 'orbit': 7531, 'santos': 7532, 'exporters': 7533, 'oceans': 7534, 'sung': 7535, 'tommy': 7536, 'immunity': 7537, 'requiring': 7538, 'reshuffle': 7539, 'negligence': 7540, 'legend': 7541, 'expenditures': 7542, 'imbalance': 7543, 'wardak': 7544, 'automatically': 7545, 'pill': 7546, 'operators': 7547, 'mudslide': 7548, 'slide': 7549, 'purchases': 7550, 'exxonmobil': 7551, 'knows': 7552, 'minster': 7553, 'confession': 7554, 'opera': 7555, 'suggestion': 7556, 'comprises': 7557, 'hilla': 7558, 'mohsen': 7559, 'outspoken': 7560, 'oversees': 7561, 'kozulin': 7562, 'impressive': 7563, 'arrange': 7564, 'dividing': 7565, 'scoring': 7566, 'observance': 7567, 'hikers': 7568, 'chalabi': 7569, 'retained': 7570, 'usual': 7571, 'zalmay': 7572, 'chase': 7573, 'phil': 7574, 'favors': 7575, 'wishes': 7576, 'retailer': 7577, 'videos': 7578, 'tea': 7579, 'mall': 7580, 'posters': 7581, 'jamal': 7582, 'embezzling': 7583, 'shetty': 7584, 'recommend': 7585, 'enclaves': 7586, '\x96': 7587, 'melilla': 7588, 'cheek': 7589, 'relationships': 7590, 'judgment': 7591, 'machines': 7592, 'faisal': 7593, 'prescription': 7594, 'mesa': 7595, 'underwater': 7596, 'manned': 7597, 'financed': 7598, 'assembled': 7599, 'coincides': 7600, 'lucia': 7601, 'prohibited': 7602, 'smokers': 7603, 'mercy': 7604, 'jimmy': 7605, 'thaw': 7606, 'diversification': 7607, 'busiest': 7608, 'functions': 7609, 'perfect': 7610, 'provoked': 7611, 'lighting': 7612, 'consolidate': 7613, 'alliances': 7614, 'allegation': 7615, 'automatic': 7616, 'unemployed': 7617, 'inclusion': 7618, 'migration': 7619, 'bandits': 7620, 'ivo': 7621, 'murray': 7622, 'gender': 7623, 'pregnant': 7624, 'salt': 7625, 'hire': 7626, 'displays': 7627, 'exists': 7628, 'skies': 7629, 'rushing': 7630, 'brutality': 7631, 'kurram': 7632, 'specialist': 7633, 'naked': 7634, 'hamdi': 7635, 'issac': 7636, 'warlord': 7637, 'praying': 7638, 'adha': 7639, '1961': 7640, 'achieving': 7641, 'quartet': 7642, 'turkmen': 7643, 'ante': 7644, 'boundary': 7645, 'explored': 7646, 'periods': 7647, 'cheered': 7648, 'smallest': 7649, 'touring': 7650, 'sake': 7651, 'caution': 7652, 'gogh': 7653, 'bangalore': 7654, 'stimulate': 7655, 'smart': 7656, 'architect': 7657, 'resist': 7658, 'abide': 7659, 'compassion': 7660, 'enact': 7661, 'captive': 7662, 'sikh': 7663, 'marwan': 7664, '9th': 7665, 'walid': 7666, 'signals': 7667, 'paralysis': 7668, 'apple': 7669, 'metals': 7670, 'heated': 7671, 'vacant': 7672, 'biological': 7673, 'substances': 7674, 'paktia': 7675, 'prestigious': 7676, 'hip': 7677, 'muscle': 7678, 'vanunu': 7679, 'sheik': 7680, 'moments': 7681, 'bells': 7682, 'coincided': 7683, 'discourage': 7684, 'conspiring': 7685, 'undermined': 7686, 'radicals': 7687, 'weaknesses': 7688, 'regimes': 7689, 'jolo': 7690, 'economics': 7691, 'barrage': 7692, 'petrobras': 7693, 'analyst': 7694, 'certified': 7695, 'mauritius': 7696, 'submarine': 7697, 'slums': 7698, 'federated': 7699, 'outlook': 7700, 'equatorial': 7701, 'intimidated': 7702, 'alassane': 7703, 'narrowed': 7704, 'aerial': 7705, 'galan': 7706, 'tone': 7707, 'feelings': 7708, 'reinstate': 7709, 'curbing': 7710, 'distribute': 7711, 'spike': 7712, 'excess': 7713, 'possess': 7714, 'bekaa': 7715, 'commando': 7716, 'lieutenants': 7717, 'nam': 7718, 'mandatory': 7719, 'gases': 7720, 'maher': 7721, 'instructed': 7722, 'suspicions': 7723, 'taped': 7724, 'sudden': 7725, 'attractive': 7726, 'tool': 7727, 'recruited': 7728, 'meshaal': 7729, 'unusually': 7730, 'cafes': 7731, 'marijuana': 7732, 'khalis': 7733, 'strictly': 7734, 'adnan': 7735, 'dulaimi': 7736, 'blankets': 7737, 'trio': 7738, 'environmentally': 7739, 'vow': 7740, 'withheld': 7741, 'automotive': 7742, 'overhead': 7743, 'unsuccessfully': 7744, '1929': 7745, 'transformation': 7746, 'constituent': 7747, 'iii': 7748, 'inquired': 7749, 'indicators': 7750, 'grieving': 7751, 'govern': 7752, 'venezuelans': 7753, 'reinforcements': 7754, 'barracks': 7755, 'bulldozers': 7756, 'sincere': 7757, 'confined': 7758, 'cycle': 7759, 'petersen': 7760, 'feud': 7761, 'suppression': 7762, 'lung': 7763, 'maung': 7764, 'perino': 7765, 'ore': 7766, 'fernando': 7767, 'martian': 7768, 'feature': 7769, 'assassinations': 7770, 'beheadings': 7771, 'wetlands': 7772, 'cheering': 7773, 'wealthiest': 7774, 'monaco': 7775, 'casino': 7776, 'gambling': 7777, 'feeding': 7778, 'deserve': 7779, 'cried': 7780, 'answered': 7781, 'thwart': 7782, 'horns': 7783, 'converted': 7784, 'importing': 7785, 'enhancing': 7786, 'tractor': 7787, 'privacy': 7788, 'skin': 7789, 'dumping': 7790, 'citrus': 7791, 'fruits': 7792, 'volunteer': 7793, 'elizabeth': 7794, 'joy': 7795, 'cathedral': 7796, 'domestically': 7797, 'method': 7798, 'miss': 7799, 'apartments': 7800, 'camera': 7801, 'ravalomanana': 7802, 'blows': 7803, 'oregon': 7804, 'slash': 7805, 'chaotic': 7806, 'spoken': 7807, 'hutus': 7808, 'tariq': 7809, 'sympathizers': 7810, 'gatherings': 7811, 'nepali': 7812, 'persuading': 7813, 'insult': 7814, 'assaulting': 7815, 'finnish': 7816, 'mend': 7817, 'karl': 7818, 'timothy': 7819, 'publisher': 7820, '1956': 7821, 'prague': 7822, 'hanged': 7823, 'agha': 7824, 'ahmadi': 7825, 'downgraded': 7826, 'pockets': 7827, 'unfounded': 7828, 'crushing': 7829, 'nominate': 7830, 'blazes': 7831, 'havel': 7832, 'desmond': 7833, 'tutu': 7834, 'tube': 7835, 'manger': 7836, 'flare': 7837, 'siblings': 7838, 'ak': 7839, 'seem': 7840, 'shutting': 7841, 'mechanism': 7842, 'mill': 7843, 'reviewed': 7844, 'reagan': 7845, 'otherwise': 7846, 'disapprove': 7847, 'disruption': 7848, 'sarah': 7849, 'combines': 7850, 'plata': 7851, 'uss': 7852, 'mohamad': 7853, 'husin': 7854, 'formula': 7855, 'fuad': 7856, 'prudent': 7857, 'color': 7858, 'fifty': 7859, 'khabarovsk': 7860, 'creditors': 7861, 'compatriot': 7862, 'valuable': 7863, 'commemorations': 7864, 'maps': 7865, 'update': 7866, 'crimea': 7867, 'lucrative': 7868, 'dehydration': 7869, 'roadblocks': 7870, 'qaim': 7871, 'bodily': 7872, 'aiming': 7873, 'kibo': 7874, 'petitioned': 7875, '1965': 7876, 'beaches': 7877, '102': 7878, 'overrun': 7879, 'tasked': 7880, 'permitted': 7881, 'equals': 7882, 'utilities': 7883, 'frogs': 7884, 'dmitry': 7885, 'robbed': 7886, 'nicotine': 7887, 'dong': 7888, 'concerning': 7889, 'accomplished': 7890, 'trends': 7891, 'marshall': 7892, 'yunnan': 7893, 'uri': 7894, 'shaky': 7895, 'yahoo': 7896, 'switch': 7897, 'opinions': 7898, '190': 7899, 'totally': 7900, 'depending': 7901, 'ceased': 7902, 'newsweek': 7903, 'prachanda': 7904, 'bananas': 7905, 'lu': 7906, 'hanoun': 7907, 'productive': 7908, 'blue': 7909, 'collects': 7910, 'lawlessness': 7911, 'kiribati': 7912, 'exclaimed': 7913, 'obtaining': 7914, 'jurors': 7915, 'leaked': 7916, 'strains': 7917, 'printing': 7918, 'demonstrating': 7919, 'infiltrated': 7920, 'physicians': 7921, 'endorsement': 7922, 'prosecuted': 7923, 'bipartisan': 7924, 'crises': 7925, 'vilks': 7926, 'snap': 7927, 'h5': 7928, '31st': 7929, 'consulates': 7930, 'lage': 7931, 'slower': 7932, 'fundamental': 7933, 'deeper': 7934, 'merged': 7935, 'gambia': 7936, 'counterterrorism': 7937, 'unnecessary': 7938, 'silent': 7939, 'bathroom': 7940, 'canberra': 7941, 'anatolia': 7942, 'encourages': 7943, 'digital': 7944, 'ideology': 7945, 'fireworks': 7946, 'martina': 7947, 'unrelated': 7948, 'occurs': 7949, 'desecrated': 7950, 'dragan': 7951, 'andorra': 7952, 'actors': 7953, 'jazz': 7954, 'fraction': 7955, 'unlawful': 7956, 'discoveries': 7957, 'physically': 7958, 'izmir': 7959, 'pumping': 7960, 'fault': 7961, 'statehood': 7962, 'detail': 7963, 'structures': 7964, 'hamza': 7965, 'scare': 7966, 'gamal': 7967, 'powered': 7968, 'suleiman': 7969, 'silvio': 7970, 'shocks': 7971, 'abdelaziz': 7972, 'fugitives': 7973, 'rauf': 7974, 'moustapha': 7975, 'mistaken': 7976, 'occasionally': 7977, 'postage': 7978, 'handicrafts': 7979, 'extraction': 7980, 'actively': 7981, 'editorial': 7982, 'cool': 7983, 'cameron': 7984, 'explode': 7985, 'roberto': 7986, 'roy': 7987, 'upgraded': 7988, 'sometime': 7989, 'shamil': 7990, 'restrictive': 7991, 'sect': 7992, 'astronomers': 7993, 'cyber': 7994, 'turkeys': 7995, "yar'adua": 7996, 'airbase': 7997, 'lt': 7998, 'ruz': 7999, 'shock': 8000, 'pneumonia': 8001, 'survive': 8002, 'ratio': 8003, 'vegetables': 8004, 'kite': 8005, 'accelerating': 8006, 'pet': 8007, 'panels': 8008, 'renounced': 8009, 'expenses': 8010, 'ul': 8011, 'alabama': 8012, 'dagestan': 8013, 'nickel': 8014, 'retaliate': 8015, 'resting': 8016, 'sessions': 8017, 'hebei': 8018, 'automakers': 8019, 'clubs': 8020, 'km': 8021, 'fiercely': 8022, 'tall': 8023, 'mistreated': 8024, 'pemex': 8025, 'rank': 8026, 'scared': 8027, 'theaters': 8028, 'countryman': 8029, 'loeb': 8030, 'alerted': 8031, 'admission': 8032, 'swing': 8033, 'hindi': 8034, 'subcommittee': 8035, 'oaxaca': 8036, 'elephant': 8037, 'culling': 8038, 'reyes': 8039, 'garment': 8040, 'lawrence': 8041, 'haider': 8042, 'spilled': 8043, 'marchers': 8044, 't': 8045, '147': 8046, 'undecided': 8047, 'berenson': 8048, 'diaz': 8049, 'ritual': 8050, 'reverend': 8051, 'taxation': 8052, 'zhejiang': 8053, 'depot': 8054, 'gah': 8055, 'otunbayeva': 8056, 'cracking': 8057, 'connect': 8058, 'abruptly': 8059, 'czechoslovakia': 8060, 'citigroup': 8061, 'ghad': 8062, 'gheit': 8063, 'chances': 8064, 'trigger': 8065, 'levee': 8066, 'rooting': 8067, 'buyers': 8068, 'aig': 8069, 'publications': 8070, 'cooked': 8071, 'mediated': 8072, 'wiesenthal': 8073, 'unexpected': 8074, 'moyo': 8075, 'debut': 8076, 'coordinating': 8077, 'las': 8078, 'hilary': 8079, 'grammy': 8080, 'rooms': 8081, 'receipt': 8082, 'troubles': 8083, 'seemed': 8084, 'forbes': 8085, 'bleeding': 8086, 'heels': 8087, 'armitage': 8088, 'boundaries': 8089, 'lifetime': 8090, 'achievement': 8091, 'catherine': 8092, 'mobilize': 8093, 'faiths': 8094, 'descendants': 8095, 'offenders': 8096, 'seems': 8097, 'spaniards': 8098, 'rewards': 8099, 'elaine': 8100, 'sivaram': 8101, 'acknowledges': 8102, 'vacationing': 8103, 'abidjan': 8104, 'pattern': 8105, 'preaching': 8106, 'hardenne': 8107, 'prematurely': 8108, 'revoked': 8109, 'foreclosures': 8110, 'sint': 8111, 'k': 8112, 'fifteen': 8113, 'titan': 8114, 'facilitate': 8115, 'additionally': 8116, 'courageous': 8117, 'constrained': 8118, 'engagement': 8119, 'shourd': 8120, 'tombs': 8121, 'rankings': 8122, 'saturn': 8123, 'evolved': 8124, 'reaches': 8125, 'meridian': 8126, 'sheriff': 8127, 'salim': 8128, 'ratners': 8129, 'repelled': 8130, 'refrain': 8131, 'donating': 8132, 'chihuahua': 8133, 'accomplice': 8134, 'restarted': 8135, 'pork': 8136, 'guerillas': 8137, 'childhood': 8138, 'contamination': 8139, 'rawalpindi': 8140, 'kurd': 8141, 'urgently': 8142, 'glasgow': 8143, 'coral': 8144, 'krajicek': 8145, 'disastrous': 8146, 'gere': 8147, 'anglo': 8148, 'backers': 8149, 'forbids': 8150, 'ash': 8151, 'nationalized': 8152, 'mtv': 8153, 'ceuta': 8154, 'lesser': 8155, 'wings': 8156, 'rehnquist': 8157, 'antiquities': 8158, 'saberi': 8159, 'obesity': 8160, 'kadyrov': 8161, 'fujian': 8162, 'glacier': 8163, 'rowhani': 8164, 'baiji': 8165, 'skull': 8166, 'tuvalu': 8167, 'lubanga': 8168, 'obelisk': 8169, 'luck': 8170, 'passaro': 8171, 'asghari': 8172, 'abac': 8173, 'maldives': 8174, 'umbrella': 8175, 'snoop': 8176, 'dogg': 8177, 'heathrow': 8178, 'scuffled': 8179, 'bankers': 8180, 'odinga': 8181, 'cigarette': 8182, 'subcomandante': 8183, 'uttar': 8184, 'studied': 8185, 'suppress': 8186, 'salafist': 8187, 'escorted': 8188, 'longstanding': 8189, 'drag': 8190, 'talons': 8191, 'snatched': 8192, 'proceeded': 8193, 'bribery': 8194, 'volcker': 8195, 'networking': 8196, 'dies': 8197, 'barak': 8198, 'concludes': 8199, 'repairing': 8200, 'corn': 8201, 'deborah': 8202, 'tires': 8203, 'pelted': 8204, 'sangin': 8205, 'reclaim': 8206, 'forbidden': 8207, 'rode': 8208, 'difference': 8209, 'tightly': 8210, 'riders': 8211, 'hewitt': 8212, 'frenchman': 8213, 'sing': 8214, 'biathlon': 8215, 'vancouver': 8216, 'domination': 8217, 'terje': 8218, 'rein': 8219, 'kurt': 8220, 'suppressing': 8221, 'grandson': 8222, 'alexandria': 8223, 'plains': 8224, 'paved': 8225, 'fazlullah': 8226, 'recaptured': 8227, 'dereliction': 8228, 'plead': 8229, 'eaten': 8230, 'sponsors': 8231, 'worsen': 8232, 'yassin': 8233, 'philadelphia': 8234, 'resumes': 8235, 'azeri': 8236, 'renounces': 8237, 'carla': 8238, '1821': 8239, 'guitarist': 8240, 'sizeable': 8241, 'bauxite': 8242, 'introduction': 8243, 'surpassed': 8244, 'ants': 8245, 'stung': 8246, 'indeed': 8247, 'alike': 8248, 'innovation': 8249, 'indiscriminately': 8250, 'farda': 8251, 'wolfgang': 8252, 'tomas': 8253, 'continuously': 8254, 'internally': 8255, 'blank': 8256, 'swim': 8257, '325': 8258, 'reprimanded': 8259, 'commemorates': 8260, 'exchanging': 8261, 'bureaucracy': 8262, 'withstand': 8263, 'exhibit': 8264, 'mandelson': 8265, 'jerry': 8266, 'gunpoint': 8267, 'sikhs': 8268, 'disruptions': 8269, 'gems': 8270, 'overloaded': 8271, 'stated': 8272, 'kwan': 8273, 'gardens': 8274, 'qaeda': 8275, 'deserted': 8276, 'amur': 8277, 'kunduz': 8278, 'thinking': 8279, 'lithuanian': 8280, 'armies': 8281, 'victorious': 8282, 'polluted': 8283, 'minus': 8284, 'pretoria': 8285, 'shutdown': 8286, 'spla': 8287, 'disabled': 8288, 'denial': 8289, 'hardware': 8290, 'discontent': 8291, 'possessions': 8292, 'tariff': 8293, 'plantation': 8294, 'subsidized': 8295, 'averaged': 8296, 'inefficient': 8297, 'embarked': 8298, 'extractive': 8299, 'zinc': 8300, 'hood': 8301, 'intolerance': 8302, 'alarcon': 8303, 'valencia': 8304, 'cheap': 8305, 'barricades': 8306, 'precious': 8307, 'alternatives': 8308, 'fearing': 8309, 'packing': 8310, 'respective': 8311, 'kouchner': 8312, 'spate': 8313, 'torturing': 8314, 'hosseini': 8315, 'lessen': 8316, 'participates': 8317, 'safin': 8318, 'perth': 8319, 'fasher': 8320, 'pistol': 8321, 'noordin': 8322, 'treatments': 8323, 'medications': 8324, 'traces': 8325, 'interpreted': 8326, 'impacted': 8327, 'makeup': 8328, 'broadcasters': 8329, 'performances': 8330, 'survival': 8331, 'lined': 8332, 'voices': 8333, 'restructure': 8334, 'closures': 8335, 'mistook': 8336, 'thwarted': 8337, 'italians': 8338, 'reflection': 8339, 'ingredients': 8340, 'halabja': 8341, 'technological': 8342, 'shortfall': 8343, 'reconcile': 8344, 'races': 8345, 'naming': 8346, 'galaxy': 8347, 'ailments': 8348, '1918': 8349, 'dissatisfied': 8350, 'heilongjiang': 8351, 'hotly': 8352, 'refined': 8353, 'hamper': 8354, 'guide': 8355, 'overcame': 8356, 'beautiful': 8357, 'vanished': 8358, 'iskandariyah': 8359, 'holed': 8360, 'karni': 8361, 'incursions': 8362, 'ridiculous': 8363, 'reviving': 8364, 'receives': 8365, 'sheehan': 8366, 'rumors': 8367, 'riddled': 8368, 'inflated': 8369, 'habits': 8370, 'moshe': 8371, 'teachings': 8372, 'skeleton': 8373, 'technician': 8374, 'deliberate': 8375, 'clearly': 8376, 'habitats': 8377, 'demobilized': 8378, 'accelerate': 8379, 'element': 8380, 'unfortunately': 8381, 'dragged': 8382, 'commemoration': 8383, 'zanu': 8384, 'pf': 8385, 'complied': 8386, 'reigning': 8387, 'bottom': 8388, 'occurring': 8389, 'illnesses': 8390, 'spokesperson': 8391, 'beer': 8392, 'teargas': 8393, 'employing': 8394, 'averaging': 8395, 'brazilians': 8396, 'excerpts': 8397, 'signaled': 8398, 'faltering': 8399, 'positioned': 8400, 'frist': 8401, 'arranging': 8402, 'ills': 8403, 'khursheed': 8404, 'incorrect': 8405, 'rico': 8406, 'paving': 8407, 'walkout': 8408, 'stockpiles': 8409, 'viral': 8410, 'reserved': 8411, 'beckett': 8412, 'leak': 8413, 'andaman': 8414, 'coconuts': 8415, 'baghlan': 8416, 'pedro': 8417, 'nick': 8418, 'cowell': 8419, 'prospered': 8420, 'leslie': 8421, 'cautious': 8422, 'endemic': 8423, 'caspian': 8424, 'disappointment': 8425, 'bystander': 8426, 'quantity': 8427, 'livelihoods': 8428, 'governed': 8429, 'ganguly': 8430, 'bowlers': 8431, 'kumble': 8432, 'tips': 8433, 'sayed': 8434, 'fencing': 8435, 'tunceli': 8436, 'roadblock': 8437, 'complaining': 8438, 'accra': 8439, 'communists': 8440, 'dennis': 8441, 'wildfires': 8442, 'gran': 8443, 'triangle': 8444, 'outcry': 8445, 'hrw': 8446, 'danilo': 8447, 'rises': 8448, 'habitat': 8449, 'bugti': 8450, 'outdated': 8451, 'predicting': 8452, 'outages': 8453, 'utility': 8454, 'mukherjee': 8455, 'independents': 8456, 'highlights': 8457, 'tatiana': 8458, 'delegations': 8459, 'kitchen': 8460, 'featured': 8461, 'lavish': 8462, 'concentration': 8463, 'penitentiary': 8464, 'airing': 8465, 'merchant': 8466, 'resettled': 8467, 'lakes': 8468, 'dust': 8469, 'circulated': 8470, 'benchmarks': 8471, 'paulson': 8472, 'remembrance': 8473, 'wade': 8474, 'ram': 8475, 'bingu': 8476, 'foreigner': 8477, 'icrc': 8478, 'conveyed': 8479, 'mix': 8480, 'gunshot': 8481, 'bissau': 8482, 'ramon': 8483, 'freely': 8484, 'cliff': 8485, 'observatory': 8486, 'kilogram': 8487, 'cervical': 8488, 'updated': 8489, 'fundamentalism': 8490, 'buys': 8491, 'contagious': 8492, 'expelling': 8493, 'shrink': 8494, 'clementina': 8495, 'penalties': 8496, 'occasional': 8497, 'perpetual': 8498, 'induced': 8499, 'defensive': 8500, 'succeeding': 8501, 'customer': 8502, 'machimura': 8503, 'presutti': 8504, 'resettlement': 8505, 'enhanced': 8506, 'polled': 8507, 'attendance': 8508, 'gift': 8509, 'bennett': 8510, 'mehsud': 8511, 'surayud': 8512, 'sighting': 8513, 'crescent': 8514, 'meals': 8515, 'extracted': 8516, 'demarcation': 8517, 'desecration': 8518, 'interceptor': 8519, 'scrapped': 8520, 'parliamentarians': 8521, 'royalist': 8522, 'await': 8523, 'sue': 8524, 'sticks': 8525, 'whale': 8526, 'testified': 8527, 'entertainer': 8528, 'liquor': 8529, '1941': 8530, 'consented': 8531, 'accepts': 8532, 'briefed': 8533, 'credited': 8534, 'aged': 8535, 'rover': 8536, 'costly': 8537, 'fundraising': 8538, 'altitude': 8539, 'breathing': 8540, 'crocker': 8541, 'pronk': 8542, 'knocking': 8543, 'fulfill': 8544, 'converting': 8545, 'scheme': 8546, 'impressed': 8547, 'barbaric': 8548, '8th': 8549, 'pleased': 8550, 'yediot': 8551, 'sentencing': 8552, 'orphans': 8553, 'reasonable': 8554, 'costner': 8555, 'concerts': 8556, 'speaks': 8557, 'popularly': 8558, 'stockpile': 8559, 'painful': 8560, 'partnerships': 8561, 'petrochemical': 8562, 'struggles': 8563, 'anticipation': 8564, 'departments': 8565, 'purchasing': 8566, 'catches': 8567, 'ridge': 8568, 'ultimate': 8569, 'raping': 8570, 'profitable': 8571, 'preconditions': 8572, 'traditions': 8573, 'farid': 8574, 'angel': 8575, 'financially': 8576, 'zulima': 8577, 'palacio': 8578, 'specialists': 8579, 'adumim': 8580, '23rd': 8581, 'youtube': 8582, 'bowl': 8583, 'balls': 8584, 'borne': 8585, 'trincomalee': 8586, 'bucharest': 8587, 'traian': 8588, 'marc': 8589, 'convictions': 8590, 'jannati': 8591, 'theory': 8592, 'choices': 8593, 'disposal': 8594, 'ppp': 8595, 'upswing': 8596, 'parent': 8597, '1940': 8598, 'orchestrated': 8599, 'dancing': 8600, 'merely': 8601, 'nowak': 8602, 'tennessee': 8603, 'vault': 8604, 'thick': 8605, 'readings': 8606, 'sein': 8607, 'bunker': 8608, 'societies': 8609, 'erkinbayev': 8610, 'rift': 8611, 'granda': 8612, 'froze': 8613, 'unearthed': 8614, 'bags': 8615, 'dealers': 8616, 'manufacturers': 8617, 'philip': 8618, 'heather': 8619, 'mills': 8620, 'performers': 8621, 'examination': 8622, 'utah': 8623, 'maghreb': 8624, '113': 8625, 'nonpermanent': 8626, 'protester': 8627, 'openness': 8628, 'ljubicic': 8629, 'taha': 8630, 'chandrika': 8631, 'accountability': 8632, 'floating': 8633, 'pulls': 8634, 'pave': 8635, 'forging': 8636, 'pits': 8637, 'jacob': 8638, 'uae': 8639, 'vioxx': 8640, 'rolled': 8641, 'beatings': 8642, 'khalaf': 8643, 'evacuating': 8644, 'pressures': 8645, 'abolished': 8646, 'recurrent': 8647, 'fruit': 8648, 'fowl': 8649, 'wreath': 8650, 'presided': 8651, 'promptly': 8652, 'ganji': 8653, 'unanimous': 8654, 'blanco': 8655, 'tidal': 8656, 'marathon': 8657, 'fukuda': 8658, 'holder': 8659, 'paula': 8660, '27th': 8661, 'rampant': 8662, 'ravi': 8663, 'khanna': 8664, 'defiance': 8665, 'santo': 8666, 'compensated': 8667, 'nbc': 8668, 'violators': 8669, 'disneyland': 8670, 'downing': 8671, 'albums': 8672, 'discount': 8673, 'plachkov': 8674, 'duck': 8675, 'wu': 8676, 'revision': 8677, 'predominately': 8678, 'contrary': 8679, 'starbucks': 8680, 'entitled': 8681, 'curtain': 8682, 'frustration': 8683, 'mayors': 8684, 'qazi': 8685, 'masses': 8686, 'breaches': 8687, 'licensing': 8688, 'equally': 8689, 'cleanup': 8690, 'liters': 8691, 'halting': 8692, 'kiir': 8693, 'infectious': 8694, 'supplement': 8695, '191': 8696, 'nicobar': 8697, 'sindh': 8698, 'canaveral': 8699, 'safarova': 8700, 'safina': 8701, 'charm': 8702, 'wta': 8703, 'exceeding': 8704, 'natanz': 8705, 'eviction': 8706, 'transcripts': 8707, 'tighter': 8708, 'borrowers': 8709, 'maan': 8710, 'frustrated': 8711, 'mandated': 8712, 'companions': 8713, 'rang': 8714, '165': 8715, 'walchhofer': 8716, 'provocative': 8717, 'mujahedin': 8718, 'outraged': 8719, 'hijack': 8720, 'warship': 8721, 'thirteen': 8722, 'haaretz': 8723, 'stripped': 8724, 'advising': 8725, 'prudential': 8726, 'strayed': 8727, 'resilient': 8728, 'totaling': 8729, 'signatures': 8730, 'muttahida': 8731, 'yi': 8732, 'denis': 8733, '270': 8734, 'dispatch': 8735, 'mei': 8736, 'boxes': 8737, 'kot': 8738, 'besides': 8739, 'telerate': 8740, 'evolution': 8741, 'entity': 8742, 'noticed': 8743, 'proud': 8744, 'praising': 8745, 'sachs': 8746, 'infantry': 8747, 'roza': 8748, 'uzbeks': 8749, 'intercontinental': 8750, '162': 8751, 'legendary': 8752, 'safer': 8753, 'hitler': 8754, 'kidwa': 8755, 'asks': 8756, 'helpful': 8757, 'nfl': 8758, '1910': 8759, '1937': 8760, 'col': 8761, 'shall': 8762, 'armistice': 8763, 'paint': 8764, 'acid': 8765, 'boarding': 8766, 'prompt': 8767, 'photographed': 8768, 'menem': 8769, 'azhar': 8770, 'ngos': 8771, 'dar': 8772, 'wei': 8773, 'tampering': 8774, 'pricing': 8775, 'mario': 8776, 'baker': 8777, 'funerals': 8778, 'hoax': 8779, 'sort': 8780, 'nargis': 8781, 'jewelry': 8782, 'poised': 8783, 'legality': 8784, 'thorough': 8785, 'males': 8786, 'archaeologist': 8787, 'osthoff': 8788, 'shaanxi': 8789, 'hemorrhagic': 8790, 'renault': 8791, 'ferrari': 8792, 'intrusion': 8793, 'delaware': 8794, 'liverpool': 8795, 'shanxi': 8796, 'manhunt': 8797, 'sooner': 8798, 'dwyer': 8799, 'blind': 8800, 'aspirations': 8801, 'celebrity': 8802, 'janet': 8803, 'sporting': 8804, 'spills': 8805, 'cambodians': 8806, '1863': 8807, 'colom': 8808, 'metro': 8809, 'divorce': 8810, 'alliot': 8811, 'fours': 8812, 'colorful': 8813, 'theme': 8814, 'slim': 8815, 'tower': 8816, 'hubei': 8817, 'abkhaz': 8818, 'porter': 8819, 'banner': 8820, 'mirror': 8821, 'nebraska': 8822, 'boko': 8823, 'nevis': 8824, 'shirt': 8825, 'patrolled': 8826, 'newest': 8827, 'weaver': 8828, 'bandar': 8829, 'websites': 8830, 'properties': 8831, 'widening': 8832, 'clone': 8833, 'uncle': 8834, 'natsios': 8835, 'cannes': 8836, 'yuganskneftegaz': 8837, 'vomiting': 8838, 'museums': 8839, 'vukovar': 8840, 'fuels': 8841, 'precaution': 8842, 'commemorating': 8843, 'draws': 8844, 'comparing': 8845, 'stirred': 8846, 'doses': 8847, 'expresses': 8848, 'flexibility': 8849, 'latif': 8850, 'proposes': 8851, 'pak': 8852, 'wooden': 8853, 'provoke': 8854, 'kano': 8855, 'shortfalls': 8856, 'aftershock': 8857, 'assuming': 8858, 'daoud': 8859, 'moodie': 8860, 'dravid': 8861, 'atlantis': 8862, 'circulating': 8863, 'fat': 8864, 'surgical': 8865, 'zhao': 8866, 'kosumi': 8867, 'dissent': 8868, 'telephoned': 8869, 'inflicted': 8870, 'papua': 8871, 'pohamba': 8872, 'quarterfinal': 8873, 'unocal': 8874, 'ciudad': 8875, 'disqualified': 8876, 'buffer': 8877, 'trump': 8878, 'igor': 8879, 'amin': 8880, 'chapter': 8881, 'owed': 8882, 'processes': 8883, 'zones': 8884, 'uninhabited': 8885, 'ashraf': 8886, 'competed': 8887, 'kickbacks': 8888, 'favoring': 8889, 'slot': 8890, 'gibbs': 8891, 'wives': 8892, 'skier': 8893, 'mecca': 8894, '1898': 8895, 'cadmium': 8896, 'cloud': 8897, 'falkland': 8898, 'resignations': 8899, 'benigno': 8900, 'ralston': 8901, 'digit': 8902, 'curtailed': 8903, 'manslaughter': 8904, 'favorite': 8905, 'razor': 8906, 'pickens': 8907, 'loose': 8908, 'papacy': 8909, 'pristina': 8910, 'teaching': 8911, 'toiba': 8912, 'combs': 8913, 'prodi': 8914, 'chariot': 8915, 'mussa': 8916, 'hostilities': 8917, 'konare': 8918, 'pepsico': 8919, 'ears': 8920, 'surging': 8921, 'decorations': 8922, 'motorcycles': 8923, 'blogger': 8924, 'stimulant': 8925, 'paz': 8926, 'breakup': 8927, 'grievances': 8928, 'medicare': 8929, 'depressed': 8930, 'bricks': 8931, 'tusk': 8932, 'standby': 8933, 'touchdown': 8934, 'reopening': 8935, 'monk': 8936, 'nissan': 8937, 'miliband': 8938, 'alkhanov': 8939, 'slovaks': 8940, 'hamm': 8941, 'nsa': 8942, 'moore': 8943, 'mueller': 8944, 'greenland': 8945, 'eide': 8946, 'nevirapine': 8947, 'pierce': 8948, 'razuri': 8949, 'equivalent': 8950, 'nias': 8951, 'rap': 8952, 'advisers': 8953, 'transplant': 8954, 'documented': 8955, 'karim': 8956, 'seminary': 8957, 'madrassas': 8958, 'imad': 8959, '370': 8960, 'continuous': 8961, 'hamad': 8962, 'legacy': 8963, 'assuring': 8964, 'zhvania': 8965, 'systematic': 8966, 'hung': 8967, 'probes': 8968, 'crossfire': 8969, 'kfc': 8970, 'mini': 8971, 'syndrome': 8972, 'briefings': 8973, 'therapy': 8974, 'amer': 8975, 'hiring': 8976, 'generous': 8977, 'arbitrary': 8978, 'hardcourt': 8979, 'karlovic': 8980, 'unseeded': 8981, 'nieminen': 8982, 'exert': 8983, 'evacuations': 8984, 'hills': 8985, 'loud': 8986, 'rigs': 8987, 'exclude': 8988, 'decides': 8989, 'snowfall': 8990, 'sunny': 8991, 'denver': 8992, 'skubiszewski': 8993, 'crowley': 8994, 'insight': 8995, 'recipients': 8996, 'helsinki': 8997, 'dismayed': 8998, 'maulana': 8999, 'haidari': 9000, 'withholding': 9001, 'mazar': 9002, 'nikolai': 9003, 'felony': 9004, 'integrated': 9005, 'anonymous': 9006, 'buyer': 9007, 'fun': 9008, 'toronto': 9009, 'stretched': 9010, 'cancelled': 9011, 'liberalize': 9012, 'mercury': 9013, 'yourself': 9014, 'dealings': 9015, 'joschka': 9016, 'combating': 9017, 'contemporary': 9018, 'craft': 9019, 'graham': 9020, 'tools': 9021, 'r': 9022, 'ransacked': 9023, 'prevents': 9024, 'forecasts': 9025, '454': 9026, 'yahya': 9027, 'loving': 9028, 'worship': 9029, 'concentrate': 9030, 'guterres': 9031, 'titled': 9032, 'incite': 9033, 'expel': 9034, 'withdraws': 9035, 'eradicate': 9036, 'screens': 9037, 'patriotic': 9038, 'barnier': 9039, 'parallel': 9040, 'technically': 9041, 'unstable': 9042, 'osh': 9043, 'symbols': 9044, 'cardinals': 9045, 'radioactive': 9046, 'alpine': 9047, 'skiing': 9048, 'tamils': 9049, 'registering': 9050, 'nominations': 9051, 'submarines': 9052, 'informants': 9053, 'congratulate': 9054, '129': 9055, 'religions': 9056, 'forgiveness': 9057, 'spectators': 9058, 'scenes': 9059, 'mindanao': 9060, 'cooking': 9061, 'operator': 9062, 'presumed': 9063, 'primaries': 9064, 'cite': 9065, 'healthcare': 9066, 'credits': 9067, 'alarmed': 9068, 'ogaden': 9069, 'preferences': 9070, 'totaled': 9071, 'malay': 9072, 'fta': 9073, 'sluggish': 9074, 'confronting': 9075, 'gray': 9076, 'prevalence': 9077, 'relieve': 9078, 'hinder': 9079, 'establishes': 9080, 'montreal': 9081, 'solely': 9082, 'touching': 9083, 'profession': 9084, 'marching': 9085, 'magnate': 9086, 'adequately': 9087, 'gorge': 9088, 'privileged': 9089, "n'djamena": 9090, 'prasad': 9091, 'knives': 9092, 'veered': 9093, 'jumblatt': 9094, 'circulation': 9095, 'foods': 9096, 'hooded': 9097, 'edge': 9098, 'gambari': 9099, 'iftikhar': 9100, 'sochi': 9101, 'circle': 9102, 'bet': 9103, 'believers': 9104, 'requesting': 9105, 'boards': 9106, 'julian': 9107, 'laghman': 9108, 'blacks': 9109, 'strokes': 9110, 'tissue': 9111, 'reductions': 9112, 'alarm': 9113, 'meteorologists': 9114, 'shrapnel': 9115, 'gabriele': 9116, 'rini': 9117, 'retrieved': 9118, 'blizzard': 9119, 'ebadi': 9120, 'handles': 9121, 'maine': 9122, 'dir': 9123, '171': 9124, 'innocence': 9125, 'zeng': 9126, 'assert': 9127, 'sciences': 9128, 'ecowas': 9129, 'reluctant': 9130, 'earmarked': 9131, 'documentation': 9132, 'henan': 9133, 'stronach': 9134, 'grenadines': 9135, 'renamed': 9136, 'curacao': 9137, 'accommodate': 9138, 'conquer': 9139, 'dakota': 9140, 'noon': 9141, 'scrambling': 9142, 'gauge': 9143, 'sail': 9144, 'forth': 9145, 'loyalists': 9146, 'mistakes': 9147, 'intergovernmental': 9148, 'daylight': 9149, 'mobilized': 9150, 'terminal': 9151, 'saran': 9152, 'obstructing': 9153, 'proportion': 9154, 'sailed': 9155, 'immunizations': 9156, 'surgeon': 9157, '430': 9158, 'lineup': 9159, 'proves': 9160, 'miles': 9161, 'marketplace': 9162, 'kingpin': 9163, 'counterproductive': 9164, 'compelled': 9165, 'indefinite': 9166, 'scenario': 9167, 'ideal': 9168, 'lingering': 9169, 'plunge': 9170, 'revitalize': 9171, 'backer': 9172, 'contacting': 9173, 'mekong': 9174, 'methamphetamine': 9175, 'evict': 9176, 'durable': 9177, 'fortunes': 9178, 'uneven': 9179, 'contesting': 9180, 'seashore': 9181, 'fulfilled': 9182, 'constituencies': 9183, 'singing': 9184, 'praises': 9185, 'striker': 9186, 'qualify': 9187, 'towers': 9188, 'jeopardize': 9189, 'abdominal': 9190, 'lend': 9191, 'spree': 9192, 'distributors': 9193, 'relying': 9194, 'cracked': 9195, 'anxious': 9196, 'eroded': 9197, 'wise': 9198, 'nadal': 9199, 'ankle': 9200, 'liberalization': 9201, 'termination': 9202, 'wracked': 9203, 'monrovia': 9204, 'richardson': 9205, 'endorse': 9206, 'hispanic': 9207, 'wary': 9208, 'lent': 9209, 'masks': 9210, 'filmed': 9211, 'ingushetia': 9212, 'caledonia': 9213, 'tactic': 9214, 'drastically': 9215, 'asad': 9216, 'fleets': 9217, 'entreated': 9218, 'copy': 9219, 'inaugural': 9220, 'unsafe': 9221, 'shelled': 9222, 'rehman': 9223, 'discouraging': 9224, 'uniformed': 9225, 'hopefuls': 9226, 'tueni': 9227, 'nice': 9228, '1830': 9229, 'bureaucratic': 9230, 'ravine': 9231, 'arsenals': 9232, '337': 9233, 'stumps': 9234, 'matthew': 9235, 'anil': 9236, 'nabih': 9237, 'berri': 9238, 'mutilated': 9239, 'pains': 9240, 'consolidation': 9241, 'lynndie': 9242, 'bias': 9243, 'escalate': 9244, 'entrenched': 9245, 'meal': 9246, 'rainy': 9247, 'depletion': 9248, 'stabilized': 9249, 'inspected': 9250, 'forests': 9251, 'environmentalists': 9252, 'nativity': 9253, 'registry': 9254, 'unexpectedly': 9255, 'speculate': 9256, 'charred': 9257, 'assessed': 9258, 'unjustified': 9259, 'privatizations': 9260, 'javed': 9261, 'max': 9262, 'grandmother': 9263, 'treats': 9264, 'electrocuted': 9265, 'destroyer': 9266, 'clad': 9267, 'jhangvi': 9268, 'multan': 9269, 'abyan': 9270, '111': 9271, 'poles': 9272, 'spanta': 9273, 'morgan': 9274, 'sheltering': 9275, 'westward': 9276, 'underlying': 9277, 'invasions': 9278, 'tuna': 9279, 'arose': 9280, 'swam': 9281, 'alter': 9282, 'balloon': 9283, 'batteries': 9284, 'malnutrition': 9285, 'divert': 9286, 'parliamentarian': 9287, 'debating': 9288, 'henry': 9289, 'distress': 9290, 'pessimistic': 9291, 'avoided': 9292, 'motorists': 9293, 'extermination': 9294, 'hostility': 9295, 'flaws': 9296, 'syrians': 9297, 'justin': 9298, 'profiling': 9299, 'revise': 9300, 'redistribute': 9301, 'stems': 9302, 'accompanying': 9303, 'minas': 9304, 'eric': 9305, 'minimize': 9306, 'conte': 9307, 'installing': 9308, 'landmines': 9309, 'whoever': 9310, 'fog': 9311, '166': 9312, 'douste': 9313, 'blazy': 9314, 'acquire': 9315, 'logging': 9316, 'rogers': 9317, 'holders': 9318, 'educated': 9319, 'restrain': 9320, 'coaches': 9321, 'pleasure': 9322, 'complicated': 9323, 'clerk': 9324, 'pathogenic': 9325, 'kaduna': 9326, 'anders': 9327, 'rasmussen': 9328, 'pul': 9329, 'yielded': 9330, 'brands': 9331, 'patience': 9332, 'hmong': 9333, 'filling': 9334, 'whitman': 9335, 'plateau': 9336, 'loading': 9337, 'psychological': 9338, 'displacing': 9339, 'lander': 9340, 'resident': 9341, 'panjwayi': 9342, 'arming': 9343, 'reputed': 9344, 'rioted': 9345, 'bayelsa': 9346, 'berger': 9347, '1950s': 9348, 'argues': 9349, 'alexei': 9350, 'camilla': 9351, 'glimpse': 9352, 'aslam': 9353, 'initiate': 9354, 'turki': 9355, 'gotten': 9356, 'graphic': 9357, 'clampdown': 9358, 'span': 9359, 'brawl': 9360, 'presenting': 9361, 'sped': 9362, 'confrontations': 9363, 'lara': 9364, 'climb': 9365, 'affirmed': 9366, 'gilbert': 9367, 'victories': 9368, 'relinquished': 9369, 'reversal': 9370, 'competitiveness': 9371, 'sacrifices': 9372, 'leaks': 9373, 'cooling': 9374, 'plunging': 9375, 'mahinda': 9376, 'rajapakse': 9377, 'intimidate': 9378, 'azimi': 9379, 'crater': 9380, 'pregnancy': 9381, 'leta': 9382, 'fincher': 9383, 'woo': 9384, 'organizer': 9385, '1958': 9386, 'isolate': 9387, 'looters': 9388, 'enduring': 9389, 'bongo': 9390, 'bangladeshis': 9391, 'navarro': 9392, 'letting': 9393, 'unreported': 9394, 'bodman': 9395, 'refiners': 9396, 'zahir': 9397, 'finishing': 9398, 'baghdadi': 9399, 'shocking': 9400, 'devoted': 9401, 'conform': 9402, 'fahd': 9403, 'hasan': 9404, '101st': 9405, 'slept': 9406, 'constant': 9407, 'plight': 9408, 'blessing': 9409, 'breached': 9410, 'fledgling': 9411, 'mahee': 9412, 'marketing': 9413, 'reneged': 9414, 'demolition': 9415, 'ann': 9416, 'tome': 9417, 'rogue': 9418, 'alvarez': 9419, 'succeeds': 9420, 'l': 9421, 'aluminum': 9422, 'expatriate': 9423, 'comparable': 9424, 'nauru': 9425, 'autocratic': 9426, 'discounted': 9427, 'illegitimate': 9428, 'instance': 9429, 'typical': 9430, 'commissioned': 9431, 'yuganskneftegas': 9432, 'maale': 9433, 'delp': 9434, 'protective': 9435, 'anabel': 9436, 'cho': 9437, 'embrace': 9438, 'distances': 9439, 'clergy': 9440, 'fixing': 9441, 'butt': 9442, 'correctly': 9443, 'waheed': 9444, 'arshad': 9445, 'anna': 9446, 'politkovskaya': 9447, 'fred': 9448, 'mechanical': 9449, 'grozny': 9450, 'discredit': 9451, 'rudd': 9452, 'guam': 9453, 'salehi': 9454, 'nursultan': 9455, 'miranshah': 9456, 'lightly': 9457, 'hainan': 9458, '141': 9459, 'advances': 9460, 'nonetheless': 9461, 'pocket': 9462, 'tearing': 9463, 'wished': 9464, 'thief': 9465, 'raffaele': 9466, 'impunity': 9467, 'cleaner': 9468, 'h5n2': 9469, 'carmona': 9470, 'comic': 9471, 'ringleader': 9472, 'fourteen': 9473, 'brisbane': 9474, 'overwhelmed': 9475, 'blasted': 9476, 'droughts': 9477, 'comoros': 9478, 'verbal': 9479, 'courthouse': 9480, 'mccartney': 9481, 'candles': 9482, 'indonesians': 9483, 'miran': 9484, 'periodic': 9485, 'cirque': 9486, 'ca': 9487, 'baja': 9488, 'undergone': 9489, 'rupiah': 9490, 'vojislav': 9491, 'emigration': 9492, 'ecuadorian': 9493, 'tire': 9494, 'staple': 9495, 'improper': 9496, 'poorer': 9497, 'asadabad': 9498, 'balanced': 9499, 'lendu': 9500, 'barinov': 9501, 'hersh': 9502, 'athletics': 9503, 'moi': 9504, 'stall': 9505, 'knesset': 9506, 'fisheries': 9507, 'lucio': 9508, 'cement': 9509, 'outlines': 9510, 'resettle': 9511, 'bouteflika': 9512, 'camara': 9513, 'appreciation': 9514, 'prospect': 9515, 'copra': 9516, 'coins': 9517, 'medieval': 9518, '1865': 9519, 'emigrants': 9520, 'reader': 9521, 'grounding': 9522, 'pigeons': 9523, 'flattened': 9524, 'stab': 9525, 'ahmet': 9526, 'justification': 9527, 'farmland': 9528, 'photojournalist': 9529, 'reviews': 9530, 'beliefs': 9531, 'commonly': 9532, 'acquiring': 9533, 'latvian': 9534, 'raymond': 9535, 'thigh': 9536, 'identifying': 9537, 'hallums': 9538, 'shipyard': 9539, 'sustainability': 9540, 'tarasyuk': 9541, 'doubling': 9542, 'facts': 9543, '145': 9544, 'nguyen': 9545, 'illicit': 9546, 'bremer': 9547, 'smithsonian': 9548, 'wholesale': 9549, 'sultanate': 9550, 'uprisings': 9551, 'warden': 9552, 'rage': 9553, 'elementary': 9554, 'shandong': 9555, 'transmit': 9556, 'exception': 9557, 'amazon': 9558, 'madrazo': 9559, 'defunct': 9560, 'wayne': 9561, 'needy': 9562, 'unused': 9563, 'soar': 9564, 'yuriy': 9565, 'interviewer': 9566, 'shorter': 9567, 'jason': 9568, 'respectively': 9569, 'barno': 9570, 'hillah': 9571, 'beheading': 9572, 'transform': 9573, 'eldest': 9574, 'hayat': 9575, 'solutions': 9576, 'innovative': 9577, 'ron': 9578, 'survivor': 9579, 'drunk': 9580, 'forever': 9581, 'minded': 9582, 'criteria': 9583, 'introduce': 9584, 'jay': 9585, 'inch': 9586, 'teeth': 9587, 'cleaned': 9588, 'executing': 9589, 'crying': 9590, 'robotic': 9591, 'salva': 9592, 'wider': 9593, 'mao': 9594, 'discover': 9595, 'aliens': 9596, '167': 9597, 'kerala': 9598, 'stakes': 9599, 'lucie': 9600, 'dinara': 9601, 'ridden': 9602, 'economically': 9603, 'vazquez': 9604, 'montevideo': 9605, 'nevada': 9606, 'uighurs': 9607, 'uighur': 9608, 'fruitful': 9609, 'carmaker': 9610, 'robot': 9611, 'harmed': 9612, 'outsiders': 9613, 'temperature': 9614, 'diving': 9615, 'divides': 9616, 'item': 9617, 'shinzo': 9618, 'wielgus': 9619, 'telesur': 9620, 'attach': 9621, 'bode': 9622, 'rahlves': 9623, 'anton': 9624, 'appointing': 9625, 'ultimatum': 9626, 'container': 9627, 'mikheil': 9628, 'heinous': 9629, 'leftists': 9630, 'bravo': 9631, 'thin': 9632, 'blasting': 9633, 'intelogic': 9634, 'stating': 9635, 'urdu': 9636, 'sassou': 9637, 'tong': 9638, 'don': 9639, 'alston': 9640, 'alejandro': 9641, 'toledo': 9642, 'grateful': 9643, 'andrei': 9644, 'speeding': 9645, 'secularists': 9646, 'tendered': 9647, 'aloft': 9648, 'reminded': 9649, 'narrated': 9650, 'tenure': 9651, '1954': 9652, '112': 9653, 'soup': 9654, 'bratislava': 9655, 'aboul': 9656, 'hadassah': 9657, 'hemorrhage': 9658, 'function': 9659, 'historian': 9660, 'damrey': 9661, 'updates': 9662, '260': 9663, 'euros': 9664, 'frequency': 9665, 'khz': 9666, 'crimean': 9667, 'reinforced': 9668, 'paso': 9669, 'imbalances': 9670, 'decent': 9671, 'underemployment': 9672, 'tuareg': 9673, 'hail': 9674, 'durban': 9675, 'improperly': 9676, 'warmer': 9677, 'spectrum': 9678, 'bombardment': 9679, 'haifa': 9680, 'shrinking': 9681, 'monde': 9682, 'pounding': 9683, 'abdulkadir': 9684, 'es': 9685, 'salaam': 9686, 'jokonya': 9687, 'regiment': 9688, 'tremendous': 9689, 'osce': 9690, 'regardless': 9691, 'casablanca': 9692, 'issuing': 9693, 'clans': 9694, 'angolan': 9695, 'indirectly': 9696, 'tsang': 9697, 'intent': 9698, 'ruegen': 9699, 'abductees': 9700, 'ottawa': 9701, 'susanne': 9702, 'thoughts': 9703, 'polynesian': 9704, 'dynamic': 9705, 'writers': 9706, 'spam': 9707, 'thank': 9708, 'hyderabad': 9709, 'akihito': 9710, '1944': 9711, 'reservoir': 9712, 'glory': 9713, 'conocophillips': 9714, 'clinics': 9715, 'turk': 9716, 'grief': 9717, 'sided': 9718, 'eavesdropping': 9719, 'rulings': 9720, 'ancic': 9721, 'hekmatyar': 9722, 'emmanuel': 9723, 'akitani': 9724, 'husaybah': 9725, '320': 9726, 'wang': 9727, 'mohamud': 9728, 'posting': 9729, 'sixty': 9730, 'measuring': 9731, 'joyful': 9732, 'ghazi': 9733, 'parole': 9734, 'phnom': 9735, 'penh': 9736, 'myth': 9737, 'nuns': 9738, 'oscar': 9739, 'symonds': 9740, 'michele': 9741, 'batsman': 9742, 'rogge': 9743, 'sebastian': 9744, 'lowering': 9745, 'advertisements': 9746, 'ad': 9747, 'uefa': 9748, 'postponing': 9749, 'kansas': 9750, 'slave': 9751, 'anguilla': 9752, 'brutally': 9753, 'parkinson': 9754, 'duelfer': 9755, 'karnataka': 9756, 'accreditation': 9757, 'budapest': 9758, 'adhere': 9759, 'gestures': 9760, 'overturn': 9761, 'effectiveness': 9762, 'relieved': 9763, 'opener': 9764, 'reunited': 9765, 'flocks': 9766, 'processed': 9767, 'amorim': 9768, 'maarten': 9769, 'fifths': 9770, 'antilles': 9771, 'constitute': 9772, '1964': 9773, 'sonia': 9774, 'beside': 9775, 'flurry': 9776, 'earn': 9777, 'frances': 9778, 'pinera': 9779, 'seated': 9780, 'relics': 9781, 'saints': 9782, 'weeklong': 9783, 'bauer': 9784, 'conquered': 9785, 'hawass': 9786, 'enormous': 9787, 'eager': 9788, 'suppressed': 9789, 'husseinov': 9790, 'provocation': 9791, 'huygens': 9792, 'roque': 9793, 'genetic': 9794, 'egg': 9795, 'abandoning': 9796, 'rightly': 9797, 'sampling': 9798, '1939': 9799, 'dp': 9800, 'bakiev': 9801, 'brasilia': 9802, 'turbulent': 9803, 'mental': 9804, 'confronted': 9805, 'unsealed': 9806, 'foreclosure': 9807, 'cruz': 9808, 'fend': 9809, 'profitability': 9810, 'renewing': 9811, 'prefecture': 9812, 'sorry': 9813, 'demolished': 9814, 'daughters': 9815, 'outage': 9816, 'anatoly': 9817, 'haq': 9818, 'kampala': 9819, 'undetermined': 9820, 'pat': 9821, 'protein': 9822, '132': 9823, 'takers': 9824, 'disturbed': 9825, 'inspire': 9826, 'widen': 9827, 'bacteria': 9828, 'caps': 9829, 'dprk': 9830, '1950': 9831, 'myung': 9832, 'bak': 9833, 'guyana': 9834, 'abolition': 9835, 'downplayed': 9836, 'auspices': 9837, 'cdc': 9838, 'trans': 9839, 'bakery': 9840, 'nujoma': 9841, 'dawei': 9842, 'construct': 9843, 'scarce': 9844, 'outer': 9845, 'renewal': 9846, 'bull': 9847, 'mentioning': 9848, 'anfal': 9849, 'shortened': 9850, 'suffocation': 9851, 'barcodes': 9852, 'bolivians': 9853, 'silvan': 9854, 'padilla': 9855, 'lone': 9856, 'shaft': 9857, 'mardi': 9858, 'gras': 9859, 'ngwira': 9860, 'iles': 9861, 'dolphin': 9862, 'stewart': 9863, 'goats': 9864, 'donation': 9865, 'preacher': 9866, 'infamous': 9867, 'seismologists': 9868, 'tianjin': 9869, '550': 9870, 'protects': 9871, 'referees': 9872, 'colder': 9873, 'belief': 9874, 'auckland': 9875, 'leasing': 9876, 'flour': 9877, '106': 9878, 'chaco': 9879, '1932': 9880, 'noise': 9881, 'bei': 9882, 'caucus': 9883, 'privileges': 9884, 'gatlin': 9885, 'aided': 9886, '1955': 9887, 'backlash': 9888, 'wheeler': 9889, 'agca': 9890, 'elton': 9891, 'poisonous': 9892, 'smooth': 9893, 'securities': 9894, 'francis': 9895, 'qari': 9896, 'abubakar': 9897, 'borrow': 9898, 'cordoned': 9899, 'haas': 9900, 'hydrocarbons': 9901, 'conquest': 9902, 'halliburton': 9903, 'advocating': 9904, 'danielle': 9905, 'manning': 9906, 'fences': 9907, 'cardenas': 9908, 'lip': 9909, 'rohmer': 9910, 'absent': 9911, 'erik': 9912, 'solheim': 9913, 'runners': 9914, 'rezko': 9915, 'interrogations': 9916, 'adjust': 9917, 'consciousness': 9918, 'conferred': 9919, 'pluto': 9920, 'fillon': 9921, 'abusive': 9922, 'consult': 9923, 'molina': 9924, 'zia': 9925, 'isle': 9926, 'aswat': 9927, 'wielding': 9928, 'raffarin': 9929, 'mukasey': 9930, 'jos': 9931, 'homicide': 9932, 'zaidi': 9933, 'sandinista': 9934, 'hercules': 9935, 'ramda': 9936, 'rsf': 9937, 'concede': 9938, 'djindjic': 9939, 'sticking': 9940, 'insecurity': 9941, 'expansionary': 9942, 'filipinos': 9943, 'mashaie': 9944, 'constituency': 9945, '101': 9946, 'breakfast': 9947, 'stern': 9948, 'exams': 9949, 'jameson': 9950, 'grill': 9951, 'fiery': 9952, '747': 9953, 'bakri': 9954, 'cosatu': 9955, 'mullen': 9956, 'traded': 9957, 'affiliates': 9958, 'fda': 9959, 'moiseyev': 9960, 'reyna': 9961, 'steele': 9962, 'acceptable': 9963, 'witty': 9964, 'flea': 9965, 'pius': 9966, 'ltte': 9967, 'axum': 9968, 'pastrana': 9969, 'dome': 9970, 'papadopoulos': 9971, 'designs': 9972, 'srebotnik': 9973, 'mourn': 9974, 'nurses': 9975, 'nun': 9976, 'badghis': 9977, 'mikati': 9978, 'fitting': 9979, 'jankovic': 9980, 'falun': 9981, 'gong': 9982, 'gamsakhurdia': 9983, 'asteroid': 9984, 'bishara': 9985, 'upscale': 9986, 'python': 9987, 'cerkez': 9988, 'trench': 9989, 'tripura': 9990, 'jolie': 9991, 'mowlam': 9992, 'defect': 9993, 'partition': 9994, 'hospitalization': 9995, 'x': 9996, 'railways': 9997, 'disturbance': 9998, 'raila': 9999, '\x85': 10000, '\x94': 10001, 'truly': 10002, 'academics': 10003, 'attendees': 10004, 'hiriart': 10005, 'chiapas': 10006, 'mysterious': 10007, 'execute': 10008, 'contradicts': 10009, 'kinds': 10010, 'foundations': 10011, 'immunization': 10012, 'specially': 10013, 'dire': 10014, 'deprived': 10015, 'sleep': 10016, 'diversifying': 10017, 'siphoning': 10018, 'constraints': 10019, 'unequal': 10020, 'peasant': 10021, 'lesson': 10022, 'potato': 10023, 'sacks': 10024, 'pink': 10025, 'mansion': 10026, 'geoff': 10027, 'testifying': 10028, 'kupwara': 10029, 'fried': 10030, 'sanders': 10031, 'platforms': 10032, 'pleas': 10033, 'frivolous': 10034, 'comfortable': 10035, 'filipino': 10036, 'conferences': 10037, 'alex': 10038, 'beset': 10039, 'inexpensive': 10040, 'abed': 10041, 'aghazadeh': 10042, 'complications': 10043, 'exhumed': 10044, 'rolling': 10045, 'lincoln': 10046, 'raiders': 10047, 'lleyton': 10048, 'florian': 10049, 'andreas': 10050, 'jarkko': 10051, 'kenneth': 10052, 'mandy': 10053, 'specialty': 10054, 'enforcing': 10055, 'injected': 10056, 'tuz': 10057, 'visibility': 10058, 'sympathy': 10059, 'archaeological': 10060, 'exercising': 10061, 'assemble': 10062, 'humiliating': 10063, 'robbing': 10064, 'deepen': 10065, 'proving': 10066, 'boosts': 10067, 'piano': 10068, 'plague': 10069, 'cartoonist': 10070, 'editors': 10071, 'azeris': 10072, 'mi': 10073, 'thrift': 10074, 'fertile': 10075, 'highlands': 10076, 'alumina': 10077, 'estates': 10078, 'injustice': 10079, 'nest': 10080, 'trampled': 10081, 'paintings': 10082, 'artwork': 10083, 'clay': 10084, 'plain': 10085, 'prijedor': 10086, 'blessings': 10087, 'abraham': 10088, 'nicosia': 10089, 'impartial': 10090, 'telecommunication': 10091, 'provider': 10092, 'diwaniyah': 10093, 'ruined': 10094, 'dioxin': 10095, 'groin': 10096, 'bye': 10097, 'kommersant': 10098, 'sinhalese': 10099, 'missionary': 10100, 'relaxed': 10101, 'qalqiliya': 10102, 'nimroz': 10103, 'naqib': 10104, 'adamkus': 10105, 'vastly': 10106, 'complying': 10107, 'pullback': 10108, 'swaths': 10109, 'firmly': 10110, 'criticisms': 10111, 'challengers': 10112, 'hiroyuki': 10113, 'hosoda': 10114, 'occurrence': 10115, 'saddened': 10116, 'ike': 10117, 'chooses': 10118, 'icon': 10119, 'franklin': 10120, 'roosevelt': 10121, 'paralyzed': 10122, 'shabelle': 10123, 'seller': 10124, 'premises': 10125, 'widened': 10126, 'phosphates': 10127, 'catalyst': 10128, 'dec': 10129, 'spared': 10130, 'connections': 10131, 'neglect': 10132, 'diminished': 10133, 'peaked': 10134, 'module': 10135, 'soyuz': 10136, 'gaps': 10137, 'gholam': 10138, 'haddad': 10139, 'endanger': 10140, 'sino': 10141, 'exceptional': 10142, 'censor': 10143, 'jams': 10144, 'airfield': 10145, 'typhoons': 10146, 'splinter': 10147, 'gleneagles': 10148, 'implicates': 10149, 'roaming': 10150, 'assignments': 10151, 'bombmaker': 10152, 'hopman': 10153, 'hijacker': 10154, 'surrendering': 10155, 'accomplices': 10156, 'avenge': 10157, 'subpoena': 10158, 'responses': 10159, 'madeleine': 10160, 'farewell': 10161, 'cholesterol': 10162, 'bayji': 10163, 'guided': 10164, 'drawings': 10165, 'objection': 10166, 'foes': 10167, 'prohibiting': 10168, 'instrument': 10169, 'vigilant': 10170, 'uphold': 10171, 'imposition': 10172, 'tracked': 10173, 'fundamentalist': 10174, 'recorder': 10175, 'tijuana': 10176, 'blatant': 10177, 'zawahri': 10178, 'suspensions': 10179, 'punishments': 10180, 'superiors': 10181, 'hindering': 10182, 'reacting': 10183, 'unconditional': 10184, 'haste': 10185, '22nd': 10186, 'withhold': 10187, 'satisfactory': 10188, '1783': 10189, 'slovenes': 10190, 'distanced': 10191, 'absorbed': 10192, 'mainstays': 10193, 'ins': 10194, 'complicate': 10195, 'prefer': 10196, 'bramble': 10197, 'hedge': 10198, 'vain': 10199, 'personality': 10200, 'junk': 10201, 'auditing': 10202, 'annulled': 10203, 'corrected': 10204, 'surges': 10205, 'mulford': 10206, 'shyam': 10207, 'maize': 10208, 'semlow': 10209, 'mastery': 10210, 'harriet': 10211, 'democratization': 10212, 'secondhand': 10213, 'rugova': 10214, 'contrast': 10215, 'cindy': 10216, 'aye': 10217, 'daniele': 10218, 'repubblica': 10219, 'sentiments': 10220, 'reversing': 10221, 'covertly': 10222, 'signaling': 10223, 'tnk': 10224, 'enrique': 10225, 'ramos': 10226, '227': 10227, 'oumarou': 10228, 'amadou': 10229, 'nardiello': 10230, 'rejoin': 10231, 'instill': 10232, 'paddy': 10233, 'bow': 10234, 'assure': 10235, 'tender': 10236, 'anemic': 10237, 'railroad': 10238, 'specifics': 10239, 'chinamasa': 10240, '25th': 10241, 'inconclusive': 10242, 'moussaoui': 10243, 'accelerated': 10244, 'davos': 10245, 'stormy': 10246, 'availability': 10247, 'clamp': 10248, 'seventeen': 10249, 'petty': 10250, 'diversity': 10251, 'siberian': 10252, 'feast': 10253, 'kings': 10254, 'infiltration': 10255, 'imposes': 10256, 'tries': 10257, 'normalize': 10258, 'erosion': 10259, 'aggravated': 10260, 'affiliate': 10261, 'universe': 10262, 'authenticated': 10263, 'hispanics': 10264, 'caucuses': 10265, 'revelers': 10266, 'pack': 10267, 'samba': 10268, 'costumes': 10269, 'showdown': 10270, 'filibusters': 10271, 'jetliners': 10272, 'geo': 10273, 'sirnak': 10274, 'growers': 10275, 'rewrite': 10276, 'gallup': 10277, 'beachfront': 10278, 'dishes': 10279, 'punching': 10280, 'handcuffs': 10281, 'ekmeleddin': 10282, 'ihsanoglu': 10283, 'rove': 10284, 'recapture': 10285, 'spiraling': 10286, 'absolutely': 10287, 'implicating': 10288, 'impede': 10289, 'introducing': 10290, 'heal': 10291, 'prescribe': 10292, 'bitterly': 10293, 'lamented': 10294, 'skirmish': 10295, 'ejected': 10296, 'lagging': 10297, 'requirement': 10298, 'exhaustion': 10299, 'spends': 10300, 'ghanaian': 10301, 'conceived': 10302, 'occupy': 10303, 'mistreating': 10304, 'decapitated': 10305, 'likelihood': 10306, 'amanullah': 10307, 'differs': 10308, 'sulawesi': 10309, 'tenerife': 10310, 'wildfire': 10311, 'autocracy': 10312, 'subsided': 10313, 'chart': 10314, 'applause': 10315, 'microphone': 10316, 'supervised': 10317, 'yasukuni': 10318, 'liners': 10319, 'kate': 10320, 'mccann': 10321, 'celebrities': 10322, 'regarded': 10323, 'occupants': 10324, 'prosecuting': 10325, 'ibero': 10326, 'smoothly': 10327, 'snapped': 10328, 'ken': 10329, 'faulty': 10330, 'schemes': 10331, 'cheema': 10332, 'jirga': 10333, 'precinct': 10334, 'pensions': 10335, 'sanctioned': 10336, 'cracks': 10337, 'residency': 10338, 'bypass': 10339, 'recovers': 10340, 'collective': 10341, 'pranab': 10342, 'bombarded': 10343, 'skepticism': 10344, 'intensifying': 10345, 'barring': 10346, 'bargaining': 10347, 'chip': 10348, 'pairs': 10349, 'grabs': 10350, 'pipe': 10351, 'unverifiable': 10352, 'sprayed': 10353, 'dadfar': 10354, 'qureshi': 10355, 'mere': 10356, 'pegged': 10357, 'procurement': 10358, 'remarkable': 10359, 'cleansing': 10360, 'perished': 10361, 'hurts': 10362, 'nile': 10363, 'frame': 10364, 'boroujerdi': 10365, 'desk': 10366, 'forgiven': 10367, 'luge': 10368, 'shattering': 10369, 'swollen': 10370, 'shrank': 10371, 'salih': 10372, 'josh': 10373, 'yankees': 10374, 'ropes': 10375, 'sounded': 10376, 'feuding': 10377, 'lessons': 10378, 'impression': 10379, 'spirits': 10380, 'geneina': 10381, 'mathieu': 10382, 'babies': 10383, 'jaroslaw': 10384, 'sickened': 10385, 'malisse': 10386, 'guillermo': 10387, 'plaguing': 10388, 'sheets': 10389, 'practicing': 10390, 'treasure': 10391, 'bold': 10392, 'print': 10393, 'protections': 10394, 'badr': 10395, 'cristina': 10396, 'rebate': 10397, 'sana': 10398, 'yousifi': 10399, 'incurable': 10400, 'restraints': 10401, 'conceded': 10402, 'crane': 10403, 'widows': 10404, 'sprint': 10405, 'der': 10406, 'canyon': 10407, 'ecosystem': 10408, 'downstream': 10409, 'discovering': 10410, 'philippe': 10411, 'ingrid': 10412, 'publicity': 10413, 'konstantin': 10414, 'luzon': 10415, 'weightlifting': 10416, 'convertible': 10417, 'nominal': 10418, 'efta': 10419, 'grey': 10420, 'vigorous': 10421, 'pounds': 10422, 'centralized': 10423, 'tow': 10424, 'insured': 10425, 'softball': 10426, 'cure': 10427, 'improves': 10428, 'disinfected': 10429, 'applies': 10430, 'fogh': 10431, 'battering': 10432, 'nova': 10433, 'grows': 10434, 'nobutaka': 10435, 'nimeiri': 10436, 'gunship': 10437, 'airstrip': 10438, 'bryan': 10439, 'friction': 10440, 'fitzgerald': 10441, 'dresden': 10442, 'yorker': 10443, 'plame': 10444, 'flipped': 10445, 'drenched': 10446, 'garmser': 10447, 'tangerang': 10448, 'transmissible': 10449, 'zhari': 10450, 'expands': 10451, 'warmest': 10452, 'olli': 10453, 'heinonen': 10454, 'mau': 10455, '1952': 10456, 'undersea': 10457, 'rattle': 10458, 'retracted': 10459, 'onlookers': 10460, 'relation': 10461, 'sherif': 10462, 'accumulated': 10463, 'wo': 10464, 'inviting': 10465, 'headlines': 10466, 'provoking': 10467, 'recount': 10468, 'greenpeace': 10469, '280': 10470, 'loyalty': 10471, 'katowice': 10472, 'wine': 10473, 'nearest': 10474, 'carat': 10475, 'sidr': 10476, 'derives': 10477, 'felix': 10478, 'privatize': 10479, 'unhappy': 10480, 'pasture': 10481, 'punishing': 10482, 'thereafter': 10483, 'barbara': 10484, 'disgusting': 10485, 'secede': 10486, 'yu': 10487, 'adams': 10488, 'stabbing': 10489, 'overthrown': 10490, 'yong': 10491, 'herbert': 10492, 'specified': 10493, 'oppressed': 10494, 'strides': 10495, 'joaquin': 10496, 'valls': 10497, 'bishops': 10498, 'gravity': 10499, '1924': 10500, 'segolene': 10501, 'encounter': 10502, 'loaned': 10503, 'susan': 10504, 'overhaul': 10505, 'poppies': 10506, 'unwelcome': 10507, 'comrades': 10508, 'ridicule': 10509, 'demolish': 10510, 'jalgaon': 10511, 'seals': 10512, 'hearts': 10513, 'therefore': 10514, 'evicted': 10515, 'scenarios': 10516, 'advises': 10517, 'stuttgart': 10518, 'vaz': 10519, 'almeida': 10520, 'torrijos': 10521, 'splitting': 10522, 'versus': 10523, 'repatriate': 10524, 'arkansas': 10525, 'horseback': 10526, 'begged': 10527, 'physicist': 10528, 'bucket': 10529, 'solved': 10530, 'modeled': 10531, 'harmony': 10532, 'billingslea': 10533, 'creates': 10534, 'roadmap': 10535, 'ultranationalist': 10536, 'charcoal': 10537, 'confederations': 10538, 'julia': 10539, 'robertson': 10540, 'pascal': 10541, 'lakshman': 10542, 'kadirgamar': 10543, 'aslan': 10544, 'consistently': 10545, 'unconfirmed': 10546, 'shaikh': 10547, 'facilitating': 10548, 'nano': 10549, 'sermon': 10550, 'federico': 10551, 'lombardi': 10552, 'samir': 10553, 'rabbi': 10554, 'judaism': 10555, 'hearted': 10556, 'sundown': 10557, 'reed': 10558, 'oct': 10559, 'delphi': 10560, 'rebounding': 10561, '1814': 10562, '1905': 10563, 'referenda': 10564, 'rpf': 10565, 'bent': 10566, 'kigali': 10567, 'edwards': 10568, 'frontrunner': 10569, 'inspiration': 10570, 'chittagong': 10571, 'drain': 10572, 'quadruple': 10573, 'tournaments': 10574, 'breath': 10575, 'variant': 10576, 'reformers': 10577, 'obligation': 10578, 'bernie': 10579, 'disagree': 10580, 'killers': 10581, 'titanosaurs': 10582, 'jordanians': 10583, 'dodd': 10584, 'departed': 10585, 'receding': 10586, 'instructions': 10587, 'airlifted': 10588, 'pleading': 10589, 'contend': 10590, 'swede': 10591, 'robin': 10592, 'frequencies': 10593, 'tsunamis': 10594, 'artificial': 10595, 'slipping': 10596, 'rated': 10597, 'wiping': 10598, 'partisan': 10599, 'pays': 10600, '230': 10601, 'airlift': 10602, 'nabil': 10603, 'jolted': 10604, 'bam': 10605, 'administering': 10606, 'excluded': 10607, 'nouakchott': 10608, '144': 10609, 'defaulted': 10610, 'navigation': 10611, 'sgrena': 10612, 'engulfed': 10613, 'nath': 10614, 'truckers': 10615, 'ride': 10616, 'transforming': 10617, 'lukoil': 10618, 'neverland': 10619, 'lausanne': 10620, 'accorded': 10621, 'jeep': 10622, 'clogged': 10623, 'elias': 10624, 'mena': 10625, 'olsson': 10626, 'wreck': 10627, 'brazzaville': 10628, 'deployments': 10629, 'folk': 10630, 'casey': 10631, 'sweeps': 10632, 'shoppers': 10633, 'erez': 10634, 'subdue': 10635, 'consensual': 10636, 'defines': 10637, 'invite': 10638, 'debates': 10639, 'fatwa': 10640, 'taba': 10641, '1928': 10642, 'urine': 10643, 'enable': 10644, 'undercut': 10645, 'bedouin': 10646, 'stir': 10647, 'frightened': 10648, 'jakob': 10649, 'atta': 10650, 'teamed': 10651, 'translation': 10652, '155': 10653, 'tokelau': 10654, 'underdeveloped': 10655, 'encouragement': 10656, 'dwellings': 10657, 'arlington': 10658, '137': 10659, 'escaping': 10660, 'homelands': 10661, 'ruthless': 10662, 'necdet': 10663, 'sezer': 10664, 'swedes': 10665, 'consuming': 10666, 'projections': 10667, '1867': 10668, 'flies': 10669, 'kathleen': 10670, 'noguchi': 10671, 'defused': 10672, 'streamline': 10673, 'affluent': 10674, 'fairness': 10675, 'quitting': 10676, 'premiere': 10677, 'convincing': 10678, 'agreeing': 10679, 'domingo': 10680, 'resurfaced': 10681, 'pornography': 10682, 'appropriately': 10683, 'menatep': 10684, 'overshot': 10685, 'pumped': 10686, 'hizbul': 10687, 'figueredo': 10688, 'sabotaged': 10689, 'presentation': 10690, 'softer': 10691, 'hockey': 10692, '1946': 10693, 'reestablished': 10694, 'shane': 10695, 'trafficked': 10696, 'fortune': 10697, 'ah': 10698, 'qingdao': 10699, 'kemal': 10700, 'cancellations': 10701, 'visible': 10702, 'fared': 10703, 'petroecuador': 10704, 'basin': 10705, 'institutional': 10706, 'pri': 10707, 'ailment': 10708, 'electing': 10709, 'conclusions': 10710, 'masterminds': 10711, 'lovato': 10712, 'citgo': 10713, '169': 10714, 'publishes': 10715, 'noriega': 10716, 'neutralized': 10717, 'joel': 10718, 'personalities': 10719, 'caches': 10720, 'ho': 10721, '80th': 10722, 'equitable': 10723, 'nights': 10724, 'majlis': 10725, 'petroleos': 10726, 'portfolio': 10727, 'impending': 10728, 'hugh': 10729, 'contributor': 10730, 'alleviate': 10731, 'peacocks': 10732, 'annoyed': 10733, 'claws': 10734, 'auditors': 10735, 'waterborne': 10736, 'sir': 10737, 'unreasonable': 10738, 'spearheaded': 10739, 'cats': 10740, 'asians': 10741, 'seasons': 10742, 'injure': 10743, 'moro': 10744, 'slumping': 10745, 'u2': 10746, 'kalam': 10747, 'clears': 10748, 'distinctive': 10749, 'carl': 10750, 'streak': 10751, 'gregory': 10752, 'psychiatric': 10753, 'zulia': 10754, 'warner': 10755, 'dominguez': 10756, 'raced': 10757, 'pretty': 10758, 'khel': 10759, 'airlifting': 10760, 'marco': 10761, 'tours': 10762, 'applicants': 10763, 'bruce': 10764, 'golding': 10765, 'nathan': 10766, 'justine': 10767, 'commuters': 10768, 'miner': 10769, 'wiretapping': 10770, 'wiretaps': 10771, 'das': 10772, 'gateway': 10773, 'peasants': 10774, 'roots': 10775, 'homosexuality': 10776, 'primerica': 10777, 'endowed': 10778, 'relied': 10779, 'secretive': 10780, 'opted': 10781, 'disguise': 10782, 'sum': 10783, 'statue': 10784, 'xdr': 10785, 'clarification': 10786, 'angrily': 10787, 'spinal': 10788, 'reimposed': 10789, 'tshabalala': 10790, 'vary': 10791, 'rhetoric': 10792, 'fiction': 10793, 'sway': 10794, 'beast': 10795, 'rajoelina': 10796, 'advocated': 10797, 'stretching': 10798, 'melted': 10799, 'subaru': 10800, 'demobilization': 10801, 'zahar': 10802, 'banker': 10803, 'gearing': 10804, 'sleeping': 10805, 'charsadda': 10806, 'kam': 10807, 'unexploded': 10808, 'painted': 10809, 'trace': 10810, 'edelman': 10811, 'ackerman': 10812, 'harvesting': 10813, 'manpower': 10814, 'purely': 10815, 'guiana': 10816, 'sugarcane': 10817, 'debated': 10818, '254': 10819, 'climbing': 10820, 'emotional': 10821, 'lobbyist': 10822, 'knowing': 10823, 'reprinting': 10824, 'depiction': 10825, 'reprinted': 10826, 'pashtuns': 10827, 'teammate': 10828, 'deplored': 10829, 'nguesso': 10830, 'galle': 10831, 'lankans': 10832, 'laughed': 10833, 'bannu': 10834, 'cursed': 10835, 'karroubi': 10836, 'recordings': 10837, 'ghulam': 10838, 'choking': 10839, 'steven': 10840, 'reelection': 10841, 'canisters': 10842, 'catching': 10843, 'gem': 10844, 'backyard': 10845, 'rand': 10846, '1815': 10847, 'colonized': 10848, '1951': 10849, 'subdued': 10850, 'johndroe': 10851, 'xi': 10852, 'battalion': 10853, 'tolerated': 10854, 'camped': 10855, 'choreographer': 10856, 'nobody': 10857, 'traced': 10858, 'degrading': 10859, 'slovak': 10860, 'pyramid': 10861, 'painting': 10862, 'farouk': 10863, 'weighing': 10864, 'hindus': 10865, 'birthplace': 10866, 'atrophy': 10867, 'capped': 10868, 'commended': 10869, 'zuloaga': 10870, 'peyton': 10871, 'onboard': 10872, 'equus': 10873, 'waterways': 10874, 'bare': 10875, 'minimal': 10876, 'agrarian': 10877, 'oxen': 10878, 'lamb': 10879, 'oh': 10880, 'enabling': 10881, 'thirst': 10882, 'certificate': 10883, 'softening': 10884, 'pretext': 10885, 'vandalism': 10886, 'dale': 10887, 'reassured': 10888, 'forbid': 10889, 'infecting': 10890, 'usaid': 10891, 'longwang': 10892, 'cartagena': 10893, 'paerson': 10894, 'differ': 10895, 'installment': 10896, 'sharma': 10897, 'escorting': 10898, 'reckless': 10899, 'sofia': 10900, 'albert': 10901, 'comprised': 10902, 'grammys': 10903, 'dignity': 10904, 'orderly': 10905, 'guitar': 10906, 'endeavour': 10907, 'salvadoran': 10908, 'intellectual': 10909, 'rebuilt': 10910, 'rafiq': 10911, 'exploitable': 10912, 'peg': 10913, '1957': 10914, 'wrath': 10915, 'hammered': 10916, 'micheletti': 10917, 'reclusive': 10918, 'earner': 10919, 'theo': 10920, 'bouyeri': 10921, 'normalization': 10922, 'sighted': 10923, 'medecins': 10924, 'bihar': 10925, 'derbez': 10926, 'eighteen': 10927, 'nyamwasa': 10928, 'benn': 10929, 'dock': 10930, 'scorpions': 10931, 'reconstruct': 10932, 'parks': 10933, 'planner': 10934, 'memoir': 10935, 'symbolic': 10936, '193': 10937, 'hispaniola': 10938, 'bore': 10939, 'summits': 10940, 'larry': 10941, 'dd': 10942, "dunkin'": 10943, 'palm': 10944, 'decreasing': 10945, '1936': 10946, 'franco': 10947, 'seventy': 10948, 'sixteen': 10949, 'corner': 10950, 'tzipi': 10951, 'gallon': 10952, 'animated': 10953, 'referee': 10954, 'singers': 10955, 'lips': 10956, 'sa': 10957, 'unwanted': 10958, 'expertise': 10959, 'jing': 10960, 'guatemalan': 10961, 'miroslav': 10962, 'nastase': 10963, 'pensioners': 10964, 'maneuver': 10965, 'bosch': 10966, 'boskovski': 10967, 'chee': 10968, 'hwa': 10969, 'islami': 10970, '218': 10971, 'conventions': 10972, 'ashkelon': 10973, 'beattie': 10974, 'nur': 10975, 'aegean': 10976, 'timberlake': 10977, 'ripping': 10978, 'township': 10979, 'hamadi': 10980, 'segments': 10981, 'principally': 10982, 'adjustment': 10983, 'alam': 10984, 'dhekelia': 10985, 'fold': 10986, 'fatigue': 10987, 'interrupting': 10988, 'pedophile': 10989, 'henri': 10990, 'arias': 10991, 'backdrop': 10992, 'concealed': 10993, 'typically': 10994, 'wrestler': 10995, 'purge': 10996, 'steroid': 10997, 'cauldron': 10998, 'simultaneously': 10999, 'pouring': 11000, 'chlorine': 11001, 'suitcase': 11002, 'allowance': 11003, 'sums': 11004, 'napolitano': 11005, 'flatly': 11006, 'kumar': 11007, 'rests': 11008, 'snowstorm': 11009, 'ndc': 11010, 'safeguards': 11011, 'quang': 11012, 'incitement': 11013, 'culminated': 11014, 'unabated': 11015, 'owe': 11016, 'policymakers': 11017, 'concerted': 11018, 'seminar': 11019, 'jamie': 11020, 'hudson': 11021, 'sen': 11022, 'spiral': 11023, 'recalling': 11024, 'explains': 11025, 'warmup': 11026, 'kicking': 11027, 'drums': 11028, "'re": 11029, 'decisive': 11030, 'vine': 11031, 'mood': 11032, 'mule': 11033, 'handgun': 11034, 'listeners': 11035, 'beck': 11036, 'ferrero': 11037, 'ortiz': 11038, 'kazemi': 11039, 'jaffer': 11040, 'laxman': 11041, 'wicket': 11042, 'defying': 11043, 'panicked': 11044, 'restated': 11045, 'awaited': 11046, 'mile': 11047, 'eprdf': 11048, 'fossil': 11049, 'fuji': 11050, 'daimlerchrysler': 11051, 'zebra': 11052, 'copei': 11053, 'couples': 11054, 'obvious': 11055, 'files': 11056, 'austin': 11057, 'stalin': 11058, 'stagnated': 11059, '122': 11060, 'niue': 11061, 'ant': 11062, 'honey': 11063, 'cream': 11064, 'dove': 11065, 'battlefield': 11066, 'aftershocks': 11067, 'bakool': 11068, 'sevastopol': 11069, 'nabi': 11070, 'fattal': 11071, 'ilham': 11072, 'armstrong': 11073, 'trick': 11074, 'nonproliferation': 11075, 'earning': 11076, 'tumors': 11077, 'horizons': 11078, 'sii': 11079, 'bonuses': 11080, 'stuck': 11081, 'answering': 11082, 'nerve': 11083, 'rent': 11084, 'sandstorm': 11085, 'frigate': 11086, 'models': 11087, 'pittsburgh': 11088, 'finalizing': 11089, 'jerzy': 11090, 'robredo': 11091, 'ayad': 11092, 'iraqiya': 11093, 'forty': 11094, 'claus': 11095, 'bonus': 11096, 'perisic': 11097, 'erratic': 11098, 'preceding': 11099, 'presides': 11100, 'secrecy': 11101, 'atoll': 11102, 'lash': 11103, 'intercept': 11104, 'technique': 11105, 'siti': 11106, 'supari': 11107, 'subways': 11108, 'funneling': 11109, 'stoning': 11110, 'devil': 11111, 'ugandans': 11112, 'capitalized': 11113, 'accuracy': 11114, 'inaccuracies': 11115, '117': 11116, 'deadlines': 11117, 'stabbed': 11118, 'din': 11119, 'charkhi': 11120, 'sketchy': 11121, 'ursula': 11122, 'prohibit': 11123, 'hospitality': 11124, 'fats': 11125, 'domino': 11126, 'verifying': 11127, 'pig': 11128, 'provocations': 11129, 'ensuing': 11130, '1920s': 11131, 'draped': 11132, 'disciplined': 11133, 'bajram': 11134, 'ceku': 11135, 'foster': 11136, 'janata': 11137, 'corridor': 11138, 'infants': 11139, 'backward': 11140, 'julio': 11141, 'designation': 11142, 'legalized': 11143, 'corporations': 11144, 'subjects': 11145, 'discusses': 11146, 'mortality': 11147, 'renowned': 11148, '139': 11149, 'arena': 11150, 'leaning': 11151, 'khaznawi': 11152, 'bearing': 11153, 'gusts': 11154, 'diyarbakir': 11155, 'perspective': 11156, 'macapagal': 11157, 'clot': 11158, 'suits': 11159, 'apparatus': 11160, 'tajik': 11161, 'underscore': 11162, 'ostrich': 11163, 'reprehensible': 11164, 'diwali': 11165, 'hun': 11166, 'discounts': 11167, 'informing': 11168, 'burundians': 11169, 'nagasaki': 11170, 'atom': 11171, 'consequence': 11172, 'tumor': 11173, 'delimit': 11174, 'kanu': 11175, 'disparities': 11176, 'su': 11177, 'deceased': 11178, 'kyodo': 11179, 'lena': 11180, 'groenefeld': 11181, 'clemency': 11182, 'kai': 11183, 'inundated': 11184, 'juventus': 11185, 'clunkers': 11186, 'suez': 11187, 'comprise': 11188, 'sham': 11189, 'jumping': 11190, 'pitcairn': 11191, 'akrotiri': 11192, 'embroiled': 11193, 'undertook': 11194, 'degradation': 11195, 'davenport': 11196, 'mutually': 11197, 'practical': 11198, 'bouterse': 11199, 'shilpa': 11200, 'kissed': 11201, 'cords': 11202, 'cord': 11203, 'worn': 11204, 'bayan': 11205, 'jabr': 11206, 'ariana': 11207, 'temples': 11208, 'collins': 11209, 'warhead': 11210, 'orissa': 11211, 'ningbo': 11212, 'racing': 11213, 'newer': 11214, 'pilgrimage': 11215, '1921': 11216, '107': 11217, 'sutherland': 11218, 'friedan': 11219, 'infect': 11220, 'cannon': 11221, 'abortions': 11222, 'engineered': 11223, 'destabilizing': 11224, 'dairy': 11225, 'ramush': 11226, 'careful': 11227, 'coastlines': 11228, 'exceeds': 11229, 'hoon': 11230, 'commentary': 11231, 'camel': 11232, 'misery': 11233, 'inaccessible': 11234, 'garrison': 11235, 'wet': 11236, 'ourselves': 11237, 'mutua': 11238, 'abdallah': 11239, 'outline': 11240, 'adkins': 11241, 'homecoming': 11242, 'bolstered': 11243, 'weigh': 11244, '1492': 11245, 'paths': 11246, 'choudhury': 11247, 'negatively': 11248, 'futures': 11249, 'solider': 11250, 'carlo': 11251, 'corporal': 11252, 'converging': 11253, 'giants': 11254, 'usher': 11255, 'associations': 11256, 'desai': 11257, 'wisdom': 11258, 'oo': 11259, 'bollea': 11260, 'zetas': 11261, 'lease': 11262, 'kizza': 11263, 'sadness': 11264, 'paisley': 11265, 'sinn': 11266, 'fein': 11267, 'recommending': 11268, 'publicized': 11269, 'mate': 11270, 'depictions': 11271, 'kulov': 11272, '1872': 11273, 'commercially': 11274, 'fearfully': 11275, 'hemp': 11276, 'foca': 11277, '1922': 11278, 'g7': 11279, 'medic': 11280, 'horror': 11281, 'ardzinba': 11282, 'khadjimba': 11283, 'pedestrians': 11284, 'toys': 11285, 'dallas': 11286, 'bahr': 11287, 'affection': 11288, 'injection': 11289, 'insistence': 11290, 'genetically': 11291, 'oumar': 11292, 'deterioration': 11293, 'nevzlin': 11294, 'val': 11295, 'swearing': 11296, 'parachinar': 11297, '203': 11298, 'sphere': 11299, 'trapping': 11300, 'duekoue': 11301, 'ramzan': 11302, 'videotapes': 11303, 'epsilon': 11304, 'doctrine': 11305, 'zaragoza': 11306, 'preserved': 11307, 'wheelchair': 11308, 'weaker': 11309, 'avert': 11310, 'sanchez': 11311, 'pa': 11312, 'crept': 11313, 'assumes': 11314, 'shoving': 11315, 'harbi': 11316, 'bed': 11317, 'ipad': 11318, 'eternal': 11319, 'yearly': 11320, 'seniors': 11321, 'maiduguri': 11322, 'lobby': 11323, '109': 11324, 'hogg': 11325, 'sudharmono': 11326, 'photographers': 11327, 'seselj': 11328, 'curve': 11329, '444': 11330, 'slaying': 11331, 'tomatoes': 11332, 'firearms': 11333, 'rivalry': 11334, 'trent': 11335, 'uhm': 11336, 'spacewalks': 11337, 'slots': 11338, 'betting': 11339, 'bluefin': 11340, 'measles': 11341, 'karbouli': 11342, 'drinks': 11343, 'malpractice': 11344, 'josef': 11345, 'overflowed': 11346, 'lebedev': 11347, 'repaired': 11348, 'defy': 11349, 'haul': 11350, 'creatures': 11351, 'playoffs': 11352, 'roofs': 11353, 'andijon': 11354, 'owl': 11355, 'scam': 11356, 'di': 11357, 'solitary': 11358, 'comeback': 11359, 'stein': 11360, 'foolish': 11361, 'thorn': 11362, 'reply': 11363, 'jeffrey': 11364, 'echoupal': 11365, 'stirring': 11366, 'dean': 11367, 'buckovski': 11368, 'clinical': 11369, 'bolt': 11370, 'shenzhou': 11371, 'worshipers': 11372, 'vajpayee': 11373, 'traveler': 11374, 'cobain': 11375, 'martyrdom': 11376, 'craybas': 11377, 'bengali': 11378, 'melt': 11379, 'pole': 11380, 'parma': 11381, 'anheuser': 11382, 'busch': 11383, 'caldera': 11384, 'qantas': 11385, 'chang': 11386, 'antibiotics': 11387, 'materazzi': 11388, 'newseum': 11389, 'benesova': 11390, 'bild': 11391, "l'aquila": 11392, 'acosta': 11393, 'banderas': 11394, 'viper': 11395, 'foreman': 11396, 'advani': 11397, 'karabilah': 11398, 'satterfield': 11399, 'sliding': 11400, 'sperling': 11401, 'lapdog': 11402, 'nist': 11403, 'adverse': 11404, 'terrain': 11405, 'ellison': 11406, 'groundhog': 11407, 'convergence': 11408, 'lithium': 11409, 'hondo': 11410, 'guernsey': 11411, 'fayaz': 11412, 'karpinski': 11413, 'opel': 11414, 'mattel': 11415, 'fleihan': 11416, 'aguilar': 11417, 'zinser': 11418, 'boudhiba': 11419, 'arauca': 11420, 'czechs': 11421, 'rabbis': 11422, 'beiring': 11423, 'mprp': 11424, 'echeverria': 11425, 'certificates': 11426, 'qanuni': 11427, 'shotgun': 11428, 'buechel': 11429, 'fouad': 11430, 'designers': 11431, 'spaceshipone': 11432, 'designer': 11433, 'richter': 11434, 'entourage': 11435, 'lounge': 11436, 'disclosure': 11437, 'vying': 11438, 'congresswoman': 11439, 'promoter': 11440, 'abd': 11441, 'oppression': 11442, '290': 11443, 'normandy': 11444, 'thani': 11445, 'khalifa': 11446, 'generates': 11447, 'extraordinarily': 11448, 'contrive': 11449, 'zurab': 11450, 'leisure': 11451, 'grandfather': 11452, 'sack': 11453, 'benon': 11454, 'satisfaction': 11455, 'lit': 11456, 'mock': 11457, 'poonch': 11458, 'kentucky': 11459, 'effigy': 11460, 'bahadur': 11461, 'deuba': 11462, 'hotbed': 11463, 'endorsements': 11464, 'leukemia': 11465, 'bone': 11466, 'himalayas': 11467, 'displacement': 11468, 'gholamreza': 11469, 'juvenile': 11470, 'eyewitnesses': 11471, 'zvornik': 11472, 'sistan': 11473, 'thunder': 11474, 'topping': 11475, 'ignacio': 11476, 'chela': 11477, 'serra': 11478, 'carlsen': 11479, 'tune': 11480, 'unborn': 11481, 'incompatible': 11482, '1559': 11483, 'mughal': 11484, 'doctorate': 11485, 'overtures': 11486, 'inseparable': 11487, 'collapses': 11488, 'snowstorms': 11489, 'statesman': 11490, 'lyudmila': 11491, 'connecting': 11492, 'skyrocketing': 11493, 'reliant': 11494, 'cosmonauts': 11495, 'demoted': 11496, 'simonyi': 11497, 'defiant': 11498, 'stuffed': 11499, 'toughen': 11500, 'curriculum': 11501, 'merit': 11502, 'downs': 11503, 'readying': 11504, 'manuscript': 11505, 'beethoven': 11506, 'sotheby': 11507, 'library': 11508, 'palmer': 11509, 'duet': 11510, '1920': 11511, 'reluctance': 11512, 'saeed': 11513, 'sanader': 11514, 'ltd': 11515, '212': 11516, 'pursues': 11517, 'wrangling': 11518, 'infested': 11519, 'natives': 11520, 'cooler': 11521, '1838': 11522, 'pete': 11523, 'devalued': 11524, 'carib': 11525, 'vigorously': 11526, 'performs': 11527, 'gallery': 11528, 'barrett': 11529, 'orphanage': 11530, 'endangering': 11531, 'hectare': 11532, 'rigid': 11533, '210': 11534, 'colliding': 11535, 'tying': 11536, '1933': 11537, 'scandinavian': 11538, 'sneak': 11539, 'streamlining': 11540, 'materialize': 11541, 'fleming': 11542, 'hardship': 11543, 'embedded': 11544, 'indira': 11545, 'arbiter': 11546, 'agitation': 11547, 'jamming': 11548, 'portable': 11549, 'supportive': 11550, 'completes': 11551, 'meaningful': 11552, 'schoolchildren': 11553, 'uncommon': 11554, 'consultant': 11555, 'superstar': 11556, 'recurring': 11557, 'mordechai': 11558, 'tolled': 11559, 'expressions': 11560, 'kalashnikov': 11561, 'hitch': 11562, 'paperwork': 11563, 'objectivity': 11564, 'troubling': 11565, 'millimeters': 11566, 'rife': 11567, 'precondition': 11568, 'editions': 11569, 'condolence': 11570, 'rations': 11571, 'vegetable': 11572, 'prizes': 11573, 'chemistry': 11574, 'alfred': 11575, 'banquet': 11576, 'literary': 11577, 'hypothetical': 11578, 'nears': 11579, 'restricts': 11580, 'yoh': 11581, 'materialized': 11582, 'legs': 11583, 'timex': 11584, 'cane': 11585, 'napoleonic': 11586, 'strategically': 11587, 'vi': 11588, 'centrally': 11589, 'fdi': 11590, 'thermal': 11591, 'micronesia': 11592, 'fsm': 11593, '2023': 11594, 'payouts': 11595, 'tears': 11596, 'attractions': 11597, 'forgive': 11598, 'reservists': 11599, 'imaginary': 11600, 'motorbike': 11601, 'contention': 11602, 'dawa': 11603, 'enforced': 11604, 'tramway': 11605, 'feat': 11606, 'monastery': 11607, 'attraction': 11608, 'slaughtering': 11609, 'qasab': 11610, 'gripping': 11611, 'koirala': 11612, 'curtailing': 11613, '143': 11614, 'throats': 11615, 'diminishing': 11616, 'nighttime': 11617, 'karshi': 11618, 'khanabad': 11619, 'issa': 11620, 'taste': 11621, 'calorie': 11622, 'inmate': 11623, 'lance': 11624, 'caraballo': 11625, 'topic': 11626, 'waterfowl': 11627, 'marat': 11628, 'normalizing': 11629, 'uda': 11630, 'authentic': 11631, 'azhari': 11632, 'kapisa': 11633, 'headscarves': 11634, 'secretaries': 11635, 'senses': 11636, 'headaches': 11637, 'reflecting': 11638, 'tangible': 11639, 'speculators': 11640, 'pointing': 11641, 'disagrees': 11642, 'perceptions': 11643, 'ceiling': 11644, 'meddling': 11645, 'stricter': 11646, 'indecent': 11647, 'supervise': 11648, 'snyder': 11649, 'honiara': 11650, 'asserts': 11651, 'shirin': 11652, 'instantly': 11653, 'unlicensed': 11654, 'pisanu': 11655, 'submission': 11656, 'presently': 11657, 'anraat': 11658, 'coinciding': 11659, 'naturally': 11660, 'retroactive': 11661, 'rationing': 11662, 'mls': 11663, 'ac': 11664, '76th': 11665, 'incompetence': 11666, 'taxpayer': 11667, 'kwasniewski': 11668, 'valdas': 11669, 'alphabet': 11670, 'proponents': 11671, '625': 11672, 'manfred': 11673, 'colonization': 11674, 'slovene': 11675, 'acceded': 11676, 'severing': 11677, 'quarrel': 11678, 'traveller': 11679, 'splendid': 11680, 'ellsworth': 11681, 'mwenda': 11682, 'broadly': 11683, 'sterilization': 11684, 'centimeter': 11685, 'overseen': 11686, 'promotes': 11687, 'intermittent': 11688, 'sixto': 11689, 'ninh': 11690, 'zhaoxing': 11691, 'chengdu': 11692, 'clashing': 11693, 'lobbing': 11694, 'shantytown': 11695, 'blackouts': 11696, 'generators': 11697, 'stray': 11698, 'shihab': 11699, 'kilinochchi': 11700, 'freighter': 11701, 'chitral': 11702, 'ahbash': 11703, 'sponsoring': 11704, 'clarify': 11705, 'arbil': 11706, 'asthma': 11707, 'lisbon': 11708, 'elevated': 11709, 'beni': 11710, 'cordon': 11711, 'gravely': 11712, 'claude': 11713, 'staunch': 11714, 'resurgence': 11715, 'militarized': 11716, 'directions': 11717, 'orlandez': 11718, 'gamboa': 11719, 'lords': 11720, 'distorting': 11721, 'harms': 11722, 'dudley': 11723, 'monterrey': 11724, 'lodged': 11725, 'rebates': 11726, 'yaalon': 11727, 'farce': 11728, 'outlet': 11729, 'retirees': 11730, 'slap': 11731, 'forcefully': 11732, 'almallah': 11733, 'dabas': 11734, 'magistrate': 11735, 'montedison': 11736, 'pursuant': 11737, 'density': 11738, 'crab': 11739, 'mustard': 11740, 'chidambaram': 11741, 'colonists': 11742, 'chiweshe': 11743, 'inclusive': 11744, 'consultation': 11745, 'pharaohs': 11746, 'maazou': 11747, 'defender': 11748, 'abel': 11749, 'pushes': 11750, 'hormone': 11751, 'yadav': 11752, 'transmissions': 11753, 'zacarias': 11754, 'grapes': 11755, 'manganese': 11756, 'beverages': 11757, 'disgruntled': 11758, 'welcoming': 11759, 'component': 11760, 'interruptions': 11761, 'ceyhan': 11762, 'deeds': 11763, 'regulation': 11764, 'fitness': 11765, 'infiltrating': 11766, 'ming': 11767, 'toe': 11768, 'rebounds': 11769, 'abhisit': 11770, 'dispersing': 11771, 'deforestation': 11772, 'infidels': 11773, 'endorses': 11774, 'portland': 11775, 'sambadrome': 11776, 'squeeze': 11777, 'collaborating': 11778, 'owen': 11779, 'commits': 11780, 'hashimi': 11781, 'vengeance': 11782, 'bikindi': 11783, 'composed': 11784, 'lyrics': 11785, 'baathist': 11786, 'soured': 11787, 'defusing': 11788, 'hence': 11789, 'averted': 11790, 'anticipates': 11791, 'marti': 11792, 'offend': 11793, 'konan': 11794, 'mian': 11795, 'tactical': 11796, 'tegucigalpa': 11797, 'markey': 11798, 'accessories': 11799, 'cosmetic': 11800, 'technologically': 11801, 'consumed': 11802, 'antonella': 11803, 'liberalizing': 11804, 'reared': 11805, 'unwilling': 11806, 'federally': 11807, 'pashtun': 11808, 'disregard': 11809, '124': 11810, 'sourav': 11811, 'surpassing': 11812, 'countrymen': 11813, 'juma': 11814, 'segment': 11815, 'discomfort': 11816, 'inspiring': 11817, 'emirate': 11818, 'defenders': 11819, 'volunteered': 11820, 'skip': 11821, 'exploited': 11822, 'canaria': 11823, 'moderated': 11824, 'arsonists': 11825, 'vaclav': 11826, 'authors': 11827, 'soros': 11828, 'overlooking': 11829, 'cheers': 11830, 'infiltrate': 11831, 'gerry': 11832, 'absurd': 11833, 'bench': 11834, 'javad': 11835, 'haqqani': 11836, 'honorary': 11837, 'positively': 11838, 'novosti': 11839, 'condom': 11840, 'salazar': 11841, 'janica': 11842, 'spilling': 11843, 'sanctuaries': 11844, 'absentee': 11845, 'gubernatorial': 11846, 'christine': 11847, 'ruin': 11848, 'stretches': 11849, 'explicitly': 11850, 'khurmatu': 11851, 'bastion': 11852, 'arteries': 11853, 'tripled': 11854, 'firefights': 11855, 'announcements': 11856, 'shalit': 11857, 'marinin': 11858, 'totmianina': 11859, 'poiree': 11860, 'miracle': 11861, 'rented': 11862, "l'equipe": 11863, 'erected': 11864, '1500': 11865, 'sheltered': 11866, 'rangin': 11867, 'komuri': 11868, 'tsvangirai': 11869, 'mehmood': 11870, 'integra': 11871, 'exercised': 11872, 'hammer': 11873, 'advantages': 11874, 'lagged': 11875, 'leste': 11876, 'markedly': 11877, 'duchy': 11878, 'fluctuations': 11879, 'raven': 11880, 'desired': 11881, 'supposing': 11882, 'washing': 11883, 'silk': 11884, 'financier': 11885, 'bible': 11886, 'stationary': 11887, 'trailing': 11888, 'norad': 11889, 'peterson': 11890, 'preston': 11891, 'niccum': 11892, 'samantha': 11893, 'lantos': 11894, 'barham': 11895, 'sirens': 11896, 'trek': 11897, 'assassin': 11898, 'mates': 11899, 'mislead': 11900, 'listing': 11901, 'lefevre': 11902, 'staffer': 11903, 'teens': 11904, 'kojo': 11905, 'upbeat': 11906, 'centanni': 11907, 'olaf': 11908, 'wiig': 11909, 'caption': 11910, 'instigate': 11911, 'delray': 11912, 'xavier': 11913, 'humanely': 11914, 'jemua': 11915, 'soy': 11916, 'behead': 11917, 'immune': 11918, 'glaxosmithkline': 11919, 'atmar': 11920, 'separatism': 11921, 'hygienic': 11922, 'mughani': 11923, 'lansana': 11924, 'plenty': 11925, 'ancestry': 11926, 'sculptures': 11927, 'andresen': 11928, 'muzadi': 11929, 'bhumibol': 11930, 'adulyadej': 11931, 'spiegel': 11932, 'adre': 11933, 'sediment': 11934, 'tallest': 11935, 'timed': 11936, 'bisengimina': 11937, 'confess': 11938, 'ensuring': 11939, 'contempt': 11940, 'anyway': 11941, 'suing': 11942, '175': 11943, 'placement': 11944, 'franc': 11945, '2000s': 11946, 'considerably': 11947, 'tan': 11948, 'accustomed': 11949, 'blackboard': 11950, 'chalk': 11951, 'northward': 11952, 'khushab': 11953, 'cautiously': 11954, 'warri': 11955, 'ijaw': 11956, 'screenings': 11957, 'joyce': 11958, 'mujuru': 11959, 'vacancy': 11960, 'ariane': 11961, 'adrian': 11962, 'yanjin': 11963, 'echoing': 11964, 'valerie': 11965, 'betty': 11966, 'knowingly': 11967, 'marlon': 11968, 'shelves': 11969, 'tightening': 11970, 'soe': 11971, 'wanting': 11972, 'chechens': 11973, 'chulanont': 11974, 'abstaining': 11975, 'sunset': 11976, 'scorching': 11977, 'ineffectiveness': 11978, 'ages': 11979, 'bilfinger': 11980, 'flushed': 11981, 'duchess': 11982, 'cornwall': 11983, 'tasnim': 11984, 'amarah': 11985, 'relocated': 11986, 'lynn': 11987, 'ihab': 11988, 'krishna': 11989, 'yelled': 11990, 'ritchie': 11991, 'presumptive': 11992, 'hafs': 11993, 'babar': 11994, 'combatant': 11995, 'biologist': 11996, 'reformists': 11997, 'accuser': 11998, 'concession': 11999, 'pigeon': 12000, 'jeweler': 12001, 'tareq': 12002, 'plentiful': 12003, 'reinsurance': 12004, 'amphibious': 12005, 'capitalist': 12006, 'domain': 12007, 'madagonia': 12008, 'ensued': 12009, 'garnered': 12010, 'malicious': 12011, 'overpowered': 12012, 'mahabad': 12013, 'petra': 12014, 'arch': 12015, 'litani': 12016, 'prevail': 12017, 'izzadeen': 12018, 'disruptive': 12019, 'checking': 12020, 'layers': 12021, '90th': 12022, 'leveled': 12023, 'fagih': 12024, 'prop': 12025, 'deterrent': 12026, 'stifle': 12027, 'walker': 12028, 'moody': 12029, 'defaults': 12030, 'sensitivities': 12031, 'mai': 12032, 'terminals': 12033, 'lifts': 12034, 'tract': 12035, 'serviceman': 12036, 'macy': 12037, 'characters': 12038, 'endorsing': 12039, 'putumayo': 12040, 'extortion': 12041, '184': 12042, 'modifications': 12043, 'ahronot': 12044, 'dismay': 12045, 'ambushing': 12046, 'blantyre': 12047, 'translated': 12048, 'lilongwe': 12049, 'lenient': 12050, 'parish': 12051, 'moderates': 12052, '65th': 12053, 'annexation': 12054, 'kfar': 12055, 'sailor': 12056, 'villaraigosa': 12057, 'figaro': 12058, 'unsustainable': 12059, 'qiyue': 12060, 'kaka': 12061, 'jung': 12062, "'ve": 12063, 'ntawukulilyayo': 12064, 'bernardo': 12065, '1st': 12066, 'citadel': 12067, 'sept': 12068, 'hobbled': 12069, 'overdependence': 12070, 'impediment': 12071, 'blueprint': 12072, 'sponsorship': 12073, 'subsidy': 12074, 'necessities': 12075, 'mined': 12076, 'casamance': 12077, 'undeclared': 12078, 'pretense': 12079, 'sorely': 12080, 'mathematician': 12081, 'relevant': 12082, 'ashcroft': 12083, 'restarting': 12084, 'tossed': 12085, 'helm': 12086, 'ceo': 12087, 'genuine': 12088, 'planche': 12089, 'wana': 12090, 'embarrassment': 12091, 'admiration': 12092, 'coping': 12093, 'feasible': 12094, 'inflammatory': 12095, 'sulaymaniya': 12096, 'hampshire': 12097, 'regrettable': 12098, 'toxicology': 12099, 'sealing': 12100, 'bounced': 12101, 'catalonia': 12102, 'classical': 12103, 'eighty': 12104, 'languages': 12105, 'tabloid': 12106, 'icc': 12107, 'derived': 12108, 'ineffective': 12109, 'grabbed': 12110, 'elevator': 12111, 'eckhard': 12112, 'stocking': 12113, 'timeline': 12114, 'badakhshan': 12115, 'exploring': 12116, 'ion': 12117, 'eduard': 12118, 'atrocity': 12119, 'tapped': 12120, '257': 12121, 'mammoth': 12122, 'verdicts': 12123, 'instigating': 12124, 'schelling': 12125, 'aumann': 12126, 'sumaidaie': 12127, 'sabbath': 12128, 'noble': 12129, 'kyaw': 12130, 'varies': 12131, 'muhammed': 12132, 'sunrise': 12133, 'everyday': 12134, 'campus': 12135, 'plc': 12136, '149': 12137, 'demographic': 12138, 'attributable': 12139, 'likewise': 12140, '2016': 12141, 'viking': 12142, 'norwegians': 12143, 'nationalism': 12144, 'consist': 12145, 'monkeys': 12146, 'danced': 12147, 'spectacle': 12148, 'nuts': 12149, 'laughter': 12150, 'unleashed': 12151, 'taji': 12152, 'douchevina': 12153, 'mauresmo': 12154, 'flamingo': 12155, 'milder': 12156, 'virulent': 12157, 'strengthens': 12158, 'ambition': 12159, 'pipes': 12160, 'sur': 12161, 'erupt': 12162, 'opposite': 12163, 'hauling': 12164, 'mac': 12165, 'drama': 12166, 'leash': 12167, 'karrada': 12168, 'greed': 12169, 'hazem': 12170, 'shaalan': 12171, 'colombians': 12172, 'dinosaurs': 12173, 'bones': 12174, 'tails': 12175, 'fielding': 12176, 'rajasthan': 12177, 'guerrero': 12178, 'amritsar': 12179, 'problematic': 12180, 'ploy': 12181, 'desperately': 12182, 'podium': 12183, 'ferrer': 12184, 'atp': 12185, 'pale': 12186, '195': 12187, 'vows': 12188, 'postwar': 12189, 'adversely': 12190, 'kadhimiya': 12191, 'aquifer': 12192, 'seismic': 12193, 'coretta': 12194, 'ovarian': 12195, 'bunkers': 12196, 'jessen': 12197, 'kostunica': 12198, 'hamma': 12199, 'dsm': 12200, 'barthelemy': 12201, 'attracts': 12202, 'abundant': 12203, 'prix': 12204, 'frelimo': 12205, 'delicate': 12206, 'armando': 12207, 'questionable': 12208, 'remittance': 12209, 'domenech': 12210, 'respecting': 12211, 'prevalent': 12212, 'manifesto': 12213, 'desperation': 12214, 'flexible': 12215, 'reprisals': 12216, 'dc': 12217, 'cavaco': 12218, 'cap': 12219, 'protectionism': 12220, 'muhajer': 12221, 'landmarks': 12222, 'socialists': 12223, 'hygiene': 12224, 'premature': 12225, 'chennai': 12226, 'rallying': 12227, 'collapsing': 12228, 'drainage': 12229, 'kenteris': 12230, 'thanou': 12231, 'systemic': 12232, 'arap': 12233, 'gomez': 12234, 'gallbladder': 12235, 'shinui': 12236, 'unimaginable': 12237, 'errant': 12238, 'busan': 12239, 'sandy': 12240, 'swimming': 12241, 'motorist': 12242, 'greenback': 12243, 'qawasmi': 12244, 'lure': 12245, 'infiltrations': 12246, 'incidence': 12247, 'liable': 12248, 'tentatively': 12249, 'explorer': 12250, 'levey': 12251, 'cornerstone': 12252, 'fallout': 12253, 'umm': 12254, 'kellenberger': 12255, 'throwers': 12256, 'perjury': 12257, 'contradictory': 12258, 'denominated': 12259, 'onset': 12260, 'dried': 12261, 'ec': 12262, 'default': 12263, 'emu': 12264, '1885': 12265, 'bloated': 12266, 'mongolian': 12267, 'dug': 12268, 'sown': 12269, 'barber': 12270, 'simeus': 12271, 'harmless': 12272, 'peugeot': 12273, 'adjourn': 12274, 'anhui': 12275, 'cowardly': 12276, 'curling': 12277, 'arturo': 12278, 'setbacks': 12279, 'grids': 12280, 'holbrooke': 12281, 'relocation': 12282, 'quebec': 12283, 'dominion': 12284, 'referendums': 12285, 'wrap': 12286, 'liaison': 12287, 'tragic': 12288, 'parcel': 12289, 'stranding': 12290, 'tarongoy': 12291, 'jeff': 12292, 'gdansk': 12293, 'mansour': 12294, 'destabilized': 12295, 'adventure': 12296, 'hilltop': 12297, 'expecting': 12298, 'stagnant': 12299, 'kenichiro': 12300, 'sasae': 12301, '80s': 12302, 'sacrificed': 12303, 'detecting': 12304, '248': 12305, 'incurred': 12306, 'plummeted': 12307, 'kajaki': 12308, 'harvests': 12309, 'correspondents': 12310, '228': 12311, 'fist': 12312, 'ahlu': 12313, 'tribesman': 12314, 'oshkosh': 12315, '352': 12316, 'chassis': 12317, 'hizballah': 12318, 'remarkably': 12319, 'awakened': 12320, 'bite': 12321, 'ross': 12322, 'sirte': 12323, 'cologne': 12324, 'mausoleum': 12325, 'ghorbanpour': 12326, 'masood': 12327, 'reinforce': 12328, 'sucumbios': 12329, 'orinoco': 12330, 'goodluck': 12331, 'notify': 12332, 'notification': 12333, 'merida': 12334, '780': 12335, 'unpunished': 12336, 'precursor': 12337, 'hafun': 12338, 'sand': 12339, 'granada': 12340, 'rider': 12341, 'danube': 12342, 'tenth': 12343, 'populist': 12344, 'roar': 12345, 'evaluating': 12346, 'boyfriend': 12347, '1795': 12348, 'partitioned': 12349, 'unlawfully': 12350, 'tolerant': 12351, 'siddiqui': 12352, 'ubaydi': 12353, 'culminate': 12354, 'emptied': 12355, 'chaman': 12356, 'extort': 12357, 'daschle': 12358, 'amal': 12359, 'npt': 12360, 'swung': 12361, 'insurer': 12362, 'bhutanese': 12363, 'indo': 12364, 'defined': 12365, 'inefficiencies': 12366, '1902': 12367, 'administer': 12368, 'bolted': 12369, 'lisa': 12370, 'examples': 12371, 'inland': 12372, 'juveniles': 12373, 'headscarf': 12374, 'nitrogen': 12375, 'ridiculed': 12376, 'fig': 12377, 'yasuo': 12378, 'radicalism': 12379, 'milf': 12380, 'pure': 12381, 'airs': 12382, 'spaceship': 12383, 'geldof': 12384, 'grim': 12385, 'refers': 12386, 'tammy': 12387, 'wizard': 12388, 'restructured': 12389, 'rosales': 12390, 'pennetta': 12391, 'solis': 12392, 'compassionate': 12393, 'floral': 12394, 'vigilance': 12395, 'ruptured': 12396, 'repayment': 12397, 'astana': 12398, 'serhiy': 12399, 'holovaty': 12400, 'suitable': 12401, 'datta': 12402, 'bertie': 12403, 'ahern': 12404, 'belfast': 12405, 'overturning': 12406, 'formality': 12407, 'solemn': 12408, 'yasir': 12409, 'adil': 12410, 'metropolitan': 12411, 'morgenthau': 12412, 'glorifies': 12413, 'mishandling': 12414, 'shenzhen': 12415, 'textbooks': 12416, 'uruguayan': 12417, 'acceptance': 12418, 'kashmiris': 12419, 'guise': 12420, 'griffin': 12421, 'netted': 12422, 'paracha': 12423, 'slip': 12424, 'plotted': 12425, 'mashhadani': 12426, 'irbil': 12427, 'disagreed': 12428, 'aspects': 12429, 'dublin': 12430, 'flushing': 12431, 'ernest': 12432, 'serena': 12433, 'combine': 12434, 'yachts': 12435, 'crj': 12436, 'backpack': 12437, 'imprisoning': 12438, 'aquatic': 12439, 'description': 12440, 'marketed': 12441, 'dipped': 12442, 'benefiting': 12443, 'civic': 12444, 'sculptor': 12445, 'juno': 12446, 'certainly': 12447, 'hollow': 12448, 'ai': 12449, 'screened': 12450, 'projectiles': 12451, 'alzheimer': 12452, 'sellers': 12453, 'skidded': 12454, 'attitude': 12455, 'telegram': 12456, 'veal': 12457, 'uncensored': 12458, 'admitting': 12459, 'collaborated': 12460, 'mouthpiece': 12461, 'vinci': 12462, 'disclaimer': 12463, 'maier': 12464, 'fritz': 12465, 'forensics': 12466, 'harmonious': 12467, 'forbidding': 12468, 'overran': 12469, 'filmyard': 12470, 'deposit': 12471, 'pulp': 12472, 'mombasa': 12473, 'pills': 12474, 'petter': 12475, 'solberg': 12476, 'auc': 12477, 'plagues': 12478, 'lahiya': 12479, 'gurgenidze': 12480, 'barricade': 12481, 'jabaliya': 12482, 'veneman': 12483, 'cimoszewicz': 12484, 'trucking': 12485, 'littered': 12486, 'vigil': 12487, 'ruiz': 12488, 'semitic': 12489, 'premeditated': 12490, 'nyan': 12491, 'marty': 12492, 'krill': 12493, 'administrations': 12494, 'guadeloupe': 12495, 'reunion': 12496, 'fourths': 12497, 'beware': 12498, 'mossad': 12499, 'tamara': 12500, 'gansu': 12501, 'quami': 12502, 'insults': 12503, 'palma': 12504, 'vines': 12505, 'airmen': 12506, 'emergencies': 12507, 'rotate': 12508, 'kaibyshev': 12509, 'lauderdale': 12510, 'reservations': 12511, 'resembles': 12512, 'farsi': 12513, 'avenue': 12514, 'mujahedeen': 12515, 'underneath': 12516, 'neumann': 12517, 'phoned': 12518, 'portrays': 12519, 'showcasing': 12520, 'showcase': 12521, 'mort': 12522, 'earliest': 12523, 'crypt': 12524, 'harshly': 12525, 'conspirators': 12526, 'pulwama': 12527, 'cereal': 12528, 'shortcomings': 12529, 'sar': 12530, 'phenomenon': 12531, 'dynastic': 12532, 'cede': 12533, 'orientation': 12534, 'kooyong': 12535, 'tentative': 12536, 'izvestia': 12537, 'prolong': 12538, 'ninevah': 12539, 'wipe': 12540, 'mutation': 12541, 'interceptors': 12542, 'kidd': 12543, 'vientiane': 12544, 'ablaze': 12545, 'complicating': 12546, '720': 12547, 'elimination': 12548, 'adb': 12549, 'tanzanian': 12550, 'dheere': 12551, 'poisons': 12552, 'diluted': 12553, 'underscored': 12554, 'resolutely': 12555, 'exploratory': 12556, 'dreamliner': 12557, 'nippon': 12558, 'hamdania': 12559, 'orbiter': 12560, 'harassing': 12561, 'petro': 12562, 'succumbed': 12563, 'abundance': 12564, 'oakland': 12565, 'preference': 12566, 'boers': 12567, 'locomotives': 12568, 'constitutionally': 12569, 'intensively': 12570, 'standstill': 12571, 'selfish': 12572, 'reign': 12573, 'swooped': 12574, 'deserves': 12575, 'afflicted': 12576, 'samuels': 12577, 'crease': 12578, 'steyn': 12579, 'towed': 12580, 'crewmembers': 12581, 'unconditionally': 12582, 'retake': 12583, 'experiences': 12584, 'interrupt': 12585, 'jailing': 12586, 'franks': 12587, 'tireless': 12588, 'adolf': 12589, 'valenzuela': 12590, 'franz': 12591, 'dorfmeister': 12592, 'versions': 12593, 'obeidi': 12594, 'bribe': 12595, 'downplaying': 12596, 'tornado': 12597, 'haley': 12598, 'barbour': 12599, 'counties': 12600, 'malindi': 12601, 'vermont': 12602, 'kuo': 12603, 'bergersen': 12604, 'dongzhou': 12605, 'savannah': 12606, 'georgetown': 12607, 'churns': 12608, 'vegas': 12609, 'duff': 12610, 'realizes': 12611, 'mindful': 12612, 'sounds': 12613, 'anarchy': 12614, 'tasks': 12615, 'dextre': 12616, 'closes': 12617, 'havoc': 12618, 'reining': 12619, 'manigat': 12620, 'savimbi': 12621, 'possesses': 12622, 'revival': 12623, '1942': 12624, 'razak': 12625, 'bamako': 12626, 'jem': 12627, 'khalil': 12628, 'notices': 12629, 'warren': 12630, 'buffett': 12631, 'hsieh': 12632, 'andras': 12633, 'batiz': 12634, 'rattled': 12635, 'bingol': 12636, 'eagles': 12637, 'nadia': 12638, 'venues': 12639, 'kokang': 12640, 'levin': 12641, 'sans': 12642, 'frontieres': 12643, 'labado': 12644, 'influx': 12645, 'insulza': 12646, 'recessed': 12647, 'ngabo': 12648, 'hasina': 12649, 'inhuman': 12650, 'professors': 12651, 'octopus': 12652, 'creature': 12653, 'counternarcotics': 12654, 'expeditions': 12655, 'toppling': 12656, 'firecrackers': 12657, 'jovic': 12658, 'vieira': 12659, 'pistols': 12660, 'luggage': 12661, 'trafficker': 12662, 'relax': 12663, 'fiber': 12664, 'patagonian': 12665, 'toothfish': 12666, '213': 12667, 'overflights': 12668, 'kites': 12669, 'vanquished': 12670, 'cock': 12671, '138': 12672, 'telecom': 12673, 'kewell': 12674, 'aussies': 12675, 'inconsistent': 12676, 'merk': 12677, 'stuff': 12678, 'zedong': 12679, 'empress': 12680, 'plowed': 12681, 'prostate': 12682, 'bright': 12683, 'dai': 12684, 'chopan': 12685, 'exaggerated': 12686, 'depicts': 12687, 'jesse': 12688, 'racist': 12689, 'ruth': 12690, 'scooter': 12691, 'poaching': 12692, 'bunia': 12693, 'bralo': 12694, 'najim': 12695, 'mironov': 12696, 'cppcc': 12697, 'useful': 12698, 'retaliatory': 12699, 'categorically': 12700, 'gulbuddin': 12701, 'commandeered': 12702, '131': 12703, 'schultz': 12704, 'demonstrates': 12705, 'endangers': 12706, 'arish': 12707, 'pavarotti': 12708, 'upstream': 12709, 'adaado': 12710, 'jiangxi': 12711, 'costume': 12712, 'convene': 12713, 'duarte': 12714, '223': 12715, 'newmont': 12716, 'focal': 12717, 'candy': 12718, 'savin': 12719, 'cent': 12720, 'surpluses': 12721, 'pol': 12722, 'amounted': 12723, 'falklands': 12724, 'wayside': 12725, 'shame': 12726, 'foxes': 12727, 'spider': 12728, 'tallies': 12729, 'entries': 12730, 'greg': 12731, 'schulte': 12732, 'pinault': 12733, 'ugly': 12734, 'dismissing': 12735, 'statute': 12736, 'breeding': 12737, 'yousef': 12738, 'phrase': 12739, 'luo': 12740, 'pitch': 12741, 'mutates': 12742, 'extravagant': 12743, 'civilization': 12744, 'mok': 12745, 'lipsky': 12746, 'pumps': 12747, 'voluntary': 12748, 'sinking': 12749, 'undertaken': 12750, 'acquisitions': 12751, 'dharmeratnam': 12752, 'tamilnet': 12753, 'columnist': 12754, 'staying': 12755, 'disbursing': 12756, 'qin': 12757, 'detection': 12758, 'nonprofit': 12759, 'speculated': 12760, 'fights': 12761, 'hooliganism': 12762, 'damn': 12763, 'robbers': 12764, 'gagged': 12765, 'classroom': 12766, '126': 12767, 'villager': 12768, "b'tselem": 12769, 'rabat': 12770, '375': 12771, 'connie': 12772, 'eradicated': 12773, 'breakdown': 12774, 'bab': 12775, 'foxx': 12776, 'unpredictable': 12777, 'ashdown': 12778, 'scaling': 12779, 'cropper': 12780, 'automobiles': 12781, 'homage': 12782, 'evaluation': 12783, 'edict': 12784, 'recipient': 12785, 'baton': 12786, 'thad': 12787, 'inducted': 12788, 'inductees': 12789, 'competent': 12790, 'mitrovica': 12791, 'seceded': 12792, 'disadvantaged': 12793, 'celso': 12794, 'manas': 12795, 'enactment': 12796, 'bunch': 12797, 'muentefering': 12798, 'smiled': 12799, 'presley': 12800, 'memphis': 12801, 'graceland': 12802, 'shariat': 12803, 'hymns': 12804, 'colombant': 12805, 'bowled': 12806, 'wong': 12807, 'reforming': 12808, 'qomi': 12809, 'naji': 12810, 'eden': 12811, 'kutesa': 12812, 'costing': 12813, 'golovin': 12814, 'marta': 12815, 'marion': 12816, 'brink': 12817, 'delegate': 12818, 'gilles': 12819, 'srichaphan': 12820, '206': 12821, 'extinction': 12822, 'rituals': 12823, 'biblical': 12824, 'crucifixion': 12825, 'liberians': 12826, 'unchallenged': 12827, 'bolstering': 12828, 'enlisted': 12829, 'fabricated': 12830, 'fictional': 12831, 'clearance': 12832, 'rms': 12833, 'mongol': 12834, 'russo': 12835, '1904': 12836, 'shifted': 12837, 'legitimacy': 12838, 'uninhabitable': 12839, 'topped': 12840, 'passion': 12841, 'lime': 12842, 'cottage': 12843, 'durbin': 12844, 'machar': 12845, 'rezaei': 12846, 'mostafa': 12847, 'stun': 12848, 'constantinople': 12849, 'daunting': 12850, 'clause': 12851, 'velasco': 12852, 'bot': 12853, 'zahi': 12854, 'saqqara': 12855, 'marriott': 12856, 'milk': 12857, 'russert': 12858, 'undefeated': 12859, 'elmar': 12860, 'oppressive': 12861, 'aliyev': 12862, 'inherit': 12863, 'relaying': 12864, 'cassini': 12865, 'rooftops': 12866, 'feasibility': 12867, 'engagements': 12868, 'overpass': 12869, 'hostess': 12870, 'woods': 12871, 'longevity': 12872, 'breakthroughs': 12873, 'ghor': 12874, 'madrassa': 12875, 'foxy': 12876, 'rapper': 12877, 'schoolgirl': 12878, 'indianapolis': 12879, 'writes': 12880, 'upside': 12881, 'chiyangwa': 12882, 'kenny': 12883, 'disapproval': 12884, 'judged': 12885, 'principe': 12886, 'incredible': 12887, 'manipulated': 12888, 'comedian': 12889, 'pryor': 12890, 'florence': 12891, 'conducts': 12892, 'extraditing': 12893, 'tolo': 12894, 'shaer': 12895, '360': 12896, 'replenish': 12897, 'ferencevych': 12898, 'definitive': 12899, 'imran': 12900, 'kyiv': 12901, 'phyu': 12902, 'szmajdzinski': 12903, 'zionism': 12904, 'ruhollah': 12905, 'khomeini': 12906, 'stanisic': 12907, 'outs': 12908, 'crafting': 12909, 'blackmail': 12910, 'cables': 12911, 'arid': 12912, 'workshop': 12913, 'blasphemy': 12914, 'defaming': 12915, 'punishable': 12916, 'saarc': 12917, '142': 12918, 'confusion': 12919, 'simkins': 12920, 'barretto': 12921, 'rhythm': 12922, 'incomprehensible': 12923, 'spit': 12924, 'anticipate': 12925, 'ahtisaari': 12926, 'ging': 12927, 'cultivated': 12928, 'rivalries': 12929, 'du': 12930, 'lambert': 12931, 'leonel': 12932, 'helen': 12933, 'weisfield': 12934, 'qalqilya': 12935, 'gerald': 12936, 'fluctuates': 12937, 'payroll': 12938, 'mixture': 12939, 'vendors': 12940, 'interrogating': 12941, 'lana': 12942, 'aces': 12943, 'preside': 12944, 'perkins': 12945, 'hajj': 12946, 'milton': 12947, 'obote': 12948, 'murat': 12949, 'descended': 12950, 'reunify': 12951, 'schumer': 12952, 'jilin': 12953, 'compiled': 12954, '192': 12955, 'rahul': 12956, 'anchored': 12957, '136': 12958, 'hans': 12959, 'cermak': 12960, 'mladen': 12961, 'markac': 12962, 'floated': 12963, 'ericsson': 12964, 'leaflets': 12965, 'adversaries': 12966, 'blades': 12967, 'starring': 12968, 'mutating': 12969, 'nad': 12970, 'sadio': 12971, 'grappling': 12972, 'equity': 12973, 'defamation': 12974, 'clouds': 12975, 'nickname': 12976, 'hits': 12977, 'uncover': 12978, 'portrait': 12979, 'handsome': 12980, 'apprehended': 12981, 'kujundzic': 12982, 'ahram': 12983, 'kazimierz': 12984, 'marcinkiewicz': 12985, '361': 12986, 'rok': 12987, 'demilitarized': 12988, '1493': 12989, '1648': 12990, 'assumption': 12991, 'importation': 12992, 'plantations': 12993, 'persisted': 12994, 'anticorruption': 12995, 'abrupt': 12996, 'reefs': 12997, 'beja': 12998, 'commands': 12999, '173': 13000, 'elsa': 13001, 'shortwave': 13002, '159': 13003, 'valleys': 13004, 'jawad': 13005, 'denktash': 13006, 'klebnikov': 13007, 'hanover': 13008, 'declassified': 13009, 'autobiography': 13010, 'norms': 13011, 'definition': 13012, 'overuse': 13013, 'isaias': 13014, 'lublin': 13015, 'synagogue': 13016, 'legislatures': 13017, 'severance': 13018, 'defeating': 13019, 'geographical': 13020, 'boar': 13021, 'helpless': 13022, 'belts': 13023, 'fan': 13024, 'ozawa': 13025, 'brett': 13026, 'prabhakaran': 13027, 'wyoming': 13028, 'chahine': 13029, 'imperialism': 13030, 'solaiman': 13031, 'cnooc': 13032, 'forceful': 13033, 'pollutants': 13034, 'sepat': 13035, 'weathered': 13036, 'voyage': 13037, 'mariam': 13038, 'inventory': 13039, 'mack': 13040, 'sabeel': 13041, 'kafeel': 13042, 'haneef': 13043, 'soliciting': 13044, 'destined': 13045, 'informer': 13046, 'smashing': 13047, 'cohen': 13048, 'hazards': 13049, 'andreev': 13050, 'appease': 13051, 'coleco': 13052, 'anjouan': 13053, 'azali': 13054, 'moreover': 13055, 'bursting': 13056, '1861': 13057, 'benito': 13058, 'mussolini': 13059, 'fascist': 13060, 'azerbaijani': 13061, 'encamped': 13062, 'impediments': 13063, 'chodo': 13064, 'maronite': 13065, 'blog': 13066, 'ilo': 13067, '24th': 13068, 'tomorrow': 13069, 'memorials': 13070, 'bakara': 13071, 'yuschenko': 13072, 'nasiriyah': 13073, 'ansa': 13074, 'sized': 13075, 'bellerive': 13076, 'spreads': 13077, 'analyze': 13078, 'vx': 13079, 'emergence': 13080, 'plenary': 13081, 'kirk': 13082, 'tops': 13083, 'luncheon': 13084, 'peters': 13085, 'guber': 13086, '1840': 13087, 'eligibility': 13088, 'unreliable': 13089, 'kiel': 13090, 'hydrographic': 13091, 'latitude': 13092, 'automated': 13093, 'ought': 13094, 'drunken': 13095, 'quoting': 13096, 'chorus': 13097, 'filtering': 13098, 'regulating': 13099, 'worded': 13100, 'yields': 13101, 'choe': 13102, 'peng': 13103, 'stanley': 13104, 'zinedine': 13105, '157': 13106, 'saves': 13107, 'tirin': 13108, 'farraj': 13109, 'libbi': 13110, 'insein': 13111, 'pad': 13112, 'equator': 13113, 'convey': 13114, 'asserted': 13115, 'stalls': 13116, 'paralympic': 13117, 'chongqing': 13118, 'wheels': 13119, 'decker': 13120, 'prototype': 13121, 'boosters': 13122, 'britons': 13123, '70s': 13124, 'starred': 13125, 'rhymes': 13126, 'kanyarukiga': 13127, 'xerox': 13128, 'crum': 13129, 'forster': 13130, 'comparison': 13131, 'transshipment': 13132, 'hooper': 13133, 'southernmost': 13134, 'ensured': 13135, 'obstructed': 13136, 'huntsmen': 13137, 'revolutionaries': 13138, 'unveil': 13139, 'stick': 13140, 'happens': 13141, 'removes': 13142, 'tribunals': 13143, '540': 13144, 'dunem': 13145, 'discharges': 13146, 'cheeks': 13147, 'frail': 13148, 'directive': 13149, 'aharonot': 13150, 'chileans': 13151, 'murungi': 13152, 'michoacan': 13153, 'shihri': 13154, 'umar': 13155, 'commuted': 13156, 'nottingham': 13157, 'raich': 13158, 'soonthorn': 13159, 'botched': 13160, 'socio': 13161, 'bowed': 13162, 'fonseka': 13163, 'expedition': 13164, '1914': 13165, 'wellington': 13166, 'obscenity': 13167, 'dilute': 13168, 'administers': 13169, 'controllers': 13170, 'ordaz': 13171, 'heroes': 13172, 'obstruction': 13173, 'sprinter': 13174, 'azima': 13175, 'shakeri': 13176, 'samawa': 13177, 'guerilla': 13178, 'subordinates': 13179, 'scarcely': 13180, 'tremors': 13181, 'destructive': 13182, 'regev': 13183, 'landfill': 13184, 'interactive': 13185, 'verez': 13186, 'bencomo': 13187, 'nyathi': 13188, 'filter': 13189, 'jockeys': 13190, 'horne': 13191, 'georges': 13192, 'dominates': 13193, 'yams': 13194, 'bind': 13195, 'occupies': 13196, 'tramp': 13197, 'carved': 13198, 'verification': 13199, 'mengistu': 13200, '156': 13201, 'archives': 13202, 'preview': 13203, 'trader': 13204, 'deporting': 13205, 'chacon': 13206, 'akerson': 13207, 'turnaround': 13208, 'shoddy': 13209, 'bel': 13210, 'intersection': 13211, 'broadband': 13212, 'continuation': 13213, 'sinbad': 13214, 'wikipedia': 13215, 'kye': 13216, 'dinners': 13217, 'supervisor': 13218, 'outposts': 13219, 'intact': 13220, 'bread': 13221, 'taino': 13222, 'dahlan': 13223, 'mahmud': 13224, 'excluding': 13225, 'limitations': 13226, 'crunch': 13227, 'monkey': 13228, 'saluted': 13229, '154': 13230, 'houghton': 13231, 'soulja': 13232, 'sidelined': 13233, 'recorders': 13234, 'bagosora': 13235, 'immunized': 13236, 'routed': 13237, 'sparrows': 13238, 'leeward': 13239, 'yoadimnadji': 13240, 'dell': 13241, 'veerman': 13242, 'emphasizing': 13243, 'jebel': 13244, 'marra': 13245, 'farooq': 13246, 'dense': 13247, 'scaled': 13248, 'novel': 13249, 'humane': 13250, 'blagojevic': 13251, 'logical': 13252, 'betraying': 13253, 'jafarzadeh': 13254, 'rakhine': 13255, 'median': 13256, 'jassar': 13257, 'speedskater': 13258, 'endure': 13259, 'teaches': 13260, 'reasonably': 13261, 'kopra': 13262, 'guaranteeing': 13263, 'unionist': 13264, 'fodder': 13265, 'confer': 13266, 'ruggiero': 13267, 'cannons': 13268, 'jane': 13269, 'fonda': 13270, 'scolded': 13271, 'massey': 13272, 'charleston': 13273, 'ansip': 13274, 'explaining': 13275, 'yugansk': 13276, 'asses': 13277, 'blanketed': 13278, 'rechnitzer': 13279, 'gamma': 13280, 'elgon': 13281, 'exempt': 13282, 'avigdor': 13283, 'shepherds': 13284, 'yayi': 13285, 'emulate': 13286, 'extract': 13287, 'heed': 13288, 'recommends': 13289, 'romano': 13290, 'reinstatement': 13291, 'todovic': 13292, 'alexandre': 13293, 'wikileaks': 13294, 'arian': 13295, 'reschedule': 13296, 'depth': 13297, 'vladislav': 13298, 'vicious': 13299, 'pollsters': 13300, '2013': 13301, 'forge': 13302, 'disappear': 13303, 'reprocessing': 13304, 'invading': 13305, 'compounded': 13306, 'siraj': 13307, 'eldawoody': 13308, 'observes': 13309, 'mas': 13310, 'wit': 13311, 'alias': 13312, 'initials': 13313, 'lao': 13314, 'filmmakers': 13315, 'suli': 13316, 'katyusha': 13317, 'naqoura': 13318, 'nooyi': 13319, 'cholmondeley': 13320, 'sympathetic': 13321, 'neighbour': 13322, 'farmyard': 13323, 'shake': 13324, 'seibu': 13325, 'sogo': 13326, 'diary': 13327, 'suspecting': 13328, 'abating': 13329, 'blacklisted': 13330, '100ths': 13331, 'enlargement': 13332, 'sars': 13333, 'emptive': 13334, 'billboards': 13335, 'blonska': 13336, 'shaways': 13337, 'kareem': 13338, 'hamed': 13339, '373': 13340, 'erode': 13341, 'raouf': 13342, 'cud': 13343, 'hebrides': 13344, 'cultures': 13345, 'didier': 13346, 'bedside': 13347, 'misconduct': 13348, 'detachment': 13349, 'hobart': 13350, 'introduces': 13351, 'reprisal': 13352, 'skirmishes': 13353, 'gwyneth': 13354, 'fisher': 13355, 'portray': 13356, 'retreated': 13357, 'lukic': 13358, 'sling': 13359, 'lament': 13360, 'cries': 13361, 'orator': 13362, 'unblotted': 13363, 'escutcheon': 13364, 'foy': 13365, 'misusing': 13366, 'sibal': 13367, 'campuses': 13368, 'dharamsala': 13369, 'martic': 13370, 'duke': 13371, 'detainment': 13372, 'toss': 13373, 'hoggard': 13374, 'panesar': 13375, 'baba': 13376, 'banditry': 13377, 'dictate': 13378, 'abqaiq': 13379, 'detector': 13380, 'arbor': 13381, 'murderers': 13382, 'empires': 13383, 'manipulation': 13384, 'contra': 13385, 'rope': 13386, 'pastor': 13387, 'divorced': 13388, 'pitting': 13389, 'retroviral': 13390, 'tac': 13391, 'nutrients': 13392, 'dimona': 13393, 'nasdaq': 13394, 'cac': 13395, 'dax': 13396, 'ounce': 13397, 'tomohito': 13398, 'counterfeit': 13399, 'affordable': 13400, 'baki': 13401, 'dunham': 13402, 'alexis': 13403, 'haro': 13404, 'overheating': 13405, 'predictions': 13406, 'cutbacks': 13407, 'concluding': 13408, 'saadi': 13409, 'yedioth': 13410, 'ahronoth': 13411, 'privatized': 13412, 'fundamentals': 13413, 'lows': 13414, 'plummeting': 13415, 'mortally': 13416, 'avalanches': 13417, 'consisting': 13418, 'misguided': 13419, 'gao': 13420, 'shara': 13421, '20s': 13422, 'dream': 13423, 'pretending': 13424, 'variation': 13425, 'hawks': 13426, 'outlaw': 13427, 'explorers': 13428, 'capitalism': 13429, 'rejoined': 13430, 'reaffirm': 13431, 'schuessel': 13432, 'sample': 13433, 'aisha': 13434, 'assailed': 13435, 'taya': 13436, 'leigh': 13437, 'liang': 13438, 'identifies': 13439, 'shadi': 13440, 'unaccounted': 13441, 'raffle': 13442, 'aol': 13443, 'distinct': 13444, 'estrada': 13445, 'premiums': 13446, 'exposing': 13447, 'tareen': 13448, 'inserted': 13449, 'abbott': 13450, 'xii': 13451, 'platon': 13452, 'alexeyev': 13453, 'kappes': 13454, 'quarterback': 13455, 'thu': 13456, 'mende': 13457, 'merchandise': 13458, 'cozumel': 13459, 'guttenberg': 13460, 'schneiderhan': 13461, 'cheapest': 13462, 'atolls': 13463, 'altered': 13464, 'origins': 13465, 'gymnast': 13466, 'backgrounds': 13467, 'curator': 13468, 'shula': 13469, 'laughing': 13470, 'believing': 13471, 'chambliss': 13472, 'rohrabacher': 13473, '121': 13474, 'lions': 13475, 'touchdowns': 13476, 'tablet': 13477, 'seaside': 13478, '3d': 13479, 'fronts': 13480, 'waive': 13481, 'shrines': 13482, 'storming': 13483, 'saudis': 13484, 'zurich': 13485, '380': 13486, 'superjumbo': 13487, 'alu': 13488, 'sadeq': 13489, 'horrified': 13490, 'kramer': 13491, 'abizaid': 13492, 'booster': 13493, 'rowing': 13494, 'adolfo': 13495, 'smyr': 13496, 'confidentiality': 13497, 'skulls': 13498, 'oceanic': 13499, 'turtles': 13500, 'equatoria': 13501, 'facilitated': 13502, 'banana': 13503, 'uloum': 13504, 'berman': 13505, 'aka': 13506, 'acapulco': 13507, 'rood': 13508, 'akayeva': 13509, 'reactions': 13510, 'approves': 13511, 'genoino': 13512, 'nestle': 13513, 'schoch': 13514, 'bruhin': 13515, 'baptist': 13516, 'vuitton': 13517, 'canterbury': 13518, 'archive': 13519, 'flashing': 13520, 'tombstone': 13521, 'manar': 13522, 'iqbal': 13523, 'gene': 13524, 'conducive': 13525, 'yakubov': 13526, 'peso': 13527, 'afforded': 13528, 'groaned': 13529, 'rehn': 13530, 'manipulate': 13531, 'demonizing': 13532, 'haze': 13533, 'lugar': 13534, 'satisfy': 13535, 'dad': 13536, 'broadened': 13537, 'arsala': 13538, 'sheet': 13539, 'glaciers': 13540, 'corpus': 13541, 'christi': 13542, 'redraw': 13543, 'combining': 13544, 'agitated': 13545, 'walikale': 13546, 'exotic': 13547, 'maaleh': 13548, 'nullified': 13549, '224': 13550, 'khayam': 13551, 'cola': 13552, 'cse': 13553, 'stringent': 13554, 'organs': 13555, 'miljus': 13556, 'bankrupt': 13557, 'balaguer': 13558, 'catholicism': 13559, 'vasp': 13560, 'raviglione': 13561, 'eavesdrop': 13562, '256': 13563, 'midway': 13564, 'nwr': 13565, 'shbak': 13566, 'gasquet': 13567, 'mirnyi': 13568, 'byzantine': 13569, 'guild': 13570, 'cate': 13571, 'blanchett': 13572, 'europol': 13573, 'nhs': 13574, 'paktiawal': 13575, '163': 13576, 'verveer': 13577, 'naimi': 13578, 'ellice': 13579, 'tonga': 13580, 'zanzibar': 13581, 'fowler': 13582, 'myself': 13583, 'disengagement': 13584, 'shas': 13585, 'abolish': 13586, 'kononov': 13587, 'solecki': 13588, 'montagnards': 13589, 'tokage': 13590, 'kindergarten': 13591, 'hound': 13592, 'clauses': 13593, 'jo': 13594, 'negotiation': 13595, 'matesi': 13596, 'quist': 13597, 'petkoff': 13598, 'larose': 13599, 'arising': 13600, 'depended': 13601, 'anp': 13602, 'hares': 13603, '258': 13604, 'realizing': 13605, 'gration': 13606, 'saqib': 13607, 'nazareth': 13608, 'jamaican': 13609, 'emigrated': 13610, 'clarifies': 13611, 'meoni': 13612, 'benisede': 13613, 'jimenez': 13614, 'bergman': 13615, 'idema': 13616, 'hornets': 13617, 'rezai': 13618, 'preventive': 13619, '29th': 13620, 'olpc': 13621, 'samper': 13622, 'methane': 13623, 'vortex': 13624, 'pokhrel': 13625, 'ahnlund': 13626, 'clemens': 13627, 'frightening': 13628, 'housedog': 13629, 'collier': 13630, 'ousting': 13631, 'greeting': 13632, 'zim': 13633, 'merapi': 13634, 'merimee': 13635, 'demirel': 13636, 'aalam': 13637, 'fiat': 13638, 'kamin': 13639, 'pamphlets': 13640, 'ghost': 13641, 'mswati': 13642, 'soprano': 13643, 'costeira': 13644, 'rowers': 13645, 'azizuddin': 13646, 'pitt': 13647, 'diouf': 13648, 'minuteman': 13649, 'mirrors': 13650, 'mongols': 13651, 'hen': 13652, 'bittok': 13653, 'ney': 13654, 'johanns': 13655, 'quail': 13656, 'phrases': 13657, 'nightingale': 13658, 'kindhearts': 13659, 'rhee': 13660, 'sbs': 13661, 'prints': 13662, 'inoperable': 13663, 'murtha': 13664, 'rostropovich': 13665, 'monte': 13666, 'heifer': 13667, 'worm': 13668, 'ferrying': 13669, 'bottlenecks': 13670, 'sow': 13671, 'trophy': 13672, 'geir': 13673, 'countless': 13674, 'calvin': 13675, 'broadus': 13676, 'crips': 13677, 'calamity': 13678, 'engages': 13679, 'complimentary': 13680, 'overtaking': 13681, 'peruvians': 13682, 'comandante': 13683, 'ramona': 13684, 'zapatistas': 13685, 'rumored': 13686, 'mask': 13687, 'concept': 13688, 'immunize': 13689, '2015': 13690, 'barakat': 13691, 'keyser': 13692, 'assessments': 13693, '2030': 13694, 'responsibly': 13695, 'stays': 13696, 'nicephore': 13697, 'soglo': 13698, 'ignorance': 13699, 'sheds': 13700, 'rebuffed': 13701, 'snows': 13702, 'accessible': 13703, 'dia': 13704, '1819': 13705, 'qatari': 13706, 'backbone': 13707, 'outlying': 13708, 'unskilled': 13709, 'bundle': 13710, 'rendered': 13711, 'wasted': 13712, 'tetiana': 13713, 'koprowicz': 13714, 'consul': 13715, 'steadfastly': 13716, 'udhampur': 13717, 'brisk': 13718, 'superpower': 13719, 'qalat': 13720, 'flaming': 13721, 'downplay': 13722, 'baduel': 13723, 'formalize': 13724, 'condemnations': 13725, 'transplants': 13726, 'jama': 13727, 'dora': 13728, 'formidable': 13729, 'sweilam': 13730, 'payrolls': 13731, 'indicator': 13732, 'tend': 13733, 'census': 13734, 'petrocaribe': 13735, 'jaafar': 13736, 'diabetics': 13737, 'exhuming': 13738, 'garage': 13739, 'skeletons': 13740, 'massacred': 13741, 'revere': 13742, 'hrbaty': 13743, 'adelaide': 13744, 'onslaught': 13745, 'bouncing': 13746, 'hernych': 13747, 'junaid': 13748, 'philippoussis': 13749, 'seppi': 13750, 'florent': 13751, 'residences': 13752, 'skiers': 13753, 'stalling': 13754, 'arif': 13755, 'h1': 13756, 'balata': 13757, 'clout': 13758, 'omi': 13759, 'measurable': 13760, 'sandstorms': 13761, 'runways': 13762, 'krzysztof': 13763, 'visionary': 13764, 'taunting': 13765, 'bujumbura': 13766, 'fnl': 13767, 'remanded': 13768, 'ismael': 13769, 'oleg': 13770, 'privilege': 13771, 'gourmet': 13772, 'idb': 13773, 'enrolled': 13774, 'composer': 13775, 'ink': 13776, 'pencil': 13777, 'gara': 13778, 'portrayed': 13779, 'centerpiece': 13780, 'zenith': 13781, 'depleted': 13782, 'daltrey': 13783, '1563': 13784, 'townshend': 13785, 'barely': 13786, 'volatility': 13787, 'inherited': 13788, 'owing': 13789, 'nutmeg': 13790, 'philosopher': 13791, 'providence': 13792, 'perish': 13793, 'reflections': 13794, 'chaldean': 13795, 'pablo': 13796, 'chips': 13797, 'seville': 13798, 'mansoorain': 13799, 'excellence': 13800, 'propulsion': 13801, 'kariye': 13802, 'lindsey': 13803, 'howes': 13804, 'huot': 13805, 'wat': 13806, 'eisenman': 13807, 'evokes': 13808, 'archer': 13809, 'extrajudicial': 13810, 'farmhouse': 13811, 'proportionately': 13812, 'encounters': 13813, 'lax': 13814, 'daybreak': 13815, 'itv': 13816, 'reeling': 13817, 'kevljani': 13818, 'safavi': 13819, 'allah': 13820, 'dimitris': 13821, 'christofias': 13822, 'centenary': 13823, 'burge': 13824, 'sattler': 13825, 'wired': 13826, "o'hara": 13827, 'limiting': 13828, 'brushing': 13829, 'azamiyah': 13830, 'amiriyah': 13831, 'eutelsat': 13832, 'infertility': 13833, 'patent': 13834, 'competitor': 13835, 'shaab': 13836, 'overdue': 13837, 'redeploy': 13838, 'khurshid': 13839, 'hardliners': 13840, 'tcdd': 13841, '268': 13842, 'regrouped': 13843, 'eluded': 13844, 'divulging': 13845, 'bazaar': 13846, 'innocents': 13847, 'bagpipes': 13848, 'lawn': 13849, 'shanksville': 13850, 'pokhara': 13851, 'legitimize': 13852, 'specifies': 13853, 'precision': 13854, 'collaborators': 13855, 'jailhouse': 13856, 'debby': 13857, 'stephanides': 13858, 'siphoned': 13859, 'devised': 13860, 'settling': 13861, 'nascent': 13862, 'cans': 13863, 'jang': 13864, 'appalling': 13865, 'prohibits': 13866, 'canned': 13867, 'warehouses': 13868, 'shallow': 13869, 'pens': 13870, 'philanthropist': 13871, '1896': 13872, '1901': 13873, 'unexplored': 13874, 'rehabilitate': 13875, 'lecturer': 13876, 'gai': 13877, 'disability': 13878, 'integrate': 13879, 'disabilities': 13880, 'rai': 13881, 'beneficiaries': 13882, 'amiin': 13883, 'illiteracy': 13884, 'disparity': 13885, 'fluctuating': 13886, 'swings': 13887, 'undeveloped': 13888, 'taunted': 13889, 'headlights': 13890, 'unjust': 13891, 'remind': 13892, 'apples': 13893, 'lonely': 13894, 'graner': 13895, 'sabrina': 13896, 'orbits': 13897, 'capsule': 13898, '2018': 13899, 'landings': 13900, 'enslaved': 13901, 'preempt': 13902, 'taksim': 13903, 'spans': 13904, 'monasteries': 13905, 'burton': 13906, 'austrians': 13907, 'casts': 13908, 'girija': 13909, 'saadoun': 13910, 'skirted': 13911, 'suffocating': 13912, 'stopover': 13913, 'takeoff': 13914, 'azarov': 13915, 'waits': 13916, 'bury': 13917, 'nanmadol': 13918, 'tchiroma': 13919, 'examinations': 13920, 'bakassi': 13921, 'advancement': 13922, 'flavinols': 13923, 'motorway': 13924, 'intermediary': 13925, 'abandons': 13926, 'precedents': 13927, 'schalkwyk': 13928, '2025': 13929, 'deif': 13930, 'humiliation': 13931, 'teimuraz': 13932, 'gabashvili': 13933, 'subur': 13934, 'sugiarto': 13935, 'shin': 13936, 'intoxicated': 13937, 'infringe': 13938, 'rudolf': 13939, 'apostolic': 13940, 'eucharist': 13941, 'assadullah': 13942, 'parwan': 13943, 'crucifixes': 13944, 'reverence': 13945, 'oxford': 13946, 'researcher': 13947, 'facial': 13948, 'thinning': 13949, 'disturbing': 13950, 'exceptions': 13951, 'circumvent': 13952, 'wording': 13953, 'inconvenient': 13954, 'rohani': 13955, 'nevsky': 13956, 'prospekt': 13957, 'cater': 13958, 'interpretation': 13959, 'ghoul': 13960, 'bust': 13961, 'holmes': 13962, 'summons': 13963, 'certify': 13964, 'zaman': 13965, 'cds': 13966, 'laptops': 13967, 'sardinia': 13968, 'bologna': 13969, 'ideologies': 13970, 'pretrial': 13971, 'motions': 13972, 'drawdown': 13973, 'marginalized': 13974, 'qinghong': 13975, 'trinidad': 13976, 'tobago': 13977, 'widower': 13978, 'zimmermann': 13979, 'grubbs': 13980, 'schrock': 13981, 'yves': 13982, 'chauvin': 13983, 'molecules': 13984, 'reproduce': 13985, 'impassable': 13986, 'branded': 13987, 'tottenham': 13988, 'stint': 13989, 'teammates': 13990, 'achilles': 13991, 'bowen': 13992, 'aleksander': 13993, 'caicos': 13994, 'eilat': 13995, 'armorgroup': 13996, 'outburst': 13997, 'enters': 13998, '108': 13999, '1719': 14000, 'eurozone': 14001, 'zeta': 14002, 'invoked': 14003, 'threshold': 14004, 'mutiny': 14005, 'aggrieved': 14006, 'proportional': 14007, 'leases': 14008, 'soils': 14009, 'reins': 14010, 'saddled': 14011, 'foil': 14012, 'dear': 14013, 'flourishing': 14014, 'boxing': 14015, 'accountant': 14016, 'assignment': 14017, 'doomed': 14018, 'erben': 14019, 'kanyabayonga': 14020, 'fragments': 14021, 'dwellers': 14022, 'urgency': 14023, 'renovation': 14024, 'hottest': 14025, 'appliances': 14026, 'yunis': 14027, 'forgives': 14028, 'humiliations': 14029, '386': 14030, 'somalians': 14031, 'miltzow': 14032, 'unloaded': 14033, 'torgelow': 14034, 'qualities': 14035, 'hamidzada': 14036, 'misunderstanding': 14037, 'aleem': 14038, 'siddique': 14039, 'ntv': 14040, 'forget': 14041, 'nonsmokers': 14042, 'implies': 14043, 'suef': 14044, 'baden': 14045, 'rationale': 14046, 'hypertension': 14047, 'squared': 14048, '722': 14049, 'rajouri': 14050, 'juncker': 14051, 'judgments': 14052, 'boasted': 14053, 'plo': 14054, 'hayward': 14055, 'skeptical': 14056, 'leon': 14057, 'mezni': 14058, 'jendayi': 14059, 'frazer': 14060, 'khawaza': 14061, 'desires': 14062, 'layer': 14063, 'glitch': 14064, 'tumbled': 14065, 'stave': 14066, 'seyni': 14067, 'yanukovich': 14068, 'inadmissible': 14069, 'sliders': 14070, 'lund': 14071, 'hydrogen': 14072, 'unrealistic': 14073, 'tachileik': 14074, '756': 14075, 'carnage': 14076, 'ignite': 14077, 'degraded': 14078, 'revitalizing': 14079, 'jumpstart': 14080, 'erbamont': 14081, 'blaise': 14082, 'compaore': 14083, 'stubbornly': 14084, 'recreation': 14085, 'meadow': 14086, 'happiness': 14087, 'groom': 14088, 'alas': 14089, 'scoundrel': 14090, 'pitching': 14091, 'excessively': 14092, 'preparedness': 14093, 'festivals': 14094, 'montserrat': 14095, 'qualifiers': 14096, 'surprising': 14097, 'stunning': 14098, 'niamey': 14099, 'stade': 14100, 'essam': 14101, 'stallone': 14102, 'nagpur': 14103, 'oral': 14104, 'histories': 14105, 'radiation': 14106, 'ulcers': 14107, 'alcoholic': 14108, 'feeds': 14109, 'hartford': 14110, 'equip': 14111, 'repatriating': 14112, 'renovating': 14113, 'holes': 14114, 'solo': 14115, "t'bilisi": 14116, 'akhalkalaki': 14117, 'capitalize': 14118, 'entirety': 14119, 'gratitude': 14120, 'alexy': 14121, 'yakutsk': 14122, 'epiphany': 14123, 'iacovou': 14124, 'sadrist': 14125, 'boomed': 14126, '266': 14127, 'agoa': 14128, 'firewood': 14129, 'persuaded': 14130, 'outlandish': 14131, 'anytime': 14132, 'penal': 14133, 'filibuster': 14134, 'priscilla': 14135, '2019': 14136, 'mankind': 14137, 'prevailed': 14138, 'heaven': 14139, 'righteous': 14140, 'consulted': 14141, 'juvenal': 14142, 'habyarimana': 14143, 'attaché': 14144, 'decreed': 14145, 'henceforth': 14146, 'illustrate': 14147, 'eliezer': 14148, 'ueberroth': 14149, 'arises': 14150, 'singly': 14151, 'yasin': 14152, 'attendants': 14153, 'varieties': 14154, 'wagner': 14155, 'valle': 14156, 'stripping': 14157, 'redefine': 14158, 'ilam': 14159, 'tang': 14160, 'undertaking': 14161, 'drunkenness': 14162, 'kaliningrad': 14163, 'refuted': 14164, 'tribespeople': 14165, 'deutsche': 14166, 'welle': 14167, 'musayyib': 14168, 'atrocious': 14169, 'pronounced': 14170, 'mourned': 14171, 'rendition': 14172, 'kearny': 14173, 'irrigated': 14174, 'nina': 14175, 'simone': 14176, 'margins': 14177, 'barba': 14178, 'berdimuhamedow': 14179, 'polyglot': 14180, 'frog': 14181, 'lame': 14182, 'wrinkled': 14183, 'snake': 14184, 'filberts': 14185, 'grasped': 14186, 'readily': 14187, 'malakand': 14188, '28th': 14189, 'damper': 14190, 'entice': 14191, 'himat': 14192, 'cheerful': 14193, 'kwame': 14194, 'nkrumah': 14195, 'gongadze': 14196, 'maltreatment': 14197, 'mistrial': 14198, 'bowman': 14199, 'bahraini': 14200, 'aiyar': 14201, 'pangandaran': 14202, 'skipped': 14203, 'herds': 14204, '5000': 14205, 'scorched': 14206, 'robinson': 14207, 'nasal': 14208, 'bless': 14209, 'hinted': 14210, 'timely': 14211, 'canceling': 14212, 'saboteurs': 14213, 'blessed': 14214, 'devout': 14215, 'emotions': 14216, 'publicize': 14217, 'ditch': 14218, 'vaeedi': 14219, '787': 14220, 'facilitator': 14221, 'guiding': 14222, 'glenn': 14223, 'antitrust': 14224, '633': 14225, 'domodedovo': 14226, 'ria': 14227, 'guess': 14228, 'millimeter': 14229, 'balochistan': 14230, '146': 14231, 'ignores': 14232, 'kryvorizhstal': 14233, 'woefully': 14234, 'providers': 14235, '429': 14236, 'locales': 14237, 'indoors': 14238, 'trades': 14239, 'concealing': 14240, 'conscripted': 14241, 'shallah': 14242, 'gilad': 14243, 'randomly': 14244, 'salerno': 14245, 'duel': 14246, 'maxim': 14247, 'snowboarding': 14248, 'kelly': 14249, 'encompass': 14250, '676': 14251, 'rossi': 14252, '387': 14253, 'compiling': 14254, 'shoved': 14255, 'pediatricians': 14256, '85th': 14257, 'mullaittivu': 14258, 'upheaval': 14259, 'duncan': 14260, 'denounces': 14261, 'hallwood': 14262, 'masoum': 14263, 'piped': 14264, 'repository': 14265, 'idps': 14266, 'plumage': 14267, 'pools': 14268, 'habit': 14269, 'nettle': 14270, 'boldly': 14271, 'philosophy': 14272, 'hiking': 14273, 'draining': 14274, 'ninety': 14275, 'aerospace': 14276, 'extorting': 14277, 'ransoms': 14278, 'trainers': 14279, 'davies': 14280, 'override': 14281, 'derailment': 14282, 'mangled': 14283, 'griffal': 14284, 'joye': 14285, 'quinn': 14286, 'medalists': 14287, 'myles': 14288, '161': 14289, 'erin': 14290, 'courtney': 14291, 'yang': 14292, 'bhat': 14293, 'stroll': 14294, 'colored': 14295, 'marlins': 14296, 'shofar': 14297, 'birkenau': 14298, 'cassim': 14299, '530': 14300, 'sterility': 14301, 'spotlight': 14302, 'padden': 14303, 'lapses': 14304, '177': 14305, 'delgado': 14306, 'oliver': 14307, 'raad': 14308, 'hashim': 14309, 'darren': 14310, 'capping': 14311, 'laith': 14312, 'rockslide': 14313, 'manshiyet': 14314, 'nasr': 14315, 'rockslides': 14316, 'grains': 14317, 'sidnaya': 14318, 'scanned': 14319, 'spear': 14320, 'karabila': 14321, 'experimental': 14322, 'gardasil': 14323, 'cancerous': 14324, 'hpvs': 14325, 'kiraithe': 14326, 'hanif': 14327, 'hai': 14328, 'puppets': 14329, 'milliyet': 14330, 'willingly': 14331, 'everglades': 14332, 'behnam': 14333, 'nateghi': 14334, 'tearful': 14335, 'raphael': 14336, '298': 14337, 'laps': 14338, 'itno': 14339, 'mulino': 14340, 'kempthorne': 14341, 'regulates': 14342, 'rightwing': 14343, 'gabriel': 14344, 'unthinkable': 14345, 'stunt': 14346, 'kosachyov': 14347, 'faxed': 14348, 'shein': 14349, 'competitions': 14350, '153': 14351, 'incorporation': 14352, 'harmonize': 14353, 'readmitted': 14354, 'float': 14355, 'depreciate': 14356, 'sanction': 14357, 'localities': 14358, '1848': 14359, '1874': 14360, 'jealous': 14361, 'omen': 14362, 'loudly': 14363, 'wondered': 14364, 'caw': 14365, 'tanner': 14366, 'smell': 14367, 'inconvenience': 14368, 'centre': 14369, 'dissemble': 14370, 'pond': 14371, 'shantytowns': 14372, 'leaned': 14373, 'scotia': 14374, 'varied': 14375, '116': 14376, 'sook': 14377, 'omdurman': 14378, 'chapters': 14379, 'alienated': 14380, 'barges': 14381, 'wisconsin': 14382, 'derailing': 14383, 'educating': 14384, 'transatlantic': 14385, 'kar': 14386, 'seismological': 14387, 'hillsides': 14388, 'guizhou': 14389, 'repealing': 14390, 'regressing': 14391, 'substitute': 14392, 'deflected': 14393, 'shaktoi': 14394, 'hakimullah': 14395, 'schapelle': 14396, 'corby': 14397, 'toughest': 14398, 'savic': 14399, 'visegrad': 14400, 'recreated': 14401, 'vargas': 14402, 'importer': 14403, 'kamchatka': 14404, 'remorse': 14405, 'unfit': 14406, 'mutilation': 14407, 'circumcision': 14408, 'abusers': 14409, 'nyala': 14410, 'naturalized': 14411, 'marseille': 14412, 'analyzing': 14413, 'uproar': 14414, 'kuznetsov': 14415, 'fabricating': 14416, 'massacring': 14417, 'princess': 14418, 'dragomir': 14419, 'airstrips': 14420, 'yusef': 14421, 'manchester': 14422, 'muteiry': 14423, 'fawaz': 14424, 'nineteen': 14425, 'mara': 14426, 'salvatrucha': 14427, 'chandler': 14428, 'yacht': 14429, 'accumulation': 14430, 'ningxia': 14431, 'barzan': 14432, 'divorcing': 14433, 'pamela': 14434, 'exempts': 14435, '197': 14436, 'inconsistencies': 14437, 'blindness': 14438, 'saqiz': 14439, 'radioed': 14440, 'mammals': 14441, 'drown': 14442, 'molested': 14443, 'narsingh': 14444, 'disqualify': 14445, 'overly': 14446, 'exposition': 14447, 'homing': 14448, 'fetched': 14449, 'jewelers': 14450, 'moriarty': 14451, 'arable': 14452, 'bozize': 14453, 'persist': 14454, 'sparsely': 14455, 'jalil': 14456, 'greedily': 14457, 'mortal': 14458, 'agony': 14459, 'windfall': 14460, 'desiring': 14461, 'novakatka': 14462, 'novakatkan': 14463, 'novakatkans': 14464, 'charki': 14465, 'heidar': 14466, 'moslehi': 14467, 'expediency': 14468, '404': 14469, 'electrician': 14470, 'bibles': 14471, 'slit': 14472, 'malatya': 14473, 'demonstrator': 14474, 'intensity': 14475, 'appeasing': 14476, 'taunt': 14477, 'affront': 14478, 'punched': 14479, 'shoulders': 14480, 'cremation': 14481, 'uncertainties': 14482, 'stakeholder': 14483, 'formations': 14484, 'mystery': 14485, 'wheeled': 14486, 'overweight': 14487, 'obese': 14488, 'clearer': 14489, 'bonny': 14490, 'overboard': 14491, 'birmingham': 14492, 'circulatory': 14493, 'antonovs': 14494, 'naypyidaw': 14495, 'hwan': 14496, 'alperon': 14497, 'careers': 14498, 'balloons': 14499, 'resemble': 14500, 'hat': 14501, 'lamppost': 14502, 'bayrou': 14503, 'patron': 14504, 'leyva': 14505, 'caverns': 14506, 'hapoalim': 14507, 'schwab': 14508, 'lender': 14509, 'lars': 14510, 'bountiful': 14511, 'osuji': 14512, 'comprising': 14513, 'bragg': 14514, 'bolivarian': 14515, 'orchestrate': 14516, 'liberated': 14517, 'exterminate': 14518, 'darom': 14519, 'evaded': 14520, 'kato': 14521, 'sustaining': 14522, 'possibilities': 14523, '44th': 14524, 'soo': 14525, 'wonderful': 14526, 'professionalism': 14527, 'norman': 14528, 'swords': 14529, 'righteousness': 14530, 'rebellious': 14531, 'arusha': 14532, 'demagogue': 14533, 'fidelity': 14534, 'kane': 14535, 'tunisians': 14536, 'terminated': 14537, 'modernizing': 14538, 'emphasis': 14539, 'competes': 14540, 'furniture': 14541, 'paints': 14542, 'tiles': 14543, 'outflows': 14544, 'cushion': 14545, 'varying': 14546, 'abdoulaye': 14547, 'horseman': 14548, 'faculty': 14549, 'sink': 14550, 'melissa': 14551, 'schoomaker': 14552, 'triumph': 14553, 'cleland': 14554, 'steer': 14555, 'leumi': 14556, 'stockpiling': 14557, 'enticed': 14558, 'slovenian': 14559, 'obeid': 14560, 'sutanto': 14561, 'batu': 14562, 'masterminding': 14563, '69th': 14564, 'fiorina': 14565, 'hp': 14566, 'resisting': 14567, 'vintsuk': 14568, 'vyachorka': 14569, 'quashing': 14570, 'keen': 14571, 'preceded': 14572, 'mireya': 14573, 'moscoso': 14574, 'servers': 14575, 'mejia': 14576, 'waterway': 14577, 'baikal': 14578, 'twins': 14579, 'fiancee': 14580, 'monoxide': 14581, 'adriano': 14582, 'ronaldinho': 14583, 'naftogaz': 14584, 'sullivan': 14585, 'ekaterina': 14586, 'peer': 14587, 'aiko': 14588, 'nakamura': 14589, 'cassation': 14590, 'yoon': 14591, 'jeong': 14592, 'pianist': 14593, 'irina': 14594, 'leverage': 14595, 'calmed': 14596, 'notable': 14597, 'cricketers': 14598, 'intentionally': 14599, 'imitation': 14600, 'aleppo': 14601, 'sofyan': 14602, 'edited': 14603, 'ambiguous': 14604, 'rick': 14605, 'misunderstood': 14606, 'pattaya': 14607, 'homelessness': 14608, 'crowns': 14609, 'atagi': 14610, 'adamantly': 14611, 'faizabad': 14612, 'kohistan': 14613, 'jeanne': 14614, 'sorin': 14615, 'miscoci': 14616, 'ovidiu': 14617, 'ohanesian': 14618, 'crnogorac': 14619, 'banja': 14620, 'luka': 14621, 'minustah': 14622, 'mansoor': 14623, 'ayodhya': 14624, 'atoms': 14625, 'anatolian': 14626, 'mathematically': 14627, 'runoffs': 14628, 'galina': 14629, 'dogged': 14630, 'firebombing': 14631, 'yosef': 14632, 'hsann': 14633, 'subsidizing': 14634, 'greedy': 14635, 'packaging': 14636, 'discontinued': 14637, 'pretax': 14638, '133': 14639, 'reorganize': 14640, 'fertility': 14641, 'municipalities': 14642, 'chronically': 14643, 'deepest': 14644, 'upheavals': 14645, 'culminating': 14646, 'retribution': 14647, 'zaire': 14648, 'rout': 14649, 'perfumes': 14650, 'apt': 14651, 'till': 14652, 'forgot': 14653, 'robes': 14654, 'huntsman': 14655, 'basket': 14656, 'abstain': 14657, 'dragging': 14658, 'inaction': 14659, 'fluid': 14660, 'claudia': 14661, 'complication': 14662, 'ted': 14663, 'greats': 14664, 'parker': 14665, 'hooker': 14666, 'eyesight': 14667, 'surgeons': 14668, 'lasts': 14669, 'flashpoint': 14670, 'golf': 14671, 'vera': 14672, 'amelie': 14673, 'stewardship': 14674, 'dealership': 14675, 'daring': 14676, 'phony': 14677, 'hyung': 14678, 'araujo': 14679, 'maltreating': 14680, 'rampaging': 14681, 'seclusion': 14682, 'grinning': 14683, 'sunk': 14684, 'compliant': 14685, 'respirator': 14686, 'inhumane': 14687, 'bayaman': 14688, 'kara': 14689, 'queensland': 14690, 'nicknamed': 14691, 'cooper': 14692, 'ranchers': 14693, 'necks': 14694, 'rishawi': 14695, 'bluntly': 14696, 'possessed': 14697, 'mozambican': 14698, 'anjar': 14699, 'wagah': 14700, 'kurzban': 14701, 'depleting': 14702, 'berdych': 14703, 'novak': 14704, 'djokovic': 14705, 'verdasco': 14706, 'nikolay': 14707, 'davydenko': 14708, 'branislav': 14709, 'warplane': 14710, 'cartosat': 14711, 'hamsat': 14712, 'sriharokota': 14713, 'pslav': 14714, 'askari': 14715, 'platero': 14716, 'synagogues': 14717, 'advertise': 14718, 'weary': 14719, 'altercation': 14720, 'mansur': 14721, 'panicking': 14722, 'fairer': 14723, 'deepening': 14724, 'resorted': 14725, 'turkmens': 14726, 'depress': 14727, 'steepest': 14728, 'ryazanov': 14729, 'sundance': 14730, 'monica': 14731, 'surgeries': 14732, 'rays': 14733, 'pyinmana': 14734, 'soren': 14735, 'vuk': 14736, 'draskovic': 14737, 'gereshk': 14738, 'sid': 14739, 'outlay': 14740, 'guebuza': 14741, 'disqualification': 14742, 'terminate': 14743, 'discouraged': 14744, 'awori': 14745, 'giuliana': 14746, 'blockaded': 14747, 'conserve': 14748, 'necessarily': 14749, 'laments': 14750, 'stove': 14751, 'onitsha': 14752, 'anambra': 14753, 'scuffle': 14754, 'sparks': 14755, 'overshadowed': 14756, 'repercussions': 14757, 'persecuting': 14758, 'calmer': 14759, 'succumb': 14760, 'shahristani': 14761, 'timeframe': 14762, 'whites': 14763, 'molesting': 14764, 'mannar': 14765, 'squandered': 14766, 'tiebreaker': 14767, 'vliegen': 14768, 'seymour': 14769, 'drowning': 14770, 'electrocution': 14771, 'sprinters': 14772, 'render': 14773, 'provisionally': 14774, 'multimillion': 14775, 'napoleon': 14776, 'grooming': 14777, 'dn': 14778, 'ethnicity': 14779, 'pedophiles': 14780, 'halls': 14781, 'infiltrators': 14782, '950': 14783, 'straining': 14784, 'truckloads': 14785, 'weakest': 14786, 'baluchis': 14787, 'mendez': 14788, 'soweto': 14789, '423': 14790, 'constitutes': 14791, 'attiya': 14792, 'migrated': 14793, 'builds': 14794, 'lutfullah': 14795, 'mashal': 14796, 'newborn': 14797, 'yinghua': 14798, 'cubs': 14799, 'authenticate': 14800, 'algerians': 14801, 'facilitation': 14802, 'daytime': 14803, 'neglecting': 14804, 'dadis': 14805, 'erecting': 14806, 'knock': 14807, 'celebratory': 14808, 'turpan': 14809, 'offshoot': 14810, 'elco': 14811, '1829': 14812, 'protracted': 14813, 'hydrocarbon': 14814, 'saparmurat': 14815, 'nyyazow': 14816, 'pearls': 14817, 'rekindled': 14818, 'tongue': 14819, 'thistles': 14820, 'kari': 14821, 'reveals': 14822, 'dumarsais': 14823, '134': 14824, 'symbolizing': 14825, 'thais': 14826, 'pacts': 14827, 'disadvantage': 14828, 'looms': 14829, 'citroen': 14830, 'dongfeng': 14831, 'uniting': 14832, 'strips': 14833, 'crumpton': 14834, '324': 14835, 'paired': 14836, 'relocating': 14837, 'immigrated': 14838, 'dictatorial': 14839, 'rejections': 14840, 'riga': 14841, 'tirah': 14842, 'azar': 14843, 'fracture': 14844, 'bride': 14845, 'sting': 14846, 'switched': 14847, 'vitaly': 14848, 'churkin': 14849, 'tattoo': 14850, 'storied': 14851, 'bordered': 14852, 'hefty': 14853, 'walesa': 14854, 'speedy': 14855, 'nida': 14856, 'simpson': 14857, 'ashley': 14858, 'stalinist': 14859, 'kharazzi': 14860, 'kocharian': 14861, 'characterizing': 14862, '216': 14863, 'thanh': 14864, 'hoa': 14865, 'nalchik': 14866, 'reliable': 14867, 'maulvi': 14868, 'decentralized': 14869, 'resilience': 14870, 'gonzalo': 14871, 'dimming': 14872, 'nonessential': 14873, 'riches': 14874, 'pyramids': 14875, 'cube': 14876, 'candlelight': 14877, 'maarib': 14878, 'aqili': 14879, 'phasing': 14880, 'midsized': 14881, 'muscat': 14882, 'qaboos': 14883, 'reshuffled': 14884, 'ostensible': 14885, 'antigovernment': 14886, 'dredging': 14887, 'locks': 14888, 'wonder': 14889, 'grudge': 14890, 'metin': 14891, 'kaplan': 14892, 'ataturk': 14893, 'basilan': 14894, 'precipitation': 14895, 'zionist': 14896, 'lucky': 14897, 'marshals': 14898, 'roasted': 14899, 'nicole': 14900, 'mitofsky': 14901, 'umaru': 14902, 'edged': 14903, 'medellin': 14904, 'assassinating': 14905, 'punk': 14906, 'mest': 14907, 'matt': 14908, 'label': 14909, 'disbanding': 14910, 'blumenthal': 14911, 'swimmers': 14912, '956': 14913, 'markos': 14914, 'kongsak': 14915, 'bangguo': 14916, 'mebki': 14917, 'bouake': 14918, 'ivorians': 14919, 'contestants': 14920, 'ljoekelsoey': 14921, 'jumps': 14922, '788': 14923, 'widhoelzl': 14924, 'gunboats': 14925, 'madden': 14926, 'charlotte': 14927, 'halfway': 14928, 'drinkers': 14929, 'hamburgers': 14930, 'hey': 14931, 'download': 14932, 'hamburg': 14933, 'yazidi': 14934, 'sinjar': 14935, 'qasim': 14936, 'saeedi': 14937, 'disorders': 14938, 'prussia': 14939, 'levies': 14940, 'nioka': 14941, 'spied': 14942, 'amisom': 14943, 'bahonar': 14944, 'vahidi': 14945, 'vahid': 14946, 'dilapidated': 14947, 'sajjad': 14948, 'sharki': 14949, 'shadid': 14950, 'thoroughly': 14951, 'depots': 14952, 'culmination': 14953, 'belatedly': 14954, 'gala': 14955, 'danes': 14956, '937': 14957, 'wrongfully': 14958, 'purged': 14959, 'rafts': 14960, 'banjul': 14961, 'privatizing': 14962, 'simplify': 14963, 'autopsy': 14964, 'cyclical': 14965, 'thune': 14966, 'employer': 14967, 'ed': 14968, 'xingning': 14969, 'harmonized': 14970, 'mutahida': 14971, 'betterment': 14972, 'retaining': 14973, 'consolidated': 14974, 'tub': 14975, 'baked': 14976, 'weddings': 14977, 'milling': 14978, 'leather': 14979, 'jute': 14980, '1907': 14981, 'formalized': 14982, 'unhcr': 14983, 'jigme': 14984, 'wangchuck': 14985, 'abdicated': 14986, 'renegotiated': 14987, 'preferential': 14988, 'borrowed': 14989, 'richly': 14990, 'sour': 14991, 'skimming': 14992, 'vents': 14993, 'containment': 14994, 'minors': 14995, 'gallows': 14996, 'hoshide': 14997, 'plaintiffs': 14998, 'mayardit': 14999, 'purity': 15000, 'subscribers': 15001, 'disappearing': 15002, 'folly': 15003, '199': 15004, 'selections': 15005, 'bono': 15006, 'ha': 15007, 'vinh': 15008, 'nadu': 15009, 'dissipating': 15010, 'oz': 15011, 'mgm': 15012, 'dvds': 15013, 'patterson': 15014, 'firearm': 15015, 'conspirator': 15016, 'hammad': 15017, 'hilda': 15018, 'makeover': 15019, 'dhahran': 15020, 'frequented': 15021, 'gustavo': 15022, 'feeder': 15023, 'pitches': 15024, 'shurpayev': 15025, 'reestablish': 15026, 'arboleda': 15027, 'malfunctioned': 15028, 'strangled': 15029, 'untapped': 15030, 'observation': 15031, 'piled': 15032, 'protestants': 15033, 'vandalized': 15034, 'sergio': 15035, 'viera': 15036, 'mello': 15037, 'wirayuda': 15038, 'albar': 15039, 'diplomatically': 15040, 'disturbances': 15041, 'unaware': 15042, 'tolls': 15043, 'misunderstandings': 15044, 'supermarket': 15045, 'tabare': 15046, 'meddle': 15047, 'heydari': 15048, 'inevitable': 15049, 'shaping': 15050, 'balances': 15051, 'voicing': 15052, 'vested': 15053, 'factual': 15054, 'electronically': 15055, 'email': 15056, 'turkic': 15057, 'explores': 15058, 'chandrayaan': 15059, 'sensors': 15060, 'structuring': 15061, 'iscuande': 15062, 'ocampo': 15063, 'duped': 15064, 'reassure': 15065, 'sulaimaniya': 15066, 'foe': 15067, 'quadrupled': 15068, 'aliases': 15069, 'conditional': 15070, 'taifi': 15071, 'baotou': 15072, 'bakr': 15073, 'intercepting': 15074, 'wrongful': 15075, 'hurtado': 15076, 'roth': 15077, 'murderous': 15078, 'builders': 15079, '976': 15080, 'developers': 15081, 'malls': 15082, 'subsidiaries': 15083, 'liabilities': 15084, 'fijians': 15085, 'smarter': 15086, 'realize': 15087, 'mcc': 15088, 'violently': 15089, 'regulated': 15090, 'flourishes': 15091, 'gum': 15092, 'flesh': 15093, 'statues': 15094, 'gods': 15095, 'nearer': 15096, 'outrun': 15097, 'realities': 15098, 'sadly': 15099, 'ample': 15100, 'retrieve': 15101, 'spray': 15102, 'lifestyle': 15103, 'lobbed': 15104, 'causalities': 15105, 'militarily': 15106, 'mingled': 15107, 'fireball': 15108, 'wasteful': 15109, 'quo': 15110, 'namibians': 15111, 'grimes': 15112, 'angelo': 15113, 'sodano': 15114, 'vladivostok': 15115, 'navies': 15116, 'natal': 15117, 'hassani': 15118, 'stoned': 15119, 'belaroussi': 15120, 'wakil': 15121, 'muttawakil': 15122, 'stanislaw': 15123, 'izarra': 15124, '520': 15125, 'lapd': 15126, 'probed': 15127, 'eimiller': 15128, 'jayden': 15129, 'daron': 15130, 'strobl': 15131, 'stressing': 15132, 'daud': 15133, 'cooperative': 15134, 'strive': 15135, 'forgo': 15136, 'aero': 15137, 'limmt': 15138, 'miramax': 15139, 'shakespeare': 15140, 'bidders': 15141, 'weinstein': 15142, 'stanizai': 15143, 'ronnie': 15144, 'andry': 15145, 'najibullah': 15146, 'mwangura': 15147, 'seafarers': 15148, 'kismayo': 15149, 'inconceivable': 15150, 'fradkov': 15151, '760': 15152, 'proposing': 15153, 'byrd': 15154, 'sebastien': 15155, 'choong': 15156, 'hurriyah': 15157, 'marcus': 15158, 'malfunction': 15159, 'guajira': 15160, 'connects': 15161, 'inquest': 15162, 'routing': 15163, 'bernama': 15164, 'patricia': 15165, 'massing': 15166, 'atenco': 15167, 'tamin': 15168, 'nuristani': 15169, 'underscores': 15170, 'untouched': 15171, 'tarnished': 15172, 'searchers': 15173, 'parliaments': 15174, 'dabaa': 15175, 'barot': 15176, 'newark': 15177, 'julie': 15178, 'ulises': 15179, 'resigns': 15180, 'anxiety': 15181, 'semitism': 15182, 'interfaith': 15183, 'daylam': 15184, 'camilo': 15185, 'razali': 15186, 'asher': 15187, 'maximize': 15188, 'specialized': 15189, 'hampers': 15190, 'susceptibility': 15191, 'plank': 15192, 'brook': 15193, 'lest': 15194, 'dynamics': 15195, 'hrytsenko': 15196, 'catering': 15197, 'symbolically': 15198, 'ch': 15199, 'foment': 15200, 'revolutions': 15201, 'shielding': 15202, 'bekasi': 15203, 'okinawa': 15204, 'abhorrent': 15205, 'caring': 15206, 'gerais': 15207, 'casket': 15208, 'lori': 15209, 'tupac': 15210, 'amaru': 15211, 'keesler': 15212, 'patented': 15213, 'outrageous': 15214, 'framed': 15215, 'dishonest': 15216, 'odd': 15217, 'unlimited': 15218, 'nesterenko': 15219, 'proceeding': 15220, 'mourino': 15221, 'tellez': 15222, 'hizb': 15223, 'whitcomb': 15224, 'servicemembers': 15225, 'galleries': 15226, 'myuran': 15227, 'sukumaran': 15228, 'jalawla': 15229, 'embraced': 15230, 'antalya': 15231, 'emily': 15232, 'henochowicz': 15233, 'bartering': 15234, 'quezon': 15235, 'emptying': 15236, 'empowered': 15237, 'alluvial': 15238, 'hides': 15239, '1806': 15240, 'norm': 15241, 'exodus': 15242, 'socioeconomic': 15243, 'hardened': 15244, 'unifying': 15245, 'strangle': 15246, 'thereupon': 15247, 'bother': 15248, 'sukhoi': 15249, 'detailing': 15250, 'geoffrey': 15251, 'allotted': 15252, 'inked': 15253, 'pacifist': 15254, 'hangzhou': 15255, 'reinforcement': 15256, 'laborer': 15257, 'modify': 15258, 'somber': 15259, 'payload': 15260, 'fragmented': 15261, 'undemocratic': 15262, 'fatma': 15263, 'incentive': 15264, 'broadway': 15265, 'dolls': 15266, 'choreography': 15267, 'hafez': 15268, 'parisian': 15269, 'nanny': 15270, 'marjah': 15271, 'thefts': 15272, 'shed': 15273, 'swindler': 15274, 'mets': 15275, 'grandchild': 15276, 'medically': 15277, '481': 15278, 'guangxi': 15279, 'naeem': 15280, 'noor': 15281, 'brace': 15282, 'qassem': 15283, 'payloads': 15284, 'exomars': 15285, 'flowed': 15286, 'adopting': 15287, 'consultative': 15288, 'scrapping': 15289, 'globovision': 15290, 'pendleton': 15291, 'trumped': 15292, 'mhz': 15293, 'abid': 15294, 'suraiwi': 15295, 'chained': 15296, 'embankment': 15297, 'mentions': 15298, 'boots': 15299, 'accrue': 15300, '1886': 15301, '1899': 15302, 'boycotts': 15303, 'thinned': 15304, 'dukedom': 15305, '1603': 15306, '1854': 15307, 'modernize': 15308, 'sakhalin': 15309, 'gupta': 15310, 'longed': 15311, 'devouring': 15312, 'exertions': 15313, 'gentleman': 15314, 'excused': 15315, 'enlist': 15316, 'aswan': 15317, 'abul': 15318, 'appreciates': 15319, 'denunciations': 15320, 'rabin': 15321, 'theodore': 15322, 'neo': 15323, 'newlands': 15324, 'chanderpaul': 15325, '297': 15326, 'ntini': 15327, 'www': 15328, 'gov': 15329, 'livingstone': 15330, 'lusaka': 15331, 'effigies': 15332, 'humidity': 15333, 'transmitting': 15334, 'seif': 15335, 'faulting': 15336, 'energetic': 15337, 'thales': 15338, 'cotonou': 15339, 'novo': 15340, '7th': 15341, 'precautionary': 15342, 'tributes': 15343, 'eichmann': 15344, 'shiller': 15345, 'subprime': 15346, 'solving': 15347, 'grenoble': 15348, 'robber': 15349, 'swirling': 15350, 'kleinkirchheim': 15351, 'klammer': 15352, 'michaela': 15353, 'finishes': 15354, 'anja': 15355, 'khristenko': 15356, 'panetta': 15357, 'lembe': 15358, 'bukavu': 15359, 'kenyon': 15360, 'tornadoes': 15361, 'reburied': 15362, 'rodrigues': 15363, 'scares': 15364, 'malachite': 15365, 'determines': 15366, 'rhode': 15367, 'tai': 15368, 'qiang': 15369, 'katzenellenbogen': 15370, 'erect': 15371, 'surpass': 15372, 'keidel': 15373, 'carnegie': 15374, 'endowment': 15375, 'gauging': 15376, 'camila': 15377, 'guerra': 15378, 'incessant': 15379, 'gypsy': 15380, 'logs': 15381, 'ry': 15382, 'cooder': 15383, 'beloved': 15384, 'brick': 15385, 'destiny': 15386, 'disband': 15387, 'lanes': 15388, 'bbl': 15389, 'jonas': 15390, 'arrears': 15391, 'borneo': 15392, 'wanderings': 15393, 'glided': 15394, 'pricked': 15395, 'useless': 15396, 'min': 15397, 'ashr': 15398, 'hydara': 15399, 'martinez': 15400, 'balakot': 15401, 'frees': 15402, 'jade': 15403, 'ying': 15404, 'jeou': 15405, 'jeopardy': 15406, '012': 15407, 'maharastra': 15408, 'guirassy': 15409, 'karliova': 15410, 'tectonic': 15411, 'mercenaries': 15412, 'teodoro': 15413, 'baltica': 15414, 'pillars': 15415, 'peat': 15416, 'wolves': 15417, 'sates': 15418, 'hui': 15419, 'cohesion': 15420, 'salizhan': 15421, 'sharipov': 15422, 'leroy': 15423, 'chiao': 15424, 'branko': 15425, 'decay': 15426, 'psychic': 15427, 'kimchi': 15428, 'cabbage': 15429, 'dish': 15430, 'achin': 15431, 'sarath': 15432, 'portfolios': 15433, 'essay': 15434, 'rear': 15435, 'clocked': 15436, 'mclaren': 15437, 'mercedes': 15438, 'wurz': 15439, 'raikkonen': 15440, 'trails': 15441, 'falcon': 15442, 'drifted': 15443, 'frayed': 15444, 'shunned': 15445, 'trumpet': 15446, 'daya': 15447, 'nino': 15448, 'sanha': 15449, 'bandung': 15450, '698': 15451, 'salinas': 15452, 'edgar': 15453, 'norte': 15454, 'contrasted': 15455, 'campo': 15456, 'raiding': 15457, 'unicorp': 15458, 'cara': 15459, 'defection': 15460, 'profited': 15461, 'petronas': 15462, 'malays': 15463, '1925': 15464, '376': 15465, '552': 15466, 'guarantor': 15467, 'stamping': 15468, 'neigh': 15469, 'brilliant': 15470, 'indolent': 15471, 'hog': 15472, 'cocks': 15473, 'skulked': 15474, 'crowed': 15475, 'boasting': 15476, 'marjayoun': 15477, 'declarations': 15478, 'markus': 15479, 'insulted': 15480, 'morsink': 15481, 'michiko': 15482, 'saipan': 15483, 'fading': 15484, 'torrent': 15485, 'bassist': 15486, 'vista': 15487, 'acclaim': 15488, 'ruben': 15489, 'gonzalez': 15490, 'memin': 15491, 'pinguin': 15492, 'immensely': 15493, 'offended': 15494, 'demeanor': 15495, 'conservationists': 15496, 'guji': 15497, 'borena': 15498, 'shakiso': 15499, 'arero': 15500, 'yabello': 15501, 'virunga': 15502, 'segway': 15503, 'lowers': 15504, 'ndjabu': 15505, 'dwain': 15506, 'freelance': 15507, 'rodina': 15508, 'impaired': 15509, 'llodra': 15510, 'koukalova': 15511, 'countrywoman': 15512, 'johan': 15513, 'tarulovski': 15514, 'foodstuffs': 15515, 'malcolm': 15516, 'reception': 15517, 'deploys': 15518, 'conferring': 15519, 'striving': 15520, 'ashdod': 15521, 'gilchrist': 15522, 'olympio': 15523, 'andiwal': 15524, 'apologizes': 15525, 'levinson': 15526, 'locating': 15527, '243': 15528, 'centurion': 15529, 'anthrax': 15530, 'impacts': 15531, 'dynamite': 15532, 'sderot': 15533, 'southerners': 15534, 'cutoff': 15535, 'boiled': 15536, 'reassuring': 15537, 'talent': 15538, 'quakes': 15539, 'beiji': 15540, 'paulos': 15541, 'faraj': 15542, 'nineveh': 15543, 'blackmailed': 15544, 'baber': 15545, 'mcdaniel': 15546, 'ecosystems': 15547, 'yokota': 15548, 'alfonso': 15549, '340': 15550, 'logos': 15551, 'detonators': 15552, 'arsenic': 15553, 'diver': 15554, 'conn': 15555, 'adjustments': 15556, 'stimulated': 15557, 'ushering': 15558, 'indochina': 15559, 'hardships': 15560, 'semblance': 15561, 'norodom': 15562, 'aboriginal': 15563, '1770': 15564, 'capt': 15565, 'qom': 15566, 'ascension': 15567, 'perceiving': 15568, 'deprivation': 15569, 'thriller': 15570, 'grossing': 15571, 'ideological': 15572, 'gossip': 15573, 'hamilton': 15574, 'hayek': 15575, 'compatible': 15576, 'volt': 15577, '16s': 15578, 'manual': 15579, '351': 15580, 'manipur': 15581, 'defenseless': 15582, 'athlete': 15583, 'gayle': 15584, 'internationals': 15585, 'occured': 15586, 'penetrate': 15587, 'balkenende': 15588, 'dragons': 15589, 'torchbearer': 15590, 'constructively': 15591, 'jowhar': 15592, 'medusa': 15593, 'maksim': 15594, 'awakening': 15595, 'sexuality': 15596, 'examines': 15597, 'zheng': 15598, 'ads': 15599, 'denuclearization': 15600, 'donkey': 15601, 'unanswered': 15602, 'ulf': 15603, 'henricsson': 15604, 'claudio': 15605, 'tenet': 15606, 'unwarranted': 15607, 'deactivated': 15608, 'nitrate': 15609, 'nikiforov': 15610, 'staffing': 15611, 'sous': 15612, 'bois': 15613, 'radicalization': 15614, 'madhav': 15615, 'yadana': 15616, 'realtors': 15617, 'helgesen': 15618, 'mackay': 15619, 'contrasts': 15620, 'sukhumi': 15621, 'millenium': 15622, 'protectionist': 15623, 'trousers': 15624, 'knit': 15625, 'underwear': 15626, 'vedomosti': 15627, 'muifa': 15628, 'ngai': 15629, 'thua': 15630, 'thien': 15631, 'hue': 15632, 'bonfoh': 15633, 'offending': 15634, 'hadson': 15635, 'melnichenko': 15636, 'unwillingness': 15637, 'couch': 15638, 'enthusiasm': 15639, 'echo': 15640, 'tellers': 15641, 'whispers': 15642, 'replies': 15643, 'breeze': 15644, 'discriminatory': 15645, 'enshrines': 15646, 'guy': 15647, 'gazans': 15648, 'streamed': 15649, 'fostering': 15650, 'yousaf': 15651, 'sizably': 15652, 'alerts': 15653, 'lobbied': 15654, 'velach': 15655, "d'affaires": 15656, 'mtetwa': 15657, 'gulu': 15658, 'cooperated': 15659, 'ghalib': 15660, 'kubba': 15661, 'investigates': 15662, 'haiphong': 15663, 'benedetti': 15664, 'blige': 15665, 'pasadena': 15666, 'naacp': 15667, 'csw': 15668, 'chiang': 15669, 'whitney': 15670, 'surprisingly': 15671, 'biennial': 15672, 'jumbo': 15673, 'architecture': 15674, 'minarets': 15675, 'hatem': 15676, 'budgets': 15677, 'hovered': 15678, 'unjustly': 15679, 'traumatic': 15680, '655': 15681, 'understandable': 15682, 'reside': 15683, 'arghandab': 15684, 'feith': 15685, 'scheuer': 15686, 'inflamed': 15687, 'scouts': 15688, 'blacklist': 15689, 'sulphur': 15690, 'sheng': 15691, 'baqubah': 15692, 'gillard': 15693, 'shiloh': 15694, 'harbors': 15695, '1891': 15696, 'dpp': 15697, '1888': 15698, '1900': 15699, 'strolling': 15700, 'lofty': 15701, 'quoth': 15702, 'morsel': 15703, 'seldom': 15704, 'drawer': 15705, "'d": 15706, 'recruiter': 15707, 'mules': 15708, 'hitched': 15709, 'trawlers': 15710, 'elvis': 15711, 'dancer': 15712, 'magerovskiy': 15713, "ba'ath": 15714, 'transmitter': 15715, 'rages': 15716, 'valdes': 15717, 'nico': 15718, 'beatle': 15719, 'donatella': 15720, 'versace': 15721, 'syndicated': 15722, 'insider': 15723, 'gaunt': 15724, 'mishandled': 15725, 'batumi': 15726, 'louise': 15727, 'araque': 15728, 'kiryenko': 15729, 'shurja': 15730, 'kolkata': 15731, 'wasim': 15732, 'keeper': 15733, 'mahendra': 15734, 'looted': 15735, 'yorkers': 15736, 'nathalie': 15737, 'dechy': 15738, 'marrero': 15739, 'bartoli': 15740, 'pascual': 15741, '537': 15742, 'cheating': 15743, 'ginepri': 15744, 'muller': 15745, 'paradorn': 15746, 'h7': 15747, 'congratulatory': 15748, 'quagga': 15749, 'hunted': 15750, 'endeavor': 15751, 'impeach': 15752, 'yearlong': 15753, 'zabol': 15754, 'oils': 15755, 'supper': 15756, 'apostles': 15757, 'resurrection': 15758, 'booth': 15759, 'almaty': 15760, 'myna': 15761, 'antoine': 15762, 'flavia': 15763, 'patty': 15764, 'schnyder': 15765, 'arraigned': 15766, 'gary': 15767, 'waivers': 15768, 'distributes': 15769, 'principalities': 15770, 'gorbachev': 15771, 'inadvertently': 15772, 'splintered': 15773, 'antigua': 15774, 'bedding': 15775, 'hemispheric': 15776, 'whelp': 15777, 'collectors': 15778, 'proxy': 15779, 'hudur': 15780, 'masorin': 15781, 'registers': 15782, 'moin': 15783, 'qalibaf': 15784, 'bartholomew': 15785, 'insurmountable': 15786, 'crusaders': 15787, 'najam': 15788, 'briston': 15789, 'gilead': 15790, 'collaborate': 15791, 'dose': 15792, 'harvard': 15793, 'yaqoob': 15794, 'ne': 15795, 'artifacts': 15796, 'servant': 15797, 'coffins': 15798, 'jurist': 15799, 'asfandyar': 15800, 'idle': 15801, 'nationalizing': 15802, 'maternity': 15803, 'garnering': 15804, 'appointees': 15805, 'caldwell': 15806, 'electorate': 15807, 'muscles': 15808, 'delight': 15809, 'helmets': 15810, 'chelyabinsk': 15811, 'rounding': 15812, 'doe': 15813, 'misfortune': 15814, 'invasive': 15815, 'uterine': 15816, 'fibroid': 15817, 'dave': 15818, 'heineman': 15819, 'saifullah': 15820, 'belongings': 15821, 'uproot': 15822, 'probation': 15823, 'unify': 15824, '2a': 15825, 'profane': 15826, 'cetin': 15827, 'rotation': 15828, 'pen': 15829, 'inspecting': 15830, 'decorated': 15831, '1912': 15832, 'partisans': 15833, 'deficiencies': 15834, '1856': 15835, '1878': 15836, 'ceausescu': 15837, 'draconian': 15838, 'prgf': 15839, 'nomadic': 15840, '1916': 15841, 'spraying': 15842, 'mosquitoes': 15843, 'ziemer': 15844, '1938': 15845, 'yonts': 15846, 'ambulance': 15847, 'kusadasi': 15848, 'tak': 15849, 'qassam': 15850, 'ravaging': 15851, 'spawned': 15852, 'influencing': 15853, 'rezayee': 15854, 'booking': 15855, 'merrill': 15856, 'lynch': 15857, 'brokerage': 15858, 'memories': 15859, 'hartmann': 15860, 'hamdan': 15861, 'ui': 15862, 'turboprop': 15863, 'chant': 15864, 'philipp': 15865, 'coerced': 15866, 'totals': 15867, 'razed': 15868, 'bi': 15869, 'safehouse': 15870, 'kambakhsh': 15871, 'dissolving': 15872, 'shoreline': 15873, 'spanned': 15874, 'tito': 15875, 'beached': 15876, 'martti': 15877, 'trillions': 15878, 'bets': 15879, 'bathrooms': 15880, 'alerting': 15881, 'greens': 15882, 'nigerians': 15883, 'momcilo': 15884, 'cinema': 15885, 'aug': 15886, 'downgrading': 15887, 'peers': 15888, 'tipped': 15889, 'revamp': 15890, 'uneasy': 15891, 'stimulating': 15892, 'consequently': 15893, 'incorporate': 15894, 'awaits': 15895, 'polynesia': 15896, 'distinguished': 15897, 'deficient': 15898, 'shipwrecked': 15899, 'awoke': 15900, 'plow': 15901, 'cd': 15902, 'scrambled': 15903, 'superior': 15904, 'reconvene': 15905, 'fadillah': 15906, 'probability': 15907, 'akmatbayev': 15908, 'substation': 15909, 'chubais': 15910, 'wesley': 15911, 'legg': 15912, 'mason': 15913, '86th': 15914, 'querrey': 15915, '43rd': 15916, 'nightfall': 15917, 'bat': 15918, 'org': 15919, 'muqdadiyah': 15920, 'converge': 15921, 'kazakh': 15922, 'assassins': 15923, 'ancestral': 15924, 'insolent': 15925, 'dervis': 15926, 'eroglu': 15927, 'hinge': 15928, 'tillman': 15929, 'culprit': 15930, 'sampled': 15931, 'demolitions': 15932, 'chepas': 15933, 'mallahi': 15934, 'tawhid': 15935, '255': 15936, 'tendulkar': 15937, 'dinesh': 15938, 'doda': 15939, 'schlegel': 15940, 'krajina': 15941, 'smear': 15942, 'raging': 15943, 'mourns': 15944, 'shia': 15945, 'disturbia': 15946, 'penguin': 15947, 'surf': 15948, 'plassnik': 15949, 'pluralistic': 15950, 'heartland': 15951, 'sud': 15952, 'orellana': 15953, 'condoms': 15954, 'firestorm': 15955, 'homily': 15956, 'dangerously': 15957, 'doboj': 15958, 'streptococcus': 15959, 'suis': 15960, '174': 15961, 'millennia': 15962, 'cheonan': 15963, 'coat': 15964, 'leninist': 15965, 'jagan': 15966, 'unequivocally': 15967, 'chiluba': 15968, 'usd': 15969, 'placard': 15970, 'shopkeeper': 15971, 'salesman': 15972, 'pozarevac': 15973, 'milorad': 15974, 'mash': 15975, 'vandalizing': 15976, 'shebaa': 15977, '480': 15978, 'grossed': 15979, 'themes': 15980, 'agim': 15981, 'lutfi': 15982, 'angering': 15983, 'bargal': 15984, 'dams': 15985, 'encryption': 15986, '543': 15987, 'bharatiya': 15988, 'thanking': 15989, 'bolani': 15990, 'waili': 15991, 'aigle': 15992, 'azur': 15993, 'stifling': 15994, 'robben': 15995, 'writings': 15996, 'centrifuge': 15997, 'evangelist': 15998, 'wholly': 15999, 'assertions': 16000, 'godfather': 16001, 'sensible': 16002, 'statutes': 16003, 'interstate': 16004, 'fingerprint': 16005, 'fingerprinting': 16006, 'trunk': 16007, 'anne': 16008, 'sufficiently': 16009, 'susy': 16010, 'tekunan': 16011, 'quest': 16012, 'recycled': 16013, 'baking': 16014, 'unfriendly': 16015, 'deserters': 16016, 'thriving': 16017, 'hifikepunye': 16018, 'windhoek': 16019, 'swapo': 16020, 'canals': 16021, 'irrawaddy': 16022, 'exorbitant': 16023, 'kph': 16024, 'peoria': 16025, 'gayoom': 16026, 'nasheed': 16027, 'elevation': 16028, 'tsz': 16029, 'remotely': 16030, 'demarcated': 16031, 'coordinates': 16032, 'tracts': 16033, 'frg': 16034, 'gdr': 16035, 'intervening': 16036, 'vultures': 16037, 'crows': 16038, 'gored': 16039, 'vanallen': 16040, 'wead': 16041, 'triangular': 16042, '3rd': 16043, 'velupillai': 16044, 'lakhdaria': 16045, 'fomenting': 16046, 'kerch': 16047, 'nashville': 16048, 'yekiti': 16049, 'maashouq': 16050, 'kameshli': 16051, 'encountering': 16052, 'converts': 16053, 'bidder': 16054, 'defining': 16055, 'fade': 16056, 'comfort': 16057, 'goers': 16058, 'lags': 16059, '646': 16060, 'calin': 16061, 'tariceanu': 16062, 'siirt': 16063, 'scanners': 16064, 'massed': 16065, 'trainees': 16066, 'cylinders': 16067, 'everybody': 16068, 'clots': 16069, 'secretariat': 16070, 'francesca': 16071, 'chess': 16072, 'liberals': 16073, 'emomali': 16074, 'rakhmon': 16075, 'finisher': 16076, 'mexicans': 16077, 'reorganization': 16078, 'unsecured': 16079, 'reorganized': 16080, 'effected': 16081, 'dioceses': 16082, '1825': 16083, 'consisted': 16084, 'amerindian': 16085, 'bubble': 16086, 'sicily': 16087, 'eec': 16088, 'conserving': 16089, 'choked': 16090, 'resigning': 16091, 'instituting': 16092, 'kheir': 16093, 'negate': 16094, 'firings': 16095, 'karasin': 16096, 'toussaint': 16097, 'sfeir': 16098, 'unfiltered': 16099, 'click': 16100, 'ignited': 16101, 'bilis': 16102, 'amsterdam': 16103, 'hyundai': 16104, 'kifaya': 16105, 'forgery': 16106, 'misquoting': 16107, 'postsays': 16108, 'merging': 16109, 'nizar': 16110, 'trabelsi': 16111, 'vasil': 16112, 'instructors': 16113, 'ivashov': 16114, 'duc': 16115, 'luong': 16116, 'chipmaker': 16117, 'golborne': 16118, 'shafts': 16119, 'precedes': 16120, 'purple': 16121, 'beads': 16122, 'batlle': 16123, 'drained': 16124, 'sodium': 16125, 'escorts': 16126, 'simmering': 16127, 'blueprints': 16128, 'risked': 16129, 'kandani': 16130, 'miyazaki': 16131, 'pajhwok': 16132, 'blechschmidt': 16133, 'consolidating': 16134, 'yulija': 16135, 'kosachev': 16136, 'awake': 16137, 'malignant': 16138, 'jon': 16139, 'litigation': 16140, 'eparses': 16141, 'integral': 16142, 'kerguelen': 16143, 'ile': 16144, 'satisfying': 16145, 'kenyatta': 16146, 'fractured': 16147, 'dislodge': 16148, 'narc': 16149, 'lighthouse': 16150, 'qar': 16151, 'solvent': 16152, 'vakhitov': 16153, 'korans': 16154, 'drifting': 16155, 'sara': 16156, 'mathew': 16157, 'surveying': 16158, 'chelsea': 16159, 'cows': 16160, 'lawbreakers': 16161, 'hotmail': 16162, 'bio': 16163, '336': 16164, 'hon': 16165, 'okah': 16166, 'bisphenol': 16167, 'liver': 16168, 'rachid': 16169, 'oxygen': 16170, '36th': 16171, 'michaella': 16172, 'wessels': 16173, 'kiefer': 16174, 'dent': 16175, 'preservation': 16176, 'grouped': 16177, 'unnerved': 16178, 'westinghouse': 16179, 'préval': 16180, 'mobutu': 16181, 'legitimizing': 16182, 'asadullah': 16183, 'shiekh': 16184, 'alcantara': 16185, 'technicians': 16186, 'pervasive': 16187, 'depict': 16188, 'iskander': 16189, 'urumqi': 16190, 'sleek': 16191, 'magnetic': 16192, 'rental': 16193, 'borrower': 16194, '295': 16195, 'paige': 16196, 'kollock': 16197, 'chester': 16198, 'gunsmoke': 16199, 'qualifications': 16200, 'emmy': 16201, 'taher': 16202, 'busta': 16203, 'uncooperative': 16204, 'recreational': 16205, 'amateur': 16206, 'inaccurate': 16207, 'staunchly': 16208, 'freight': 16209, 'overfishing': 16210, 'mutineers': 16211, 'tahitian': 16212, 'vestige': 16213, 'qarase': 16214, 'bainimarama': 16215, 'cpa': 16216, 'hounds': 16217, 'pin': 16218, 'kung': 16219, '1911': 16220, 'hani': 16221, 'calabar': 16222, 'kirilenko': 16223, 'seemingly': 16224, 'beachside': 16225, 'exciting': 16226, 'surfers': 16227, 'elham': 16228, 'fundamentally': 16229, 'sepp': 16230, 'blatter': 16231, 'trim': 16232, 'kissing': 16233, 'looped': 16234, 'troedsson': 16235, 'tends': 16236, 'alloceans': 16237, 'smigun': 16238, 'snowboard': 16239, 'sheibani': 16240, 'saderat': 16241, 'jabalya': 16242, 'arthritis': 16243, 'akash': 16244, 'chandipur': 16245, 'bhubaneshwar': 16246, 'drinan': 16247, 'congestive': 16248, 'unsure': 16249, 'shaking': 16250, 'stratfor': 16251, 'turkestan': 16252, 'adumin': 16253, 'westernmost': 16254, 'rocking': 16255, 'githongo': 16256, 'asghar': 16257, 'everywhere': 16258, 'abdulmutallab': 16259, 'cloning': 16260, 'suk': 16261, 'deceiving': 16262, 'scuffles': 16263, 'wengen': 16264, '706': 16265, 'practically': 16266, 'plates': 16267, 'ganei': 16268, 'oic': 16269, 'caved': 16270, 'drastic': 16271, 'tyre': 16272, 'lanterns': 16273, 'souls': 16274, 'horrific': 16275, 'janakpur': 16276, 'rajapaksa': 16277, 'visual': 16278, 'apiece': 16279, 'shedding': 16280, 'reaped': 16281, 'fiscally': 16282, 'attaining': 16283, '1857': 16284, 'biodiversity': 16285, '1903': 16286, 'alfredo': 16287, 'celtic': 16288, 'rebellions': 16289, 'ulster': 16290, 'maiberger': 16291, 'smelter': 16292, 'politicizing': 16293, 'groundwork': 16294, 'feminine': 16295, 'mystique': 16296, 'groundbreaking': 16297, 'husbands': 16298, 'goushmane': 16299, 'mindoro': 16300, 'albay': 16301, 'mayon': 16302, 'loaning': 16303, 'paves': 16304, 'blockades': 16305, 'eugene': 16306, 'prescribed': 16307, 'hyde': 16308, 'irrigation': 16309, 'altitudes': 16310, 'rainstorms': 16311, 'napa': 16312, 'swelling': 16313, 'indict': 16314, 'tu': 16315, 'elusive': 16316, 'prithvi': 16317, 'persecuted': 16318, 'dyes': 16319, 'conceal': 16320, 'kuril': 16321, '561': 16322, 'taxpayers': 16323, 'aia': 16324, 'hakkari': 16325, 'wilmington': 16326, 'graduation': 16327, 'benita': 16328, 'breaststroke': 16329, 'meningitis': 16330, 'midler': 16331, 'celine': 16332, 'dion': 16333, 'headliner': 16334, 'inaugurating': 16335, 'colosseum': 16336, 'vaile': 16337, 'vuvuzelas': 16338, 'robots': 16339, 'lighter': 16340, 'chikunova': 16341, 'danforth': 16342, 'chesnot': 16343, 'malbrunot': 16344, 'wazirstan': 16345, 'accessing': 16346, 'rak': 16347, 'uninterrupted': 16348, 'unload': 16349, 'earners': 16350, 'genius': 16351, 'correctness': 16352, 'saiki': 16353, 'bwakira': 16354, 'sherzai': 16355, 'weed': 16356, 'narcotic': 16357, 'bribed': 16358, 'beds': 16359, 'fanning': 16360, '284': 16361, 'kapilvastu': 16362, 'directing': 16363, 'prostitution': 16364, 'haile': 16365, 'politicize': 16366, 'mutilating': 16367, 'arak': 16368, 'ensures': 16369, 'torah': 16370, 'overcrowding': 16371, 'tine': 16372, 'deshu': 16373, 'kakar': 16374, 'mujaddedi': 16375, 'cpp': 16376, 'ilbo': 16377, 'erroneous': 16378, 'forwarded': 16379, 'rosh': 16380, 'hashanah': 16381, 'strangling': 16382, 'icelandic': 16383, 'stanford': 16384, 'chesney': 16385, 'chill': 16386, 'clarence': 16387, 'gatemouth': 16388, 'shmatko': 16389, 'malda': 16390, 'tyranny': 16391, 'levan': 16392, 'gachechiladze': 16393, 'flowstations': 16394, 'chevrontexaco': 16395, 'eindhoven': 16396, 'fargo': 16397, 'squatters': 16398, 'plainclothes': 16399, 'dushanbe': 16400, 'seafood': 16401, 'cake': 16402, 'rechargeable': 16403, 'volume': 16404, 'exterminated': 16405, '1834': 16406, 'geographically': 16407, 'ethanol': 16408, 'expiration': 16409, 'statist': 16410, 'workshops': 16411, '41st': 16412, 'geography': 16413, 'viceroyalty': 16414, '1822': 16415, 'offspring': 16416, 'handsomest': 16417, 'tenderness': 16418, 'allot': 16419, 'scattering': 16420, 'demobilize': 16421, 'aiff': 16422, 'morale': 16423, 'z': 16424, 'refine': 16425, 'reparation': 16426, 'militarism': 16427, 'stumbling': 16428, 'divers': 16429, 'bancoro': 16430, 'liquidity': 16431, '538': 16432, 'fadilah': 16433, 'payne': 16434, 'receptionist': 16435, 'occupiers': 16436, 'confrontational': 16437, 'naisse': 16438, '311': 16439, 'tap': 16440, 'lashing': 16441, 'massacres': 16442, 'zahedan': 16443, 'novelist': 16444, 'booker': 16445, 'orphaned': 16446, 'granddaughter': 16447, 'vidoje': 16448, 'jundollah': 16449, 'adapt': 16450, 'ogun': 16451, 'torun': 16452, 'revisit': 16453, 'barley': 16454, 'cauvin': 16455, 'ojea': 16456, 'quintana': 16457, 'hogan': 16458, 'clearwater': 16459, 'shelor': 16460, 'graziano': 16461, 'joey': 16462, 'emphasizes': 16463, 'samoa': 16464, 'nkurunziza': 16465, 'overland': 16466, 'disgrace': 16467, 'gwan': 16468, 'commandoes': 16469, 'mauled': 16470, 'beings': 16471, 'fini': 16472, 'koichi': 16473, 'wakata': 16474, 'patterns': 16475, 'crossroads': 16476, 'philosophical': 16477, 'claire': 16478, '247': 16479, 'fulfills': 16480, 'straits': 16481, 'hafeez': 16482, 'heir': 16483, 'ascend': 16484, 'chrysanthemum': 16485, 'rosa': 16486, 'floats': 16487, 'climatic': 16488, 'slutskaya': 16489, 'skaters': 16490, 'entrants': 16491, 'elena': 16492, 'technocrats': 16493, 'creativity': 16494, 'distinguish': 16495, 'ronaldo': 16496, 'roseneft': 16497, 'fiercest': 16498, 'calcutta': 16499, 'ghettos': 16500, 'diddy': 16501, 'mogul': 16502, 'salvage': 16503, 'bosco': 16504, 'katutsi': 16505, 'kulyab': 16506, 'ordinance': 16507, '348': 16508, 'subsidizes': 16509, 'peanuts': 16510, 'boni': 16511, 'dampened': 16512, 'squid': 16513, 'wool': 16514, 'dane': 16515, 'eco': 16516, 'jackdaw': 16517, 'envy': 16518, 'whir': 16519, 'daw': 16520, 'assented': 16521, 'sowing': 16522, 'hopping': 16523, 'despised': 16524, 'pausing': 16525, 'nixon': 16526, 'panhandle': 16527, 'hallandale': 16528, 'boating': 16529, 'inscribed': 16530, 'timoshenko': 16531, 'tut': 16532, 'taif': 16533, 'stephanie': 16534, 'breaching': 16535, 'argentines': 16536, 'harrison': 16537, 'bahlul': 16538, 'diminish': 16539, 'backpacks': 16540, 'luton': 16541, 'ramazan': 16542, 'sami': 16543, 'understood': 16544, 'novin': 16545, 'mesbah': 16546, 'douglas': 16547, 'badakshan': 16548, '186': 16549, 'kharj': 16550, 'sinmun': 16551, 'somaliland': 16552, 'renovations': 16553, 'reauthorize': 16554, 'redeployment': 16555, 'hezb': 16556, 'olympia': 16557, 'patan': 16558, 'stanishev': 16559, 'odom': 16560, '181': 16561, 'diverting': 16562, 'embolden': 16563, 'fushun': 16564, 'rob': 16565, 'flagship': 16566, 'frazier': 16567, 'heist': 16568, 'discretion': 16569, 'malegaon': 16570, 'minivan': 16571, 'trailer': 16572, 'undocumented': 16573, 'rizeigat': 16574, 'aliu': 16575, 'hinges': 16576, 'sariyev': 16577, 'soro': 16578, 'annapolis': 16579, 'languishing': 16580, 'bulky': 16581, 'smiling': 16582, 'creator': 16583, 'wag': 16584, 'shahidi': 16585, 'annulment': 16586, 'dormant': 16587, 'uninsured': 16588, 'bales': 16589, 'pastures': 16590, 'roxana': 16591, 'detached': 16592, 'chairwoman': 16593, 'casings': 16594, 'apache': 16595, 'yankee': 16596, 'frontline': 16597, 'pbs': 16598, 'arc': 16599, 'grace': 16600, 'nuri': 16601, 'potent': 16602, 'inquiries': 16603, 'interpretations': 16604, 'surroundings': 16605, 'lunchtime': 16606, 'excrement': 16607, 'preachers': 16608, 'motivation': 16609, 'revelry': 16610, 'rancher': 16611, 'apondi': 16612, 'maastricht': 16613, '1697': 16614, '1804': 16615, 'postponements': 16616, 'grievously': 16617, 'devoured': 16618, 'surfeited': 16619, 'nursing': 16620, 'chains': 16621, 'kezerashvili': 16622, 'sahibzada': 16623, 'anis': 16624, 'kanow': 16625, 'clinched': 16626, 'salami': 16627, 'tor': 16628, 'skied': 16629, 'ivica': 16630, 'darkazanli': 16631, 'entrepreneur': 16632, 'unfolding': 16633, 'revoking': 16634, 'undermines': 16635, 'ibaraki': 16636, 'sabawi': 16637, 'jvp': 16638, 'bleak': 16639, 'unhelpful': 16640, 'swastika': 16641, 'tamimi': 16642, 'rowsch': 16643, 'inflationary': 16644, 'ustarkhanova': 16645, 'inflame': 16646, 'unprofessional': 16647, 'envisions': 16648, 'penetrating': 16649, 'tauran': 16650, 'pluralism': 16651, 'nita': 16652, 'extinct': 16653, 'loosened': 16654, 'ratsiraka': 16655, 'lessened': 16656, 'melody': 16657, 'stool': 16658, 'newell': 16659, 'letten': 16660, 'kenjic': 16661, 'petitioning': 16662, 'pitted': 16663, 'amezcua': 16664, 'minds': 16665, 'sardar': 16666, 'barometer': 16667, 'georgians': 16668, 'gregorio': 16669, 'alec': 16670, 'chita': 16671, '\x93': 16672, 'pakhtunkhwa': 16673, 'handcuffed': 16674, 'bands': 16675, 'discharge': 16676, 'bartlett': 16677, 'spiked': 16678, 'haroon': 16679, 'paltrow': 16680, 'folha': 16681, 'fraenzi': 16682, 'infromation': 16683, 'nutritional': 16684, 'blanket': 16685, 'sprawling': 16686, 'expatriates': 16687, 'tributary': 16688, 'yangtze': 16689, 'vest': 16690, 'ncri': 16691, '630': 16692, '55th': 16693, 'baloyi': 16694, 'hop': 16695, 'yearend': 16696, 'intifada': 16697, 'oak': 16698, 'hearty': 16699, 'organ': 16700, 'scorn': 16701, 'tired': 16702, 'partitioning': 16703, 'machetes': 16704, 'haram': 16705, 'ghani': 16706, 'phases': 16707, 'undetected': 16708, 'debriefed': 16709, 'zenani': 16710, 'computing': 16711, 'browsing': 16712, 'conferencing': 16713, 'gadget': 16714, 'conson': 16715, 'floodwalls': 16716, 'berkeley': 16717, 'minke': 16718, 'anesthetic': 16719, 'bharti': 16720, 'shopkeepers': 16721, 'unaffected': 16722, 'ethem': 16723, 'erdagi': 16724, 'horses': 16725, 'amazed': 16726, 'deception': 16727, 'sangakkara': 16728, 'divisive': 16729, 'pregnancies': 16730, 'roe': 16731, 'legalizing': 16732, 'sha': 16733, 'hailu': 16734, 'ammar': 16735, 'royalty': 16736, 'irreparable': 16737, 'muntadar': 16738, 'nhk': 16739, 'tsa': 16740, 'grassroots': 16741, 'overshadows': 16742, 'enroll': 16743, 'subjecting': 16744, 'commons': 16745, 'births': 16746, 'muntazer': 16747, 'invalid': 16748, '1919': 16749, 'polarized': 16750, 'soaked': 16751, 'faint': 16752, 'scampered': 16753, 'woe': 16754, 'overtime': 16755, 'quran': 16756, 'terry': 16757, 'rath': 16758, 'defamatory': 16759, 'nenbutsushu': 16760, 'mobs': 16761, 'waxman': 16762, 'suharto': 16763, 'viewing': 16764, 'unopposed': 16765, 'aes': 16766, 'breakdowns': 16767, 'emperors': 16768, 'heirs': 16769, 'trusted': 16770, 'sinopec': 16771, 'vitoria': 16772, 'datafolha': 16773, 'zoran': 16774, 'milenkovic': 16775, 'covic': 16776, 'baluyevsky': 16777, 'clyburn': 16778, 'dusan': 16779, 'tesic': 16780, 'hargreaves': 16781, 'ranges': 16782, 'edouard': 16783, 'cautions': 16784, 'mountainside': 16785, 'stupid': 16786, 'marriages': 16787, 'gujarat': 16788, 'diseased': 16789, 'tucson': 16790, 'nabbed': 16791, 'garner': 16792, 'obey': 16793, 'denials': 16794, 'adherence': 16795, 'cfa': 16796, 'jeopardized': 16797, 'breadfruit': 16798, 'spoil': 16799, 'modestly': 16800, 'heap': 16801, 'misfortunes': 16802, 'yours': 16803, 'bell': 16804, 'protocols': 16805, 'giddens': 16806, 'barman': 16807, 'naqba': 16808, 'counterinsurgency': 16809, 'ravix': 16810, 'makhachkala': 16811, 'returnees': 16812, 'incapacitated': 16813, 'moviemaking': 16814, 'reinforces': 16815, 'tarnishing': 16816, 'wasting': 16817, 'heater': 16818, 'arg': 16819, 'kakdwip': 16820, 'methodology': 16821, 'attache': 16822, 'exploding': 16823, 'escapees': 16824, 'resorting': 16825, 'kohlu': 16826, 'passover': 16827, 'motivating': 16828, 'deir': 16829, 'caterpillar': 16830, 'vacationers': 16831, 'pago': 16832, 'gardener': 16833, 'watered': 16834, 'fork': 16835, 'swamp': 16836, 'alarming': 16837, 'chemist': 16838, 'coupled': 16839, 'bertone': 16840, 'mudasir': 16841, 'notoriously': 16842, 'sarin': 16843, 'informant': 16844, '26th': 16845, 'invaders': 16846, 'frisked': 16847, 'communicate': 16848, 'conscience': 16849, 'curbs': 16850, 'literacy': 16851, 'refueling': 16852, 'stakic': 16853, 'warranted': 16854, 'kharazi': 16855, 'civility': 16856, 'reconstructive': 16857, 'ipsos': 16858, 'midterm': 16859, 'answers': 16860, 'activated': 16861, 'wwf': 16862, 'eighties': 16863, 'sever': 16864, 'deserter': 16865, 'townspeople': 16866, 'sibneft': 16867, 'nomads': 16868, 'comatose': 16869, 'unconscious': 16870, 'initiating': 16871, 'maybe': 16872, 'archery': 16873, 'dreams': 16874, 'enclosed': 16875, 'bray': 16876, 'linguistic': 16877, '1839': 16878, 'knife': 16879, 'bolshevik': 16880, 'ferdinand': 16881, 'edsa': 16882, 'corazon': 16883, 'kowal': 16884, 'franken': 16885, 'layoffs': 16886, 'et': 16887, "terre'blanche": 16888, 'stemmed': 16889, 'mailed': 16890, 'communal': 16891, 'poroshenko': 16892, 'relaxing': 16893, 'kilimo': 16894, 'habib': 16895, 'zctu': 16896, 'czar': 16897, 'okala': 16898, 'brownback': 16899, 'massouda': 16900, 'enthusiastic': 16901, 'rod': 16902, 'yards': 16903, 'kaman': 16904, 'hasten': 16905, 'relaunch': 16906, 'yury': 16907, 'gambira': 16908, 'mandalay': 16909, 'orchestrating': 16910, 'bandundu': 16911, 'navigate': 16912, 'nickelodeon': 16913, 'signifies': 16914, 'trivial': 16915, 'meltdown': 16916, 'theodor': 16917, 'zu': 16918, 'ou': 16919, 'sabouri': 16920, 'disposition': 16921, 'seamen': 16922, 'ttf': 16923, 'nz': 16924, 'vulnerability': 16925, 'remoteness': 16926, 'lobster': 16927, 'reuniting': 16928, 'flute': 16929, 'signboard': 16930, 'unwittingly': 16931, 'dashed': 16932, 'terribly': 16933, 'reindeer': 16934, 'deserved': 16935, 'rudolph': 16936, 'cylinder': 16937, 'jumpers': 16938, 'beltrame': 16939, 'chiapolino': 16940, 'fractures': 16941, 'concussion': 16942, 'montas': 16943, 'skewed': 16944, 'arawak': 16945, 'slows': 16946, 'siphiwe': 16947, 'safa': 16948, 'sedibe': 16949, 'memorable': 16950, 'spanning': 16951, 'zaken': 16952, 'cascade': 16953, 'faisalabad': 16954, 'sidique': 16955, 'sidon': 16956, 'shehade': 16957, 'housekeeper': 16958, 'patriots': 16959, 'brammertz': 16960, 'utmost': 16961, 'rightful': 16962, 'geagea': 16963, 'liquids': 16964, 'nazif': 16965, 'saxby': 16966, 'waking': 16967, 'comfortably': 16968, 'abercrombie': 16969, 'silenced': 16970, 'sprout': 16971, 'ivanic': 16972, 'pluck': 16973, '321': 16974, 'nagano': 16975, 'abdulaziz': 16976, 'intending': 16977, 'colts': 16978, 'phillip': 16979, 'hotline': 16980, 'zinjibar': 16981, 'noaman': 16982, 'gomaa': 16983, 'swear': 16984, 'aqaba': 16985, 'aspiazu': 16986, 'rubina': 16987, 'dimensional': 16988, 'modell': 16989, 'nausea': 16990, 'feudal': 16991, 'ohrid': 16992, '1609': 16993, '1820s': 16994, 'raft': 16995, 'unknowingly': 16996, 'splm': 16997, 'django': 16998, 'reinhardt': 16999, 'bossa': 17000, 'aneurysm': 17001, 'cerberus': 17002, 'straightened': 17003, 'buhriz': 17004, 'gasses': 17005, 'unscheduled': 17006, 'babil': 17007, 'qurans': 17008, 'mcadams': 17009, 'brokering': 17010, 'tamir': 17011, 'soleil': 17012, 'persson': 17013, 'laila': 17014, 'freivalds': 17015, 'sharpest': 17016, 'cerveny': 17017, 'dtp': 17018, 'salmonella': 17019, 'rash': 17020, 'jamaat': 17021, 'summoning': 17022, 'des': 17023, 'danilovich': 17024, 'idled': 17025, 'mpla': 17026, 'dos': 17027, 'unita': 17028, 'helena': 17029, 'wig': 17030, 'bald': 17031, 'abstained': 17032, 'yogi': 17033, 'bathtub': 17034, 'invented': 17035, '1850': 17036, '1875': 17037, 'spammers': 17038, 'upgrading': 17039, 'mohammadi': 17040, 'jeffery': 17041, 'orion': 17042, 'dances': 17043, 'surfaces': 17044, 'turf': 17045, 'successors': 17046, 'ligament': 17047, 'magazzeni': 17048, 'sermons': 17049, 'christa': 17050, 'mcauliffe': 17051, 'liftoff': 17052, 'cycling': 17053, 'scilingo': 17054, 'recanted': 17055, 'cult': 17056, 'embracing': 17057, 'patzi': 17058, 'interahamwe': 17059, 'zhou': 17060, 'rings': 17061, 'hilton': 17062, '580': 17063, 'petrova': 17064, 'postcard': 17065, 'qaumi': 17066, 'crackdowns': 17067, 'baptiste': 17068, 'rumbo': 17069, 'propio': 17070, 'courier': 17071, 'samburu': 17072, 'boasts': 17073, '1870s': 17074, 'mahdist': 17075, '1236': 17076, 'dare': 17077, 'unregistered': 17078, 'shaath': 17079, 'staggered': 17080, 'abstentions': 17081, 'spite': 17082, 'sk': 17083, 'adulthood': 17084, 'camouflage': 17085, 'lowey': 17086, 'yunesi': 17087, 'jamaicans': 17088, '100th': 17089, 'bermet': 17090, 'steam': 17091, 'trickle': 17092, 'coexist': 17093, 'kamel': 17094, 'gollnisch': 17095, 'disputing': 17096, 'nullify': 17097, 'discotheques': 17098, 'trademark': 17099, 'bernd': 17100, 'nogaideli': 17101, 'murphy': 17102, 'anglican': 17103, 'rowan': 17104, 'mostar': 17105, 'krzyzewski': 17106, 'grocery': 17107, 'coalitions': 17108, '265': 17109, 'tremor': 17110, 'halter': 17111, 'courted': 17112, '439': 17113, 'cimpl': 17114, 'getty': 17115, 'bc': 17116, 'fayfi': 17117, 'veiled': 17118, 'destroys': 17119, 'bjp': 17120, 'granite': 17121, 'prediction': 17122, 'ovation': 17123, 'stumbled': 17124, 'likened': 17125, 'warriors': 17126, 'decisively': 17127, 'indiscriminate': 17128, 'ecotourism': 17129, 'loads': 17130, 'galloped': 17131, 'kohdamani': 17132, 'ajmal': 17133, 'alamzai': 17134, 'toilets': 17135, 'nirvana': 17136, 'wed': 17137, 'realistic': 17138, 'puebla': 17139, 'herceptin': 17140, 'drugmaker': 17141, 'intelligent': 17142, 'pm': 17143, 'radius': 17144, 'alluding': 17145, 'badawi': 17146, 'obliged': 17147, 'tahhar': 17148, 'pietersen': 17149, 'islamophobia': 17150, 'xenophobia': 17151, 'peipah': 17152, 'tassos': 17153, 'ashes': 17154, 'nme': 17155, 'confiscation': 17156, 'khagrachhari': 17157, 'karradah': 17158, 'zelenovic': 17159, 'globally': 17160, 'aggravating': 17161, 'sidi': 17162, 'cheikh': 17163, 'abdallahi': 17164, 'afro': 17165, 'halifax': 17166, 'simulating': 17167, 'goldschmidt': 17168, 'rizgar': 17169, 'unpaid': 17170, 'interpret': 17171, 'millionaire': 17172, 'hopkins': 17173, 'karate': 17174, 'eddie': 17175, 'enzo': 17176, 'redline': 17177, 'budweiser': 17178, 'immense': 17179, 'shifts': 17180, 'hib': 17181, 'placards': 17182, 'mukakibibi': 17183, 'umurabyo': 17184, 'hatf': 17185, 'asb': 17186, 'asagoe': 17187, 'a380s': 17188, 'silently': 17189, 'kalma': 17190, 'acropolis': 17191, 'straying': 17192, 'expedite': 17193, 'atwood': 17194, 'artificially': 17195, 'hajdib': 17196, 'hycamtin': 17197, 'loj': 17198, 'cowboy': 17199, 'destinations': 17200, 'ecc': 17201, 'rmi': 17202, 'lackluster': 17203, 'unsettled': 17204, '1930': 17205, 'popes': 17206, 'interreligious': 17207, 'slater': 17208, 'mcqueen': 17209, 'saturated': 17210, 'dormitory': 17211, 'unforgivable': 17212, 'panyu': 17213, 'kuti': 17214, 'vivanco': 17215, 'lustiger': 17216, 'admits': 17217, 'faults': 17218, 'habsadeh': 17219, 'shargudud': 17220, 'feliciano': 17221, 'kimunya': 17222, 'ein': 17223, 'behave': 17224, 'lockerbie': 17225, 'brew': 17226, 'akhmad': 17227, 'sulaimaniyah': 17228, 'hyperinflation': 17229, 'refuges': 17230, 'vegetation': 17231, 'aruba': 17232, 'footing': 17233, 'jaques': 17234, 'harbhajan': 17235, '332': 17236, 'aviator': 17237, 'sideways': 17238, 'duress': 17239, 'verbally': 17240, 'gunning': 17241, 'arafa': 17242, 'schatten': 17243, 'embryonic': 17244, 'beleaguered': 17245, 'explosively': 17246, 'efps': 17247, 'healing': 17248, 'adhamiya': 17249, 'significance': 17250, 'iveta': 17251, 'rtl': 17252, 'downriver': 17253, 'inflexible': 17254, 'drel': 17255, '46th': 17256, 'contradict': 17257, 'caricatures': 17258, 'disgust': 17259, 'jabouri': 17260, 'invests': 17261, 'yomiuri': 17262, 'counselor': 17263, 'gunshots': 17264, '710': 17265, 'convicts': 17266, 'muqrin': 17267, 'squash': 17268, 'twigs': 17269, 'trod': 17270, 'talha': 17271, 'ulemas': 17272, 'betrayed': 17273, 'dovish': 17274, 'blocs': 17275, 'unilaterally': 17276, 'akhund': 17277, 'jinnah': 17278, 'reese': 17279, 'rdx': 17280, 'gomes': 17281, 'renunciation': 17282, 'taiba': 17283, 'verizon': 17284, 'kgb': 17285, 'sukhorenko': 17286, 'proclaiming': 17287, 'sandwich': 17288, 'smearing': 17289, 'luca': 17290, 'cantv': 17291, 'yasar': 17292, 'tolling': 17293, 'wept': 17294, 'echoed': 17295, 'wajama': 17296, 'gojko': 17297, 'flourished': 17298, 'riza': 17299, 'bourguiba': 17300, 'viable': 17301, 'alderman': 17302, 'raccoon': 17303, 'outpouring': 17304, 'dicaprio': 17305, 'sparing': 17306, 'kakooza': 17307, 'dye': 17308, 'insulation': 17309, '617': 17310, 'gulag': 17311, 'ri': 17312, 'bextra': 17313, 'passersby': 17314, 'donetsk': 17315, 'belligerence': 17316, 'circa': 17317, 'tenaris': 17318, 'natasha': 17319, 'couso': 17320, 'maori': 17321, 'coleman': 17322, 'brandished': 17323, 'rigorous': 17324, 'usage': 17325, 'wraps': 17326, 'botanical': 17327, 'faroe': 17328, 'taboo': 17329, 'favourite': 17330, 'lap': 17331, 'dhimbil': 17332, 'hawiye': 17333, 'fawzi': 17334, 'geithner': 17335, 'tytler': 17336, 'enticing': 17337, 'equate': 17338, 'sugiura': 17339, '453': 17340, 'lewthwaite': 17341, 'lid': 17342, 'greeks': 17343, 'eidsvig': 17344, 'choir': 17345, 'jac': 17346, 'ricci': 17347, 'hulya': 17348, 'kocyigit': 17349, 'profound': 17350, 'shameful': 17351, 'doghmush': 17352, 'gebran': 17353, 'nikola': 17354, 'paracel': 17355, 'bailouts': 17356, 'dwindling': 17357, 'fasten': 17358, 'overtaken': 17359, 'amusement': 17360, 'compare': 17361, 'ludlam': 17362, 'glacial': 17363, 'danuri': 17364, 'spontaneous': 17365, '1908': 17366, 'auwaerter': 17367, 'cyril': 17368, 'dirt': 17369, 'incited': 17370, 'mummy': 17371, 'sarcophagus': 17372, 'theyab': 17373, 'beatified': 17374, 'sainthood': 17375, 'prizren': 17376, 'lakers': 17377, 'leavitt': 17378, 'interwar': 17379, 'voronin': 17380, 'aie': 17381, 'budgeted': 17382, 'tedder': 17383, 'onerepublic': 17384, 'beatboxing': 17385, 'mistaking': 17386, 'karel': 17387, 'osvaldo': 17388, 'skill': 17389, 'hortefeux': 17390, 'unfolded': 17391, 'nicanor': 17392, 'faeldon': 17393, 'altar': 17394, 'sardenberg': 17395, 'validity': 17396, 'infrared': 17397, 'tf': 17398, 'jungles': 17399, 'voltage': 17400, 'shams': 17401, 'tomar': 17402, 'northernmost': 17403, 'mora': 17404, 'announces': 17405, 'descriptions': 17406, 'comedians': 17407, 'waldron': 17408, 'encompasses': 17409, 'butcher': 17410, 'mcneill': 17411, 'seyranlioglu': 17412, 'astros': 17413, 'comerio': 17414, 'compares': 17415, 'punxsutawney': 17416, 'copa': 17417, 'nassif': 17418, 'klein': 17419, 'maariv': 17420, 'rohingya': 17421, 'bmg': 17422, 'kabylie': 17423, 'ieds': 17424, 'blackmailer': 17425, 'floquet': 17426, 'lek': 17427, 'alimony': 17428, 'mcgrady': 17429, 'cavaliers': 17430, 'assists': 17431, 'empowers': 17432, 'mayan': 17433, 'persians': 17434, 'shevardnadze': 17435, 'acc': 17436, 'imply': 17437, 'fool': 17438, 'ticona': 17439, 'chute': 17440, 'libel': 17441, 'sodomy': 17442, 'ahvaz': 17443, 'chamrouen': 17444, 'colleges': 17445, 'sonntag': 17446, 'sics': 17447, 'sapporo': 17448, 'jeremic': 17449, 'cyclist': 17450, 'duggal': 17451, 'atmospheric': 17452, 'dodik': 17453, 'balasingham': 17454, 'pacheco': 17455, 'merchants': 17456, 'ashtiani': 17457, 'lashes': 17458, 'adultery': 17459, 'surano': 17460, 'eruptions': 17461, 'espersen': 17462, 'baghran': 17463, 'flaring': 17464, 'commissions': 17465, 'kahar': 17466, 'screaming': 17467, 'documenting': 17468, 'cummings': 17469, 'sacirbey': 17470, 'detonator': 17471, 'resembling': 17472, 'thura': 17473, 'mahn': 17474, 'ghormley': 17475, 'janice': 17476, 'kabungulu': 17477, 'flagrant': 17478, 'interesting': 17479, 'herrington': 17480, 'unbeaten': 17481, 'junoon': 17482, 'falu': 17483, 'rodham': 17484, 'dulaymi': 17485, 'poleo': 17486, 'mezerhane': 17487, 'lott': 17488, 'witt': 17489, 'digits': 17490, 'redmond': 17491, 'atambayev': 17492, 'absalon': 17493, 'kotkai': 17494, 'datanalisis': 17495, 'piazza': 17496, 'schwarzenburg': 17497, 'quds': 17498, 'darmawan': 17499, 'magazines': 17500, 'bumper': 17501, 'falcons': 17502, 'rams': 17503, 'invalidate': 17504, 'kubis': 17505, 'tain': 17506, 'marinellis': 17507, 'soundly': 17508, 'bengalis': 17509, 'fundraiser': 17510, 'goatherd': 17511, 'pulikovsky': 17512, 'lively': 17513, 'herd': 17514, 'sonata': 17515, 'follower': 17516, 'puteh': 17517, 'nursery': 17518, 'abia': 17519, 'ge': 17520, 'hints': 17521, 'tunisian': 17522, 'mentally': 17523, 'volodymyr': 17524, 'bernal': 17525, 'sopranos': 17526, 'reprocess': 17527, 'velvet': 17528, 'udd': 17529, 'falash': 17530, 'mura': 17531, 'songwriters': 17532, 'nominating': 17533, 'balart': 17534, 'antwerp': 17535, 'henman': 17536, 'mashhad': 17537, 'silos': 17538, 'implicate': 17539, 'congressmen': 17540, 'sammarei': 17541, 'westminster': 17542, 'torkham': 17543, 'seine': 17544, 'forklift': 17545, 'avril': 17546, 'lavigne': 17547, 'krasniqi': 17548, 'nicaraguans': 17549, 'mpp': 17550, 'disbursement': 17551, 'partridge': 17552, 'earnestly': 17553, 'mohaqiq': 17554, 'lazarevic': 17555, 'adi': 17556, 'abeto': 17557, 'purposely': 17558, 'culprits': 17559, 'netting': 17560, 'jaime': 17561, 'lloyd': 17562, 'olmo': 17563, 'lazaro': 17564, 'chanet': 17565, 'ushakov': 17566, 'siddiq': 17567, 'surkhanpha': 17568, 'kiriyenko': 17569, 'megawatts': 17570, 'zolfaghari': 17571, 'certainty': 17572, 'kostiw': 17573, 'distraction': 17574, 'tetanus': 17575, 'dabous': 17576, 'yanbu': 17577, 'howlwadaag': 17578, 'karua': 17579, 'murungaru': 17580, 'meek': 17581, 'ssr': 17582, 'truss': 17583, 'katharine': 17584, 'pellets': 17585, 'avid': 17586, 'montiglio': 17587, 'slaughterhouse': 17588, 'dushevina': 17589, 'razzano': 17590, 'tarmac': 17591, 'disembark': 17592, 'grytviken': 17593, 'nm': 17594, 'caste': 17595, 'stepanek': 17596, 'lilienfeld': 17597, 'udf': 17598, 'costello': 17599, 'aleksandar': 17600, 'yevpatoria': 17601, 'rumbling': 17602, 'rappers': 17603, 'achakzai': 17604, 'halloween': 17605, 'afars': 17606, 'flavors': 17607, 'lipstick': 17608, 'ear': 17609, 'abetting': 17610, 'cellist': 17611, 'constrain': 17612, 'shawal': 17613, 'syvkovych': 17614, 'eminem': 17615, 'mathers': 17616, 'fuller': 17617, 'grasshoppers': 17618, 'nambiar': 17619, 'equations': 17620, 'approximation': 17621, 'gospel': 17622, 'pilgrim': 17623, 'druze': 17624, 'tokar': 17625, 'fay': 17626, "l'aquilla": 17627, 'middlesbrough': 17628, 'essebar': 17629, 'ekici': 17630, 'kohl': 17631, 'annul': 17632, 'resolute': 17633, 'shambles': 17634, 'cardiac': 17635, 'catheterization': 17636, 'inserting': 17637, 'catheter': 17638, 'plug': 17639, 'burt': 17640, 'rutan': 17641, 'ansari': 17642, 'mojave': 17643, 'krona': 17644, 'wipha': 17645, 'haarde': 17646, 'affray': 17647, 'whisky': 17648, 'gritty': 17649, 'grignon': 17650, 'icg': 17651, 'akwei': 17652, 'thompson': 17653, 'losers': 17654, 'strengths': 17655, 'forums': 17656, 'deadliness': 17657, 'lourdes': 17658, 'ollanta': 17659, 'humala': 17660, 'tzotzil': 17661, 'darul': 17662, 'uloom': 17663, 'deoband': 17664, 'falsely': 17665, 'spurs': 17666, 'bulletproof': 17667, 'remodeled': 17668, 'eddin': 17669, 'yarkas': 17670, 'veronique': 17671, 'gspc': 17672, 'humiliated': 17673, 'liquefied': 17674, 'endeavors': 17675, 'tonnage': 17676, '1800s': 17677, 'pearling': 17678, 'attain': 17679, 'misdirected': 17680, 'revitalization': 17681, 'aclu': 17682, 'admiring': 17683, 'ungrateful': 17684, 'deliverer': 17685, 'marveled': 17686, 'pit': 17687, 'clutched': 17688, 'mathematicians': 17689, 'nostalgia': 17690, 'grammar': 17691, 'blacksmith': 17692, 'toughened': 17693, 'rigors': 17694, 'blacksmithing': 17695, 'potatoes': 17696, 'overlooked': 17697, 'overcharges': 17698, 'audits': 17699, 'bathed': 17700, 'ribbon': 17701, 'makepeace': 17702, 'morrell': 17703, 'brakes': 17704, 'quicken': 17705, 'evaluate': 17706, 'vesna': 17707, 'harlan': 17708, 'patrons': 17709, 'kabobs': 17710, 'pizza': 17711, 'rahimgul': 17712, 'sarawan': 17713, 'carve': 17714, 'toshiyuki': 17715, 'takano': 17716, 'arrows': 17717, 'shays': 17718, 'continual': 17719, 'burnt': 17720, 'pilotless': 17721, 'cancers': 17722, 'marrow': 17723, 'autoimmune': 17724, 'villarreal': 17725, 'nayef': 17726, 'forthcoming': 17727, 'busload': 17728, 'vans': 17729, 'suhaila': 17730, 'snagovo': 17731, 'baluchestan': 17732, 'bikers': 17733, 'sinatra': 17734, 'dominik': 17735, 'braces': 17736, 'rameez': 17737, 'annie': 17738, 'lennox': 17739, 'parishes': 17740, 'biathletes': 17741, 'transfusions': 17742, 'sneaked': 17743, 'jehan': 17744, 'pronouncements': 17745, 'churned': 17746, 'programmers': 17747, 'shigeru': 17748, 'appreciate': 17749, 'folks': 17750, 'balmy': 17751, 'snowman': 17752, 'khormato': 17753, 'arbaeen': 17754, 'backlog': 17755, 'graveyards': 17756, 'lush': 17757, 'magezi': 17758, 'triumfalnaya': 17759, 'alexeyeva': 17760, 'sakharov': 17761, 'allahabad': 17762, 'eject': 17763, 'adraskan': 17764, 'megan': 17765, 'ambuhl': 17766, 'summary': 17767, 'soyuzcapsule': 17768, 'abdurahman': 17769, 'fyodor': 17770, 'yurchikhin': 17771, 'kotov': 17772, 'likes': 17773, 'spaceflight': 17774, 'gagarin': 17775, 'tyurin': 17776, 'alegria': 17777, 'sunita': 17778, 'cropped': 17779, 'beard': 17780, 'svinarov': 17781, 'illegals': 17782, 'reconciled': 17783, 'propagandists': 17784, 'hateful': 17785, 'weathering': 17786, 'ups': 17787, 'cr': 17788, '787s': 17789, 'cathay': 17790, 'mustapha': 17791, 'ludwig': 17792, 'fuge': 17793, 'auctioneers': 17794, 'theological': 17795, '1826': 17796, 'annotations': 17797, 'crayon': 17798, 'rediscovery': 17799, 'reassessment': 17800, 'deaf': 17801, '1827': 17802, 'mortazavi': 17803, 'mana': 17804, 'neyestani': 17805, 'poked': 17806, 'tabriz': 17807, 'cockroach': 17808, 'peineta': 17809, 'foothold': 17810, 'trustco': 17811, 'llion': 17812, 'tampa': 17813, 'devolution': 17814, 'colonizing': 17815, 'swamps': 17816, 'cartago': 17817, 'disintegrated': 17818, 'offstage': 17819, 'bronchitis': 17820, 'trawling': 17821, 'venetiaan': 17822, 'tamed': 17823, 'waned': 17824, '1498': 17825, 'uncolonized': 17826, '1762': 17827, 'cacao': 17828, 'ringleaders': 17829, 'reinstituted': 17830, 'endless': 17831, 'shipwreck': 17832, 'inveighed': 17833, 'perchance': 17834, 'indulging': 17835, 'wand': 17836, 'hast': 17837, 'thyself': 17838, 'rami': 17839, 'tayyah': 17840, 'picasso': 17841, "o'keeffe": 17842, 'primimoda': 17843, 'ndimyake': 17844, 'mwakalyelye': 17845, 'hardcourts': 17846, 'carson': 17847, 'sopore': 17848, '542': 17849, 'orbited': 17850, 'schwehm': 17851, 'interplanetary': 17852, 'miniaturized': 17853, 'abdikadir': 17854, 'lafoole': 17855, 'evade': 17856, 'bristol': 17857, 'huon': 17858, 'angor': 17859, 'puth': 17860, 'lim': 17861, 'thierse': 17862, '711': 17863, 'unadorned': 17864, 'slabs': 17865, 'similarly': 17866, 'surma': 17867, 'sunamganj': 17868, 'caymans': 17869, 'esad': 17870, 'bajramovic': 17871, 'omarska': 17872, 'keraterm': 17873, 'wrested': 17874, 'obedience': 17875, 'scripture': 17876, 'talents': 17877, 'generosity': 17878, 'salvaged': 17879, 'generously': 17880, 'miillion': 17881, 'mural': 17882, 'deh': 17883, 'rawood': 17884, 'revising': 17885, 'faruq': 17886, 'qaddumi': 17887, 'moualem': 17888, 'itu': 17889, 'macrumors': 17890, 'keyboard': 17891, 'freetown': 17892, 'syphon': 17893, 'contaminant': 17894, 'interregnum': 17895, 'conclave': 17896, 'obtainable': 17897, 'toxins': 17898, 'radiological': 17899, 'needles': 17900, 'demining': 17901, 'deminers': 17902, 'iranativu': 17903, 'skater': 17904, 'dashing': 17905, 'whistle': 17906, 'blower': 17907, 'ghazala': 17908, 'naama': 17909, 'exaggerate': 17910, 'ascendant': 17911, 'wreathlaying': 17912, 'presumably': 17913, '636': 17914, '677': 17915, 'reaffirming': 17916, 'designates': 17917, 'europeanunion': 17918, 'razek': 17919, 'majaidie': 17920, 'shielded': 17921, 'teshiktosh': 17922, 'karasu': 17923, 'chapel': 17924, 'embarks': 17925, 'jae': 17926, 'carmen': 17927, 'stoves': 17928, 'physics': 17929, 'cages': 17930, 'gavutu': 17931, 'scratches': 17932, 'sunburn': 17933, 'undernourished': 17934, 'unisa': 17935, 'democratize': 17936, 'delano': 17937, 'zemedkun': 17938, 'tekle': 17939, 'wardheer': 17940, 'generalized': 17941, 'classifications': 17942, 'categories': 17943, 'maurits': 17944, 'nassau': 17945, '1715': 17946, '1810': 17947, 'adawe': 17948, 'saed': 17949, 'alleviating': 17950, 'underdevelopment': 17951, 'adapting': 17952, 'residing': 17953, 'towering': 17954, 'antiquated': 17955, 'vlore': 17956, 'perpetuity': 17957, 'reinvest': 17958, 'columbite': 17959, 'tantalite': 17960, 'parting': 17961, 'rite': 17962, 'topologist': 17963, 'doughnut': 17964, 'javal': 17965, 'harman': 17966, 'roskosmos': 17967, 'timidria': 17968, 'ates': 17969, 'bondage': 17970, 'kahraman': 17971, 'sadikoglu': 17972, 'adversity': 17973, 'serzh': 17974, 'sarkisian': 17975, 'vorotan': 17976, 'tatev': 17977, 'soften': 17978, 'manipulates': 17979, 'janabi': 17980, 'fequiere': 17981, 'betrand': 17982, 'siegouekou': 17983, 'mykola': 17984, 'suck': 17985, 'speeded': 17986, 'heave': 17987, 'oxidant': 17988, 'a9': 17989, 'stirling': 17990, 'pivotal': 17991, 'hypothermia': 17992, 'input': 17993, 'postings': 17994, 'marthinus': 17995, 'captures': 17996, 'swore': 17997, 'rana': 17998, 'bhagwandas': 17999, 'lyons': 18000, 'tendonitis': 18001, 'svetlana': 18002, 'kuznetsova': 18003, 'perceive': 18004, 'impulse': 18005, 'islamiah': 18006, 'netanya': 18007, 'proudly': 18008, 'albright': 18009, 'scharping': 18010, '765': 18011, 'gregorian': 18012, 'conspicuous': 18013, 'skullcaps': 18014, 'mismanaging': 18015, 'devotion': 18016, 'finest': 18017, 'hasty': 18018, 'disproportionately': 18019, 'journals': 18020, 'lancetand': 18021, 'lancet': 18022, 'neurology': 18023, 'counteracting': 18024, 'rothwell': 18025, 'numbness': 18026, 'slurred': 18027, 'prohibitions': 18028, 'disprove': 18029, 'salons': 18030, 'parlors': 18031, 'unsuitable': 18032, 'hairdresser': 18033, 'nulcear': 18034, 'addicts': 18035, 'squalid': 18036, 'brutalized': 18037, 'realignment': 18038, 'commissioners': 18039, 'deliberations': 18040, 'mcpherson': 18041, 'hover': 18042, 'hurriyet': 18043, 'pech': 18044, 'younus': 18045, 'giuseppe': 18046, 'cagliari': 18047, 'petronio': 18048, 'fresco': 18049, 'misanthropic': 18050, 'frans': 18051, 'rotterdam': 18052, 'bureaucrats': 18053, 'renaming': 18054, 'sectarianism': 18055, 'discrepancy': 18056, 'roadway': 18057, 'evi': 18058, 'sachenbacher': 18059, 'endurance': 18060, 'epo': 18061, 'kikkan': 18062, 'randall': 18063, 'leif': 18064, 'plastics': 18065, 'metathesis': 18066, 'rearranged': 18067, 'catalysts': 18068, 'hotspur': 18069, 'offseason': 18070, 'tendon': 18071, 'hotspurs': 18072, 'redknapp': 18073, 'barahona': 18074, 'switching': 18075, 'cocked': 18076, 'dengfeng': 18077, 'akerfeldt': 18078, 'wallowing': 18079, 'gingl': 18080, 'caribs': 18081, 'crnojevic': 18082, 'theocracy': 18083, 'princes': 18084, '1852': 18085, 'looser': 18086, 'basutoland': 18087, 'basuto': 18088, 'hegang': 18089, 'moshoeshoe': 18090, 'letsie': 18091, 'aegis': 18092, 'arisen': 18093, 'jaws': 18094, 'saddle': 18095, 'bridled': 18096, 'theirs': 18097, 'pomegranate': 18098, 'boastful': 18099, 'disputings': 18100, 'gloves': 18101, 'tongues': 18102, 'pugilists': 18103, 'statistician': 18104, 'frost': 18105, 'whispered': 18106, 'kfm': 18107, 'sedition': 18108, 'rory': 18109, 'irishman': 18110, 'manta': 18111, 'evolves': 18112, 'opt': 18113, 'stockhlom': 18114, 'harold': 18115, 'pinter': 18116, 'mocked': 18117, 'shahr': 18118, 'kord': 18119, 'raz': 18120, 'binh': 18121, 'nana': 18122, 'effah': 18123, 'apenteng': 18124, 'precede': 18125, 'conditioning': 18126, 'rot': 18127, 'resold': 18128, 'kissufin': 18129, 'spotting': 18130, 'farmlands': 18131, 'context': 18132, 'conductive': 18133, 'alwi': 18134, 'vied': 18135, '176': 18136, 'kush': 18137, 'merka': 18138, 'turnabout': 18139, 'tigray': 18140, 'amhara': 18141, 'oromia': 18142, 'humayun': 18143, 'persona': 18144, 'grata': 18145, 'bilge': 18146, 'mardin': 18147, 'allotment': 18148, 'besir': 18149, 'atalay': 18150, 'farmaner': 18151, 'hatched': 18152, 'candle': 18153, 'storey': 18154, 'calmly': 18155, 'bins': 18156, 'buyouts': 18157, 'hourly': 18158, 'ettore': 18159, 'francesco': 18160, 'sequi': 18161, 'responsiblity': 18162, 'worrisome': 18163, 'gears': 18164, 'juncture': 18165, 'dubious': 18166, 'khalikov': 18167, 'needing': 18168, 'barranquilla': 18169, 'obstruct': 18170, 'reciprocated': 18171, 'barrios': 18172, 'reynaldo': 18173, 'larrazabal': 18174, 'collusion': 18175, 'nureddin': 18176, 'kidwai': 18177, 'alertness': 18178, 'conceivable': 18179, 'timessays': 18180, 'kehla': 18181, 'staving': 18182, 'bunji': 18183, 'dogharoun': 18184, 'salahaddin': 18185, 'zafaraniyah': 18186, 'reutersnews': 18187, 'swapping': 18188, 'swapped': 18189, 'subterranean': 18190, 'headline': 18191, 'vh1': 18192, 'sensex': 18193, 'hama': 18194, 'aichatou': 18195, 'mindaoudou': 18196, 'albade': 18197, 'abouba': 18198, 'mahamane': 18199, 'lamine': 18200, 'zeine': 18201, 'admired': 18202, 'unforseeable': 18203, 'arbitrator': 18204, 'bobsled': 18205, 'altenberg': 18206, 'zach': 18207, 'whistleblower': 18208, 'revealing': 18209, 'neutron': 18210, 'pointless': 18211, 'implicitly': 18212, 'beersheba': 18213, 'underestimated': 18214, 'nawzad': 18215, 'caffeine': 18216, 'tablets': 18217, 'grapple': 18218, 'ponds': 18219, 'fixes': 18220, 'dahoun': 18221, 'gignor': 18222, 'tabarre': 18223, 'moutaz': 18224, 'slough': 18225, 'mohannad': 18226, 'farfetched': 18227, 'advertised': 18228, 'volta': 18229, 'securely': 18230, 'burkinabe': 18231, 'tame': 18232, 'kuna': 18233, 'lag': 18234, 'genoese': 18235, 'fortress': 18236, '1215': 18237, 'grimaldi': 18238, '1297': 18239, '1331': 18240, '1419': 18241, 'linkup': 18242, 'scenery': 18243, 'forsaking': 18244, 'adapted': 18245, 'contentment': 18246, 'currycombing': 18247, 'rubbing': 18248, 'oats': 18249, 'rochester': 18250, 'ny': 18251, 'fervently': 18252, 'styling': 18253, 'gel': 18254, 'alarmist': 18255, 'advisories': 18256, '1632': 18257, 'froce': 18258, 'redistricting': 18259, 'incorporating': 18260, 'frenzy': 18261, 'lawmaking': 18262, 'menas': 18263, 'landholdings': 18264, '154th': 18265, 'général': 18266, 'kountché': 18267, 'blistering': 18268, 'ouwa': 18269, 'shafy': 18270, 'goalkeeper': 18271, 'hadary': 18272, 'bordeaux': 18273, 'equalizer': 18274, 'midfield': 18275, 'fathallah': 18276, 'offside': 18277, 'inauspicious': 18278, 'soufriere': 18279, 'sylvester': 18280, 'laloo': 18281, 'shores': 18282, 'sickness': 18283, 'hemorrhages': 18284, 'dislodged': 18285, 'jabella': 18286, 'hazelnuts': 18287, 'nonalcoholic': 18288, 'merciful': 18289, 'tufail': 18290, 'matoo': 18291, 'erzerum': 18292, 'kars': 18293, 'mattoo': 18294, 'apprehension': 18295, 'simplified': 18296, 'invincible': 18297, 'neediest': 18298, 'isi': 18299, 'pinning': 18300, 'igniting': 18301, 'discarding': 18302, 'compromises': 18303, 'sore': 18304, 'getter': 18305, 'vejjajiva': 18306, 'somchai': 18307, 'wongsawat': 18308, 'shirted': 18309, 'sainworla': 18310, 'veritas': 18311, 'butty': 18312, 'beaming': 18313, 'revolutionized': 18314, 'tended': 18315, 'repentance': 18316, 'sensual': 18317, 'culminates': 18318, 'indulgence': 18319, '1853': 18320, '1864': 18321, 'ordeal': 18322, 'teleconference': 18323, 'noumea': 18324, '1055': 18325, '1002': 18326, 'wafted': 18327, 'persecutors': 18328, 'stationing': 18329, 'unceasing': 18330, 'indissoluble': 18331, 'habitations': 18332, 'edelist': 18333, 'archival': 18334, 'transcript': 18335, 'abound': 18336, 'discern': 18337, 'zaraqawi': 18338, 'sanjaya': 18339, 'baru': 18340, 'stoppages': 18341, 'alitalia': 18342, 'bormio': 18343, 'orchid': 18344, 'cauca': 18345, 'cedatos': 18346, 'inefficiency': 18347, 'centralize': 18348, 'wpmf': 18349, '680': 18350, 'outbursts': 18351, 'loi': 18352, 'rashakai': 18353, 'khata': 18354, 'manhandling': 18355, 'deserting': 18356, 'matti': 18357, 'vanhanen': 18358, 'paraded': 18359, 'maysan': 18360, 'muthana': 18361, 'redeployed': 18362, 'abijan': 18363, 'wandering': 18364, 'nicobari': 18365, 'emaciated': 18366, 'esmatullah': 18367, 'alizai': 18368, 'neshin': 18369, 'chora': 18370, 'unproven': 18371, 'complexes': 18372, 'desalinization': 18373, 'toulouse': 18374, 'esquisabel': 18375, 'urtuzaga': 18376, 'mushir': 18377, 'senseless': 18378, 'dea': 18379, 'gibran': 18380, 'alaina': 18381, 'tepid': 18382, 'dixie': 18383, 'chicks': 18384, 'charisma': 18385, 'goody': 18386, 'payable': 18387, 'flemings': 18388, 'walloons': 18389, 'oases': 18390, 'tabaldo': 18391, 'tribally': 18392, 'ashgabat': 18393, 'racy': 18394, 'sensation': 18395, 'redenomination': 18396, 'manat': 18397, 'bulwark': 18398, 'janos': 18399, 'kadar': 18400, 'goulash': 18401, 'marsh': 18402, 'pretend': 18403, 'gait': 18404, 'chink': 18405, 'fatas': 18406, 'synchronize': 18407, 'nadhem': 18408, 'bradman': 18409, 'ricky': 18410, 'ponting': 18411, 'zaheer': 18412, 'saqeb': 18413, 'cordons': 18414, 'barbed': 18415, '050': 18416, 'calories': 18417, 'pacifism': 18418, 'speculative': 18419, 'notches': 18420, 'downgrades': 18421, 'quan': 18422, 'caller': 18423, 'arraignment': 18424, 'georgy': 18425, 'maltreat': 18426, 'hastert': 18427, 'centraql': 18428, 'mani': 18429, 'shankar': 18430, 'jadoon': 18431, '57th': 18432, '588': 18433, 'kravchenko': 18434, 'tomini': 18435, 'gorontalo': 18436, 'barreled': 18437, '668': 18438, 'tutwiler': 18439, 'rangers': 18440, 'incinerated': 18441, 'caregivers': 18442, 'mlada': 18443, 'fronta': 18444, 'dnes': 18445, 'intake': 18446, 'armchair': 18447, 'tracheotomy': 18448, 'bethelehem': 18449, 'mardan': 18450, 'considerations': 18451, 'parlimentary': 18452, 'duluiyah': 18453, 'extinguished': 18454, 'guardsman': 18455, 'sawers': 18456, 'paktiya': 18457, 'lien': 18458, 'bullhorn': 18459, 'hubbard': 18460, 'feldstein': 18461, '357': 18462, 'neelie': 18463, 'kroes': 18464, 'snarling': 18465, 'sheremetyevo': 18466, 'slicked': 18467, 'encased': 18468, 'taxis': 18469, 'originate': 18470, 'touchstone': 18471, 'warms': 18472, 'raziq': 18473, 'alasay': 18474, 'spindleruv': 18475, 'mlyn': 18476, 'kathrin': 18477, 'zettel': 18478, 'marlies': 18479, 'schild': 18480, 'zvarych': 18481, 'mae': 18482, 'aborted': 18483, 'abduct': 18484, 'susceptible': 18485, 'affluence': 18486, 'inoculated': 18487, 'atheist': 18488, 'gregoire': 18489, 'barreling': 18490, 'mayhem': 18491, 'discourages': 18492, 'sidewalks': 18493, 'kilowatt': 18494, 'rasul': 18495, 'aadham': 18496, 'baucus': 18497, 'montana': 18498, 'exemption': 18499, 'rebuked': 18500, 'infringement': 18501, 'starving': 18502, 'sweet': 18503, 'avi': 18504, 'dichter': 18505, 'mhawesh': 18506, 'qadi': 18507, 'naseem': 18508, 'haji': 18509, 'freeskate': 18510, 'hao': 18511, 'liv': 18512, 'grete': 18513, 'kogelo': 18514, 'winston': 18515, 'churchill': 18516, 'maneuvering': 18517, 'maroney': 18518, 'tapes': 18519, 'valentino': 18520, 'kenenisa': 18521, 'bekele': 18522, '965': 18523, 'rumor': 18524, 'tigris': 18525, 'wildly': 18526, 'secessionists': 18527, 'concentrations': 18528, 'zamfara': 18529, 'epidemiologists': 18530, 'kidneys': 18531, 'reproductive': 18532, 'chinhoyi': 18533, 'disinfectant': 18534, 'masahiko': 18535, 'komura': 18536, 'geelani': 18537, 'quashed': 18538, 'saga': 18539, 'massively': 18540, 'unvarnished': 18541, 'snuff': 18542, 'mailing': 18543, 'entitles': 18544, 'downsized': 18545, 'overstated': 18546, 'supplemented': 18547, '1809': 18548, 'albeit': 18549, 'finns': 18550, 'initiation': 18551, 'clipperton': 18552, 'altars': 18553, 'gently': 18554, 'grasp': 18555, 'mathematics': 18556, 'pharaoh': 18557, 'helium': 18558, 'pencils': 18559, 'elevators': 18560, 'escalators': 18561, 'switches': 18562, 'diapers': 18563, 'keel': 18564, 'recharge': 18565, 'malnourished': 18566, 'elmendorf': 18567, 'drik': 18568, 'gassing': 18569, 'glyn': 18570, 'alaeddin': 18571, 'udi': 18572, 'votel': 18573, 'reemergence': 18574, 'berth': 18575, 'placid': 18576, 'grimmette': 18577, 'martinin': 18578, 'mazdzer': 18579, 'benshoofin': 18580, 'retrosi': 18581, 'hamlin': 18582, 'zablocki': 18583, 'presidium': 18584, 'hyong': 18585, 'sop': 18586, 'paek': 18587, 'veligonda': 18588, 'inayatullah': 18589, 'marshy': 18590, 'brightly': 18591, 'sodden': 18592, 'pitchers': 18593, 'stints': 18594, 'sep': 18595, 'postseason': 18596, 'wailed': 18597, 'yad': 18598, 'vashem': 18599, 'carriages': 18600, 'roma': 18601, 'gypsies': 18602, 'malawai': 18603, 'luc': 18604, 'chatel': 18605, 'objecting': 18606, 'gauthier': 18607, 'sibling': 18608, 'mouths': 18609, 'unmarried': 18610, 'palin': 18611, 'cotecna': 18612, 'siyam': 18613, 'solovtsov': 18614, 'intermediate': 18615, 'uncalled': 18616, 'mirek': 18617, 'topolanek': 18618, 'redskins': 18619, 'doubted': 18620, 'notched': 18621, 'cruised': 18622, 'gimelstob': 18623, 'marach': 18624, 'todd': 18625, 'widom': 18626, 'juhyi': 18627, 'purports': 18628, 'embraces': 18629, 'boisvert': 18630, 'winterized': 18631, 'essentially': 18632, 'ruphael': 18633, 'amen': 18634, 'benishangul': 18635, 'gumuz': 18636, 'latrines': 18637, 'contaminate': 18638, 'tribune': 18639, 'kuba': 18640, 'rats': 18641, 'limestone': 18642, 'sunflower': 18643, 'subsidize': 18644, 'rhine': 18645, 'westphalia': 18646, 'profiled': 18647, 'unauthenticated': 18648, 'bavarian': 18649, 'guenther': 18650, 'beckstein': 18651, 'papillovirus': 18652, 'lesions': 18653, 'andrej': 18654, 'hermlin': 18655, 'gerd': 18656, 'uwe': 18657, 'fleur': 18658, 'dissel': 18659, 'gush': 18660, 'katif': 18661, 'muthmahien': 18662, '183': 18663, 'watchlist': 18664, '244': 18665, 'aggressors': 18666, 'abdulatif': 18667, 'sener': 18668, 'guinean': 18669, 'glades': 18670, 'wading': 18671, 'alligators': 18672, 'lane': 18673, 'awesome': 18674, 'sanctioning': 18675, 'chronicles': 18676, 'feminist': 18677, 'frode': 18678, 'ruhpolding': 18679, 'rösch': 18680, 'greis': 18681, 'misses': 18682, 'nahdlatul': 18683, 'ulama': 18684, 'hasyim': 18685, 'abakar': 18686, 'dirk': 18687, 'lever': 18688, 'glen': 18689, 'skyscraper': 18690, 'darien': 18691, 'superintendent': 18692, 'motives': 18693, 'bulldozer': 18694, 'usmani': 18695, 'daynunay': 18696, 'betweens': 18697, 'teheran': 18698, 'pled': 18699, 'gikoro': 18700, 'arlete': 18701, 'ramaroson': 18702, 'organisation': 18703, 'doncasters': 18704, 'monteiro': 18705, 'enshrine': 18706, 'yuganskneftgaz': 18707, 'retractable': 18708, 'redeem': 18709, 'coupon': 18710, 'disrepute': 18711, 'brake': 18712, 'decelerated': 18713, 'expenditure': 18714, 'hoard': 18715, 'iwf': 18716, '1291': 18717, 'cantons': 18718, '1499': 18719, 'perching': 18720, 'cawed': 18721, 'foreboded': 18722, 'cry': 18723, 'unpleasant': 18724, 'implacable': 18725, 'pelt': 18726, 'inning': 18727, 'math': 18728, 'jaji': 18729, 'ratifying': 18730, 'hanan': 18731, 'raufi': 18732, 'piercing': 18733, 'sari': 18734, 'inhale': 18735, 'smoker': 18736, 'addictive': 18737, 'lslamic': 18738, 'tham': 18739, 'krabok': 18740, 'msika': 18741, 'muzenda': 18742, 'emmerson': 18743, 'mnangawa': 18744, 'quentier': 18745, 'cyrus': 18746, 'laywers': 18747, 'prayerful': 18748, 'fenty': 18749, 'zhaotong': 18750, 'bu': 18751, 'khairul': 18752, 'amri': 18753, 'agu': 18754, 'casmir': 18755, 'mahyadi': 18756, 'panggabean': 18757, 'osako': 18758, 'subpoenas': 18759, 'rossendorf': 18760, 'momir': 18761, 'oilrig': 18762, 'getulio': 18763, 'ironically': 18764, 'kitgum': 18765, 'bigombe': 18766, 'drinkable': 18767, 'defillo': 18768, 'nonbinding': 18769, '83rd': 18770, 'hashish': 18771, 'genital': 18772, 'genitalia': 18773, 'hemorrhaging': 18774, 'invitations': 18775, 'iftars': 18776, 'morphine': 18777, 'kibar': 18778, 'delinquency': 18779, 'baume': 18780, 'teikoku': 18781, 'characteristics': 18782, 'plundering': 18783, 'reparations': 18784, 'dedicate': 18785, 'diana': 18786, '182': 18787, 'refinement': 18788, 'fuheid': 18789, 'nashmi': 18790, 'khobar': 18791, 'racketeering': 18792, 'suburban': 18793, 'fists': 18794, 'salute': 18795, 'rachel': 18796, 'armada': 18797, 'mashruh': 18798, 'mahara': 18799, 'dialysis': 18800, 'anat': 18801, 'dolev': 18802, 'zhongwei': 18803, 'sitaula': 18804, 'reappearing': 18805, 'hassem': 18806, 'ramsey': 18807, 'headfirst': 18808, 'snowbank': 18809, 'roughed': 18810, 'marrying': 18811, 'garissa': 18812, 'oranges': 18813, 'commodies': 18814, 'discrepancies': 18815, 'dili': 18816, 'martinho': 18817, 'gusmao': 18818, 'horta': 18819, 'olo': 18820, 'deregulation': 18821, 'uncontrolled': 18822, 'sudi': 18823, 'yalahow': 18824, 'edgware': 18825, 'congratulating': 18826, 'concides': 18827, 'notifying': 18828, 'brandenburg': 18829, 'collisions': 18830, 'stefanie': 18831, 'werner': 18832, 'anchorage': 18833, 'bedroom': 18834, 'arjun': 18835, 'disqualifications': 18836, 'compelling': 18837, 'silesia': 18838, 'gemstone': 18839, 'auctioned': 18840, 'bested': 18841, 'hancock': 18842, 'moussaieff': 18843, 'gemstones': 18844, 'vivid': 18845, 'boron': 18846, 'crystal': 18847, 'revoke': 18848, 'identifications': 18849, 'anticipating': 18850, 'horbach': 18851, 'ubangi': 18852, 'shari': 18853, 'tumultuous': 18854, 'misrule': 18855, 'ange': 18856, 'patasse': 18857, 'tacit': 18858, '1892': 18859, '1915': 18860, 'makin': 18861, 'tarawa': 18862, 'garrisons': 18863, '1943': 18864, 'oversupply': 18865, 'jilani': 18866, 'contagion': 18867, 'nook': 18868, 'intruded': 18869, 'stranger': 18870, 'antipathy': 18871, 'apologise': 18872, 'madagonians': 18873, 'chagrined': 18874, 'fergana': 18875, 'nonexistent': 18876, 'uncontained': 18877, 'christiane': 18878, 'berthiaume': 18879, 'navi': 18880, 'pillay': 18881, 'taint': 18882, 'amputation': 18883, 'heckling': 18884, 'anjem': 18885, 'choudary': 18886, 'ghurabaa': 18887, 'zirve': 18888, 'wrestling': 18889, 'trabzon': 18890, 'blabague': 18891, 'marboulaye': 18892, 'tram': 18893, 'diluting': 18894, 'inhibit': 18895, 'roving': 18896, 'panorama': 18897, 'descend': 18898, 'adolescent': 18899, 'ik': 18900, 'obudu': 18901, 'oshiomole': 18902, 'healthier': 18903, 'cardiovascular': 18904, 'aggressor': 18905, 'estimating': 18906, 'adjusters': 18907, 'ravage': 18908, 'underestimating': 18909, 'dhafra': 18910, '2s': 18911, '380th': 18912, 'expeditionary': 18913, 'mizhir': 18914, 'yousi': 18915, 'mukhtaran': 18916, 'contractual': 18917, 'biscaglia': 18918, 'overtook': 18919, 'ashia': 18920, 'scriptures': 18921, 'camillo': 18922, 'ruini': 18923, 'cardio': 18924, 'urinary': 18925, 'soh': 18926, 'antonov': 18927, 'sok': 18928, 'chol': 18929, 'doo': 18930, "ya'akov": 18931, 'lamp': 18932, 'wayward': 18933, 'almanza': 18934, 'logarda': 18935, 'dror': 18936, 'soku': 18937, 'females': 18938, 'interviewers': 18939, 'yoram': 18940, 'haham': 18941, 'mobsters': 18942, 'vibrant': 18943, 'roemer': 18944, 'kazem': 18945, 'vaziri': 18946, 'hamaneh': 18947, 'momammad': 18948, 'amy': 18949, 'katz': 18950, 'fabian': 18951, 'adolphus': 18952, 'wabara': 18953, '273': 18954, 'welsh': 18955, 'hlinethaya': 18956, 'roses': 18957, 'orphan': 18958, 'liz': 18959, 'rosenberg': 18960, '412': 18961, 'athanase': 18962, 'seromba': 18963, 'nandurbar': 18964, 'navapur': 18965, 'heihe': 18966, 'blagoveshchensk': 18967, 'undesirable': 18968, 'pardoning': 18969, 'scouring': 18970, 'angelus': 18971, 'loves': 18972, 'llc': 18973, 'qualifies': 18974, 'takatoshi': 18975, 'toufik': 18976, 'hanouichi': 18977, 'mohcine': 18978, 'bouarfa': 18979, 'jew': 18980, 'meknes': 18981, 'fez': 18982, 'trilateral': 18983, 'gadahn': 18984, 'tibaijuka': 18985, 'zimbabweans': 18986, 'charting': 18987, 'mentality': 18988, 'daioyu': 18989, 'senkaku': 18990, 'japanto': 18991, 'scoreless': 18992, 'abalo': 18993, '53rd': 18994, 'ahn': 18995, 'sputtered': 18996, 'kember': 18997, 'loney': 18998, 'harmeet': 18999, 'sooden': 19000, 'damiao': 19001, 'fradique': 19002, 'awarding': 19003, 'doubtful': 19004, 'gisagara': 19005, 'braved': 19006, 'complemented': 19007, 'cubana': 19008, 'walbrecher': 19009, 'ineligible': 19010, 'feedstock': 19011, 'ceramics': 19012, 'fabrics': 19013, 'untaxed': 19014, 'harmonizing': 19015, 'occupier': 19016, 'exploit': 19017, 'overstaffed': 19018, 'afloat': 19019, 'senegambia': 19020, 'envisaged': 19021, 'mfdc': 19022, 'snared': 19023, 'homewards': 19024, 'leap': 19025, 'cofer': 19026, 'installs': 19027, 'builder': 19028, 'bribing': 19029, 'janez': 19030, 'jansa': 19031, 'roused': 19032, 'booby': 19033, 'retrieving': 19034, 'hewlett': 19035, 'packard': 19036, 'carly': 19037, 'printer': 19038, 'compaq': 19039, 'wayman': 19040, 'syarhei': 19041, 'antonchyk': 19042, 'unsanctioned': 19043, 'insincere': 19044, 'shanwei': 19045, 'soleiman': 19046, 'tolima': 19047, 'antioquia': 19048, 'simba': 19049, 'banadir': 19050, 'landscape': 19051, 'shatt': 19052, 'bulba': 19053, 'payback': 19054, 'khogyani': 19055, 'espino': 19056, 'thapa': 19057, 'examiner': 19058, 'grills': 19059, 'castelgandolfo': 19060, 'nuremberg': 19061, 'frankenstadion': 19062, 'lukas': 19063, 'podolski': 19064, 'ballack': 19065, '48th': 19066, 'loser': 19067, 'leipzig': 19068, 'ukrgazenergo': 19069, 'rosukrenergo': 19070, 'bychkova': 19071, 'shahar': 19072, 'scruff': 19073, 'czink': 19074, 'berkofsky': 19075, 'firebrand': 19076, 'virtuosity': 19077, 'berkovsky': 19078, 'scot': 19079, 'riddlesberger': 19080, 'solicit': 19081, 'colonels': 19082, 'solidify': 19083, 'nationalistic': 19084, 'tendencies': 19085, 'afl': 19086, 'cio': 19087, 'unionists': 19088, 'vocation': 19089, 'pope2you': 19090, 'ringwald': 19091, 'artemisia': 19092, 'artemisian': 19093, 'mefloquine': 19094, 'firat': 19095, 'hakurk': 19096, 'warmongering': 19097, 'plymouth': 19098, 'djalil': 19099, 'careless': 19100, 'discarded': 19101, 'novaya': 19102, 'gazeta': 19103, 'volvo': 19104, 'allocating': 19105, 'paya': 19106, 'lebar': 19107, 'refuel': 19108, 'starye': 19109, 'novye': 19110, 'disinformation': 19111, 'timelines': 19112, 'monaf': 19113, 'purnomo': 19114, 'yusgiantoro': 19115, '770': 19116, 'plum': 19117, 'nasir': 19118, 'dhakla': 19119, 'juster': 19120, 'micro': 19121, 'miniature': 19122, 'christina': 19123, 'rocca': 19124, 'mundane': 19125, 'poitou': 19126, 'charentes': 19127, 'respublika': 19128, 'disparaging': 19129, 'kazakhs': 19130, 'dyrdina': 19131, 'desecrating': 19132, 'eliashiv': 19133, 'refraining': 19134, 'iftaar': 19135, 'kabal': 19136, 'danzhou': 19137, 'ripe': 19138, '118': 19139, '388': 19140, 'necessitate': 19141, 'amounting': 19142, 'projection': 19143, 'bundesbank': 19144, 'annum': 19145, 'tapered': 19146, 'olav': 19147, 'tryggvason': 19148, '994': 19149, '1397': 19150, 'cession': 19151, 'outset': 19152, 'rwandans': 19153, 'retaking': 19154, 'comparative': 19155, 'mimics': 19156, 'pupils': 19157, 'arrayed': 19158, 'courtiers': 19159, 'courtier': 19160, 'mischief': 19161, 'amidst': 19162, 'longing': 19163, 'plunder': 19164, 'wiled': 19165, 'scar': 19166, 'cavity': 19167, 'annyaso': 19168, 'onulak': 19169, 'passionate': 19170, 'charlie': 19171, 'influences': 19172, 'hillside': 19173, 'thunderstorms': 19174, 'qada': 19175, 'eastbourne': 19176, 'strapping': 19177, 'shortness': 19178, 'accomplishments': 19179, 'smoky': 19180, 'recommit': 19181, 'ozone': 19182, 'acres': 19183, 'chide': 19184, 'sidestep': 19185, 'hector': 19186, 'robberies': 19187, 'belo': 19188, 'horizonte': 19189, 'fortaleza': 19190, 'landscaping': 19191, '944': 19192, 'bamboo': 19193, 'kyu': 19194, 'interfered': 19195, 'aflame': 19196, '204': 19197, 'permitting': 19198, 'amiens': 19199, 'savigny': 19200, 'orge': 19201, 'firebomb': 19202, 'zamoanga': 19203, 'compressed': 19204, 'liquified': 19205, 'mccullogh': 19206, 'letterman': 19207, 'ibn': 19208, '64th': 19209, 'battleship': 19210, 'waver': 19211, 'mess': 19212, 'antiviral': 19213, 'fossils': 19214, 'fossilized': 19215, 'eromanga': 19216, 'weighs': 19217, 'prehistoric': 19218, 'roam': 19219, 'sauropods': 19220, 'sajida': 19221, 'dreadfully': 19222, 'barmer': 19223, 'anchorwoman': 19224, 'chilpancingo': 19225, 'sos': 19226, 'dissatisified': 19227, 'soderling': 19228, 'finale': 19229, 'finalist': 19230, 'gael': 19231, 'monfils': 19232, 'potro': 19233, 'photography': 19234, 'jovicevic': 19235, 'spaceport': 19236, 'heavier': 19237, 'precise': 19238, '5400': 19239, 'homicides': 19240, 'badri': 19241, 'silencers': 19242, 'neftaly': 19243, 'deceptive': 19244, 'tar': 19245, 'labeling': 19246, 'aspect': 19247, 'altria': 19248, 'funneled': 19249, 'counterterrorist': 19250, 'contestant': 19251, 'limb': 19252, 'sleeve': 19253, 'prosthesis': 19254, 'viva': 19255, 'curtains': 19256, 'underfoot': 19257, 'akwa': 19258, 'ibom': 19259, 'afren': 19260, '3600': 19261, 'mukhtar': 19262, 'yilmaz': 19263, 'resit': 19264, 'isik': 19265, 'hawija': 19266, 'scarcity': 19267, 'condolezza': 19268, 'cbo': 19269, 'threefold': 19270, 'negar': 19271, 'kerman': 19272, 'torbat': 19273, 'heydariyeh': 19274, 'rehab': 19275, 'wonderland': 19276, '570': 19277, 'dui': 19278, 'unconventional': 19279, 'defensible': 19280, 'fallback': 19281, 'khair': 19282, 'shuja': 19283, 'malian': 19284, 'nouadhibou': 19285, '235': 19286, 'villas': 19287, 'inhibits': 19288, 'hadj': 19289, 'ondimba': 19290, 'stonemason': 19291, 'marinus': 19292, '301': 19293, 'marxism': 19294, 'renamo': 19295, 'joaquim': 19296, 'chissano': 19297, 'emilio': 19298, 'silverstone': 19299, 'dollarization': 19300, 'uphill': 19301, 'startup': 19302, 'saltillo': 19303, 'cameroonian': 19304, 'kilted': 19305, 'playlists': 19306, 'mwiraria': 19307, 'unveiling': 19308, 'bellamy': 19309, 'baixinglou': 19310, 'chaoyang': 19311, 'diners': 19312, 'waiters': 19313, 'backtrack': 19314, 'hausas': 19315, 'ibos': 19316, 'anibal': 19317, 'correo': 19318, 'caroni': 19319, 'styled': 19320, 'deploring': 19321, 'jela': 19322, 'franceschi': 19323, 'hampton': 19324, 'alexi': 19325, 'nenets': 19326, 'acquittal': 19327, 'kristof': 19328, 'downpour': 19329, 'konstantinos': 19330, 'iaaf': 19331, 'pentastar': 19332, 'dodge': 19333, 'amiri': 19334, 'shiite': 19335, 'retract': 19336, 'safwat': 19337, 'hamstring': 19338, 'athletic': 19339, 'marian': 19340, 'oprea': 19341, 'dmitrij': 19342, 'valukevic': 19343, 'flocked': 19344, 'televisions': 19345, 'staggering': 19346, 'overture': 19347, 'scuttle': 19348, 'dimension': 19349, 'priesthood': 19350, 'hierarchy': 19351, 'swiftly': 19352, 'pilings': 19353, 'ninewa': 19354, '2900': 19355, 'jeered': 19356, 'joan': 19357, 'baez': 19358, 'vigils': 19359, 'incumbents': 19360, 'damiri': 19361, 'tayyeb': 19362, 'ayoub': 19363, 'fewest': 19364, 'cfco': 19365, 'pointe': 19366, 'noire': 19367, 'aab': 19368, 'ghum': 19369, 'fnj': 19370, 'worsens': 19371, 'dhabi': 19372, 'trusts': 19373, 'painkiller': 19374, 'diploma': 19375, 'haggling': 19376, 'immigrate': 19377, 'unveils': 19378, 'carmakers': 19379, 'badran': 19380, 'wheel': 19381, 'suv': 19382, 'zookeepers': 19383, 'incubator': 19384, 'jingguo': 19385, 'nurse': 19386, 'gomoa': 19387, 'buduburam': 19388, 'rabiah': 19389, 'cousins': 19390, 'catalina': 19391, 'perpetuate': 19392, 'ingestion': 19393, 'excludes': 19394, 'haiman': 19395, 'netzarim': 19396, 'morag': 19397, 'ateret': 19398, 'unamid': 19399, 'listens': 19400, 'jurists': 19401, 'arbitrarily': 19402, 'fayssal': 19403, 'mekdad': 19404, 'veracity': 19405, 'tikriti': 19406, 'disc': 19407, '690': 19408, '551': 19409, 'balkh': 19410, 'mihtarlam': 19411, 'openings': 19412, 'stringers': 19413, 'rockford': 19414, 'fasteners': 19415, 'outweighs': 19416, 'creditor': 19417, 'dwindled': 19418, 'dilma': 19419, 'rousseff': 19420, 'confine': 19421, 'souvenir': 19422, 'remitted': 19423, 'khurasan': 19424, 'merv': 19425, 'boon': 19426, 'plaintiff': 19427, 'gurbanguly': 19428, 'accumulating': 19429, 'heathens': 19430, 'peking': 19431, 'translate': 19432, 'pang': 19433, 'devils': 19434, 'barbarity': 19435, 'incensed': 19436, 'wager': 19437, 'janitor': 19438, 'organist': 19439, 'pews': 19440, 'conakry': 19441, 'squeezed': 19442, "o'keefe": 19443, 'incredibly': 19444, 'celestial': 19445, 'astronomical': 19446, 'overrule': 19447, 'mulgueta': 19448, 'debalk': 19449, 'newcastle': 19450, 'drownings': 19451, 'unknowns': 19452, 'doves': 19453, 'origami': 19454, 'folded': 19455, 'occassion': 19456, 'picnics': 19457, 'cemeteries': 19458, 'xian': 19459, 'han': 19460, 'wuhan': 19461, 'yakaghund': 19462, 'wheelchairs': 19463, 'extricate': 19464, 'bilingual': 19465, 'ashti': 19466, 'dangtu': 19467, 'identical': 19468, 'stampa': 19469, 'parisi': 19470, 'trajectory': 19471, 'miliant': 19472, 'iskandariya': 19473, 'graduated': 19474, 'sulaymaniyeh': 19475, 'emerli': 19476, 'maref': 19477, 'mirpur': 19478, 'sacramento': 19479, 'scurrying': 19480, 'conditioners': 19481, 'mahmood': 19482, 'developmentaly': 19483, 'moghaddasi': 19484, '640': 19485, 'stockpiled': 19486, 'michaelle': 19487, 'adrienne': 19488, 'turnover': 19489, 'awacs': 19490, 'margraten': 19491, 'erased': 19492, 'vermilion': 19493, 'kohat': 19494, 'mizuki': 19495, 'tomiaki': 19496, 'marathoner': 19497, 'radcliffe': 19498, 'lancaster': 19499, 'tzachi': 19500, 'hanegbi': 19501, 'anarchist': 19502, 'kaesong': 19503, 'drum': 19504, 'bugle': 19505, 'pageant': 19506, 'twilight': 19507, 'feuer': 19508, 'entrapment': 19509, 'plaza': 19510, 'encircled': 19511, 'revava': 19512, 'demarcating': 19513, 'abyei': 19514, 'kashmirs': 19515, 'saffir': 19516, 'viewership': 19517, 'musicalphenomenon': 19518, 'zac': 19519, 'efron': 19520, 'tisdale': 19521, 'spawning': 19522, 'defeatism': 19523, 'mockery': 19524, 'higüey': 19525, 'faxas': 19526, 'quand': 19527, 'quoc': 19528, 'kabardino': 19529, 'balkaria': 19530, 'exploiters': 19531, 'exponential': 19532, 'tehrik': 19533, 'hadithah': 19534, 'blitz': 19535, 'natonski': 19536, 'adamantios': 19537, 'vassilakis': 19538, 'recommitted': 19539, 'skeptics': 19540, 'hatta': 19541, 'radjasa': 19542, 'microsystems': 19543, 'beitar': 19544, 'illit': 19545, 'efrat': 19546, 'marcelo': 19547, 'antezana': 19548, 'disposing': 19549, 'cheated': 19550, 'user': 19551, 'castle': 19552, 'sleet': 19553, 'bout': 19554, 'decimate': 19555, 'dhusamareb': 19556, 'marergur': 19557, 'waljama': 19558, 'strewn': 19559, 'jeny': 19560, 'frias': 19561, 'attachés': 19562, 'evangelicals': 19563, 'apprehend': 19564, 'mainz': 19565, 'wis': 19566, 'deere': 19567, 'omanis': 19568, 'hafiz': 19569, "ba'th": 19570, 'alawite': 19571, 'jarome': 19572, 'iginla': 19573, 'dany': 19574, 'heatley': 19575, 'doan': 19576, "da'ra": 19577, 'legalization': 19578, 'quelling': 19579, 'tjarnqvist': 19580, 'henrik': 19581, 'sedin': 19582, 'hemispheres': 19583, 'aragonite': 19584, 'sands': 19585, 'mechanic': 19586, 'imprudent': 19587, 'imprudence': 19588, 'thoughtful': 19589, 'vicissitudes': 19590, 'nap': 19591, 'cosily': 19592, 'slumber': 19593, 'barked': 19594, 'muttering': 19595, 'betsy': 19596, 'gallop': 19597, 'jinan': 19598, 'pillar': 19599, 'caliph': 19600, 'caliphate': 19601, 'lobbies': 19602, 'alireza': 19603, 'jamshidi': 19604, 'soheil': 19605, 'farshad': 19606, 'scout': 19607, 'bastani': 19608, 'ghalawiya': 19609, 'sleeper': 19610, 'isselmou': 19611, 'liberate': 19612, "o'hare": 19613, 'lago': 19614, 'agrio': 19615, 'valiant': 19616, 'prd': 19617, '598': 19618, 'gores': 19619, '673': 19620, 'melchior': 19621, 'ndadaye': 19622, 'kalenga': 19623, 'ramadhani': 19624, 'analyzed': 19625, 'telephoning': 19626, 'maverick': 19627, 'embarrass': 19628, 'nabaie': 19629, 'inspects': 19630, 'desolation': 19631, 'currents': 19632, 'dianne': 19633, 'sawyer': 19634, 'dune': 19635, 'sainct': 19636, 'thessaloniki': 19637, 'kyprianou': 19638, 'wanthana': 19639, 'jared': 19640, 'cotter': 19641, 'sloan': 19642, 'vie': 19643, 'jumper': 19644, 'mitterndorf': 19645, 'faultless': 19646, '207': 19647, '762': 19648, 'morgenstern': 19649, '752': 19650, 'planica': 19651, 'overpayments': 19652, 'unlocked': 19653, 'footlocker': 19654, 'gambled': 19655, 'baylesa': 19656, 'pricey': 19657, '4th': 19658, 'avoiding': 19659, 'briskly': 19660, 'ballad': 19661, 'pharrell': 19662, 'downloads': 19663, 'themed': 19664, 'dakhil': 19665, 'yazidis': 19666, 'gentry': 19667, '1772': 19668, '279': 19669, 'comparatively': 19670, 'burundian': 19671, 'onyango': 19672, 'omollo': 19673, 'ochami': 19674, 'marzieh': 19675, 'dastjerdi': 19676, 'underclass': 19677, 'indulge': 19678, 'seeduzzaman': 19679, 'elahi': 19680, 'bakhsh': 19681, 'soomro': 19682, 'fakhar': 19683, 'dignitary': 19684, 'undated': 19685, 'krzanich': 19686, 'chi': 19687, 'minh': 19688, 'evaluates': 19689, 'presenter': 19690, 'ohn': 19691, 'bo': 19692, 'zin': 19693, 'khun': 19694, 'sai': 19695, 'nib': 19696, 'bused': 19697, 'communiqué': 19698, 'halemi': 19699, 'pathology': 19700, 'autopsies': 19701, 'fukusho': 19702, 'shinobu': 19703, 'hasegawa': 19704, 'disillusioned': 19705, 'zonen': 19706, 'rodney': 19707, 'melville': 19708, 'ganey': 19709, 'firimbi': 19710, '111th': 19711, 'rendell': 19712, 'daxing': 19713, 'meizhou': 19714, 'domineering': 19715, 'whittaker': 19716, 'notting': 19717, '306': 19718, '415': 19719, 'underperforming': 19720, 'basotho': 19721, 'mineworkers': 19722, 'canning': 19723, 'herding': 19724, 'drawback': 19725, '362': 19726, 'precipitously': 19727, 'sinchulu': 19728, 'ceding': 19729, 'whereby': 19730, 'krone': 19731, 'singye': 19732, 'khesar': 19733, 'namgyel': 19734, 'thimphu': 19735, 'loosening': 19736, 'venturing': 19737, 'moulting': 19738, 'strutted': 19739, 'cheat': 19740, 'striding': 19741, 'pecked': 19742, 'plucked': 19743, 'plumes': 19744, 'jays': 19745, 'behaviour': 19746, 'seagull': 19747, 'gullet': 19748, 'spendthrift': 19749, 'pawned': 19750, 'cloak': 19751, 'solemnly': 19752, 'bougainville': 19753, 'oily': 19754, 'skimmers': 19755, 'hookup': 19756, '1890s': 19757, 'pensacola': 19758, 'leased': 19759, 'gushing': 19760, '322': 19761, 'dues': 19762, 'guesthouse': 19763, 'tegua': 19764, 'manifestation': 19765, 'executes': 19766, 'stamped': 19767, 'newscaster': 19768, 'malfeasance': 19769, 'allan': 19770, 'kemakeza': 19771, 'reestablishing': 19772, 'ramsi': 19773, 'fossum': 19774, 'garan': 19775, 'akihiko': 19776, 'shower': 19777, 'foliage': 19778, 'despoiling': 19779, 'denuded': 19780, 'deceive': 19781, 'democratizing': 19782, 'bossaso': 19783, 'vaunting': 19784, 'fearlessness': 19785, 'pained': 19786, 'silvestre': 19787, 'afable': 19788, 'enterprising': 19789, 'fearless': 19790, 'modernizes': 19791, 'schearf': 19792, 'reasoned': 19793, 'swicord': 19794, 'signatories': 19795, 'rui': 19796, 'zhu': 19797, 'houze': 19798, 'pu': 19799, 'endeavoured': 19800, 'lundestad': 19801, '168': 19802, 'roswell': 19803, 'anh': 19804, 'tay': 19805, 'duong': 19806, 'pondicherry': 19807, 'rails': 19808, 'mehrabpur': 19809, 'welded': 19810, 'darkness': 19811, 'meterologists': 19812, 'roaring': 19813, 'spyglass': 19814, 'outdo': 19815, 'triumphs': 19816, 'icahn': 19817, 'plavia': 19818, 'levar': 19819, "jam'iyyat": 19820, 'saheeh': 19821, 'samana': 19822, 'latina': 19823, 'scapegoating': 19824, 'criminalizes': 19825, 'alienating': 19826, 'flocking': 19827, 'facelift': 19828, 'modernity': 19829, 'cubanization': 19830, 'amhed': 19831, 'osbek': 19832, 'castillo': 19833, 'diamondbacks': 19834, 'francisely': 19835, 'bueno': 19836, 'braves': 19837, 'ilyas': 19838, 'repressed': 19839, 'hernan': 19840, 'khetaguri': 19841, 'inguri': 19842, 'jaw': 19843, 'extensively': 19844, 'signings': 19845, 'betrayal': 19846, 'definite': 19847, 'shana': 19848, 'garhi': 19849, 'ransacking': 19850, 'bula': 19851, 'hawo': 19852, 'photographic': 19853, 'baran': 19854, 'illusion': 19855, 'helmet': 19856, 'hoisting': 19857, 'aftab': 19858, 'sherpao': 19859, 'lodi': 19860, 'zazai': 19861, 'muniz': 19862, 'maywand': 19863, 'hosada': 19864, 'mobbed': 19865, 'butts': 19866, 'canes': 19867, 'oncologist': 19868, 'meddles': 19869, 'interferes': 19870, 'suppresses': 19871, 'fathers': 19872, 'judith': 19873, 'latham': 19874, 'dateline': 19875, 'isro': 19876, 'madhavan': 19877, 'nair': 19878, 'array': 19879, 'salvation': 19880, 'sayidat': 19881, 'nejat': 19882, 'mcguffin': 19883, 'harun': 19884, 'kushayb': 19885, 'uzair': 19886, 'solicited': 19887, 'ecolog': 19888, 'summon': 19889, 'mustaqbal': 19890, 'liuguantun': 19891, 'tangshan': 19892, 'helland': 19893, 'meadows': 19894, 'stanilas': 19895, 'warwrinka': 19896, 'gulbis': 19897, 'spellings': 19898, 'compilation': 19899, 'banco': 19900, 'championed': 19901, 'zabi': 19902, "gm's": 19903, 'nanhai': 19904, 'bombardier': 19905, 'wiretapped': 19906, 'semana': 19907, 'pilar': 19908, 'thugs': 19909, 'shengyou': 19910, 'feigning': 19911, 'oda': 19912, 'hok': 19913, 'buro': 19914, 'happold': 19915, 'aquatics': 19916, '476': 19917, 'balfour': 19918, 'beatty': 19919, 'reconfigured': 19920, 'chattisgarh': 19921, 'rocco': 19922, 'buttiglione': 19923, 'sin': 19924, '472': 19925, 'delisted': 19926, 'duluth': 19927, 'ga': 19928, 'adept': 19929, 'landholders': 19930, 'jubilee': 19931, 'unmasking': 19932, 'macro': 19933, 'unrwa': 19934, 'astrology': 19935, 'underestimate': 19936, 'handicapped': 19937, 'astute': 19938, 'esteem': 19939, 'mortals': 19940, 'messenger': 19941, 'fling': 19942, 'journeying': 19943, 'loosen': 19944, 'faggot': 19945, 'anticipations': 19946, 'strand': 19947, 'pursuer': 19948, 'recollecting': 19949, 'tumultous': 19950, 'heartless': 19951, 'skipper': 19952, "'t": 19953, 'convergent': 19954, 'murmured': 19955, 'marooned': 19956, 'shareman': 19957, 'hauls': 19958, 'pudong': 19959, 'deportees': 19960, 'qatada': 19961, 'lerner': 19962, 'sufa': 19963, 'nahal': 19964, 'antihistamine': 19965, 'heredity': 19966, 'batter': 19967, 'vero': 19968, 'southward': 19969, 'ticketing': 19970, 'rong': 19971, 'utilized': 19972, 'oversold': 19973, 'blackhawk': 19974, 'etienne': 19975, 'tshisekedi': 19976, 'weakness': 19977, 'coahuila': 19978, 'charai': 19979, 'persists': 19980, 'tacked': 19981, 'additions': 19982, 'earmarks': 19983, 'gauteng': 19984, 'surprises': 19985, 'manama': 19986, 'dialog': 19987, 'frederic': 19988, 'piry': 19989, 'kwazulu': 19990, 'hajim': 19991, 'mento': 19992, 'msimang': 19993, 'blacked': 19994, 'underscoring': 19995, 'ghassan': 19996, 'daglas': 19997, 'blazing': 19998, 'retaliating': 19999, 'caravan': 20000, 'hardcore': 20001, 'conscious': 20002, 'hayabullah': 20003, 'rafiqi': 20004, 'qabail': 20005, 'solders': 20006, 'repsol': 20007, 'norma': 20008, 'nonspecific': 20009, 'uncorroborated': 20010, 'hermann': 20011, 'apollo': 20012, 'ohno': 20013, 'skates': 20014, 'pechstein': 20015, 'anni': 20016, 'friesinger': 20017, 'armin': 20018, 'zoeggeler': 20019, 'resolves': 20020, 'sciri': 20021, 'echoes': 20022, 'norinco': 20023, 'zibo': 20024, 'chemet': 20025, 'hongdu': 20026, 'ounion': 20027, 'metallurgy': 20028, 'walt': 20029, '660': 20030, 'nonrefundable': 20031, 'tutor': 20032, 'iger': 20033, 'pixar': 20034, 'marvel': 20035, 'harvey': 20036, 'bloodthirsty': 20037, 'hated': 20038, 'monster': 20039, 'mccellan': 20040, '740': 20041, 'receving': 20042, 'ecuadoreans': 20043, 'tachilek': 20044, 'noel': 20045, 'imb': 20046, 'gronholm': 20047, 'immunizing': 20048, 'duaik': 20049, 'mitsubishi': 20050, 'gigi': 20051, 'galli': 20052, 'turbo': 20053, 'charger': 20054, 'valves': 20055, 'maracaibo': 20056, 'gazan': 20057, 'constituted': 20058, 'guilt': 20059, 'bickering': 20060, 'monoply': 20061, 'xp': 20062, 'lado': 20063, 'grigol': 20064, 'mgalobishvili': 20065, 'markko': 20066, 'technocrat': 20067, 'newhouse': 20068, 'sezibera': 20069, 'qassim': 20070, 'branco': 20071, 'scathing': 20072, 'giliani': 20073, 'commerical': 20074, 'traumatized': 20075, 'untreated': 20076, 'adwa': 20077, 'wlodzimierz': 20078, 'odzimierz': 20079, 'leopoldo': 20080, 'taiana': 20081, 'dhiren': 20082, 'qaisar': 20083, 'shaffi': 20084, 'nadeem': 20085, 'tarmohammed': 20086, 'esa': 20087, 'citicorp': 20088, 'graffiti': 20089, 'turner': 20090, 'durand': 20091, 'strikers': 20092, 'noses': 20093, 'stomachs': 20094, 'lauder': 20095, 'schneider': 20096, 'inforadio': 20097, 'oswaldo': 20098, 'jarrin': 20099, 'unaffiliated': 20100, 'datapoint': 20101, 'finfish': 20102, 'instabilities': 20103, 'martinique': 20104, 'mayotte': 20105, 'overpopulated': 20106, 'inefficiently': 20107, 'fy09': 20108, 'fy10': 20109, 'pulses': 20110, 'mw': 20111, 'grasping': 20112, 'idf': 20113, 'strelets': 20114, 'anatoliy': 20115, 'theoretically': 20116, 'tishrin': 20117, 'sincerity': 20118, 'zhouqu': 20119, 'watchers': 20120, 'jammed': 20121, 'gymnasium': 20122, 'jianlian': 20123, 'fouled': 20124, 'narvaez': 20125, 'locker': 20126, 'wore': 20127, "dvd's": 20128, 'sola': 20129, 'chilitepic': 20130, 'medan': 20131, 'tahseen': 20132, 'poul': 20133, 'nielsen': 20134, 'farabaugh': 20135, 'reebok': 20136, 'nord': 20137, 'eclair': 20138, 'gobernador': 20139, 'valadares': 20140, 'biloxi': 20141, 'expletive': 20142, 'bashkortostan': 20143, 'oskar': 20144, 'alekseyeva': 20145, 'rewriting': 20146, 'andrade': 20147, 'gereida': 20148, 'formalizing': 20149, 'totalitarian': 20150, 'pinchuk': 20151, 'guilin': 20152, 'wolong': 20153, 'nestrenko': 20154, 'paseo': 20155, 'reforma': 20156, 'vasconcelos': 20157, 'nadi': 20158, 'tahab': 20159, 'snarled': 20160, 'inception': 20161, 'congestion': 20162, 'conjunction': 20163, 'simulated': 20164, 'tarin': 20165, 'purim': 20166, 'galloway': 20167, 'barter': 20168, 'disrepair': 20169, 'denpasar': 20170, 'czugaj': 20171, 'stephens': 20172, 'renae': 20173, 'lambasting': 20174, 'adoring': 20175, 'divisiveness': 20176, 'canister': 20177, 'sparingly': 20178, '236': 20179, 'domenici': 20180, 'reap': 20181, 'vetoed': 20182, 'est': 20183, '576': 20184, 'barron': 20185, 'distributions': 20186, 'gini': 20187, 'coefficient': 20188, 'allotments': 20189, 'germanic': 20190, '1866': 20191, 'practiced': 20192, 'supranational': 20193, 'annals': 20194, 'overarching': 20195, 'yemenis': 20196, 'delimitation': 20197, 'huthi': 20198, 'zaydi': 20199, 'revitalized': 20200, "sana'a": 20201, 'sprain': 20202, 'racquetball': 20203, 'loosed': 20204, 'coil': 20205, 'irritated': 20206, 'rustic': 20207, 'ignorant': 20208, 'induce': 20209, 'mused': 20210, 'ilyushin': 20211, 'rosoboronexport': 20212, 'nightline': 20213, 'balboa': 20214, 'embarking': 20215, '232nd': 20216, 'risking': 20217, 'juste': 20218, 'moradi': 20219, 'avoidance': 20220, 'yoshimasa': 20221, 'hayashi': 20222, 'jinping': 20223, 'undervalued': 20224, '2nd': 20225, '502nd': 20226, 'scholarships': 20227, 'specter': 20228, 'contradicted': 20229, 'nsanje': 20230, 'pepfar': 20231, 'maroua': 20232, 'rania': 20233, 'joyous': 20234, 'eni': 20235, 'wintry': 20236, 'dictates': 20237, 'ivanovo': 20238, 'teikovo': 20239, 'countering': 20240, 'macarthur': 20241, 'parcels': 20242, 'zahraa': 20243, 'etman': 20244, 'compel': 20245, 'exuberant': 20246, 'guys': 20247, 'brides': 20248, 'chanthalangsy': 20249, 'sawang': 20250, 'frigid': 20251, 'dikweneh': 20252, 'ambulances': 20253, 'paddick': 20254, 'russell': 20255, 'madaen': 20256, 'intermediaries': 20257, 'kuwaitis': 20258, 'mutairi': 20259, 'bloemfontein': 20260, 'lieu': 20261, 'conjured': 20262, 'gasparovic': 20263, 'wholesalers': 20264, 'koufax': 20265, 'wilpon': 20266, 'causality': 20267, 'baladiyat': 20268, 'shlomo': 20269, 'mor': 20270, 'anesthesia': 20271, 'dosage': 20272, 'sedated': 20273, 'unconsciousness': 20274, 'vase': 20275, 'sutham': 20276, 'saengprathum': 20277, 'kelantan': 20278, 'polytechnic': 20279, 'backcountry': 20280, 'babri': 20281, 'rama': 20282, 'shiv': 20283, 'sena': 20284, '784': 20285, 'heishan': 20286, 'seyoum': 20287, 'mesfin': 20288, 'awan': 20289, 'cayenne': 20290, 'khanun': 20291, 'talim': 20292, 'disfigured': 20293, 'mats': 20294, 'talal': 20295, 'sattar': 20296, 'suha': 20297, 'dordain': 20298, 'shoigu': 20299, 'foul': 20300, 'interruption': 20301, 'outed': 20302, 'sad': 20303, 'revelation': 20304, 'defiled': 20305, 'diligence': 20306, 'choco': 20307, 'wines': 20308, '00e': 20309, 'airasia': 20310, 'gomhouria': 20311, 'ralia': 20312, 'thrived': 20313, '1030': 20314, 'cranks': 20315, 'thayer': 20316, 'kadhim': 20317, 'defacate': 20318, 'finely': 20319, 'armchairs': 20320, 'punitive': 20321, 'theorize': 20322, 'verbytsky': 20323, 'sandbag': 20324, 'winfield': 20325, 'inundate': 20326, 'embankments': 20327, 'ruining': 20328, 'soybeans': 20329, 'skyrocketed': 20330, 'royalists': 20331, 'sahafi': 20332, 'liquidation': 20333, 'dividends': 20334, 'redeemed': 20335, '1652': 20336, 'spice': 20337, 'trekked': 20338, 'subjugation': 20339, 'encroachments': 20340, 'boer': 20341, 'afrikaners': 20342, 'kgalema': 20343, 'motlanthe': 20344, 'promarket': 20345, 'narcotrafficking': 20346, 'delimited': 20347, 'tokugawa': 20348, 'shogunate': 20349, 'flowering': 20350, 'kanagawa': 20351, 'industrialize': 20352, 'formosa': 20353, '1931': 20354, 'manchuria': 20355, 'honshu': 20356, 'saibou': 20357, 'madhuri': 20358, 'duration': 20359, 'sahel': 20360, 'nigerien': 20361, 'growling': 20362, 'snapping': 20363, 'wrathful': 20364, 'tyrannical': 20365, 'gentle': 20366, 'panther': 20367, 'amity': 20368, 'coils': 20369, 'spat': 20370, 'slake': 20371, 'draught': 20372, 'juror': 20373, 'housecat': 20374, 'furred': 20375, 'unimaginably': 20376, 'lurking': 20377, 'yitzhak': 20378, 'leah': 20379, 'herzl': 20380, 'defaced': 20381, 'beilin': 20382, 'gurion': 20383, 'graveyard': 20384, 'sadah': 20385, 'shivnarine': 20386, '214': 20387, 'makhaya': 20388, 'cn': 20389, 'bucca': 20390, 'biographies': 20391, 'miscommunication': 20392, 'offloaded': 20393, 'cetacean': 20394, 'cabeza': 20395, 'vaca': 20396, 'shoko': 20397, 'muallem': 20398, 'sheiria': 20399, 'riad': 20400, 'dovonou': 20401, 'adjarra': 20402, 'porto': 20403, 'scheussel': 20404, 'pelting': 20405, 'incapacitating': 20406, 'poors': 20407, 'paused': 20408, 'streetcar': 20409, 'departs': 20410, 'tecnica': 20411, 'loja': 20412, 'universidad': 20413, 'andes': 20414, '068472222': 20415, 'nike': 20416, 'downhills': 20417, '882': 20418, '592': 20419, 'presses': 20420, 'jensen': 20421, 'phuket': 20422, 'yazoo': 20423, 'incomplete': 20424, 'anup': 20425, 'raj': 20426, 'jabril': 20427, 'abdulle': 20428, 'mauritian': 20429, 'grouper': 20430, 'eels': 20431, 'chaka': 20432, 'fattah': 20433, 'fills': 20434, 'gunfights': 20435, 'copacabana': 20436, 'ipanema': 20437, 'legalize': 20438, 'shen': 20439, 'gregg': 20440, 'jawid': 20441, 'tichaona': 20442, 'traitors': 20443, 'withered': 20444, 'helpe': 20445, 'chasseurs': 20446, 'volontaires': 20447, 'domingue': 20448, '1779': 20449, 'turb': 20450, 'ulent': 20451, 'passy': 20452, '2035': 20453, 'sahrawi': 20454, 'bart': 20455, 'stupak': 20456, 'grammyawards': 20457, 'domm': 20458, 'samo': 20459, 'parra': 20460, 'mientes': 20461, 'dejarte': 20462, 'amar': 20463, 'guerraas': 20464, 'bachata': 20465, 'fukuoko': 20466, 'univision': 20467, 'judgmental': 20468, 'starlet': 20469, 'richie': 20470, 'councilor': 20471, 'jiaxuan': 20472, 'lehman': 20473, 'ubs': 20474, 'stearns': 20475, 'renowed': 20476, 'farka': 20477, 'genre': 20478, 'timbuktu': 20479, 'belet': 20480, 'weyn': 20481, 'compartment': 20482, 'latifiya': 20483, 'hafidh': 20484, 'deferring': 20485, 'amani': 20486, 'wreaking': 20487, 'laissez': 20488, 'faire': 20489, 'archaic': 20490, 'entrepot': 20491, 'ballooning': 20492, 'conditioned': 20493, 'mayen': 20494, 'accrued': 20495, 'kwanza': 20496, 'depreciated': 20497, 'fishery': 20498, 'rutile': 20499, 'protectorates': 20500, 'malaya': 20501, 'sarawak': 20502, 'armourer': 20503, 'dart': 20504, 'fangs': 20505, 'insensible': 20506, 'salesmen': 20507, 'dina': 20508, 'cody': 20509, 'slumps': 20510, 'oceania': 20511, 'incidences': 20512, 'forcible': 20513, 'mabhouh': 20514, 'circulate': 20515, 'deyda': 20516, 'berezovsky': 20517, 'akhmed': 20518, 'zakayev': 20519, 'greenest': 20520, 'soothe': 20521, 'h9n2': 20522, 'subtypes': 20523, 'mansehra': 20524, 'emerges': 20525, 'lots': 20526, 'rubies': 20527, 'auctions': 20528, 'nishin': 20529, 'asphyxiated': 20530, 'salvatore': 20531, 'sagues': 20532, 'prosecutions': 20533, 'kandill': 20534, 'faultline': 20535, 'chenembiri': 20536, 'bhunu': 20537, 'ranged': 20538, 'obiang': 20539, 'nguema': 20540, 'unspoiled': 20541, 'maciej': 20542, 'nowicki': 20543, 'rospuda': 20544, 'pristine': 20545, 'bog': 20546, 'lynx': 20547, 'augustow': 20548, 'fayoum': 20549, 'michalak': 20550, 'thuan': 20551, 'clouded': 20552, 'harass': 20553, 'hofstad': 20554, 'nansan': 20555, 'brader': 20556, 'soothing': 20557, 'teng': 20558, 'perpetrating': 20559, 'erkki': 20560, 'tuomioja': 20561, 'haisori': 20562, 'destabilization': 20563, 'rugigana': 20564, 'faustin': 20565, 'kayumba': 20566, 'develops': 20567, 'izzat': 20568, 'douri': 20569, 'ration': 20570, 'cosmonaut': 20571, 'grujic': 20572, 'decadence': 20573, 'aspire': 20574, 'perfection': 20575, 'oberhausen': 20576, 'legged': 20577, 'mussels': 20578, 'peacemaker': 20579, 'reconstructing': 20580, 'wayaobao': 20581, 'zichang': 20582, 'howells': 20583, '277': 20584, 'kantathi': 20585, 'supamongkhon': 20586, 'spicy': 20587, 'garlicky': 20588, 'epitomizes': 20589, 'alzouma': 20590, 'yada': 20591, 'adamou': 20592, 'cuvette': 20593, 'heath': 20594, 'anura': 20595, 'bandaranaike': 20596, 'amunugama': 20597, 'mediocre': 20598, 'peril': 20599, 'isaiah': 20600, 'unpublished': 20601, 'authored': 20602, '294': 20603, 'surpasses': 20604, '2927': 20605, 'polarize': 20606, 'interlagos': 20607, '060092593': 20608, '050474537': 20609, 'kimi': 20610, 'realistically': 20611, 'schumacher': 20612, 'quails': 20613, 'prolific': 20614, 'functional': 20615, 'joao': 20616, 'billboard': 20617, 'vocals': 20618, 'lamm': 20619, 'loughnane': 20620, 'sandagiri': 20621, 'malam': 20622, 'bacai': 20623, 'toddler': 20624, 'yushu': 20625, 'guangrong': 20626, 'kamau': 20627, 'artur': 20628, 'marina': 20629, 'otalora': 20630, 'condoleeza': 20631, 'ludicrous': 20632, 'barricaded': 20633, 'shangla': 20634, 'sarfraz': 20635, 'naeemi': 20636, 'kingsbridge': 20637, 'donuts': 20638, 'dunkin': 20639, '468': 20640, 'randolph': 20641, 'casinos': 20642, 'cepa': 20643, 'pataca': 20644, 'riskier': 20645, 'revisions': 20646, '1889': 20647, '586': 20648, 'euphausia': 20649, 'superba': 20650, '027': 20651, 'dissostichus': 20652, 'eleginoides': 20653, 'bass': 20654, '910': 20655, '591': 20656, '396': 20657, 'ccamlr': 20658, 'unregulated': 20659, '799': 20660, 'iaato': 20661, 'sideline': 20662, 'uniosil': 20663, 'furthering': 20664, 'fatherland': 20665, 'wintertime': 20666, 'curled': 20667, 'tee': 20668, 'olden': 20669, 'enchanted': 20670, 'imitate': 20671, 'dull': 20672, 'industrious': 20673, 'poetry': 20674, 'honoured': 20675, 'compiler': 20676, 'volumes': 20677, 'tabulated': 20678, 'lustily': 20679, 'behold': 20680, 'goeth': 20681, 'calamitously': 20682, 'prowl': 20683, 'dwell': 20684, 'whichever': 20685, 'quarrelling': 20686, 'pep': 20687, 'redirect': 20688, 'groomed': 20689, 'sim': 20690, 'unicom': 20691, '628': 20692, 'westerly': 20693, 'siegler': 20694, 'fouls': 20695, 'nrc': 20696, 'handelsblad': 20697, 'henk': 20698, 'forested': 20699, 'lays': 20700, 'orlando': 20701, 'cachaito': 20702, 'buena': 20703, 'compay': 20704, 'segundo': 20705, 'nurja': 20706, 'euthanized': 20707, 'pets': 20708, 'cares': 20709, 'hindawi': 20710, 'duluiya': 20711, 'machinea': 20712, 'jaatanni': 20713, 'taadhii': 20714, 'upright': 20715, 'gyroscopes': 20716, 'tricky': 20717, 'scooters': 20718, 'warrantless': 20719, 'mascots': 20720, 'floribert': 20721, 'integrationist': 20722, 'precedence': 20723, 'rigoberta': 20724, 'menchu': 20725, 'jokers': 20726, 'forcers': 20727, 'contradicting': 20728, 'cheats': 20729, 'dhia': 20730, 'assimilating': 20731, 'visually': 20732, 'ordina': 20733, 'den': 20734, 'taping': 20735, 'klara': 20736, 'ljube': 20737, 'ljubotno': 20738, 'skopje': 20739, 'radisson': 20740, 'militarization': 20741, 'telecast': 20742, 'weaponization': 20743, 'singnaghi': 20744, 'tugboats': 20745, 'mavi': 20746, 'marmaraout': 20747, 'neuilly': 20748, 'marja': 20749, 'jalani': 20750, 'kish': 20751, 'duffle': 20752, 'ashwell': 20753, 'dwayne': 20754, 'jerome': 20755, 'germ': 20756, 'rihab': 20757, 'huda': 20758, 'amash': 20759, 'marwa': 20760, 'brookings': 20761, 'camaraderie': 20762, 'outdoors': 20763, 'monaliza': 20764, 'noormohammadi': 20765, 'porous': 20766, 'thunderous': 20767, 'uthmani': 20768, 'funnel': 20769, 'luciano': 20770, 'modena': 20771, 'polyclinic': 20772, 'pancreatic': 20773, 'aficionados': 20774, 'tenor': 20775, 'minghe': 20776, 'salad': 20777, 'crooner': 20778, 'shrugged': 20779, 'suichuan': 20780, 'mala': 20781, 'shorish': 20782, 'akre': 20783, 'bijeel': 20784, 'shaikan': 20785, 'rovi': 20786, 'sarta': 20787, 'dihok': 20788, 'chimneys': 20789, 'embarrased': 20790, 'halftime': 20791, 'performer': 20792, 'jacksonville': 20793, 'mccarthy': 20794, 'facets': 20795, 'contenders': 20796, 'baya': 20797, 'rahho': 20798, 'archeologist': 20799, 'energize': 20800, 'verifiably': 20801, 'pontchartrain': 20802, 'megumi': 20803, 'faking': 20804, 'mocks': 20805, 'hamesh': 20806, 'koreb': 20807, 'trawler': 20808, 'hmawbi': 20809, 'gracious': 20810, 'bijbehera': 20811, 'amor': 20812, 'almagro': 20813, 'buyat': 20814, 'aridi': 20815, 'bugojno': 20816, 'twa': 20817, 'stethem': 20818, 'lovers': 20819, '410': 20820, 'guinness': 20821, 'yerevan': 20822, '587': 20823, 'elah': 20824, 'dufour': 20825, 'novi': 20826, 'alessandria': 20827, 'piemonte': 20828, 'stamford': 20829, 'magnified': 20830, 'nonrecurring': 20831, 'valuation': 20832, 'geodynamic': 20833, 'kos': 20834, 'astypaleia': 20835, 'excels': 20836, 'sufficiency': 20837, 'contractions': 20838, 'khmers': 20839, 'angkor': 20840, 'cham': 20841, '1887': 20842, 'pot': 20843, 'normalcy': 20844, 'contending': 20845, 'sihanouk': 20846, 'sihamoni': 20847, 'ageing': 20848, 'fy06': 20849, 'earns': 20850, 'penning': 20851, 'antagonist': 20852, 'leisurely': 20853, 'sauntering': 20854, 'cheer': 20855, 'schemed': 20856, 'tailless': 20857, 'brush': 20858, 'spun': 20859, 'shahab': 20860, 'salma': 20861, 'valentina': 20862, 'paloma': 20863, 'ventanazul': 20864, 'goldwyn': 20865, 'ppr': 20866, 'labels': 20867, 'gucci': 20868, 'balenciaga': 20869, 'puma': 20870, '159th': 20871, 'paynesville': 20872, 'congotown': 20873, 'needlessly': 20874, 'antagonizes': 20875, 'uncalculated': 20876, 'melih': 20877, 'gokcek': 20878, 'drapchi': 20879, 'otton': 20880, '187': 20881, 'heraldo': 20882, 'munoz': 20883, 'imphal': 20884, 'okram': 20885, 'ibobi': 20886, '282': 20887, 'hussey': 20888, 'lbw': 20889, 'meng': 20890, 'diuretic': 20891, 'hua': 20892, 'swimmer': 20893, 'ouyang': 20894, 'kunpeng': 20895, '281': 20896, 'paced': 20897, '267': 20898, 'authorites': 20899, 'outlaws': 20900, 'bulava': 20901, 'stavropol': 20902, 'delanoe': 20903, 'coe': 20904, 'suite': 20905, 'yimou': 20906, 'perina': 20907, 'artibonite': 20908, 'refocus': 20909, 'azimbek': 20910, 'beknazarov': 20911, 'nepotism': 20912, 'adolescence': 20913, 'adolescents': 20914, 'reciprocate': 20915, 'verifiable': 20916, 'yichang': 20917, 'wanzhou': 20918, 'downpours': 20919, 'mujhava': 20920, 'commonplace': 20921, 'reshape': 20922, 'broadening': 20923, 'ojedokun': 20924, 'screeners': 20925, 'baggage': 20926, 'glut': 20927, 'unsold': 20928, 'giovanni': 20929, 'fava': 20930, 'lawmakes': 20931, 'overdrafts': 20932, 'kallenberger': 20933, 'bellinger': 20934, 'ammonium': 20935, 'anfo': 20936, 'deactivate': 20937, 'backups': 20938, 'maccabi': 20939, 'betar': 20940, 'dinamo': 20941, 'nikolas': 20942, 'clichy': 20943, 'businesswomen': 20944, 'memento': 20945, 'sherpa': 20946, 'unfurled': 20947, 'rededicate': 20948, 'commemorated': 20949, 'fundraisers': 20950, 'itinerary': 20951, 'thamilselvan': 20952, 'vidar': 20953, 'gagra': 20954, 'subzero': 20955, 'slippery': 20956, 'motels': 20957, 'exclusion': 20958, 'haruna': 20959, 'idrissu': 20960, 'softened': 20961, 'adopts': 20962, 'jaua': 20963, 'speculating': 20964, 'paralyzing': 20965, 'tri': 20966, 'daklak': 20967, 'suicidal': 20968, 'allots': 20969, 'immorality': 20970, 'repealed': 20971, 'shoichi': 20972, 'nakagawa': 20973, 'penn': 20974, 'roundly': 20975, 'testifies': 20976, 'reimbursed': 20977, 'amortization': 20978, 'lingered': 20979, 'precipitated': 20980, '1623': 20981, 'rebelled': 20982, 'sekou': 20983, 'sekouba': 20984, 'konate': 20985, 'conde': 20986, 'nafta': 20987, 'absorbs': 20988, 'buffeted': 20989, 'capitalization': 20990, 'stuffing': 20991, 'quills': 20992, 'aux': 20993, 'dames': 20994, 'wearily': 20995, 'wallets': 20996, 'etc': 20997, 'plaster': 20998, 'noticeable': 20999, 'confidently': 21000, 'rowdy': 21001, 'busied': 21002, 'flap': 21003, 'stapler': 21004, 'stapled': 21005, 'debilitating': 21006, 'addendum': 21007, 'debriefing': 21008, 'mekorot': 21009, 'bursa': 21010, 'compatriots': 21011, 'modifies': 21012, 'enhances': 21013, 'detriment': 21014, 'ishac': 21015, 'diwan': 21016, 'qusai': 21017, 'wahab': 21018, 'bahrainian': 21019, 'satisfies': 21020, 'verhofstadt': 21021, 'sol': 21022, 'tuzla': 21023, 'gijs': 21024, 'vries': 21025, 'muriel': 21026, 'degauque': 21027, 'anyama': 21028, 'gendarmes': 21029, 'newman': 21030, 'flagrantly': 21031, 'semester': 21032, 'tulane': 21033, 'trailers': 21034, 'moinuddin': 21035, 'puri': 21036, 'affirming': 21037, 'fayyum': 21038, 'giza': 21039, 'filip': 21040, 'tkachev': 21041, 'krasnodar': 21042, 'beatrice': 21043, 'norton': 21044, 'toby': 21045, 'harnden': 21046, 'simmonds': 21047, 'workings': 21048, 'vetoes': 21049, 'sorting': 21050, 'sabotaging': 21051, 'schmit': 21052, 'zabayda': 21053, 'chitchai': 21054, 'wannasathit': 21055, 'durango': 21056, 'resembled': 21057, 'incendiary': 21058, 'pardo': 21059, 'sharjee': 21060, 'frangiskos': 21061, 'ragoussis': 21062, 'castoff': 21063, 'cinderella': 21064, 'dreamgirls': 21065, 'miro': 21066, 'yousifiyah': 21067, 'simplifying': 21068, 'ler': 21069, 'minesweepers': 21070, 'airdrops': 21071, 'karkh': 21072, 'ogero': 21073, 'compromised': 21074, 'regaining': 21075, 'ping': 21076, 'kun': 21077, 'ghangzhou': 21078, 'yat': 21079, 'nangjing': 21080, 'slideshow': 21081, 'sgt': 21082, 'mounts': 21083, '74th': 21084, 'researching': 21085, 'malwiya': 21086, 'jagged': 21087, 'abbassid': 21088, 'alps': 21089, 'closeness': 21090, 'studded': 21091, 'multitudes': 21092, 'transfusion': 21093, 'vincennes': 21094, 'rockers': 21095, 'lynyrd': 21096, 'skynyrd': 21097, 'butch': 21098, 'kievenaar': 21099, 'blondie': 21100, 'trumpeter': 21101, 'herb': 21102, 'alpert': 21103, 'moss': 21104, 'founders': 21105, 'lafayette': 21106, 'novosibirsk': 21107, 'stymied': 21108, 'inspires': 21109, 'hubris': 21110, 'anonymously': 21111, 'festive': 21112, 'decked': 21113, 'zhanjun': 21114, 'accredited': 21115, 'binjie': 21116, 'embraer': 21117, 'ramping': 21118, "sh'ite": 21119, 'huaren': 21120, 'simmons': 21121, 'fayyaz': 21122, 'cobbled': 21123, 'mortgages': 21124, 'refinancing': 21125, 'customized': 21126, 'simulates': 21127, 'juliana': 21128, 'weisgan': 21129, 'bahamian': 21130, 'nyasaland': 21131, 'hastings': 21132, 'kamuzu': 21133, 'sprat': 21134, 'umpire': 21135, 'orchard': 21136, 'ripening': 21137, 'quench': 21138, 'paces': 21139, 'tempting': 21140, 'despise': 21141, 'butlers': 21142, 'chauffeurs': 21143, 'backfired': 21144, 'obscene': 21145, 'tourniquet': 21146, 'noticing': 21147, 'slyly': 21148, 'fractions': 21149, 'andrea': 21150, 'nahles': 21151, 'kajo': 21152, 'wasserhoevel': 21153, 'solbes': 21154, 'constructing': 21155, 'firehouses': 21156, 'mm': 21157, 'wesson': 21158, 'visitor': 21159, 'unfastened': 21160, 'journeyed': 21161, 'sergey': 21162, 'tiffany': 21163, 'stiegler': 21164, 'rebecca': 21165, 'naturalization': 21166, 'tanith': 21167, 'belbin': 21168, 'shagh': 21169, 'initialed': 21170, 'seydou': 21171, 'diarra': 21172, 'thundershowers': 21173, 'rounder': 21174, 'nel': 21175, 'boeta': 21176, 'dippenaar': 21177, 'wai': 21178, 'stylized': 21179, 'sci': 21180, 'fi': 21181, '2047': 21182, 'bernando': 21183, 'vavuniya': 21184, 'bogdanchikov': 21185, 'allegra': 21186, 'anorexia': 21187, 'zimmerman': 21188, 'estanged': 21189, 'correspond': 21190, 'inject': 21191, 'otri': 21192, 'pramoni': 21193, 'frechette': 21194, 'plaque': 21195, '616': 21196, 'delighted': 21197, 'holing': 21198, 'sohail': 21199, 'tanvir': 21200, 'dhoni': 21201, 'waw': 21202, 'barge': 21203, 'trudging': 21204, 'jogged': 21205, 'biked': 21206, 'emilie': 21207, 'loit': 21208, 'ruano': 21209, 'bleach': 21210, 'cereals': 21211, 'smerdon': 21212, 'mrksic': 21213, 'radic': 21214, 'veselin': 21215, 'sljivancanin': 21216, '534': 21217, 'reforestation': 21218, 'robby': 21219, 'yeu': 21220, 'tzuoo': 21221, 'neurosurgeon': 21222, 'nihon': 21223, 'keizai': 21224, 'pioneered': 21225, 'economical': 21226, 'gwangju': 21227, 'tomislav': 21228, 'nikolic': 21229, 'qua': 21230, 'subspecies': 21231, 'reinhold': 21232, 'rau': 21233, 'pakitka': 21234, 'thrust': 21235, 'submitting': 21236, 'diocese': 21237, 'wash': 21238, 'liturgy': 21239, 'disapproved': 21240, 'clamping': 21241, 'rightists': 21242, 'coaltion': 21243, 'ceramic': 21244, 'murugupillai': 21245, 'jenita': 21246, 'jeyarajah': 21247, 'crested': 21248, 'galam': 21249, 'playground': 21250, 'aviaries': 21251, 'ghanem': 21252, 'deflecting': 21253, 'nuria': 21254, 'llagostera': 21255, 'vives': 21256, 'aryanto': 21257, 'boedihardjo': 21258, 'aip': 21259, 'hidayat': 21260, 'ciamis': 21261, 'regency': 21262, 'salik': 21263, 'firdaus': 21264, 'misno': 21265, 'lauro': 21266, 'savors': 21267, 'martinis': 21268, 'chef': 21269, 'rep': 21270, 'freer': 21271, '558': 21272, 'clotting': 21273, 'defendents': 21274, 'zubeidi': 21275, 'hasbrouk': 21276, 'debenture': 21277, '158': 21278, '666': 21279, 'zalkin': 21280, '608': 21281, '413': 21282, '967': 21283, '809': 21284, 'muscovy': 21285, 'absorb': 21286, 'romanov': 21287, '1682': 21288, '1725': 21289, 'hegemony': 21290, 'defeats': 21291, 'lenin': 21292, 'iosif': 21293, 'glasnost': 21294, 'perestroika': 21295, 'buttressed': 21296, 'seaports': 21297, 'dislocation': 21298, 'barbuda': 21299, 'spencer': 21300, 'antiguan': 21301, 'yawning': 21302, 'disadvantages': 21303, 'abject': 21304, 'equaling': 21305, 'gardening': 21306, 'reengagement': 21307, 'mis': 21308, 'outlays': 21309, 'coconut': 21310, 'pitied': 21311, 'bough': 21312, 'fowling': 21313, 'lambs': 21314, 'pupil': 21315, 'lookout': 21316, 'famishing': 21317, 'babe': 21318, 'sneered': 21319, "i'd": 21320, 'compliment': 21321, 'riek': 21322, 'homestead': 21323, 'nyongwa': 21324, 'ankunda': 21325, 'remarked': 21326, 'weakly': 21327, 'jolt': 21328, 'fy08': 21329, 'tartus': 21330, 'decimated': 21331, 'doodipora': 21332, 'azad': 21333, 'baqer': 21334, 'constitutions': 21335, 'ecumenical': 21336, 'chrysostom': 21337, 'nazianzen': 21338, '1054': 21339, 'sacking': 21340, '1204': 21341, 'salome': 21342, 'zurabishvili': 21343, 'inforamtion': 21344, 'institutionalizing': 21345, 'squibb': 21346, 'sustiva': 21347, 'viread': 21348, 'emtriva': 21349, 'replication': 21350, 'malaki': 21351, 'hiker': 21352, 'untied': 21353, 'bittersweet': 21354, 'concertacion': 21355, 'foxley': 21356, '1824': 21357, 'notari': 21358, 'involuntarily': 21359, 'tubes': 21360, 'murals': 21361, 'excavation': 21362, 'mummies': 21363, 'scribe': 21364, 'motorized': 21365, 'rickshaw': 21366, 'hello': 21367, 'collectivization': 21368, 'prodemocracy': 21369, 'chronicled': 21370, 'skated': 21371, 'moallem': 21372, 'appalled': 21373, 'barbarism': 21374, 'composition': 21375, 'docks': 21376, 'banbury': 21377, 'lenghty': 21378, 'hayam': 21379, 'zahri': 21380, 'borroto': 21381, 'contemplating': 21382, 'glittering': 21383, 'gemelli': 21384, 'inflammation': 21385, 'minya': 21386, 'unsanitary': 21387, 'simulation': 21388, 'followup': 21389, 'solidifies': 21390, 'oft': 21391, "o'er": 21392, 'descending': 21393, 'mick': 21394, 'jagger': 21395, 'oprah': 21396, 'winfrey': 21397, 'golfer': 21398, 'consitutional': 21399, 'spearker': 21400, 'reversible': 21401, 'minimally': 21402, 'embolization': 21403, 'hysterectomy': 21404, 'shrinks': 21405, 'rowed': 21406, 'fibroids': 21407, 'alimport': 21408, 'qilla': 21409, 'arrogance': 21410, 'inga': 21411, 'marchand': 21412, 'spitting': 21413, 'counseling': 21414, 'manicurists': 21415, 'slightest': 21416, 'plotter': 21417, 'reassessed': 21418, '384': 21419, 'maligned': 21420, 'supremacy': 21421, 'bereavement': 21422, 'haswa': 21423, 'jib': 21424, 'dial': 21425, 'stefan': 21426, 'mellar': 21427, 'deniers': 21428, 'hikmet': 21429, '°c': 21430, 'mashonaland': 21431, 'godfrey': 21432, 'dzvairo': 21433, 'itai': 21434, 'marchi': 21435, 'karidza': 21436, 'tendai': 21437, 'matambanadzo': 21438, 'naad': 21439, 'mangwana': 21440, 'krygyz': 21441, 'dubrovka': 21442, 'haden': 21443, 'maclellan': 21444, 'surrey': 21445, 'feniger': 21446, 'xenophobic': 21447, 'combative': 21448, 'lsi': 21449, 'sp': 21450, 'wallachia': 21451, 'moldavia': 21452, 'suzerainty': 21453, '1859': 21454, '1862': 21455, 'transylvania': 21456, 'axis': 21457, 'soviets': 21458, 'abdication': 21459, 'nicolae': 21460, 'securitate': 21461, 'concessional': 21462, 'rescheduling': 21463, '1876': 21464, 'tsarist': 21465, 'akaev': 21466, 'otunbaeva': 21467, 'interethnic': 21468, 'lark': 21469, 'uninterred': 21470, 'crest': 21471, 'hillock': 21472, 'monstrous': 21473, 'refrained': 21474, 'posthumous': 21475, 'deft': 21476, 'degenerative': 21477, 'bowie': 21478, 'merle': 21479, 'haggard': 21480, 'jessye': 21481, 'chinooks': 21482, 'rotor': 21483, 'wadajir': 21484, 'hodan': 21485, 'reconciling': 21486, 'seacoast': 21487, 'ezzedin': 21488, 'smoldering': 21489, 'parched': 21490, 'smog': 21491, 'sonthi': 21492, 'boonyaratglin': 21493, 'shaima': 21494, 'char': 21495, 'bowing': 21496, 'bombard': 21497, 'booked': 21498, 'fingerprinted': 21499, 'shrunk': 21500, 'layoff': 21501, 'citi': 21502, 'walks': 21503, 'waning': 21504, 'naha': 21505, 'mint': 21506, 'mouknass': 21507, 'brig': 21508, 'sexy': 21509, 'pertains': 21510, 'nasty': 21511, 'fsg': 21512, 'spessart': 21513, 'rheinland': 21514, 'pfalz': 21515, 'strutting': 21516, 'catwalk': 21517, 'mellon': 21518, 'siddiqi': 21519, 'beechcraft': 21520, 'zhulyany': 21521, 'intoning': 21522, 'mica': 21523, 'adde': 21524, 'slovakian': 21525, 'zsolt': 21526, 'grebe': 21527, 'peregrine': 21528, 'gabcikovo': 21529, 'upsets': 21530, 'kohlschreiber': 21531, 'transformer': 21532, 'kayettuli': 21533, 'gloomier': 21534, 'haris': 21535, 'ehsanul': 21536, 'sadequee': 21537, 'positioning': 21538, 'bheri': 21539, 'surkhet': 21540, 'takirambudde': 21541, 'firefighting': 21542, 'carpentry': 21543, 'kuomintang': 21544, 'hsiao': 21545, 'khim': 21546, 'bombmaking': 21547, 'perwiz': 21548, 'reexamine': 21549, 'stipulated': 21550, 'baribari': 21551, 'alhurra': 21552, 'repel': 21553, 'percussionist': 21554, 'conga': 21555, 'dizzy': 21556, 'gillespie': 21557, 'puente': 21558, 'salsa': 21559, 'celia': 21560, 'hopeless': 21561, 'refloating': 21562, 'refloat': 21563, 'settings': 21564, 'mythical': 21565, 'winding': 21566, 'borg': 21567, 'risky': 21568, 'farris': 21569, 'redrawing': 21570, 'separates': 21571, 'pierced': 21572, 'attributes': 21573, 'sahab': 21574, 'europrean': 21575, 'renate': 21576, 'kuenast': 21577, 'kuhn': 21578, 'batasuna': 21579, 'qinglin': 21580, 'mersin': 21581, 'counterkidnapping': 21582, 'thatcher': 21583, 'pathe': 21584, 'productions': 21585, 'dj': 21586, 'frears': 21587, 'mirren': 21588, 'outbid': 21589, 'ratner': 21590, 'sweetened': 21591, 'acceptances': 21592, 'rupee': 21593, 'overvalued': 21594, 'amortizing': 21595, 'eff': 21596, 'erm2': 21597, 'bounce': 21598, 'supplanted': 21599, 'overstaffing': 21600, 'mortgaged': 21601, 'specializes': 21602, 'conformity': 21603, '245': 21604, 'qamdo': 21605, 'bern': 21606, 'prized': 21607, 'behest': 21608, 'mururoa': 21609, 'microenterprises': 21610, 'buffetings': 21611, 'reproaches': 21612, 'calmness': 21613, 'fury': 21614, 'issawiya': 21615, 'criminalizing': 21616, 'tainting': 21617, 'erich': 21618, 'circumventing': 21619, 'rumbek': 21620, 'fidler': 21621, 'cutler': 21622, 'tynychbek': 21623, 'transferable': 21624, 'tula': 21625, 'escravos': 21626, 'entitlements': 21627, 'chookiat': 21628, 'ophaswongse': 21629, 'grosjean': 21630, 'danai': 21631, 'udomchoke': 21632, 'oudsema': 21633, 'scan': 21634, 'proclaim': 21635, 'accordingly': 21636, 'purification': 21637, 'thirteenth': 21638, 'jailings': 21639, 'batted': 21640, 'shaharyar': 21641, 'moveon': 21642, 'inzamam': 21643, 'mina': 21644, 'jamarat': 21645, 'grueling': 21646, 'bodied': 21647, 'communion': 21648, 'wasit': 21649, 'mwesige': 21650, 'shaka': 21651, 'ssali': 21652, 'sutalinov': 21653, 'objectionable': 21654, 'cancelation': 21655, 'herman': 21656, 'rompuy': 21657, 'edinburgh': 21658, 'chhattisgarh': 21659, 'raipur': 21660, 'jharkhand': 21661, 'fratto': 21662, 'indices': 21663, 'dehui': 21664, 'tulsi': 21665, 'giri': 21666, 'mostafavi': 21667, 'jude': 21668, 'genes': 21669, 'proteins': 21670, 'josefa': 21671, 'baeza': 21672, 'aston': 21673, 'mulally': 21674, 'khamis': 21675, 'dahab': 21676, 'sachin': 21677, 'kartik': 21678, 'tallied': 21679, 'brar': 21680, 'shamsbari': 21681, 'wreaked': 21682, 'blockage': 21683, 'sidakan': 21684, 'rex': 21685, 'walheim': 21686, 'orbital': 21687, 'outing': 21688, 'crewmates': 21689, 'leopold': 21690, 'eyharts': 21691, 'plundered': 21692, 'handsets': 21693, 'umpires': 21694, 'hashimzai': 21695, 'obeyed': 21696, 'nsc': 21697, 'selecting': 21698, 'explicit': 21699, 'theatergoers': 21700, 'suspense': 21701, 'disturbiaunseated': 21702, 'peeping': 21703, 'labeouf': 21704, 'morse': 21705, 'dreamworks': 21706, 'distributor': 21707, 'lebeouf': 21708, 'transformers': 21709, 'hualan': 21710, 'satan': 21711, 'interwoven': 21712, 'salif': 21713, 'ousamane': 21714, 'ngom': 21715, 'taraba': 21716, 'suspends': 21717, 'encampment': 21718, 'afflicting': 21719, 'imperative': 21720, 'oblivious': 21721, 'destitute': 21722, 'huts': 21723, 'supplemental': 21724, 'recounted': 21725, 'blueberry': 21726, 'erasmus': 21727, 'avenging': 21728, '246': 21729, 'oleksiy': 21730, 'ivchenko': 21731, 'ukrainy': 21732, 'predrag': 21733, "shi'te": 21734, 'dulles': 21735, 'omission': 21736, 'rostock': 21737, 'heiligendamm': 21738, 'rostok': 21739, 'gargash': 21740, 'sift': 21741, 'displaying': 21742, 'passages': 21743, 'bara': 21744, 'nuys': 21745, 'calif': 21746, 'realestate': 21747, 'fluctuation': 21748, '38th': 21749, 'punctuated': 21750, 'bartolomeo': 21751, '1784': 21752, 'gustavia': 21753, 'repurchased': 21754, 'appellations': 21755, 'populace': 21756, 'collectivity': 21757, 'hereditary': 21758, 'premiers': 21759, 'promulgation': 21760, 'plurality': 21761, 'overruled': 21762, 'gridlock': 21763, 'jhala': 21764, 'khanal': 21765, 'indentured': 21766, 'ethnocultural': 21767, 'cheddi': 21768, 'bharrat': 21769, 'jagdeo': 21770, 'rhodesia': 21771, '1923': 21772, 'spratly': 21773, 'overlaps': 21774, 'reef': 21775, 'vucelic': 21776, 'mira': 21777, 'markovic': 21778, 'remembrances': 21779, 'trauma': 21780, 'blockbuster': 21781, 'thermopylae': 21782, 'copied': 21783, 'amnesties': 21784, 'absolve': 21785, 'watchful': 21786, 'ziyang': 21787, 'magnolia': 21788, 'fatmir': 21789, 'sejdiu': 21790, 'waseem': 21791, 'sohrab': 21792, 'goth': 21793, 'cartographers': 21794, 'haziri': 21795, 'ethic': 21796, 'puntland': 21797, 'elyor': 21798, 'ganiyev': 21799, 'alvarezes': 21800, '261': 21801, 'kwanzaa': 21802, 'wintery': 21803, 'qader': 21804, 'jassim': 21805, 'sherwan': 21806, 'gaulle': 21807, 'qarabaugh': 21808, 'klerk': 21809, 'jafaari': 21810, 'commend': 21811, 'torshin': 21812, 'shepel': 21813, 'disapproves': 21814, 'gimble': 21815, 'papa': 21816, 'mariann': 21817, 'boel': 21818, 'zhenchuan': 21819, 'breakers': 21820, 'hurling': 21821, "n'guesso": 21822, 'permissible': 21823, 'gvero': 21824, 'mocny': 21825, 'accosted': 21826, 'idrac': 21827, 'sustains': 21828, 'landfills': 21829, 'afeworki': 21830, 'rethink': 21831, 'organic': 21832, 'cookies': 21833, 'nasariyah': 21834, 'lattes': 21835, 'untitled': 21836, 'cobos': 21837, 'schudrich': 21838, 'scrolls': 21839, 'rabinnical': 21840, 'merbau': 21841, 'undisturbed': 21842, 'admhaiyah': 21843, 'momen': 21844, 'ye': 21845, 'heliodoro': 21846, 'reclassify': 21847, 'bloating': 21848, 'dot': 21849, 'riverbanks': 21850, 'profiteers': 21851, 'essentials': 21852, 'lecturers': 21853, 'knu': 21854, 'ghazaliyah': 21855, 'policing': 21856, 'hovers': 21857, 'chequers': 21858, 'kalamazoo': 21859, 'mich': 21860, 'maumoon': 21861, 'embark': 21862, 'badme': 21863, 'eebc': 21864, 'immersed': 21865, 'advent': 21866, 'expended': 21867, 'heel': 21868, 'mitigating': 21869, 'radicova': 21870, 'agonies': 21871, 'fiercer': 21872, 'unto': 21873, 'gasping': 21874, 'grudges': 21875, 'tusks': 21876, 'growled': 21877, 'cowards': 21878, 'majesty': 21879, 'marry': 21880, 'proliferating': 21881, 'ichiro': 21882, 'audiotapes': 21883, 'doug': 21884, 'pathologically': 21885, 'liar': 21886, '179': 21887, '343': 21888, 'csongrad': 21889, '196': 21890, 'abnormally': 21891, 'ratnayke': 21892, 'bruised': 21893, 'cosmopolitan': 21894, 'multiculturalism': 21895, 'etihad': 21896, 'bastille': 21897, 'inalienable': 21898, 'instructor': 21899, 'basij': 21900, 'vigilantes': 21901, 'azov': 21902, 'volganeft': 21903, 'sulfur': 21904, 'cary': 21905, 'porpoises': 21906, 'tuneup': 21907, 'fallouj': 21908, 'petrodar': 21909, 'conglomerate': 21910, 'arbab': 21911, 'basir': 21912, 'rajah': 21913, 'zamboanga': 21914, 'inlets': 21915, 'fumes': 21916, 'petrechemical': 21917, 'interpreting': 21918, 'taitung': 21919, 'plowing': 21920, 'guardedly': 21921, 'grips': 21922, 'regrettably': 21923, 'channeled': 21924, 'culls': 21925, '555': 21926, 'wisely': 21927, 'krugman': 21928, 'trichet': 21929, 'boao': 21930, 'mowaffaq': 21931, 'rubaie': 21932, 'formulas': 21933, 'phillips': 21934, 'battleground': 21935, 'sinaloa': 21936, 'emitted': 21937, 'nosedive': 21938, 'creators': 21939, '890': 21940, 'popov': 21941, 'poncet': 21942, 'barcode': 21943, 'clerks': 21944, 'barcoded': 21945, 'chewing': 21946, 'invalidated': 21947, 'redoing': 21948, 'nails': 21949, 'jam': 21950, 'refiling': 21951, 'sacrilegious': 21952, 'valdivia': 21953, 'ultrasound': 21954, 'unicameral': 21955, 'verkhovna': 21956, 'rada': 21957, 'immobility': 21958, 'nycz': 21959, 'dreaded': 21960, 'uthai': 21961, 'burying': 21962, 'petrovka': 21963, 'bakyt': 21964, 'seitov': 21965, 'ramechhap': 21966, 'trailed': 21967, 'schiavone': 21968, 'kasparov': 21969, 'kasyanov': 21970, 'magnet': 21971, 'disparate': 21972, 'transcends': 21973, 'superseded': 21974, 'blond': 21975, 'haired': 21976, 'telephones': 21977, 'jokhang': 21978, 'gongga': 21979, 'rabdhure': 21980, 'inflate': 21981, 'finalists': 21982, 'stockholders': 21983, 'ranger': 21984, 'avon': 21985, 'glitches': 21986, 'patch': 21987, 'craze': 21988, 'moheli': 21989, 'fomboni': 21990, 'rotates': 21991, 'sambi': 21992, 'bacar': 21993, 'anjouanais': 21994, 'comoran': 21995, 'curia': 21996, 'mementos': 21997, 'countercoups': 21998, 'widest': 21999, 'empower': 22000, 'lowlands': 22001, 'entrant': 22002, 'wavered': 22003, 'tallinn': 22004, 'forefront': 22005, 'outperformed': 22006, 'notch': 22007, 'boediono': 22008, 'continuity': 22009, 'peatlands': 22010, 'trailblazing': 22011, 'redd': 22012, 'suppose': 22013, 'err': 22014, 'testament': 22015, 'interred': 22016, 'terrorizing': 22017, 'morally': 22018, 'commute': 22019, 'basing': 22020, 'grigory': 22021, 'lighthouses': 22022, 'bachelor': 22023, 'rewarded': 22024, '93rd': 22025, 'redirected': 22026, 'redirecting': 22027, 'hack': 22028, 'drummond': 22029, 'firecracker': 22030, 'pallipat': 22031, 'sweets': 22032, 'snacks': 22033, 'lamps': 22034, '518': 22035, '346': 22036, 'unverified': 22037, 'rizkar': 22038, 'pawn': 22039, 'steadfast': 22040, 'covenant': 22041, 'deposited': 22042, 'byelection': 22043, 'disbarred': 22044, 'rollout': 22045, 'hennes': 22046, 'mauritz': 22047, 'ab': 22048, 'purses': 22049, 'risque': 22050, 'conical': 22051, 'bras': 22052, 'fellowships': 22053, 'orphanages': 22054, 'recalls': 22055, 'reliability': 22056, 'honda': 22057, 'sniffing': 22058, 'sherry': 22059, 'fining': 22060, 'classification': 22061, 'goddess': 22062, 'beijings': 22063, 'hacksaws': 22064, 'transports': 22065, 'muse': 22066, '620': 22067, '777': 22068, 'kochi': 22069, 'baziv': 22070, 'slauta': 22071, 'kharkiv': 22072, 'yevhen': 22073, 'kushnaryov': 22074, 'rousing': 22075, 'ryzhkov': 22076, 'yevgeny': 22077, 'primakov': 22078, 'phan': 22079, 'khai': 22080, 'tran': 22081, 'giustra': 22082, 'osterholm': 22083, 'katsuya': 22084, 'okada': 22085, 'naoto': 22086, 'kan': 22087, 'laurence': 22088, 'boring': 22089, 'literally': 22090, 'feather': 22091, 'boas': 22092, 'connick': 22093, 'chao': 22094, 'congratuatlions': 22095, 'posht': 22096, 'rud': 22097, 'artemisinin': 22098, 'bala': 22099, 'boluk': 22100, 'midestern': 22101, 'newport': 22102, 'hydroxide': 22103, 'reverses': 22104, 'definitely': 22105, 'falciparum': 22106, 'plastered': 22107, "ba'athists": 22108, 'sui': 22109, 'nawab': 22110, 'maliky': 22111, 'jaghato': 22112, 'nahim': 22113, 'malawian': 22114, 'willy': 22115, 'mwaluka': 22116, 'artisans': 22117, 'hotspots': 22118, 'adijon': 22119, 'warrior': 22120, 'tendering': 22121, 'taaf': 22122, 'archipelagos': 22123, 'crozet': 22124, 'fauna': 22125, 'adelie': 22126, 'slice': 22127, "mutharika's": 22128, 'exhibited': 22129, 'goodall': 22130, 'gondwe': 22131, 'oresund': 22132, 'bosporus': 22133, 'seaway': 22134, 'jomo': 22135, 'toroitich': 22136, 'multiethnic': 22137, 'rainbow': 22138, 'uhuru': 22139, 'conciliatory': 22140, 'odm': 22141, 'powersharing': 22142, 'eliminates': 22143, 'willis': 22144, 'islets': 22145, 'beacons': 22146, 'gladly': 22147, 'traitor': 22148, 'nay': 22149, 'wallow': 22150, 'converged': 22151, 'owning': 22152, 'jeungsan': 22153, 'dhi': 22154, 'nautical': 22155, 'listen': 22156, '7115': 22157, '9885': 22158, '11705': 22159, '11725': 22160, 'voanews': 22161, 'depressing': 22162, 'argentinean': 22163, 'angie': 22164, 'sanclemente': 22165, 'valenica': 22166, 'airat': 22167, 'hae': 22168, 'panmunjom': 22169, 'meadowbrook': 22170, 'ozlam': 22171, 'sanabel': 22172, 'obsessed': 22173, 'alexeyenko': 22174, 'ordnance': 22175, 'swiergosz': 22176, 'vaguely': 22177, 'fortinet': 22178, 'irawaddy': 22179, 'valery': 22180, 'sitnikov': 22181, 'enacts': 22182, 'fitzpatrick': 22183, 'habbaniya': 22184, 'mandates': 22185, 'airlifts': 22186, 'benghazi': 22187, 'heightening': 22188, 'thae': 22189, 'bok': 22190, 'mandarin': 22191, 'tuo': 22192, 'bpa': 22193, 'ilhami': 22194, 'gafir': 22195, 'abdelkader': 22196, 'loyalties': 22197, 'nimnim': 22198, 'grieves': 22199, 'talangama': 22200, 'shuai': 22201, 'stosur': 22202, 'arthurs': 22203, '1850s': 22204, 'disrespectful': 22205, 'dissipated': 22206, 'tookie': 22207, 'inspirational': 22208, 'playmaker': 22209, 'wrecking': 22210, 'fulayfill': 22211, 'geyer': 22212, 'cavalry': 22213, 'jeb': 22214, 'rené': 22215, 'sanderson': 22216, 'rony': 22217, 'trooper': 22218, 'sese': 22219, 'seko': 22220, 'shajoy': 22221, 'jesper': 22222, 'helsoe': 22223, 'feyzabad': 22224, 'yazdi': 22225, 'mahendranagar': 22226, 'bager': 22227, 'uspi': 22228, 'guardians': 22229, 'lntelligence': 22230, 'altcantara': 22231, 'vsv': 22232, 'voto': 22233, 'bernales': 22234, 'likening': 22235, 'gear': 22236, 'practitioners': 22237, 'tarnish': 22238, 'detractors': 22239, 'wishers': 22240, 'paralympics': 22241, 'statisticians': 22242, 'gymnasiums': 22243, 'chrome': 22244, 'oversized': 22245, 'velocity': 22246, '575': 22247, 'streaked': 22248, '515': 22249, '581': 22250, 'levitates': 22251, 'alstom': 22252, 'horsepower': 22253, '786': 22254, 'alleviation': 22255, 'citibank': 22256, 'revelations': 22257, 'mayo': 22258, 'environments': 22259, '\x91': 22260, '\x92': 22261, 'decathlon': 22262, 'limping': 22263, 'mccloud': 22264, 'ani': 22265, 'lectures': 22266, 'trevor': 22267, 'jianchao': 22268, 'lounderma': 22269, 'unnerve': 22270, 'hanun': 22271, 'spoiled': 22272, 'explanations': 22273, 'cristobal': 22274, 'casas': 22275, 'uribana': 22276, 'panzhihua': 22277, 'tit': 22278, 'tat': 22279, 'gaspard': 22280, 'bulldozing': 22281, 'nyange': 22282, 'schooled': 22283, '492': 22284, 'cutthroat': 22285, '1767': 22286, '1790': 22287, 'outmigration': 22288, '233': 22289, 'cemented': 22290, 'melanesian': 22291, 'melanesians': 22292, 'fijian': 22293, 'laisenia': 22294, 'commodore': 22295, 'voreqe': 22296, 'influxes': 22297, 'sprang': 22298, 'snatch': 22299, 'brawny': 22300, 'refrigerator': 22301, 'doorbell': 22302, 'sibaie': 22303, 'moustafa': 22304, 'yafei': 22305, 'condone': 22306, 'amil': 22307, 'adamiya': 22308, 'villa': 22309, 'andré': 22310, 'nesnera': 22311, 'korea\x92s': 22312, 'pectoral': 22313, 'booed': 22314, 'domachowska': 22315, 'berms': 22316, 'surfboard': 22317, 'emphasize': 22318, 'swells': 22319, 'posses': 22320, 'gholamhossein': 22321, 'convenient': 22322, 'theoretical': 22323, '6000': 22324, 'daimler': 22325, 'desi': 22326, 'varanasi': 22327, 'meerut': 22328, 'endeavourhas': 22329, 'undocked': 22330, 'exterior': 22331, 'goodbyes': 22332, 'hatches': 22333, 'recycling': 22334, 'expectant': 22335, 'umbilical': 22336, 'suna': 22337, 'afewerki': 22338, 'ontario': 22339, 'coastguard': 22340, 'spyros': 22341, 'kristina': 22342, 'marit': 22343, 'bjoergen': 22344, 'hilde': 22345, 'pedersen': 22346, 'genders': 22347, 'speedskating': 22348, 'ebrahim': 22349, 'supersedes': 22350, 'defer': 22351, 'textbook': 22352, 'overlooks': 22353, 'calculation': 22354, 'characterization': 22355, 'cynical': 22356, '880': 22357, 'azzedine': 22358, 'belkadi': 22359, 'kerimli': 22360, 'pflp': 22361, 'saadat': 22362, 'rehavam': 22363, 'zeevi': 22364, 'splattered': 22365, 'pettigrew': 22366, 'bowling': 22367, 'rolando': 22368, 'otoniel': 22369, 'guevara': 22370, 'samahdna': 22371, 'runup': 22372, 'libertador': 22373, "o'higgins": 22374, 'valparaiso': 22375, 'intelcenter': 22376, 'heeding': 22377, 'energized': 22378, 'kiraitu': 22379, 'payday': 22380, 'divine': 22381, 'summed': 22382, 'barbecues': 22383, 'bulandshahr': 22384, 'leil': 22385, 'atheists': 22386, 'lambeth': 22387, 'misappropriation': 22388, 'veterinarian': 22389, 'veils': 22390, 'khori': 22391, 'lauberhorn': 22392, 'alleys': 22393, 'bib': 22394, '589': 22395, 'rittipakdee': 22396, 'unvetted': 22397, 'gorges': 22398, 'yuanmu': 22399, 'overpopulation': 22400, 'delegitimize': 22401, 'amrullah': 22402, 'militaristic': 22403, 'motoyasu': 22404, 'candlelit': 22405, 'lantern': 22406, 'itahari': 22407, 'astonished': 22408, 'hongyao': 22409, 'arafura': 22410, 'merauke': 22411, 'headcount': 22412, 'gelles': 22413, 'wertheim': 22414, 'schroder': 22415, '875': 22416, 'suitors': 22417, 'igad': 22418, 'trimmed': 22419, 'kubilius': 22420, 'cutback': 22421, 'eskom': 22422, 'necessitating': 22423, 'empowerment': 22424, 'guano': 22425, 'navassa': 22426, 'rested': 22427, 'panamanians': 22428, '1811': 22429, 'lowland': 22430, 'stroessner': 22431, 'omra': 22432, 'norsemen': 22433, 'boru': 22434, '1014': 22435, 'repressions': 22436, 'andrews': 22437, 'trotted': 22438, 'scudded': 22439, 'optimist': 22440, 'pessimist': 22441, '857': 22442, '772': 22443, 'loder': 22444, 'crusader': 22445, 'misdemeanor': 22446, 'sobriety': 22447, 'misdmeanor': 22448, 'keifer': 22449, 'metropolis': 22450, 'baishiyao': 22451, 'feminism': 22452, 'psychology': 22453, 'housewife': 22454, 'unfulfilled': 22455, 'abdouramane': 22456, 'conviasa': 22457, 'sidor': 22458, 'margarita': 22459, 'defections': 22460, 'portraits': 22461, 'yvon': 22462, 'neptune': 22463, 'jocelerme': 22464, 'privert': 22465, 'protestors': 22466, 'naryn': 22467, 'pour': 22468, 'onshore': 22469, 'changbei': 22470, 'petrochina': 22471, 'unreleased': 22472, 'pneumonic': 22473, '2500': 22474, 'tawilla': 22475, 'widens': 22476, 'trimester': 22477, 'incest': 22478, 'addington': 22479, 'hannah': 22480, 'canaries': 22481, 'utor': 22482, 'whipping': 22483, 'huddled': 22484, 'sludge': 22485, 'walayo': 22486, 'hepatitis': 22487, 'cerda': 22488, 'disappearances': 22489, 'belkhadem': 22490, 'ugalde': 22491, 'magistrates': 22492, 'milky': 22493, 'magellanic': 22494, 'fragmentary': 22495, 'parnaz': 22496, 'haleh': 22497, 'esfandiari': 22498, 'woodrow': 22499, 'kian': 22500, 'tajbakhsh': 22501, 'malibu': 22502, 'concentrating': 22503, 'ohlmert': 22504, 'qasr': 22505, 'uncomplicated': 22506, 'patu': 22507, 'cull': 22508, 'extolling': 22509, 'diyar': 22510, 'nourished': 22511, 'sonoma': 22512, 'receded': 22513, 'rained': 22514, 'shieh': 22515, 'jhy': 22516, 'wey': 22517, 'cheng': 22518, 'shu': 22519, 'ezzedine': 22520, 'contemplate': 22521, 'aggravate': 22522, 'idriz': 22523, 'balaj': 22524, 'lahi': 22525, 'brahimaj': 22526, 'pune': 22527, '503': 22528, '816': 22529, 'misquoted': 22530, 'magnitudes': 22531, 'tidjane': 22532, 'thiam': 22533, 'exiting': 22534, 'recife': 22535, 'gabgbo': 22536, 'sculpture': 22537, 'gadgets': 22538, 'matteo': 22539, 'duran': 22540, 'walder': 22541, 'brendan': 22542, 'kosuke': 22543, 'kitajima': 22544, 'omaha': 22545, 'medley': 22546, 'katie': 22547, 'hoff': 22548, 'freestyle': 22549, 'regents': 22550, 'innovations': 22551, 'themba': 22552, 'demirbag': 22553, 'forgave': 22554, 'bette': 22555, 'caesar': 22556, 'bawdy': 22557, 'meglen': 22558, 'probable': 22559, 'insiders': 22560, 'cher': 22561, '545': 22562, 'trumpets': 22563, 'reduces': 22564, 'ambient': 22565, 'racers': 22566, 'experimenting': 22567, 'underage': 22568, 'underfed': 22569, 'jockey': 22570, 'johnny': 22571, 'unpremeditated': 22572, 'garbage': 22573, 'akhrorkhodzha': 22574, 'tolipkhodzhayev': 22575, 'offline': 22576, 'statesmen': 22577, 'tearfully': 22578, 'doaba': 22579, 'hangu': 22580, 'klaus': 22581, 'toepfer': 22582, 'riverside': 22583, 'profiles': 22584, '377': 22585, 'mushtaq': 22586, 'bechuanaland': 22587, 'preserves': 22588, '1895': 22589, 'reverted': 22590, 'democratized': 22591, 'watermelons': 22592, 'quarry': 22593, 'subsuming': 22594, 'legislated': 22595, 'reasoning': 22596, 'overgrazing': 22597, 'linen': 22598, 'travelling': 22599, 'superb': 22600, 'unconcern': 22601, 'characteristic': 22602, 'contemptuously': 22603, 'bark': 22604, 'birch': 22605, 'gump': 22606, 'overheard': 22607, 'pie': 22608, 'disbursed': 22609, 'akitaka': 22610, 'hammering': 22611, 'creatively': 22612, 'tankbuster': 22613, 'kheyal': 22614, 'baaz': 22615, 'gardez': 22616, 'cantarell': 22617, 'administrators': 22618, 'ilayan': 22619, 'duda': 22620, 'mendonca': 22621, 'floors': 22622, 'crumbled': 22623, 'inferior': 22624, 'correcting': 22625, 'dengue': 22626, 'larvae': 22627, 'breed': 22628, 'chul': 22629, 'woon': 22630, '626': 22631, "n'zerekore": 22632, 'yentai': 22633, 'bhaikaji': 22634, 'ghimire': 22635, 'prostitutes': 22636, 'handicaps': 22637, 'pioneering': 22638, 'lagham': 22639, 'chaudry': 22640, 'sebastiao': 22641, 'veloso': 22642, 'abdali': 22643, 'powder': 22644, 'keg': 22645, 'servicmen': 22646, 'jennings': 22647, 'borrows': 22648, 'cuomo': 22649, 'brokers': 22650, 'wemple': 22651, 'stoffel': 22652, 'clarified': 22653, 'kerem': 22654, 'yaracuy': 22655, 'lapi': 22656, 'carabobo': 22657, 'henrique': 22658, 'salas': 22659, 'jailbreak': 22660, 'banghazi': 22661, 'unbeatable': 22662, 'abdi': 22663, 'dagoberto': 22664, 'swirled': 22665, 'narrowing': 22666, 'redistributed': 22667, 'witch': 22668, 'discriminate': 22669, 'muthaura': 22670, 'kospi': 22671, 'noureddine': 22672, 'marshmallow': 22673, 'yam': 22674, 'sibghatullah': 22675, 'marshmallows': 22676, 'hilario': 22677, 'davide': 22678, 'alluded': 22679, 'psychiatrist': 22680, 'carreno': 22681, 'backyards': 22682, 'talhi': 22683, 'sughayr': 22684, 'khashiban': 22685, 'freezes': 22686, 'frantic': 22687, 'chosun': 22688, 'kuduna': 22689, 'abdulhamid': 22690, 'cough': 22691, 'lubroth': 22692, 'collateral': 22693, 'demise': 22694, 'awe': 22695, 'wolde': 22696, 'meshesha': 22697, 'ozcan': 22698, 'disrupts': 22699, 'aena': 22700, 'belching': 22701, 'abrasive': 22702, 'minar': 22703, 'lecture': 22704, 'yongbyong': 22705, 'temptations': 22706, 'parachute': 22707, 'zydeco': 22708, 'cajun': 22709, 'okie': 22710, 'dokie': 22711, 'stomp': 22712, 'dandy': 22713, 'zaw': 22714, 'masud': 22715, 'murshidabad': 22716, 'greatness': 22717, 'bullring': 22718, 'theofilos': 22719, 'malenchenko': 22720, 'kula': 22721, 'babel': 22722, 'psv': 22723, 'afellay': 22724, 'sota': 22725, 'hirayama': 22726, '68th': 22727, 'omotoyossi': 22728, '32nd': 22729, '59th': 22730, '5th': 22731, 'hilltops': 22732, 'evictions': 22733, 'thuggish': 22734, 'fitch': 22735, 'aa': 22736, 'bbb': 22737, 'shidiac': 22738, 'lbc': 22739, 'judging': 22740, 'configuring': 22741, 'brining': 22742, 'eloy': 22743, '820': 22744, 'talbak': 22745, 'nazarov': 22746, 'rakhmonov': 22747, 'purina': 22748, '422': 22749, 'greenville': 22750, 'cincinnati': 22751, 'eveready': 22752, '1494': 22753, '1655': 22754, 'mohmmed': 22755, '461': 22756, 'distortions': 22757, 'rigidities': 22758, 'nejad': 22759, 'fended': 22760, 'ace': 22761, 'decaying': 22762, 'neglected': 22763, 'telecoms': 22764, 'kazakhstani': 22765, 'tenge': 22766, 'overreliance': 22767, 'petrochemicals': 22768, 'lucayan': 22769, '1647': 22770, 'inca': 22771, '1533': 22772, '1717': 22773, 'nosed': 22774, 'hairless': 22775, 'laugh': 22776, 'dearest': 22777, 'additives': 22778, 'recede': 22779, '828': 22780, 'jixi': 22781, 'nehe': 22782, 'floruan': 22783, 'barbey': 22784, 'fataki': 22785, 'pradip': 22786, 'carmo': 22787, 'fernandes': 22788, 'deandre': 22789, 'crank': 22790, 'itunes': 22791, 'globalized': 22792, 'pinpoint': 22793, 'abductee': 22794, 'admited': 22795, 'contrite': 22796, 'funk': 22797, 'khayrat': 22798, 'shater': 22799, 'linguists': 22800, 'theoneste': 22801, 'codefendants': 22802, '200s': 22803, 'giordani': 22804, 'recoverable': 22805, 'azeglio': 22806, 'ciampi': 22807, 'prestige': 22808, '264': 22809, 'inhumanely': 22810, 'aaron': 22811, 'galindo': 22812, 'nandrolone': 22813, 'somebody': 22814, 'rebirth': 22815, 'unwavering': 22816, 'arinze': 22817, 'easterly': 22818, '1800': 22819, 'aktham': 22820, 'ennals': 22821, 'somalian': 22822, 'hatching': 22823, 'naise': 22824, 'embodied': 22825, 'bussereau': 22826, 'perfectly': 22827, 'plummets': 22828, 'finalizes': 22829, 'modification': 22830, 'barney': 22831, 'disseminating': 22832, 'platinum': 22833, 'stylist': 22834, 'tameka': 22835, 'smalls': 22836, 'carme': 22837, 'yoadimadji': 22838, 'wilds': 22839, 'silicon': 22840, 'outsourcing': 22841, 'numbering': 22842, 'capua': 22843, 'shih': 22844, 'chien': 22845, 'wahid': 22846, 'golo': 22847, 'moun': 22848, 'omari': 22849, '276': 22850, 'sociology': 22851, 'razumkov': 22852, 'croatians': 22853, 'zadar': 22854, 'zabihullah': 22855, 'mujahed': 22856, 'canyons': 22857, 'naxalites': 22858, 'exemplifies': 22859, 'welcometousa': 22860, 'interagency': 22861, 'guides': 22862, 'leaner': 22863, 'trimming': 22864, 'underreporting': 22865, 'moratinos': 22866, 'kiran': 22867, 'inheritance': 22868, 'magnificent': 22869, 'breadth': 22870, 'acuteness': 22871, 'crumbling': 22872, 'anita': 22873, 'genocidal': 22874, 'embera': 22875, 'jihadists': 22876, 'blend': 22877, 'norfolk': 22878, 'receptive': 22879, 'dictators': 22880, 'multiply': 22881, 'auditorium': 22882, 'decontaminating': 22883, 'boone': 22884, 'oilman': 22885, 'reno': 22886, 'laser': 22887, 'sadrists': 22888, 'loach': 22889, 'shakes': 22890, 'palme': 22891, "d'or": 22892, 'flanders': 22893, 'inarritu': 22894, 'volver': 22895, 'collectively': 22896, 'narino': 22897, 'cao': 22898, 'gangchuan': 22899, 'butheetaung': 22900, 'hulk': 22901, 'supra': 22902, 'extricated': 22903, 'bayfront': 22904, 'rawi': 22905, 'jibouri': 22906, 'champ': 22907, 'johann': 22908, 'koss': 22909, 'hidalgo': 22910, 'heriberto': 22911, 'lazcano': 22912, 'retaken': 22913, 'primitive': 22914, 'caroline': 22915, 'bulls': 22916, 'pastured': 22917, 'guileful': 22918, 'feasted': 22919, '237': 22920, 'bentegeat': 22921, 'refusals': 22922, 'granville': 22923, 'abdelrahman': 22924, 'rahama': 22925, 'confessing': 22926, 'ireju': 22927, 'bares': 22928, 'furious': 22929, 'messaging': 22930, 'dalailama': 22931, 'evan': 22932, 'floundering': 22933, 'script': 22934, 'xijing': 22935, "xi'an": 22936, 'eyebrow': 22937, 'recluse': 22938, 'disfigurement': 22939, 'tirelessly': 22940, 'denuclearize': 22941, 'gianfranco': 22942, 'bezoti': 22943, 'marshburn': 22944, '40th': 22945, 'tuning': 22946, 'macchiavello': 22947, 'overthrowing': 22948, 'narrows': 22949, 'maud': 22950, 'chastelain': 22951, 'unitary': 22952, 'borys': 22953, 'lutsenko': 22954, 'tsushko': 22955, 'staunchest': 22956, 'despises': 22957, 'tabloids': 22958, 'soap': 22959, 'operas': 22960, 'alien': 22961, '982': 22962, 'vovk': 22963, 'rs': 22964, '12m': 22965, 'kapustin': 22966, 'yar': 22967, 'strings': 22968, 'lenten': 22969, 'packs': 22970, 'grassland': 22971, 'demostrators': 22972, 'skate': 22973, 'evgeny': 22974, 'plushenko': 22975, 'sokolova': 22976, 'viktoria': 22977, 'volchkova': 22978, 'navka': 22979, 'kostomarov': 22980, 'dancers': 22981, 'greener': 22982, 'equalize': 22983, 'shota': 22984, 'utiashvili': 22985, 'turbine': 22986, 'poti': 22987, 'antics': 22988, 'capp': 22989, 'wooten': 22990, 'raleigh': 22991, 'manchin': 22992, 'gazette': 22993, 'sago': 22994, 'fractious': 22995, 'define': 22996, 'marker': 22997, 'estonian': 22998, 'ruutel': 22999, 'andrus': 23000, 'juhan': 23001, 'andriy': 23002, 'shevchenko': 23003, 'balking': 23004, 'qeshm': 23005, 'unhitches': 23006, '1600': 23007, 'unintentional': 23008, 'demonized': 23009, 'molded': 23010, 'managerial': 23011, 'plouffe': 23012, 'misallocation': 23013, 'renegotiate': 23014, 'wmd': 23015, 'adhamiyah': 23016, 'waziriyah': 23017, 'muscular': 23018, 'shafi': 23019, 'vahiuddin': 23020, 'inward': 23021, 'cartoonists': 23022, 'civilizations': 23023, 'bandage': 23024, 'retreating': 23025, 'dissipate': 23026, 'coasts': 23027, 'coppola': 23028, 'limon': 23029, 'sirisia': 23030, 'sabaot': 23031, 'yisrael': 23032, 'beitenu': 23033, 'goverment': 23034, 'calf': 23035, 'displeased': 23036, 'newfoundland': 23037, 'sq': 23038, 'cashew': 23039, 'hut': 23040, 'haunch': 23041, 'mutton': 23042, 'kernels': 23043, 'undp': 23044, 'dahomey': 23045, 'clamor': 23046, 'outsider': 23047, 'cumulative': 23048, 'nigh': 23049, 'furnish': 23050, 'dampen': 23051, 'abated': 23052, 'perch': 23053, 'entangled': 23054, 'fleece': 23055, 'fluttered': 23056, 'clipped': 23057, 'woodcutter': 23058, 'expedient': 23059, 'importunities': 23060, 'suitor': 23061, 'cheerfully': 23062, 'toothless': 23063, 'clawless': 23064, 'woodman': 23065, 'repent': 23066, 'aisle': 23067, 'messed': 23068, 'crapping': 23069, 'tayr': 23070, 'filsi': 23071, 'solemly': 23072, 'obsolete': 23073, 'shalikashvili': 23074, 'tacitly': 23075, 'cardboard': 23076, 'deliberating': 23077, 'savo': 23078, 'comprehend': 23079, 'distracted': 23080, 'balikesir': 23081, 'antoin': 23082, 'dom': 23083, 'afrik': 23084, 'tutankhamun': 23085, 'skyline': 23086, 'demerits': 23087, 'misused': 23088, 'blanked': 23089, 'emmen': 23090, 'otalvaro': 23091, '52nd': 23092, 'lionel': 23093, 'messi': 23094, 'doetinchem': 23095, 'taye': 23096, 'taiwo': 23097, 'kolesnikov': 23098, '331': 23099, 'wirajuda': 23100, 'sassi': 23101, '347': 23102, 'oviedo': 23103, 'norbert': 23104, 'lammert': 23105, 'girona': 23106, 'bigots': 23107, 'apure': 23108, 'catalonians': 23109, 'rocio': 23110, 'esteban': 23111, 'cooperativa': 23112, 'boniface': 23113, 'localized': 23114, 'aftenposten': 23115, 'noneducational': 23116, 'proliferate': 23117, 'regina': 23118, 'reichenm': 23119, 'reassurance': 23120, 'infomation': 23121, '569': 23122, 'mohean': 23123, 'nodar': 23124, 'khashba': 23125, 'voided': 23126, 'gali': 23127, 'majmaah': 23128, 'assorted': 23129, 'rodon': 23130, 'hargeisa': 23131, 'maigao': 23132, 'paksitan': 23133, '1718': 23134, 'safta': 23135, 'naysayers': 23136, 'cynics': 23137, 'strenuously': 23138, 'balancing': 23139, 'assumptions': 23140, 'disturbingly': 23141, 'relays': 23142, 'merajudeen': 23143, 'squander': 23144, 'hospitalizations': 23145, 'gripped': 23146, 'hurry': 23147, '50th': 23148, 'ysidro': 23149, 'diameter': 23150, 'sinuiju': 23151, 'portman': 23152, 'iiss': 23153, 'hails': 23154, 'tipping': 23155, 'badaun': 23156, 'organiation': 23157, 'shahawar': 23158, 'matin': 23159, 'brooklyn': 23160, 'stoked': 23161, 'impress': 23162, "qur'an": 23163, 'scud': 23164, 'showering': 23165, 'thankful': 23166, 'agendas': 23167, 'bradford': 23168, 'ernst': 23169, 'disburses': 23170, 'kasami': 23171, 'shab': 23172, 'barat': 23173, '1621': 23174, 'sophistication': 23175, 'cpsc': 23176, 'chieftain': 23177, 'darfuri': 23178, 'eissa': 23179, 'ghazal': 23180, 'tandem': 23181, 'jebaliya': 23182, 'podemos': 23183, 'akylbek': 23184, 'ricans': 23185, 'plebiscites': 23186, 'guei': 23187, 'blatantly': 23188, 'disaffected': 23189, 'linas': 23190, 'marcoussis': 23191, 'guillaume': 23192, 'ouagadougou': 23193, 'reintegration': 23194, 'dramane': 23195, 'pleasant': 23196, 'nonpolluting': 23197, 'thrives': 23198, 'jurisdictions': 23199, 'monopolies': 23200, 'computerized': 23201, 'tamper': 23202, 'biometric': 23203, 'reassert': 23204, 'optician': 23205, 'telescopes': 23206, 'munificent': 23207, 'patronage': 23208, 'kangaroo': 23209, 'awkwardly': 23210, 'pouch': 23211, 'desirous': 23212, 'deceitful': 23213, 'insupportable': 23214, 'cheerless': 23215, 'unappreciated': 23216, 'implored': 23217, 'endow': 23218, 'resentment': 23219, 'fulness': 23220, 'thereof': 23221, 'incaudate': 23222, 'chin': 23223, 'wags': 23224, 'gratification': 23225, 'charon': 23226, 'geology': 23227, 'kuiper': 23228, 'payenda': 23229, 'rocketed': 23230, 'caves': 23231, 'subverting': 23232, 'mahamadou': 23233, 'issoufou': 23234, 'sarturday': 23235, 'hassas': 23236, 'checkpost': 23237, 'khela': 23238, 'barbershops': 23239, 'jabber': 23240, 'belgaum': 23241, 'mohamuud': 23242, 'musse': 23243, 'gurage': 23244, 'dastardly': 23245, 'responsibilty': 23246, 'ossetian': 23247, 'katsina': 23248, 'acquittals': 23249, 'convict': 23250, 'mazda': 23251, 'outsold': 23252, 'volkswagen': 23253, 'agadez': 23254, 'mnj': 23255, 'crusted': 23256, 'immobilized': 23257, 'drifts': 23258, 'siding': 23259, 'antonin': 23260, 'scalia': 23261, 'hermogenes': 23262, 'esperon': 23263, 'dulmatin': 23264, 'patek': 23265, 'suspiciously': 23266, 'behaving': 23267, 'stopper': 23268, 'evin': 23269, 'hats': 23270, 'cobs': 23271, 'recognizable': 23272, 'pests': 23273, 'monsanto': 23274, 'frattini': 23275, 'colllins': 23276, 'strangely': 23277, 'ineffectively': 23278, 'galveston': 23279, 'aurangabad': 23280, 'berrones': 23281, 'handkerchiefs': 23282, 'colors': 23283, 'dazzled': 23284, 'documentaries': 23285, 'restless': 23286, 'controller': 23287, 'sunda': 23288, 'volcanos': 23289, 'encircling': 23290, 'gaule': 23291, 'dabaan': 23292, 'baalbek': 23293, 'ural': 23294, 'distorted': 23295, 'unbiased': 23296, 'migrate': 23297, 'roshan': 23298, 'pepsi': 23299, 'indra': 23300, 'madras': 23301, 'undergraduate': 23302, 'graduate': 23303, 'yale': 23304, 'skillfully': 23305, 'sightings': 23306, 'congregate': 23307, 'grower': 23308, 'masjid': 23309, 'eyewitness': 23310, 'forgiving': 23311, 'geologist': 23312, 'piotr': 23313, 'stanczak': 23314, 'geofizyka': 23315, 'krakow': 23316, 'contributors': 23317, 'rehearsing': 23318, 'penitence': 23319, 'unami': 23320, 'muga': 23321, 'delamere': 23322, 'harbored': 23323, 'nonassociated': 23324, '2022': 23325, 'causeway': 23326, 'amerindians': 23327, 'annihilated': 23328, 'revolted': 23329, "l'ouverture": 23330, 'inaugurate': 23331, 'neighbours': 23332, 'avarice': 23333, 'avaricious': 23334, 'envious': 23335, 'vices': 23336, 'spectator': 23337, 'scratched': 23338, 'jog': 23339, 'enables': 23340, 'ivaylo': 23341, 'kalfin': 23342, 'calculated': 23343, 'preparatory': 23344, 'convenience': 23345, 'afghani': 23346, 'magloire': 23347, 'retailing': 23348, 'davit': 23349, 'nomura': 23350, 'kasai': 23351, 'occidental': 23352, 'constatin': 23353, '217': 23354, 'irin': 23355, 'burials': 23356, 'dominating': 23357, 'ilya': 23358, 'kovalchuk': 23359, 'goaltender': 23360, 'evgeni': 23361, 'nabokov': 23362, 'shutout': 23363, 'suleimaniya': 23364, 'abolishes': 23365, 'tier': 23366, '482': 23367, 'm1': 23368, 'belfort': 23369, 'whistler': 23370, 'zurbriggen': 23371, 'nkosazana': 23372, 'dlamini': 23373, 'transitioning': 23374, 'extraditions': 23375, 'revert': 23376, 'mamoun': 23377, 'unstoppable': 23378, 'paule': 23379, 'kieny': 23380, 'irreversible': 23381, 'zuckerberg': 23382, 'billionaires': 23383, 'bacterium': 23384, 'ubiquitous': 23385, 'frenk': 23386, 'guni': 23387, 'rusere': 23388, 'otto': 23389, 'finishers': 23390, 'cpj': 23391, '090': 23392, 'prosper': 23393, 'alcoa': 23394, 'santuario': 23395, 'guatica': 23396, 'restrepo': 23397, 'reintegrate': 23398, 'downbeat': 23399, 'hausa': 23400, 'impressions': 23401, 'showered': 23402, 'unprotected': 23403, 'intercourse': 23404, 'contingency': 23405, 'verge': 23406, 'clinching': 23407, 'huckabee': 23408, 'sayyed': 23409, 'tantawi': 23410, 'bioterrorism': 23411, 'purifying': 23412, 'accommodating': 23413, 'navtej': 23414, 'sarna': 23415, 'fascists': 23416, 'neon': 23417, 'sizes': 23418, 'underfunding': 23419, 'baltasar': 23420, 'garzon': 23421, 'ramzi': 23422, 'binalshibh': 23423, 'tahar': 23424, 'ezirouali': 23425, 'heptathlon': 23426, 'natalia': 23427, 'dobrynska': 23428, 'alwan': 23429, 'baquoba': 23430, 'hourmadji': 23431, 'doumgor': 23432, 'chadians': 23433, 'khaleda': 23434, 'tarique': 23435, 'taka': 23436, 'zhuang': 23437, 'collaborative': 23438, 'cabezas': 23439, 'unsatisfactory': 23440, 'zeros': 23441, 'dzhennet': 23442, 'abdurakhmanova': 23443, 'umalat': 23444, 'magomedov': 23445, 'markha': 23446, 'leashes': 23447, 'illustrates': 23448, 'relented': 23449, 'reappointing': 23450, 'aberrahman': 23451, 'catastrophes': 23452, 'happening': 23453, 'busters': 23454, 'gmt': 23455, 'andrey': 23456, 'denisov': 23457, 'underline': 23458, "madai'ni": 23459, 'overrunning': 23460, '249': 23461, 'particulate': 23462, 'cavic': 23463, 'azizullah': 23464, 'lodin': 23465, 'evangelical': 23466, 'zelenak': 23467, 'nipayia': 23468, 'asir': 23469, 'handwriting': 23470, 'shorja': 23471, 'inflaming': 23472, 'uedf': 23473, 'vaccinating': 23474, 'jeanet': 23475, 'goot': 23476, 'infectiousness': 23477, 'thereby': 23478, 'inoculation': 23479, '1765': 23480, 'manx': 23481, 'gaelic': 23482, 'idi': 23483, 'promulgated': 23484, 'amending': 23485, 'respectable': 23486, 'antananarivo': 23487, 'simplification': 23488, 'mitigated': 23489, 'graduates': 23490, 'vineyards': 23491, 'spades': 23492, 'mattocks': 23493, 'repaid': 23494, 'superabundant': 23495, 'mechanisms': 23496, 'surety': 23497, 'pleases': 23498, 'begging': 23499, 'coward': 23500, 'cookstown': 23501, 'tyrone': 23502, 'paralysed': 23503, 'waist': 23504, 'negligent': 23505, 'bernazzani': 23506, 'nacion': 23507, 'mladjen': 23508, 'vojkovici': 23509, 'fraught': 23510, 'kiwayu': 23511, 'ta': 23512, 'tuol': 23513, 'sleng': 23514, 'duch': 23515, 'adan': 23516, 'mirrored': 23517, 'despondent': 23518, 'boulevard': 23519, 'vasilily': 23520, 'filipchuk': 23521, 'thespians': 23522, 'shakespeareans': 23523, 'zaher': 23524, 'lifeline': 23525, 'guideline': 23526, 'vaccinated': 23527, 'rosal': 23528, 'deserts': 23529, 'greenery': 23530, 'sonntags': 23531, 'blick': 23532, 'reassigned': 23533, 'millerwise': 23534, 'dyck': 23535, 'hierarchical': 23536, 'staffed': 23537, 'pleshkov': 23538, 'krasnokamensk': 23539, 'forays': 23540, 'harcharik': 23541, 'angioplasty': 23542, 'impeding': 23543, 'schedules': 23544, 'hadjiya': 23545, 'terrorize': 23546, 'fldr': 23547, 'handler': 23548, 'canine': 23549, 'soiling': 23550, 'ketzer': 23551, 'emptively': 23552, 'halfun': 23553, '30s': 23554, 'niyazov': 23555, 'schulz': 23556, 'corroborate': 23557, 'chevallier': 23558, 'shek': 23559, 'jarrah': 23560, 'danny': 23561, 'ayalon': 23562, 'coldplay': 23563, 'rude': 23564, 'paolo': 23565, 'opined': 23566, 'nicest': 23567, 'testy': 23568, 'ringtone': 23569, 'edging': 23570, '068171296': 23571, '068217593': 23572, 'aufdenblatten': 23573, '068263889': 23574, '068275463': 23575, '782': 23576, '685': 23577, 'redesigned': 23578, 'slope': 23579, 'sangju': 23580, '4000': 23581, 'mexicali': 23582, 'marghzar': 23583, 'underfunded': 23584, 'unprepared': 23585, 'comrade': 23586, 'nigerla': 23587, 'shoppertrak': 23588, 'rct': 23589, 'quietly': 23590, 'elaborating': 23591, 'chaparhar': 23592, 'radioactivity': 23593, 'narim': 23594, 'alcolac': 23595, 'vwr': 23596, 'thermo': 23597, 'maruf': 23598, 'bakhit': 23599, 'fayez': 23600, 'minia': 23601, 'sights': 23602, 'exxon': 23603, 'reaping': 23604, 'hers': 23605, 'cheadle': 23606, 'remixed': 23607, 'benham': 23608, 'natagehi': 23609, 'paratroopers': 23610, 'excursion': 23611, 'landale': 23612, 'mirko': 23613, 'norac': 23614, 'ademi': 23615, 'medak': 23616, 'gall': 23617, 'bladder': 23618, 'reims': 23619, 'penjwin': 23620, 'durham': 23621, 'taxiways': 23622, 'hornafrik': 23623, 'abdirahman': 23624, 'dinari': 23625, 'sreten': 23626, 'vascular': 23627, '1726': 23628, '1828': 23629, 'tupamaros': 23630, 'frente': 23631, 'amplio': 23632, 'freest': 23633, 'salam': 23634, 'fayyad': 23635, 'uptick': 23636, 'mandeb': 23637, 'hormuz': 23638, 'malacca': 23639, 'cranes': 23640, 'plowlands': 23641, 'brandishing': 23642, 'forsook': 23643, 'liliput': 23644, 'earnest': 23645, 'suffice': 23646, 'groan': 23647, 'finger': 23648, 'misdeeds': 23649, 'whitewash': 23650, 'mortification': 23651, 'unsettling': 23652, 'buyout': 23653, 'breakout': 23654, 'kuru': 23655, 'wareng': 23656, 'barakin': 23657, 'ladi': 23658, 'anwarul': 23659, 'usman': 23660, 'unseated': 23661, 'railed': 23662, 'wadia': 23663, 'jaish': 23664, 'hafsa': 23665, 'hiked': 23666, 'busier': 23667, 'quelled': 23668, 'yaounde': 23669, 'cancels': 23670, 'fasted': 23671, 'nidal': 23672, 'saada': 23673, 'amona': 23674, 'overstaying': 23675, 'culpable': 23676, 'grandchildren': 23677, 'hrd': 23678, 'kapil': 23679, 'linux': 23680, 'perito': 23681, 'glacieres': 23682, 'spectacular': 23683, 'abdolsamad': 23684, 'khorramshahi': 23685, 'shatters': 23686, 'interception': 23687, 'arjangi': 23688, 'elliott': 23689, 'abrams': 23690, 'welch': 23691, 'catandunes': 23692, 'crusade': 23693, 'satirical': 23694, 'rockefeller': 23695, 'zaldivar': 23696, 'gusting': 23697, 'treacherous': 23698, 'pile': 23699, 'trnava': 23700, 'compensating': 23701, 'tuition': 23702, 'biak': 23703, 'tremendously': 23704, 'substantiate': 23705, 'supposition': 23706, 'involuntary': 23707, 'callup': 23708, 'chimango': 23709, '188': 23710, 'asgiriya': 23711, 'kandy': 23712, 'seam': 23713, 'spinner': 23714, 'monty': 23715, 'collingwood': 23716, 'prasanna': 23717, 'jayawardene': 23718, 'alastair': 23719, 'gana': 23720, 'kingibe': 23721, 'intrude': 23722, 'unintended': 23723, 'conception': 23724, 'liken': 23725, 'latifullah': 23726, 'zukang': 23727, 'shawel': 23728, 'mosaic': 23729, 'farthest': 23730, 'snapshot': 23731, 'bang': 23732, 'turbans': 23733, 'mature': 23734, 'galactic': 23735, 'sorts': 23736, 'clarity': 23737, 'nom': 23738, 'guerre': 23739, 'masons': 23740, 'muqataa': 23741, 'morality': 23742, 'headdress': 23743, 'wearers': 23744, 'escalates': 23745, 'shaaban': 23746, 'plantings': 23747, 'headgear': 23748, 'preventative': 23749, 'pronounces': 23750, 'protégé': 23751, 'suited': 23752, 'jusuf': 23753, 'kalla': 23754, 'mukmin': 23755, 'mammy': 23756, 'fare': 23757, 'mohtarem': 23758, 'manzur': 23759, 'disengage': 23760, 'adamant': 23761, 'ducked': 23762, 'masterminded': 23763, 'hangings': 23764, 'khazaee': 23765, 'tightens': 23766, 'reluctantly': 23767, '1713': 23768, 'utrecht': 23769, 'gibraltarians': 23770, 'tripartite': 23771, 'cooperatively': 23772, 'noncolonial': 23773, 'sporadically': 23774, 'durrani': 23775, '1747': 23776, 'notional': 23777, 'experiment': 23778, 'tottering': 23779, 'relentless': 23780, 'ladin': 23781, 'bonn': 23782, 'saavedra': 23783, 'mitch': 23784, 'buoyant': 23785, 'strange': 23786, 'lacerated': 23787, 'belabored': 23788, 'tonight': 23789, 'toil': 23790, 'waggon': 23791, 'rut': 23792, 'exertion': 23793, 'tuba': 23794, 'firmn': 23795, 'hotbeds': 23796, 'mitsumi': 23797, 'puli': 23798, 'obscure': 23799, 'mizhar': 23800, '618': 23801, 'varema': 23802, 'pummeling': 23803, 'northwesterly': 23804, 'vitamin': 23805, 'therapies': 23806, 'matthias': 23807, 'bounnyang': 23808, 'vorachit': 23809, 'ome': 23810, 'taj': 23811, 'macedonians': 23812, '463': 23813, 'counterattack': 23814, 'yuganskeneftegaz': 23815, 'ramin': 23816, 'mahmanparast': 23817, 'adhaim': 23818, 'emissary': 23819, 'censors': 23820, 'golkar': 23821, 'jonah': 23822, 'brass': 23823, '532': 23824, '864': 23825, '406': 23826, 'electricidad': 23827, 'edc': 23828, 'nationalizations': 23829, 'mouthing': 23830, 'articulated': 23831, 'iadb': 23832, 'crimping': 23833, 'mikasa': 23834, 'concubines': 23835, 'saidi': 23836, 'tohid': 23837, 'bighi': 23838, 'henghameh': 23839, 'somaieh': 23840, 'nosrati': 23841, 'matinpour': 23842, '239': 23843, 'espirito': 23844, 'catu': 23845, 'bahia': 23846, 'gasene': 23847, 'dejan': 23848, 'bugsy': 23849, 'nebojsa': 23850, 'instrumental': 23851, 'hajime': 23852, 'massaki': 23853, 'mutalib': 23854, 'convening': 23855, 'procedural': 23856, 'gamble': 23857, 'andar': 23858, 'rejoining': 23859, 'madelyn': 23860, 'impregnating': 23861, 'matabeleland': 23862, 'counterfeiting': 23863, 'geologists': 23864, 'confessions': 23865, 'censored': 23866, 'piot': 23867, 'pears': 23868, 'shoe': 23869, 'baitullah': 23870, 'perpetuating': 23871, 'ericq': 23872, 'les': 23873, 'cayes': 23874, 'attainable': 23875, 'augustin': 23876, 'welt': 23877, 'saroki': 23878, 'bolder': 23879, 'herve': 23880, 'morin': 23881, 'areva': 23882, 'silbert': 23883, 'californian': 23884, 'chuck': 23885, 'hamel': 23886, 'corrosion': 23887, 'prudhoe': 23888, 'implanted': 23889, 'manufactures': 23890, 'syncardia': 23891, 'eskisehir': 23892, 'kiliclar': 23893, 'nazer': 23894, 'osprey': 23895, 'reacts': 23896, 'unfold': 23897, 'weblogs': 23898, 'blogs': 23899, 'aleim': 23900, 'gothenburg': 23901, 'scandinavia': 23902, 'gungoren': 23903, 'savage': 23904, 'olusegan': 23905, 'trouncing': 23906, 'prefers': 23907, 'taker': 23908, 'younes': 23909, 'megawatt': 23910, 'deutchmark': 23911, 'dinar': 23912, 'infusion': 23913, 'criminality': 23914, 'chernobyl': 23915, 'riverine': 23916, 'semidesert': 23917, 'devaluation': 23918, 'predominate': 23919, 'ranches': 23920, 'melons': 23921, 'exemptions': 23922, 'booty': 23923, 'witnessing': 23924, 'learns': 23925, 'beg': 23926, 'requite': 23927, 'kindness': 23928, 'flattered': 23929, 'messes': 23930, 'eats': 23931, 'behaves': 23932, 'cubapetroleo': 23933, 'cnbc': 23934, 'coercive': 23935, 'conniving': 23936, 'dismisses': 23937, 'doubting': 23938, 'chests': 23939, 'beltran': 23940, 'shyloh': 23941, 'entangle': 23942, '405': 23943, 've': 23944, 'falluja': 23945, 'conduit': 23946, 'remissaninthe': 23947, 'roared': 23948, 'formulations': 23949, 'differing': 23950, '2050': 23951, 'polluting': 23952, 'bitar': 23953, 'bitarov': 23954, 'adilgerei': 23955, 'magomedtagirov': 23956, 'xianghe': 23957, 'salang': 23958, 'belgians': 23959, 'statoil': 23960, 'krasnoyarsk': 23961, 'assortment': 23962, 'subtracted': 23963, 'mawlawi': 23964, 'masah': 23965, 'ulema': 23966, 'cambridge': 23967, 'azocar': 23968, 'bollywood': 23969, 'kiteswere': 23970, 'silverman': 23971, 'deifies': 23972, 'militarist': 23973, 'demonstrably': 23974, 'antony': 23975, 'ambareesh': 23976, 'jayprakash': 23977, 'narain': 23978, 'rashtriya': 23979, 'dal': 23980, 'jabel': 23981, 'creutzfeldt': 23982, 'bovine': 23983, 'spongiform': 23984, 'encephalopathy': 23985, 'alienation': 23986, 'victimize': 23987, 'loosely': 23988, 'smash': 23989, 'delivers': 23990, 'samho': 23991, 'qater': 23992, 'frontal': 23993, 'aboutorabi': 23994, 'fard': 23995, 'seeker': 23996, 'joongang': 23997, 'esfandiar': 23998, 'exits': 23999, 'fang': 24000, 'llam': 24001, 'impassioned': 24002, 'vandemoortele': 24003, 'defies': 24004, '1004': 24005, 'maghazi': 24006, 'thun': 24007, 'statistical': 24008, 'lindy': 24009, 'makubalo': 24010, 'olesgun': 24011, 'miserable': 24012, 'turkana': 24013, 'rongai': 24014, 'marigat': 24015, 'mogotio': 24016, 'corresponding': 24017, 'sledge': 24018, 'hammers': 24019, 'structurally': 24020, 'unsound': 24021, 'seabees': 24022, 'shujaat': 24023, 'zamir': 24024, 'asef': 24025, 'shawkat': 24026, 'bumpy': 24027, 'firebase': 24028, 'ripley': 24029, 'baskin': 24030, 'bypassed': 24031, 'inroads': 24032, 'wefaq': 24033, 'botanic': 24034, 'partnered': 24035, 'orchids': 24036, 'splash': 24037, 'brilliance': 24038, 'shahid': 24039, 'durjoy': 24040, 'bangla': 24041, 'bogra': 24042, 'demographer': 24043, 'spouse': 24044, 'mu': 24045, 'guangzhong': 24046, 'fetus': 24047, 'heartened': 24048, 'shies': 24049, 'kanan': 24050, 'duffy': 24051, 'balah': 24052, 'swerve': 24053, 'aweil': 24054, 'hanifa': 24055, '733': 24056, 'g20': 24057, 'azizi': 24058, 'jalali': 24059, 'benoit': 24060, 'maimed': 24061, 'ceel': 24062, 'waaq': 24063, 'campsites': 24064, 'schoolgirls': 24065, 'motorbikes': 24066, 'bundled': 24067, 'iapa': 24068, 'overhauling': 24069, 'entitlement': 24070, 'exceedingly': 24071, 'samoan': 24072, 'togoland': 24073, 'facade': 24074, 'rpt': 24075, 'continually': 24076, 'tile': 24077, 'prospering': 24078, 'tilemaker': 24079, 'shine': 24080, 'bolting': 24081, 'abstraction': 24082, 'pickle': 24083, 'spectacles': 24084, 'needless': 24085, 'lens': 24086, 'wharf': 24087, 'stumped': 24088, 'predator': 24089, 'brewed': 24090, 'adhesive': 24091, 'togetherness': 24092, 'monosodium': 24093, 'glue': 24094, 'shimbun': 24095, 'valero': 24096, 'prejudice': 24097, 'tarcisio': 24098, 'furor': 24099, 'priestly': 24100, 'celibacy': 24101, 'galo': 24102, 'chiriboga': 24103, 'dries': 24104, 'buehring': 24105, 'gujri': 24106, 'gopal': 24107, 'slams': 24108, 'tolerating': 24109, 'animists': 24110, 'heineken': 24111, 'pingdingshan': 24112, 'coded': 24113, 'correspondence': 24114, 'deciphering': 24115, 'endeavouris': 24116, 'endeavourundocked': 24117, 'educate': 24118, 'hell': 24119, 'wook': 24120, 'gainers': 24121, 'performace': 24122, 'concacaf': 24123, 'hamarjajab': 24124, 'bridged': 24125, 'khatibi': 24126, 'flourish': 24127, 'abilities': 24128, 'rangeen': 24129, 'milomir': 24130, 'h7n3': 24131, 'droppings': 24132, 'thyroid': 24133, '47s': 24134, 'slayings': 24135, 'zvyagintsev': 24136, 'parulski': 24137, 'smolensk': 24138, 'djinnit': 24139, 'unacceptably': 24140, 'grossman': 24141, 'sliced': 24142, 'hacked': 24143, 'canons': 24144, 'glad': 24145, 'unravel': 24146, 'fabric': 24147, 'calculators': 24148, 'bookmaking': 24149, 'khurul': 24150, 'kalmykia': 24151, 'expectation': 24152, 'khouna': 24153, 'haidallah': 24154, 'maaouiya': 24155, 'advertisement': 24156, 'expansive': 24157, 'communicated': 24158, 'fares': 24159, 'firmest': 24160, 'mediating': 24161, 'pepper': 24162, 'truncheons': 24163, 'beyazit': 24164, 'sushi': 24165, 'jinling': 24166, 'spiraled': 24167, 'hagino': 24168, 'uji': 24169, 'shfaram': 24170, 'renen': 24171, 'schorr': 24172, 'shooter': 24173, 'kach': 24174, 'belhadj': 24175, 'inoculate': 24176, 'ziyad': 24177, 'desouki': 24178, 'vaccinators': 24179, 'macheke': 24180, 'aeneas': 24181, 'chigwedere': 24182, 'lci': 24183, 'react': 24184, 'relate': 24185, 'incur': 24186, 'directives': 24187, 'raiser': 24188, 'picnic': 24189, 'kickball': 24190, 'volleyball': 24191, 'crafts': 24192, 'creek': 24193, 'roast': 24194, 'fixings': 24195, '241': 24196, '2661': 24197, 'jcfundrzr': 24198, 'colonizers': 24199, '1906': 24200, 'condominium': 24201, '963': 24202, 'benelux': 24203, 'agrochemicals': 24204, 'aral': 24205, 'stagnation': 24206, 'curtailment': 24207, '1935': 24208, 'insurgencies': 24209, 'conqueror': 24210, 'flapped': 24211, 'exultingly': 24212, 'pounced': 24213, 'undisputed': 24214, 'comission': 24215, 'sportsmen': 24216, 'coho': 24217, 'walleye': 24218, 'reproduced': 24219, 'muskie': 24220, 'kowalski': 24221, 'laotian': 24222, 'harkin': 24223, 'dispose': 24224, 'waiver': 24225, 'suicides': 24226, 'uday': 24227, 'numaniya': 24228, 'domed': 24229, 'cykla': 24230, 'assailant': 24231, 'fathi': 24232, 'nuaimi': 24233, 'void': 24234, 'firmer': 24235, 'jiang': 24236, 'jufeng': 24237, 'bildnewspaper': 24238, 'fastening': 24239, 'ornament': 24240, 'align': 24241, 'propped': 24242, 'robustly': 24243, 'getters': 24244, 'mizarkhel': 24245, '737s': 24246, 'rashad': 24247, '356': 24248, 'defaulting': 24249, 'disbursements': 24250, 'pontificate': 24251, 'delegated': 24252, 'urbi': 24253, 'orbi': 24254, 'supremacist': 24255, 'mahlangu': 24256, 'afrikaner': 24257, 'bludgeoned': 24258, 'fenced': 24259, '259': 24260, 'labs': 24261, 'kaletra': 24262, 'generic': 24263, 'overpricing': 24264, 'muted': 24265, 'malaysians': 24266, 'vacations': 24267, 'caucasian': 24268, 'teresa': 24269, 'borcz': 24270, 'harkat': 24271, 'zihad': 24272, 'ranbir': 24273, 'dayers': 24274, 'acrimonious': 24275, 'chrysostomos': 24276, 'heretic': 24277, 'poachers': 24278, 'skins': 24279, 'imperialist': 24280, 'crucified': 24281, 'neiva': 24282, 'jebii': 24283, '1582': 24284, 'noncompliant': 24285, 'yunlin': 24286, 'mariane': 24287, 'odierno': 24288, 'bobbie': 24289, 'vihemina': 24290, 'prout': 24291, 'wrought': 24292, 'singling': 24293, 'chibebe': 24294, 'seige': 24295, 'tobie': 24296, 'homosexual': 24297, 'discriminated': 24298, 'insights': 24299, 'sulik': 24300, 'rebut': 24301, 'broncos': 24302, 'undrafted': 24303, 'jake': 24304, 'plummer': 24305, 'kicker': 24306, 'elam': 24307, 'receiver': 24308, 'samie': 24309, 'corpse': 24310, 'kits': 24311, 'luzhkov': 24312, 'satanic': 24313, 'htay': 24314, 'ashin': 24315, 'hkamti': 24316, 'sagaing': 24317, 'willian': 24318, 'sadiq': 24319, 'slimy': 24320, 'messiest': 24321, 'dispenser': 24322, 'goo': 24323, 'sandler': 24324, 'stiller': 24325, 'wannabe': 24326, 'youthful': 24327, 'lighthearted': 24328, 'imprisioned': 24329, '1745': 24330, 'xinqian': 24331, 'adjusted': 24332, 'asadollah': 24333, 'obligates': 24334, 'leftwing': 24335, 'relates': 24336, 'buraydah': 24337, 'singur': 24338, 'uttarakhand': 24339, 'chapfika': 24340, 'zvakwana': 24341, 'anguillan': 24342, 'intercommunal': 24343, 'trnc': 24344, 'impetus': 24345, 'acquis': 24346, 'forbade': 24347, 'projecting': 24348, 'tunes': 24349, 'leaping': 24350, 'perverse': 24351, 'merrily': 24352, 'goblet': 24353, 'jarring': 24354, 'zeal': 24355, 'naturedly': 24356, 'benefactor': 24357, 'gnawed': 24358, 'orioles': 24359, 'baltimore': 24360, 'outfield': 24361, 'glowing': 24362, 'proboscis': 24363, 'pinna': 24364, 'stefano': 24365, 'predazzo': 24366, 'belluno': 24367, 'spleen': 24368, 'cavalese': 24369, 'someday': 24370, 'zwelinzima': 24371, 'vavi': 24372, 'uz': 24373, 'mushahid': 24374, 'squadron': 24375, 'shawn': 24376, 'brightest': 24377, 'enming': 24378, 'aspiring': 24379, 'olympian': 24380, 'sagging': 24381, 'gdps': 24382, '1672': 24383, 'inevitably': 24384, 'simmers': 24385, 'policharki': 24386, 'khaisor': 24387, 'symbolism': 24388, 'heighten': 24389, 'enviable': 24390, 'xinmiao': 24391, 'qing': 24392, '1644': 24393, 'adwar': 24394, 'rabiyaa': 24395, 'shehzad': 24396, 'tanweer': 24397, 'hasib': 24398, 'slid': 24399, 'uncovering': 24400, 'taser': 24401, 'giuliani': 24402, 'hema': 24403, 'serge': 24404, 'acutely': 24405, 'ilna': 24406, 'sajedinia': 24407, 'karoubi': 24408, 'liner': 24409, "ma'ariv": 24410, 'heidelberg': 24411, 'intestine': 24412, 'hoekstra': 24413, 'diane': 24414, 'feinstein': 24415, 'dozing': 24416, 'bailey': 24417, 'videolink': 24418, 'neil': 24419, 'horrifying': 24420, 'counseled': 24421, 'acorn': 24422, 'heroism': 24423, 'ignace': 24424, 'schops': 24425, "voa's": 24426, 'acorns': 24427, 'mistletoe': 24428, 'irremediable': 24429, 'insure': 24430, 'mikerevic': 24431, 'dismissals': 24432, 'flax': 24433, 'boded': 24434, "pe'at": 24435, 'sadeh': 24436, 'lastly': 24437, 'darts': 24438, 'dormitories': 24439, 'disobey': 24440, 'credence': 24441, 'stubb': 24442, 'wisest': 24443, 'odde': 24444, 'leaflet': 24445, 'solitude': 24446, 'mixing': 24447, 'ayyub': 24448, 'khakrez': 24449, 'touts': 24450, 'haleem': 24451, '329': 24452, '913': 24453, '781': 24454, 'cowboys': 24455, 'julius': 24456, 'madhya': 24457, 'buzzard': 24458, 'outstrip': 24459, '205': 24460, '9942': 24461, 'vaeidi': 24462, 'soltanieh': 24463, 'provincal': 24464, 'crazy': 24465, 'pest': 24466, 'fouling': 24467, 'insect': 24468, 'extracting': 24469, 'cesar': 24470, 'abderrahaman': 24471, 'infidel': 24472, 'confiscate': 24473, 'ashland': 24474, 'gyeong': 24475, 'nuptials': 24476, 'bryant': 24477, 'sita': 24478, 'miliatry': 24479, 'garikoitz': 24480, 'pyrenees': 24481, 'wireless': 24482, 'txeroki': 24483, 'myricks': 24484, 'demotion': 24485, 'samsun': 24486, 'vertigo': 24487, 'heralds': 24488, 'filmmaking': 24489, 'replicating': 24490, 'physiology': 24491, '715': 24492, '1278': 24493, 'andorrans': 24494, '1607': 24495, 'onward': 24496, 'seu': 24497, "d'urgell": 24498, 'titular': 24499, 'benefitted': 24500, 'hellenic': 24501, 'inequities': 24502, 'keeling': 24503, 'clunie': 24504, 'cocos': 24505, '1841': 24506, 'skillful': 24507, 'meshes': 24508, 'exacted': 24509, 'promissory': 24510, '747s': 24511, 'chopper': 24512, 'locator': 24513, 'renouncing': 24514, 'crisp': 24515, 'overpaid': 24516, 'dilemma': 24517, 'athar': 24518, 'chalit': 24519, 'phukphasuk': 24520, 'thirapat': 24521, 'serirangsan': 24522, 'paulino': 24523, 'matip': 24524, 'ventura': 24525, 'dans': 24526, 'mon': 24527, 'jobim': 24528, 'acronym': 24529, 'expending': 24530, 'deviated': 24531, 'fragility': 24532, 'enrollment': 24533, 'seminars': 24534, 'confuse': 24535, 'mckiernan': 24536, 'unites': 24537, 'taza': 24538, 'dutton': 24539, 'buchan': 24540, 'curbed': 24541, 'victors': 24542, 'peacekeeers': 24543, 'demented': 24544, 'invoke': 24545, 'emission': 24546, 'fledged': 24547, 'kien': 24548, 'premiering': 24549, 'dramatizes': 24550, 'integrating': 24551, 'indifferent': 24552, 'dishonor': 24553, 'pararajasingham': 24554, 'undertake': 24555, 'yuli': 24556, 'ronit': 24557, 'tirosh': 24558, 'reconstructed': 24559, 'pieced': 24560, 'presidencies': 24561, 'goran': 24562, 'tempered': 24563, 'gag': 24564, 'colby': 24565, 'vokey': 24566, 'mcnuggets': 24567, 'abdurrahman': 24568, 'yalcinkaya': 24569, 'unger': 24570, 'budny': 24571, 'marxists': 24572, 'jacqui': 24573, 'headless': 24574, 'fitna': 24575, 'geert': 24576, 'wilders': 24577, 'quotations': 24578, 'bloom': 24579, 'narthiwat': 24580, 'sungai': 24581, 'kolok': 24582, 'carrasquero': 24583, 'kumgang': 24584, 'reunions': 24585, 'mclaughlin': 24586, 'disrespectfully': 24587, 'tabulation': 24588, 'moines': 24589, 'intuitive': 24590, 'talker': 24591, 'dismal': 24592, 'shuttered': 24593, 'humphreys': 24594, 'kozloduy': 24595, 'reinsurer': 24596, 'reinsurers': 24597, 'jetliner': 24598, 'cargolux': 24599, 'utilize': 24600, 'quieter': 24601, 'justly': 24602, 'militaries': 24603, 'dahoud': 24604, 'withstood': 24605, 'tristan': 24606, 'cunha': 24607, 'agriculturally': 24608, 'tasalouti': 24609, 'expectancy': 24610, 'vertical': 24611, 'horizontal': 24612, 'clusters': 24613, 'mitigate': 24614, 'outreach': 24615, '1667': 24616, 'nominally': 24617, '1650': 24618, 'saeedlou': 24619, 'precipice': 24620, 'endeavoring': 24621, 'willful': 24622, 'funny': 24623, 'mane': 24624, 'windy': 24625, 'charming': 24626, 'sisters': 24627, 'blandly': 24628, 'gust': 24629, 'glistening': 24630, 'billiard': 24631, 'embarrassed': 24632, 'woodchopper': 24633, 'besought': 24634, 'mahsouli': 24635, 'thoughtless': 24636, 'deity': 24637, 'salivated': 24638, 'amphitheatre': 24639, 'devour': 24640, 'honourably': 24641, 'claimant': 24642, 'berra': 24643, 'stocky': 24644, 'catcher': 24645, 'miraculous': 24646, 'bothered': 24647, 'miscreants': 24648, 'toad': 24649, 'evicting': 24650, 'query': 24651, "ha'aretz": 24652, 'utilization': 24653, 'interaction': 24654, 'unsolicited': 24655, 'server': 24656, 'disabling': 24657, 'faraa': 24658, 'yamoun': 24659, 'parental': 24660, 'hamstrung': 24661, 'workhorse': 24662, 'dhanush': 24663, 'subhadra': 24664, 'balletic': 24665, 'acrobatic': 24666, 'souq': 24667, 'ghazl': 24668, 'inoculations': 24669, 'rye': 24670, 'bluegrass': 24671, 'mown': 24672, 'fertilized': 24673, 'billed': 24674, 'flares': 24675, 'sprained': 24676, 'saddamists': 24677, 'gianni': 24678, 'piles': 24679, 'kiosks': 24680, 'expensively': 24681, 'rafidain': 24682, 'finsbury': 24683, 'notoriety': 24684, 'earl': 24685, 'columbiadisintegred': 24686, 'showings': 24687, 'disciplines': 24688, 'drugged': 24689, 'headlining': 24690, 'differed': 24691, 'erian': 24692, 'complains': 24693, 'poster': 24694, 'ezzat': 24695, 'itv1': 24696, 'suleimaniyah': 24697, 'salah': 24698, 'reservist': 24699, 'genitals': 24700, 'kerkorian': 24701, 'ghosn': 24702, 'contry': 24703, 'transporation': 24704, '263': 24705, '299': 24706, 'diagnostic': 24707, 'utterly': 24708, 'irrelevant': 24709, 'orjiako': 24710, 'hassanpour': 24711, 'abdolvahed': 24712, 'hiva': 24713, 'botimar': 24714, 'asou': 24715, 'guocong': 24716, 'grossly': 24717, 'jamuna': 24718, '051655093': 24719, 'camille': 24720, 'karolina': 24721, 'sprem': 24722, 'harkleroad': 24723, 'mujahadeen': 24724, 'adjourns': 24725, 'gaston': 24726, 'gaudio': 24727, 'kanaan': 24728, 'parlemannews': 24729, 'alyaty': 24730, 'melange': 24731, 'edison': 24732, 'natama': 24733, 'unavoidable': 24734, 'wannian': 24735, 'whereas': 24736, 'hanyuan': 24737, 'zhengyu': 24738, 'dung': 24739, 'halo': 24740, 'trenches': 24741, 'lining': 24742, 'specialize': 24743, 'validated': 24744, 'si': 24745, 'cumple': 24746, 'mvr': 24747, 'depressions': 24748, '1851': 24749, 'rustlers': 24750, 'pokot': 24751, 'letimalo': 24752, 'combing': 24753, 'bend': 24754, 'wilderness': 24755, 'archipelagoes': 24756, 'aquarium': 24757, 'vlado': 24758, 'cathy': 24759, 'majtenyi': 24760, 'uwezo': 24761, 'theatre': 24762, 'colonize': 24763, 'rum': 24764, 'distilling': 24765, 'croix': 24766, 'mindaugas': 24767, '1386': 24768, '1569': 24769, 'abortive': 24770, 'variations': 24771, 'gonsalves': 24772, 'limbs': 24773, 'notarized': 24774, 'hint': 24775, 'pinions': 24776, 'biceps': 24777, 'pugiliste': 24778, 'ladders': 24779, 'stepstools': 24780, 'demontrators': 24781, 'qidwa': 24782, 'pall': 24783, 'sugars': 24784, 'diet': 24785, 'shelley': 24786, 'schlender': 24787, 'hammond': 24788, 'obamas': 24789, 'tayeb': 24790, 'musbah': 24791, 'pilgrimages': 24792, 'inflicting': 24793, 'khar': 24794, 'hacari': 24795, 'santander': 24796, 'honked': 24797, 'circled': 24798, 'unleaded': 24799, 'kalameh': 24800, 'sabz': 24801, 'refiner': 24802, 'bazian': 24803, 'dahuk': 24804, 'injustices': 24805, 'impulses': 24806, 'disloyalty': 24807, 'olds': 24808, 'juristictions': 24809, 'kimonos': 24810, 'ud': 24811, 'gabla': 24812, 'concocted': 24813, 'animosity': 24814, 'appropriations': 24815, 'jalalzadeh': 24816, 'cai': 24817, 'guo': 24818, 'quiang': 24819, 'gunpowder': 24820, 'designing': 24821, 'firework': 24822, 'guggenheim': 24823, 'yiru': 24824, '7000': 24825, 'tanoh': 24826, 'bouna': 24827, 'abderahmane': 24828, 'vezzaz': 24829, 'tintane': 24830, 'otay': 24831, 'interrogate': 24832, 'moken': 24833, 'banged': 24834, 'offerings': 24835, 'loftis': 24836, 'schilling': 24837, 'asafa': 24838, 'usain': 24839, 'haniyah': 24840, 'farhat': 24841, 'cautioning': 24842, 'rematch': 24843, 'gels': 24844, 'cabins': 24845, 'aidar': 24846, 'sequence': 24847, 'tyson': 24848, 'sonora': 24849, 'anxiously': 24850, 'liwei': 24851, 'naser': 24852, 'earthen': 24853, 'raba': 24854, 'borderline': 24855, 'spearheading': 24856, 'tarso': 24857, 'genro': 24858, 'treasurer': 24859, 'dirceu': 24860, 'reshuffling': 24861, 'timer': 24862, 'gaudy': 24863, 'props': 24864, 'muqudadiyah': 24865, 'silo': 24866, 'kicks': 24867, 'relais': 24868, 'prommegger': 24869, 'heinz': 24870, 'inniger': 24871, 'jaquet': 24872, '460': 24873, 'kohli': 24874, 'daniela': 24875, 'meuli': 24876, 'pomagalski': 24877, 'isabelle': 24878, 'blanc': 24879, 'ibb': 24880, 'jibla': 24881, 'bruno': 24882, 'acholi': 24883, 'aphaluck': 24884, 'bhatiasevi': 24885, '292': 24886, 'vomit': 24887, 'saliva': 24888, 'dhess': 24889, 'shalabi': 24890, 'dagma': 24891, 'djamel': 24892, 'dusseldorf': 24893, 'abdalla': 24894, 'trademarks': 24895, 'counterfeiters': 24896, 'alois': 24897, 'soldaten': 24898, 'cavernous': 24899, 'armory': 24900, 'lekki': 24901, 'decommission': 24902, 'acehnese': 24903, 'resent': 24904, 'burdzhanadze': 24905, '42nd': 24906, 'translates': 24907, 'shaleyste': 24908, 'cart': 24909, 'reconcilation': 24910, 'alimov': 24911, 'berkin': 24912, 'chaika': 24913, 'emigre': 24914, 'pavel': 24915, 'ryaguzov': 24916, 'annualized': 24917, 'jalbire': 24918, 'retires': 24919, 'shearer': 24920, 'initiators': 24921, 'middleman': 24922, 'lupolianski': 24923, 'marshal': 24924, 'slavonia': 24925, 'interlarded': 24926, 'downturns': 24927, 'vagaries': 24928, 'statutory': 24929, 'gateways': 24930, 'vulnerabilities': 24931, 'alleviated': 24932, 'eater': 24933, 'ashamed': 24934, 'admirer': 24935, 'hairs': 24936, 'zealous': 24937, 'dazzling': 24938, 'boisterous': 24939, 'tilt': 24940, 'kalachen': 24941, 'rapporteur': 24942, '557': 24943, '522': 24944, 'enroute': 24945, 'whatsoever': 24946, 'xiamen': 24947, 'kaohsiung': 24948, 'lambasted': 24949, 'unsavory': 24950, 'dunking': 24951, 'filthy': 24952, 'workable': 24953, 'jocic': 24954, 'floodwater': 24955, 'marble': 24956, 'sculpted': 24957, 'voulgarakis': 24958, 'popovkin': 24959, 'jabir': 24960, 'jubran': 24961, 'machinists': 24962, 'insulin': 24963, 'glucose': 24964, 'snipers': 24965, 'carrizales': 24966, 'atal': 24967, 'bihari': 24968, 'bhartiya': 24969, 'earle': 24970, 'prosecutorial': 24971, 'pornographic': 24972, 'passwords': 24973, 'proliferators': 24974, 'teshome': 24975, 'toga': 24976, 'structur': 24977, 'unsaturated': 24978, 'clog': 24979, 'applauding': 24980, 'hubs': 24981, 'artistic': 24982, 'sahar': 24983, 'sepehri': 24984, 'evangelina': 24985, 'elizondo': 24986, 'phantom': 24987, '915': 24988, 'konarak': 24989, 'furnished': 24990, 'spaces': 24991, 'wmo': 24992, 'factored': 24993, 'salaheddin': 24994, 'talib': 24995, 'nader': 24996, 'shaban': 24997, 'seaport': 24998, '1750': 24999, 'lithuanians': 25000, 'chipaque': 25001, 'policymaking': 25002, 'sabirjon': 25003, 'kwaito': 25004, 'derivative': 25005, 'mbalax': 25006, 'youssou': 25007, "n'dour": 25008, 'stiffly': 25009, 'fracturing': 25010, 'inequalities': 25011, 'batna': 25012, 'roulette': 25013, 'irresponsibly': 25014, 'sittwe': 25015, 'birdflu': 25016, 'sparrowhawk': 25017, 'jubilation': 25018, 'unfunded': 25019, 'specializing': 25020, "jama'at": 25021, "qu'ran": 25022, 'nahda': 25023, 'rusafa': 25024, 'latifiyah': 25025, 'aps': 25026, 'intercede': 25027, '151': 25028, 'austere': 25029, 'pachyderm': 25030, 'foments': 25031, 'perception': 25032, 'favoritism': 25033, 'crimp': 25034, 'arbour': 25035, 'pastoral': 25036, 'nomadism': 25037, 'sedentary': 25038, 'quarreled': 25039, 'literate': 25040, 'indebtedness': 25041, 'saa': 25042, 'duhalde': 25043, 'bottomed': 25044, 'audacious': 25045, 'understating': 25046, 'exacerbating': 25047, 'dominica': 25048, 'geothermal': 25049, 'lightening': 25050, 'luxuries': 25051, 'overburdened': 25052, 'intensely': 25053, 'shining': 25054, 'quarreling': 25055, 'hopped': 25056, 'antagonists': 25057, 'comb': 25058, 'fahim': 25059, 'emroz': 25060, 'didadgah': 25061, 'rodong': 25062, 'wenesday': 25063, 'organizational': 25064, 'faulted': 25065, 'responders': 25066, 'gijira': 25067, 'fdic': 25068, 'tartous': 25069, 'rainstorm': 25070, 'bean': 25071, 'flannel': 25072, 'sweaters': 25073, 'smells': 25074, 'patat': 25075, 'jabor': 25076, 'dalian': 25077, 'tian': 25078, 'neng': 25079, 'meishan': 25080, 'vent': 25081, 'reformer': 25082, 'lukewarm': 25083, 'mosleshi': 25084, '637': 25085, 'texmelucan': 25086, 'ayar': 25087, 'postpones': 25088, 'lazio': 25089, 'fiorentina': 25090, 'serie': 25091, 'handpicking': 25092, 'relegation': 25093, 'demotions': 25094, '164': 25095, 'fulani': 25096, 'herdsmen': 25097, 'creationism': 25098, 'gymnastics': 25099, 'healed': 25100, 'molucca': 25101, 'ternate': 25102, 'vivanews': 25103, 'rotator': 25104, 'cuff': 25105, 'keywords': 25106, 'chat': 25107, 'accessed': 25108, 'storing': 25109, 'wiretap': 25110, 'pbsnewshour': 25111, 'alternates': 25112, 'mighty': 25113, 'najjar': 25114, 'meza': 25115, 'oleksander': 25116, 'horobets': 25117, 'despicable': 25118, 'wallet': 25119, 'rfid': 25120, 'biographical': 25121, 'bezsmertniy': 25122, 'terrence': 25123, 'corina': 25124, 'morariu': 25125, 'mashona': 25126, 'qutbi': 25127, 'toluca': 25128, 'alistair': 25129, '367': 25130, '323': 25131, 'aristolbulo': 25132, 'isturiz': 25133, 'timergarah': 25134, 'sibbi': 25135, 'streamlines': 25136, 'siphon': 25137, 'pullouts': 25138, 'rouen': 25139, 'manifestations': 25140, 'disproportionally': 25141, 'overrepresented': 25142, 'pekanbara': 25143, 'islamophobic': 25144, 'underdocumented': 25145, 'underreported': 25146, 'hexaflouride': 25147, 'tackling': 25148, 'tindouf': 25149, 'yudhyono': 25150, 'lekima': 25151, 'guanglie': 25152, 'mesopotamia': 25153, 'abnormalities': 25154, 'haves': 25155, 'nots': 25156, 'keith': 25157, 'snorted': 25158, 'ingesting': 25159, 'cremated': 25160, 'cared': 25161, 'bert': 25162, 'famously': 25163, 'petitions': 25164, 'dissenting': 25165, 'edmund': 25166, 'vacated': 25167, 'strikingly': 25168, 'sews': 25169, 'devra': 25170, 'robitaille': 25171, 'fliers': 25172, 'washingtonians': 25173, 'mousa': 25174, "'80s": 25175, 'irrelevent': 25176, 'valuables': 25177, 'statments': 25178, 'baghaichhari': 25179, 'gravesite': 25180, 'khanty': 25181, 'mansiisk': 25182, 'padded': 25183, 'burdensome': 25184, 'po': 25185, 'psl': 25186, 'straddling': 25187, 'overexploitation': 25188, 'deepwater': 25189, 'kjetil': 25190, 'aamodt': 25191, '110474537': 25192, 'concessionary': 25193, 'ladder': 25194, 'deepened': 25195, 'parity': 25196, '110625': 25197, 'maaouya': 25198, 'mauritanians': 25199, 'moor': 25200, 'berber': 25201, "qa'ida": 25202, 'aqim': 25203, 'combi': 25204, 'jar': 25205, 'smeared': 25206, 'suffocated': 25207, 'expiring': 25208, 'fondles': 25209, 'nurtures': 25210, 'hates': 25211, 'neglects': 25212, 'caressed': 25213, 'smothered': 25214, 'nurtured': 25215, 'banjo': 25216, 'plotters': 25217, 'alishar': 25218, 'mirajuddin': 25219, 'giro': 25220, 'rignot': 25221, 'kenedy': 25222, 'neal': 25223, 'unmonitored': 25224, 'josslyn': 25225, 'aberle': 25226, 'incarceration': 25227, 'readied': 25228, 'homayun': 25229, 'khamail': 25230, 'reassess': 25231, 'wnba': 25232, 'playoff': 25233, 'hawi': 25234, 'peeling': 25235, 'rides': 25236, 'khao': 25237, 'lak': 25238, 'trumpeting': 25239, 'trunks': 25240, 'calamities': 25241, 'perishable': 25242, 'dodger': 25243, 'alison': 25244, 'forges': 25245, 'sportscar': 25246, 'irwindale': 25247, 'speedway': 25248, 'deuce': 25249, 'bigalow': 25250, 'sadek': 25251, 'enzos': 25252, 'brewing': 25253, 'bottler': 25254, 'brewer': 25255, 'coltan': 25256, 'cassiterite': 25257, 'diaoyu': 25258, 'unguarded': 25259, 'perimeter': 25260, 'leftover': 25261, 'retroactively': 25262, 'shuffle': 25263, '541': 25264, 'proportions': 25265, 'dharmendra': 25266, 'rajaratnam': 25267, 'sasa': 25268, 'radak': 25269, 'ovcara': 25270, 'saidati': 25271, 'publishers': 25272, 'agnes': 25273, 'uwimana': 25274, 'disobedience': 25275, '462': 25276, '745': 25277, 'arenas': 25278, 'uladi': 25279, 'mazlan': 25280, 'jusoh': 25281, 'resonated': 25282, 'deepens': 25283, 'katarina': 25284, 'shinboue': 25285, 'leanne': 25286, 'lubiani': 25287, '87th': 25288, 'a380': 25289, 'midair': 25290, 'rolls': 25291, 'royce': 25292, 'turbines': 25293, 'volleys': 25294, 'bani': 25295, 'navarre': 25296, 'corpsman': 25297, 'kor': 25298, 'affirmation': 25299, 'yankham': 25300, 'kengtung': 25301, 'countdown': 25302, 'manan': 25303, '452': 25304, 'edible': 25305, 'kavkazcenter': 25306, 'khalim': 25307, 'sadulayev': 25308, 'rivaling': 25309, 'telethons': 25310, 'unesco': 25311, 'parthenon': 25312, '392': 25313, '485': 25314, 'clamped': 25315, 'drywall': 25316, 'helipad': 25317, 'kruif': 25318, 'bedfordshire': 25319, 'bedford': 25320, 'skyjackers': 25321, '726': 25322, 'bestseller': 25323, 'nanking': 25324, 'pesticides': 25325, 'residue': 25326, 'statistically': 25327, 'kennedys': 25328, 'jacqueline': 25329, 'onassis': 25330, 'stillborn': 25331, 'repose': 25332, 'hkd': 25333, 'konstanz': 25334, 'suitcases': 25335, 'dortmund': 25336, 'koblenz': 25337, 'kazmi': 25338, 'outnumbering': 25339, '8000': 25340, 'cisplatin': 25341, 'cervix': 25342, 'prolonging': 25343, 'hina': 25344, 'jutarnji': 25345, 'bats': 25346, 'diligently': 25347, 'shy': 25348, 'casual': 25349, 'grinstein': 25350, 'rafat': 25351, 'costliest': 25352, 'burdens': 25353, 'downsizing': 25354, 'cooled': 25355, 'psa': 25356, 'nagorno': 25357, 'karabakh': 25358, 'chiefdoms': 25359, 'springboard': 25360, '1844': 25361, 'dominicans': 25362, 'leonidas': 25363, 'trujillo': 25364, '1870': 25365, 'circumscribed': 25366, 'lateran': 25367, 'concordat': 25368, 'primacy': 25369, 'profess': 25370, 'ungrudgingly': 25371, 'commiserated': 25372, 'surely': 25373, 'celma': 25374, 'illustrious': 25375, 'inhabitant': 25376, 'mc': 25377, "'70s": 25378, 'bullitt': 25379, 'papillon': 25380, 'memorabilia': 25381, 'boyhood': 25382, 'yung': 25383, 'meaningless': 25384, 'graz': 25385, 'nozari': 25386, 'hamshahri': 25387, 'shargh': 25388, 'chow': 25389, 'chilled': 25390, 'fuzhou': 25391, 'jessica': 25392, 'fiance': 25393, 'xtr': 25394, 'ludford': 25395, 'hanjiang': 25396, 'mashjid': 25397, 'jalna': 25398, 'osaid': 25399, 'falluji': 25400, 'najdi': 25401, 'newsletter': 25402, 'sawt': 25403, 'disseminate': 25404, 'jihadist': 25405, 'khaleeq': 25406, 'tumbling': 25407, 'kel': 25408, 'seun': 25409, 'fela': 25410, 'nnamdi': 25411, 'moweta': 25412, 'leo': 25413, 'cheaply': 25414, 'undercutting': 25415, 'bomblets': 25416, 'hyper': 25417, 'clearances': 25418, 'assistants': 25419, 'dubs': 25420, 'discriminating': 25421, 'disregarding': 25422, 'laguna': 25423, 'aynin': 25424, 'haaretzthat': 25425, 'zigana': 25426, 'gumushane': 25427, 'surround': 25428, 'intersect': 25429, 'semiarid': 25430, 'arla': 25431, 'loses': 25432, 'chretien': 25433, 'secularist': 25434, 'koksal': 25435, 'toptan': 25436, 'rim': 25437, 'disowning': 25438, 'notre': 25439, 'dame': 25440, 'patriarchal': 25441, 'khalayleh': 25442, 'doomsday': 25443, 'gobe': 25444, 'squadrons': 25445, 'uprooting': 25446, 'conclusive': 25447, 'waded': 25448, 'unprovoked': 25449, 'hamidreza': 25450, 'estefan': 25451, 'thalia': 25452, 'jamrud': 25453, 'venevision': 25454, 'ovidio': 25455, 'cuesta': 25456, 'baramullah': 25457, 'srinigar': 25458, 'assembling': 25459, 'bikaner': 25460, 'sketch': 25461, 'amos': 25462, 'esmat': 25463, 'klain': 25464, '599': 25465, 'emanate': 25466, 'dahl': 25467, 'froeshaug': 25468, '4x5': 25469, 'atiku': 25470, 'lai': 25471, 'immoral': 25472, 'yambio': 25473, 'seyi': 25474, 'memene': 25475, 'kerosene': 25476, 'veil': 25477, 'ramdi': 25478, 'deepak': 25479, 'gurung': 25480, 'voyages': 25481, 'bago': 25482, 'kyauk': 25483, 'kanyutkwin': 25484, 'allert': 25485, '428': 25486, 'sounding': 25487, 'kleiner': 25488, 'caufield': 25489, 'byers': 25490, 'blossomed': 25491, 'machakos': 25492, 'drank': 25493, 'makutano': 25494, 'methanol': 25495, "chang'aa": 25496, 'palpa': 25497, 'bitlis': 25498, 'pejak': 25499, 'cessation': 25500, 'hawaiian': 25501, 'papahanaumokuakea': 25502, 'terrestrial': 25503, 'corals': 25504, 'shellfish': 25505, 'seabirds': 25506, 'insects': 25507, 'colon': 25508, 'traverse': 25509, 'firsts': 25510, 'aruban': 25511, 'dip': 25512, 'frequenting': 25513, 'lamentation': 25514, 'fuss': 25515, 'batsmen': 25516, '493': 25517, 'intimidating': 25518, 'intra': 25519, 'sucre': 25520, 'olivier': 25521, 'rochus': 25522, 'ramstein': 25523, 'pontifical': 25524, 'poupard': 25525, 'castel': 25526, 'gandolfo': 25527, 'theorized': 25528, 'othman': 25529, 'swank': 25530, 'portrayal': 25531, 'katherine': 25532, 'hepburn': 25533, 'freeman': 25534, 'shaloub': 25535, 'orbach': 25536, 'ensemble': 25537, 'quirky': 25538, 'jpmorgan': 25539, 'midfielder': 25540, 'ramming': 25541, 'wael': 25542, 'rubaei': 25543, 'izzariya': 25544, 'embryos': 25545, 'preclude': 25546, 'underlings': 25547, 'coercion': 25548, 'impropriety': 25549, '127': 25550, 'fadela': 25551, 'chaib': 25552, 'sufferer': 25553, '315': 25554, 'kandili': 25555, 'anatolianews': 25556, 'erzurum': 25557, 'governorates': 25558, 'qods': 25559, 'shorten': 25560, 'editorials': 25561, 'gye': 25562, 'wafd': 25563, 'baleno': 25564, 'fatigues': 25565, 'revote': 25566, 'picnicking': 25567, 'aleg': 25568, 'qadis': 25569, 'na': 25570, 'jarmila': 25571, 'gajdosova': 25572, 'ting': 25573, 'tiantian': 25574, 'scandalized': 25575, 'anymore': 25576, 'mains': 25577, 'mechanics': 25578, 'ana': 25579, 'rauchenstein': 25580, 'sabaa': 25581, 'spelled': 25582, 'guzzling': 25583, 'lobes': 25584, 'sedatives': 25585, 'soir': 25586, 'acquaint': 25587, 'inflammable': 25588, 'consumes': 25589, 'philanthropy': 25590, 'loopholes': 25591, 'hutton': 25592, 'forgivable': 25593, 'faras': 25594, 'aqidi': 25595, 'joanne': 25596, 'fahad': 25597, 'cured': 25598, 'melanne': 25599, 'epidemics': 25600, 'nanjing': 25601, 'haerbaling': 25602, 'divulge': 25603, 'crafted': 25604, 'pennies': 25605, 'tsholotsho': 25606, 'chinotimba': 25607, 'inbalance': 25608, 'madero': 25609, 'otis': 25610, 'powerlines': 25611, 'whiskey': 25612, 'abolishing': 25613, 'commutes': 25614, 'footprints': 25615, 'khuzestan': 25616, 'saudia': 25617, 'jules': 25618, 'guere': 25619, 'influnce': 25620, 'majzoub': 25621, 'samkelo': 25622, 'mokhine': 25623, 'jehangir': 25624, 'mirza': 25625, 'enthusiastically': 25626, 'acknowledgment': 25627, 'thrifty': 25628, 'handmade': 25629, 'polynesians': 25630, 'micronesians': 25631, 'vanilla': 25632, 'tongan': 25633, 'upturn': 25634, 'tanganyika': 25635, 'minimizing': 25636, 'negation': 25637, '1010': 25638, 'tifa': 25639, 'fashioning': 25640, 'thrush': 25641, 'intently': 25642, 'upwards': 25643, 'swoon': 25644, 'purposed': 25645, 'unawares': 25646, 'snares': 25647, 'ahmedou': 25648, 'ashayier': 25649, 'kouk': 25650, "sa'dun": 25651, 'hamduni': 25652, 'hmas': 25653, 'fitted': 25654, 'turnbull': 25655, 'awantipora': 25656, 'saldanha': 25657, 'lateef': 25658, 'adegbite': 25659, 'tenets': 25660, 'darkest': 25661, 'zarqwai': 25662, 'scrubbed': 25663, 'hangar': 25664, 'annex': 25665, 'sabri': 25666, 'annexing': 25667, '335': 25668, 'treetops': 25669, 'compliments': 25670, 'obaidullah': 25671, 'lal': 25672, 'subcontinent': 25673, 'patched': 25674, 'vassily': 25675, 'slandered': 25676, 'yoshie': 25677, 'sato': 25678, 'yokosuka': 25679, 'kitty': 25680, 'assessors': 25681, 'vimpelcom': 25682, 'jaunpur': 25683, 'patna': 25684, 'hexogen': 25685, 'unclaimed': 25686, 'bavaria': 25687, 'allay': 25688, 'aggressions': 25689, '58th': 25690, 'wrecked': 25691, 'shadows': 25692, 'shines': 25693, 'aijalon': 25694, 'unhindered': 25695, 'kasab': 25696, 'faizullah': 25697, 'akhbar': 25698, 'youm': 25699, 'sects': 25700, 'jamia': 25701, 'americanism': 25702, '049': 25703, 'stepan': 25704, 'georgi': 25705, 'parvanov': 25706, 'prolongs': 25707, 'normalized': 25708, 'hennadiy': 25709, 'vasylyev': 25710, 'epa': 25711, 'ecweru': 25712, 'swahili': 25713, 'mulongoti': 25714, 'bududa': 25715, 'header': 25716, 'khedira': 25717, '82nd': 25718, 'mesut': 25719, 'oezil': 25720, 'edinson': 25721, 'cavani': 25722, 'equalized': 25723, 'forlan': 25724, 'marcell': 25725, 'jansen': 25726, 'resettling': 25727, 'unsubstantiated': 25728, 'categorized': 25729, 'shegag': 25730, 'karo': 25731, 'hudaidah': 25732, '411': 25733, 'badoer': 25734, 'maranello': 25735, 'reggio': 25736, 'emilia': 25737, 'venice': 25738, 'ignites': 25739, 'scoop': 25740, 'footprint': 25741, 'subsurface': 25742, '679': 25743, '447': 25744, 'buyukanit': 25745, 'wadowice': 25746, 'knees': 25747, 'worshipped': 25748, 'readies': 25749, 'gurirab': 25750, 'redistribution': 25751, 'sensing': 25752, 'petting': 25753, 'binyamina': 25754, 'symptom': 25755, 'chilling': 25756, 'bullying': 25757, 'deprive': 25758, 'mastering': 25759, 'wabho': 25760, 'electricial': 25761, 'trusting': 25762, 'triumf': 25763, 'vikings': 25764, 'easternmost': 25765, 'kwajalein': 25766, 'usaka': 25767, '1881': 25768, 'paroled': 25769, 'offender': 25770, 'joachim': 25771, 'ruecker': 25772, 'repressing': 25773, 'unmatched': 25774, 'zine': 25775, 'abidine': 25776, 'tunis': 25777, 'ghannouchi': 25778, "m'bazaa": 25779, 'fluctuated': 25780, 'terrible': 25781, 'zoological': 25782, 'tales': 25783, 'graze': 25784, 'tidings': 25785, 'whence': 25786, 'entertained': 25787, 'boatmen': 25788, 'emotion': 25789, 'yielding': 25790, 'gasped': 25791, 'wretched': 25792, 'perilous': 25793, 'renoun': 25794, 'elves': 25795, 'subordinate': 25796, 'leonardo': 25797, 'overaggressive': 25798, 'ching': 25799, 'hsi': 25800, 'mistreats': 25801, 'baltim': 25802, 'kafr': 25803, 'zigazag': 25804, 'entebbe': 25805, 'jovica': 25806, 'simatovic': 25807, 'insidious': 25808, 'nihilism': 25809, 'fanatic': 25810, 'copts': 25811, 'nightly': 25812, 'renegotiating': 25813, 'cone': 25814, 'foam': 25815, 'disintegration': 25816, 'goldsmith': 25817, "u'zayra": 25818, 'larkin': 25819, 'khayber': 25820, 'hyuck': 25821, 'format': 25822, 'worthless': 25823, 'dae': 25824, 'hamash': 25825, 'levied': 25826, 'prescriptions': 25827, 'somavia': 25828, 'spelling': 25829, 'sayings': 25830, 'borjomi': 25831, 'jaca': 25832, 'pyeongchang': 25833, 'salzburg': 25834, 'questionnaire': 25835, 'accommodations': 25836, '6th': 25837, 'buddhism': 25838, 'anuradhapura': 25839, 'polonnaruwa': 25840, '1070': 25841, '1200': 25842, 'mcchrystal': 25843, 'pinerolo': 25844, '1500s': 25845, 'glides': 25846, 'circular': 25847, 'briquettes': 25848, 'norpro': 25849, 'xingguang': 25850, '1796': 25851, '1802': 25852, 'alain': 25853, 'pellegrini': 25854, 'ceylon': 25855, 'lubero': 25856, 'guehenno': 25857, 'eelam': 25858, 'overshadow': 25859, 'kofoworola': 25860, 'anguish': 25861, 'cual': 25862, 'ojeda': 25863, 'borges': 25864, 'proficiency': 25865, 'indoctrinate': 25866, 'bounds': 25867, 'weekends': 25868, 'gravel': 25869, 'kihonge': 25870, 'supervising': 25871, 'wreaths': 25872, 'yugoslavian': 25873, 'dacic': 25874, 'colleen': 25875, 'drillings': 25876, 'pedraz': 25877, 'taras': 25878, 'portsyuk': 25879, 'buenaventura': 25880, 'chieftains': 25881, 'waitangi': 25882, 'npa': 25883, '1843': 25884, 'tsotne': 25885, 'zviad': 25886, 'hajan': 25887, 'lapsed': 25888, 'printemps': 25889, '464': 25890, 'dispatching': 25891, 'vampire': 25892, 'refill': 25893, 'trespassing': 25894, 'seini': 25895, 'leniency': 25896, 'rebuke': 25897, 'khamas': 25898, 'hajem': 25899, 'bole': 25900, 'nihal': 25901, 'denigrates': 25902, 'ruble': 25903, 'steward': 25904, 'codes': 25905, 'göteborg': 25906, 'krisztina': 25907, 'nagy': 25908, 'tiechui': 25909, 'paramedics': 25910, 'irrawady': 25911, 'fazul': 25912, 'stanezai': 25913, 'impervious': 25914, 'buster': 25915, 'lioness': 25916, 'harden': 25917, 'drift': 25918, 'easley': 25919, 'laszlo': 25920, 'solyom': 25921, 'mufti': 25922, 'shuttles': 25923, 'jalula': 25924, 'yourselves': 25925, 'amerli': 25926, 'petrol': 25927, 'invariably': 25928, 'passers': 25929, 'leveraged': 25930, 'maltese': 25931, 'faroese': 25932, 'moorish': 25933, 'dynasties': 25934, "sa'adi": 25935, '1578': 25936, 'alaouite': 25937, '1860': 25938, 'internationalized': 25939, 'tangier': 25940, 'bicameral': 25941, 'moderately': 25942, 'hobbles': 25943, 'overspending': 25944, 'overborrowing': 25945, 'stables': 25946, 'licked': 25947, 'dainty': 25948, 'blinking': 25949, 'stroked': 25950, 'commenced': 25951, 'prancing': 25952, 'pitchforks': 25953, 'clumsy': 25954, 'jesting': 25955, 'joke': 25956, 'summers': 25957, 'natured': 25958, 'shibis': 25959, 'llasa': 25960, 'radhia': 25961, 'achouri': 25962, 'trily': 25963, 'showdowns': 25964, 'reprimands': 25965, 'sanaa': 25966, 'coleand': 25967, 'supertanker': 25968, 'limburg': 25969, 'supervises': 25970, 'dmitrivev': 25971, 'prestwick': 25972, 'jagdish': 25973, 'yongbyon': 25974, 'renaissance': 25975, 'kedallah': 25976, 'younous': 25977, 'purging': 25978, 'demeans': 25979, 'gratuitous': 25980, 'instructional': 25981, 'kuta': 25982, 'taormina': 25983, 'suggestive': 25984, 'taboos': 25985, 'menstrual': 25986, 'unidentifed': 25987, 'provocateurs': 25988, '385': 25989, 'falsifying': 25990, 'shootouts': 25991, 'eastward': 25992, 'alternately': 25993, 'hendrik': 25994, 'taatgen': 25995, 'ambrosio': 25996, 'intakes': 25997, 'wastewater': 25998, 'yingde': 25999, 'reservoirs': 26000, 'neurological': 26001, 'knockout': 26002, 'strongmen': 26003, 'yardenna': 26004, 'dwelling': 26005, 'courtyard': 26006, 'annunciation': 26007, 'copenhagen': 26008, 'enacting': 26009, 'snub': 26010, 'siachen': 26011, 'kalikot': 26012, 'seiken': 26013, 'spouses': 26014, 'torres': 26015, 'yake': 26016, '867': 26017, 'headbands': 26018, '577': 26019, '969': 26020, '729': 26021, 'germaine': 26022, 'fanatics': 26023, 'twisted': 26024, 'holdouts': 26025, 'aurobindo': 26026, 'pharma': 26027, 'lamivudine': 26028, 'zidovudine': 26029, 'regimen': 26030, 'patents': 26031, 'sharpened': 26032, 'daley': 26033, 'rahm': 26034, 'repays': 26035, 'liempde': 26036, 'nuriye': 26037, 'kesbir': 26038, 'climbs': 26039, 'cot': 26040, 'breasts': 26041, 'exam': 26042, 'malakhel': 26043, 'osmani': 26044, 'aubenas': 26045, 'reopens': 26046, 'xinjuan': 26047, 'bruises': 26048, 'georg': 26049, 'trondheim': 26050, 'vague': 26051, 'bernt': 26052, 'pedophilia': 26053, 'takeifa': 26054, 'keita': 26055, 'shyrock': 26056, '675': 26057, 'architectural': 26058, 'burgeoning': 26059, 'hinting': 26060, 'managua': 26061, 'disavowed': 26062, 'murr': 26063, 'thuggery': 26064, 'michuki': 26065, 'bitten': 26066, 'neskovic': 26067, 'bijeljina': 26068, 'amagasaki': 26069, 'osaka': 26070, 'milososki': 26071, 'beneficial': 26072, 'mia': 26073, 'crvenkovski': 26074, 'gruevski': 26075, 'swerved': 26076, 'althing': 26077, '930': 26078, 'askja': 26079, 'pattle': 26080, 'woody': 26081, '1788': 26082, 'islanders': 26083, 'mechanized': 26084, 'rutte': 26085, 'industrialization': 26086, 'soles': 26087, 'idols': 26088, 'unlucky': 26089, 'pedestal': 26090, 'hardly': 26091, 'sultans': 26092, 'splendour': 26093, 'dukeness': 26094, 'liege': 26095, 'gorgeous': 26096, 'jewel': 26097, 'gleaming': 26098, 'badgesty': 26099, 'catarrh': 26100, 'rabbit': 26101, 'obstructs': 26102, 'cheyenne': 26103, 'showers': 26104, 'wednesdays': 26105, 'upholding': 26106, 'abbasali': 26107, 'kadkhodai': 26108, 'tahreer': 26109, 'peshmerga': 26110, 'christman': 26111, 'starved': 26112, 'menoufia': 26113, '43nd': 26114, 'unasur': 26115, 'basile': 26116, 'casmoussa': 26117, 'guenael': 26118, 'rodier': 26119, 'attributing': 26120, 'colonia': 26121, 'cachet': 26122, 'burrow': 26123, 'gino': 26124, 'casassa': 26125, 'repeals': 26126, 'segun': 26127, 'odegbami': 26128, 'nfa': 26129, 'salisu': 26130, 'hendarso': 26131, 'sumatran': 26132, 'religiously': 26133, '269': 26134, 'alienate': 26135, 'hazel': 26136, 'blears': 26137, 'khalidiyah': 26138, 'bakwa': 26139, 'ziba': 26140, 'sharq': 26141, 'awsat': 26142, 'tempers': 26143, 'designating': 26144, 'excited': 26145, 'motel': 26146, 'dietary': 26147, 'hauled': 26148, 'pragmatists': 26149, 'copes': 26150, 'revisited': 26151, 'stargazers': 26152, 'wd5': 26153, 'megaton': 26154, 'exhumation': 26155, 'glorious': 26156, 'johns': 26157, 'fabrizio': 26158, 'kiffa': 26159, 'motorcyclists': 26160, 'charter97': 26161, 'despres': 26162, 'ktm': 26163, '8956': 26164, 'ceremon': 26165, 'flabbergasted': 26166, 'asparagus': 26167, 'acidic': 26168, 'vapor': 26169, 'cassettes': 26170, 'augment': 26171, 'mcbride': 26172, 'vocalist': 26173, 'nichols': 26174, 'craig': 26175, 'wiseman': 26176, 'peformed': 26177, 'mcgraw': 26178, 'gretchen': 26179, 'horizon': 26180, 'kris': 26181, 'kristofferson': 26182, 'zamani': 26183, 'speedboats': 26184, 'coherent': 26185, 'nyein': 26186, 'ko': 26187, 'naing': 26188, 'appropriated': 26189, 'fadhila': 26190, 'perverted': 26191, 'cctv': 26192, 'nozzles': 26193, 'salomi': 26194, 'reads': 26195, 'blackwater': 26196, 'ire': 26197, 'benedicto': 26198, 'commercio': 26199, 'zevallos': 26200, 'dee': 26201, 'boersma': 26202, 'serfdom': 26203, 'dumitru': 26204, 'newsroom': 26205, 'formalities': 26206, 'dens': 26207, 'airdrop': 26208, 'ingmar': 26209, 'actresses': 26210, 'ullman': 26211, 'bibi': 26212, 'andersson': 26213, 'faro': 26214, 'strawberries': 26215, 'madness': 26216, 'tinged': 26217, 'melancholy': 26218, 'humor': 26219, 'iconic': 26220, 'knight': 26221, 'bylaw': 26222, 'thg': 26223, 'tetrahydrogestrinone': 26224, 'boa': 26225, 'mailbox': 26226, 'measurer': 26227, 'machining': 26228, 'invent': 26229, 'braille': 26230, 'nanotechnology': 26231, 'cybersecurity': 26232, 'grid': 26233, 'standardized': 26234, 'hoses': 26235, 'mummified': 26236, 'earthenware': 26237, 'teir': 26238, 'atoun': 26239, 'habsburg': 26240, 'emmerick': 26241, 'visions': 26242, 'mel': 26243, 'gibson': 26244, 'ludovica': 26245, 'angelis': 26246, 'beatification': 26247, 'directorate': 26248, 'touted': 26249, 'handovers': 26250, 'vigilante': 26251, 'edwin': 26252, 'workload': 26253, 'principlists': 26254, 'reem': 26255, 'zeid': 26256, 'khazaal': 26257, 'gheorghe': 26258, 'flutur': 26259, 'caraorman': 26260, 'huy': 26261, 'nga': 26262, 'locus': 26263, 'moldovan': 26264, 'dniester': 26265, 'slavic': 26266, 'transnistria': 26267, 'pcrm': 26268, 'reconstituted': 26269, 'barren': 26270, 'heyday': 26271, '1755': 26272, 'crocodile': 26273, 'murderer': 26274, 'bosom': 26275, 'coldness': 26276, 'grin': 26277, 'coals': 26278, 'pleasures': 26279, 'thawed': 26280, 'civilly': 26281, 'foreshadows': 26282, '940': 26283, 'bedingfield': 26284, 'dreaming': 26285, 'beatboxers': 26286, 'dispenses': 26287, 'anybody': 26288, 'kapondi': 26289, 'joshua': 26290, 'kutuny': 26291, 'wilfred': 26292, 'machage': 26293, 'dragmulla': 26294, 'hurl': 26295, 'marts': 26296, 'elvira': 26297, 'nabiullina': 26298, 'gucht': 26299, 'shoaib': 26300, 'festus': 26301, 'okonkwo': 26302, 'attends': 26303, 'amed': 26304, 'snag': 26305, 'exclusively': 26306, 'loophole': 26307, 'kordofan': 26308, 'sla': 26309, 'ghubaysh': 26310, 'federations': 26311, 'edel': 26312, 'checkup': 26313, 'poet': 26314, 'rivero': 26315, 'espinoso': 26316, 'chepe': 26317, 'conspired': 26318, 'gulzar': 26319, 'nabeel': 26320, 'shamin': 26321, 'uddin': 26322, 'treasures': 26323, 'dakhlallah': 26324, 'ideals': 26325, 'brice': 26326, 'flashlight': 26327, 'interrogator': 26328, 'azmi': 26329, 'enkhbayar': 26330, 'cali': 26331, 'barco': 26332, 'bogata': 26333, 'headache': 26334, 'tagging': 26335, 'mastered': 26336, 'doused': 26337, 'blizzards': 26338, 'herders': 26339, 'afder': 26340, 'liban': 26341, 'gode': 26342, 'deteriorate': 26343, 'atomstroyexport': 26344, 'blowtorch': 26345, 'deteriorates': 26346, 'kisangani': 26347, 'kidnapper': 26348, 'temur': 26349, 'pasted': 26350, 'krivtsov': 26351, 'iraqna': 26352, 'posh': 26353, 'makati': 26354, 'deluxe': 26355, 'ghorian': 26356, 'navfor': 26357, 'harardhere': 26358, 'sacrificial': 26359, 'ubt': 26360, 'trampling': 26361, 'keck': 26362, 'vortices': 26363, 'teenaged': 26364, 'mastung': 26365, 'naushki': 26366, 'wellhead': 26367, 'cawthorne': 26368, 'dignified': 26369, 'emails': 26370, 'flakus': 26371, 'weird': 26372, 'mingora': 26373, 'neolithic': 26374, 'republished': 26375, 'alok': 26376, 'lauded': 26377, 'palocci': 26378, 'rato': 26379, 'ringing': 26380, 'tet': 26381, 'hermosa': 26382, 'myitsone': 26383, 'kachin': 26384, 'humam': 26385, 'hammoudi': 26386, 'narayan': 26387, 'huerta': 26388, 'knut': 26389, 'elfriede': 26390, 'jelinek': 26391, 'irreparably': 26392, 'horace': 26393, 'engdahl': 26394, 'madison': 26395, 'tinted': 26396, 'glasses': 26397, 'lennon': 26398, 'lyricist': 26399, 'taupin': 26400, 'serenaded': 26401, 'whoopie': 26402, 'goldberg': 26403, 'portraying': 26404, 'shaheen': 26405, 'nexus': 26406, 'cheecha': 26407, 'watni': 26408, 'corvettes': 26409, 'overruns': 26410, 'sipah': 26411, 'sahaba': 26412, 'husbandry': 26413, 'conscientious': 26414, 'entrepreneurship': 26415, 'supplements': 26416, 'pretended': 26417, 'lameness': 26418, 'pulse': 26419, 'mouthful': 26420, 'vexation': 26421, 'sup': 26422, 'flagon': 26423, 'insert': 26424, 'requital': 26425, 'pager': 26426, 'anesthetist': 26427, 'stethoscope': 26428, 'dangling': 26429, 'hardfought': 26430, '286': 26431, '252': 26432, 'tuncay': 26433, 'heroic': 26434, 'tabinda': 26435, 'kamiya': 26436, 'outfielders': 26437, 'sammy': 26438, 'sosa': 26439, 'leagues': 26440, 'hander': 26441, 'strikeouts': 26442, 'cy': 26443, 'rippled': 26444, 'deauville': 26445, 'kouguell': 26446, 'padang': 26447, 'daraga': 26448, 'durian': 26449, 'catanduanes': 26450, 'enezi': 26451, 'kut': 26452, 'rescind': 26453, 'ryon': 26454, 'dawoud': 26455, 'rasheed': 26456, 'thawing': 26457, 'predictor': 26458, 'stump': 26459, 'carryover': 26460, 'candlemas': 26461, 'wwii': 26462, 'blared': 26463, 'dabbagh': 26464, 'keynote': 26465, 'complementary': 26466, 'genome': 26467, 'dripped': 26468, 'notion': 26469, 'atttacks': 26470, 'hurriya': 26471, 'kufa': 26472, 'boodai': 26473, 'swamped': 26474, 'tearfund': 26475, 'andrhra': 26476, 'ar': 26477, 'rutbah': 26478, 'khurram': 26479, 'mehran': 26480, 'msn': 26481, 'bing': 26482, 'sufi': 26483, 'sufis': 26484, 'heretics': 26485, 'turkman': 26486, 'qaratun': 26487, 'khairuddin': 26488, '590': 26489, 'yair': 26490, 'landlords': 26491, 'mykolaiv': 26492, 'chasing': 26493, 'loyalist': 26494, 'lvf': 26495, 'tyrants': 26496, 'oppress': 26497, 'rocker': 26498, 'springsteen': 26499, 'repertoire': 26500, 'idolwinner': 26501, 'refrigerated': 26502, 'abass': 26503, 'barbero': 26504, 'neutralizing': 26505, 'tessa': 26506, 'jowell': 26507, 'expose': 26508, 'baojing': 26509, 'jianhao': 26510, 'blackmailing': 26511, 'maurice': 26512, '88th': 26513, 'somme': 26514, 'triomphe': 26515, 'pongpa': 26516, 'wasser': 26517, 'backup': 26518, 'irreconcilable': 26519, 'tracy': 26520, 'lebron': 26521, 'pacers': 26522, 'croshere': 26523, 'steals': 26524, 'dunleavy': 26525, 'faridullah': 26526, 'kani': 26527, 'wam': 26528, 'interivew': 26529, 'jianzhong': 26530, 'surkh': 26531, 'nangahar': 26532, 'penalize': 26533, 'baptismal': 26534, 'arranges': 26535, 'shutters': 26536, 'portico': 26537, 'rites': 26538, 'asserting': 26539, 'sarabjit': 26540, 'moods': 26541, 'retirements': 26542, 'politicized': 26543, 'derg': 26544, 'selassie': 26545, 'kingdoms': 26546, 'colchis': 26547, 'kartli': 26548, 'iberia': 26549, '330s': 26550, 'oceanographic': 26551, 'circumpolar': 26552, 'mingle': 26553, 'discrete': 26554, 'ecologic': 26555, 'concentrates': 26556, 'approximates': 26557, '1631': 26558, 'retook': 26559, '1633': 26560, 'amongst': 26561, 'roamed': 26562, 'amused': 26563, 'frighten': 26564, 'perched': 26565, 'beak': 26566, 'wily': 26567, 'stratagem': 26568, 'complexion': 26569, 'deservedly': 26570, 'deceitfully': 26571, 'refute': 26572, 'reproached': 26573, 'luxuriate': 26574, 'depicted': 26575, 'zuhair': 26576, 'reapprove': 26577, 'abiya': 26578, 'rizvan': 26579, 'chitigov': 26580, 'shali': 26581, 'rescinded': 26582, 'judo': 26583, 'repatriation': 26584, 'esperanza': 26585, 'copiapo': 26586, 'shadowed': 26587, 'nazran': 26588, 'zyazikov': 26589, 'jafri': 26590, 'venomous': 26591, 'vindicated': 26592, 'samara': 26593, 'arsamokov': 26594, 'ingush': 26595, 'condominiums': 26596, 'equipping': 26597, 'interacted': 26598, 'kayhan': 26599, 'pinas': 26600, 'schoof': 26601, 'ayala': 26602, 'alabang': 26603, 'baikonur': 26604, 'cosmodrome': 26605, 'pythons': 26606, 'kandal': 26607, 'wedded': 26608, 'kroung': 26609, 'pich': 26610, 'cage': 26611, 'subscribe': 26612, 'animism': 26613, 'inhabit': 26614, 'inanimate': 26615, 'snakes': 26616, 'neth': 26617, 'vy': 26618, 'dharmsala': 26619, 'acknowledging': 26620, 'worthwhile': 26621, 'rosaries': 26622, 'scorpion': 26623, 'venom': 26624, 'selectively': 26625, '516': 26626, 'howell': 26627, 'enlarged': 26628, 'franchises': 26629, 'echocardiograms': 26630, 'knicks': 26631, 'eddy': 26632, 'curry': 26633, 'irregular': 26634, 'heartbeat': 26635, 'hoiberg': 26636, 'guenter': 26637, 'verheugen': 26638, 'sheeting': 26639, 'coveted': 26640, 'entitle': 26641, 'salar': 26642, 'uyuni': 26643, 'dahir': 26644, 'aweys': 26645, 'powerless': 26646, 'tvn': 26647, 'stainmeier': 26648, 'butmir': 26649, 'eufor': 26650, 'karameh': 26651, 'dwarfed': 26652, 'pressewednesday': 26653, 'bud': 26654, 'selig': 26655, 'fehr': 26656, 'sox': 26657, 'sama': 26658, 'murillo': 26659, 'hokkaido': 26660, 'stiffer': 26661, 'carphedon': 26662, 'murcia': 26663, 'gerolsteiner': 26664, 'issori': 26665, 'scourge': 26666, 'buoys': 26667, 'thammararoj': 26668, 'killer': 26669, 'dario': 26670, 'kordic': 26671, 'ganiyu': 26672, 'adewale': 26673, 'badoosh': 26674, 'babaker': 26675, 'zibari': 26676, 'lajcak': 26677, 'clamps': 26678, 'anand': 26679, 'sterilized': 26680, 'nimal': 26681, 'siripala': 26682, 'salvaging': 26683, 'cabs': 26684, 'fortunate': 26685, 'feasts': 26686, 'costan': 26687, 'emphatically': 26688, 'sakineh': 26689, 'starve': 26690, 'inexplicable': 26691, '5\xa0storm': 26692, 'measurement': 26693, 'measurements': 26694, 'outcries': 26695, 'shinsei': 26696, 'maru': 26697, 'compensatiion': 26698, 'bayu': 26699, 'krisnamurthi': 26700, 'parviz': 26701, 'davoodi': 26702, 'vollsmose': 26703, 'odense': 26704, 'conversions': 26705, 'evangelization': 26706, 'volcanologist': 26707, 'lene': 26708, 'understates': 26709, 'thankot': 26710, 'bhairahawa': 26711, 'geophysical': 26712, 'sparse': 26713, 'congratulation': 26714, 'summarizes': 26715, 'pollutes': 26716, 'afghanis': 26717, 'waistcoat': 26718, 'stained': 26719, 'biakand': 26720, 'sunnah': 26721, '215': 26722, 'aulnay': 26723, 'eker': 26724, 'mellot': 26725, 'korangal': 26726, 'shon': 26727, 'meckfessel': 26728, 'fadlallah': 26729, 'dispel': 26730, 'koyair': 26731, 'drunkedness': 26732, 'crosses': 26733, 'hossam': 26734, 'zaki': 26735, 'sadat': 26736, 'outstripped': 26737, 'legia': 26738, 'elijah': 26739, 'missteps': 26740, 'maas': 26741, 'muhamed': 26742, 'garza': 26743, 'superdome': 26744, 'workouts': 26745, 'trinity': 26746, 'alamodome': 26747, 'herbal': 26748, 'palnoo': 26749, 'kulgam': 26750, 'siasi': 26751, 'unprincipled': 26752, 'coldest': 26753, 'mikulski': 26754, 'shyster': 26755, 'uhstad': 26756, 'hardliner': 26757, 'indicative': 26758, '3700': 26759, 'bermel': 26760, 'slumped': 26761, 'bargains': 26762, 'resales': 26763, 'kibembi': 26764, 'shryock': 26765, 'carcasses': 26766, 'manifest': 26767, 'portoroz': 26768, 'obita': 26769, 'rostov': 26770, 'checkups': 26771, 'dhangadi': 26772, 'reclaimed': 26773, 'fragmentation': 26774, 'nesterenk': 26775, 'cruiser': 26776, 'chabanenko': 26777, 'rearming': 26778, 'harith': 26779, 'tayseer': 26780, 'endeavouron': 26781, 'spacewalking': 26782, 'reaffirms': 26783, 'bergner': 26784, 'rish': 26785, 'ashfaq': 26786, 'parvez': 26787, 'kayani': 26788, 'fatou': 26789, 'bensouda': 26790, 'hemingway': 26791, 'brighton': 26792, 'everado': 26793, 'tobasco': 26794, 'montiel': 26795, 'handpicked': 26796, 'ofakim': 26797, 'resurrected': 26798, 'tajouri': 26799, 'campaigner': 26800, 'polska': 26801, 'diminishes': 26802, 'obsession': 26803, 'ethnomusicologist': 26804, 'q': 26805, 'nabarro': 26806, 'mirwaiz': 26807, 'vilsack': 26808, 'kucinich': 26809, 'nationalizes': 26810, 'eugenio': 26811, 'anez': 26812, 'nunez': 26813, 'romani': 26814, 'lately': 26815, 'distract': 26816, 'morshed': 26817, 'ldp': 26818, 'responsive': 26819, 'jawed': 26820, 'ludin': 26821, 'sordid': 26822, 'horticulture': 26823, 'evolving': 26824, 'derive': 26825, 'onerous': 26826, 'jdx': 26827, 'multilaterals': 26828, 'exacerbates': 26829, 'tarmiyah': 26830, 'judaidah': 26831, 'ratios': 26832, 'mingling': 26833, 'zalinge': 26834, 'minimized': 26835, 'demoralized': 26836, 'disinvest': 26837, 'suleyman': 26838, 'aysegul': 26839, 'esenler': 26840, 'bourgas': 26841, 'egebank': 26842, 'joblessness': 26843, 'haridy': 26844, 'mirdamadi': 26845, 'pakhtoonkhaw': 26846, 'iteere': 26847, 'tracing': 26848, 'almazbek': 26849, 'appointee': 26850, 'hashem': 26851, 'ashibli': 26852, 'manie': 26853, 'clerq': 26854, 'torching': 26855, 'jumbled': 26856, 'acclaimed': 26857, 'avant': 26858, 'garde': 26859, 'yakov': 26860, 'chernikhov': 26861, '274': 26862, 'antiques': 26863, 'hermitage': 26864, 'gilded': 26865, 'ladle': 26866, 'gastrostomy': 26867, 'zoba': 26868, 'yass': 26869, 'saloumi': 26870, 'nkunda': 26871, 'rutshuru': 26872, 'disproportionate': 26873, 'cordero': 26874, 'montezemolo': 26875, 'corriere': 26876, 'della': 26877, 'sera': 26878, 'disregards': 26879, 'bajur': 26880, 'resealed': 26881, 'squeezing': 26882, 'workforces': 26883, 'democratia': 26884, 'channu': 26885, 'torchbearers': 26886, 'massaua': 26887, 'livio': 26888, 'berruti': 26889, 'palazzo': 26890, 'citta': 26891, 'kissem': 26892, 'tchangai': 26893, 'walla': 26894, 'lome': 26895, 'emmanual': 26896, 'townsend': 26897, 'jackets': 26898, 'frenchmen': 26899, 'choi': 26900, 'youn': 26901, 'jin': 26902, 'spilt': 26903, 'unjustifiable': 26904, 'beruit': 26905, 'odyssey': 26906, 'indignation': 26907, 'boulder': 26908, 'zwally': 26909, 'bioethics': 26910, 'sperm': 26911, 'kompas': 26912, 'iwan': 26913, 'rois': 26914, 'underlined': 26915, 'ridding': 26916, 'untrue': 26917, '1452': 26918, '459': 26919, 'decried': 26920, 'vadym': 26921, 'chuprun': 26922, 'preach': 26923, 'namik': 26924, 'infuriated': 26925, 'abdulla': 26926, 'shahin': 26927, 'pegs': 26928, 'pagonis': 26929, 'habila': 26930, 'bluefields': 26931, 'steelers': 26932, 'chargers': 26933, 'seahawks': 26934, 'tarija': 26935, '308': 26936, 'afari': 26937, 'djan': 26938, 'brong': 26939, 'ahafo': 26940, 'npp': 26941, 'duping': 26942, 'fleeced': 26943, 'ziauddin': 26944, 'gilgit': 26945, 'conoco': 26946, 'gela': 26947, 'bezhuashvili': 26948, 'abiding': 26949, 'rebuffing': 26950, '602': 26951, 'universally': 26952, 'originates': 26953, 'latgalians': 26954, 'panabaj': 26955, 'moviegoers': 26956, 'irate': 26957, 'propriety': 26958, 'bhagwagar': 26959, 'sensationalizing': 26960, 'revalue': 26961, 'churn': 26962, '345': 26963, 'anatol': 26964, 'liabedzka': 26965, 'krasovsky': 26966, '1776': 26967, 'confederacy': 26968, 'buoyed': 26969, 'desolate': 26970, 'indisputably': 26971, '1614': 26972, 'trappers': 26973, 'beerenberg': 26974, 'winters': 26975, 'victorian': 26976, 'awkward': 26977, 'wajed': 26978, 'harangued': 26979, 'nineva': 26980, 'pushpa': 26981, 'dahal': 26982, 'dasain': 26983, 'predetermined': 26984, 'stunned': 26985, 'reverberated': 26986, 'downfall': 26987, 'prabtibha': 26988, 'patil': 26989, 'fallujans': 26990, 'swazis': 26991, 'grudgingly': 26992, 'backslid': 26993, 'gagging': 26994, 'cesme': 26995, 'ksusadasi': 26996, 'bassel': 26997, 'sanitary': 26998, 'typhoid': 26999, 'morelos': 27000, 'disdain': 27001, 'lugansville': 27002, 'espiritu': 27003, 'bankroll': 27004, '1845': 27005, 'chandpur': 27006, 'apologies': 27007, 'giorgio': 27008, 'exhort': 27009, 'darwin': 27010, 'resurrect': 27011, 'adana': 27012, 'disparage': 27013, 'enraged': 27014, 'idolatry': 27015, 'eventide': 27016, 'selective': 27017, 'snowed': 27018, 'strangers': 27019, 'abundantly': 27020, 'qubad': 27021, 'ingratitude': 27022, 'restriction': 27023, 'hindrance': 27024, 'iwc': 27025, 'hunts': 27026, 'nahrawan': 27027, 'becher': 27028, "sa'eed": 27029, 'comptroller': 27030, 'feeble': 27031, 'compassionately': 27032, 'advertisers': 27033, 'abayi': 27034, 'kourou': 27035, 'moans': 27036, 'tonic': 27037, 'nutrient': 27038, 'helios': 27039, 'optical': 27040, 'parasol': 27041, 'halts': 27042, 'mistrust': 27043, 'fixation': 27044, 'reminder': 27045, 'hedi': 27046, 'yousseff': 27047, 'planners': 27048, 'fanned': 27049, 'andreu': 27050, 'hearse': 27051, 'lytvyn': 27052, 'ulrich': 27053, 'wilhelm': 27054, 'juventud': 27055, 'rebelde': 27056, 'conjwayo': 27057, 'tiempo': 27058, 'mauricio': 27059, 'zapata': 27060, 'sununu': 27061, 'dangabari': 27062, 'svalbard': 27063, 'wainganga': 27064, 'jens': 27065, 'stoltenberg': 27066, 'environmentalist': 27067, 'wangari': 27068, 'maathai': 27069, 'capsize': 27070, 'bhandara': 27071, '925': 27072, 'janan': 27073, 'souray': 27074, 'gloom': 27075, 'lashkargah': 27076, 'tables': 27077, 'pham': 27078, 'thrive': 27079, 'painstakingly': 27080, 'anchor': 27081, 'ulsan': 27082, 'mobster': 27083, 'hangout': 27084, 'satriale': 27085, 'manny': 27086, 'condominum': 27087, 'serial': 27088, 'authentication': 27089, 'demolishing': 27090, 'pals': 27091, 'episode': 27092, 'gref': 27093, 'pai': 27094, 'f15': 27095, 'dohuk': 27096, 'okay': 27097, 'insurers': 27098, 'caters': 27099, 'caymanians': 27100, 'preoccupied': 27101, 'sudeten': 27102, 'ruthenians': 27103, 'reich': 27104, 'truncated': 27105, 'ruthenia': 27106, 'siam': 27107, 'chinnawat': 27108, 'shahdi': 27109, 'mohanna': 27110, 'wetchachiwa': 27111, 'confiscating': 27112, 'cleavages': 27113, 'politic': 27114, 'marginal': 27115, 'underinvestment': 27116, 'vitality': 27117, 'dombrovskis': 27118, 'meekness': 27119, 'gentleness': 27120, 'temper': 27121, 'altogether': 27122, 'boldness': 27123, 'bridle': 27124, 'dread': 27125, 'valynkin': 27126, 'mainichi': 27127, 'amelia': 27128, 'renting': 27129, 'lehava': 27130, 'rentals': 27131, 'lifestyles': 27132, 'muttur': 27133, 'hanssen': 27134, 'indescribable': 27135, 'horrors': 27136, 'miracles': 27137, 'e2': 27138, 'pingtung': 27139, 'flattening': 27140, 'shanties': 27141, 'browne': 27142, 'inductee': 27143, 'masser': 27144, 'burgie': 27145, 'bobby': 27146, 'teddy': 27147, 'randazzo': 27148, 'dylan': 27149, 'catalog': 27150, 'induction': 27151, 'vindicates': 27152, 'septum': 27153, 'cerebral': 27154, 'piling': 27155, 'orenburg': 27156, '2017': 27157, 'polo': 27158, 'rugby': 27159, 'roller': 27160, 'equestrian': 27161, 'equine': 27162, 'hallams': 27163, 'muammar': 27164, 'divas': 27165, 'potts': 27166, 'angelina': 27167, 'naankuse': 27168, 'dedication': 27169, 'baboons': 27170, 'leopards': 27171, 'dalia': 27172, 'itzik': 27173, 'seasonally': 27174, 'tropics': 27175, 'climates': 27176, 'modifying': 27177, 'acidity': 27178, 'salinity': 27179, 'idaho': 27180, 'taepodong': 27181, 'geoscience': 27182, 'spewing': 27183, 'plume': 27184, 'gulfport': 27185, 'sancha': 27186, 'waterworks': 27187, 'changqi': 27188, 'jubilant': 27189, 'neve': 27190, 'dekalim': 27191, 'commissioning': 27192, 'mo': 27193, 'layered': 27194, 'hospice': 27195, 'radiotherapy': 27196, 'shelve': 27197, 'iskan': 27198, 'harithiya': 27199, 'brazen': 27200, 'nourollah': 27201, 'niaraki': 27202, 'dousing': 27203, 'fuselage': 27204, 'tupolev': 27205, 'airtour': 27206, 'logjams': 27207, 'ballenas': 27208, 'defrauding': 27209, 'psychologically': 27210, 'unfurling': 27211, 'ayham': 27212, 'mujaheddin': 27213, 'distinction': 27214, 'radhika': 27215, 'coomaraswamy': 27216, 'cormac': 27217, 'delmas': 27218, 'challengerspace': 27219, 'donovan': 27220, 'watan': 27221, 'blackburn': 27222, 'basically': 27223, 'sneaking': 27224, 'burqa': 27225, 'yoshinori': 27226, 'ono': 27227, 'yvelines': 27228, 'hauts': 27229, "d'": 27230, 'oise': 27231, 'zheijiang': 27232, 'inscriptions': 27233, 'phoenixes': 27234, 'etched': 27235, 'porcelain': 27236, 'derek': 27237, 'whibley': 27238, 'bedrooms': 27239, 'sauna': 27240, 'disarms': 27241, 'noranit': 27242, 'setabutr': 27243, 'amass': 27244, 'sigou': 27245, 'solidaria': 27246, 'salvadorans': 27247, 'vulgar': 27248, 'gniljane': 27249, 'selim': 27250, 'transocean': 27251, 'trident': 27252, 'channeling': 27253, 'indus': 27254, 'fused': 27255, 'aryan': 27256, 'scythians': 27257, 'satisfactorily': 27258, 'marginalization': 27259, 'rocky': 27260, 'brightens': 27261, 'microchips': 27262, 'idiot': 27263, 'drunkard': 27264, 'clinging': 27265, 'chinchilla': 27266, 'chinggis': 27267, 'khaan': 27268, 'eurasian': 27269, 'steppe': 27270, 'mongolians': 27271, 'waemu': 27272, 'bakers': 27273, 'concealment': 27274, 'nibble': 27275, 'tendrils': 27276, 'rustling': 27277, 'arrow': 27278, 'maltreated': 27279, 'partridges': 27280, 'recompense': 27281, 'scruple': 27282, 'pondered': 27283, 'matched': 27284, '425': 27285, 'touches': 27286, 'closings': 27287, 'nasab': 27288, 'haqooq': 27289, 'zan': 27290, 'vujadin': 27291, 'popovic': 27292, 'milutinovic': 27293, 'sainovic': 27294, 'dragoljub': 27295, 'ojdanic': 27296, 'moses': 27297, 'lottery': 27298, 'refinances': 27299, 'cyanide': 27300, 'cashed': 27301, 'tavis': 27302, 'smiley': 27303, 'imagine': 27304, 'evading': 27305, 'conscription': 27306, 'asmara': 27307, 'qadir': 27308, 'sinak': 27309, 'muadham': 27310, 'brillantes': 27311, 'kilju': 27312, 'cabin': 27313, 'spinetta': 27314, 'cricketer': 27315, 'mauro': 27316, 'vechchio': 27317, 'sharks': 27318, 'stalking': 27319, 'crittercam': 27320, 'saola': 27321, 'hachijo': 27322, 'habibur': 27323, 'gaddafi': 27324, 'thilan': 27325, 'samaraweera': 27326, 'tharanga': 27327, 'paranavithana': 27328, 'misleading': 27329, 'devise': 27330, 'sisli': 27331, 'compounding': 27332, 'agence': 27333, 'presse': 27334, 'riskiest': 27335, 'womb': 27336, 'veterinarians': 27337, 'farhatullah': 27338, 'kypriano': 27339, 'kristin': 27340, 'halvorsen': 27341, 'crave': 27342, 'leong': 27343, 'lazy': 27344, 'mandera': 27345, 'prep': 27346, 'skelleftea': 27347, 'carli': 27348, 'dribbled': 27349, 'footed': 27350, 'ricocheted': 27351, 'pia': 27352, 'sundhage': 27353, 'cups': 27354, 'severity': 27355, 'kazemeini': 27356, 'disapora': 27357, 'speeds': 27358, 'parallels': 27359, 'lagunas': 27360, 'chacahua': 27361, 'cabo': 27362, 'corrientes': 27363, 'guatemalans': 27364, 'tabasco': 27365, 'mengal': 27366, 'salem': 27367, 'respectfully': 27368, '355': 27369, 'overtake': 27370, 'latvians': 27371, 'erase': 27372, 'clinch': 27373, 'kyat': 27374, 'ronco': 27375, 'doled': 27376, 'highness': 27377, 'royals': 27378, 'scimitar': 27379, 'graduating': 27380, 'sandhurst': 27381, 'accompany': 27382, 'isna': 27383, 'nie': 27384, 'frailty': 27385, 'decriminalizing': 27386, 'sweltering': 27387, 'tursunov': 27388, 'edgardo': 27389, 'massa': 27390, 'gremelmayr': 27391, 'bjorn': 27392, 'phau': 27393, 'teesta': 27394, 'ecology': 27395, 'ganges': 27396, 'damadola': 27397, 'scold': 27398, 'cuzco': 27399, 'reckon': 27400, 'incwala': 27401, 'swazi': 27402, 'nhlanhla': 27403, 'nhlabatsi': 27404, 'mbabane': 27405, 'hms': 27406, 'seawater': 27407, 'yunus': 27408, 'tadjoura': 27409, 'loudspeakers': 27410, 'ophir': 27411, 'pines': 27412, 'relinquish': 27413, 'rosemond': 27414, 'pradel': 27415, 'indiscretion': 27416, 'shoplifting': 27417, 'husseindoust': 27418, 'destablize': 27419, 'streamlined': 27420, 'weiner': 27421, 'lobbyists': 27422, 'tenzin': 27423, 'taklha': 27424, 'attribute': 27425, 'vials': 27426, 'profiting': 27427, 'ciampino': 27428, 'martha': 27429, 'goldenberg': 27430, 'kendrick': 27431, 'cong': 27432, 'superhighway': 27433, 'shady': 27434, 'loitering': 27435, 'boyle': 27436, '1860s': 27437, 'sughd': 27438, 'deluge': 27439, 'khujand': 27440, 'rasht': 27441, 'sedimentary': 27442, 'basins': 27443, 'garments': 27444, 'overflowing': 27445, 'damsel': 27446, 'reclining': 27447, 'forgetting': 27448, 'nurture': 27449, 'curious': 27450, 'sandbags': 27451, 'learnt': 27452, 'escapes': 27453, 'chevy': 27454, 'gallegos': 27455, 'sheldon': 27456, '232': 27457, 'honesty': 27458, '728': 27459, 'arnoldo': 27460, 'aleman': 27461, 'discredited': 27462, 'sandanista': 27463, '54th': 27464, 'dikes': 27465, 'vicinity': 27466, 'flashpoints': 27467, 'osnabrueck': 27468, 'processor': 27469, 'westland': 27470, 'hallmark': 27471, 'usda': 27472, 'vani': 27473, 'contreras': 27474, 'averting': 27475, 'jeopardizes': 27476, '34th': 27477, 'socially': 27478, 'hatim': 27479, '1657': 27480, 'basset': 27481, 'megrahi': 27482, 'mans': 27483, 'saydiyah': 27484, 'neighbourhood': 27485, 'lightened': 27486, 'lateral': 27487, 'limped': 27488, '2100': 27489, 'edt': 27490, 'doubly': 27491, 'aoun': 27492, 'sulieman': 27493, 'ivana': 27494, 'lisjak': 27495, 'gaz': 27496, 'byes': 27497, 'virginie': 27498, 'kveta': 27499, 'peschke': 27500, 'tsvetana': 27501, 'pironkova': 27502, 'rae': 27503, 'bareli': 27504, '70th': 27505, 'rajiv': 27506, 'safeguarding': 27507, 'refurbished': 27508, 'bike': 27509, 'walkways': 27510, 'sorted': 27511, 'crawl': 27512, 'unidentifiied': 27513, 'gruesome': 27514, "'n": 27515, 'caliber': 27516, 'chairperson': 27517, 'jaoko': 27518, 'recounting': 27519, 'episodes': 27520, 'pheasants': 27521, 'townships': 27522, 'reinvigorate': 27523, 'doi': 27524, 'talaeng': 27525, 'quadriplegic': 27526, 'clips': 27527, 'streaming': 27528, 'outward': 27529, 'sunspots': 27530, '489': 27531, 'forgotten': 27532, 'jaber': 27533, 'wafa': 27534, 'saboor': 27535, 'hadhar': 27536, 'leatherback': 27537, 'fascinate': 27538, 'roams': 27539, 'nesting': 27540, 'leatherbacks': 27541, 'tubigan': 27542, 'joko': 27543, 'suyono': 27544, 'intensifies': 27545, 'apostates': 27546, 'qais': 27547, 'shameri': 27548, 'sabotages': 27549, 'rekindle': 27550, 'lawfully': 27551, 'empt': 27552, 'seche': 27553, 'otari': 27554, 'unleash': 27555, 'miscalculated': 27556, 'zap': 27557, 'ararat': 27558, 'mazlum': 27559, 'mattei': 27560, 'nuimei': 27561, 'listened': 27562, '\x97': 27563, 'monuc': 27564, 'anyplace': 27565, 'catapulted': 27566, 'showcased': 27567, 'madhoun': 27568, 'leveling': 27569, 'kumtor': 27570, 'cis': 27571, 'recapitalization': 27572, 'cycles': 27573, 'shackleton': 27574, 'fated': 27575, 'aviary': 27576, 'wont': 27577, 'peacock': 27578, 'bajura': 27579, 'accham': 27580, 'kohistani': 27581, 'ahadi': 27582, 'locusts': 27583, 'zinder': 27584, 'castes': 27585, 'fortis': 27586, 'stella': 27587, 'artois': 27588, 'radek': 27589, 'johansson': 27590, 'tulkarm': 27591, 'maclang': 27592, 'batad': 27593, 'banaue': 27594, 'ifugao': 27595, 'legaspi': 27596, 'starmagazine': 27597, 'distorts': 27598, 'muasher': 27599, 'safehaven': 27600, 'wachira': 27601, 'waruru': 27602, '73rd': 27603, 'holodomor': 27604, 'kiwis': 27605, 'scorers': 27606, '201': 27607, 'ashraful': 27608, 'napier': 27609, 'detective': 27610, 'colt': 27611, 'cobra': 27612, 'revolver': 27613, 'definitively': 27614, 'holster': 27615, 'aussie': 27616, 'flyway': 27617, 'yuanshi': 27618, 'xianliang': 27619, 'sprees': 27620, 'gedo': 27621, 'bakili': 27622, 'muluzi': 27623, '365': 27624, 'yuvraj': 27625, 'araft': 27626, 'goodbye': 27627, 'saca': 27628, 'shaowu': 27629, 'chairmen': 27630, 'mumps': 27631, 'rubella': 27632, 'autism': 27633, 'upali': 27634, 'ealier': 27635, 'affiliations': 27636, 'hypocrisy': 27637, 'gardena': 27638, 'guay': 27639, '442': 27640, '420': 27641, 'aksel': 27642, 'svindal': 27643, '417': 27644, 'configured': 27645, 'nahum': 27646, 'repeating': 27647, 'pera': 27648, 'petrasevic': 27649, 'vukov': 27650, 'csatia': 27651, 'metullah': 27652, 'teeple': 27653, 'unspent': 27654, 'helmund': 27655, 'hushiar': 27656, 'facilitates': 27657, 'loveland': 27658, 'butchers': 27659, 'unionize': 27660, 'acetylene': 27661, 'basement': 27662, 'monsoons': 27663, 'bong': 27664, 'saqlawiyah': 27665, 'nampo': 27666, 'samad': 27667, 'kojak': 27668, 'sulaiman': 27669, 'pashtoonkhwa': 27670, 'milli': 27671, 'cholily': 27672, '221': 27673, 'chakul': 27674, 'consent': 27675, 'abune': 27676, 'squares': 27677, 'sicko': 27678, 'humaneness': 27679, 'harshest': 27680, 'wealthier': 27681, 'heeds': 27682, 'https': 27683, 'celebritiesforcharity': 27684, 'raffles': 27685, 'netraffle': 27686, 'cfm': 27687, 'airfare': 27688, 'costumed': 27689, 'urchins': 27690, 'porch': 27691, 'treaters': 27692, 'gravelly': 27693, 'beggars': 27694, 'decorating': 27695, 'upshot': 27696, 'dusk': 27697, 'ghouls': 27698, 'starlets': 27699, 'distasteful': 27700, 'nisr': 27701, 'khantumani': 27702, '178': 27703, 'moravia': 27704, 'magyarization': 27705, 'issas': 27706, 'gouled': 27707, 'aptidon': 27708, 'guelleh': 27709, 'socializing': 27710, 'terrified': 27711, 'slew': 27712, 'pounce': 27713, 'remedy': 27714, 'doosnoswair': 27715, 'oliveira': 27716, '567': 27717, 'onion': 27718, 'chili': 27719, 'eggplant': 27720, 'smoked': 27721, 'trout': 27722, 'spaghetti': 27723, 'parmesan': 27724, 'spinach': 27725, 'avocado': 27726, 'custodian': 27727, 'squeegee': 27728, 'educators': 27729, 'believer': 27730, 'séances': 27731, 'merriment': 27732, 'fooling': 27733, 'gullible': 27734, 'poke': 27735, 'mansions': 27736, 'scenic': 27737, '314': 27738, 'amharic': 27739, 'stinging': 27740, 'misled': 27741, 'rotfeld': 27742, 'surrenders': 27743, 'onal': 27744, 'ulus': 27745, 'cabello': 27746, 'youssifiyeh': 27747, 'kamdesh': 27748, 'abbreviations': 27749, 'translations': 27750, 'transplanting': 27751, 'casualities': 27752, 'rawhi': 27753, 'fattouh': 27754, 'corners': 27755, '407': 27756, 'francs': 27757, 'jokic': 27758, 'murghab': 27759, 'conductor': 27760, 'mstislav': 27761, 'moderating': 27762, '39th': 27763, 'adrien': 27764, 'houngbedji': 27765, 'vishnevskaya': 27766, 'laughable': 27767, 'laiyan': 27768, 'jiaotong': 27769, 'tp': 27770, 'rovnag': 27771, 'abdullayev': 27772, 'filya': 27773, 'implications': 27774, 'nabucco': 27775, 'bypassing': 27776, 'colchester': 27777, 'shabat': 27778, 'jabbar': 27779, 'jeremy': 27780, 'hobbs': 27781, 'khasavyurt': 27782, 'longyan': 27783, 'mensehra': 27784, 'dialed': 27785, 'gibb': 27786, 'guadalupe': 27787, 'escamilla': 27788, 'mota': 27789, 'rajapakshe': 27790, 'kentung': 27791, 'leonella': 27792, 'sgorbati': 27793, 'disable': 27794, 'amerzeb': 27795, 'beats': 27796, 'slinging': 27797, 'irresistable': 27798, 'shrek': 27799, 'grosses': 27800, 'bourj': 27801, 'abi': 27802, 'haidar': 27803, 'puccio': 27804, 'vince': 27805, 'spadea': 27806, 'taik': 27807, 'melli': 27808, 'proliferator': 27809, 'agartala': 27810, 'sébastien': 27811, 'citroën': 27812, 'xsara': 27813, 'wrc': 27814, 'duval': 27815, 'grönholm': 27816, 'auriol': 27817, 'submachine': 27818, 'estranged': 27819, 'hailie': 27820, 'remarried': 27821, 'recouped': 27822, 'continents': 27823, 'waldner': 27824, 'tycze': 27825, 'substantiated': 27826, 'establishments': 27827, 'ventilation': 27828, 'passive': 27829, 'petropavlosk': 27830, 'kamchatskii': 27831, 'fedora': 27832, 'adventurer': 27833, 'spielberg': 27834, 'paramount': 27835, 'indy': 27836, 'mangos': 27837, 'avocados': 27838, '1634': 27839, 'bonaire': 27840, 'isla': 27841, 'refineria': 27842, 'lied': 27843, 'burner': 27844, 'housekeeping': 27845, 'whiten': 27846, 'blacken': 27847, 'harnessed': 27848, 'tormented': 27849, 'yoke': 27850, 'smile': 27851, 'idleness': 27852, 'lighten': 27853, 'labors': 27854, 'hive': 27855, 'tasted': 27856, 'honeycomb': 27857, 'brute': 27858, 'brayed': 27859, 'cudgelling': 27860, 'fright': 27861, 'irrationality': 27862, 'lakeside': 27863, 'vijay': 27864, 'silly': 27865, 'clapping': 27866, 'luay': 27867, 'zaid': 27868, 'sander': 27869, 'maddalena': 27870, 'sardinian': 27871, 'humberto': 27872, 'valbuena': 27873, 'caqueta': 27874, '1890': 27875, 'dorsey': 27876, 'mahalia': 27877, '13010': 27878, 'majdal': 27879, 'matara': 27880, 'muhsin': 27881, 'matwali': 27882, 'atwah': 27883, 'intoxicating': 27884, 'maysoon': 27885, 'damaluji': 27886, 'massum': 27887, 'homeowner': 27888, 'micky': 27889, 'rosenfeld': 27890, 'talansky': 27891, 'congratulations': 27892, 'barka': 27893, 'kousalyan': 27894, 'shipyards': 27895, 'disillusionment': 27896, 'pictured': 27897, 'kira': 27898, 'plastinina': 27899, 'mikhailova': 27900, 'emma': 27901, 'sarrata': 27902, 'chronicle': 27903, 'jamahiriyah': 27904, 'pariah': 27905, 'walled': 27906, 'sevilla': 27907, 'maresca': 27908, '78th': 27909, '84th': 27910, 'fabiano': 27911, 'frédéric': 27912, 'kanouté': 27913, 'collemaggio': 27914, 'tarsyuk': 27915, 'adriatic': 27916, 'slovenians': 27917, 'dagger': 27918, 'stashes': 27919, 'unleashing': 27920, 'atilla': 27921, 'onna': 27922, 'shkin': 27923, 'abruzzo': 27924, 'cruelty': 27925, 'amicable': 27926, 'remnant': 27927, 'fades': 27928, 'animist': 27929, 'gil': 27930, 'hoffman': 27931, 'helmut': 27932, 'reunifying': 27933, '238': 27934, 'verbeke': 27935, 'intercepts': 27936, 'symbolize': 27937, 'ishaq': 27938, 'alako': 27939, 'rahmatullah': 27940, 'nazari': 27941, 'robinsons': 27942, 'quentin': 27943, 'tarantino': 27944, 'grindhouse': 27945, 'debuted': 27946, 'tracker': 27947, 'dergarabedian': 27948, 'takhar': 27949, 'icelanders': 27950, 'bootleg': 27951, 'reykjavik': 27952, 'marginalize': 27953}
defaultdict(<class 'int'>, {'of': 20221, 'country': 1931, 'and': 16861, 'iraq': 1673, 'marched': 65, 'that': 6054, 'through': 513, 'demand': 219, 'in': 22128, 'demonstrators': 132, 'from': 4355, 'the': 36488, 'thousands': 491, 'to': 18293, 'london': 277, 'protest': 236, 'withdrawal': 154, 'british': 593, 'have': 5287, 'troops': 1191, 'war': 954, 'wednesday': 1258, 'surveillance': 51, 'functioning': 11, 'parts': 169, 'say': 4148, 'system': 260, 'iranian': 376, 'officials': 3364, 'plant': 107, 'access': 125, 'after': 2722, 'begins': 51, 'an': 4030, 'they': 2273, 'sealed': 19, 'sensitive': 30, 'expect': 66, 'get': 169, 'iaea': 71, 'offensive': 149, 'militants': 1051, 'believed': 175, 'region': 851, 'are': 3583, 'earlier': 826, 'waziristan': 82, 'tribal': 167, 'many': 598, 'military': 1981, 'nearby': 107, 'avoid': 82, 'pounded': 15, 'militant': 457, 'fled': 154, 'taliban': 277, 'hideouts': 16, 'where': 651, 'south': 985, 'helicopter': 110, 'saturday': 1151, 'orakzai': 19, 'gunships': 18, 'hour': 127, 'police': 1771, 'standoff': 31, 'tense': 17, 'riot': 46, 'with': 5214, 'left': 350, 'long': 399, 'a': 18078, 'australian': 116, 'ferrying': 2, 'coordinator': 13, 'province': 934, 'crews': 18, 'remote': 99, 'western': 539, 'indonesian': 109, 'helicopters': 69, 's': 4204, 'aceh': 54, 'sunday': 1212, 'not': 2645, 'egeland': 14, 'jan': 64, 'areas': 305, 'relief': 199, 'said': 5264, 'supplies': 162, 'n': 855, 'u': 5024, 'ground': 82, 'food': 354, 'out': 1083, 'reach': 124, 'can': 499, 'people': 2722, 'mr': 2942, 'indonesia': 217, 'need': 146, 'maldives': 6, 'figures': 72, '8': 257, 'million': 833, 'assistance': 141, 'latest': 232, '1': 723, 'sri': 141, 'lanka': 92, 'greatest': 19, 'india': 490, 'show': 220, 'he': 3899, 'underwater': 7, 'massive': 144, 'tsunami': 151, 'triggered': 75, 'earthquake': 158, 'has': 6954, 'africa': 347, 'millions': 144, 'week': 1364, 'asia': 239, 'last': 1977, 'it': 3578, 'affected': 92, "'s": 9788, 'some': 1087, 'dead': 358, '000': 1129, 'known': 335, '27': 106, 'lack': 103, 'stressed': 50, 'but': 2512, 'remain': 236, 'bottlenecks': 2, 'is': 6407, 'challenge': 54, 'rushed': 23, 'official': 743, 'being': 568, 'infrastructure': 100, 'aid': 469, 'formerly': 26, 'lebanese': 205, 'attempt': 150, 'blast': 338, 'bomb': 718, 'sectarian': 59, 'strife': 15, 'torn': 54, 'neighborhood': 60, 'friday': 1277, 'as': 3701, 'condemning': 18, 'christian': 95, 'beirut': 94, 'sow': 2, 'politicians': 79, 'while': 624, 'destroying': 30, 'at': 4457, 'united': 2019, 'prime': 1118, 'spirit': 12, 'attempts': 63, 'string': 31, 'voiced': 22, 'nations': 1012, 'siniora': 9, 'their': 1719, 'fouad': 3, 'resolute': 1, 'summit': 243, 'minister': 1805, 'such': 417, 'anger': 24, 'new': 2085, 'york': 284, 'preventing': 30, 'residential': 15, '20': 409, 'more': 2258, 'injured': 238, 'others': 626, 'on': 6738, 'which': 1611, 'place': 376, 'killed': 2831, 'person': 141, 'street': 83, 'late': 568, 'than': 1828, 'one': 1790, 'took': 435, 'was': 4533, 'other': 1514, 'bombings': 242, 'rafik': 52, 'lebanon': 295, 'february': 263, 'series': 263, 'former': 987, 'explosion': 226, 'suffered': 102, 'since': 1353, 'hariri': 101, 'syria': 288, 'before': 719, 'detlev': 11, 'his': 3141, 'mehlis': 18, 'accused': 486, 'return': 260, 'damascus': 69, 'several': 912, 'killing': 583, 'investigator': 16, 'days': 525, 'interview': 154, 'widely': 65, 'about': 1534, 'comes': 234, 'syrian': 226, 'involvement': 115, 'assassination': 131, 'iceland': 10, 'shambles': 1, 'economy': 570, 'financial': 292, 'crisis': 254, 'global': 317, 'heart': 67, 'israeli': 942, 'procedure': 23, 'close': 237, 'minor': 32, 'tiny': 19, 'discovered': 116, 'treatment': 105, 'ariel': 75, 'stroke': 34, 'undergo': 12, 'will': 3248, 'hole': 10, 'medical': 170, 'thursday': 1330, 'for': 7940, 'during': 1223, 'sharon': 158, 'month': 1124, 'doctors': 100, 'defect': 4, 'describe': 14, 'chambers': 13, 'upper': 39, 'partition': 4, 'between': 1036, 'birth': 32, 'into': 1151, 'catheter': 1, 'cardiac': 1, 'blood': 58, 'inserting': 1, 'vessel': 53, 'device': 40, 'umbrella': 6, 'involves': 24, 'catheterization': 1, 'plug': 1, 'like': 140, 'make': 331, 'recovery': 81, 'full': 143, 'returned': 138, '25': 259, 'emergency': 216, 'hospitalization': 4, 'work': 359, 'december': 311, 'caused': 216, 'any': 507, 'permanent': 84, 'damage': 120, '10': 552, 'private': 153, 'prize': 59, 'designers': 3, 'first': 1126, 'promote': 59, 'rocket': 157, 'space': 154, 'burst': 13, 'manned': 7, 'received': 163, 'created': 75, 'tourism': 115, 'behalf': 21, 'state': 1408, 'ansari': 1, 'accepted': 43, 'x': 4, 'team': 239, 'rutan': 1, 'designer': 3, 'money': 219, 'ceremony': 113, 'missouri': 9, 'trophy': 2, 'awards': 20, 'burt': 1, 'spaceshipone': 3, 'fly': 25, 'win': 157, 'kilometers': 267, 'off': 562, '100': 223, 'period': 101, 'earth': 68, 'two': 2905, 'least': 1457, 'had': 1465, 'twice': 45, 'above': 53, 'early': 481, 'spacecraft': 17, 'mojave': 1, 'lifting': 20, 'october': 248, 'september': 320, 'flights': 80, 'california': 94, 'its': 2541, 'desert': 15, 'made': 687, 'krona': 1, 'three': 1409, 'major': 433, 'value': 58, 'soared': 24, 'plunged': 36, 'unemployment': 106, 'collapsed': 52, 'banks': 70, 'weight': 19, 'equivalent': 5, 'vehicle': 190, 'carry': 84, 'pilot': 28, 'passengers': 71, 'co': 67, 'financed': 7, 'paul': 130, 'allen': 10, 'founder': 25, 'corporation': 44, 'microsoft': 24, 'north': 877, '14': 200, 'says': 4602, 'korea': 482, '09': 10, 'flooding': 65, 'homes': 186, 'destroyed': 131, 'wipha': 1, 'typhoon': 47, 'hectares': 15, 'crops': 33, 'by': 4276, 'agency': 800, 'news': 757, 'reported': 481, 'monday': 1264, 'kcna': 8, 'floods': 65, 'public': 369, 'or': 885, 'damaged': 81, 'also': 2307, 'buildings': 92, 'roads': 54, 'railways': 4, 'washed': 16, 'bridges': 11, 'deaths': 217, 'report': 803, 'mention': 16, 'did': 409, 'injuries': 103, 'rains': 86, 'capital': 728, 'heavy': 189, 'most': 641, 'part': 638, 'pyongyang': 129, 'occurred': 198, 'southwestern': 76, 'including': 808, 'severe': 69, 'missing': 173, '600': 66, '00': 216, 'displaced': 95, 'nias': 5, 'ocean': 86, 'strong': 193, 'panic': 12, 'no': 998, 'islands': 185, 'under': 742, 'sumatra': 14, 'scale': 76, 'gave': 117, '6': 203, '7': 273, 'island': 298, 'quake': 98, 'preliminary': 41, 'geological': 18, 'richter': 3, 'estimate': 29, 'strength': 39, 'tuesday': 1392, 'epicenter': 9, 'survey': 71, 'morning': 94, 'geir': 2, 'call': 191, 'elections': 752, 'resign': 43, 'refused': 148, 'haarde': 1, 'cause': 112, '900': 20, 'march': 339, 'producing': 62, 'countless': 2, '26': 142, 'experienced': 38, 'earthquakes': 18, 'both': 440, 'stands': 36, '28': 89, 'tragedy': 23, 'them': 584, 'toll': 151, 'death': 500, '76': 28, 'nearly': 501, 'still': 383, '50': 286, 'feared': 31, 'listed': 19, 'five': 691, 'been': 2834, 'star': 58, 'disturbance': 4, 'britain': 332, 'arrested': 467, 'dogg': 5, 'airport': 111, 'heathrow': 6, 'rap': 5, 'associates': 13, 'snoop': 5, 'born': 83, 'broadus': 2, 'musician': 16, 'told': 683, 'calvin': 2, 'who': 1942, 'were': 3355, 'media': 394, 'held': 590, 'violent': 133, 'disorder': 9, 'charges': 441, 'affray': 1, 'members': 646, 'entourage': 3, 'name': 117, 'when': 1224, 'group': 1303, 'lounge': 3, 'class': 29, 'flight': 54, 'concert': 23, 'perform': 10, 'denied': 222, 'waiting': 29, 'threw': 50, 'scuffled': 6, 'free': 277, 'whisky': 1, 'bottles': 10, 'duty': 35, 'later': 511, 'store': 27, 'songs': 11, 'southern': 855, 'gritty': 1, 'crips': 2, 'gang': 40, 'streets': 88, 'life': 171, 'member': 349, 'reflect': 25, 'economic': 708, 'blames': 32, 'bankers': 6, 'calamity': 2, 'commercial': 60, 'spare': 14, 'afghan': 785, 'president': 3261, 'anyone': 55, 'engages': 2, 'activity': 128, 'would': 1109, 'fired': 274, 'hamid': 115, 'high': 534, 'spying': 43, 'countries': 804, 'warns': 27, 'ranking': 41, 'karzai': 150, 'involved': 187, 'parliament': 501, 'dismissed': 85, 'sworn': 36, 'indicate': 61, 'newly': 58, 'disclosure': 3, 'meeting': 590, 'nor': 37, 'action': 205, 'lunch': 12, 'evidence': 136, 'against': 1237, 'even': 129, 'if': 711, 'punished': 14, 'trial': 243, 'foreign': 1100, 'television': 285, 'be': 2450, 'shown': 47, 'put': 183, 'found': 472, 'taleban': 555, 'led': 650, '2001': 232, 'ousted': 145, 'afghanistan': 1030, 'presidential': 391, 'election': 694, 'won': 247, '2004': 241, 'waging': 14, 'administration': 292, 'now': 389, 'insurgency': 126, 'kofi': 101, 'secretary': 535, 'government': 3176, 'four': 839, 'raila': 4, 'opposition': 576, 'deal': 364, 'kenyan': 64, 'mwai': 17, 'trying': 282, 'general': 717, 'odinga': 6, 'kibaki': 36, 'broker': 12, 'annan': 162, 'weeks': 336, 'un': 66, 'leading': 188, 'concentrated': 10, 'arrangement': 12, 'agreement': 424, 'negotiations': 172, 'sharing': 53, 'power': 534, 'transitional': 37, 'fund': 119, 'billion': 355, 'international': 1078, 'monetary': 53, 'loan': 29, 'multi': 47, 'dollar': 104, 'forced': 175, 'ask': 44, 'finalize': 8, 'addressed': 29, 'must': 281, 'complimentary': 2, 'sets': 48, 'recent': 561, 'issues': 205, 'detailed': 17, 'francois': 11, 'icg': 1, 'grignon': 1, 'program': 598, 'director': 133, 'tackle': 11, 'reporter': 42, 'constitutional': 126, 'task': 22, '\x85': 3, 'dispute': 153, 'akwei': 1, 'electoral': 103, 'reform': 143, 'needed': 137, 'thompson': 1, 'because': 617, 'voa': 235, '\x94': 3, 'legal': 133, 'discuss': 268, 'losers': 1, 'telephone': 40, 'stronger': 45, 'stake': 19, 'demonstrate': 13, 'political': 716, 'transition': 35, 'screening': 9, 'legislation': 100, 'attack': 989, 'require': 36, 'terrorist': 363, 'cities': 149, 'all': 778, 'bush': 972, 'sea': 142, 'deemed': 18, 'risk': 80, 'cargo': 43, 'signed': 296, 'provide': 172, 'air': 269, 'homeland': 57, 'signing': 47, 'teams': 57, 'advisers': 5, 'doing': 73, 'protect': 102, 'security': 1593, 'enemy': 39, 'everything': 22, 'dangerous': 67, 'counter': 82, 'terrorism': 292, 'what': 559, 'called': 1009, 'bill': 205, 'commission': 252, 'states': 1503, 'recommendations': 20, 'measures': 163, 'independent': 185, 'investigated': 31, 'attacks': 1066, '11': 303, 'those': 564, '4': 247, 'transit': 32, 'grant': 36, 'include': 268, 'upgrade': 11, 'given': 129, 'ships': 69, 'planes': 59, 'years': 1007, 'bound': 37, 'next': 619, 'mandate': 31, 'within': 207, 'toward': 157, 'burmese': 102, 'reconciliation': 47, 'aung': 72, 'democracy': 233, 'kyi': 65, 'national': 739, 'calling': 216, 'advocate': 9, 'citizens': 154, 'san': 117, 'her': 559, 'year': 2163, 'suu': 65, 'she': 488, 'struggle': 58, 'force': 429, '2011': 70, 'together': 108, 'asked': 194, 'words': 61, 'statement': 717, 'strengths': 1, 'world': 1311, 'overtaking': 2, 'become': 170, 'experts': 178, 'health': 573, '2010': 209, 'disease': 198, 'cancer': 59, 'house': 584, 'old': 509, 'released': 528, '65': 41, 'democratic': 360, 'arrest': 201, 'reforms': 152, 'burma': 298, '13': 206, 'seven': 353, 'november': 268, 'criticized': 191, 'victory': 108, 'claimed': 281, 'rulers': 17, 'just': 311, 'overwhelming': 22, 'decades': 159, 'social': 122, 'establish': 50, 'well': 346, 'achieve': 21, 'truly': 4, 'networks': 23, 'talks': 993, '2': 457, '200': 179, 'leaders': 701, 'engage': 20, 'again': 156, 'prisoners': 234, 'conflict': 248, 'academics': 4, 'assembled': 7, 'activists': 154, 'poverty': 106, 'warning': 142, 'clinton': 119, 'address': 162, 'initiative': 51, 'coincides': 7, 'conference': 266, 'assembly': 140, 'millennium': 22, 'opening': 101, 'focus': 104, 'significant': 96, 'simply': 18, 'problems': 177, 'talk': 32, 'concrete': 15, 'secure': 59, 'day': 907, 'pledges': 22, 'ways': 78, 'participants': 20, 'pledge': 45, 'organizers': 40, 'back': 279, 'different': 50, 'forums': 1, 'progress': 130, 'required': 42, 'deputy': 178, 'expected': 598, 'attendees': 4, 'shimon': 14, 'blair': 128, 'speakers': 11, 'israel': 905, 'peres': 29, 'tony': 90, 'tightened': 11, 'spot': 21, 'second': 478, 'ballot': 20, 'vying': 3, 'peruvian': 26, 'further': 201, 'run': 348, 'gap': 30, 'candidates': 149, 'narrow': 23, 'organization': 457, 'smoking': 21, 'behind': 121, 'rising': 113, 'developing': 113, 'cigarette': 5, 'deadliness': 1, 'factor': 17, 'growing': 150, 'issued': 295, 'votes': 86, '96': 11, 'percent': 593, 'pro': 263, 'garcia': 12, 'counted': 27, 'business': 179, '90': 96, 'alan': 19, 'center': 267, 'congresswoman': 3, 'flores': 12, 'less': 132, 'lourdes': 1, 'leads': 26, 'whose': 99, 'attributed': 22, 'living': 128, 'surge': 34, 'starting': 47, 'support': 469, 'impact': 58, 'tally': 14, 'apparently': 76, 'among': 434, 'peruvians': 2, 'abroad': 74, '9': 181, 'half': 215, 'announced': 495, 'april': 193, '30': 374, 'final': 242, 'results': 212, 'vote': 495, 'candidate': 179, 'take': 425, 'nationalist': 22, 'ollanta': 1, 'humala': 1, 'either': 61, '31': 103, 'pinochet': 32, 'children': 289, 'augusto': 12, 'wife': 79, 'bail': 40, 'detained': 328, 'evasion': 24, 'tax': 128, 'chilean': 35, 'authorities': 1186, 'freed': 127, 'dictator': 71, 'adult': 12, 'dollars': 156, 'investigation': 255, 'kept': 42, 'lucia': 7, 'bank': 463, 'hiriart': 4, 'accounts': 79, 'child': 79, 'fifth': 74, 'daughter': 45, 'whereabouts': 10, 'unknown': 57, 'charged': 184, 'leaving': 114, 'located': 80, 'prohibited': 7, 'indicted': 64, 'fraud': 135, 'hiding': 46, 'allegedly': 118, 'thought': 57, '40': 232, 'china': 967, 'alone': 29, 'live': 86, 'smokers': 7, 'rights': 563, 'rule': 168, '1970s': 29, 'mid': 95, 'faces': 114, 'related': 129, 'human': 562, 'ordered': 221, 'fit': 13, 'stand': 71, 'enough': 127, 'so': 369, 'court': 688, 'do': 276, 'healthy': 20, 'lawyers': 80, 'mexico': 197, 'died': 590, 'leader': 885, 'zapatista': 8, 'female': 53, 'sources': 114, 'movement': 189, 'rebel': 314, 'ramona': 2, 'comandante': 2, 'subcomandante': 6, 'zapatistas': 2, 'piece': 22, 'saying': 785, 'fighter': 50, 'marcos': 8, 'lost': 142, 'stop': 295, 'tour': 105, 'six': 589, 'nationwide': 66, 'announcement': 146, 'chiapas': 4, 'came': 390, 'kidney': 15, 'rumored': 2, 'nature': 27, 'immediately': 154, 'clear': 212, 'transplant': 5, 'once': 113, 'mysterious': 4, 'promoter': 3, 'indian': 330, 'tzotzil': 1, 'women': 272, 'wearing': 33, 'black': 84, 'longtime': 25, 'appeared': 110, 'mask': 2, 'ski': 12, 'this': 1595, 'bid': 59, 'hideout': 30, 'january': 389, 'influence': 50, 'jungle': 10, 'begin': 183, 'emerged': 24, '12': 294, 'estimated': 127, 'diagnosed': 20, 'form': 161, 'representatives': 125, 'islamic': 413, 'release': 301, 'carroll': 28, 'relations': 237, 'jill': 17, 'journalist': 118, 'washington': 551, 'council': 467, 'american': 718, 'kidnapped': 211, 'appealed': 46, 'based': 385, 'baghdad': 758, 'abductors': 16, 'unharmed': 37, 'influential': 32, 'record': 225, 'iraqi': 1076, 'culture': 27, 'documented': 5, 'arab': 236, 'reporting': 56, 'respect': 39, 'objective': 9, 'custody': 123, 'eight': 317, 'there': 816, 'fate': 44, 'word': 50, 'kidnappers': 84, 'detainees': 171, 'threat': 167, 'execute': 4, 'unless': 84, 'following': 474, 'senior': 231, 'son': 86, 'defense': 477, 'ministry': 557, 'meanwhile': 456, 'brigadier': 24, 'forces': 1322, 'kill': 121, 'threatened': 158, 'arabiya': 9, 'al': 1140, 'sabah': 8, 'videotape': 44, 'coalition': 479, 'cooperating': 17, 'karim': 5, 'abd': 3, 'northern': 565, 'denounced': 39, 'muslim': 360, 'anti': 351, 'attending': 55, 'groups': 509, 'uttar': 6, '150': 72, 'clerics': 36, 'scholars': 8, 'deoband': 1, 'top': 536, 'darul': 1, 'pradesh': 16, 'uloom': 1, 'declaration': 38, 'seminary': 5, 'innocent': 26, 'targeting': 77, 'islam': 110, 'peace': 636, 'contradicts': 4, 'concept': 2, 'condemned': 115, 'mercy': 7, 'oppression': 3, 'violence': 754, 'described': 102, 'religion': 25, 'kinds': 4, 'hunt': 29, 'launched': 195, 'pakistani': 468, 'insurgents': 546, 'die': 40, 'predicts': 10, 'community': 169, 'however': 274, 'ensure': 65, 'harassed': 8, 'schools': 81, 'muslims': 162, 'link': 29, 'madrassas': 5, 'whenever': 8, 'religious': 147, 'studied': 6, 'having': 105, 'acts': 28, 'lives': 114, 'bars': 13, 'terror': 150, 'falsely': 1, 'spending': 130, 'pledged': 116, 'campaign': 224, 'vaccinate': 8, 'poorest': 41, 'gates': 46, 'norway': 75, 'hope': 100, 'invest': 16, 'donors': 59, 'melinda': 12, '750': 11, 'vaccines': 11, 'spurs': 1, 'immunization': 4, 'foundations': 4, 'governments': 127, 'foundation': 36, '290': 3, 'alliance': 158, 'immunize': 2, 'hopes': 123, '2015': 2, 'cost': 106, 'estimates': 62, 'diseases': 29, 'each': 234, 'easily': 41, 'basic': 43, 'prevented': 39, 'begun': 111, 'suspected': 536, 'helping': 95, 'qaida': 472, 'spain': 174, 'plan': 435, '24': 120, 'glass': 11, 'bulletproof': 1, 'madrid': 32, 'defendants': 51, 'greater': 84, 'tuberculosis': 12, 'aids': 107, 'soon': 139, 'number': 471, 'predicted': 30, 'combined': 36, 'malaria': 26, 'courtroom': 13, 'specially': 4, 'trials': 30, 'remodeled': 1, 'built': 68, 'providing': 76, 'armed': 236, 'multiple': 27, 'eddin': 1, 'yarkas': 1, 'imad': 5, 'barakat': 2, 'cell': 55, 'spanish': 123, 'finalized': 12, 'suspects': 292, 'details': 173, 'organized': 53, 'sentenced': 96, 'convicted': 109, 'prosecutors': 131, '60': 163, 'prison': 305, 'asking': 47, 'parliamentary': 268, 'monitor': 53, 'strip': 253, 'kidnappings': 48, 'union': 660, 'gaza': 540, 'preparing': 63, 'palestinian': 918, 'foreigners': 63, 'observers': 52, 'west': 479, 'european': 714, 'despite': 254, 'chief': 461, 'keyser': 2, 'taking': 199, 'observer': 11, 'making': 194, 'necessary': 74, 'de': 131, 'precautions': 10, 'veronique': 1, 'regular': 32, 'assessments': 2, 'eu': 352, 'met': 336, 'sign': 99, 'send': 93, 'bad': 55, 'adding': 30, 'monitors': 53, 'commitment': 48, 'workers': 409, 'jerusalem': 94, 'towns': 80, 'spoke': 132, 'headed': 59, 'reporters': 326, 'ms': 178, 'campaigning': 22, 'voting': 95, 'east': 374, 'citing': 48, 'permit': 19, 'law': 284, 'refusal': 26, 'conditions': 134, 'delay': 84, 'order': 169, 'dire': 4, 'may': 641, 'rise': 92, 'patients': 50, 'dying': 14, '17': 192, '2030': 2, 'process': 242, 'stays': 2, 'track': 47, 'monitored': 16, 'concerned': 94, 'liberia': 38, 'delegation': 100, 'post': 258, 'act': 102, 'responsibly': 2, 'jimmy': 7, 'urged': 251, 'soglo': 2, 'carter': 24, 'nicephore': 2, 'continue': 311, 'benin': 21, 'voter': 29, 'extensive': 34, 'runoff': 43, 'education': 109, 'added': 142, 'poll': 125, 'widespread': 82, 'understanding': 20, 'ballots': 58, 'problem': 63, 'casting': 13, 'ignorance': 2, 'cited': 41, 'choice': 24, 'america': 170, 'joint': 173, 'includes': 121, 'institute': 31, 'europe': 272, 'gathered': 113, 'sheds': 2, "shi'ite": 324, 'main': 266, 'our': 37, 'referred': 40, 'remarks': 87, 'kabul': 152, 'destruction': 66, 'wants': 159, 'mosque': 118, 'refer': 26, 'rebuffed': 2, 'offers': 20, 'similar': 152, 'documents': 70, 'reports': 737, 'liberties': 23, 'obtained': 32, 'civil': 251, 'suppress': 6, 'abuse': 99, 'tried': 153, 'saw': 70, 'increase': 233, 'nato': 504, 'dramatic': 10, 'thousand': 72, 'thaw': 7, 'mountain': 31, 'accessible': 2, 'months': 438, 'coming': 115, 'snows': 2, 'paris': 124, 'crackdown': 100, 'france': 321, 'planning': 121, 'nine': 210, 'normandy': 3, 'french': 398, 'allegiance': 9, 'links': 87, 'gspc': 1, 'algerian': 30, 'declared': 161, 'combat': 110, 'salafist': 6, 'camp': 183, 'base': 232, 'interrogation': 26, 'guards': 100, 'bay': 71, 'escorted': 6, 'guantanamo': 99, 'naval': 69, 'us': 188, 'ray': 13, 'detainee': 23, 'pentagon': 59, 'cuba': 230, 'home': 418, 'tribunal': 124, 'combatants': 9, 'determined': 42, 'sent': 208, 'sudan': 356, 'saudi': 197, 'third': 316, 'another': 569, 'jordan': 92, 'arabia': 109, 'cleared': 32, 'review': 90, 'types': 12, 'administrative': 26, 'deprived': 4, 'humiliated': 1, 'assaulted': 8, 'staff': 111, 'dia': 2, 'intelligence': 267, 'incidents': 82, 'witnessed': 10, 'sleep': 4, 'moroccan': 28, 'transferred': 48, 'eighth': 21, 'oil': 1018, 'revenue': 60, 'declining': 41, 'resources': 104, 'low': 150, 'yemen': 46, 'highly': 69, 'dependent': 40, 'income': 93, '70': 136, 'gdp': 173, 'roughly': 37, 'petroleum': 70, 'investment': 154, 'diversifying': 4, 'initiated': 12, 'effects': 28, 'bolster': 18, 'non': 175, '2006': 206, 'sectors': 63, 'designed': 68, '2009': 203, 'effort': 227, 'gas': 291, 'diversification': 7, 'liquefied': 1, 'exported': 10, 'natural': 162, 'imf': 70, 'established': 77, 'efforts': 417, 'approved': 232, 'towards': 57, 'friends': 39, '370': 5, 'aims': 22, 'august': 250, 'face': 220, 'difficult': 61, 'challenges': 63, 'continues': 148, 'growth': 319, 'these': 91, 'water': 226, 'population': 138, 'term': 263, 'ambitious': 16, 'endeavors': 1, 'rate': 137, 'singapore': 47, 'colony': 33, 'trading': 113, '1819': 2, 'founded': 31, 'malaysian': 20, '1963': 9, 'federation': 51, 'separated': 14, 'joined': 116, 'became': 176, 'prosperous': 20, 'capita': 34, 'terms': 66, 'subsequently': 25, 'tonnage': 1, 'handled': 20, 'port': 139, 'per': 171, 'busiest': 7, 'equal': 21, 'personnel': 104, 'e': 80, 'mails': 16, 'special': 175, 'included': 107, 'complaints': 36, 'qatar': 33, '1800s': 1, 'transformed': 10, 'revenues': 58, 'ruled': 159, 'family': 194, 'mainly': 89, 'poor': 176, 'itself': 61, 'pearling': 1, 'noted': 45, 'thani': 3, 'protectorate': 14, '1980s': 53, 'siphoning': 4, 'crippled': 8, 'amir': 13, 'continuous': 5, 'qatari': 2, '1972': 12, '1990s': 82, 'overthrew': 18, 'hamad': 5, 'bin': 99, 'him': 638, 'coup': 97, 'bloodless': 10, 'khalifa': 3, 'current': 172, '1995': 90, 'resolved': 36, 'border': 627, 'bahrain': 25, 'longstanding': 6, 'disputes': 37, 'highest': 118, 'attain': 1, 'enabled': 8, '2007': 184, 'agriculture': 127, 'forestry': 10, 'african': 433, 'car': 402, 'outlying': 2, 'subsistence': 31, 'central': 480, 'remains': 217, 'republic': 258, 'backbone': 2, 'sector': 205, 'generates': 3, 'agricultural': 94, 'diamond': 15, '16': 176, 'export': 101, 'accounted': 12, 'timber': 14, 'industry': 164, 'earnings': 49, 'unskilled': 2, 'development': 300, 'legacy': 5, 'important': 128, 'landlocked': 14, 'transportation': 40, 'macroeconomic': 15, 'constraints': 4, 'largely': 103, 'misdirected': 1, 'policies': 122, 'position': 70, 'fighting': 592, 'drag': 6, 'opponents': 70, 'revitalization': 1, 'factional': 16, 'federal': 221, 'aclu': 1, 'freedom': 107, 'request': 112, 'comply': 23, 'information': 309, 'unequal': 4, 'distribution': 43, 'extraordinarily': 3, 'partially': 9, 'humanitarian': 116, 'needs': 92, 'grants': 17, 'only': 482, 'meet': 351, 'trap': 8, 'bird': 520, 'captured': 191, 'peasant': 4, 'set': 472, 'admiring': 1, 'eagle': 31, 'much': 233, 'bundle': 2, 'deliverer': 1, 'prove': 19, 'flew': 34, 'talons': 6, 'seeing': 40, 'head': 323, 'wall': 62, 'sitting': 14, 'safe': 67, 'snatched': 6, 'ungrateful': 1, 'fall': 89, 'rose': 121, 'pursuit': 12, 'let': 56, 'fallen': 38, 'rendered': 2, 'pieces': 17, 'up': 1091, 'service': 250, 'marveled': 1, 'find': 99, 'same': 231, 'man': 408, 'went': 175, 'ass': 33, 'mutual': 12, 'entered': 102, 'partnership': 36, 'fox': 91, 'protection': 74, 'forest': 12, 'far': 178, 'proceeded': 6, 'lion': 52, 'capture': 47, 'harm': 23, 'danger': 28, 'promised': 122, 'approached': 18, 'contrive': 3, 'imminent': 9, 'pit': 1, 'arranged': 10, 'should': 376, 'upon': 86, 'deep': 51, 'assuring': 5, 'then': 258, 'georgian': 49, 'fair': 77, 'upcoming': 80, 'supports': 41, 'zhvania': 5, 'ukraine': 204, 'ukrainian': 96, 'zurab': 3, 'attacked': 216, 'leisure': 3, 'secured': 15, 'clutched': 1, 'your': 57, 'never': 104, 'trust': 29, 'mathematicians': 1, 'lose': 22, 'functions': 7, 'nostalgia': 1, 'lesson': 4, 'grammar': 1, 'you': 144, 'perfect': 7, 'past': 347, 'present': 76, 'blacksmith': 1, 'grandfather': 3, 'shop': 14, 'tell': 30, 'rigors': 1, 'himself': 140, 'how': 233, 'toughened': 1, 'my': 52, 'blacksmithing': 1, 'worked': 73, 'used': 359, 'me': 33, 'boy': 63, 'could': 776, 'arms': 143, 'sack': 3, 'hold': 249, 'extend': 52, 'outside': 320, 'straight': 48, 'sides': 155, 'potato': 2, '5': 374, 'pound': 13, 'hand': 99, 'lift': 34, 'finally': 33, 'eventually': 38, 'sacks': 2, 'got': 42, 'potatoes': 1, 'putting': 34, 'minutes': 59, 'started': 86, 'reportedly': 62, 'internal': 63, 'audit': 11, 'overlooked': 1, 'advance': 42, 'copies': 9, 'overcharges': 1, 'wasted': 2, "'": 398, 'contractors': 32, 'audits': 1, 'sevan': 8, 'benon': 3, 'office': 330, 'published': 154, 'aide': 41, 'ran': 31, 'criticize': 14, 'visiting': 77, 'currently': 141, 'comment': 111, 'voice': 56, 'systematic': 5, 'reveal': 14, 'corruption': 211, 'associated': 134, 'bribery': 6, 'portions': 15, 'press': 228, 'times': 171, 'investigate': 56, 'panel': 76, 'named': 137, 'billions': 31, 'regime': 89, 'corrupt': 15, 'hussein': 135, 'allegations': 164, 'saddam': 183, 'diverted': 8, '1996': 65, 'help': 532, 'gulf': 159, 'volcker': 6, 'heads': 61, 'wrongdoing': 21, 'cut': 214, 'artillery': 40, 'sites': 66, 'airstrikes': 35, '53': 37, 'wounded': 705, 'soldiers': 764, 'jaffna': 12, 'peninsula': 71, 'strikes': 113, 'tiger': 51, 'lankan': 46, 'fire': 425, 'rebels': 687, 'tamil': 80, 'provoked': 7, 'area': 495, 'trapped': 42, 'evacuate': 19, '800': 49, 'civilians': 316, 'ship': 107, 'stranded': 23, 'georgia': 126, 'satisfaction': 3, 'visit': 437, 'expressed': 207, 'began': 431, 'great': 93, 'interest': 121, 'separate': 283, 'tens': 127, 'lighting': 7, 'fight': 169, 'bathed': 1, 'attention': 55, 'white': 319, 'breast': 13, 'pink': 3, 'networking': 6, 'barack': 98, 'obama': 239, 'evening': 53, 'mansion': 4, 'lit': 3, 'recognition': 25, 'awareness': 20, 'executive': 82, 'site': 209, 'twitter': 13, 'proclamation': 8, 'aimed': 263, 'numerous': 45, 'large': 289, 'ribbon': 1, 'hung': 5, 'society': 40, 'annually': 30, 'claims': 106, '19': 120, 'worldwide': 110, 'traffic': 43, 'every': 109, 'seconds': 42, 'accident': 84, 'average': 63, 'someone': 24, 'dies': 6, 'condoleezza': 97, 'rice': 224, 'level': 157, 'advisor': 34, 'priority': 24, 'conclusion': 15, 'road': 129, 'safety': 97, 'calls': 251, '2008': 247, 'issue': 173, 'amid': 105, 'plans': 374, 'tetiana': 2, 'koprowicz': 2, 'improve': 92, 'diplomat': 76, 'ismail': 37, 'haniyeh': 22, 'bbc': 23, 'johnston': 8, 'case': 246, 'hamas': 417, 'richard': 38, 'consul': 2, 'makepeace': 1, 'kidnapping': 65, 'boycott': 60, 'focused': 38, 'policy': 197, 'represent': 26, 'change': 164, 'resolution': 127, 'abduction': 22, 'time': 573, 'permanently': 11, 'integrity': 16, 'regards': 11, 'russian': 527, 'affairs': 101, 'quoted': 96, 'enclave': 28, 'own': 177, 'recognizes': 19, 'territorial': 35, 'abkhazia': 26, 'ballistic': 20, 'short': 118, 'range': 86, 'missile': 161, 'rockets': 92, 'agreed': 314, 'missiles': 102, 'committee': 209, 'barak': 6, 'robert': 93, 'counterpart': 67, 'proposed': 142, 'ehud': 52, 'iran': 1240, 'geoff': 4, 'possibly': 30, 'spokesman': 732, 'morrell': 1, 'able': 112, 'territories': 60, 'facing': 92, 'withdrawing': 37, '2005': 177, 'territory': 194, 'revolution': 50, 'chavez': 366, 'rejected': 211, 'voters': 125, 'brakes': 1, 'venezuelan': 236, 'hugo': 154, 'socialist': 40, 'constitution': 275, 'quicken': 1, 'turn': 58, 'try': 110, 'mistake': 26, 'pace': 27, 'haven': 17, 'weekly': 72, 'venezuela': 355, 'broadcast': 103, 'referendum': 161, 'deciding': 9, 'evaluate': 1, 'proceed': 11, 'way': 237, 'party': 790, 'regional': 175, 'consolidate': 7, 'package': 57, 'turned': 124, 'down': 536, '18': 195, 'criminal': 94, 'over': 1291, 'alleged': 249, 'belarus': 45, 'probes': 5, 'riots': 49, 'concludes': 6, 'authoritarian': 10, '15': 352, 'vesna': 1, 'belarusian': 25, 'alexander': 41, 'lukashenko': 39, 'jailed': 60, 'wake': 37, 're': 161, 'overall': 67, 'protests': 286, 'capturing': 9, 'consecutive': 25, '80': 145, 'fourth': 122, 'closed': 126, 'fraudulent': 18, 'polls': 111, 'strongly': 62, 'alliances': 7, 'hearings': 28, 'confirmation': 39, 'heavily': 94, 'nominee': 37, 'repairing': 6, 'strained': 32, 'question': 48, 'senate': 170, 'powell': 43, 'hours': 219, 'nomination': 53, 'colin': 24, 'succeed': 31, 'decision': 273, 'tough': 28, 'direction': 17, 'spent': 81, 'testifying': 4, 'invade': 8, 'questions': 37, 'handling': 37, 'junichiro': 26, 'korean': 235, 'koizumi': 43, 'funeral': 69, 'seoul': 46, 'stopping': 29, 'japanese': 202, 'protesters': 218, 'mock': 3, 'give': 205, 'maintained': 29, 'timetable': 28, 'declined': 97, 'steadfastly': 2, 'believes': 63, 'building': 228, 'spreading': 37, 'goals': 32, 'around': 306, 'globe': 11, 'involving': 66, 'kashmir': 192, 'districts': 36, 'gunbattles': 11, 'udhampur': 2, 'erupted': 83, 'raided': 60, 'poonch': 3, 'along': 305, 'clashes': 141, 'kupwara': 4, 'district': 232, 'youths': 21, 'battled': 17, 'crossfire': 5, 'incident': 271, 'civilian': 186, 'residents': 206, 'kashmiri': 29, 'manmohan': 39, 'singh': 76, 'separatists': 53, 'prominent': 66, 'attend': 98, 'famous': 25, 'kfc': 5, 'chain': 16, 'fried': 4, 'fast': 39, 'chicken': 21, 'embassy': 202, 'park': 30, 'surrounded': 35, 'picture': 24, 'coffin': 13, 'empty': 14, 'carried': 221, 'hundreds': 295, 'near': 902, 'sanders': 3, 'harlan': 1, 'david': 66, 'patrons': 1, 'kentucky': 3, 'colonel': 44, 'better': 104, 'know': 50, 'restaurant': 21, 'brisk': 2, 'serves': 11, 'pizza': 1, 'alongside': 22, 'kabobs': 1, 'association': 97, 'ends': 27, 'sarawan': 1, 'inspired': 13, 'rahimgul': 1, 'narrates': 15, 'brian': 15, 'superpower': 2, 'approve': 61, 'platforms': 4, 'sales': 127, 'benjamin': 22, 'locally': 10, 'netanyahu': 32, 'satellites': 13, 'research': 77, 'mini': 5, 'market': 247, 'primary': 43, 'increased': 195, 'focusing': 24, 'newspaper': 337, '250': 45, 'quotes': 165, 'capability': 11, 'division': 28, 'total': 137, 'carve': 1, 'collaboration': 8, 'possibility': 41, 'companies': 215, 'toshiyuki': 1, 'takano': 1, 'ambassador': 174, 'residence': 26, 'effigy': 3, 'front': 132, 'burned': 51, 'fell': 151, 'slightly': 53, 'direct': 84, '22': 117, '0': 84, 'commerce': 45, 'slipped': 9, 'indication': 17, '88': 23, 'contracted': 39, '97': 12, 'gives': 39, '64': 41, 'grew': 51, 'future': 196, 'inflows': 11, 'although': 136, 'build': 151, 'investments': 36, 'slowing': 15, 'respiratory': 10, '2003': 343, 'syndrome': 5, 'spring': 23, 'outbreak': 175, 'acute': 10, '43': 25, 'qalat': 2, 'zabul': 37, 'ambushed': 55, 'guns': 25, 'machine': 22, 'patrol': 129, 'weapons': 512, 'locations': 19, 'sniper': 9, 'small': 253, 'propelled': 22, 'grenades': 41, 'failed': 214, 'flaming': 2, 'arrows': 1, 'maoist': 75, 'city': 1103, 'kathmandu': 43, 'nepal': 131, 'ban': 202, 'disrupted': 28, 'demonstration': 40, 'pleas': 4, 'increasingly': 55, 'mass': 144, 'end': 460, 'demanding': 110, 'bloody': 34, 'imposed': 112, 'killings': 85, 'sher': 8, 'maoists': 24, 'deuba': 3, 'resume': 124, 'ahead': 254, 'deadline': 64, 'escalated': 12, 'bahadur': 3, 'monarchy': 45, 'replace': 87, 'communist': 151, 'majority': 223, 'conspiracy': 30, 'congressman': 30, 'tom': 27, 'charge': 137, 'reinstated': 12, 'republican': 100, 'frivolous': 4, 'manufactured': 17, 'speaking': 186, 'indictment': 27, 'longer': 63, 'comfortable': 4, 'lawmaker': 48, 'books': 15, 'critics': 95, 'downplay': 2, 'tokyo': 87, 'history': 87, 'controlled': 97, 'runs': 57, 'sentiment': 11, 'japan': 363, 'approval': 104, 'atrocities': 24, 'wartime': 14, 'continual': 1, 'ethical': 8, 'christopher': 29, 'go': 184, 'beyond': 27, 'cnn': 37, 'sometimes': 24, 'shays': 1, 'jury': 31, 'violating': 37, 'finance': 78, 'grand': 49, 'texas': 93, 'stepped': 89, 'corporate': 11, 'allegation': 7, 'misuse': 10, 'centers': 61, 'donations': 25, 'prices': 402, 'rome': 43, 'discussed': 70, 'bans': 18, 'trade': 404, 'lifted': 43, 'barriers': 15, 'reduced': 58, 'commodities': 16, 'doubled': 14, 'wheat': 13, 'highs': 27, 'corn': 6, 'philippines': 44, 'hard': 108, 'hit': 367, 'haiti': 181, 'sending': 78, 'families': 110, 'immigrants': 60, 'haitian': 72, 'filipino': 4, 'deborah': 6, 'story': 51, 'block': 55, 'jazeera': 53, 'harder': 12, 'network': 149, 'arabic': 28, 'operate': 29, 'unmanned': 23, 'look': 40, 'allied': 35, 'considering': 74, 'conferences': 4, 'renew': 22, 'employees': 83, 'briefings': 5, 'visas': 8, 'tied': 25, 'move': 250, 'suspend': 50, 'owns': 15, 'closing': 43, 'doha': 10, 'obstacles': 13, 'ties': 180, 'municipal': 26, 'separatist': 92, 'numbers': 44, 'threats': 84, 'sporadic': 15, 'stones': 19, 'srinagar': 25, 'pelted': 6, 'burnt': 1, 'tires': 6, 'stages': 16, 'councils': 16, 'town': 515, 'chose': 18, 'stage': 48, '29': 66, 'caracas': 70, 'pilotless': 1, 'raul': 36, 'baduel': 2, 'scores': 43, 'northwestern': 103, 'eastern': 403, 'bombing': 220, 'bombs': 126, 'crowded': 35, 'explosions': 71, 'almost': 148, 'blasts': 68, 'across': 316, 'daily': 105, 'officers': 270, 'sunni': 200, 'cleric': 84, 'soldier': 247, 'backed': 183, 'helmand': 112, 'clash': 104, 'fierce': 31, 'sangin': 6, 'dozens': 124, 'stormed': 47, 'checkpoint': 79, 'jets': 47, 'maintaining': 22, 'aging': 16, 'f': 26, 'positions': 66, 'dropping': 25, 'line': 119, '11th': 21, 'insurgent': 140, 'hotbed': 3, 'islamist': 110, 'regions': 88, 'treaties': 12, 'russia': 587, 'duma': 17, 'ossetia': 20, 'lower': 118, 'ratified': 19, 'breakaway': 35, 'moscow': 214, 'endorsements': 3, 'keep': 128, 'unanimously': 18, 'friendship': 9, 'voted': 138, 'formalize': 2, 'diplomatic': 129, 'lawmakers': 297, 'swept': 45, 'recognized': 32, 'shortly': 86, 'reclaim': 6, 'drew': 28, 'condemnations': 2, 'invasion': 85, 'respond': 47, 'sanctions': 234, 'station': 203, 'sergei': 37, 'lavrov': 22, 'deployment': 52, 'opposes': 33, 'responsibility': 259, 'assume': 15, 'trouble': 26, 'blocking': 29, 'leukemia': 3, 'marrow': 1, 'cancers': 1, 'stem': 34, 'transplants': 2, 'therapy': 5, 'successfully': 34, 'treated': 51, 'bone': 3, 'cells': 14, 'jama': 2, 'study': 88, 'finds': 13, 'journal': 28, 'autoimmune': 1, 'alex': 4, 'villarreal': 1, 'forming': 35, 'pullout': 39, 'gain': 59, 'labor': 167, 'rival': 117, 'overnight': 58, 'palestinians': 226, 'egypt': 268, 'moving': 76, 'opened': 217, 'forbidden': 6, 'rafah': 22, 'men': 501, 'smugglers': 19, 'terrorists': 164, 'spotted': 17, 'shot': 259, 'hospital': 244, 'negotiators': 61, 'stalled': 45, 'nuclear': 979, 'abu': 148, 'musab': 48, 'zarqawi': 72, 'amer': 5, 'web': 66, 'officer': 156, 'nayef': 1, 'holy': 88, 'claim': 116, 'gunmen': 313, 'dora': 2, 'provincial': 114, 'rode': 6, 'bodyguards': 33, 'gunned': 24, 'governor': 142, 'armored': 24, 'struck': 129, 'roadside': 237, 'elsewhere': 131, 'bomber': 213, 'separately': 104, 'rammed': 18, 'suicide': 420, 'failure': 69, 'kurds': 53, 'phase': 25, 'dialogue': 69, 'difference': 6, 'forthcoming': 1, 'marks': 44, 'responsible': 118, 'success': 34, 'beginning': 83, 'arabs': 41, "shi'ites": 48, 'violations': 56, 'ongoing': 73, 'pose': 20, 'key': 260, 'increasing': 80, 'prepare': 40, 'positive': 85, 'determination': 10, 'quarterly': 8, 'beset': 4, 'formidable': 2, 'uranium': 175, 'suspension': 41, 'sought': 65, 'kharrazi': 10, 'tehran': 338, 'pressing': 33, 'enrichment': 121, 'kamal': 15, 'always': 28, 'activities': 173, 'germany': 278, 'hostages': 95, 'pleaded': 58, 'busload': 1, 'driver': 80, 'egyptian': 170, 'construction': 150, 'cairo': 95, 'company': 411, 'wounding': 144, 'bus': 117, 'firm': 86, 'sweilam': 2, 'deadly': 249, 'surrendered': 30, 'mahmoud': 235, 'veteran': 16, 'rampage': 10, 'stopped': 78, 'driving': 47, 'rifle': 12, 'automatic': 7, 'suddenly': 15, 'shooting': 92, 'investigating': 117, 'motive': 22, 'july': 282, 'indicating': 8, '71': 13, 'employers': 11, 'recover': 42, 'recession': 77, 'jobs': 114, 'slow': 70, 'stayed': 13, 'shows': 114, 'department': 375, 'unchanged': 9, 'hiring': 5, 'closely': 46, 'figure': 66, 'watch': 90, 'payrolls': 2, 'bulk': 15, 'economists': 46, 'job': 79, 'stability': 92, 'big': 37, 'unemployed': 7, 'consumer': 76, 'tend': 2, 'worried': 28, 'employment': 31, 'spend': 25, 'indicator': 2, 'faith': 21, 'decide': 50, 'whether': 217, 'good': 136, 'gesture': 17, 'suspended': 116, 'census': 2, 'cuts': 68, 'bureau': 49, 'due': 209, 'couple': 35, 'hired': 11, 'conduct': 63, 'temporary': 43, 'count': 30, '44': 25, 'decade': 97, 'invited': 35, 'offering': 54, 'caribbean': 56, 'join': 115, 'inexpensive': 4, 'preval': 41, 'rene': 21, 'elect': 67, 'offer': 124, 'petrocaribe': 2, 'purchase': 30, 'generous': 5, 'inclusion': 7, 'payment': 25, 'options': 26, 'donate': 12, 'diesel': 13, 'hospitals': 44, 'fuel': 241, 'use': 282, 'promises': 23, 'elected': 153, 'haitians': 10, 'inaugurated': 11, 'tibetan': 53, 'refugees': 109, 'chinese': 444, 'batons': 11, 'dispersed': 8, 'monks': 16, 'enriched': 29, 'loaded': 14, 'detention': 131, 'trucks': 41, 'vans': 1, 'demonstrations': 104, 'lhasa': 13, 'tibet': 56, '400': 70, 'deeply': 26, 'arrests': 75, 'detentions': 14, 'arbitrary': 5, 'himalayas': 3, 'tibetans': 16, 'fleeing': 23, 'route': 40, '21': 123, 'mostly': 156, 'abed': 4, 'policeman': 50, 'jaafar': 2, 'unhurt': 9, 'suhaila': 1, 'escaped': 69, 'displacement': 3, 'migration': 7, 'cabinet': 188, 'straw': 32, 'jalal': 46, 'jack': 26, 'talabani': 62, 'dominated': 73, 'energy': 405, 'europeans': 26, 'speed': 40, 'gholamreza': 3, 'aghazadeh': 4, 'atomic': 150, 'ibrahim': 50, 'jaafari': 27, 'choosing': 11, 'affair': 8, 'hostage': 72, 'jordanian': 46, 'diabetics': 2, 'helps': 13, 'complications': 4, 'managing': 14, 'studies': 20, 'tightly': 6, 'sugar': 37, 'type': 24, 'diabetes': 15, 'funded': 30, 'juvenile': 3, 'carol': 17, 'pearson': 16, 'grave': 42, '36': 49, 'bodies': 168, 'exhuming': 2, 'balkan': 16, 'herzegovina': 40, 'bosnia': 74, 'forensic': 9, 'contain': 53, 'zvornik': 3, 'garage': 2, 'serb': 93, 'victims': 273, 'snagovo': 1, 'eyewitnesses': 3, 'village': 159, 'bosnian': 96, 'buried': 45, 'corpses': 8, 'already': 218, '300': 111, 'exhumed': 4, 'finding': 56, 'graves': 12, 'skeletons': 2, 'scientific': 21, 'today': 246, 'achievements': 9, 'srebrenica': 27, 'massacred': 2, 'boys': 30, 'southeast': 121, 'taken': 248, 'bandits': 7, 'abducting': 8, 'blocked': 52, 'vehicles': 97, 'baluchestan': 1, 'sistan': 3, 'few': 149, 'available': 56, 'rolling': 4, 'honoring': 12, 'holiday': 97, 'riders': 5, 'parade': 25, 'thunder': 3, 'memorial': 44, 'motorcycle': 24, 'battle': 101, 'vietnam': 90, 'veterans': 15, 'lincoln': 4, 'gathering': 51, 'bikers': 1, 'pop': 26, 'raiders': 3, 'sinatra': 1, 'musical': 15, 'tribute': 10, 'singer': 47, 'revere': 2, 'nancy': 8, 'event': 102, 'honors': 9, 'specifically': 20, 'hrbaty': 2, 'championships': 20, 'seeded': 44, 'advanced': 40, 'adelaide': 2, 'tennis': 58, 'dominik': 1, 'slovakia': 26, 'hewitt': 6, 'australia': 136, 'lleyton': 4, 'round': 158, 'hardcourt': 5, 'katrina': 139, 'louisiana': 30, 'braces': 1, 'onslaught': 2, 'landfall': 17, 'hurricane': 251, 'coast': 296, 'apr': 39, 'jun': 39, 'dropped': 102, 'czech': 67, 'hernych': 2, 'beat': 50, '04': 15, '06': 80, 'feb': 49, 'bouncing': 2, 'easier': 24, 'rameez': 1, 'topping': 3, '07': 39, 'junaid': 2, 'mar': 48, 'martin': 34, 'alberto': 25, 'players': 44, 'blake': 8, 'james': 45, 'defeated': 61, 'matches': 20, 'chela': 3, 'upset': 25, 'karlovic': 5, 'ivo': 7, '03': 21, 'croatia': 64, 'argentina': 71, 'losing': 19, 'ignacio': 3, 'juan': 22, 'mayer': 8, 'florian': 4, 'kenneth': 4, 'unseeded': 5, 'advancing': 14, 'philippoussis': 2, 'italy': 130, 'serra': 3, 'murray': 7, 'danish': 53, 'seppi': 2, 'andreas': 4, 'mark': 82, 'frenchman': 6, 'nieminen': 5, 'player': 47, 'andy': 12, 'jarkko': 4, 'finland': 41, 'florent': 2, 'carlsen': 3, 'hiv': 69, 'devastating': 46, 'amnesty': 88, 'inequality': 8, 'contributing': 9, 'gender': 7, 'factors': 11, 'highlighting': 10, 'tune': 3, 'sing': 6, 'lennox': 1, 'annie': 1, 'charity': 31, 'mothers': 12, 'unborn': 3, 'especially': 61, 'does': 211, 'pregnant': 7, 'learned': 17, 'affects': 11, 'trip': 183, 'mandy': 4, 'clark': 11, 'disaster': 89, 'authorizes': 8, 'appropriate': 14, 'coordinate': 20, 'parishes': 1, 'athletes': 14, 'olympic': 149, 'drug': 231, 'games': 116, 'raid': 139, 'seized': 197, 'quarters': 22, 'surprise': 26, 'austrian': 25, 'cross': 139, 'biathlon': 6, 'materials': 48, 'italian': 113, 'sweep': 16, 'biathletes': 1, 'skiers': 2, 'residences': 2, 'tests': 128, 'competition': 41, 'conducted': 75, 'searched': 19, 'unannounced': 8, 'treat': 16, 'consistent': 9, 'offense': 8, 'laws': 85, 'doping': 18, 'equipment': 78, 'austria': 49, 'walter': 13, 'connected': 17, 'probe': 133, 'lake': 19, 'transfusions': 1, 'olympics': 75, 'banned': 90, 'vancouver': 6, 'ioc': 18, 'suspicion': 38, 'salt': 7, '2002': 186, 'performing': 15, 'turin': 31, 'coach': 15, 'restoring': 20, 'existence': 16, 'incompatible': 3, 'domination': 6, 'sovereignty': 62, 'militias': 82, 'rein': 6, 'inability': 9, 'exert': 5, 'roed': 11, 'stalling': 2, 'terje': 6, 'larsen': 11, 'envoy': 92, 'control': 354, 'implementing': 18, 'disarming': 13, '1559': 3, 'hezbollah': 134, '32': 61, 'evacuations': 5, 'lying': 22, 'preparation': 14, 'neighboring': 195, 'storm': 223, 'mississippi': 21, 'camps': 54, 'hills': 5, 'delivered': 43, 'deployed': 78, 'surrender': 32, 'contractor': 22, 'leave': 144, 'army': 504, 'loud': 5, 'century': 155, 'red': 100, '17th': 34, 'fort': 19, 'delhi': 86, 'ago': 346, 'muhammad': 45, '2000': 117, 'sentence': 70, 'arif': 2, 'handed': 68, 'role': 188, 'sneaked': 1, 'complex': 37, 'palace': 24, 'emperor': 15, 'mughal': 3, 'shah': 23, 'jehan': 1, 'independence': 277, 'symbol': 12, 'pronouncements': 1, 'speeches': 13, 'extended': 65, 'formally': 87, 'congress': 235, 'continuing': 85, 'poses': 10, 'informed': 33, 'waters': 72, 'season': 112, 'churned': 1, 'evacuated': 34, 'warm': 15, 'rigs': 5, 'atlantic': 47, 'hostile': 37, 'interests': 47, 'actions': 67, 'renewed': 55, 'existing': 30, 'expire': 19, 'isolated': 22, 'junta': 34, 'visited': 118, 'assistant': 35, 'campbell': 8, 'kurt': 6, 'effectively': 17, 'exclude': 5, 'league': 79, 'quota': 18, 'requests': 19, 'reached': 165, 'specialty': 4, 'accepting': 10, 'b': 26, 'h1': 2, 'immigration': 63, 'until': 357, 'wait': 23, 'services': 170, 'visa': 25, 'scientists': 97, 'allows': 45, 'skills': 14, 'skilled': 12, 'computer': 43, 'hire': 7, 'programmers': 1, 'engineers': 32, 'normally': 13, 'granted': 58, 'slowly': 33, 'northwest': 113, 'winds': 75, 'moved': 74, '185': 9, 'kilometer': 51, 'decides': 5, 'addition': 61, 'additional': 90, 'doctorate': 3, 'master': 11, 'college': 29, 'university': 65, 'permits': 18, 'degree': 10, 'bian': 21, 'chen': 43, 'taiwan': 178, 'shui': 19, 'strait': 12, 'guarantee': 11, 'willing': 52, 'appeal': 94, 'code': 23, 'create': 100, 'inseparable': 3, 'taiwanese': 14, 'agrees': 10, 'overtures': 3, 'speak': 40, 'moves': 43, 'seize': 20, 'makes': 60, 'push': 75, 'diplomats': 122, 'possible': 253, 'allies': 95, 'persuade': 13, 'compromise': 25, 'condition': 108, 'anonymity': 12, 'board': 122, 'governors': 15, 'vienna': 58, 'florida': 80, 'slammed': 14, 'accuses': 63, 'seeking': 165, 'denies': 116, 'facilities': 127, 'allow': 216, 'inspectors': 42, 'obligations': 11, 'bar': 16, 'visits': 52, 'refugee': 93, 'sparking': 22, 'badly': 22, 'shootout': 32, 'spokeswoman': 95, 'balata': 2, 'operation': 258, 'dawn': 23, 'pre': 56, 'nablus': 23, 'enforcing': 4, 'displays': 7, 'imposing': 19, 'step': 111, 'bloodshed': 13, 'test': 137, 'local': 419, 'clout': 2, 'seen': 154, 'finished': 53, 'unofficial': 9, 'ruling': 255, 'abbas': 241, '104': 11, 'fatah': 142, '61': 18, 'completed': 68, 'lead': 175, 'suppressing': 6, 'withdraw': 92, 'jericho': 16, 'operations': 274, 'marines': 75, 'iraqis': 127, 'kurdish': 213, 'sunnis': 24, 'sky': 9, 'ready': 111, 'depends': 31, 'rapidly': 31, 'infected': 105, 'virus': 346, 'provinces': 94, 'injected': 4, 'autonomous': 33, 'spread': 140, 'transmission': 17, 'exists': 7, 'situation': 185, 'malaysia': 48, 'warnings': 23, 'shigeru': 1, 'omi': 2, 'asian': 155, '500': 159, 'percentage': 23, 'records': 38, 'cambodia': 32, 'appreciate': 1, 'none': 28, 'enjoying': 8, 'folks': 1, 'victoria': 8, 'christmas': 83, 'centimeters': 27, 'usually': 37, 'unprecedented': 24, 'balmy': 1, 'snow': 57, 'weather': 123, 'measurable': 2, 'snowfall': 5, '1973': 18, '86': 9, '1917': 8, 'enjoyed': 12, 'freezing': 24, 'snowman': 1, 'skies': 7, 'sunny': 5, 'temperatures': 42, 'forecast': 20, 'tuz': 4, 'kirkuk': 72, 'khormato': 1, 'detonated': 74, 'samarra': 19, 'blew': 79, 'karbala': 25, 'arbaeen': 1, 'come': 194, 'gather': 20, 'pilgrims': 49, 'tight': 35, 'imam': 16, 'centuries': 52, 'mourning': 24, 'walked': 28, 'grandson': 6, 'revered': 8, 'prophet': 58, 'mohammad': 78, 'sandstorms': 2, 'battered': 14, 'rain': 68, 'hardest': 23, 'storms': 22, 'wind': 19, 'brought': 121, 'collapse': 57, 'alexandria': 6, 'factory': 37, 'frequent': 46, 'follow': 57, 'collapses': 3, 'relatively': 39, 'blamed': 177, 'rules': 64, 'reopened': 17, 'ports': 23, 'accidents': 40, 'visibility': 4, 'limited': 86, 'denver': 5, 'travel': 158, 'colorado': 11, 'shut': 81, 'snowstorms': 3, 'plains': 6, 'ending': 96, 'formal': 62, 'truce': 67, 'mountainous': 37, 'common': 96, 'highways': 13, 'delivery': 62, 'postal': 10, 'runways': 2, 'open': 180, 'delays': 22, 'airlines': 43, 'backlog': 1, 'travelers': 21, 'rushing': 7, 'poland': 102, 'sympathy': 4, 'solidarity': 31, 'krzysztof': 2, 'skubiszewski': 5, 'j': 15, 'paved': 6, 'eventual': 14, 'membership': 90, 'p': 27, 'crowley': 5, 'statesman': 3, 'visionary': 2, 'age': 73, '83': 16, 'occupied': 71, 'facto': 15, 'interrupted': 12, 'communism': 8, '1989': 60, '1993': 46, 'geographic': 8, 'extraordinary': 12, 'headquarters': 81, 'revealed': 25, 'archaeological': 4, 'green': 36, 'lush': 1, 'niger': 110, 'graveyards': 1, 'insight': 5, 'sahara': 19, 'sisco': 17, 'kony': 11, 'joseph': 37, 'ugandan': 61, 'magezi': 1, 'commanded': 8, 'lieutenant': 42, 'southwest': 42, 'juba': 8, 'chris': 15, 'clashed': 49, 'heading': 44, 'congo': 141, 'fighters': 192, 'gunman': 24, 'witnesses': 206, 'lord': 18, 'resistance': 66, 'pursue': 36, 'lra': 13, 'warrants': 14, 'notorious': 20, 'brutality': 7, 'uganda': 60, 'uprising': 54, 'activist': 33, '82': 16, 'winning': 54, 'triumfalnaya': 1, 'square': 62, 'right': 157, 'vladimir': 90, 'shouted': 8, 'putin': 146, 'slogans': 36, 'reversed': 11, 'lyudmila': 3, 'alexeyeva': 1, 'dissident': 17, 'sakharov': 1, 'recipients': 5, 'founding': 19, 'helsinki': 5, 'organizations': 88, 'oldest': 12, 'kurram': 7, 'authority': 150, 'attacking': 36, 'targets': 95, 'assemble': 4, 'dismayed': 5, 'exercising': 4, 'peacefully': 20, 'speech': 120, 'recognize': 56, 'universal': 15, 'defend': 48, 'strict': 24, 'valley': 66, 'swat': 39, 'impose': 44, 'shells': 17, 'allahabad': 1, 'maulana': 5, 'loyal': 34, 'eject': 1, 'fazlullah': 6, 'checkpoints': 20, 'truck': 85, 'closer': 42, 'peak': 15, 'strategic': 46, 'recaptured': 6, 'gains': 31, 'islamists': 21, 'fm': 9, 'radio': 185, 'angry': 37, 'demonstrated': 31, 'effect': 77, 'connecting': 3, 'jalalabad': 10, 'demanded': 95, 'bring': 148, 'skyrocketing': 3, 'aside': 24, 'setting': 43, 'pakistan': 855, 'kazakhstan': 44, 'buy': 76, 'reliant': 3, 'imports': 72, 'afghans': 46, 'slowed': 27, 'recently': 222, 'exports': 191, 'concerns': 195, 'abducted': 115, 'herat': 20, 'believe': 129, 'disappeared': 46, 'nepalese': 26, 'traveling': 99, 'worker': 69, 'adraskan': 1, 'scandal': 57, 'dereliction': 6, 'guilty': 103, 'prisoner': 48, 'ghraib': 46, 'summary': 1, 'ambuhl': 1, 'plea': 24, 'specialist': 6, 'martial': 15, 'megan': 1, 'tourist': 51, 'cosmonauts': 3, 'docked': 8, 'carrying': 197, 'pay': 123, 'demoted': 3, 'maryland': 15, 'plead': 6, 'woman': 188, 'stemming': 14, 'prevent': 142, 'faced': 41, 'naked': 7, 'taunting': 2, 'condemnation': 12, 'photographs': 37, 'humiliating': 4, 'liberation': 76, 'burundi': 28, 'bujumbura': 2, 'fnl': 2, 'intervened': 10, 'robbing': 4, 'broken': 41, 'accord': 82, 'connection': 114, 'ali': 150, 'haidari': 5, 'soyuzcapsule': 1, 'tip': 15, 'acting': 44, 'mosul': 108, 'guardsmen': 9, 'destabilize': 17, 'interim': 148, 'allawi': 47, 'iyad': 26, 'remanded': 2, 'transport': 52, 'suspect': 147, 'ismael': 2, 'abdurahman': 1, 'withholding': 5, 'helped': 113, '56': 23, 'bombers': 89, 'patrolling': 15, 'scheduled': 306, 'extradition': 36, 'hearing': 60, 'hamdi': 7, 'issac': 7, 'osman': 15, 'kotov': 1, 'billionaire': 13, 'oleg': 2, 'software': 18, 'simonyi': 3, 'yurchikhin': 1, 'charles': 37, 'fyodor': 1, 'citizen': 45, 'ethiopian': 95, 'descent': 16, 'warlord': 7, 'abdul': 67, 'narrowly': 17, 'rashid': 23, 'dostum': 9, 'praying': 7, 'festival': 41, 'eid': 27, 'adha': 7, 'purportedly': 8, 'omar': 64, 'mullah': 37, 'exchange': 155, 'lay': 35, 'radical': 55, 'agencies': 117, 'enter': 76, 'likes': 1, 'defiant': 3, 'resolutions': 9, 'watchdog': 15, 'overwhelmingly': 27, 'ahmadinejad': 140, 'peaceful': 136, 'insists': 46, 'accuse': 62, 'spaceflight': 1, 'paid': 87, 'privilege': 2, 'pointed': 14, 'disarmed': 9, 'comments': 176, 'arsenal': 11, 'cooperation': 186, 'confirmed': 327, 'forward': 59, 'generate': 25, 'enrich': 19, 'electricity': 97, 'ghazni': 30, 'interpreter': 17, 'nationalities': 19, 'i': 163, 'sharif': 24, 'mazar': 5, 'german': 188, 'explosives': 153, 'stuffed': 3, 'tajikistan': 16, 'staged': 49, 'eaten': 6, '1961': 7, 'gourmet': 2, 'anniversary': 109, 'gagarin': 1, 'dinner': 21, 'yuri': 11, 'khan': 77, 'midday': 8, 'prayers': 41, 'ahmad': 39, 'khost': 32, 'worshippers': 20, 'blame': 46, 'bilateral': 78, 'opportunities': 24, 'deepen': 4, '16th': 32, 'relationship': 36, 'differences': 55, 'critical': 76, 'soviet': 121, 'mikhail': 35, 'tyurin': 1, 'williams': 27, 'astronauts': 39, 'miguel': 9, 'alegria': 1, 'sunita': 1, 'crew': 89, 'lopez': 29, 'friendly': 26, 'dozen': 51, 'baquba': 33, 'wanted': 155, 'showing': 62, 'cropped': 1, 'photos': 33, 'beard': 1, 'hair': 16, 'bulgaria': 27, 'bulgarian': 14, 'nikolai': 5, 'probably': 42, 'svinarov': 1, 'agent': 35, 'investigations': 20, 'chance': 50, 'illegals': 1, 'toughen': 3, 'controversial': 109, 'limit': 41, 'debate': 56, 'regarding': 24, 'middle': 214, 'implementation': 19, 'map': 30, 'deport': 9, 'illegal': 165, 'guest': 13, 'provisions': 20, 'fewer': 19, 'passed': 143, 'passes': 12, 'reconciled': 1, 'felony': 5, 'emphasized': 9, 'illegally': 65, 'sparked': 87, 'raise': 89, 'rebuild': 43, 'working': 223, 'adopt': 15, 'curriculum': 3, 'teachers': 38, 'inter': 20, 'train': 91, 'idb': 2, 'enrolled': 2, 'classes': 11, 'school': 130, 'moreno': 8, 'luis': 45, 'arrived': 164, 'improved': 54, 'ability': 36, 'read': 26, 'hear': 22, 'mail': 22, 'phone': 48, 'determine': 61, 'formed': 70, 'best': 78, 'promoting': 35, 'done': 47, 'values': 22, 'propagandists': 1, 'hateful': 1, 'depicting': 12, 'decisions': 25, 'hindered': 8, 'diplomacy': 21, 'acknowledged': 43, 'proving': 4, 'emerge': 11, 'merit': 3, 'forged': 10, 'ally': 53, 'angeles': 52, 'los': 54, 'nation': 409, 'though': 65, 'khartoum': 55, 'sponsors': 6, 'cites': 16, 'data': 56, 'shared': 25, 'provided': 104, 'list': 113, 'flown': 23, 'removed': 55, 'vision': 12, 'side': 73, 'achieving': 7, 'palestine': 14, 'laden': 101, 'osama': 65, 'decline': 65, 'stay': 76, '3': 344, 'slowdown': 25, 'economies': 41, 'particularly': 61, 'integrated': 5, 'weathering': 1, 'ease': 49, 'downs': 3, 'affect': 34, 'diversify': 18, 'benefited': 8, 'ups': 1, 'commodity': 15, 'increases': 30, 'markets': 91, 'price': 170, 'carrier': 21, 'charter': 36, 'kong': 97, 'purchased': 19, 'boeing': 33, 'airways': 9, 'worth': 74, 'hong': 103, 'cr': 1, 'expand': 59, 'airliners': 13, 'bought': 27, '787s': 1, '737': 8, 'boosts': 4, 'manufacturer': 10, 'cathay': 1, 'flag': 24, 'aircraft': 175, 'pacific': 84, 'status': 70, 'crack': 20, 'institutions': 55, 'protesting': 50, 'stations': 84, 'broke': 147, 'curfew': 20, 'worsen': 6, 'pipeline': 72, 'largest': 291, 'refineries': 28, 'exploded': 173, 'darfur': 325, 'rape': 38, 'readying': 3, 'murder': 99, 'sudanese': 146, 'troubled': 68, 'justice': 192, 'agents': 55, 'mohamed': 53, 'reuters': 77, 'abuses': 54, 'yassin': 6, 'vowed': 73, 'humanity': 44, 'crimes': 223, 'punish': 22, 'consider': 83, 'proposal': 129, 'mustapha': 1, 'measure': 120, 'refuse': 8, 'van': 45, 'auction': 17, 'ludwig': 1, 'beethoven': 3, 'sold': 58, 'composer': 2, 'fuge': 1, 'manuscript': 3, 'gross': 11, 'palmer': 3, 'philadelphia': 6, 'buyer': 5, 'theological': 1, 'library': 3, 'auctioneers': 1, 'anonymous': 5, 'sotheby': 3, 'document': 72, 'performed': 12, 'piano': 4, 'flat': 10, '1826': 1, 'version': 21, 'page': 18, 'duet': 3, 'quartet': 7, 'written': 32, 'annotations': 1, 'crayon': 1, 'pencil': 2, 'ink': 2, 'brown': 46, 'reassessment': 1, 'rediscovery': 1, 'music': 60, 'complete': 63, 'continued': 174, 'wrote': 27, '1827': 1, 'deaf': 1, 'prior': 34, 'eta': 34, 'basque': 20, 'foiled': 13, 'resumes': 6, 'plot': 73, 'attackers': 73, 'brigades': 36, 'planned': 199, '1920': 3, 'plague': 4, 'session': 86, 'testify': 9, 'torture': 80, 'dujail': 19, '1982': 26, '140': 30, 'restricted': 18, 'repeatedly': 86, 'classified': 21, 'material': 56, 'forcing': 51, 'howard': 30, 'john': 220, 'vital': 23, 'granting': 20, 'initially': 38, 'resisted': 10, 'share': 121, 'reluctance': 3, 'june': 249, 'gara': 2, 'deliver': 37, 'commitments': 16, 'restrictions': 70, 'paper': 45, 'cartoon': 14, 'mana': 1, 'saeed': 3, 'editors': 4, 'mortazavi': 1, 'prosecutor': 85, 'neyestani': 1, 'cartoonist': 4, 'poked': 1, 'azerbaijan': 29, 'ethnic': 192, 'fun': 5, 'tabriz': 1, 'azeris': 4, 'portrayed': 2, 'cockroach': 1, 'azeri': 5, 'rioting': 18, 'tear': 19, 'disperse': 21, 'language': 42, 'turkish': 214, 'centerpiece': 2, '2012': 30, 'target': 84, 'stadium': 30, 'la': 18, 'host': 76, 'peineta': 1, 'jakarta': 50, 'comprehensive': 29, '1976': 20, 'solution': 31, 'sustainable': 10, 'participation': 34, 'covers': 9, 'arrangements': 9, 'momentum': 8, 'gained': 47, 'devastated': 57, 'attacker': 34, 'protecting': 31, 'tensions': 115, 'yet': 146, 'rich': 79, 'competing': 21, 'turkmen': 6, 'foothold': 1, 'maintains': 17, 'jose': 76, 'offered': 107, 'renounces': 6, 'rodriguez': 44, 'zapatero': 21, 'yugoslavia': 24, 'brief': 44, 'croatian': 37, 'sanader': 3, 'attended': 55, 'carla': 6, 'postponed': 59, 'zagreb': 9, 'ponte': 10, 'gotovina': 17, 'del': 27, 'ante': 7, 'ministers': 192, 'warn': 26, 'fully': 64, 'hague': 75, 'compliance': 12, 'presence': 91, 'maintain': 64, 'boundary': 7, 'peacekeepers': 120, 'mission': 244, '1960s': 17, 'confirm': 45, 'invaded': 14, '1978': 12, 'search': 91, '212': 3, 'shareholders': 13, 'llion': 1, 'toronto': 5, 'royal': 45, 'acquisition': 15, 'ltd': 3, 'corp': 18, 'trustco': 1, 'mi': 4, 'holding': 148, 'thrift': 4, 'obtain': 16, 'transaction': 8, 'expects': 56, 'regulatory': 12, 'historically': 8, 'played': 63, 'science': 29, 'literature': 9, 'kingdom': 64, 'stretched': 5, 'empire': 45, '19th': 45, 'zenith': 2, 'surface': 31, 'depleted': 2, 'wars': 56, 'seriously': 53, '20th': 35, 'uk': 59, 'irish': 28, 'dismantling': 13, 'rebuilding': 31, 'modern': 27, 'pursues': 3, 'approach': 27, 'commonwealth': 21, 'active': 45, 'ireland': 29, '1999': 80, 'wales': 8, 'scottish': 11, 'ill': 29, 'roger': 14, 'daltrey': 2, 'cancelled': 5, 'tampa': 1, 'latter': 11, 'wrangling': 3, 'devolution': 1, 'costa': 40, 'rica': 31, 'pirate': 9, 'natives': 3, 'raids': 95, 'combination': 13, 'swamps': 1, 'mosquito': 8, 'heat': 20, 'explored': 7, 'unsuccessful': 13, 'colonizing': 1, 'infested': 3, 'brutal': 21, 'proved': 13, 'initial': 59, '1563': 2, 'cooler': 3, 'highlands': 4, 'settlement': 90, 'fertile': 4, 'cartago': 1, 'remained': 55, '1821': 6, 'jointly': 13, '1838': 3, 'proclaimed': 12, 'disintegrated': 1, 'periods': 7, 'marred': 23, '1949': 22, 'dissolved': 23, 'expanded': 36, 'industries': 45, 'technology': 106, 'standard': 28, 'crowd': 68, 'offstage': 1, 'barely': 2, 'guitarist': 6, 'bronchitis': 1, '63': 21, 'song': 20, 'pete': 3, 'suffering': 64, 'townshend': 2, 'ownership': 18, 'land': 178, 'fishing': 83, 'trawling': 1, 'refuge': 24, 'occur': 9, 'vulnerable': 28, 'mining': 65, 'accounting': 14, 'gold': 78, '85': 37, 'volatility': 2, 'mineral': 12, 'alumina': 4, 'inherited': 2, 'fiscal': 68, 'ronald': 14, 'inflation': 73, 'venetiaan': 1, 'deficit': 77, 'raised': 95, 'tamed': 1, 'quickly': 66, 'taxes': 54, 'implemented': 22, 'attempted': 41, 'austerity': 9, 'owing': 2, 'sizeable': 6, 'belgium': 33, 'suriname': 10, 'netherlands': 74, 'bauxite': 6, 'projects': 93, 'earned': 28, 'waned': 1, 'picked': 29, 'budget': 111, 'boosting': 33, 'reduce': 96, 'devalued': 3, 'currency': 68, 'cheered': 7, 'rescheduled': 8, 'structural': 18, 'depend': 18, 'introduction': 6, 'medium': 14, 'prospects': 27, 'liberalize': 5, 'columbus': 15, 'grenada': 8, 'inhabited': 11, '1498': 1, 'carib': 3, 'uncolonized': 1, 'indians': 14, 'slaves': 16, 'settled': 35, 'estates': 4, 'imported': 38, '1762': 1, 'production': 219, 'vigorously': 3, 'nutmeg': 2, 'crop': 19, 'surpassed': 6, 'cacao': 1, '1967': 26, 'autonomy': 65, 'smallest': 7, 'attained': 11, 'hemisphere': 21, '1974': 21, '1983': 15, 'marxist': 16, 'cuban': 145, 'ringleaders': 1, 'reinstituted': 1, 'touring': 7, 'wire': 8, 'album': 16, 'endless': 1, 'ivan': 11, 'causing': 63, 'drowned': 15, 'shipwreck': 1, 'philosopher': 2, 'shore': 19, 'injustice': 4, 'sailing': 11, 'perchance': 1, 'inveighed': 1, 'perish': 2, 'sake': 7, 'providence': 2, 'persons': 16, 'whole': 23, 'standing': 41, 'ants': 6, 'nest': 4, 'indulging': 1, 'reflections': 2, 'trampled': 4, 'foot': 26, 'climbed': 26, 'stung': 6, 'mercury': 5, 'thyself': 1, 'dealings': 5, 'yourself': 5, 'hast': 1, 'presented': 54, 'wand': 1, 'striking': 10, 'indeed': 6, 'judge': 182, 'manner': 11, 'tulkarem': 15, 'apartment': 32, 'tayyah': 1, 'shootings': 20, 'rami': 1, 'recruitment': 9, 'establishment': 20, 'restart': 15, 'hampering': 13, 'ahmed': 74, 'yasser': 21, 'qureia': 24, 'arafat': 44, 'things': 23, 'want': 166, 'quiet': 18, 'sends': 11, 'message': 91, 'aggression': 15, 'band': 27, 'performs': 3, 'fischer': 8, 'joschka': 5, 'criticism': 108, 'churches': 25, 'simultaneous': 13, 'chaldean': 2, 'planting': 15, 'armenian': 12, 'casualties': 134, 'church': 108, 'minority': 72, 'root': 21, 'extreme': 16, 'caution': 7, 'concern': 152, 'result': 86, 'committed': 75, 'combating': 5, 'strike': 220, 'alike': 6, 'serious': 108, 'committing': 21, 'worsened': 9, 'uruzgan': 37, 'kandahar': 154, 'art': 25, 'often': 112, 'pablo': 2, 'paintings': 4, 'picasso': 1, "o'keeffe": 1, 'mind': 13, 'gogh': 7, 'talking': 13, 'names': 33, 'contemporary': 5, 'vincent': 8, 'artists': 10, 'body': 155, 'artwork': 4, 'we': 55, 'gallery': 3, 'primimoda': 1, 'tells': 24, 'ndimyake': 1, 'mwakalyelye': 1, 'chips': 2, 'maker': 24, 'investing': 9, 'biggest': 69, 'chairman': 113, 'intel': 13, 'bangalore': 7, 'employs': 16, 'generally': 32, 'respected': 8, 'innovation': 6, 'going': 61, 'oriented': 14, 'overseas': 37, 'stimulate': 7, '700': 57, 'invested': 12, 'operating': 73, 'barrett': 3, 'grow': 38, 'davis': 20, 'cup': 117, 'tournament': 83, 'hardcourts': 1, 'carson': 1, 'start': 124, 'title': 36, 'tie': 11, 'squad': 25, 'clay': 4, 'seville': 2, 'crown': 13, 'previous': 109, 'match': 100, 'romania': 27, 'winner': 72, 'criticizes': 8, 'ceasefire': 64, 'policemen': 137, 'sopore': 1, 'indiscriminately': 6, 'hurled': 14, 'mansoorain': 2, 'portion': 26, 'merger': 32, 'technologies': 10, 'crash': 92, 'moon': 55, 'lunar': 13, '542': 1, 'utc': 11, 'excellence': 2, 'volcanic': 8, 'smart': 7, 'plain': 4, 'orbited': 1, 'schwehm': 1, 'gerhard': 27, 'manager': 14, 'excessive': 13, 'jammu': 13, 'justify': 9, 'missions': 27, 'interplanetary': 1, 'electric': 19, 'solar': 21, 'propulsion': 2, 'instruments': 13, 'miniaturized': 1, 'examine': 15, 'orbiting': 15, 'craft': 5, 'mogadishu': 96, 'orphanage': 3, 'somalia': 264, 'lafoole': 1, 'kariye': 2, 'yusuf': 16, 'assailants': 30, 'abdikadir': 1, 'unidentified': 73, 'endangering': 3, 'rely': 17, 'catastrophe': 13, 'warned': 232, 'recovering': 18, 'describes': 17, 'iranians': 24, 'receive': 64, 'lieberman': 14, 'senator': 117, 'joe': 15, 'intends': 21, 'senators': 31, 'mccain': 48, 'introduced': 35, 'lindsey': 2, 'graham': 5, 'censorship': 10, 'tools': 5, 'online': 17, 'authorize': 10, 'evade': 1, 'funds': 95, 'programming': 11, 'cover': 36, 'persian': 30, 'funding': 67, 'broadcaster': 14, 'capacity': 53, 'sponsored': 40, 'wave': 56, 'satellite': 46, 'farda': 5, 'boost': 100, 'clearing': 27, 'cambodian': 18, 'mines': 39, 'huot': 2, 'england': 56, 'howes': 2, 'khmer': 10, 'rouge': 13, 'removing': 15, 'wat': 2, 'bristol': 1, 'huon': 1, 'temple': 14, 'angor': 1, 'showed': 111, 'executed': 34, '52': 39, 'lim': 1, 'puth': 1, 'personal': 40, 'freedoms': 17, 'notes': 25, 'minorities': 16, 'ii': 112, 'nazis': 9, 'jews': 41, 'dedicated': 18, 'dignitaries': 11, 'survivors': 72, 'berlin': 31, 'murdered': 18, 'nazi': 22, 'wolfgang': 6, 'memory': 8, 'thierse': 1, 'speaker': 54, 'alive': 32, 'holocaust': 25, 'hectare': 3, 'slabs': 1, '711': 1, 'evokes': 2, 'peter': 48, 'rigid': 3, 'consists': 16, 'architect': 7, 'eisenman': 2, 'unadorned': 1, 'underground': 39, 'discipline': 11, 'honor': 38, 'jewish': 132, 'size': 35, 'arguments': 9, 'design': 12, 'norwegian': 35, 'interference': 19, '210': 3, 'continuously': 6, 'tomas': 6, 'archer': 2, 'repeated': 55, 'politically': 35, 'motivated': 31, 'extrajudicial': 2, 'similarly': 1, 'bangladesh': 72, 'situations': 13, 'provides': 47, 'r': 5, 'internally': 6, 'c': 44, 'departure': 14, 'suspicious': 22, 'peacekeeping': 123, 'volatile': 35, 'resist': 7, 'pressure': 145, 'receiving': 54, 'ransacked': 5, 'jacques': 39, 'bernard': 18, 'farmhouse': 2, 'manipulating': 8, 'supported': 45, 'claiming': 32, 'outright': 10, 'proportionately': 2, 'divide': 14, 'blank': 6, 'internationally': 28, 'brokered': 26, 'boat': 61, '37': 39, 'guerrillas': 45, 'encounters': 2, 'mideast': 14, 'prevents': 5, 'ashore': 16, 'male': 23, 'swim': 6, 'surma': 1, 'colliding': 3, 'northeastern': 82, 'capsized': 14, 'river': 105, 'dhaka': 13, 'sunamganj': 1, 'northeast': 51, '240': 8, 'rivers': 21, 'regulations': 37, 'lax': 2, 'shape': 10, 'cayman': 10, 'depression': 17, 'tropical': 69, 'strengthen': 70, 'wilma': 19, '21st': 10, 'tying': 3, '1933': 3, 'daybreak': 2, 'centered': 36, '55': 49, '325': 6, 'sustained': 41, 'itv': 2, 'denouncing': 11, 'recognizing': 9, 'abide': 7, 'likely': 140, 'jamaica': 14, 'caymans': 1, 'forecasts': 5, 'reeling': 2, 'sailors': 14, 'mine': 96, 'compound': 63, 'batticaloa': 9, 'damaging': 23, 'grenade': 48, 'parking': 8, 'cease': 98, 'monitoring': 42, 'scandinavian': 3, 'unarmed': 12, 'reprimanded': 6, '69': 16, 'concluded': 30, '454': 5, 'esad': 1, 'croat': 12, 'kevljani': 2, 'bajramovic': 1, 'prijedor': 4, 'inmates': 39, 'keraterm': 1, 'omarska': 1, 'recovered': 55, 'himalayan': 26, 'winter': 67, '220': 14, 'sneak': 3, 'joining': 30, 'mottaki': 24, 'manouchehr': 18, 'idea': 27, 'ambitions': 32, 'rahim': 10, 'yahya': 5, 'safavi': 2, 'revolutionary': 65, 'intervention': 17, 'dealing': 36, 'cbs': 13, 'option': 24, 'greetings': 10, 'celebrate': 36, 'god': 20, 'blessings': 4, 'loving': 5, 'remember': 14, 'occasion': 13, 'abraham': 4, 'thanks': 18, 'considered': 85, 'wrested': 1, 'legislature': 33, 'obedience': 1, 'commemorates': 6, 'willingness': 15, 'sacrifice': 12, 'according': 68, 'scripture': 1, 'allah': 2, 'exchanging': 6, 'engaging': 10, 'worship': 5, 'gifts': 11, 'hopeful': 23, 'talents': 1, 'compassion': 7, 'generosity': 1, 'dimitris': 2, 'christofias': 2, 'cyprus': 46, 'treaty': 98, '49': 22, 'mediterranean': 22, 'favor': 57, 'nicosia': 4, 'ratify': 14, 'streamlining': 3, 'enact': 7, 'bureaucracy': 6, 'defeat': 60, 'advocates': 21, 'unclear': 71, 'salvaged': 1, 'lands': 20, 'designate': 14, 'creating': 34, 'harsh': 28, 'ravaged': 19, 'withstand': 6, 'concentrate': 5, 'zone': 73, 'antonio': 24, 'remark': 15, 'commissioner': 43, 'guterres': 5, 'reviewing': 14, 'cold': 39, 'materialize': 3, 'hunger': 44, 'hosting': 13, 'generously': 1, 'muzaffarabad': 13, 'repay': 12, 'miillion': 1, 'toured': 10, 'fleming': 3, 'secret': 113, 'imperial': 11, 'centenary': 2, 'exhibition': 17, 'marking': 48, 'museum': 38, 'writer': 11, 'bond': 8, 'ian': 9, 'exhibit': 6, 'titled': 5, 'burge': 2, 'eyes': 11, 'contains': 14, 'linked': 164, 'fallujah': 51, 'sattler': 2, 'shipments': 21, 'hardship': 3, 'textile': 27, 'mandelson': 6, 'searching': 49, 'ammunition': 28, 'letters': 20, 'embedded': 3, 'property': 30, 'pledging': 14, 'mural': 1, 'wired': 2, 'assault': 57, "o'hara": 2, 'deh': 1, 'jerry': 6, 'rawood': 1, 'light': 66, 'gun': 37, 'convoy': 145, 'upsurge': 21, 'revising': 1, 'beijing': 314, 'followed': 102, 'limiting': 2, 'rescued': 41, 'engineer': 16, 'chad': 102, 'gunpoint': 6, 'pilots': 10, 'captive': 7, 'sikh': 7, 'sikhs': 6, '160': 24, '1984': 27, 'incite': 5, 'gandhi': 11, 'indira': 3, 'compensation': 30, 'politician': 40, 'quotas': 22, 'customs': 45, 'filled': 21, 'items': 31, 'clothing': 23, 'withdraws': 5, 'race': 75, 'popular': 118, 'marwan': 7, 'expel': 5, 'barghouti': 12, 'challenger': 19, 'faruq': 1, 'qaddumi': 1, 'goes': 28, '45': 58, 'serving': 49, 'papers': 14, 'filed': 84, '9th': 7, 'revive': 25, 'powers': 78, 'negotiate': 39, 'brushing': 2, 'statements': 41, 'moualem': 1, 'walid': 7, 'olmert': 84, 'suppliers': 10, 'arbiter': 3, 'assad': 44, 'impartial': 4, 'mediate': 18, 'bashar': 34, 'allowing': 72, 'goods': 95, 'consequences': 21, 'retailers': 13, 'doubts': 13, 'play': 85, 'lacks': 18, 'entire': 53, 'golan': 9, 'heights': 11, 'coordinated': 34, 'unit': 92, 'azamiyah': 2, 'amiriyah': 2, 'agitation': 3, 'shortages': 44, 'higher': 115, 'delaying': 19, 'broadcasts': 24, 'communications': 43, 'jamming': 3, 'interfering': 17, 'signals': 7, 'telecommunication': 4, 'blaming': 21, 'source': 76, 'soil': 49, 'indicated': 39, 'eutelsat': 2, 'provider': 4, 'itu': 1, 'disputed': 94, 'disruptions': 6, 'means': 55, 'geneva': 45, 'polio': 36, 'ivory': 79, 'faso': 16, 'nigeria': 180, 'burkina': 16, 'producers': 20, 'harming': 8, '170': 21, 'cases': 226, 'vaccine': 36, 'infertility': 2, 'eradicate': 5, 'hampered': 20, 'contaminated': 16, 'nervous': 11, 'paralysis': 7, 'touch': 13, 'screen': 14, 'portable': 3, 'add': 15, 'devices': 31, 'computers': 24, 'phones': 16, 'website': 44, 'developments': 50, 'apple': 7, 'patent': 2, 'com': 8, 'macrumors': 1, 'watches': 13, 'beneath': 20, 'display': 17, 'screens': 4, 'saving': 11, 'windows': 18, 'competitor': 2, 'keyboard': 1, 'mouse': 7, 'counterparts': 22, 'regained': 12, 'diwaniyah': 4, 'militiamen': 23, 'sirleaf': 20, 'ellen': 15, 'liberian': 20, 'johnson': 29, 'pair': 14, 'congolese': 45, 'kabila': 19, 'mrs': 27, 'reconstruction': 76, 'debt': 131, 'supportive': 3, 'emerging': 25, 'ruined': 4, 'patriotic': 5, 'kurdistan': 40, 'diyala': 21, 'mohammed': 112, 'ramadan': 37, 'nouri': 43, 'maliki': 63, '23': 97, 'shaab': 2, 'michel': 10, 'barnier': 5, 'implement': 37, 'discussing': 35, 'stabilizing': 9, 'resigned': 84, 'motion': 28, 'confidence': 80, 'troop': 34, 'withdrawals': 9, 'withdrawn': 32, 'batch': 10, 'parallel': 5, 'handing': 13, 'allowed': 137, 'completes': 3, 'faction': 44, 'ramallah': 35, 'overdue': 2, 'salaries': 15, 'wing': 56, 'televised': 36, 'sadr': 39, 'moqtada': 19, 'ended': 192, 'ordering': 16, 'redeploy': 2, 'interior': 146, 'confrontation': 17, 'responded': 53, 'resignation': 81, 'kyrgyzstan': 47, 'deposed': 31, 'accept': 70, 'akayev': 16, 'askar': 12, 'technically': 5, '75': 46, 'letter': 92, 'deputies': 10, 'traveled': 52, 'videotaped': 8, 'osh': 5, 'unstable': 5, 'islamabad': 112, 'boucher': 9, 'meetings': 83, 'kasuri': 12, 'pervez': 82, 'musharraf': 168, 'khurshid': 2, 'hardliners': 2, 'import': 24, 'adopted': 56, 'gems': 6, 'metals': 7, 'embargo': 43, 'brussels': 43, 'freeze': 32, 'assets': 59, 'subject': 32, 'relatives': 51, 'meaningful': 3, 'sierra': 17, 'leone': 17, 'exact': 16, 'unable': 32, 'schoolchildren': 3, 'freetown': 1, 'syphon': 1, 'maritime': 24, 'frequently': 40, 'ignored': 20, 'boats': 33, 'lacking': 8, 'overloaded': 6, 'uncommon': 3, 'standards': 51, 'viktor': 88, 'treating': 19, 'dioxin': 4, 'tcdd': 2, 'yushchenko': 133, 'harmful': 14, 'poisoned': 16, 'substance': 10, 'orange': 13, 'contaminant': 1, 'normal': 35, 'yanukovych': 57, 'midst': 11, 'heated': 7, 'interviewed': 11, 'supreme': 163, 'overturned': 15, 'flawed': 26, 'dumped': 20, 'stamp': 18, 'pope': 207, 'vatican': 85, 'tradition': 27, 'vacant': 7, 'crossed': 46, 'image': 27, 'keys': 14, 'see': 100, 'symbols': 5, 'papal': 7, 'traditional': 50, 'stamps': 11, 'interregnum': 1, 'valid': 8, 'cardinals': 5, 'conclave': 1, 'capable': 29, 'detonating': 8, 'radioactive': 5, 'dirty': 11, 'chemical': 79, 'biological': 7, 'proliferation': 43, 'desire': 24, 'annual': 134, 'cia': 99, 'using': 148, 'stated': 6, 'toxins': 1, 'obtainable': 1, 'improvised': 11, 'substances': 7, 'radiological': 1, 'pursuing': 28, 'convinced': 9, 'clandestine': 9, 'factions': 63, 'hebron': 13, 'settle': 16, 'larger': 56, 'smaller': 46, 'ninth': 21, 'divided': 75, 'drove': 54, 'infection': 28, '268': 2, 'partly': 14, 'consultant': 3, 'lee': 30, 'dr': 32, 'facility': 121, 'paktika': 21, 'sexual': 32, 'needles': 1, 'twelve': 8, 'paktia': 7, 'demining': 1, 'deminers': 1, 'iranativu': 1, 'navy': 70, 'mount': 26, 'regrouped': 2, 'career': 41, 'pulled': 49, 'superstar': 3, 'medal': 22, 'kwan': 6, 'prestigious': 7, 'eluded': 2, 'michelle': 10, 'skater': 1, 'dashing': 1, 'recurring': 3, 'strain': 199, 'injury': 43, 'hip': 7, 'muscle': 7, 'groin': 4, 'withdrew': 55, 'herself': 19, 'ever': 73, 'native': 39, 'onto': 21, 'bye': 4, 'bronze': 12, 'titles': 9, 'silver': 14, 'medals': 15, 'downhill': 15, 'awarded': 25, 'anticipated': 8, 'skiing': 5, 'events': 64, 'alpine': 5, 'whistle': 1, 'vanunu': 7, 'blower': 1, 'mordechai': 3, 'unauthorized': 8, 'giving': 82, 'divulging': 2, 'barred': 36, 'contacts': 18, 'resort': 68, 'targeted': 110, 'gardens': 6, 'el': 88, 'ghazala': 1, 'sheik': 7, 'naama': 1, 'bazaar': 2, 'sharm': 16, 'hotel': 60, 'outdoor': 10, 'cafe': 9, 'packed': 35, 'busy': 32, 'summer': 51, 'vacation': 12, 'tourists': 60, 'hotels': 27, '34': 54, 'exaggerate': 1, 'mastermind': 14, 'surfaced': 13, 'audiotape': 8, 'internet': 110, 'aim': 22, 'seats': 141, 'minute': 44, 'ascendant': 1, 'tape': 47, 'ayatollah': 41, 'crush': 16, 'approving': 11, 'sistani': 9, 'stronghold': 60, 'fought': 64, 'innocents': 2, 'authenticity': 12, 'completely': 30, 'little': 94, 'moments': 7, 'silence': 22, 'ceremonies': 34, 'marked': 50, 'reading': 15, 'bagpipes': 2, 'hijacked': 36, 'moment': 27, 'bells': 7, 'tolled': 3, 'colombo': 26, 'raged': 8, 'biden': 17, 'vice': 184, 'lawn': 2, 'qaeda': 6, 'wreathlaying': 1, 'crashed': 60, '93': 15, 'pennsylvania': 10, 'shanksville': 2, 'plane': 110, 'intended': 59, 'hijackers': 9, 'presumably': 1, 'hitting': 26, 'gyanendra': 35, 'rally': 104, 'king': 179, 'pokhara': 2, 'coincided': 7, 'parties': 184, 'businesses': 74, 'deserted': 6, 'accompanied': 18, 'tamils': 5, 'registering': 5, 'nominations': 5, 'legitimize': 2, 'absolute': 21, 'submarines': 4, 'sell': 53, 'starts': 21, 'kommersant': 4, '677': 1, 'amur': 6, 'older': 13, '636': 1, 'contract': 58, 'supplied': 15, 'prompted': 53, 'expressions': 3, 'kalashnikov': 3, 'weaponry': 9, 'rifles': 17, 'deals': 53, 'critic': 24, 'discrimination': 24, 'complain': 11, 'sinhalese': 4, 'dick': 36, 'cheney': 70, 'stabilize': 23, 'abdullah': 81, 'strategy': 54, 'brazil': 142, 'drugs': 63, 'smuggling': 55, 'shoot': 17, 'colombia': 209, 'traffickers': 24, 'bogota': 17, 'reaffirming': 1, 'expansion': 46, 'brazilian': 53, 'steps': 60, 'error': 13, 'missionary': 4, 'peru': 67, 'dismantled': 11, 'inside': 94, 'hitch': 3, 'commanders': 41, 'transfer': 44, 'paperwork': 3, 'relaxed': 4, 'procedures': 19, 'controls': 48, 'bethlehem': 17, 'qalqiliya': 4, 'extremists': 61, 'sabotage': 18, 'halt': 67, 'macedonia': 30, 'designates': 1, 'albania': 15, 'eligible': 31, 'kunduz': 5, 'rural': 34, 'nimroz': 4, 'repatriated': 9, 'defectors': 12, 'thinking': 6, 'example': 15, 'informants': 5, 'executions': 11, 'discourage': 7, 'specifies': 2, 'returns': 27, 'koreans': 32, 'precision': 2, 'airstrike': 34, 'elder': 13, 'ripped': 37, 'naqib': 4, 'specify': 15, 'agenda': 42, 'europeanunion': 1, 'satisfied': 9, 'manuel': 29, 'bloc': 62, 'barroso': 14, 'rejecting': 29, 'chechnya': 31, 'latvia': 32, 'agreements': 72, 'estonia': 16, 'seek': 94, 'urge': 23, 'settlements': 40, 'mortars': 27, 'abdel': 28, 'razek': 1, 'majaidie': 1, 'response': 145, 'shelling': 14, 'gunfire': 38, 'jail': 66, 'jailhouse': 2, 'masked': 15, 'collaborators': 2, 'zawahiri': 35, 'ayman': 28, 'lithuania': 22, 'congratulate': 5, 'schroeder': 49, 'optimism': 8, 'chancellor': 66, 'adamkus': 4, 'lithuanian': 6, 'congratulated': 15, 'conversation': 13, 'partners': 31, 'modernization': 8, 'objectivity': 3, 'questioned': 63, 'vastly': 4, 'characterized': 16, 'opponent': 21, 'ernesto': 9, 'forecasters': 61, 'thinks': 15, 'urging': 91, 'posed': 18, 'debby': 2, 'weakened': 20, 'miami': 26, 'cape': 23, 'verde': 8, 'addis': 16, 'ababa': 15, 'unity': 63, 'explain': 16, 'overthrow': 42, 'zenawi': 10, 'meles': 9, 'conspiring': 7, 'suggested': 44, 'commit': 16, 'genocide': 88, '129': 5, 'treason': 17, 'journalists': 152, 'disciplinary': 8, 'shielded': 1, 'troubling': 3, 'extremely': 30, 'prosecution': 36, 'undermined': 7, 'proceedings': 33, 'stephanides': 2, 'cypriot': 26, 'nationals': 60, 'impoverished': 29, 'siphoned': 2, 'devised': 2, 'away': 133, 'complying': 4, 'pullback': 4, 'millimeters': 2, 'tank': 25, 'stealing': 14, 'happy': 18, 'disarmament': 27, 'firmly': 4, 'swaths': 4, 'huge': 79, 'kyrgyz': 18, 'andijan': 11, 'uzbekistan': 38, 'teshiktosh': 1, 'karasu': 1, 'villages': 69, 'karimov': 12, 'uzbek': 32, 'radicals': 7, 'restraint': 11, 'roman': 53, 'catholic': 71, 'chapel': 1, 'religions': 5, 'forgiveness': 5, 'pontiff': 51, 'promotion': 15, 'armies': 6, 'catholics': 17, 'evil': 11, 'love': 17, 'settling': 2, 'turkey': 348, 'objections': 19, 'retain': 16, 'luxembourg': 23, 'negotiating': 19, 'framework': 15, 'agree': 73, 'officially': 64, 'midnight': 19, 'indirect': 13, 'associate': 15, 'mubarak': 78, 'hosni': 42, 'supporters': 194, 'embarks': 1, 'gadhafi': 20, 'oath': 15, 'moammar': 11, 'libyan': 29, '77': 21, 'nationally': 9, 'nascent': 2, 'legislative': 57, 'irregularities': 28, 'rife': 3, 'criticisms': 4, 'challengers': 4, 'fans': 20, 'football': 77, 'soccer': 31, 'travels': 14, 'guarantees': 13, 'qualifying': 9, 'spectators': 5, 'protected': 13, 'safely': 11, 'precondition': 3, 'hosoda': 4, 'hiroyuki': 4, 'scenes': 5, 'mob': 11, 'game': 55, 'throwing': 18, 'cameras': 8, 'cans': 2, 'field': 63, 'victorious': 6, 'path': 26, 'rare': 37, 'occurrence': 4, 'drones': 13, 'detect': 11, 'flying': 50, 'defenses': 11, 'weaknesses': 7, 'looking': 78, 'programs': 95, 'editions': 3, 'launching': 40, 'restricting': 11, 'reactor': 41, 'grade': 30, 'dated': 10, 'circumstances': 18, 'jae': 1, 'passing': 46, 'samuel': 14, 'saddened': 4, 'jang': 2, 'condolence': 3, 'repressive': 10, 'regimes': 7, 'practice': 39, 'extent': 10, 'exercises': 28, 'philippine': 43, 'carmen': 1, 'mindanao': 5, 'chanted': 19, 'jolo': 7, 'sayyaf': 15, 'animal': 34, 'solomon': 9, 'dolphins': 9, 'appalling': 2, 'prohibits': 2, 'training': 107, 'feed': 21, 'wfp': 24, 'devastation': 13, 'gustav': 10, 'ike': 4, 'hurricanes': 25, 'beans': 12, 'vegetable': 3, 'canned': 2, 'rations': 3, 'fish': 39, 'supply': 95, 'liquid': 9, 'stoves': 1, 'warehouses': 2, 'cooking': 5, 'storage': 13, 'winners': 13, 'announcing': 10, 'oslo': 10, 'nobel': 50, 'sweden': 61, 'medicine': 26, 'chemistry': 3, 'physics': 1, 'prizes': 3, 'cages': 1, 'shallow': 2, 'polluted': 6, 'pens': 2, 'gavutu': 1, 'overcrowded': 12, 'animals': 26, 'economics': 7, 'instituted': 9, 'philanthropist': 2, '1968': 10, 'alfred': 3, 'swedish': 33, 'check': 19, 'banquet': 3, '1896': 2, 'choose': 29, 'laureate': 20, 'chooses': 4, 'literary': 3, '1901': 2, 'barrage': 7, 'rounds': 35, 'mortar': 42, 'offices': 88, 'houses': 57, 'fortified': 11, 'landed': 38, 'embassies': 32, 'suffer': 22, 'scratches': 1, 'sunburn': 1, 'undernourished': 1, 'appear': 56, 'temporarily': 59, '38': 46, 'postpone': 16, 'fields': 32, 'offshore': 45, 'outgoing': 33, 'inacio': 20, 'da': 31, 'signature': 13, 'luiz': 19, 'silva': 32, 'lula': 20, 'ventures': 8, 'petrobras': 7, 'owned': 105, 'sole': 13, 'operator': 5, 'unexplored': 2, 'meters': 67, 'reserves': 55, 'southeastern': 96, 'below': 60, 'potentially': 22, 'floor': 14, 'barrels': 72, 'provision': 12, 'opinion': 65, 'wrong': 29, 'americans': 199, 'wild': 39, 'rehabilitate': 2, 'abc': 29, 'advantage': 27, 'indicates': 37, 'republicans': 37, 'equipped': 9, 'handle': 8, 'point': 98, 'democrats': 102, 'presumed': 5, 'hypothetical': 3, '51': 23, 'nears': 3, 'primaries': 5, 'hillary': 25, 'cite': 5, 'healthcare': 5, 'margin': 23, 'minus': 6, 'adults': 16, 'points': 74, 'plus': 16, 'castro': 126, 'welcomed': 42, 'fidel': 60, 'ailing': 15, 'brother': 85, 'tomb': 12, 'variety': 22, 'simon': 16, 'bolivar': 13, 'icon': 4, 'transported': 10, 'partner': 48, 'latin': 75, 'mixed': 28, 'pact': 48, 'reaction': 20, 'elbaradei': 33, 'praised': 72, 'undermine': 25, 'conducting': 50, '1998': 77, 'restricts': 3, 'federalism': 16, 'garang': 30, 'analyst': 7, 'yoh': 3, 'unisa': 1, 'credits': 5, 'gai': 2, 'lecturer': 2, 'pretoria': 6, 'advocacy': 13, 'shutdown': 6, 'somali': 148, 'materialized': 3, 'spla': 5, 'democratize': 1, 'roosevelt': 4, 'integrate': 2, 'disabled': 6, 'disability': 2, 'franklin': 4, 'award': 54, 'polish': 61, 'delano': 1, 'lech': 12, 'kaczynski': 17, 'disabilities': 2, 'guaranteed': 13, 'convention': 19, 'legs': 3, 'paralyzed': 4, '1945': 15, 'closure': 32, 'baidoa': 19, 'shabelle': 4, 'alarmed': 5, 'ogaden': 5, 'ethiopia': 113, 'zemedkun': 1, 'wardheer': 1, 'denial': 6, 'tekle': 1, 'hardware': 6, 'purported': 22, 'somalis': 19, 'bordering': 41, 'demands': 105, 'threatening': 64, 'kuwaiti': 16, 'rai': 2, 'previously': 112, 'confirming': 8, 'certified': 7, 'dominant': 20, '275': 16, '128': 8, 'briefly': 54, 'younis': 17, 'roof': 22, 'firing': 52, 'discontent': 6, 'strapped': 11, 'cash': 57, 'renounce': 20, "n't": 62, 'quantities': 10, 'produced': 46, 'possessions': 6, 'certain': 56, 'virgin': 7, 'petition': 20, 'timex': 3, 'inc': 18, 'generalized': 1, 'changes': 90, 'preferences': 5, 'requested': 47, 'covered': 26, 'tariff': 6, 'classifications': 1, '58': 26, 'categories': 1, 'decided': 89, 'potential': 78, 'producer': 62, 'priced': 10, 'seller': 4, 'battery': 10, 'operated': 36, 'thailand': 121, 'beneficiaries': 2, 'representative': 57, '1988': 29, 'totaled': 5, 'maurits': 1, 'portuguese': 13, 'nassau': 1, 'mauritius': 7, 'prince': 70, 'malay': 5, 'dutch': 101, '10th': 18, 'establishing': 21, 'cane': 3, 'overseeing': 16, 'plantation': 6, 'assumed': 34, '1715': 1, '1810': 1, 'napoleonic': 3, 'playing': 39, 'collection': 9, 'submarine': 7, 'strategically': 3, 'incomes': 10, 'attracted': 25, 'considerable': 14, 'stable': 55, 'adawe': 1, 'amiin': 2, 'premises': 4, 'saed': 1, 'apparel': 9, 'creole': 8, 'morocco': 56, 'manufacturing': 61, 'benefits': 48, 'remittances': 30, 'proximity': 8, 'costs': 75, 'phosphate': 11, 'exporter': 25, 'pursued': 11, 'vi': 3, 'steady': 19, 'performance': 34, 'industrial': 77, 'fta': 4, 'rates': 90, 'illiteracy': 2, 'subsidized': 6, 'slums': 7, 'urban': 22, 'replacing': 11, 'alleviating': 1, 'underdevelopment': 1, 'housing': 81, 'expanding': 27, 'reducing': 38, 'adapting': 1, 'widened': 4, 'sluggish': 5, 'deficits': 17, 'improving': 44, 'confronting': 5, 'moroccans': 9, 'products': 82, 'phosphates': 4, 'young': 104, 'wealth': 36, 'disparity': 2, 'centrally': 3, 'explanation': 11, 'averaged': 6, 'curb': 28, 'attracting': 17, 'gray': 5, 'crime': 79, 'residing': 1, 'dec': 4, 'offset': 13, 'catalyst': 4, 'albanians': 16, 'towering': 1, 'greece': 41, 'plots': 15, 'inefficient': 6, 'prevalence': 5, 'farming': 24, 'primarily': 33, 'hydropower': 9, 'reliance': 14, 'antiquated': 1, 'contribute': 27, 'environment': 57, 'inadequate': 27, 'lowest': 24, 'fdi': 3, 'climate': 61, 'embarked': 6, 'vlore': 1, 'lines': 57, 'kosovo': 96, 'generation': 13, 'completion': 11, 'montenegro': 22, 'relieve': 5, 'thermal': 3, 'barrier': 20, 'rail': 14, 'spared': 4, 'plagued': 28, 'exploiting': 11, 'except': 20, 'deposits': 19, 'connections': 4, 'hinder': 5, 'adequate': 18, 'location': 35, 'original': 25, '1986': 18, 'compact': 13, 'fsm': 1, 'payouts': 3, '2023': 2, 'establishes': 5, 'perpetuity': 1, 'micronesia': 3, 'amended': 9, 'contributions': 23, 'federated': 7, 'fragile': 13, 'appears': 58, 'reduction': 39, 'outlook': 7, 'discovery': 41, 'fluctuating': 2, 'contributed': 36, 'swings': 2, 'exploitation': 9, 'components': 15, 'dominate': 15, 'livelihood': 11, 'equatorial': 7, 'successive': 9, 'cocoa': 22, 'diminished': 4, 'intention': 25, 'reinvest': 1, 'neglect': 4, 'guinea': 31, 'mismanagement': 11, 'transparency': 16, 'extractive': 6, 'candidacy': 17, 'tantalite': 1, 'undeveloped': 2, 'zinc': 6, 'diamonds': 14, 'columbite': 1, 'peaked': 4, 'taunted': 2, 'canada': 103, 'boss': 8, 'montreal': 5, 'gone': 36, 'tears': 3, 'parting': 1, 'attractions': 3, 'solely': 5, 'pray': 16, 'forgive': 3, 'neck': 8, 'rite': 1, 'touching': 5, 'reservists': 3, 'abusing': 21, 'w': 19, 'please': 7, 'drive': 47, 'character': 10, 'george': 81, 'headlights': 2, 'night': 123, 'kerry': 13, 'doughnut': 1, 'coffee': 20, 'topologist': 1, 'unjust': 2, 'lot': 15, 'apples': 2, 'condemn': 8, 'remind': 2, 'profession': 5, 'lonely': 2, 'very': 96, 'imaginary': 3, 'courts': 37, 'sabrina': 2, 'hood': 6, 'graner': 2, 'instead': 76, 'sergeant': 14, 'harman': 1, 'javal': 1, 'trips': 18, 'orbits': 2, 'roskosmos': 1, 'capsule': 2, 'quote': 28, 'ton': 17, 'siberia': 9, '2018': 2, 'launch': 111, 'module': 4, 'engine': 15, 'replacement': 14, 'scrutiny': 11, 'rough': 10, 'phased': 8, 'soyuz': 4, 'landings': 2, 'cancellation': 10, 'timidria': 1, 'mali': 38, 'ates': 1, 'intimidated': 7, 'bondage': 1, 'slavery': 11, 'enslaved': 2, 'saharan': 16, 'routes': 20, 'reason': 53, 'venue': 8, 'generations': 9, 'africans': 20, 'embattled': 13, 'ouattara': 15, 'laurent': 19, 'gbagbo': 28, 'alassane': 7, 'presidency': 86, 'refusing': 14, 'yield': 12, 'organizing': 20, 'mbeki': 16, 'thabo': 12, 'dispatched': 13, 'restore': 63, 'meant': 49, 'split': 46, 'motorbike': 3, 'guard': 73, 'preempt': 2, 'leftist': 66, 'istanbul': 27, 'taksim': 2, 'marching': 5, 'flowers': 8, 'limits': 34, 'fear': 79, 'observances': 10, '1977': 10, 'thirty': 9, 'narrowed': 7, 'consideration': 17, 'mahdi': 12, 'adel': 9, 'contention': 3, 'leaves': 41, 'dawa': 3, 'runner': 12, 'belong': 14, '48': 26, 'challenging': 15, 'shipping': 21, 'ransom': 60, 'magnate': 5, 'kahraman': 1, 'sadikoglu': 1, 'enforced': 3, 'adequately': 5, 'addressing': 21, 'intolerance': 6, 'gaps': 4, 'racism': 11, 'adversity': 1, 'strasbourg': 13, 'essential': 20, 'continent': 55, '46': 32, 'brings': 30, 'longest': 14, 'aerial': 7, 'tramway': 3, 'armenia': 14, 'mountains': 24, 'serzh': 1, 'sarkisian': 1, 'tatev': 1, 'monastery': 3, 'vorotan': 1, 'highway': 41, 'feat': 3, 'gorge': 5, 'linking': 31, 'spans': 2, 'engineering': 13, 'monasteries': 2, 'attraction': 3, 'borders': 122, 'develop': 122, 'gholam': 4, 'alarcon': 6, 'haddad': 4, 'ricardo': 15, 'resumed': 60, 'thanked': 25, 'burton': 2, 'dan': 14, 'indiana': 10, 'intervene': 13, 'coordination': 22, 'colombian': 106, 'mexican': 100, 'endanger': 4, 'break': 64, 'deadlock': 13, 'alternative': 29, 'insisted': 30, 'privileged': 5, 'object': 12, 'austrians': 2, 'unacceptable': 26, 'goal': 65, 'proposals': 50, 'soften': 1, 'neighbor': 24, 'cooperate': 55, 'delayed': 56, 'commander': 181, 'slaughtering': 3, 'qasab': 3, 'eln': 16, 'francisco': 19, 'galan': 7, 'andres': 22, 'valencia': 6, 'locals': 10, 'sons': 18, 'successful': 45, 'practices': 21, 'worries': 17, 'buildup': 9, 'tone': 7, 'sino': 4, 'casts': 2, 'jintao': 43, 'hu': 80, 'arrive': 30, 'unfairly': 10, 'cheap': 6, 'manipulates': 1, 'changed': 39, 'gripping': 3, 'bringing': 57, 'disarm': 42, 'deby': 23, 'authorized': 25, 'idriss': 13, "n'djamena": 5, 'oust': 22, 'exceptional': 4, 'decree': 19, 'censor': 4, 'curtail': 11, 'command': 57, 'remove': 38, 'prasad': 5, 'koirala': 3, 'represents': 22, 'girija': 2, 'feelings': 7, 'curtailing': 3, 'reinstate': 7, 'paramilitary': 36, 'grown': 29, 'rallies': 26, 'curbing': 7, 'contact': 97, 'representing': 24, 'janabi': 1, 'represented': 17, 'colleague': 8, 'saadoun': 2, 'impossible': 12, 'deteriorating': 20, 'lawyer': 63, '143': 3, 'murders': 20, 'adjourned': 15, 'tel': 21, 'alert': 37, 'aviv': 20, 'entry': 34, 'barricades': 6, 'jams': 4, 'cultivation': 12, 'importance': 26, 'opium': 33, 'fequiere': 1, 'places': 35, 'polling': 47, 'distribute': 7, 'cards': 11, 'patrick': 11, 'identification': 11, 'preparations': 20, 'balloting': 25, 'aristide': 58, 'betrand': 1, 'jean': 58, 'calm': 31, 'shipment': 10, 'shoes': 12, 'traders': 22, 'tunnel': 27, 'blockade': 33, 'skirted': 2, 'suffocating': 2, 'israelis': 71, 'ivorian': 9, 'siegouekou': 1, 'happened': 69, 'smashed': 12, 'throats': 3, 'knives': 5, 'doors': 9, 'precious': 6, 'ingredient': 9, 'chocolate': 9, 'drilling': 16, 'barrel': 101, 'pipelines': 15, 'rita': 22, 'eased': 14, 'analysts': 132, 'spike': 7, 'falling': 51, 'unlike': 9, 'diminishing': 3, 'cutting': 46, 'excess': 7, 'refining': 18, 'veered': 5, 'flames': 13, 'runway': 11, 'aboard': 35, 'conflicting': 19, 'fatalities': 23, 'scene': 74, 'stopover': 2, 'originated': 9, 'amman': 18, 'aviation': 26, 'alternatives': 6, '115': 11, 'takeoff': 2, 'mykola': 1, 'azarov': 2, 'threaten': 22, 'suck': 1, 'neither': 44, 'possess': 7, 'waits': 2, 'hybrid': 13, 'deploy': 22, 'speeded': 1, 'tougher': 13, 'heroin': 21, 'battling': 52, 'directly': 41, 'nighttime': 3, 'karshi': 3, 'khanabad': 3, 'aftermath': 22, 'airfield': 4, 'criticizing': 24, 'bury': 2, 'fearing': 6, 'mudslides': 33, 'typhoons': 4, 'assessing': 8, 'powerful': 76, 'packing': 6, 'nanmadol': 2, 'heave': 1, 'arroyo': 21, 'gloria': 14, 'homeless': 46, 'hungry': 9, 'shelter': 42, 'rescue': 103, 'bekaa': 7, 'remaining': 72, 'en': 9, 'jumblatt': 5, 'cameroon': 17, 'issa': 3, 'respective': 6, 'tchiroma': 2, 'examinations': 2, 'bakassi': 2, 'commando': 6, 'marine': 56, 'splinter': 4, 'vessels': 26, 'ukrainians': 14, 'brain': 27, 'circulation': 5, 'findings': 28, 'advancement': 2, 'flow': 32, 'flavinols': 2, 'oxidant': 1, 'eating': 28, 'researchers': 40, 'bitter': 16, 'taste': 3, 'massacre': 35, 'testing': 36, 'foods': 5, 'calorie': 3, 'motorway': 2, 'scotland': 19, 'gleneagles': 3, 'a9': 1, 'stirling': 1, 'objects': 12, 'rocks': 18, 'hooded': 5, 'railway': 13, 'edge': 5, 'g': 33, 'canceled': 58, 'outbreaks': 50, 'surrounding': 40, 'turmoil': 22, 'kouchner': 6, 'strengthening': 27, 'pivotal': 1, 'afp': 33, 'hypothermia': 1, 'hospitalized': 35, 'exposure': 13, 'majid': 9, 'implicates': 4, 'basra': 45, 'execution': 35, 'hassan': 61, 'rangoon': 45, 'real': 72, 'supplier': 11, 'gambari': 5, 'spate': 6, 'escape': 49, 'inmate': 3, 'edward': 8, 'caraballo': 3, 'torturing': 6, 'running': 119, 'lance': 3, 'lieutenants': 7, 'pain': 14, 'hosseini': 6, 'lessen': 6, 'irna': 18, 'intermediary': 2, 'swiss': 44, 'supporting': 68, 'date': 96, 'expressing': 19, 'abandons': 2, 'apparent': 39, 'participates': 6, 'acknowledge': 11, 'exist': 20, 'reject': 31, 'stress': 8, 'makeshift': 8, 'shelters': 15, 'tent': 10, 'roaming': 4, 'offenses': 21, 'rapes': 8, 'topic': 3, 'input': 1, 'immediate': 112, 'volunteers': 22, 'serve': 57, 'notified': 11, 'postings': 1, 'cable': 21, 'dates': 11, 'thomas': 31, 'harry': 23, 'dismissal': 13, 'extra': 27, 'assignments': 4, 'precedents': 2, 'directed': 29, '1969': 8, 'nam': 7, 'aligned': 13, 'sidelines': 25, 'havana': 50, 'm': 31, 'fugitive': 26, 'warming': 38, 'coal': 44, 'emissions': 33, 'dioxide': 9, 'carbon': 21, 'schalkwyk': 2, 'efficiency': 11, 'marthinus': 1, 'mandatory': 7, 'greenhouse': 18, 'gases': 6, 'captures': 1, 'stores': 21, '2025': 2, 'hands': 41, 'deif': 2, 'humiliation': 2, 'removal': 12, 'nothing': 65, 'shaken': 10, 'iftikhar': 5, 'chaudhry': 15, 'abused': 20, 'judiciary': 18, 'interfere': 10, 'rana': 1, 'bhagwandas': 1, 'swore': 1, 'flu': 571, 'h5n1': 215, 'domestic': 108, 'poultry': 121, 'waterfowl': 3, 'dark': 10, 'bombmaker': 4, 'video': 126, 'shadow': 19, 'profile': 24, 'verified': 17, 'farm': 100, 'lyons': 1, 'lab': 18, 'slaughtered': 19, 'illness': 37, 'birds': 185, 'fears': 39, 'perth': 6, 'safin': 6, 'knee': 11, 'hopman': 4, 'persistent': 13, 'marat': 3, 'champion': 65, 'tendonitis': 1, 'struggled': 16, 'masters': 7, 'shanghai': 22, 'rather': 46, 'replaced': 39, 'kuznetsova': 1, 'teimuraz': 2, 'gabashvili': 2, 'svetlana': 1, 'serbia': 56, 'perceive': 1, 'occupying': 11, 'defuse': 15, 'convince': 10, 'pull': 42, 'impulse': 1, 'normalizing': 3, 'sochi': 5, 'chadian': 21, 'hijacker': 4, 'surrendering': 4, 'passenger': 44, '103': 10, 'fasher': 6, 'pistol': 6, 'asylum': 42, 'noordin': 6, 'extremist': 40, 'java': 26, 'subur': 2, 'sugiarto': 2, 'accomplices': 4, 'circle': 5, 'inner': 9, 'jemaah': 10, 'arm': 17, 'islamiah': 1, 'nightclub': 9, '202': 12, 'bali': 31, 'medics': 9, 'avenge': 4, 'netanya': 1, 'jihad': 67, 'shaul': 12, 'mofaz': 15, 'maher': 7, 'bet': 5, 'uda': 3, 'caught': 60, 'shin': 2, 'branch': 20, 'detain': 9, 'commented': 29, 'tass': 13, 'intoxicated': 2, 'itar': 13, 'authentic': 3, 'governmental': 23, 'responding': 25, 'nutrition': 8, 'famine': 19, 'drought': 39, 'ethnically': 8, 'yugoslav': 31, 'subpoena': 4, 'slobodan': 13, 'milosevic': 40, 'signs': 51, 'encourage': 41, 'proudly': 1, 'separation': 12, 'believers': 5, 'infringe': 2, 'requesting': 5, 'testimony': 29, 'responses': 4, 'writing': 21, 'judges': 42, 'instructed': 7, 'rudolf': 2, 'witness': 28, 'scharping': 1, 'albright': 1, 'madeleine': 4, 'counsel': 10, 'appointed': 60, 'failing': 58, 'conflicts': 32, 'counts': 25, 'pardoned': 11, 'pardon': 9, 'boards': 5, '765': 1, 'pardons': 10, 'eve': 52, 'celebration': 18, 'celebrated': 29, 'rest': 60, 'specific': 33, 'apostolic': 2, 'eucharist': 2, 'gregorian': 1, 'julian': 5, 'follows': 63, 'calendar': 11, 'examining': 9, 'afar': 16, 'applications': 8, 'chickens': 60, 'kapisa': 3, 'detected': 35, 'assadullah': 2, 'azhari': 3, 'laboratory': 42, 'samples': 43, 'logar': 8, 'nangarhar': 15, 'laghman': 5, 'suspicions': 7, 'parwan': 2, 'fao': 11, 'sharp': 39, 'conspicuous': 1, 'skullcaps': 1, 'headscarves': 3, 'controversy': 18, 'crucifixes': 2, 'donald': 54, 'mismanaging': 1, 'rumsfeld': 75, 'remembered': 8, 'carolina': 27, 'worst': 89, 'secretaries': 3, 'supporter': 14, 'farewell': 4, 'devotion': 1, 'finest': 1, 'hasty': 1, 'senses': 3, 'properly': 20, 'reverence': 2, 'too': 97, 'pulling': 16, 'themselves': 60, 'reiterated': 11, 'failures': 12, 'levels': 75, 'taped': 7, 'orleans': 94, 'dangers': 9, 'blacks': 5, 'disproportionately': 1, 'quick': 20, 'dramatically': 20, 'strokes': 4, 'lancetand': 1, 'lancet': 1, 'neurology': 1, 'journals': 1, 'counteracting': 1, 'vast': 29, 'oxford': 2, 'researcher': 2, 'rothwell': 1, 'symptoms': 22, 'professionals': 15, 'care': 101, 'aggressive': 25, 'tissue': 5, 'causes': 37, 'numbness': 1, 'slurred': 1, 'sudden': 7, 'headaches': 3, 'partial': 20, 'facial': 2, 'cholesterol': 3, 'thinning': 2, 'medications': 6, 'treatments': 6, 'crude': 144, 'reflecting': 3, 'inventories': 18, 'decreased': 17, '35': 73, 'cents': 51, '68': 14, 'tenths': 15, 'publishing': 17, 'cartoons': 46, 'bayji': 4, 'rubble': 27, 'munitions': 8, 'observed': 23, 'guided': 4, 'emile': 16, 'condolences': 21, 'express': 20, 'slain': 20, 'lahoud': 25, 'mourners': 20, 'drawings': 4, 'project': 100, 'traces': 6, '05': 13, 'recorded': 34, 'easing': 20, 'investors': 69, 'weakening': 10, 'tangible': 3, 'attractive': 7, 'speculators': 3, 'ben': 20, 'pointing': 3, 'bernanke': 16, 'interpreted': 6, 'weaken': 19, 'reductions': 5, 'exporting': 33, 'opec': 59, 'cartel': 25, 'pump': 8, 'gerard': 12, 'bertrand': 29, 'latortue': 14, 'objection': 4, 'publication': 19, 'disagrees': 3, 'blasphemous': 9, 'exile': 57, 'followers': 22, 'foes': 4, 'ignore': 9, 'prohibiting': 4, 'disturbing': 2, 'perceptions': 3, 'trend': 13, 'exceptions': 2, 'prohibitions': 1, 'tool': 7, 'instrument': 4, 'alarm': 5, 'circumvent': 2, '33': 39, 'wording': 2, 'inconvenient': 2, 'meteorologists': 5, 'strengthened': 22, 'category': 18, 'opportunity': 46, 'missed': 25, 'bahamas': 13, '120': 44, 'khaled': 18, 'recruited': 7, 'meshaal': 7, 'viewed': 12, 'assassinated': 20, 'unusually': 7, 'nordic': 9, 'affecting': 9, 'baltic': 14, 'aziz': 54, 'shaukat': 18, 'buses': 22, 'produce': 75, 'purposes': 40, 'negotiator': 45, 'length': 11, 'rohani': 2, 'vigilant': 4, 'uphold': 4, 'imposition': 4, 'fails': 21, 'petersburg': 12, 'shrapnel': 5, 'mcdonald': 10, 'st': 35, 'injuring': 35, 'ceiling': 3, 'shattered': 10, 'secretly': 48, 'environmental': 63, 'disprove': 1, 'investigators': 75, 'prospekt': 2, 'nevsky': 2, 'table': 14, 'tracked': 4, 'airspace': 21, 'drone': 35, 'ten': 25, 'meddling': 3, 'cater': 2, 'stricter': 3, 'parlors': 1, 'beauty': 9, 'enforce': 14, 'salons': 1, 'interpretation': 2, 'asefi': 16, 'reza': 22, 'installation': 8, 'cafes': 7, 'unsuitable': 1, 'shops': 37, 'brand': 10, 'fundamentalist': 4, 'hairdresser': 1, 'impacted': 6, 'ghoul': 2, 'makeup': 6, 'violate': 29, 'nulcear': 1, 'alleges': 13, 'parchin': 10, 'photographer': 12, 'gabriele': 5, 'torsello': 10, 'libya': 35, 'advised': 15, 'kind': 25, 'indecent': 3, 'broadcasters': 5, 'broadcasting': 25, 'content': 13, 'supervise': 3, 'films': 20, 'conservative': 70, 'principles': 12, 'platform': 19, 'performances': 6, 'sharply': 72, '1979': 28, 'appointment': 20, 'snyder': 3, 'rini': 5, 'survival': 6, 'honiara': 3, 'downer': 10, 'businessmen': 19, '110': 17, 'retrieved': 5, 'wreckage': 21, 'recorder': 4, 'airliner': 15, 'box': 11, 'blizzard': 5, 'survived': 53, 'asserts': 3, 'addicts': 1, 'rehabilitation': 13, 'clients': 12, 'lined': 6, 'tijuana': 4, 'identify': 42, 'gangs': 35, 'cartels': 14, 'calderon': 20, 'engaged': 33, 'felipe': 21, 'marijuana': 7, 'tons': 54, '105': 14, 'bust': 2, 'holmes': 2, 'horn': 16, 'outskirts': 24, 'squalid': 1, 'munich': 8, 'brutalized': 1, 'amount': 46, 'shirin': 3, 'ebadi': 5, 'blatant': 4, 'summons': 2, 'voices': 6, 'complaint': 16, 'doubt': 21, 'handles': 5, 'noting': 22, 'matters': 14, 'cast': 49, 'bases': 50, 'restructure': 6, 'installations': 15, 'jersey': 16, 'deliberations': 1, 'virginia': 20, 'commissioners': 1, 'realignment': 1, 'closures': 6, 'mcpherson': 1, 'recommended': 25, 'maine': 5, 'hawaii': 9, 'trained': 33, 'zawahri': 4, 'save': 37, 'submit': 17, 'certify': 2, 'husband': 42, 'instantly': 3, 'transporting': 20, 'planted': 29, 'mistook': 6, 'toy': 8, 'shaped': 10, 'dir': 5, 'zaman': 2, 'placed': 69, 'deliberately': 11, 'accidentally': 18, 'taxi': 11, 'degrees': 18, 'celsius': 13, 'hover': 1, 'cds': 2, 'hurriyet': 1, 'unlicensed': 3, 'laptops': 2, 'pech': 1, 'kunar': 34, 'nationality': 17, 'whom': 47, 'younus': 1, 'khalis': 7, 'thwarted': 6, 'pisanu': 3, 'giuseppe': 1, 'bologna': 2, 'subway': 40, 'cagliari': 1, 'milan': 11, 'basilica': 11, 'sardinia': 2, 'expelled': 19, 'petronio': 1, 'tycoon': 14, 'conviction': 21, 'khodorkovsky': 40, 'features': 16, 'fresco': 1, 'insulting': 10, 'italians': 6, 'regulate': 8, 'strictly': 7, 'submission': 3, 'amendments': 21, 'suggestions': 11, 'presently': 3, 'oversight': 11, 'branches': 8, 'various': 52, 'kremlin': 38, 'reflection': 6, 'misanthropic': 1, 'ideologies': 2, 'afford': 13, 'crucial': 28, 'businessman': 22, 'selling': 46, 'complicity': 8, 'pretrial': 2, 'ingredients': 6, 'frans': 1, 'anraat': 3, 'rotterdam': 1, 'richest': 9, 'retaliation': 27, 'backing': 43, 'chemicals': 17, '62': 17, 'defendant': 20, 'halabja': 6, 'girl': 74, 'underway': 26, 'motions': 2, 'fatality': 10, '73': 31, '171': 5, '59': 24, 'drawdown': 2, 'coinciding': 3, 'opposed': 59, 'innocence': 5, 'bureaucrats': 1, 'renaming': 1, 'advice': 9, 'arresting': 17, 'adnan': 7, 'shocked': 8, 'dulaimi': 7, 'sectarianism': 1, 'marginalized': 2, 'giant': 68, 'yukos': 52, 'technological': 6, 'qinghong': 2, 'zeng': 5, 'exploration': 26, 'shortfall': 6, 'trinidad': 2, 'tobago': 2, 'arriving': 36, 'tikrit': 28, 'blankets': 7, 'wrapped': 13, 'balad': 21, 'discrepancy': 1, 'reconcile': 6, 'shortage': 27, 'suspending': 18, 'roadway': 1, 'festivities': 11, 'bicycle': 13, 'inquiry': 32, 'bhutto': 23, 'benazir': 12, 'widower': 2, 'creation': 39, 'asif': 20, 'fact': 21, 'ki': 26, 'zardari': 31, 'medalist': 11, 'reasons': 40, 'au': 94, 'evi': 1, 'sachenbacher': 1, 'epo': 1, 'endurance': 1, 'naturally': 3, 'kikkan': 1, 'leif': 1, 'zimmermann': 2, 'randall': 1, 'suspensions': 4, 'punishments': 4, 'retroactive': 3, 'races': 6, 'worse': 26, 'superiors': 4, 'assert': 5, 'repairs': 10, 'refinery': 27, 'view': 30, 'optimistic': 17, 'scattered': 9, 'yves': 2, 'h': 16, 'grubbs': 2, 'chauvin': 2, 'schrock': 2, 'academy': 19, 'sciences': 5, 'stockholm': 10, 'plastics': 1, 'environmentally': 7, 'trio': 7, 'metathesis': 1, 'molecules': 2, '1971': 10, 'rearranged': 1, 'developed': 69, 'catalysts': 1, 'reproduce': 2, 'efficient': 18, 'centrifuges': 13, 'scientist': 21, 'qadeer': 8, 'impassable': 2, 'hindering': 4, 'irresponsible': 11, 'virtual': 9, 'secrets': 15, 'father': 76, 'togo': 35, 'ecowas': 5, 'installed': 31, 'faure': 10, 'togolese': 13, 'gnassingbe': 29, 'reacting': 4, 'eyadema': 11, 'naming': 6, 'branded': 2, 'violation': 34, 'seizure': 22, 'premier': 12, 'hotspur': 1, 'stint': 2, 'beckham': 9, 'tottenham': 2, 'club': 29, 'english': 44, 'rationing': 3, 'galaxy': 6, 'hoped': 26, 'teammates': 2, 'mls': 3, 'ac': 3, 'achilles': 2, 'offseason': 1, 'tendon': 1, 'tore': 17, 'reluctant': 5, 'hotspurs': 1, 'redknapp': 1, '76th': 3, 'unconditional': 4, 'birthday': 20, 'tin': 12, 'without': 280, 'write': 14, 'chronic': 11, 'ailments': 6, 'proper': 17, 'propaganda': 21, 'bombed': 27, 'tanker': 23, 'khyber': 17, 'attached': 20, 'regularly': 21, 'pass': 62, 'inspector': 19, 'earmarked': 5, 'bowen': 2, 'stuart': 8, 'documentation': 5, 'haste': 4, 'incompetence': 3, 'indications': 8, 'account': 64, 'militia': 89, 'designated': 24, 'taxpayer': 3, 'difficulties': 18, 'vow': 7, 'kiev': 39, 'repeat': 14, 'presidents': 56, 'aleksander': 2, 'valdas': 3, 'kwasniewski': 3, 'dominican': 20, 'alpha': 13, 'barahona': 1, 'maximum': 24, '22nd': 4, 'breaking': 21, 'alphabet': 3, 'greek': 69, 'caicos': 2, 'turks': 16, 'consumption': 17, 'cleaning': 11, 'switching': 1, 'proponents': 3, 'clean': 35, 'stiff': 12, 'mistakenly': 13, 'henan': 5, 'miners': 55, 'eilat': 2, 'weapon': 42, 'cocked': 1, 'chest': 12, 'realized': 8, 'withheld': 7, 'armorgroup': 2, 'employee': 23, 'withhold': 4, 'guardian': 14, 'stepping': 19, 'magna': 7, 'mcalpine': 8, 'stronach': 5, 'frank': 19, 'automotive': 7, 'xinhua': 126, 'dengfeng': 1, 'outburst': 2, 'satisfactory': 4, 'profit': 34, 'achieved': 34, 'overhead': 7, 'stephen': 18, 'akerfeldt': 1, 'downturn': 30, 'load': 12, 'enters': 2, 'declines': 12, 'wallowing': 1, 'canadian': 77, 'dividend': 8, 'shares': 52, '125': 10, 'yesterday': 18, '625': 3, 'stock': 83, 'controlling': 13, 'unsuccessfully': 7, 'shareholder': 8, 'seat': 71, 'throughout': 83, 'manfred': 3, 'gingl': 1, 'personally': 13, 'assisted': 10, 'restructuring': 23, '108': 2, 'consulting': 9, 'colonization': 3, '1719': 2, 'caribs': 1, 'saint': 31, '18th': 24, 'ceded': 16, '1783': 4, 'grenadines': 5, 'indies': 9, '1962': 19, '1960': 26, 'hungarian': 23, 'dissolution': 9, 'austro': 9, 'slovene': 3, 'multinational': 15, '1929': 7, 'croats': 14, 'serbs': 24, 'slovenes': 4, '1918': 6, 'slovenia': 18, 'distanced': 4, 'dissatisfied': 6, '1991': 86, 'exercise': 37, 'succeeded': 24, 'transformation': 7, 'historical': 8, 'acceded': 3, 'eurozone': 2, 'dynasty': 9, 'serbian': 58, 'ottoman': 16, 'subsequent': 28, 'principality': 9, 'zeta': 2, '15th': 19, 'crnojevic': 1, '1852': 1, 'theocracy': 1, 'princes': 1, 'bishop': 9, 'secular': 26, 'constituent': 7, 'absorbed': 4, 'looser': 1, '1992': 37, 'invoked': 2, 'exceeded': 9, 'threshold': 2, 'severing': 3, 'declare': 21, 'lesotho': 9, 'basutoland': 1, '1966': 8, 'renamed': 5, 'basuto': 1, 'heilongjiang': 6, 'hegang': 1, 'exiled': 22, 'iii': 7, 'moshoeshoe': 1, 'letsie': 1, '1990': 61, 'restored': 38, 'aegis': 1, 'contentious': 10, 'botswana': 12, 'mutiny': 2, 'relative': 22, 'hotly': 6, 'proportional': 2, 'contested': 16, 'applied': 12, 'aggrieved': 2, 'mainstays': 4, 'enjoys': 11, 'compared': 54, 'accommodate': 5, 'curacao': 5, 'excellent': 10, 'tankers': 13, 'harbor': 14, 'refined': 6, 'single': 59, 'leases': 2, 'ins': 4, 'cave': 8, 'deadliest': 52, 'attempting': 27, 'soils': 2, 'hamper': 6, 'complicate': 4, 'pension': 18, 'systems': 47, 'budgetary': 8, 'horse': 14, 'hunter': 20, 'arisen': 1, 'quarrel': 3, 'revenge': 11, 'stag': 8, 'guide': 6, 'jaws': 1, 'reins': 2, 'iron': 15, 'saddle': 1, 'conquer': 4, 'saddled': 2, 'bridled': 1, 'overcame': 6, 'mouth': 16, 'friend': 24, 'prefer': 4, 'bit': 17, 'spur': 11, 'theirs': 1, 'violated': 22, 'vietnamese': 21, 'raising': 50, 'foil': 2, 'tree': 25, 'pomegranate': 1, 'beautiful': 6, 'height': 9, 'dear': 2, 'boastful': 1, 'bramble': 4, 'hedge': 4, 'disputings': 1, 'vain': 4, 'traveller': 3, 'flourishing': 2, 'splendid': 3, 'orders': 45, 'fill': 15, 'inquired': 7, 'boxing': 2, 'replied': 35, 'gloves': 1, 'pugilists': 1, 'tongues': 1, 'personality': 4, 'accountant': 2, 'statistician': 1, 'generals': 27, 'ellsworth': 3, 'dakota': 5, 'scrambling': 5, 'heard': 42, 'noon': 5, 'frost': 1, 'commanding': 8, 'curfews': 13, 'musicians': 11, 'whispered': 1, 'o': 9, 'gauge': 5, 'index': 43, 'indicators': 7, 'predict': 18, 'prompting': 23, 'andrew': 17, 'kfm': 1, 'mwenda': 3, 'junk': 4, 'sedition': 1, 'museveni': 22, 'yoweri': 13, 'assignment': 2, 'rory': 1, 'vanished': 6, 'irishman': 1, 'airports': 22, 'iskandariyah': 6, 'counting': 21, 'auditing': 4, 'migrants': 36, 'ecuadorean': 10, 'sank': 9, 'hoping': 31, 'airplane': 10, 'participating': 20, 'ecuador': 73, 'manta': 1, 'doomed': 2, 'sail': 5, 'ivanov': 29, 'broadly': 3, 'evolves': 1, 'participate': 43, '12th': 13, 'sterilization': 3, 'reverse': 23, 'surgery': 55, 'opt': 1, 'students': 86, 'grieving': 7, 'parents': 30, 'quell': 16, 'govern': 7, 'strategies': 9, 'divisions': 12, 'globalization': 10, 'resolving': 27, 'stockhlom': 1, 'harold': 1, 'pinter': 1, 'era': 31, 'centimeter': 3, 'mocked': 1, 'shahr': 1, 'kord': 1, 'incentives': 43, 'javier': 16, 'solana': 25, 'mitchell': 17, 'launches': 12, 'holds': 45, 'saeb': 11, 'erekat': 11, 'forth': 5, 'shuttle': 44, 'firefight': 19, 'gunfight': 13, 'implicated': 19, 'raz': 1, 'loyalists': 5, 'hunting': 28, 'neighbors': 48, 'venezuelans': 7, 'annulled': 4, 'corrected': 4, 'mistakes': 5, 'amend': 11, 'overseen': 3, 'promotes': 3, 'landmark': 22, 'turnout': 11, 'erben': 2, 'drop': 61, 'intermittent': 3, 'sixto': 3, 'theft': 11, 'kanyabayonga': 2, 'units': 29, 'reinforcements': 7, 'kinshasa': 15, 'hutu': 31, 'rwandan': 45, 'rwanda': 58, '1994': 62, 'zoellick': 22, 'tested': 65, 'ninh': 3, 'binh': 1, 'learning': 11, 'intergovernmental': 5, 'li': 17, 'zhaoxing': 3, 'jiabao': 12, 'wen': 17, 'chengdu': 3, 'janeiro': 15, 'rio': 19, 'clashing': 3, 'daylight': 5, 'providencia': 10, 'lobbing': 3, 'exchanged': 12, 'shantytown': 3, 'downtown': 17, 'fragments': 2, 'barracks': 7, 'mobilized': 5, 'slum': 11, 'complained': 32, 'dwellers': 2, 'kills': 14, 'apologized': 18, 'nana': 1, 'apenteng': 1, 'effah': 1, 'drafting': 18, 'resolve': 87, 'precede': 1, 'urgency': 2, 'chirac': 49, 'cubans': 24, 'renovation': 2, 'blackouts': 3, 'plants': 38, 'ones': 23, 'generators': 3, 'electrical': 19, 'conditioning': 1, 'hottest': 2, 'appliances': 2, 'surges': 4, 'rot': 1, 'admitted': 53, 'resold': 1, 'radios': 11, 'bullets': 12, 'stray': 3, 'siad': 13, 'barre': 16, 'crossing': 87, 'fence': 23, 'kissufin': 1, 'spotting': 1, 'holed': 6, 'terminal': 5, 'karni': 6, 'tanks': 28, 'bulldozers': 7, 'farmlands': 1, 'yunis': 2, 'incursions': 6, 'welcomes': 8, 'morales': 54, 'evo': 22, 'humiliations': 2, 'forgives': 2, 'bolivian': 24, 'inauguration': 24, 'welcome': 24, 'coca': 17, 'eradication': 9, 'uses': 20, 'campaigned': 8, 'cocaine': 26, 'sure': 30, 'bolivia': 62, 'plotting': 46, 'institution': 12, 'ridiculous': 6, 'apart': 22, 'regret': 11, 'mulford': 4, 'regrets': 9, 'context': 1, 'sincere': 7, 'inappropriate': 10, 'shyam': 4, 'summoned': 14, 'saran': 5, 'conductive': 1, 'inspection': 15, 'factories': 21, 'searches': 13, 'moderate': 53, 'densely': 11, 'populated': 18, 'confined': 7, 'uncovered': 32, 'laboratories': 8, 'cycle': 7, 'spark': 17, 'hub': 13, 'defending': 23, '74': 12, 'welfare': 18, 'shihab': 3, 'alwi': 1, 'magnitude': 50, 'measured': 10, 'deliveries': 19, 'obstructing': 5, 'deny': 32, 'petersen': 7, 'kilinochchi': 3, 'assess': 19, 'reviving': 6, 'progressive': 17, '225': 10, 'unification': 24, 'vied': 1, '386': 2, '176': 1, 'allocated': 8, 'proportion': 5, 'receives': 6, 'kush': 1, 'hindu': 18, 'somalians': 2, 'hijacking': 10, 'boarded': 9, 'merka': 1, 'unloaded': 2, 'miltzow': 2, 'afternoon': 38, 'maize': 4, 'torgelow': 2, 'freighter': 3, 'semlow': 4, 'kenya': 120, 'sailed': 5, 'donated': 17, 'peshawar': 24, 'chitral': 3, 'felt': 12, 'inspect': 13, 'turnabout': 1, 'brothers': 18, 'ahbash': 3, 'refuses': 12, 'draft': 77, 'sponsoring': 3, 'veto': 27, 'oppose': 55, 'saad': 13, 'vaccination': 12, 'damages': 21, 'oromia': 1, 'tigray': 1, 'amhara': 1, 'kicked': 13, 'immunizations': 5, 'judicial': 28, 'experience': 30, 'appeals': 37, 'alito': 25, 'mastery': 4, 'qualities': 2, 'disappointed': 11, 'retiring': 10, 'sandra': 11, "o'connor": 12, 'lacked': 9, 'nominated': 31, 'miers': 8, 'harriet': 4, 'colleagues': 22, 'humayun': 1, 'hamidzada': 2, 'grata': 1, 'persona': 1, 'tribes': 12, 'misunderstanding': 2, 'siddique': 2, 'clarify': 3, 'aleem': 2, 'wedding': 9, 'ankara': 40, 'bilge': 1, 'mardin': 1, 'allotment': 1, 'atalay': 1, 'besir': 1, 'ntv': 2, 'clan': 11, 'feud': 6, 'democratization': 4, 'farmaner': 1, 'forget': 2, 'suppression': 7, 'legislators': 22, 'extending': 32, 'moo': 11, 'roh': 15, 'endorsed': 31, 'hyun': 11, 'contingent': 21, 'assigned': 8, 'arbil': 3, 'smoke': 17, 'sections': 9, 'nonsmokers': 2, 'restaurants': 15, 'secondhand': 4, 'surgeon': 5, 'tobacco': 15, 'really': 10, 'lung': 7, 'asthma': 3, 'infant': 8, 'infections': 19, 'prevention': 16, '430': 5, 'portugal': 32, 'weekend': 27, 'lisbon': 3, 'charities': 8, 'ticket': 10, 'lineup': 5, 'wide': 53, 'incoming': 16, 'implies': 2, 'nominees': 18, 'objected': 9, 'motorcade': 13, 'rugova': 4, 'am': 24, 'blown': 18, 'window': 15, 'schedule': 30, 'proves': 5, 'elements': 16, 'hundred': 33, 'crawford': 10, 'ranch': 28, 'elevated': 3, 'shift': 15, 'capitals': 8, 'hatched': 1, 'theater': 13, 'candle': 1, 'suef': 2, 'stampede': 10, 'beni': 3, 'exit': 16, 'triggering': 24, 'burning': 32, 'storey': 1, 'blaze': 15, '350': 17, 'farah': 14, 'contrast': 4, '60th': 18, 'encountered': 16, 'calmly': 1, 'hall': 37, 'baden': 1, 'merkel': 58, 'protested': 34, 'angela': 31, 'detaining': 12, 'cordon': 3, 'cindy': 4, 'rationale': 2, 'sheehan': 6, 'bins': 1, 'trash': 8, 'absence': 13, 'generating': 9, 'shwe': 21, 'aye': 4, 'maung': 7, 'hosted': 18, 'routine': 18, 'suffers': 15, 'hypertension': 2, 'gravely': 3, 'rumors': 6, 'squared': 2, 'motors': 24, 'loss': 76, 'gm': 14, 'posted': 66, 'quarter': 88, '722': 2, 'hourly': 1, 'buyouts': 1, 'automaker': 11, 'struggling': 51, 'cars': 67, 'dana': 8, 'perino': 7, 'competitive': 11, 'daniele': 4, 'repubblica': 4, 'mastrogiacomo': 10, 'sequi': 1, 'ettore': 1, 'francesco': 1, 'covering': 21, 'rajouri': 2, 'miles': 5, 'responsiblity': 1, 'influenza': 19, 'avian': 58, 'understands': 8, 'sentiments': 4, 'rivals': 32, 'purpose': 16, 'explained': 8, 'mediation': 10, 'battles': 21, 'capabilities': 17, 'sydney': 21, 'historic': 34, 'marketplace': 5, 'forum': 31, 'ore': 7, 'negotiated': 14, 'zealand': 51, 'mired': 9, 'elderly': 15, 'bullet': 11, 'riddled': 6, 'else': 18, 'note': 20, 'remnants': 16, 'reversing': 4, '57': 28, 'juncker': 2, 'staunch': 3, 'claude': 3, 'denmark': 45, 'worrisome': 1, 'gears': 1, 'resurgence': 3, 'juncture': 1, 'prepares': 19, 'ouster': 23, 'benefit': 24, 'dubious': 1, 'origin': 15, 'tainted': 9, 'criminals': 31, 'pushed': 64, 'militarized': 3, 'directions': 3, 'crossings': 14, 'khalikov': 1, 'needing': 1, 'diarrhea': 10, 'approaching': 15, 'judgments': 2, 'ministerial': 10, 'chair': 14, 'kuwait': 49, 'kingpin': 5, 'orlandez': 3, 'gamboa': 3, 'smuggled': 16, 'boasted': 2, 'lords': 3, 'covertly': 4, 'laundering': 21, 'extradited': 21, 'barranquilla': 1, 'shipped': 11, 'entities': 11, 'inflated': 6, 'confederation': 11, 'distorting': 3, 'losses': 63, 'hurt': 97, 'habits': 6, 'ultimately': 11, 'counterproductive': 5, 'structure': 25, 'salary': 10, '95': 31, 'employed': 17, 'laid': 23, 'harms': 3, 'leadership': 79, 'plo': 2, 'excuse': 9, 'obstruct': 1, 'reciprocated': 1, 'bp': 15, 'dudley': 3, 'tnk': 4, 'signaling': 4, 'hayward': 2, 'sale': 52, 'spill': 23, 'discussions': 43, 'compelled': 5, 'skeptical': 2, 'replaces': 9, 'monterrey': 3, 'gate': 13, 'enrique': 4, 'barrios': 1, 'reynaldo': 1, 'ramos': 4, 'fernando': 7, 'larrazabal': 1, 'mayor': 69, 'nuevo': 10, 'leon': 2, 'collusion': 1, 'seventh': 38, 'nigerian': 82, 'abuja': 16, 'postponement': 9, 'indefinite': 5, 'nureddin': 1, 'mezni': 2, 'logistical': 19, 'infighting': 14, 'escalating': 20, 'jendayi': 2, 'frazer': 2, 'alertness': 1, 'kidwai': 1, 'khalid': 14, 'conceivable': 1, 'scenario': 5, 'timessays': 1, 'suggests': 13, 'khawaza': 2, 'kehla': 1, 'staving': 1, 'matter': 56, 'abandoned': 29, 'dam': 25, 'lodged': 3, 'memorandum': 8, 'bunji': 1, 'hydroelectric': 8, '227': 4, 'hailed': 19, 'dogharoun': 1, '123': 10, 'khatami': 39, 'desires': 2, 'studying': 15, 'salahaddin': 1, 'zafaraniyah': 1, 'reutersnews': 1, 'swapping': 1, 'visitors': 32, 'individuals': 30, 'clothes': 15, 'questioning': 38, 'swapped': 1, 'expires': 26, 'warring': 11, 'indefinitely': 10, 'organize': 12, 'driven': 53, 'planet': 26, 'nasa': 55, 'phoenix': 14, 'mars': 29, 'ideal': 5, 'polar': 15, 'subterranean': 1, 'ice': 45, 'martian': 7, 'dig': 9, 'layer': 2, 'glitch': 2, 'landing': 24, 'ranging': 19, 'negative': 14, 'headline': 1, 'vh1': 1, 'feature': 7, 'mumbai': 31, 'nikkei': 11, 'composite': 9, 'tumbled': 2, 'sensex': 1, 'lingering': 5, 'hang': 11, 'seng': 9, 'indexes': 11, 'frankfurt': 9, 'revitalize': 5, 'plunge': 5, 'rebates': 3, 'stave': 2, 'meets': 28, 'reserve': 47, 'deploying': 10, 'moshe': 6, 'yaalon': 3, 'breakthrough': 11, 'toppled': 21, 'mamadou': 8, 'returning': 58, 'oumarou': 4, 'posts': 26, 'tandja': 15, 'seyni': 2, 'hama': 1, 'amadou': 4, 'lamine': 1, 'mindaoudou': 1, 'aichatou': 1, 'keeping': 28, 'albade': 1, 'abouba': 1, 'mahamane': 1, 'zeine': 1, 'dalai': 36, 'lama': 40, 'buddhist': 34, 'spiritual': 25, 'admired': 1, 'teachings': 6, 'kuchma': 20, 'leonid': 15, 'yanukovich': 2, 'backer': 5, 'farce': 3, 'unforseeable': 1, 'inadmissible': 2, 'tim': 8, 'nardiello': 4, 'sexually': 19, 'skeleton': 6, 'arbitrator': 1, 'bobsled': 1, 'effective': 49, 'rejoin': 4, 'altenberg': 1, 'sliders': 2, 'publicly': 33, 'lund': 2, 'zach': 1, 'whistleblower': 1, 'revealing': 1, 'technician': 6, 'contacting': 5, 'hydrogen': 2, 'warheads': 12, 'neutron': 1, 'convert': 13, 'pointless': 1, 'stories': 10, 'cameraman': 12, 'victim': 54, 'outlet': 3, 'abductions': 16, 'implicitly': 1, 'beersheba': 1, 'critically': 13, 'larijani': 20, 'rush': 11, 'settlers': 54, 'mashaal': 10, 'unrealistic': 2, 'assassinations': 7, 'resuming': 18, 'inform': 12, 'self': 57, 'underestimated': 1, 'vacuum': 8, 'ball': 12, 'richards': 10, 'eye': 15, 'nawzad': 1, 'landmine': 15, 'gunbattle': 29, 'confiscated': 21, 'hide': 14, 'tachileik': 2, 'myanmar': 17, 'mekong': 5, 'drivers': 19, 'tents': 16, 'caffeine': 1, 'tablets': 1, 'blocks': 12, 'methamphetamine': 5, 'restive': 45, 'instill': 4, 'beheadings': 7, 'deliberate': 6, '756': 2, 'hurting': 11, 'fixed': 17, 'retirees': 3, 'golden': 8, 'retirement': 37, 'grapple': 1, 'mil': 19, 'arcega': 19, 'holiest': 9, 'najaf': 15, 'carnage': 2, 'risen': 49, '66': 12, 'ignite': 2, 'clearly': 6, 'wetlands': 7, 'degraded': 2, 'pandemic': 36, 'nairobi': 40, 'ponds': 1, 'habitats': 6, 'paddy': 4, 'fixes': 1, 'isolation': 23, 'suit': 18, 'slap': 3, 'dahoun': 1, 'gignor': 1, 'cheering': 7, 'demobilized': 6, 'evict': 5, 'forcefully': 3, 'tabarre': 1, 'suburb': 20, 'whatever': 8, 'ex': 30, 'rebellion': 23, 'identified': 118, 'almallah': 3, 'moutaz': 1, 'dabas': 3, '39': 25, 'spaniard': 9, 'slough': 1, 'warrant': 24, 'bow': 4, 'magistrate': 3, 'mohannad': 1, 'passport': 11, 'revitalizing': 2, 'jumpstart': 2, 'assure': 4, 'farfetched': 1, 'alleging': 17, 'stops': 17, 'michael': 67, 'montedison': 2, 'incorporated': 8, 'pharmaceuticals': 8, 'outstanding': 21, 'v': 17, 'tender': 4, 'erbamont': 2, 'advertised': 1, '72': 21, 'pursuant': 3, 'volta': 1, 'coups': 11, 'multiparty': 13, 'compaore': 2, 'blaise': 2, '1987': 17, 'durable': 5, 'securely': 1, 'density': 3, 'ghana': 32, 'unrest': 60, 'cote': 11, 'burkinabe': 1, "d'ivoire": 9, 'seasonal': 8, 'republics': 16, 'output': 54, 'waves': 34, 'wealthiest': 7, 'credit': 54, 'fortunes': 5, 'rebound': 8, 'kuna': 1, 'tame': 1, 'nevertheless': 14, 'stubbornly': 2, 'uneven': 5, 'retains': 8, 'privatization': 23, 'lag': 1, 'stabilization': 12, 'accelerate': 6, 'accession': 11, 'anemic': 4, 'mauritania': 29, 'thirds': 38, 'annexed': 19, 'contesting': 5, 'polisario': 11, 'guerrilla': 27, 'algeria': 24, 'genoese': 1, 'monaco': 7, '1215': 1, 'fortress': 1, '1331': 1, '1419': 1, '1297': 1, 'grimaldi': 1, 'casino': 7, 'railroad': 4, 'linkup': 1, 'spurred': 15, 'gambling': 7, 'scenery': 1, 'mild': 9, 'recreation': 2, 'forsaking': 1, 'crab': 3, 'seashore': 5, 'feeding': 7, 'meadow': 2, 'ate': 9, 'adapted': 1, 'deserve': 7, 'element': 6, 'happiness': 2, 'contentment': 1, 'currycombing': 1, 'rubbing': 1, 'oats': 1, 'stole': 11, 'groom': 2, 'wish': 9, 'alas': 2, 'walking': 9, 'here': 24, 'cried': 7, 'scoundrel': 2, 'answered': 7, 'makers': 10, 'mustard': 3, 'specifics': 4, 'pressed': 10, 'might': 85, 'product': 17, 'rochester': 1, 'ny': 1, 'thing': 12, 'yellow': 10, 'pitching': 2, 'unfortunately': 6, 'prayed': 10, 'fervently': 1, 'mother': 46, 'prayer': 22, 'styling': 1, 'excessively': 2, 'gel': 1, 'dragged': 6, 'independently': 14, 'thwart': 7, 'preparedness': 2, 'chidambaram': 3, 'alarmist': 1, 'westerners': 16, 'destination': 16, 'festivals': 2, 'advisories': 1, 'holidays': 11, 'commemoration': 6, 'kitts': 7, 'colonists': 3, '1632': 1, 'montserrat': 2, 'flee': 29, 'promise': 24, 'fulfilled': 5, 'froce': 1, 'mugabe': 42, 'zimbabwe': 91, 'zanu': 6, 'chinamasa': 4, 'chiweshe': 3, 'chairmanship': 9, 'pf': 6, 'draw': 39, 'mdc': 9, 'redistricting': 1, 'constituencies': 5, 'possession': 20, 'reflects': 9, 'inclusive': 3, 'consultation': 3, 'incorporating': 1, 'substantial': 35, '25th': 4, 'frenzy': 1, '1980': 18, 'lawmaking': 1, 'complied': 6, 'surprising': 2, 'champions': 8, 'qualifiers': 2, 'reigning': 6, 'praises': 5, 'horns': 7, 'blowing': 10, 'menas': 1, 'singing': 5, 'pharaohs': 3, 'stunning': 2, 'faithful': 13, 'converted': 7, 'landholdings': 1, 'ranks': 19, 'fifa': 29, '154th': 1, 'sun': 25, 'général': 1, 'niamey': 2, 'stade': 2, 'kountché': 1, 'kick': 8, 'blistering': 1, 'abel': 3, 'striker': 5, 'ouwa': 1, 'maazou': 3, 'getting': 47, 'moussa': 15, 'shafy': 1, 'defender': 3, 'scored': 55, 'goalkeeper': 1, 'essam': 2, 'hadary': 1, 'net': 34, 'bordeaux': 1, 'midfield': 1, 'rarely': 8, 'equalizer': 1, 'offside': 1, 'fathallah': 1, 'drops': 11, 'solid': 19, '01': 12, 'bottom': 6, 'continental': 10, 'johannesburg': 17, 'inauspicious': 1, 'volcano': 16, 'soufriere': 1, 'eruption': 9, 'hosts': 14, 'qualify': 5, 'gabon': 17, 'pushes': 3, 'hometown': 13, 'governing': 34, 'intensified': 24, 'inconclusive': 4, 'hormone': 3, 'importing': 7, 'fines': 15, 'stallone': 2, 'sylvester': 1, 'room': 23, 'movie': 35, 'possessing': 8, 'enhancing': 7, 'occurring': 6, 'endured': 11, 'fine': 24, 'penalty': 32, 'unlikely': 17, 'collided': 9, 'tractor': 7, 'maharashtra': 9, 'bombay': 9, 'nagpur': 2, 'tracks': 8, 'trains': 20, 'yadav': 3, 'laloo': 1, 'punjab': 10, 'collision': 10, 'transmissions': 3, 'histories': 2, 'pages': 8, 'oral': 2, 'robust': 12, 'twin': 15, 'towers': 5, 'firefighters': 17, 'sued': 9, 'privacy': 7, 'jeopardize': 5, 'zacarias': 3, 'moussaoui': 4, 'waste': 19, 'shores': 1, 'hazardous': 8, 'containers': 10, 'sickness': 1, 'hemorrhages': 1, 'ulcers': 2, 'radiation': 2, 'illnesses': 6, 'unusual': 10, 'skin': 7, 'abdominal': 5, 'dumping': 7, 'toxic': 23, 'accelerated': 4, 'dislodged': 1, 'switzerland': 48, 'lend': 5, 'taro': 8, 'aso': 15, 'davos': 4, 'rebounded': 16, 'announce': 33, 'stormy': 4, 'jabella': 1, 'beverages': 3, 'hazelnuts': 1, 'nonalcoholic': 1, 'machinery': 13, 'grapes': 3, 'copper': 14, 'manganese': 3, 'fruits': 7, 'alcoholic': 2, 'citrus': 6, 'lengthy': 12, 'anything': 13, 'consulate': 32, 'jeddah': 17, 'merciful': 1, 'availability': 4, 'external': 42, 'banking': 53, 'risks': 19, 'improvement': 14, 'spokesperson': 6, 'convoys': 13, 'operational': 16, 'wrapping': 8, 'looks': 25, 'feeds': 2, 'connecticut': 9, 'beer': 6, 'spree': 5, 'disgruntled': 3, 'distributors': 5, 'hartford': 2, 'warehouse': 9, 'equip': 2, 'welcoming': 3, 'extension': 28, 'component': 3, 'repatriating': 2, 'havens': 11, 'restrict': 15, 'interruptions': 3, 'relying': 5, 'overcome': 20, 'renovating': 2, 'hoop': 17, 'scheffer': 19, 'jaap': 14, 'sense': 17, 'clamp': 4, 'holes': 2, 'digging': 8, 'meter': 43, 'solo': 2, 'safeguard': 9, 'islamiyah': 9, 'teenager': 25, 'seventeen': 4, 'matoo': 1, 'tufail': 1, 'kars': 1, 'erzerum': 1, "t'bilisi": 1, 'capitalize': 2, 'baku': 8, 'ceyhan': 3, 'akhalkalaki': 2, 'teargas': 6, 'mattoo': 1, 'shell': 43, 'bag': 13, '47': 23, 'entirety': 2, 'celebrating': 17, 'christians': 52, 'apprehension': 1, 'petty': 4, 'simplified': 1, 'cracked': 5, 'enforcement': 29, 'collect': 9, 'invincible': 1, 'anxious': 5, 'feels': 8, 'volunteer': 6, 'neediest': 1, 'fellow': 29, 'queen': 12, 'gratitude': 2, 'pride': 9, 'elizabeth': 7, 'highlighted': 8, 'diversity': 4, 'tolerance': 11, 'ordinary': 8, 'orthodox': 30, 'deeds': 3, 'patriarch': 8, 'alexy': 2, 'pool': 13, 'joy': 7, 'cathedral': 7, 'yakutsk': 2, 'siberian': 4, 'eroded': 5, 'surplus': 18, 'borrowing': 9, 'jesus': 25, 'kings': 4, 'benedict': 70, 'epiphany': 2, 'wise': 5, 'feast': 4, 'isi': 1, 'melbourne': 21, 'slam': 9, 'rafael': 18, 'nadal': 5, 'pinning': 1, 'domestically': 7, 'attract': 16, 'regulation': 3, 'fitness': 3, 'joins': 8, 'sharapova': 8, 'andre': 9, 'agassi': 14, 'russians': 10, 'maria': 10, 'seed': 28, 'ankle': 5, 'federer': 14, 'infiltration': 4, 'method': 7, 'burns': 19, 'nicholas': 13, 'infiltrating': 3, 'igniting': 1, 'madagascar': 10, 'discarding': 1, 'liberalization': 5, 'prepared': 47, 'concessions': 12, 'expense': 12, 'subsidies': 38, 'compromises': 1, 'iacovou': 2, 'text': 11, 'ambassadors': 15, 'abandon': 36, 'gul': 18, 'tries': 4, 'imposes': 4, 'normalize': 4, 'wounds': 23, 'mainstay': 8, 'employing': 6, 'sadrist': 2, 'vocal': 8, 'responsibilities': 10, 'transferring': 10, 'toe': 3, 'sore': 1, 'basketball': 16, 'yao': 9, 'ming': 3, 'nba': 10, 'houston': 19, 'boomed': 2, '266': 2, 'miss': 7, 'getter': 1, 'averaging': 6, 'rebounds': 3, 'shots': 26, 'expulsion': 11, '114': 9, 'termination': 5, 'requirements': 19, 'agoa': 2, 'financing': 16, 'pakistanis': 32, 'apartments': 7, 'belgian': 15, 'blow': 38, 'bangkok': 19, 'crippling': 8, 'dissolve': 9, 'abhisit': 3, 'vejjajiva': 1, 'somchai': 1, 'predecessor': 18, 'thaksin': 30, 'wongsawat': 1, 'dispersing': 3, 'shirted': 1, 'wracked': 5, 'aggravated': 4, 'deforestation': 3, 'firewood': 2, 'erosion': 4, 'weah': 23, 'monrovia': 5, 'butty': 1, 'veritas': 1, 'talked': 8, 'sainworla': 1, 'affiliate': 4, 'fix': 11, 'telescope': 10, 'hubble': 13, 'camera': 7, 'galaxies': 8, 'photo': 11, 'ring': 27, 'beaming': 1, 'repair': 20, 'ravalomanana': 7, 'aggressively': 8, 'universe': 4, 'images': 40, 'revolutionized': 1, 'aqsa': 41, 'martyrs': 35, 'persuaded': 2, '30th': 12, 'hakim': 11, 'dealt': 8, 'blows': 7, 'infidels': 3, 'endorses': 3, 'authenticated': 4, 'richardson': 5, 'endorse': 5, 'portland': 3, 'oregon': 6, 'hispanic': 5, 'tended': 1, 'hispanics': 4, 'caucuses': 4, 'delegates': 42, 'selected': 24, 'wary': 5, 'uncertain': 13, 'entering': 35, 'celebrates': 9, 'pack': 4, 'lent': 5, 'carnival': 10, 'repentance': 1, 'revelers': 4, 'dance': 12, 'samba': 4, 'parades': 10, 'sensual': 1, 'featuring': 8, 'sambadrome': 3, 'culminates': 1, 'costumes': 4, 'masks': 5, 'brazilians': 6, 'drinking': 29, 'outlandish': 2, 'squeeze': 3, 'fasting': 8, 'indulgence': 1, 'celebrations': 38, 'hanging': 14, 'beit': 12, 'considers': 35, '1853': 1, 'collaborating': 3, 'row': 14, 'excerpts': 6, 'aired': 36, 'filmed': 5, 'style': 27, 'signaled': 6, 'anytime': 2, 'faltering': 6, 'positioned': 6, 'steadily': 17, 'fed': 26, 'served': 90, '1864': 1, 'penal': 2, 'balance': 27, 'slash': 7, 'hikes': 13, 'siege': 15, 'beslan': 13, 'ingushetia': 5, 'seizing': 11, 'chaotic': 7, '330': 10, 'ordeal': 1, 'teleconference': 1, 'noumea': 1, 'caledonia': 5, 'bagram': 14, 'spoken': 7, 'filibuster': 2, 'showdown': 4, 'tactic': 5, 'frist': 6, 'priscilla': 2, 'owen': 3, 'filibusters': 4, 'changing': 12, 'drastically': 5, '2014': 10, 'commits': 3, '2019': 2, 'accordance': 12, 'asad': 4, 'hashimi': 3, 'boycotting': 8, 'arranging': 6, 'jetliners': 4, 'airbus': 17, 'surprised': 12, 'regain': 11, 'mankind': 2, 'ills': 5, 'prevailed': 2, '1055': 1, '1002': 1, 'somewhat': 11, 'cope': 17, 'fleets': 5, 'khursheed': 6, 'hatred': 12, 'vengeance': 3, 'righteous': 2, 'persecutors': 1, 'heaven': 2, 'wafted': 1, 'youth': 26, 'sports': 41, 'bikindi': 3, 'tanzania': 27, 'hutus': 7, 'composed': 3, 'encouraged': 31, 'tutsis': 16, 'habyarimana': 2, 'privately': 19, 'lyrics': 3, 'tutsi': 9, 'juvenal': 2, 'consulted': 2, 'stationing': 1, 'youssef': 9, 'tariq': 7, 'baathist': 3, 'sympathizers': 7, 'warfare': 12, 'entreated': 5, 'unceasing': 1, 'indissoluble': 1, 'jupiter': 9, 'proof': 18, 'soured': 3, 'defusing': 3, 'attorney': 65, 'spies': 13, 'vicente': 32, 'rangel': 15, 'attaché': 2, 'correa': 19, 'mentioned': 21, 'documentary': 10, 'outrage': 17, 'filmmaker': 11, 'habitations': 1, 'henceforth': 2, 'decreed': 2, 'footage': 19, 'archival': 1, 'illustrate': 2, 'edelist': 1, 'incorrect': 6, 'transcript': 1, 'copy': 5, 'film': 61, 'eliezer': 2, 'baseball': 23, 'permission': 34, 'ueberroth': 2, 'treasury': 46, 'inaugural': 5, 'classic': 10, 'hence': 3, 'arises': 2, 'abound': 1, 'singly': 1, 'discern': 1, 'rico': 5, 'puerto': 12, 'panama': 34, 'transactions': 10, 'zaraqawi': 1, 'yasin': 2, 'malik': 8, 'sanjaya': 1, 'adviser': 18, 'baru': 1, 'tv': 37, 'invitation': 15, 'convened': 8, 'channel': 36, 'geo': 4, 'warlords': 25, 'paving': 6, 'locked': 16, 'feel': 8, 'unsafe': 5, 'unions': 33, 'sirnak': 4, 'averted': 3, 'stoppages': 1, 'attendants': 2, 'alitalia': 1, 'walkout': 6, 'telecommunications': 32, 'slalom': 11, 'bormio': 1, 'bigger': 9, 'grab': 9, 'growers': 4, 'orchid': 1, 'varieties': 2, 'unique': 14, 'wagner': 2, 'valle': 2, 'alvaro': 33, 'farc': 64, 'cauca': 1, 'uribe': 46, 'swap': 10, 'paramilitaries': 18, 'rightist': 16, 'rewrite': 4, '78': 22, 'cedatos': 1, 'inefficiency': 1, 'gallup': 4, 'centralize': 1, 'stripping': 2, 'unpopular': 9, 'redefine': 2, 'shelled': 5, 'reaching': 34, 'unveiled': 29, 'epidemic': 16, 'anticipates': 3, 'gatherings': 7, 'stockpiles': 6, 'viral': 6, 'tamiflu': 8, 'beachfront': 4, 'minibus': 16, 'pkk': 65, 'jenin': 13, 'viewers': 11, 'marti': 3, 'article': 28, 'granma': 10, 'wpmf': 1, 'dishes': 4, 'nepali': 7, '680': 1, 'ilam': 2, 'persuading': 7, 'insult': 7, 'outbursts': 1, 'revolt': 15, 'pushing': 18, 'bajaur': 10, 'sam': 14, 'intense': 33, 'loi': 1, 'khata': 1, 'rashakai': 1, 'tang': 2, 'reserved': 6, 'rehman': 5, 'offensives': 8, 'discouraging': 5, 'undertaking': 2, 'militancy': 8, 'beating': 34, 'assaulting': 7, 'punching': 4, 'drunkenness': 2, 'uniformed': 5, 'pictures': 35, 'credentials': 11, 'manhandling': 1, 'handcuffs': 4, 'beaten': 19, 'looting': 9, 'deserting': 1, 'respects': 13, 'arrives': 11, 'universities': 12, 'offend': 3, 'ekmeleddin': 4, 'ihsanoglu': 4, 'finnish': 7, 'kaliningrad': 2, 'denying': 31, 'interfax': 19, 'vanhanen': 1, 'matti': 1, 'reward': 22, 'harcourt': 10, 'paraded': 1, 'mend': 7, 'refuted': 2, 'emancipation': 11, 'delta': 62, 'hoshyar': 9, 'zebari': 14, 'maysan': 1, 'beckett': 6, 'margaret': 17, 'muthana': 1, 'redeployed': 1, 'mean': 21, 'covert': 9, 'reid': 22, 'lewis': 8, 'libby': 16, 'leak': 6, 'probing': 12, 'rove': 4, 'karl': 7, 'economist': 16, 'konan': 3, 'banny': 11, 'mediators': 21, 'dakar': 9, 'abijan': 1, 'senegal': 41, 'archipelago': 15, 'andaman': 6, 'wandering': 1, 'teenagers': 13, 'flooded': 29, 'hill': 34, 'tribespeople': 2, 'nicobari': 1, 'lived': 41, 'coconuts': 6, 'emaciated': 1, 'alizai': 1, 'esmatullah': 1, 'mian': 3, 'neshin': 1, 'tactical': 3, 'recapture': 4, 'chora': 1, 'casualty': 12, 'welle': 2, 'baghlan': 6, 'deutsche': 2, 'explosive': 35, 'musayyib': 2, 'spiraling': 4, 'topics': 16, 'absolutely': 4, 'unproven': 1, 'complexes': 1, 'alonso': 10, 'desalinization': 1, 'urtuzaga': 1, 'esquisabel': 1, 'pedro': 6, 'toulouse': 1, 'mushir': 1, 'masri': 16, 'atrocious': 2, 'steinmeier': 10, 'senseless': 1, 'successor': 31, 'fatally': 9, 'honduran': 9, 'tegucigalpa': 3, 'markey': 3, 'timothy': 7, 'leg': 28, 'pronounced': 2, 'fatal': 23, 'robbery': 10, 'dea': 1, 'mcclellan': 25, 'scott': 33, 'idol': 8, 'hopefuls': 5, 'implicating': 4, 'tueni': 5, 'gibran': 1, 'mourned': 2, 'publisher': 7, 'legislator': 11, 'coincide': 13, 'nick': 6, 'nice': 5, 'charisma': 1, 'sang': 8, 'cowell': 6, 'axe': 8, 'chicks': 1, 'rendition': 2, 'dixie': 1, 'tepid': 1, 'alaina': 1, 'wto': 25, 'bids': 9, 'goody': 1, 'payable': 1, 'kearny': 2, 'accessories': 3, 'cosmetic': 3, '92': 11, 'takeover': 17, '1830': 5, 'technologically': 3, 'prospered': 6, 'flemings': 1, 'walloons': 1, 'irrigated': 2, 'intensive': 11, 'turkmenistan': 12, 'oases': 1, 'feeling': 11, 'leslie': 6, 'nina': 2, 'simone': 2, 'tabaldo': 1, 'consumed': 3, 'cotton': 13, 'employ': 8, 'workforce': 12, 'cautious': 6, 'sustain': 15, 'tribally': 1, 'ashgabat': 1, 'educational': 8, 'endemic': 6, 'statistics': 15, 'margins': 2, 'sensation': 1, 'antonella': 3, 'racy': 1, 'barba': 2, 'particular': 21, 'gasoline': 63, 'dual': 11, 'unified': 15, 'berdimuhamedow': 2, 'caspian': 6, 'redenomination': 1, 'manat': 1, 'impede': 4, 'bureaucratic': 5, 'hungary': 24, '1000': 9, 'd': 31, 'bulwark': 1, 'polyglot': 2, 'warsaw': 19, '1956': 7, 'janos': 1, 'kadar': 1, 'liberalizing': 3, 'goulash': 1, 'introducing': 4, 'confinement': 8, 'rotating': 14, 'marsh': 1, 'physician': 10, 'beasts': 9, 'frog': 2, 'heal': 4, 'gait': 1, 'pretend': 1, 'wrinkled': 2, 'prescribe': 4, 'lame': 2, 'swallow': 7, 'reared': 3, 'chink': 1, 'snake': 2, 'eat': 17, 'injunction': 8, 'filberts': 2, 'pitcher': 7, 'grasped': 2, 'disappointment': 6, 'bitterly': 4, 'lamented': 4, 'unwilling': 3, 'readily': 2, 'bystander': 6, 'quantity': 6, 'unspecified': 12, 'administered': 18, 'fatas': 1, 'federally': 3, 'livelihoods': 6, 'pashtun': 3, 'governed': 6, 'elders': 22, 'ravine': 5, 'malakand': 2, 'disregard': 3, 'poorly': 15, 'medvedev': 31, 'ratification': 12, 'dmitri': 16, 'prague': 7, 'submitted': 29, 'synchronize': 1, 'arsenals': 5, 'milestone': 8, 'skirmish': 4, 'nadhem': 1, '337': 5, 'cricket': 30, 'stumps': 5, 'nld': 12, 'landslide': 24, 'matthew': 5, 'hayden': 11, 'ganguly': 5, '28th': 2, '124': 3, 'sourav': 3, 'bradman': 1, 'surpassing': 3, 'countrymen': 3, 'ponting': 1, 'ricky': 1, 'anil': 5, '84': 19, 'kumble': 6, 'damper': 2, 'bowlers': 6, 'zaheer': 1, 'ejected': 4, 'grounds': 25, 'tips': 6, 'interviews': 19, 'entice': 2, 'prisons': 46, 'berri': 5, 'nabih': 5, 'odds': 13, 'amr': 8, 'simple': 15, 'outlining': 8, 'swift': 9, 'hanged': 7, 'juma': 3, 'describing': 10, 'trees': 22, 'himat': 2, 'mutilated': 5, 'agha': 6, 'saqeb': 1, 'sayed': 6, 'ahmadi': 7, 'cordons': 1, 'section': 31, 'segment': 3, 'rock': 40, 'fencing': 6, 'barbed': 1, 'tunceli': 6, 'outlawed': 24, 'roadblock': 6, 'unilateral': 17, 'ignoring': 8, 'complaining': 6, 'pains': 5, 'calories': 1, 'requirement': 4, '050': 1, 'lagging': 4, 'cheerful': 2, 'discomfort': 3, 'experiencing': 11, 'exhaustion': 4, 'spends': 4, 'ghanaian': 4, 'accra': 6, 'entirely': 13, 'argue': 16, 'consolidation': 5, 'gradual': 8, 'conceived': 4, 'kwame': 2, 'nkrumah': 2, 'fresh': 41, 'pacifism': 1, 'rating': 19, 'slashed': 10, 'ratings': 11, 'athens': 15, 'speculative': 1, 'bonds': 12, 'notches': 1, 'downgraded': 7, 'sovereign': 20, 'debts': 8, 'lenders': 9, 'stocks': 24, 'downgrades': 1, 'favorable': 11, 'quan': 1, 'investigative': 12, 'communists': 6, 'coverage': 24, 'caller': 1, 'occupy': 4, 'contained': 26, 'arraignment': 1, 'lynndie': 5, 'georgy': 1, 'gongadze': 2, 'maltreat': 1, 'maltreatment': 2, 'presiding': 9, 'mistrial': 2, 'mistreating': 4, 'tunisia': 10, 'bias': 5, 'dominique': 17, 'villepin': 19, 'reactors': 23, 'escalate': 5, 'decapitated': 4, 'dennis': 6, 'hastert': 1, 'inspiring': 3, 'contest': 13, 'captain': 31, 'bowman': 2, 'shifting': 8, 'pockets': 7, 'entrenched': 5, 'centraql': 1, 'bodyguard': 17, 'conversations': 10, 'bahraini': 2, 'likelihood': 4, 'emirate': 3, 'aiyar': 2, 'mani': 1, 'amanullah': 4, 'jadoon': 1, 'shankar': 1, 'pressured': 11, '57th': 1, '588': 1, 'releases': 9, 'differs': 4, 'khin': 17, 'accusations': 53, 'nyunt': 11, 'dissidents': 31, 'threatens': 22, 'defenders': 3, 'kravchenko': 1, 'intimidation': 8, 'drc': 30, 'afraid': 8, 'legitimate': 16, 'coastline': 10, 'generated': 15, 'sulawesi': 4, 'tomini': 1, 'gorontalo': 1, 'unfounded': 7, 'pangandaran': 2, 'barreled': 1, 'crushing': 7, '668': 1, 'volunteered': 3, 'skip': 3, 'reunite': 8, 'lady': 17, 'laura': 8, 'skipped': 2, 'meal': 5, 'rainfall': 11, 'depletion': 5, 'rainy': 5, 'herds': 2, 'livestock': 25, 'kenyans': 17, 'closest': 9, 'karen': 19, 'reputation': 9, 'hughes': 8, 'nominate': 7, 'requires': 35, 'advisors': 13, 'tutwiler': 1, 'exploited': 3, 'greeted': 13, 'villagers': 55, 'rangers': 1, '5000': 2, 'wildfires': 6, 'canary': 11, 'scorched': 2, 'tenerife': 4, 'gran': 6, 'stabilized': 5, 'fires': 32, 'moderated': 3, 'canaria': 3, 'inspected': 5, 'forests': 5, 'incinerated': 1, 'resorts': 11, 'blazes': 7, 'coastal': 45, 'caregivers': 1, 'environmentalists': 5, 'arsonists': 3, 'wildfire': 4, 'confessed': 15, 'havel': 7, 'vaclav': 3, 'autocracy': 4, 'desmond': 7, 'mary': 9, 'archbishop': 20, 'robinson': 2, 'soros': 3, 'tutu': 7, 'authors': 3, 'retired': 48, 'fronta': 1, 'mlada': 1, 'dnes': 1, 'subsided': 4, '1997': 52, 'chart': 4, 'speculation': 34, 'tube': 6, 'nasal': 2, 'throat': 8, 'intake': 1, 'works': 43, 'aides': 28, 'armchair': 1, 'overlooking': 3, 'bless': 2, 'cheers': 3, 'applause': 4, 'microphone': 4, 'discharged': 8, 'tracheotomy': 1, 'bethelehem': 1, 'manger': 7, 'nativity': 5, 'infiltrate': 3, 'mardan': 1, 'registry': 5, 'considerations': 1, 'tadic': 13, 'boris': 13, 'albanian': 20, 'supervised': 4, 'backs': 8, 'hinted': 2, 'supervision': 8, 'shrine': 32, 'cancel': 18, 'timely': 2, 'yasukuni': 4, 'atlanta': 19, 'audience': 20, 'referring': 21, 'parlimentary': 1, 'register': 20, 'canceling': 2, 'appoint': 20, 'liners': 4, 'flare': 7, 'why': 46, 'elaborate': 24, 'democrat': 23, 'duluiyah': 1, 'triangle': 6, 'extinguished': 1, 'saboteurs': 2, 'guardsman': 1, 'gerry': 3, 'mccann': 4, 'kate': 4, 'siblings': 7, 'blessed': 2, 'photograph': 12, 'emotions': 2, 'devout': 2, 'publicize': 2, 'disappearance': 9, 'outcry': 6, 'celebrities': 4, 'duties': 33, 'hrw': 6, 'condemns': 15, 'lists': 14, 'absurd': 3, 'contends': 9, 'revised': 17, 'bench': 3, 'declaring': 10, 'sawers': 1, 'ditch': 2, 'vaeedi': 2, 'javad': 3, 'consensus': 16, 'unexpectedly': 5, 'strongest': 14, 'jobless': 9, 'weak': 29, 'stimulus': 24, '787': 2, 'secessionist': 9, 'cultural': 22, 'haqqani': 3, 'facilitator': 2, 'paktiya': 1, 'lien': 1, 'honorary': 3, 'chan': 8, 'mainland': 42, 'greenspan': 21, 'guiding': 2, 'retire': 8, 'regarded': 4, 'speculate': 5, 'occupants': 4, 'bullhorn': 1, 'ak': 7, 'feldstein': 1, 'hubbard': 1, 'academic': 8, 'glenn': 2, 'anderson': 20, 'danilo': 6, 'charred': 5, 'positively': 3, 'prosecuting': 4, 'ibero': 4, '119': 10, 'jump': 14, 'seem': 7, 'rises': 6, 'assessed': 5, '2020': 12, 'sufficient': 16, 'egyptians': 16, 'resulting': 22, 'fined': 13, 'regulators': 21, 'antitrust': 2, '357': 1, 'neelie': 1, 'kroes': 1, 'double': 60, '633': 2, 'smoothly': 4, 'technical': 30, 'unjustified': 5, 'icy': 12, 'shutting': 7, 'snarling': 1, 'domodedovo': 2, 'sheremetyevo': 1, 'snapped': 4, 'encased': 1, 'slicked': 1, 'ria': 2, 'novosti': 3, 'taxis': 1, 'charging': 14, 'sex': 34, 'guess': 2, 'physical': 24, 'appearance': 34, '42': 28, 'condom': 3, 'karachi': 51, 'stolen': 18, 'espionage': 12, 'goodwill': 13, 'identities': 14, 'bears': 13, 'originate': 1, 'species': 22, 'ken': 4, 'endangered': 13, 'applying': 8, 'habitat': 6, 'salazar': 3, 'application': 9, 'farther': 9, 'arctic': 15, 'mechanism': 7, 'millimeter': 2, 'bear': 15, 'warms': 1, 'atmosphere': 23, 'melting': 9, 'touchstone': 1, 'balochistan': 2, 'bugti': 5, 'raziq': 1, 'quetta': 24, 'wiped': 13, 'profits': 29, 'unfair': 17, 'graft': 9, 'entrance': 18, 'homemade': 16, '146': 2, 'perceived': 10, 'outdated': 6, 'faulty': 4, 'ignores': 2, 'focuses': 8, 'payments': 44, 'bribes': 15, 'angola': 34, 'alasay': 1, 'patrols': 20, 'spindleruv': 1, 'mlyn': 1, 'kostelic': 11, 'janica': 3, 'zettel': 1, '08': 8, 'kathrin': 1, 'marlies': 1, 'schild': 1, 'circuit': 9, 'mill': 7, 'steel': 18, 'kryvorizhstal': 2, 'consortium': 9, 'reviewed': 7, 'tymoshenko': 31, 'yulia': 12, 'fairly': 11, 'privatizations': 5, 'quit': 43, 'zvarych': 1, 'canal': 21, 'schemes': 4, 'shan': 19, 'mae': 1, 'wa': 9, 'spilling': 3, 'manufacture': 10, 'frontier': 23, 'cheema': 4, 'jirga': 4, 'javed': 5, 'aborted': 1, 'abduct': 1, 'tribesmen': 21, 'freeing': 10, 'passage': 22, 'sanctuaries': 3, 'swine': 25, 'woefully': 2, 'susceptible': 1, 'everyone': 20, 'h1n1': 8, 'affluence': 1, 'wealthy': 19, 'biased': 12, 'medicines': 8, 'gets': 10, 'providers': 2, 'inoculated': 1, 'atheist': 1, '429': 2, 'confirms': 11, 'absentee': 3, 'provisional': 20, 'registered': 32, 'precinct': 3, 'christine': 3, 'gregoire': 1, 'gubernatorial': 3, 'ruin': 3, 'barreling': 1, 'mayhem': 1, 'stretches': 3, 'minnesota': 9, 'explicitly': 3, 'locales': 2, 'predicting': 6, 'discourages': 1, 'outages': 6, 'airline': 29, 'servicing': 10, 'reagan': 7, 'bloomberg': 19, 'indoors': 2, 'sidewalks': 1, 'guidance': 8, 'otherwise': 7, 'trades': 2, 'productivity': 12, 'wage': 22, 'minimum': 13, 'hike': 18, 'pensions': 4, 'steep': 13, 'utility': 6, 'users': 19, 'kilowatt': 1, 'heaviest': 8, 'conservation': 12, 'rasul': 1, 'khurmatu': 3, 'aadham': 1, 'laborers': 15, '180': 15, 'ramadi': 42, 'anbar': 50, 'max': 5, 'montana': 1, 'baucus': 1, 'basis': 37, 'exemption': 1, 'harassment': 10, 'rebuked': 1, 'bastion': 3, 'encouraging': 16, 'infringement': 1, 'arizona': 25, 'concealing': 2, 'cracks': 4, 'sanctioned': 4, 'conscripted': 2, 'residency': 4, 'disclose': 10, 'slaughter': 15, 'arteries': 3, 'bypass': 4, 'recovers': 4, 'pranab': 4, 'chaired': 8, 'mukherjee': 6, 'collective': 4, 'tripled': 3, 'morris': 9, 'greatly': 12, 'deteriorated': 10, 'severely': 22, 'flowing': 14, 'starving': 1, 'nahr': 9, 'tripoli': 13, 'bared': 10, 'surveyed': 19, 'disapprove': 7, 'flags': 17, 'bombarded': 4, 'firefights': 3, 'sweet': 1, 'disruption': 7, 'minsk': 16, 'soaring': 28, 'skepticism': 4, 'hailing': 8, 'sheikh': 46, 'announcements': 3, 'shallah': 2, 'brotherhood': 41, 'intensifying': 4, 'rejection': 9, 'politics': 40, 'barring': 4, 'independents': 6, 'chip': 4, 'bargaining': 4, 'mhawesh': 1, 'gilad': 2, 'dichter': 1, 'qadi': 1, 'shalit': 3, 'avi': 1, 'dressed': 19, 'commandos': 13, 'randomly': 2, 'akhtar': 10, 'haji': 1, 'naseem': 1, 'salerno': 2, 'retaliated': 11, 'freeskate': 1, 'pairs': 3, 'duel': 2, 'highlights': 6, 'totmianina': 3, 'maxim': 2, 'tatiana': 6, 'marinin': 3, 'hao': 1, 'zhang': 9, 'grandmother': 5, 'sarah': 7, 'thieves': 8, 'grabs': 4, 'combines': 7, 'poiree': 3, 'grete': 1, 'liv': 1, 'snowboarding': 2, 'kelly': 2, 'pipe': 4, 'skating': 10, 'arson': 8, 'suburbs': 13, 'rioters': 16, 'racial': 9, 'treats': 5, 'kogelo': 1, 'hid': 12, 'electrocuted': 5, 'americas': 12, 'plata': 7, 'opens': 9, 'democratically': 14, 'honduras': 22, 'delegations': 6, 'encompass': 2, 'nestor': 8, 'kirchner': 12, 'pirates': 84, 'churchill': 1, 'destroyer': 5, 'winston': 1, 'uss': 6, 'piracy': 22, 'kitchen': 6, 'door': 15, 'maneuvering': 1, 'sixth': 39, 'compete': 29, 'usa': 11, 'maroney': 1, 'sean': 24, 'featured': 6, 'mohamad': 7, 'miracle': 3, 'resulted': 55, 'azahari': 8, 'husin': 7, 'tapes': 1, 'rented': 3, "l'equipe": 3, 'valentino': 1, '676': 2, 'rossi': 2, '387': 2, 'formula': 7, 'kenenisa': 1, 'distance': 15, 'bekele': 1, 'compiling': 2, '81': 19, 'wimbledon': 12, '965': 1, 'bridge': 26, '450': 12, 'rumor': 1, 'shoved': 2, 'tigris': 1, 'unverifiable': 4, 'wildly': 1, 'clad': 5, 'eleven': 9, 'lashkar': 23, 'jhangvi': 5, 'multan': 5, 'yemeni': 21, 'abyan': 5, 'secessionists': 1, 'placing': 14, 'asset': 8, 'zimbabwean': 9, 'lavish': 6, 'throw': 9, 'poisoning': 10, '111': 5, 'concentrations': 1, 'zamfara': 1, 'epidemiologists': 1, 'pediatricians': 2, 'kidneys': 1, 'reproductive': 1, 'concentration': 6, 'chinhoyi': 1, 'harare': 10, 'herald': 22, '85th': 2, 'tigers': 13, 'mullaittivu': 2, 'disinfectant': 1, 'sprayed': 4, 'erected': 3, 'poles': 5, 'correspondent': 24, '1500': 3, 'sheltered': 3, 'rangin': 3, 'dadfar': 4, 'spanta': 5, 'komuri': 3, 'masahiko': 1, 'komura': 1, 'morgan': 4, 'tsvangirai': 3, 'sheltering': 5, 'mehmood': 3, 'qureshi': 4, 'gilani': 18, 'yousuf': 19, 'raza': 16, 'syed': 10, 'geelani': 1, 'hurriyat': 9, 'penitentiary': 6, 'upheaval': 2, 'quashed': 1, 'saga': 1, 'massively': 1, 'chaos': 29, 'airing': 6, 'chairs': 11, 'publicist': 8, 'duncan': 2, 'truth': 13, 'unvarnished': 1, 'snuff': 1, 'denounces': 2, 'mailing': 1, 'integra': 3, 'entitles': 1, 'exercised': 3, 'nov': 9, 'hammer': 3, 'hallwood': 2, 'operates': 13, 'merchant': 6, 'cleveland': 15, 'integration': 27, 'mere': 4, 'advantages': 3, 'eliminated': 17, 'downsized': 1, 'lagged': 3, 'overstated': 1, 'lowered': 8, 'sound': 28, 'fuad': 7, 'masoum': 2, 'pegged': 4, 'euro': 53, 'prudent': 7, 'modest': 10, 'timor': 11, 'leste': 3, 'westward': 5, 'supplemented': 1, 'piped': 2, 'convenes': 9, 'preserve': 12, 'repository': 2, 'idps': 1, 'resettled': 6, 'markedly': 3, 'procurement': 4, 'underlying': 5, '1809': 1, 'duchy': 2, 'invasions': 5, 'albeit': 1, 'finns': 1, 'remarkable': 4, 'diversified': 8, 'initiation': 1, 'fluctuations': 3, 'challenged': 20, 'equality': 19, 'clipperton': 1, 'tuna': 5, 'desired': 3, 'swan': 13, 'plumage': 2, 'raven': 3, 'altars': 1, 'supposing': 3, 'washing': 3, 'pools': 2, 'color': 7, 'lakes': 6, 'swam': 5, 'arose': 5, 'feathers': 8, 'cleansing': 4, 'perished': 4, 'habit': 2, 'alter': 5, 'nettle': 2, 'hurts': 4, 'gently': 1, 'touched': 9, 'silk': 3, 'boldly': 2, 'grasp': 1, 'soft': 11, 'dust': 6, 'philosophy': 2, 'objectives': 9, 'mathematics': 1, 'kids': 11, 'pharaoh': 1, 'bible': 3, 'financier': 3, 'nile': 4, 'helium': 1, 'stationary': 3, 'pencils': 1, 'hiking': 2, 'trailing': 3, 'elevators': 1, 'escalators': 1, 'switches': 1, 'diapers': 1, 'keel': 1, 'balloon': 4, 'batteries': 5, 'recharge': 1, 'draining': 2, 'fifty': 7, 'ninety': 2, 'malnutrition': 5, 'ethiopians': 12, 'eliminate': 18, 'sanitation': 14, 'malnourished': 1, 'norad': 2, 'maneuvers': 10, 'aerospace': 2, 'alaska': 8, 'khabarovsk': 7, 'elmendorf': 1, 'drik': 1, 'divert': 5, 'extorting': 2, 'ransoms': 2, 'trainers': 2, 'peterson': 3, 'squads': 9, 'circulated': 6, 'rallied': 27, 'shouting': 11, 'denounce': 8, 'watched': 21, 'outcome': 14, 'gassing': 1, 'glyn': 1, 'davies': 2, 'frame': 4, 'drafted': 9, 'kilograms': 18, 'boroujerdi': 4, 'alaeddin': 1, 'parliamentarian': 5, 'substantially': 18, 'debating': 5, 'binding': 12, 'securing': 10, 'benchmarks': 6, 'desk': 4, 'override': 1, 'adam': 17, 'udi': 1, 'votel': 1, 'paulson': 6, 'lending': 10, 'henry': 5, 'distress': 5, 'reemergence': 1, 'loans': 37, 'creditors': 7, 'forgiven': 4, 'derailment': 2, 'mangled': 2, 'griffal': 2, 'luge': 4, 'quinn': 2, 'joye': 2, 'preston': 3, 'doubles': 15, 'niccum': 3, 'berth': 1, 'placid': 1, 'grimmette': 1, 'martinin': 1, 'medalists': 2, 'singles': 15, '161': 2, 'mazdzer': 1, 'compatriot': 7, 'myles': 2, 'jonathan': 13, 'benshoofin': 1, 'retrosi': 1, 'erin': 2, 'zablocki': 1, 'samantha': 3, 'hamlin': 1, 'courtney': 2, 'presidium': 1, 'yang': 2, 'hyong': 1, 'sop': 1, 'paek': 1, 'lantos': 3, 'veligonda': 1, 'andhra': 8, 'resumption': 19, 'participated': 15, 'boycotted': 32, 'shattering': 4, 'lull': 9, 'beheaded': 11, 'swollen': 4, 'derailed': 9, 'shrank': 4, 'consumers': 33, 'faster': 15, 'pessimistic': 5, 'drives': 15, 'clues': 10, 'midwest': 8, 'flood': 33, 'bhat': 2, 'inayatullah': 1, 'acted': 21, 'stroll': 2, 'predominantly': 19, 'tal': 15, 'baath': 9, 'salih': 4, 'barham': 3, 'sodden': 1, 'marshy': 1, 'brightly': 1, 'colored': 2, 'valuable': 7, 'marlins': 2, 'josh': 4, 'avoided': 5, 'arbitration': 11, 'stints': 1, 'pitchers': 1, 'sep': 1, 'championship': 17, 'postseason': 1, '02': 23, 'yankees': 4, 'remembrance': 6, 'motorists': 5, 'wailed': 1, 'sirens': 3, 'yad': 1, 'commemorations': 7, 'vashem': 1, 'auschwitz': 9, 'rescuers': 40, 'ropes': 4, 'wade': 6, 'carriages': 1, 'birkenau': 2, 'sounded': 4, 'shofar': 2, 'roma': 1, 'ram': 5, 'trek': 3, 'gypsies': 1, 'takes': 65, 'extermination': 5, 'promising': 19, 'cassim': 2, 'malawai': 1, 'chilumpha': 8, 'mutharika': 18, 'bingu': 6, 'assassin': 3, 'malawi': 33, 'feuding': 4, 'mates': 3, 'lessons': 4, 'luc': 1, 'chatel': 1, 'maps': 7, 'google': 32, 'objecting': 1, 'mislead': 3, 'impression': 4, 'update': 6, 'listing': 3, 'views': 27, '530': 2, 'foreigner': 6, 'icrc': 5, 'spirits': 4, 'lefevre': 3, 'gauthier': 1, 'germans': 17, 'sibling': 1, 'sister': 18, 'geneina': 4, 'staffer': 3, 'communities': 71, 'captors': 10, 'bashir': 26, 'hostility': 5, 'obasanjo': 27, 'olusegun': 14, 'mouths': 1, 'kerekou': 9, 'mathieu': 4, 'babies': 4, 'sterility': 2, 'crimea': 7, 'palin': 1, 'unmarried': 1, 'teen': 10, 'spotlight': 2, 'padden': 2, 'teens': 3, 'conveyed': 6, 'management': 53, 'lapses': 2, 'kojo': 3, 'flaws': 5, 'correct': 11, 'lucrative': 7, 'contracts': 21, 'cotecna': 1, 'siyam': 1, 'upbeat': 3, 'olaf': 3, 'assessment': 15, 'centanni': 3, 'wiig': 3, 'steve': 13, 'solovtsov': 1, 'intermediate': 1, 'uncalled': 1, 'topolanek': 1, 'mirek': 1, 'jaroslaw': 4, 'syrians': 5, 'caption': 3, 'taylor': 25, 'wound': 8, 'apology': 15, 'gunshot': 6, 'redskins': 1, 'mix': 6, 'doubted': 1, 'cholera': 15, '177': 2, 'sickened': 4, 'bissau': 6, 'instigate': 3, 'assassinate': 12, 'beach': 17, 'notched': 1, 'delray': 3, 'paraguay': 20, 'delgado': 2, 'ramon': 6, 'cruised': 1, 'xavier': 3, 'malisse': 4, 'gimelstob': 1, 'justin': 5, 'oliver': 2, 'marach': 1, 'guillermo': 4, 'todd': 1, 'widom': 1, 'plaguing': 4, 'decrease': 8, 'ministries': 10, 'tariffs': 16, 'chile': 59, 'panamanian': 11, 'intestinal': 15, 'cousin': 10, 'sultan': 8, 'juhyi': 1, 'raad': 2, 'hashim': 2, 'purports': 1, 'sunna': 8, 'ansar': 11, 'embraces': 1, 'dining': 8, 'dehydration': 7, 'darren': 2, 'boisvert': 1, 'winterized': 1, 'distributing': 14, 'sheets': 4, 'plastic': 15, 'essentially': 1, 'revived': 8, 'lawsuit': 23, 'reconsider': 9, 'justices': 16, 'practicing': 4, 'tortured': 15, 'imprisonment': 13, 'freely': 6, 'restoration': 11, 'humanely': 3, 'eritrean': 16, 'ruphael': 1, 'jemua': 3, 'amen': 1, 'gumuz': 1, 'benishangul': 1, 'latrines': 1, 'contaminate': 1, 'eritrea': 39, 'capping': 2, 'schwarzenegger': 13, 'course': 29, 'arnold': 8, 'treasure': 4, 'bold': 4, 'pollution': 23, 'tribune': 1, 'chicago': 13, 'print': 4, 'electronic': 28, 'protections': 4, 'random': 10, 'profiling': 5, 'dictatorship': 15, 'kuba': 1, 'laith': 2, 'rats': 1, 'brigade': 15, 'badr': 4, 'rockslide': 2, 'cliff': 6, 'nasr': 2, 'manshiyet': 2, 'limestone': 1, 'debris': 25, 'rockslides': 2, 'farmers': 48, 'grains': 2, 'sunflower': 1, 'fernandez': 11, 'rebate': 4, 'seeds': 8, 'cristina': 4, 'soy': 3, 'grain': 14, 'subsidize': 1, 'constructed': 12, 'roadblocks': 7, 'revise': 5, 'redistribute': 5, 'william': 35, 'brownfield': 9, 'trafficking': 52, 'accusation': 14, 'extremism': 20, 'sana': 4, 'sidnaya': 2, 'profiled': 1, 'student': 41, 'scanned': 2, 'rhine': 1, 'stems': 5, 'westphalia': 1, 'observatory': 6, 'magazine': 49, 'interrogated': 8, 'unauthenticated': 1, 'accompanying': 5, 'yousifi': 4, 'behead': 3, 'minas': 5, 'spear': 2, 'kilogram': 6, 'qaim': 7, 'karabila': 2, 'pharmaceutical': 11, 'merck': 10, 'experimental': 2, 'cervical': 6, 'viruses': 8, 'beckstein': 1, 'guenther': 1, 'bavarian': 1, 'papillovirus': 1, 'gardasil': 2, 'cancerous': 2, 'lesions': 1, 'immune': 3, 'transmitted': 15, 'hpvs': 2, 'glaxosmithkline': 3, 'eric': 5, 'kiraithe': 2, 'andrej': 1, 'fleur': 1, 'gerd': 1, 'dissel': 1, 'uwe': 1, 'hermlin': 1, 'gush': 1, 'katif': 1, 'hanif': 2, 'atmar': 3, 'muthmahien': 1, 'hai': 2, 'puppets': 2, '183': 1, 'watchlist': 1, 'milliyet': 2, 'book': 34, 'updated': 6, 'separatism': 3, 'fundamentalism': 6, 'buys': 6, '244': 1, 'marburg': 19, 'luanda': 10, 'uige': 13, 'stricken': 17, 'incurable': 4, 'contagious': 6, 'fluids': 9, 'ebola': 16, 'hygienic': 3, 'bodily': 7, 'restraints': 3, 'affiliated': 14, 'conceded': 4, 'confident': 15, 'expelling': 6, 'willingly': 2, 'enemies': 13, 'aggressors': 1, 'accusing': 53, 'mughani': 3, 'aiming': 7, 'minimize': 5, 'sener': 1, 'abdulatif': 1, 'conte': 5, 'crane': 4, 'guinean': 1, 'lansana': 3, 'humans': 65, 'everglades': 2, 'stretch': 12, 'glades': 1, 'shrink': 6, 'wildlife': 16, '1930s': 8, 'wading': 1, 'alligators': 1, "'ll": 9, 'plenty': 3, 'sight': 10, 'lane': 1, 'awesome': 1, 'anywhere': 10, 'installing': 5, 'kibo': 7, 'toilet': 8, 'fujimori': 33, 'ancestry': 3, 'sanctioning': 1, 'embezzlement': 9, 'petitioned': 7, 'extradite': 15, 'feminist': 1, '1965': 7, 'chronicles': 1, 'sculptures': 3, 'nateghi': 2, 'behnam': 2, 'jim': 19, 'bertel': 9, 'communication': 20, 'file': 20, 'cantoni': 10, 'clementina': 6, 'widows': 4, 'tearful': 2, 'raphael': 2, 'sprint': 4, 'ruhpolding': 1, 'frode': 1, 'andresen': 3, 'penalties': 6, 'rösch': 1, 'greis': 1, 'standings': 9, '298': 2, 'misses': 1, 'laps': 2, 'thai': 47, 'bhumibol': 3, 'shinawatra': 12, 'nahdlatul': 1, 'muzadi': 3, 'ulama': 1, 'hasyim': 1, 'adulyadej': 3, '650': 10, 'spiegel': 3, 'der': 4, 'landmines': 5, 'formation': 24, 'abakar': 1, 'itno': 2, 'adre': 3, 'janjaweed': 15, 'whoever': 5, 'something': 20, 'mulino': 2, 'nephew': 10, 'liberty': 9, 'canyon': 3, 'ecosystem': 4, 'lever': 1, 'dirk': 1, 'releasing': 17, 'glen': 1, 'regulates': 2, 'kempthorne': 2, 'beaches': 7, 'downstream': 4, 'sediment': 3, 'tallest': 3, 'skyscraper': 1, 'experiments': 12, 'darien': 1, 'motives': 1, 'timed': 3, 'superintendent': 1, 'suggesting': 13, 'bulldozer': 1, 'discovering': 4, 'fog': 5, 'disagreements': 12, 'halutz': 8, 'occasional': 6, '102': 7, 'stance': 16, 'popularity': 15, '166': 5, 'congressional': 56, 'rightwing': 2, 'ingrid': 4, 'blazy': 5, 'douste': 5, 'philippe': 4, 'betancourt': 11, 'gabriel': 2, 'unthinkable': 2, 'usmani': 1, 'turban': 8, 'daynunay': 1, 'shabab': 23, 'publicity': 4, 'stunt': 2, 'overrun': 7, 'acquire': 5, 'konstantin': 4, 'kosachyov': 2, 'betweens': 1, 'telegraph': 9, 'teheran': 1, 'delivering': 14, 'falls': 8, 'bisengimina': 3, 'bargain': 9, 'pled': 1, 'gikoro': 1, 'arlete': 1, 'confess': 3, 'ramaroson': 1, 'quarantine': 9, 'aware': 20, 'organisation': 1, 'luzon': 4, 'logging': 5, 'assist': 24, 'dubai': 27, 'doncasters': 1, 'broader': 12, 'angered': 30, 'lima': 8, 'authorizing': 16, 'faxed': 2, 'santiago': 17, 'monteiro': 1, 'ensuring': 3, 'tasked': 7, 'contempt': 2, 'thein': 8, 'shein': 2, 'sentences': 37, 'permitted': 7, 'nearing': 11, 'enshrine': 1, 'bankruptcy': 14, 'jurisdiction': 14, 'yuganskneftgaz': 1, 'anyway': 3, 'firms': 34, 'gazprom': 43, 'suing': 3, 'rosneft': 12, 'competitions': 2, 'weightlifting': 3, 'owes': 8, 'placement': 3, '153': 2, '175': 3, 'preferred': 12, '148': 11, 'perpetual': 6, 'rogers': 5, 'retractable': 1, 'holders': 5, 'redeem': 1, 'conversion': 21, 'convertible': 4, 'coupon': 1, 'industrialized': 34, 'enterprise': 8, 'liechtenstein': 16, 'induced': 6, 'easy': 8, 'incorporation': 2, 'nominal': 4, 'plutonium': 12, 'disrepute': 1, 'sport': 18, 'franc': 3, 'efta': 4, 'harmonize': 2, 'grey': 4, 'model': 14, 'oecd': 12, 'educated': 5, 'uruguay': 30, '2000s': 3, 'readmitted': 2, 'brake': 1, 'decelerated': 1, 'vigorous': 4, 'expenditure': 1, 'managed': 31, 'float': 2, 'pounds': 4, 'equals': 7, 'rapid': 27, 'significantly': 26, 'utilities': 7, 'contributes': 9, 'considerably': 3, 'hoard': 1, 'secession': 19, 'depreciate': 2, 'uncertainty': 10, 'restrain': 5, 'sanction': 2, 'iwf': 1, 'coaches': 5, 'cantons': 1, '1291': 1, 'defensive': 6, 'localities': 2, 'succeeding': 6, '1499': 1, 'centralized': 4, 'modified': 13, '1848': 2, '1874': 2, 'honored': 10, 'neutrality': 9, 'omen': 2, 'jealous': 2, 'crow': 13, 'cawed': 1, 'loudly': 2, 'perching': 1, 'wondered': 2, 'journey': 12, 'cry': 1, 'foreboded': 1, 'caw': 2, 'companion': 9, 'unpleasant': 1, 'smell': 2, 'tan': 3, 'tanner': 2, 'yard': 11, 'inconvenience': 2, 'accustomed': 3, 'farmer': 24, 'implacable': 1, 'centre': 2, 'tow': 3, 'tail': 12, 'dissemble': 2, 'insured': 4, 'pelt': 1, 'frogs': 7, 'pond': 2, 'pleasure': 5, 'shantytowns': 2, 'softball': 4, 'inning': 1, 'math': 1, 'complicated': 5, "'m": 9, 'clerk': 4, 'customer': 6, 'leaned': 2, 'priest': 18, 'blackboard': 3, 'dry': 21, 'chalk': 3, 'cure': 4, 'doctor': 26, 'looked': 12, 'improves': 4, 'pathogenic': 5, 'kaduna': 5, 'jaji': 1, 'disinfected': 4, 'ratifying': 1, 'armenians': 10, 'hanan': 1, 'raufi': 1, 'piercing': 1, 'armor': 10, 'applies': 4, 'rasmussen': 5, 'anders': 5, 'fogh': 4, 'sewage': 7, 'dmitry': 7, 'ophelia': 8, 'northward': 3, 'battering': 4, 'massachusetts': 17, 'scotia': 2, 'nova': 4, 'occupation': 34, 'bystanders': 11, 'pul': 5, 'sari': 1, 'robbed': 7, 'nicotine': 6, 'cigarettes': 10, 'inhale': 1, 'smoker': 1, 'yielded': 5, 'varied': 2, '116': 2, 'brands': 5, 'addictive': 1, 'grows': 4, 'incursion': 19, 'tayyip': 31, 'patience': 5, 'recep': 31, 'erdogan': 47, 'mounting': 25, 'classify': 8, 'khushab': 3, 'cautiously': 3, 'kim': 68, 'sook': 2, 'concerning': 7, 'dong': 7, 'chung': 12, 'machimura': 6, 'nobutaka': 4, 'nimeiri': 4, 'lslamic': 1, '79': 14, 'omdurman': 2, '1985': 16, 'chapters': 2, 'sharia': 8, 'alienated': 2, 'barges': 2, 'warri': 3, 'gunship': 4, 'ijaw': 3, 'airstrip': 4, 'presutti': 6, 'carolyn': 9, 'accomplished': 7, 'hmong': 5, 'score': 11, 'resettlement': 6, 'wisconsin': 2, 'handful': 11, 'midwestern': 11, 'tham': 1, 'krabok': 1, 'enhanced': 6, 'screenings': 3, 'mujuru': 3, 'joyce': 3, 'msika': 1, 'filling': 5, 'vacancy': 3, 'muzenda': 1, 'chosen': 25, 'mnangawa': 1, 'emmerson': 1, 'derailing': 2, 'quentier': 1, 'ariane': 3, 'educating': 2, 'transatlantic': 2, 'trends': 7, 'marshall': 7, 'polled': 6, 'bryan': 4, 'whitman': 5, 'kar': 2, 'cyrus': 1, 'laywers': 1, 'prayerful': 1, 'fenty': 1, 'attendance': 6, 'adrian': 3, 'gift': 5, 'yunnan': 7, 'shook': 14, 'county': 24, 'yanjin': 3, 'zhaotong': 1, 'seismological': 2, 'hillsides': 2, 'plateau': 5, 'guizhou': 2, 'uri': 7, 'bu': 1, 'scrap': 10, 'repealing': 2, 'impasse': 10, 'pick': 22, 'regressing': 2, 'staffers': 16, 'compounds': 8, 'sub': 24, 'finals': 19, 'bennett': 6, 'khairul': 1, 'amri': 1, 'daniel': 22, 'agu': 1, 'casmir': 1, 'substitute': 2, 'mahyadi': 1, 'deflected': 2, 'panggabean': 1, 'shaky': 7, 'shaktoi': 2, 'sees': 14, 'friction': 4, 'hakimullah': 2, 'recording': 18, 'mehsud': 6, 'audio': 16, 'journalism': 9, 'yahoo': 7, 'relating': 9, 'osako': 1, 'subpoenas': 1, 'australians': 12, 'smuggle': 9, 'schapelle': 2, 'corby': 2, 'fitzgerald': 4, 'operative': 16, 'identity': 27, 'toughest': 2, 'rossendorf': 1, 'dresden': 4, 'reunification': 19, 'observe': 17, 'loading': 5, 'echoing': 3, 'yorker': 4, 'psychological': 5, 'momir': 1, 'savic': 2, 'visegrad': 2, 'dayton': 16, 'oilrig': 1, 'valerie': 3, 'plame': 4, 'flipped': 4, 'drenched': 4, 'switch': 7, 'recreated': 2, 'vargas': 2, 'getulio': 1, '1953': 8, 'ironically': 1, 'rig': 11, 'unresolved': 10, 'kitgum': 1, 'bigombe': 1, 'mediator': 13, 'betty': 3, 'displacing': 5, 'knowingly': 3, 'lander': 5, 'garden': 15, 'drinkable': 1, 'owners': 20, 'shelves': 3, 'marlon': 3, 'defillo': 1, 'garmser': 4, 'importer': 2, 'readiness': 9, 'bracing': 9, 'tightening': 3, 'kamchatka': 2, 'remorse': 2, 'hanoi': 18, 'resident': 5, 'vaccinations': 9, 'opinions': 7, 'meaning': 11, 'nonbinding': 1, 'unfit': 2, 'soe': 3, 'tangerang': 4, '83rd': 1, 'becoming': 43, '190': 7, 'mutate': 17, 'transmissible': 4, 'zhari': 4, 'panjwayi': 5, 'credible': 13, 'expands': 4, 'wanting': 3, 'hashish': 1, 'fueling': 12, 'genital': 1, 'mutilation': 2, 'cruel': 10, 'genitalia': 1, 'subjected': 8, 'circumcision': 2, 'girls': 40, 'unicef': 14, 'hemorrhaging': 1, 'processing': 30, 'totally': 7, 'chechen': 30, 'chechens': 3, 'jails': 11, 'abusers': 2, 'consultations': 14, 'surayud': 6, 'chulanont': 3, 'unite': 8, 'fueled': 21, 'executives': 21, 'awaiting': 18, 'crescent': 6, 'sighting': 6, 'sunset': 3, 'abstaining': 3, 'depending': 7, 'scorching': 3, 'warmest': 3, 'koran': 16, 'meals': 6, 'iftars': 1, 'invitations': 1, 'conclude': 9, 'fitr': 12, 'nyala': 2, 'arming': 5, 'heinonen': 4, 'olli': 4, 'newspapers': 41, 'dubbed': 13, 'naturalized': 2, 'legally': 12, 'outpost': 14, 'reputed': 5, 'containing': 12, 'morphine': 1, 'cache': 19, 'enacted': 18, 'ineffectiveness': 3, 'marseille': 2, 'kibar': 1, 'housed': 12, 'delinquency': 1, 'filing': 10, 'ages': 3, 'baume': 1, 'immigrant': 16, 'rioted': 5, 'bayelsa': 5, 'berger': 5, 'subsidiary': 13, 'bilfinger': 3, 'extracted': 6, 'warplanes': 34, 'colonial': 15, '1950s': 5, 'mau': 2, 'argues': 5, '1952': 4, '1959': 12, 'drill': 13, 'explore': 8, 'teikoku': 1, 'exclusive': 13, 'demarcation': 6, 'analyzing': 2, 'authorization': 8, 'undersea': 4, 'desecration': 6, 'ceased': 7, 'disclosed': 12, 'newsweek': 7, 'rattle': 4, 'interrogators': 21, 'flushed': 3, 'uproar': 2, 'retracted': 4, 'interceptor': 6, 'alexei': 5, 'kuznetsov': 2, 'characteristics': 1, 'fabricating': 2, 'argument': 11, 'massacring': 2, 'plundering': 1, 'resource': 15, 'reparations': 1, 'camilla': 5, 'married': 24, 'glimpse': 5, 'onlookers': 4, 'cornwall': 3, 'duchess': 3, 'dedicate': 1, 'tasnim': 3, 'aslam': 5, 'scrapped': 6, 'princess': 2, 'diana': 1, 'sarajevo': 11, 'dragomir': 2, 'corps': 27, 'relation': 4, 'radovan': 12, 'ratko': 11, 'mladic': 14, 'karadzic': 18, 'triple': 15, '182': 1, 'amarah': 3, 'gedi': 19, 'airstrips': 2, 'parliamentarians': 6, 'yusef': 2, 'abdullahi': 13, 'relocate': 12, 'relocated': 3, 'manchester': 2, 'refinement': 1, 'originally': 37, 'initiate': 5, 'imprisoned': 32, 'royalist': 6, 'supposed': 15, 'turki': 5, 'muteiry': 2, 'fuheid': 1, 'nashmi': 1, 'timing': 11, 'fawaz': 2, 'khobar': 1, 'salvatrucha': 2, 'mara': 2, 'racketeering': 1, 'nineteen': 2, 'indictments': 9, 'suburban': 1, 'salvador': 37, 'traditionally': 18, 'await': 6, 'salute': 1, 'fists': 1, 'signal': 10, 'rachel': 1, 'chandler': 2, 'seychelles': 13, 'yacht': 2, 'lynn': 3, 'warships': 10, 'armada': 1, 'hijackings': 9, 'seas': 9, 'blindfolded': 8, 'sherif': 4, 'ihab': 3, 'mashruh': 1, 'krishna': 3, 'mahara': 1, 'dialysis': 1, 'coma': 13, 'anat': 1, 'dolev': 1, 'accumulated': 4, 'accumulation': 2, 'ningxia': 2, 'zhongwei': 1, 'prachanda': 7, 'sitaula': 1, 'reappearing': 1, '130': 29, 'gotten': 5, 'graphic': 5, 'hassem': 1, 'knew': 11, 'barzan': 2, 'exchanges': 9, 'yelled': 3, 'ramsey': 1, 'clampdown': 5, 'headfirst': 1, 'snowbank': 1, 'kid': 8, 'wo': 4, 'false': 34, 'ritchie': 3, 'bob': 18, 'sue': 6, 'michigan': 8, 'roughed': 1, 'inviting': 4, 'existed': 8, 'insufficient': 10, 'actress': 21, 'pamela': 2, 'headlines': 4, 'marrying': 1, 'span': 5, 'divorcing': 2, 'provoking': 4, 'garissa': 1, 'disrupt': 34, 'yes': 9, 'oranges': 1, 'bananas': 7, 'commodies': 1, 'exempts': 2, '197': 2, 'inconsistencies': 2, 'discrepancies': 1, 'dili': 1, 'martinho': 1, 'gusmao': 1, 'recount': 4, 'horta': 1, 'olo': 1, 'lu': 7, 'brawl': 5, 'blindness': 2, 'uncontrolled': 1, 'deregulation': 1, 'sticks': 6, 'musa': 13, 'yalahow': 1, 'sudi': 1, 'presumptive': 3, 'edgware': 1, 'hafs': 3, 'congratulating': 1, 'expert': 18, 'clarke': 10, 'babar': 3, 'presenting': 5, 'labeled': 8, 'combatant': 3, 'saqiz': 2, 'concides': 1, 'sped': 5, 'tracking': 9, 'notifying': 1, 'chiefs': 19, 'radioed': 2, 'confrontations': 5, 'protocol': 19, 'moratorium': 12, 'brandenburg': 1, 'whales': 18, 'famed': 8, 'displayed': 9, 'whale': 6, 'greenpeace': 4, 'mammals': 2, 'fisherman': 15, 'nets': 7, 'collisions': 1, 'drown': 2, 'stefanie': 1, 'werner': 1, 'biologist': 3, 'whaling': 12, 'anchorage': 1, 'bedroom': 1, 'molested': 2, 'teenage': 12, 'jackson': 29, 'narsingh': 2, 'arjun': 1, 'recalled': 20, 'reformists': 3, '280': 4, 'loyalty': 4, 'disqualify': 2, 'testified': 6, 'patient': 14, 'accuser': 3, 'conservatives': 11, 'disqualifications': 1, 'defended': 44, 'compelling': 1, 'monthly': 18, 'license': 22, 'concession': 3, 'lara': 5, 'tolerate': 12, 'outlets': 13, 'overly': 2, 'rctv': 12, 'metal': 16, 'katowice': 4, 'exposition': 2, 'climb': 5, 'silesia': 1, 'homing': 2, 'pigeon': 3, 'liquor': 6, 'entertainer': 6, 'wine': 4, 'drink': 14, 'jet': 36, 'hanoun': 7, 'nearest': 4, 'mustafa': 12, 'productive': 7, 'carat': 3, 'gemstone': 1, 'auctioned': 1, 'blue': 7, 'bested': 1, 'fetched': 2, 'hancock': 1, 'jeweler': 2, 'gemstones': 1, 'collects': 7, 'moussaieff': 1, 'jewelers': 2, 'stone': 16, 'crystal': 1, 'boron': 1, 'vivid': 1, 'hashemi': 8, 'tareq': 3, 'benchmark': 8, 'dna': 14, 'revoke': 1, 'identifications': 1, 'sophisticated': 8, 'cyclone': 22, 'moriarty': 2, 'sidr': 4, 'anticipating': 1, 'bangladeshi': 22, 'plentiful': 3, 'bermuda': 10, 'horbach': 1, 'luxury': 20, 'reinsurance': 3, 'derives': 4, 'arable': 2, 'shari': 1, 'ubangi': 1, 'misrule': 1, 'lasted': 18, 'tumultuous': 1, 'ange': 1, 'patasse': 1, 'felix': 4, 'bozize': 2, 'affirmed': 5, 'tacit': 1, 'lawlessness': 7, 'persist': 2, 'countryside': 11, '1915': 1, 'gilbert': 4, '1892': 1, '1941': 6, '1943': 1, 'makin': 1, 'victories': 5, 'amphibious': 3, 'garrisons': 1, 'tarawa': 1, 'kiribati': 7, 'relinquished': 5, 'sparsely': 2, 'capitalist': 3, 'reversal': 5, 'oversupply': 1, 'actually': 20, 'reflected': 13, 'jalil': 2, 'jilani': 1, 'competitiveness': 5, 'privatize': 4, 'indebted': 11, 'contagion': 1, 'estate': 20, 'oversaw': 9, 'savings': 10, 'pressuring': 8, 'asleep': 10, 'greedily': 2, 'serpent': 12, 'nook': 1, 'turning': 29, 'mortal': 2, 'exclaimed': 7, 'agony': 2, 'windfall': 2, 'unhappy': 4, 'sacrifices': 5, 'pasture': 4, 'domain': 3, 'intruded': 1, 'desiring': 2, 'stranger': 1, 'punishing': 4, 'consented': 6, 'obtaining': 7, 'antipathy': 1, 'novakatkan': 2, 'madagonia': 3, 'novakatka': 2, 'apologise': 1, 'novakatkans': 2, 'ensued': 3, 'madagonians': 1, 'thereafter': 4, 'chagrined': 1, 'innings': 14, 'halted': 23, 'printed': 13, 'garnered': 3, 'rigged': 25, 'molestation': 8, 'santa': 15, 'barbara': 4, 'jurors': 7, 'fergana': 1, 'malicious': 3, 'disgusting': 4, 'leaks': 5, 'leaked': 7, 'nonexistent': 1, 'card': 16, 'uncontained': 1, 'disappointing': 9, 'charki': 2, 'overpowered': 3, 'heidar': 2, 'moslehi': 2, 'mahabad': 3, 'petra': 3, 'akbar': 17, 'rafsanjani': 14, 'expediency': 2, 'arch': 3, 'accepts': 6, 'cooling': 5, 'observing': 14, 'plunging': 5, 'mahinda': 5, 'briefed': 6, 'rajapakse': 5, 'burden': 22, 'berthiaume': 1, 'christiane': 1, 'litani': 3, '404': 2, 'approaches': 8, 'navi': 1, 'pillay': 1, 'taint': 1, 'intimidate': 5, 'secede': 4, 'amputation': 1, 'prevail': 3, 'thanksgiving': 21, 'depart': 9, 'credited': 6, 'azimi': 5, 'belonged': 11, 'strains': 7, 'isaf': 16, 'inciting': 11, 'heckling': 1, 'izzadeen': 3, 'anjem': 1, 'choudary': 1, 'ghurabaa': 1, 'electrician': 2, 'aged': 6, 'bibles': 2, 'malatya': 2, 'slit': 2, 'zirve': 1, 'jumped': 21, 'printing': 7, 'wrestling': 1, 'trabzon': 1, 'blabague': 1, 'marboulaye': 1, 'demonstrating': 7, 'infiltrated': 7, 'disruptive': 3, 'demonstrator': 2, 'tram': 1, 'intensity': 2, 'waged': 13, 'seeks': 22, 'appeasing': 2, 'diluting': 1, 'affront': 2, 'taunt': 2, 'behavior': 20, 'punched': 2, 'shoulders': 2, 'stomach': 9, 'cremation': 2, 'uncertainties': 2, 'inhibit': 1, 'constructive': 9, 'stakeholder': 2, 'raises': 10, 'contracting': 9, 'ducks': 19, 'checking': 3, 'crater': 5, 'roving': 1, 'panorama': 1, 'rover': 6, 'layers': 3, 'formations': 2, 'mystery': 2, 'exposed': 20, 'reconnaissance': 12, 'descend': 1, 'wheeled': 2, 'adolescent': 1, 'pregnancy': 5, 'fincher': 5, 'leta': 5, 'yu': 4, 'ik': 1, 'woo': 5, 'obudu': 1, '90th': 3, 'leveled': 3, 'passports': 9, 'hidden': 14, 'lagos': 26, 'stoppage': 8, 'adams': 4, 'oshiomole': 1, 'costly': 6, 'arabian': 12, 'riyadh': 17, 'organizer': 5, 'fagih': 3, 'learn': 16, 'manage': 10, 'healthier': 1, 'stabbing': 4, 'rahman': 13, 'aref': 8, 'overthrown': 4, '91': 8, '1958': 5, 'prop': 3, 'cardiovascular': 1, 'obese': 2, 'overweight': 2, 'smith': 29, 'deterrent': 3, 'stifle': 3, 'yong': 4, 'isolate': 5, 'breaks': 15, 'destroy': 36, 'aggressor': 1, 'il': 25, 'jong': 24, 'beef': 21, 'pending': 18, 'auto': 14, 'insurance': 44, 'estimating': 1, 'clearer': 2, 'adjusters': 1, 'becomes': 11, 'ravage': 1, 'burn': 12, 'looters': 5, 'walker': 3, 'herbert': 4, 'fundraising': 6, 'sarkozy': 44, 'moody': 3, 'mortgage': 13, 'underestimating': 1, 'defaults': 3, 'spy': 28, 'specified': 4, 'sensitivities': 3, 'emirates': 19, 'dhafra': 1, '2s': 1, 'expeditionary': 1, '380th': 1, 'enduring': 5, 'altitude': 6, 'unnamed': 28, 'oman': 18, 'haditha': 11, 'yousi': 1, 'mizhir': 1, 'bongo': 5, 'meantime': 15, 'install': 14, 'staging': 13, 'raped': 10, 'mukhtaran': 1, 'mai': 2, 'punishment': 13, 'marriage': 20, 'strides': 4, 'oppressed': 4, 'terminals': 3, 'bonny': 2, 'lifts': 3, 'contractual': 1, 'flagged': 9, 'bangladeshis': 5, 'biscaglia': 1, 'overboard': 2, 'overtook': 1, 'ashia': 1, 'hansen': 8, 'indoor': 8, 'contender': 8, 'birmingham': 2, 'breathing': 6, 'circulatory': 2, 'joaquin': 4, 'navarro': 5, 'valls': 4, 'scriptures': 1, 'ruini': 1, 'cardinal': 12, 'camillo': 1, 'bishops': 4, 'crocker': 6, 'ryan': 8, 'urinary': 1, 'fever': 23, 'cardio': 1, 'tract': 3, 'gravity': 4, 'underwent': 29, 'serviceman': 3, 'soh': 1, 'letting': 5, 'antonov': 1, 'antonovs': 2, 'pronk': 6, 'insist': 8, 'sok': 1, 'naypyidaw': 2, 'chol': 1, 'doo': 1, 'hwan': 2, 'severed': 12, 'chun': 8, "ya'akov": 1, 'alperon': 2, 'careers': 2, 'physicians': 7, 'knocking': 6, 'lamp': 1, 'macy': 3, 'wayward': 1, '1924': 4, 'characters': 3, 'resemble': 2, 'balloons': 2, 'knocked': 12, 'cat': 11, 'lamppost': 2, 'hat': 2, 'endorsing': 3, 'nicolas': 27, 'endorsement': 7, 'bayrou': 2, 'segolene': 4, 'centrist': 9, 'carlos': 34, 'logarda': 1, 'patron': 2, 'almanza': 1, 'fulfill': 6, 'putumayo': 3, 'leyva': 2, 'extortion': 3, 'dror': 1, 'compensate': 10, 'evacuation': 21, 'hardline': 13, 'nationalists': 14, 'encounter': 4, 'soku': 1, 'briton': 9, 'females': 1, 'prosecuted': 7, 'unreported': 5, 'loaned': 4, 'interviewers': 1, 'bodman': 5, 'refiners': 5, 'caverns': 2, 'heating': 13, 'surged': 23, 'converting': 6, 'yoram': 1, 'haham': 1, 'mobsters': 1, 'zahir': 5, 'scheme': 6, 'hapoalim': 2, 'customers': 15, 'mozambique': 16, 'vibrant': 1, 'impressed': 6, 'disasters': 28, 'susan': 4, 'schwab': 2, 'singled': 9, 'roemer': 1, 'bipartisan': 7, 'crises': 7, 'lender': 2, 'overhaul': 4, 'finishing': 5, '184': 3, 'nicaragua': 40, 'kazem': 1, 'hamaneh': 1, 'vaziri': 1, 'investor': 16, 'telling': 16, 'artist': 16, 'vilks': 7, 'lars': 2, 'momammad': 1, 'baghdadi': 5, 'katz': 1, 'amy': 1, 'dog': 22, 'barbaric': 6, 'shocking': 5, '14th': 11, '8th': 6, 'narcotics': 10, 'poppies': 4, 'devoted': 5, 'reopen': 19, 'harvest': 10, 'bountiful': 2, 'pleased': 6, 'kidnap': 20, 'modifications': 3, 'conform': 5, 'osuji': 2, 'fabian': 1, 'paying': 28, 'wabara': 1, 'adolphus': 1, 'ahronot': 3, 'slated': 10, 'yediot': 6, 'fahd': 5, 'comprising': 2, 'boosted': 24, 'mercantile': 8, '41': 37, 'dismay': 3, 'unwelcome': 4, 'answer': 18, '273': 1, 'welsh': 1, 'murdering': 12, 'comrades': 4, 'bragg': 2, '101st': 5, 'ambushing': 3, 'hasan': 5, 'airborne': 9, 'slept': 5, 'hlinethaya': 1, 'snap': 7, 'constant': 5, 'ridicule': 4, 'sentencing': 6, 'madonna': 11, 'orphans': 6, 'blantyre': 3, 'translated': 3, 'roses': 1, 'orphan': 1, 'lilongwe': 3, 'liz': 1, 'rosenberg': 1, 'plight': 5, 'farms': 41, 'bolivarian': 2, '412': 1, 'seromba': 1, 'lenient': 3, 'athanase': 1, 'parish': 3, 'orchestrate': 2, 'demolish': 4, 'moderates': 3, 'h5': 7, 'jalgaon': 4, 'nandurbar': 1, 'navapur': 1, 'culled': 20, 'heihe': 1, 'blagoveshchensk': 1, '65th': 3, 'liberated': 2, 'undesirable': 1, 'exterminate': 2, 'declares': 8, 'taipei': 23, 'opposing': 16, 'annexation': 3, 'fail': 23, 'guidelines': 17, 'renegade': 10, 'kfar': 3, 'darom': 2, 'committees': 11, 'pardoning': 1, 'consular': 12, 'elite': 8, 'seals': 4, 'sailor': 3, 'evaded': 2, 'scouring': 1, 'villaraigosa': 3, 'studio': 11, 'xvi': 17, 'angelus': 1, 'hearts': 4, 'loves': 1, 'blessing': 5, 'figaro': 3, 'le': 9, 'reasonable': 6, 'understand': 10, 'costner': 5, 'kevin': 19, 'breached': 5, 'fledgling': 5, 'mahee': 5, 'actor': 20, 'breach': 9, 'songwriter': 8, 'llc': 1, 'concerts': 6, 'marketing': 5, 'reneged': 5, 'qualifies': 1, 'unsustainable': 3, 'takatoshi': 1, 'kato': 2, 'therefore': 4, 'sustaining': 2, 'jew': 1, 'mohcine': 1, 'toufik': 1, 'hanouichi': 1, 'bouarfa': 1, 'acquitted': 14, 'meknes': 1, 'fez': 1, 'speaks': 6, 'trilateral': 1, 'gadahn': 1, 'fbi': 25, 'urgent': 12, 'demolition': 5, 'popularly': 6, 'evicted': 4, 'ann': 5, 'tibaijuka': 1, 'remainder': 8, 'semi': 27, 'zimbabweans': 1, 'charting': 1, 'scenarios': 4, 'mentality': 1, 'qiyue': 3, 'possibilities': 2, 'senkaku': 1, 'japanto': 1, 'daioyu': 1, 'advises': 4, 'ap': 11, 'kaka': 3, '44th': 2, 'stuttgart': 4, 'scoreless': 1, '53rd': 1, 'abalo': 1, 'ahn': 1, 'soo': 2, 'jung': 3, 'sputtered': 1, 'canadians': 15, 'wonderful': 2, "'ve": 3, 'professionalism': 2, 'norman': 2, 'kember': 1, 'loney': 1, 'harmeet': 1, 'sooden': 1, 'swords': 2, 'righteousness': 2, 'almeida': 4, 'damiao': 1, 'tome': 5, 'vaz': 4, 'sao': 14, 'doubtful': 1, 'fradique': 1, 'menezes': 12, 'awarding': 1, 'credibility': 13, 'servants': 12, 'stockpile': 6, 'rebellious': 2, 'painful': 6, '31st': 7, 'administrator': 16, 'ntawukulilyayo': 3, 'gisagara': 1, 'arusha': 2, 'prosecute': 13, 'braved': 1, 'consulates': 7, 'torrijos': 4, 'lage': 7, 'splitting': 4, 'rogue': 5, 'shield': 18, 'mobile': 40, 'complemented': 1, 'alvarez': 5, 'carriles': 19, 'posada': 18, 'bernardo': 3, 'cubana': 1, 'shannon': 8, 'demagogue': 2, 'principal': 8, 'citadel': 3, 'jr': 8, 'fidelity': 2, '1st': 3, 'walbrecher': 1, 'succeeds': 5, 'l': 5, 'kane': 2, 'tunisians': 2, 'versus': 4, 'terminated': 2, 'sept': 3, 'hobbled': 3, 'instability': 32, 'overdependence': 3, 'ineligible': 1, 'amounts': 19, 'modernizing': 2, 'emphasis': 2, 'improvements': 14, 'impediment': 3, 'blueprint': 3, 'partnerships': 6, 'receipts': 7, 'aluminum': 5, 'petrochemical': 6, 'feedstock': 1, 'competes': 2, 'struggles': 6, 'expatriate': 5, 'sponsorship': 3, 'slower': 7, 'bailout': 8, 'subsidy': 3, 'furniture': 2, 'ceramics': 1, 'paints': 2, 'relies': 15, 'fabrics': 1, 'marino': 13, 'tiles': 2, 'comparable': 5, 'repatriate': 4, 'outflows': 2, 'untaxed': 1, 'harmonizing': 1, 'fundamental': 7, 'influenced': 8, 'necessities': 3, 'occupier': 1, 'exploit': 1, 'deeper': 7, 'exhausted': 10, 'secondary': 8, 'mined': 3, 'anticipation': 6, 'cushion': 2, 'nauru': 3, 'wages': 9, 'overstaffed': 1, 'departments': 6, 'frozen': 18, 'arkansas': 4, 'afloat': 1, 'varying': 2, 'colonies': 8, 'merged': 7, 'gambia': 7, 'senegambia': 1, 'envisaged': 1, 'casamance': 3, 'mfdc': 1, 'democracies': 10, 'abdoulaye': 2, 'autocratic': 5, 'reelected': 9, 'undeclared': 3, 'homewards': 1, 'hare': 19, 'snared': 1, 'begged': 4, 'pretense': 3, 'horseback': 4, 'purchasing': 6, 'horseman': 2, 'sorely': 3, 'mathematician': 3, 'faculty': 2, 'physicist': 4, 'catches': 6, 'sink': 2, 'puts': 17, 'bucket': 2, 'leap': 1, 'sit': 9, 'melissa': 2, 'relevant': 3, 'solved': 4, 'thus': 14, 'picking': 9, 'retreat': 10, 'ashcroft': 3, 'ridge': 6, 'cofer': 1, 'counterterrorism': 7, 'schoomaker': 2, 'ultimate': 6, 'triumph': 2, 'cleland': 2, 'insisting': 8, 'isfahan': 9, 'installs': 1, 'restarting': 3, 'intentions': 18, 'appointments': 9, 'unnecessary': 7, 'steer': 2, 'leumi': 2, 'builder': 1, 'discounted': 5, 'raping': 6, 'stockpiling': 2, 'modeled': 4, 'enticed': 2, 'tossed': 3, 'bribing': 1, 'peacekeeper': 10, 'janez': 1, 'slovenian': 2, 'jansa': 1, 'notably': 8, 'roused': 1, 'obeid': 2, 'zambia': 17, 'verify': 10, 'sutanto': 2, 'batu': 2, 'rocked': 13, 'retrieving': 1, 'booby': 1, 'masterminding': 2, '69th': 2, 'tech': 24, 'packard': 1, 'hewlett': 1, 'helm': 3, 'directors': 13, 'fiorina': 2, 'carly': 1, 'printer': 1, 'hp': 2, 'profitable': 6, 'merge': 12, 'compaq': 1, 'ceo': 3, 'wayman': 1, 'resisting': 2, 'syarhei': 1, 'antonchyk': 1, 'vintsuk': 2, 'vyachorka': 2, 'unsanctioned': 1, 'milinkevich': 8, 'quashing': 2, 'genuine': 3, 'planche': 3, 'illegitimate': 5, 'principle': 14, 'wana': 3, 'preconditions': 6, 'insincere': 1, 'embarrassment': 3, 'admiration': 3, 'occasions': 12, 'traditions': 6, 'harmony': 4, 'keen': 2, 'harboring': 8, 'preceded': 2, 'sichuan': 27, 'shanwei': 1, 'guangdong': 19, 'migrant': 10, 'mireya': 2, 'moscoso': 2, 'coping': 3, 'guatemala': 31, 'belize': 8, 'farid': 6, 'soleiman': 1, 'upheld': 16, 'instance': 5, 'competitors': 9, 'servers': 2, 'mejia': 2, 'tolima': 1, 'angel': 6, 'typical': 5, 'antioquia': 1, 'victor': 13, 'billingslea': 4, 'financially': 6, 'feasible': 3, 'commissioned': 5, 'natwar': 8, 'reaffirmed': 18, 'silent': 7, 'simba': 1, 'banadir': 1, 'inflammatory': 3, 'landscape': 1, 'via': 16, 'zulima': 6, 'palacio': 6, 'shatt': 1, 'waterway': 2, 'yuganskneftegas': 5, 'bulba': 1, 'baikal': 2, 'payback': 1, 'specialists': 6, 'twins': 2, 'sulaymaniya': 3, 'quarantined': 8, 'obstacle': 8, 'creates': 4, 'slate': 8, 'maale': 5, 'adumim': 6, 'delp': 5, 'brad': 9, 'roadmap': 4, 'ultranationalist': 4, 'sacred': 8, 'sanctuary': 11, 'khogyani': 1, 'hampshire': 3, 'fiancee': 2, 'boston': 9, 'afterward': 9, 'espino': 1, 'rounded': 13, 'regrettable': 3, 'thapa': 1, 'protective': 5, 'bathroom': 7, 'charcoal': 4, 'examiner': 1, 'sealing': 3, 'grills': 1, 'toxicology': 3, 'castelgandolfo': 1, 'twenty': 11, 'monoxide': 2, 'confederations': 4, 'semifinals': 13, 'nuremberg': 1, 'ronaldinho': 2, 'adriano': 2, 'lukas': 1, 'podolski': 1, 'ballack': 1, 'frankenstadion': 1, '48th': 1, '23rd': 6, 'semifinal': 15, 'leipzig': 1, 'loser': 1, 'venture': 17, 'ukrgazenergo': 1, 'oversee': 15, 'monopoly': 15, 'naftogaz': 2, 'rosukrenergo': 1, 'sullivan': 2, 'cubic': 16, 'canberra': 7, 'garrigues': 8, 'medina': 11, 'anabel': 5, 'ekaterina': 2, 'bychkova': 1, 'nakamura': 2, 'aiko': 2, 'shahar': 1, 'peer': 2, 'bounced': 3, 'cassation': 2, 'catalonia': 3, 'scruff': 1, 'julia': 4, 'czink': 1, 'jeong': 2, 'yoon': 2, 'cho': 5, 'pianist': 2, 'berkofsky': 1, 'firebrand': 1, 'virtuosity': 1, 'classical': 3, 'charitable': 8, 'fame': 14, 'berkovsky': 1, 'robertson': 4, 'irina': 2, 'riddlesberger': 1, 'scot': 1, 'contents': 9, 'captives': 10, 'solicit': 1, 'leverage': 2, 'topple': 14, 'rooted': 9, 'anatolia': 7, 'belonging': 29, 'colonels': 1, 'launchers': 12, 'eighty': 3, 'solidify': 1, 'tendencies': 1, 'nationalistic': 1, 'populations': 14, 'afl': 1, 'cio': 1, 'unionists': 1, 'calmed': 2, 'distances': 5, 'digital': 7, 'priests': 16, 'forms': 17, 'embrace': 5, 'encourages': 7, 'clergy': 5, 'vocation': 1, 'notable': 2, 'languages': 3, 'facebook': 14, 'youtube': 6, 'pope2you': 1, 'cricketers': 2, 'fixing': 5, 'butt': 5, 'salman': 8, 'balls': 6, 'tabloid': 3, 'bowl': 6, 'intentionally': 2, 'icc': 3, 'resistant': 10, 'ringwald': 1, 'pascal': 4, 'correctly': 5, 'derived': 3, 'artemisia': 1, 'ineffective': 3, 'artemisian': 1, 'mefloquine': 1, 'medication': 12, 'borne': 6, 'firat': 1, 'hakurk': 1, 'strongholds': 17, 'warmongering': 1, 'arshad': 5, 'waheed': 5, 'grass': 9, 'oklahoma': 11, 'plymouth': 1, 'imitation': 2, 'ideology': 7, 'aleppo': 2, 'djalil': 1, 'sofyan': 2, 'edited': 2, 'ambiguous': 2, 'grabbed': 3, 'politkovskaya': 5, 'anna': 5, 'fireworks': 7, 'careless': 1, 'discarded': 1, 'gazeta': 1, 'novaya': 1, 'elevator': 3, 'trincomalee': 6, 'kadirgamar': 4, 'lakshman': 4, 'perry': 8, 'rick': 2, 'ramirez': 19, 'tunnels': 20, 'misunderstood': 2, 'eckhard': 3, 'fred': 5, 'mechanical': 5, 'martina': 7, 'professional': 21, 'hingis': 15, 'pattaya': 2, 'volvo': 1, 'homelessness': 2, 'crowns': 2, 'stocking': 3, 'allocating': 1, 'unrelated': 7, 'amendment': 28, 'paya': 1, 'lebar': 1, 'refuel': 1, 'occurs': 7, 'grozny': 5, 'atagi': 1, 'starye': 1, 'caucasus': 12, 'novye': 1, 'disinformation': 1, 'maskhadov': 10, 'discredit': 5, 'aslan': 4, 'consistently': 4, 'favored': 15, 'timeline': 3, 'timelines': 1, 'adamantly': 2, 'desecrated': 7, 'badakhshan': 3, 'faizabad': 2, 'unconfirmed': 4, 'avalanche': 8, 'kohistan': 2, 'exploring': 3, 'romanian': 20, 'bucharest': 6, 'sorin': 2, 'eduard': 3, 'ion': 3, 'ovidiu': 2, 'miscoci': 2, 'marie': 14, 'jeanne': 2, 'ohanesian': 2, 'monaf': 1, 'translator': 11, 'traian': 6, 'basescu': 9, 'collecting': 10, 'launcher': 8, 'banja': 2, 'dragan': 7, 'crnogorac': 2, 'luka': 2, 'atrocity': 3, 'purnomo': 1, 'yusgiantoro': 1, 'tapped': 3, 'rudd': 5, '770': 1, 'minustah': 2, 'plum': 1, 'marc': 6, '257': 3, 'undergoing': 13, 'shaikh': 4, 'nasir': 1, 'dhakla': 1, 'facilitating': 4, 'mansoor': 2, 'guam': 5, 'ayodhya': 2, 'convictions': 6, 'mammoth': 3, 'verdicts': 3, 'juster': 1, 'undersecretary': 19, 'nano': 4, 'biotechnology': 8, 'micro': 1, 'miniature': 1, 'atoms': 2, 'rocca': 1, 'christina': 1, 'instigating': 3, 'sermon': 4, 'jannati': 6, 'anatolian': 2, 'aumann': 3, 'schelling': 3, 'theory': 6, 'mundane': 1, 'mathematically': 2, 'choices': 6, 'runoffs': 2, 'federico': 4, 'lombardi': 4, 'poitou': 1, 'charentes': 1, 'salehi': 5, 'kazakhs': 1, 'respublika': 1, 'disparaging': 1, 'editor': 34, 'galina': 2, 'dyrdina': 1, 'lawsuits': 17, 'dogged': 2, 'firebombing': 2, 'incumbent': 15, 'nazarbayev': 9, 'nursultan': 5, 'spin': 18, 'boldak': 14, 'riding': 15, 'sumaidaie': 3, 'samir': 4, 'rabbi': 4, 'sabbath': 3, 'desecrating': 1, 'yosef': 2, 'shalom': 8, 'refraining': 1, 'electronics': 12, 'eliashiv': 1, 'violates': 9, 'ultra': 10, 'judaism': 4, 'noble': 3, 'uniforms': 14, 'aiding': 13, 'miranshah': 5, 'liter': 10, 'hearted': 4, 'poppy': 13, 'expensive': 14, 'lightly': 5, 'spokesmen': 8, 'hsann': 2, 'kyaw': 3, 'subsidizing': 2, 'varies': 3, 'muhammed': 3, 'sundown': 4, 'sunrise': 3, 'iftaar': 1, 'kabal': 1, 'everyday': 3, 'greedy': 2, 'hainan': 5, 'campus': 3, 'danzhou': 1, 'ripe': 1, 'pence': 5, 'oct': 4, '94': 12, 'plc': 3, '89': 10, '149': 3, '141': 5, 'reed': 4, '118': 1, 'packaging': 2, 'discontinued': 2, '388': 1, '133': 2, 'pretax': 2, '135': 11, 'expectations': 12, 'disposal': 6, 'ppp': 6, 'household': 10, 'demographic': 3, 'reorganize': 2, 'delphi': 4, 'fertility': 2, 'necessitate': 1, 'amounting': 1, 'transfers': 11, 'municipalities': 2, 'exceed': 10, 'chronically': 2, 'advances': 5, 'deepest': 2, 'projection': 1, 'upswing': 6, 'rebounding': 4, 'attributable': 3, 'bundesbank': 1, 'parent': 6, 'likewise': 3, '2016': 3, 'annum': 1, '994': 1, 'olav': 1, 'christianity': 11, 'adoption': 11, 'viking': 3, 'tryggvason': 1, 'tapered': 1, '1397': 1, '1814': 4, 'cession': 1, 'norwegians': 3, '1905': 4, 'nationalism': 3, 'neutral': 9, '1940': 6, 'nonetheless': 5, 'outset': 1, 'miller': 17, 'adjacent': 8, 'referenda': 4, 'preserving': 9, 'exiles': 12, 'rpf': 3, 'approximately': 23, 'exacerbated': 8, 'upheavals': 2, 'orchestrated': 6, 'culminating': 2, 'rwandans': 1, 'retribution': 2, 'zaire': 2, 'retaking': 1, 'bent': 4, 'rout': 2, 'kigali': 4, 'andorra': 7, 'comparative': 1, 'sheep': 10, 'consist': 3, 'perfumes': 2, 'monkeys': 2, 'apt': 2, 'courtiers': 1, 'pupils': 1, 'mimics': 1, 'arrayed': 1, 'danced': 3, 'mischief': 1, 'courtier': 1, 'till': 2, 'spectacle': 3, 'pocket': 5, 'nuts': 3, 'actors': 7, 'dancing': 6, 'forgot': 2, 'robes': 2, 'tearing': 5, 'laughter': 3, 'amidst': 1, 'huntsman': 2, 'dogs': 9, 'basket': 2, 'longing': 1, 'wished': 5, 'owner': 22, 'enjoy': 18, 'abstain': 2, 'honest': 11, 'thief': 5, 'plunder': 1, 'merely': 6, 'inaction': 2, 'dragging': 2, 'wiled': 1, 'edwards': 4, 'frontrunner': 4, 'lashed': 11, 'raffaele': 5, 'checked': 10, 'fluid': 2, 'scar': 1, 'cavity': 1, 'tension': 22, 'flared': 8, 'claudia': 2, 'annyaso': 1, 'complication': 2, 'populous': 11, 'onulak': 1, 'passionate': 1, 'ted': 2, 'jazz': 7, 'blues': 12, 'charlie': 1, 'influences': 1, 'greats': 2, 'parker': 2, 'hooker': 2, 'inspiration': 4, 'eyesight': 2, 'monsoon': 22, 'unleashed': 3, 'mud': 11, 'chittagong': 4, 'hillside': 1, 'thunderstorms': 1, 'lightning': 9, 'quadruple': 4, 'surgeons': 2, 'drain': 4, 'lasts': 2, 'taji': 3, 'qada': 1, 'flashpoint': 2, 'golf': 2, 'eastbourne': 1, 'douchevina': 3, 'clijsters': 8, 'vera': 2, 'tournaments': 3, 'wells': 10, 'amelie': 2, 'junior': 15, 'mauresmo': 3, 'strapping': 1, 'breath': 4, 'shortness': 1, 'impunity': 5, 'nowak': 6, 'highlight': 11, 'tennessee': 6, 'accomplishments': 1, 'smoky': 1, 'stewardship': 2, 'recommit': 1, 'cleaner': 5, 'engines': 9, 'acres': 1, 'ozone': 1, 'chide': 1, 'sidestep': 1, 'migrating': 8, 'variant': 4, 'h5n2': 5, 'milder': 3, 'flamingo': 3, 'persecution': 12, 'hector': 1, 'admiral': 20, 'carmona': 5, 'robberies': 1, 'vault': 6, 'dealership': 2, 'belo': 1, 'horizonte': 1, 'fraction': 7, 'phony': 2, 'daring': 2, 'landscaping': 1, 'fortaleza': 1, 'thick': 6, 'virulent': 3, 'readings': 6, 'ituri': 9, 'stationed': 19, 'sein': 6, 'ward': 8, 'grip': 8, 'strengthens': 3, 'bunker': 6, 'dump': 14, 'flock': 14, 'liaoning': 8, 'reformers': 4, 'societies': 5, 'ambition': 3, '944': 1, 'bamboo': 1, 'pipes': 3, 'unlawful': 7, 'hyung': 2, 'kyu': 1, 'araujo': 2, 'interfered': 1, 'maltreating': 2, '13th': 15, 'rampaging': 2, 'aflame': 1, '204': 1, 'sur': 3, 'permitting': 1, 'amiens': 1, 'savigny': 1, 'orge': 1, 'lyon': 8, 'firebomb': 1, 'younger': 18, 'erupt': 3, 'turns': 8, 'seclusion': 2, 'appearances': 9, 'zamoanga': 1, 'opposite': 3, 'liquified': 1, 'hauling': 3, 'compressed': 1, 'g8': 18, 'completing': 11, 'obligation': 4, 'comedy': 9, 'bernie': 4, 'mac': 3, 'letterman': 1, 'comic': 5, 'mccullogh': 1, 'drama': 3, 'grinning': 2, 'leash': 3, 'ibn': 1, 'disagree': 4, '64th': 1, 'pearl': 10, 'battleship': 1, 'sunk': 2, 'guests': 8, 'fleet': 23, 'waver': 1, 'argued': 23, 'ringleader': 5, 'compliant': 2, 'karrada': 3, 'mess': 1, 'greed': 3, 'renewable': 10, 'hazem': 3, 'shaalan': 3, 'appealing': 19, 'diverse': 11, 'respirator': 2, 'antiviral': 1, 'broad': 30, 'fourteen': 5, 'inhumane': 2, 'afterwards': 12, 'killers': 4, 'bayaman': 2, 'erkinbayev': 6, 'belongs': 8, 'kara': 2, 'rift': 6, 'rodrigo': 9, 'granda': 6, 'bounty': 11, 'hunters': 15, 'froze': 6, 'colombians': 3, 'dinosaurs': 3, 'fossils': 1, 'titanosaurs': 4, 'brisbane': 5, 'queensland': 2, 'fossilized': 1, 'bones': 3, 'ranchers': 2, 'nicknamed': 2, 'cooper': 2, 'eromanga': 1, 'discoveries': 7, 'weighs': 1, 'necks': 2, 'roam': 1, 'tails': 3, 'prehistoric': 1, 'sauropods': 1, 'sajida': 1, 'belt': 13, 'rishawi': 2, 'detonate': 10, 'absentia': 10, 'jordanians': 4, 'appearing': 11, 'bolton': 21, 'fielding': 3, 'bluntly': 2, 'possessed': 2, 'assertion': 8, 'dodd': 4, 'dreadfully': 1, 'departed': 4, 'mozambican': 2, 'rajasthan': 3, 'receding': 4, 'barmer': 1, 'rubber': 12, 'euphrates': 10, 'anchorwoman': 1, 'carries': 12, 'unearthed': 6, 'anjar': 2, 'uniform': 13, 'guerrero': 3, 'lahore': 21, 'amritsar': 3, 'wagah': 2, 'problematic': 3, 'chilpancingo': 1, 'instructions': 4, 'kurzban': 2, 'ira': 12, 'ploy': 3, 'desperately': 3, 'depleting': 2, 'submerged': 8, 'airlifted': 4, 'pleading': 4, 'bags': 6, 'sick': 23, 'overwhelmed': 5, 'desperate': 10, 'sos': 1, 'envoys': 15, 'rid': 9, 'podium': 3, 'dissatisified': 1, 'contend': 4, 'ferrer': 3, 'spots': 10, 'atp': 3, 'roddick': 17, 'berdych': 2, 'swede': 4, 'soderling': 1, 'robin': 4, 'novak': 2, 'djokovic': 2, 'finalist': 1, 'finale': 1, 'gael': 1, 'verdasco': 2, 'monfils': 1, 'nikolay': 2, 'argentine': 23, 'davydenko': 2, 'potro': 1, 'edition': 15, 'assisting': 10, 'photography': 1, 'jovicevic': 1, 'pale': 3, 'disrupting': 19, 'gaining': 18, 'branislav': 2, 'warplane': 2, 'cartosat': 2, 'hamsat': 2, 'sriharokota': 2, 'bengal': 18, 'pslav': 2, 'spaceport': 1, 'indigenous': 27, 'blasted': 5, 'precise': 1, 'heavier': 1, 'frequencies': 4, '5400': 1, 'homicides': 1, 'physically': 7, 'heightened': 14, '87': 14, 'dealers': 6, 'escalation': 8, 'badri': 1, 'servicemen': 9, 'askari': 2, 'priorities': 11, 'silencers': 1, 'izmir': 7, 'neftaly': 1, 'platero': 2, 'synagogues': 2, 'deceptive': 1, 'advertise': 2, 'tactics': 13, 'tar': 1, 'manufacturers': 6, 'labeling': 1, 'aspect': 1, 'advertising': 8, 'philip': 6, 'altria': 1, '195': 3, 'louis': 13, 'tsunamis': 4, 'droughts': 5, 'weary': 2, 'comoros': 5, 'barry': 8, 'verbal': 5, 'altercation': 2, 'funneled': 1, 'slight': 14, 'courthouse': 5, 'counterterrorist': 1, 'mansur': 2, 'wali': 13, 'heather': 6, 'mills': 6, 'vows': 3, 'mccartney': 5, 'stars': 12, 'limb': 1, 'artificial': 4, 'contestant': 1, 'slipping': 4, 'prosthesis': 1, 'sleeve': 1, 'viva': 1, 'fee': 10, 'rated': 4, 'curtains': 1, 'panicking': 2, 'candles': 5, 'performers': 6, 'underfoot': 1, 'mainstream': 10, 'roll': 10, 'kennedy': 30, 'ibom': 1, 'akwa': 1, 'indonesians': 5, 'afren': 1, 'steal': 8, 'fairer': 2, '3600': 1, 'gradually': 19, 'postwar': 3, 'publish': 10, 'examination': 6, 'deepening': 2, 'miran': 5, 'mukhtar': 1, 'resorted': 2, 'stood': 14, 'wiping': 4, 'adversely': 3, 'swaziland': 10, 'kadhimiya': 3, 'ambush': 45, 'yilmaz': 1, 'isik': 1, 'resit': 1, 'mehmet': 7, 'hawija': 1, 'turkmens': 2, 'knowledge': 16, 'confidential': 10, 'discussion': 16, 'pumping': 7, 'aquifer': 3, 'scarcity': 1, 'moral': 10, 'drawing': 12, 'condolezza': 1, 'steepest': 2, 'depress': 2, 'partisan': 4, 'cbo': 1, 'threefold': 1, 'ryazanov': 2, 'pays': 4, '230': 4, 'periodic': 5, 'airlift': 4, 'presents': 13, 'accurate': 8, 'nabil': 4, 'kerman': 1, 'jolted': 4, 'negar': 1, 'torbat': 1, 'heydariyeh': 1, 'fault': 7, 'seismic': 3, 'bam': 4, 'ancient': 22, 'lindsay': 11, 'utah': 6, 'lohan': 9, 'lodge': 8, 'cirque': 5, 'sundance': 2, 'alcohol': 12, 'wonderland': 1, 'rehab': 1, 'chrysler': 8, '570': 1, 'statehood': 7, 'ca': 5, 'monica': 2, 'dui': 1, 'coretta': 3, 'clinic': 14, 'baja': 5, 'diego': 7, 'rays': 2, 'surgeries': 2, 'unconventional': 1, 'levy': 8, 'mwanawasa': 14, 'undergone': 5, 'ovarian': 3, 'baseless': 8, 'starvation': 9, 'pyinmana': 2, 'zambian': 9, 'rupiah': 5, 'banda': 9, 'bunkers': 3, 'rugged': 12, 'fallback': 1, 'defensible': 1, 'belgrade': 24, 'draskovic': 2, 'jessen': 3, 'soren': 2, 'vuk': 2, 'kostunica': 3, 'vojislav': 5, 'administering': 4, 'nawaz': 12, 'excluded': 4, 'detail': 7, 'khair': 1, 'gereshk': 2, 'shuja': 1, 'malian': 1, 'hamma': 3, 'mauritanian': 8, 'sid': 2, 'ould': 12, 'maghreb': 6, 'nouakchott': 4, 'nouadhibou': 1, 'dsm': 3, '113': 6, '144': 4, '235': 1, 'guilders': 5, 'outlay': 2, 'barthelemy': 3, 'villas': 1, 'inhibits': 1, 'attracts': 3, 'ondimba': 1, 'hadj': 1, 'structures': 7, 'abundant': 3, 'nonpermanent': 6, 'prix': 3, 'stonemason': 1, 'marinus': 1, '301': 1, '1975': 18, 'prolonged': 11, 'dependence': 20, 'emigration': 5, 'frelimo': 3, 'marxism': 1, 'renamo': 1, 'chissano': 1, 'delicate': 3, 'joaquim': 1, 'emilio': 1, 'guebuza': 2, 'armando': 3, 'silverstone': 1, 'disqualification': 2, 'questionable': 3, 'defaulted': 4, 'dollarization': 1, 'discouraged': 2, 'terminate': 2, 'remittance': 3, 'flows': 9, 'ecuadorian': 5, 'lethal': 18, 'uphill': 1, 'domenech': 3, 'startup': 1, 'ceremonial': 8, 'saltillo': 1, 'respecting': 3, 'maintenance': 16, 'navigation': 4, 'tire': 5, 'cameroonian': 1, 'protester': 6, 'kilted': 1, 'playlists': 1, 'staple': 5, 'scandals': 18, 'awori': 2, 'mwiraria': 1, 'prevalent': 3, 'manifesto': 3, 'giuliana': 2, 'sgrena': 4, 'susilo': 8, 'yudhoyono': 12, 'bambang': 9, 'blockaded': 2, 'conserve': 2, 'necessarily': 2, 'unveiling': 1, 'bellamy': 1, 'desperation': 3, 'initiatives': 16, 'laments': 2, 'baixinglou': 1, 'engulfed': 4, 'chaoyang': 1, 'waiters': 1, 'diners': 1, 'improper': 5, 'stove': 2, 'flexible': 3, 'nath': 4, 'backtrack': 1, 'poorer': 5, 'mosques': 14, 'onitsha': 2, 'anambra': 2, 'hausas': 1, 'scuffle': 2, 'ibos': 1, 'sparks': 2, 'reprisals': 3, 'asadabad': 5, 'overshadowed': 2, 'dc': 3, 'truckers': 4, 'repercussions': 2, 'anibal': 1, 'cavaco': 3, 'cap': 3, 'repression': 16, 'caroni': 1, 'correo': 1, 'persecuting': 2, 'styled': 1, 'openness': 6, 'protectionism': 3, 'balanced': 5, 'muhajer': 3, 'landmarks': 3, 'hamza': 7, 'neighborhoods': 11, 'lendu': 5, 'deploring': 1, 'socialists': 3, 'calmer': 2, 'ride': 4, 'jela': 1, 'franceschi': 1, 'transforming': 4, 'balkans': 10, 'succumb': 2, 'hygiene': 3, 'hampton': 1, 'grouping': 8, 'stalemate': 13, 'informal': 16, 'nenets': 1, 'barinov': 5, 'alexi': 1, 'lukoil': 4, 'shahristani': 2, 'hussain': 9, 'perhaps': 9, 'timeframe': 2, 'premature': 3, 'recruit': 8, 'cautioned': 12, 'extends': 9, 'attorneys': 12, 'whites': 2, 'selection': 11, 'molesting': 2, 'neverland': 4, 'acquittal': 1, 'mannar': 2, '67': 16, 'chennai': 3, 'moya': 8, 'ljubicic': 6, 'squandered': 2, 'tiebreaker': 2, 'vliegen': 2, 'kristof': 1, 'seymour': 2, 'hersh': 5, 'rallying': 3, 'scare': 7, 'memo': 10, 'individual': 21, 'electrocution': 2, 'drowning': 2, 'collapsing': 3, 'downed': 8, 'downpour': 1, 'drainage': 3, 'kenteris': 3, 'konstantinos': 1, 'sprinters': 2, 'thanou': 3, 'render': 2, 'lausanne': 4, 'provisionally': 2, 'athletics': 4, 'iaaf': 1, 'accorded': 4, 'citizenship': 35, 'jeep': 4, 'dodge': 1, 'pentastar': 1, 'governance': 14, 'multimillion': 2, 'systemic': 3, 'arap': 3, 'moi': 5, 'amiri': 1, 'taha': 6, 'shiite': 1, 'clogged': 4, 'retract': 1, 'elias': 4, 'napoleon': 2, 'gomez': 3, 'safwat': 1, 'mena': 4, 'gallbladder': 3, 'gamal': 7, 'grooming': 2, 'succession': 17, 'olsson': 4, 'hamstring': 1, 'dn': 2, 'athletic': 1, 'marian': 1, 'oprea': 1, 'dmitrij': 1, 'valukevic': 1, 'chandrika': 6, 'kumaratunga': 9, 'televisions': 1, 'flocked': 1, 'ethnicity': 2, 'regard': 9, 'staggering': 1, 'deadlocked': 10, 'overture': 1, 'difficulty': 10, 'wreck': 4, 'stall': 5, 'likud': 29, 'scuttle': 1, 'defied': 10, 'shinui': 3, 'knesset': 5, 'karami': 9, 'derail': 17, 'brazzaville': 4, 'grounded': 10, 'dimension': 1, 'unimaginable': 3, 'pedophiles': 2, 'priesthood': 1, 'halls': 2, 'powered': 7, 'accountability': 6, 'hierarchy': 1, 'swiftly': 1, 'errant': 3, 'fastest': 14, 'kyoto': 17, 'fisheries': 5, 'sells': 8, 'busan': 3, 'assurances': 12, 'koreas': 23, 'gutierrez': 12, 'lucio': 3, 'examined': 10, 'infiltrators': 2, '950': 2, 'sandy': 3, 'pilings': 1, 'cement': 5, 'floating': 6, 'swimming': 3, 'pulls': 6, '2900': 1, 'ninewa': 1, 'deployments': 4, 'straining': 2, 'truckloads': 2, 'jeered': 1, 'baez': 1, 'joan': 1, 'folk': 4, 'casey': 4, 'vigils': 1, 'pave': 6, 'incumbents': 1, 'suleiman': 7, 'forging': 6, 'motorist': 3, 'tayyeb': 1, 'damiri': 1, 'posing': 9, 'greenback': 3, 'upward': 8, 'qawasmi': 3, 'ayoub': 1, 'sweeps': 4, 'fewest': 1, 'gordon': 16, 'outlined': 9, 'accountable': 9, 'fashion': 13, 'shopping': 23, 'weakest': 2, 'retail': 21, 'lure': 3, 'shoppers': 4, 'erez': 4, 'infiltrations': 3, 'cfco': 1, 'pointe': 1, 'noire': 1, 'baluchistan': 46, 'ghum': 1, 'aab': 1, 'baluchis': 2, 'waving': 9, 'fnj': 1, 'subdue': 4, 'worsens': 1, 'mendez': 2, 'pits': 6, 'zuma': 20, 'verdict': 19, 'jacob': 6, 'soweto': 2, 'apartheid': 19, 'consensual': 4, 'drawn': 23, 'incidence': 3, 'sweeping': 9, '423': 2, 'constitutes': 2, 'defines': 4, 'outlines': 5, 'invite': 4, 'dhabi': 1, 'uae': 5, 'attiya': 2, 'trusts': 1, 'painkiller': 1, 'vioxx': 6, 'liable': 3, 'diploma': 1, 'migrated': 2, 'debates': 4, 'haggling': 1, 'immigrate': 1, 'tentatively': 3, 'builds': 2, 'unveils': 1, 'ford': 21, 'carmakers': 1, 'toyota': 10, 'sinai': 16, 'badran': 1, 'wheel': 1, 'suv': 1, 'explorer': 3, 'lutfullah': 2, 'mashal': 2, 'berlusconi': 16, 'silvio': 7, 'fatwa': 4, 'zoo': 9, 'panda': 9, 'newborn': 2, 'cub': 8, 'crushed': 9, 'yinghua': 2, 'zookeepers': 1, 'incubator': 1, 'cubs': 2, 'nurse': 1, 'jingguo': 1, 'rolled': 6, 'pandas': 8, 'captivity': 16, 'gomoa': 1, 'buduburam': 1, 'resettle': 5, 'rabiah': 1, 'authenticate': 2, 'cousins': 1, 'taba': 4, 'core': 13, '1928': 4, 'catalina': 1, 'lie': 9, 'perpetuate': 1, 'urine': 4, 'beatings': 6, 'shocks': 7, 'ingestion': 1, 'algerians': 2, 'forcibly': 8, 'bouteflika': 5, 'abdelaziz': 7, 'enable': 4, 'khalaf': 6, 'facilitation': 2, 'levey': 3, 'cornerstone': 3, 'excludes': 1, 'undercut': 4, 'fallout': 3, 'bedouin': 4, 'daytime': 2, 'umm': 3, 'haiman': 1, 'fugitives': 7, 'netzarim': 1, 'evacuating': 6, 'morag': 1, 'stir': 4, 'ateret': 1, 'unamid': 1, 'frightened': 4, 'neglecting': 2, 'kellenberger': 3, 'jakob': 4, 'listens': 1, 'jurists': 1, 'dadis': 2, 'camara': 5, 'vowing': 8, 'arbitrarily': 1, 'fayssal': 1, 'erecting': 2, 'mekdad': 1, 'rauf': 7, 'shields': 8, 'knock': 2, 'throwers': 3, 'thrown': 12, 'veracity': 1, 'tikriti': 1, 'disc': 1, 'perjury': 3, 'contradictory': 3, 'celebratory': 2, 'xinjiang': 12, 'turpan': 2, 'distributed': 10, '690': 1, 'catastrophic': 8, '551': 1, 'fema': 11, 'hadley': 8, 'moustapha': 7, 'atta': 4, 'balkh': 1, 'teamed': 4, 'mihtarlam': 1, 'openings': 1, 'offshoot': 2, 'mistaken': 7, 'translation': 4, 'notice': 17, 'stringers': 1, 'occasionally': 7, 'elco': 2, 'fasteners': 1, 'rockford': 1, '155': 4, 'pressures': 6, 'outweighs': 1, 'denominated': 3, 'creditor': 1, 'onset': 3, 'dried': 3, 'dwindled': 1, 'appreciation': 5, 'dilma': 1, 'rousseff': 1, '1829': 2, 'protracted': 2, 'abolished': 6, '1981': 15, 'ec': 3, 'prospect': 5, 'voluntarily': 11, 'emu': 3, 'default': 3, 'fishermen': 18, 'confine': 1, 'tokelau': 4, 'recurrent': 6, 'copra': 5, 'postage': 7, 'coins': 5, 'souvenir': 1, 'handicrafts': 7, 'remitted': 1, 'khurasan': 1, 'medieval': 5, 'merv': 1, '1885': 3, '1865': 5, 'ussr': 12, 'underdeveloped': 4, 'extraction': 7, 'hydrocarbon': 2, 'boon': 1, 'plaintiff': 1, 'actively': 7, 'nyyazow': 2, 'saparmurat': 2, 'gurbanguly': 1, 'cook': 10, 'fruit': 6, 'pearls': 2, 'emigrants': 5, 'accumulating': 1, 'bloated': 3, 'rekindled': 2, 'encouragement': 4, 'peking': 1, 'tongue': 2, 'heathens': 1, 'translate': 1, 'editorial': 7, 'devils': 1, 'dwellings': 4, 'pang': 1, 'incensed': 1, 'mongolian': 3, 'barbarity': 1, 'wager': 1, 'reader': 5, 'dug': 3, 'sown': 3, 'thistles': 2, 'organist': 1, 'pews': 1, 'janitor': 1, 'squeezed': 1, 'barber': 3, 'kari': 2, 'conakry': 1, 'reveals': 2, "o'keefe": 1, 'celestial': 1, 'astronomical': 1, 'incredibly': 1, 'columbia': 8, 'grounding': 5, 'overrule': 1, 'simeus': 3, 'dumarsais': 2, 'arlington': 4, 'cemetery': 8, 'debalk': 1, 'harmless': 3, 'newcastle': 1, 'fowl': 6, 'mulgueta': 1, 'pigeons': 5, 'watching': 9, 'migratory': 12, 'aden': 21, '137': 4, 'drownings': 1, '134': 2, 'wreath': 6, 'unknowns': 1, 'homelands': 4, 'escaping': 4, 'ruthless': 4, 'doves': 1, 'narathiwat': 9, 'origami': 1, 'folded': 1, 'symbolizing': 2, 'thais': 2, 'andean': 12, 'occassion': 1, 'picnics': 1, 'cemeteries': 1, 'disadvantage': 2, 'pacts': 2, 'cheaper': 12, 'looms': 2, 'banning': 12, 'xian': 1, 'han': 1, 'archaeologists': 9, 'peugeot': 3, 'wuhan': 1, 'citroen': 2, 'dongfeng': 2, 'presided': 6, 'yakaghund': 1, 'mohmand': 10, 'flattened': 5, 'wheelchairs': 1, 'lawless': 8, 'extricate': 1, 'ashti': 1, 'bilingual': 1, 'adjourn': 3, 'recess': 8, 'stab': 5, 'protestant': 9, 'missionaries': 8, 'necdet': 4, 'sezer': 4, 'ahmet': 5, 'justification': 5, 'anhui': 3, 'dangtu': 1, 'produces': 19, 'uniting': 2, 'perpetrators': 10, 'cowardly': 3, 'promptly': 6, 'curling': 3, 'swedes': 4, 'identical': 1, 'landslides': 16, 'strips': 2, 'farmland': 5, 'photojournalist': 5, 'parisi': 1, 'stampa': 1, 'arturo': 3, 'pelosi': 9, 'trajectory': 1, 'setbacks': 3, 'miliant': 1, 'crumpton': 2, '324': 2, 'iskandariya': 1, 'sulaymaniyeh': 1, 'recruits': 14, 'graduated': 1, 'courses': 8, 'emerli': 1, 'maref': 1, 'consuming': 4, 'mirpur': 1, 'sacramento': 1, 'cool': 7, 'scurrying': 1, 'conditioners': 1, 'grids': 3, 'mahmood': 1, 'holbrooke': 3, 'reviews': 5, 'paired': 2, 'developmentaly': 1, 'ganji': 6, 'massoud': 8, 'moghaddasi': 1, 'articles': 16, 'negroponte': 19, '640': 1, 'projections': 4, 'stockpiled': 1, 'relocating': 2, 'relocation': 3, 'donor': 36, 'michaelle': 1, 'youngest': 10, 'adrienne': 1, 'clarkson': 8, 'quebec': 3, 'dictatorial': 2, 'immigrated': 2, 'dominion': 3, '1867': 4, 'beliefs': 5, 'turnover': 1, 'respondents': 11, 'referendums': 3, 'rejections': 2, 'unanimous': 6, 'commonly': 5, 'awacs': 1, 'acquiring': 5, 'riga': 2, 'latvian': 5, 'margraten': 1, 'flies': 4, 'kathleen': 4, 'blanco': 6, 'cameron': 7, 'erased': 1, 'vermilion': 1, 'tidal': 6, 'wrap': 3, 'nagin': 13, 'algiers': 10, 'kohat': 1, 'tirah': 2, 'raymond': 5, 'azar': 2, 'mizuki': 1, 'thigh': 5, 'marathon': 6, 'noguchi': 4, 'tomiaki': 1, 'fukuda': 6, 'marathoner': 1, 'fracture': 2, 'radcliffe': 1, 'paula': 6, 'holder': 6, 'liaison': 3, 'lancaster': 1, 'surveys': 9, 'tragic': 3, 'hanegbi': 1, 'tzachi': 1, 'parcel': 3, 'explode': 7, 'defused': 4, 'packages': 9, 'anarchist': 1, 'stranding': 3, 'kaesong': 1, 'bride': 2, 'sting': 2, 'switched': 2, 'routinely': 13, 'drills': 9, 'vitaly': 2, 'churkin': 2, 'identifying': 5, 'roberto': 7, 'tarongoy': 3, 'hallums': 5, 'roy': 7, 'tattoo': 2, 'bugle': 1, 'drum': 1, 'dating': 9, 'pageant': 1, 'twilight': 1, 'storied': 2, 'jeff': 3, 'feuer': 1, 'entrapment': 1, 'bordered': 2, 'shipyard': 5, 'gdansk': 3, 'sustainability': 5, 'hefty': 2, 'streamline': 4, 'walesa': 2, '27th': 6, 'speedy': 2, 'mansour': 3, 'affluent': 3, 'fake': 13, 'undercover': 11, 'khamenei': 23, 'tribe': 7, 'nida': 2, 'rampant': 6, 'fairness': 4, 'destabilized': 3, 'adventure': 3, 'plaza': 1, 'encircled': 1, 'revava': 1, 'hilltop': 3, 'expecting': 3, 'brent': 9, 'demarcating': 1, 'tarasyuk': 5, 'stagnant': 3, 'abyei': 1, 'kashmirs': 1, 'khanna': 6, 'ravi': 6, 'saffir': 1, 'upgraded': 7, 'simpson': 2, 'sometime': 7, 'sits': 9, 'quitting': 4, 'monarch': 12, 'moldova': 12, 'disney': 6, 'premiere': 4, 'viewership': 1, 'doubling': 5, 'tisdale': 1, 'spawning': 1, 'efron': 1, 'zac': 1, 'musicalphenomenon': 1, 'ashley': 2, 'patriot': 11, 'defeatism': 1, 'justified': 11, 'facts': 5, '80s': 3, 'sasae': 3, 'kenichiro': 3, 'stalinist': 2, 'convincing': 4, 'kharazzi': 2, 'nasser': 12, 'defiance': 6, 'mockery': 1, 'kocharian': 2, 'characterizing': 2, 'chased': 12, 'agreeing': 4, 'sacrificed': 3, 'detecting': 3, 'domingo': 4, '145': 5, 'higüey': 1, 'santo': 6, 'faxas': 1, '216': 2, 'quand': 1, 'quoc': 1, 'nguyen': 4, 'hoa': 2, 'thanh': 2, 'resurfaced': 4, '248': 3, 'shamil': 7, 'basayev': 14, 'nalchik': 2, 'kabardino': 1, 'balkaria': 1, 'exploiters': 1, 'laos': 16, 'reliable': 2, 'pornography': 4, 'exponential': 1, 'maulvi': 2, 'tehrik': 1, 'appropriately': 4, 'hadithah': 1, 'blitz': 1, 'natonski': 1, 'accords': 32, 'vassilakis': 1, 'adamantios': 1, 'recommitted': 1, 'decentralized': 2, 'compensated': 6, 'menatep': 4, 'plummeted': 3, 'incurred': 3, 'skeptics': 1, 'kajaki': 3, 'harvests': 3, 'illicit': 5, 'overshot': 4, 'nbc': 6, 'bremer': 5, 'correspondents': 3, 'hatta': 1, 'radjasa': 1, 'microsystems': 1, 'dominance': 8, '228': 3, 'beitar': 1, 'efrat': 1, 'illit': 1, 'resilience': 2, 'courage': 10, 'shoulder': 11, 'eduardo': 8, 'gonzalo': 2, 'antezana': 1, 'marcelo': 1, 'disposing': 1, 'lights': 10, 'nonessential': 2, 'dimming': 2, 'cheated': 1, 'riches': 2, 'pumped': 4, 'widow': 11, 'user': 1, 'pyramids': 2, 'castle': 1, 'smithsonian': 5, 'antarctic': 20, 'fist': 3, 'cube': 2, 'bachelet': 16, 'candlelight': 2, 'saved': 9, 'sleet': 1, 'bout': 1, 'decimate': 1, 'wholesale': 4, 'marergur': 1, 'dhusamareb': 1, 'ahlu': 3, 'waljama': 1, 'strewn': 1, 'hizbul': 4, 'promoted': 12, 'jeny': 1, 'figueredo': 4, 'frias': 1, 'mccormack': 18, 'attachés': 1, 'operatives': 9, 'maarib': 2, 'sabotaged': 4, 'tribesman': 3, 'proceeds': 9, 'evangelicals': 1, 'apprehend': 1, 'aqili': 2, 'presentation': 4, 'actual': 12, 'referral': 14, 'intend': 8, 'chamber': 14, 'mainz': 1, 'oshkosh': 2, 'wis': 1, '352': 3, 'motor': 10, 'softer': 4, 'chassis': 3, 'phasing': 2, 'midsized': 2, 'deere': 1, 'inhabitants': 11, 'muscat': 2, 'sultanate': 5, 'qaboos': 2, '1970': 8, 'restrictive': 7, 'hockey': 4, 'uprisings': 5, 'omanis': 1, 'marches': 11, 'reshuffled': 2, 'acquired': 12, '1946': 4, 'reestablished': 4, "ba'th": 1, 'sect': 7, 'alawite': 1, 'hafiz': 1, 'iginla': 1, 'shane': 4, 'heatley': 1, 'jarome': 1, 'doan': 1, 'dany': 1, 'ostensible': 2, 'hizballah': 2, 'antigovernment': 2, "da'ra": 1, 'legalization': 1, 'repeal': 11, 'quelling': 1, 'sedin': 1, 'henrik': 1, 'tjarnqvist': 1, 'remarkably': 3, 'trafficked': 3, 'hemispheres': 1, 'sands': 1, 'aragonite': 1, 'dredging': 2, 'warden': 5, 'locks': 2, 'mechanic': 1, 'imprudent': 1, 'imprudence': 1, 'vicissitudes': 1, 'wonder': 2, 'thoughtful': 1, 'fortune': 4, 'ox': 8, 'nap': 1, 'cosily': 1, 'bite': 3, 'rage': 5, 'slumber': 1, 'barked': 1, 'awakened': 3, 'muttering': 1, 'grudge': 2, 'ah': 4, 'distant': 10, 'quite': 13, 'astronomers': 7, 'ross': 3, 'elementary': 5, 'betsy': 1, 'qingdao': 4, 'gallop': 1, 'taught': 10, 'techniques': 10, 'sirte': 3, 'shandong': 5, 'jinan': 1, 'trillion': 18, 'pillar': 1, 'liberal': 19, 'kaplan': 2, 'metin': 2, 'caliph': 1, 'cologne': 3, 'mausoleum': 3, 'ataturk': 2, 'kemal': 4, 'caliphate': 1, 'lobbies': 1, 'lies': 12, 'farshad': 1, 'jamshidi': 1, 'ghorbanpour': 3, 'alireza': 1, 'soheil': 1, 'scout': 1, 'transmit': 5, 'masood': 3, 'bastani': 1, 'cyber': 7, 'basilan': 2, 'guarded': 10, 'disguised': 8, 'ghalawiya': 1, 'sleeper': 1, 'isselmou': 1, 'violators': 6, 'liberate': 1, 'cancellations': 4, "o'hare": 1, 'visible': 4, 'precipitation': 2, 'exception': 5, 'fared': 4, 'reinforce': 3, 'quito': 9, 'amazon': 5, 'petroecuador': 4, 'sucumbios': 3, 'zionist': 2, 'lago': 1, 'agrio': 1, 'lucky': 2, 'turkeys': 7, 'alternate': 10, 'marshals': 2, 'disneyland': 4, 'roasted': 2, 'valiant': 1, 'nicole': 2, 'true': 18, 'orinoco': 3, 'basin': 4, 'recommendation': 15, 'mitofsky': 2, 'obrador': 16, 'prd': 1, 'pan': 9, 'madrazo': 5, 'institutional': 4, 'pri': 4, 'goodluck': 3, 'umaru': 2, "yar'adua": 7, 'ailment': 4, 'notify': 3, 'notification': 3, 'electing': 4, '98': 10, '598': 1, 'edged': 2, 'gores': 1, 'conclusions': 4, 'airbase': 7, 'downing': 6, 'medellin': 2, 'merida': 3, '780': 3, '673': 1, 'unpunished': 3, 'melchior': 1, 'assassinating': 2, 'ndadaye': 1, 'ramadhani': 1, 'kalenga': 1, 'masterminds': 4, 'collected': 15, 'analyzed': 1, 'precursor': 3, 'punk': 2, 'telephoning': 1, 'mest': 2, 'anthony': 9, 'defunct': 5, 'lovato': 4, 'wayne': 5, 'matt': 2, 'disbanding': 2, 'label': 2, 'albums': 6, 'maverick': 1, 'needy': 5, 'blumenthal': 2, 'citgo': 4, 'discount': 6, 'embarrass': 1, 'nabaie': 1, 'shepherd': 12, 'unused': 5, 'soar': 5, 'inspects': 1, '169': 4, 'hafun': 3, 'desolation': 1, 'currents': 1, 'swimmers': 2, 'yekhanurov': 14, 'yuriy': 5, 'plachkov': 6, 'interviewer': 5, 'sawyer': 1, 'dianne': 1, 'barcelona': 10, 'shorter': 5, '956': 2, 'dune': 1, 'sand': 3, 'granada': 3, 'sainct': 1, 'wear': 8, 'rider': 2, 'swans': 12, 'danube': 3, 'veterinary': 18, 'thessaloniki': 1, 'tenth': 3, 'publishes': 4, 'duck': 6, 'kyprianou': 1, 'markos': 2, 'kongsak': 2, 'wanthana': 1, 'interested': 10, 'yala': 10, 'pattani': 10, 'gibraltar': 9, 'wu': 6, 'bangguo': 2, 'buying': 21, 'noriega': 4, 'populist': 3, 'banners': 11, 'mebki': 2, 'bouake': 2, 'revision': 6, 'ivorians': 2, 'contestants': 2, 'jared': 1, 'sloan': 1, 'jason': 5, 'cotter': 1, 'eliminating': 13, 'messages': 31, 'vie': 1, 'mitterndorf': 1, 'jumper': 1, 'ljoekelsoey': 2, 'roar': 3, '207': 1, 'faultless': 1, 'jumps': 2, '788': 2, 'morgenstern': 1, '762': 1, 'widhoelzl': 2, '752': 1, 'planica': 1, 'overpayments': 1, 'fees': 17, 'gaming': 9, 'unlocked': 1, 'footlocker': 1, 'gambled': 1, 'neutralized': 4, 'respectively': 5, 'parked': 15, 'gen': 8, 'barno': 5, 'lt': 7, 'kagame': 14, 'evaluating': 3, 'gunboats': 2, 'baylesa': 1, 'boyfriend': 3, 'joel': 4, 'charlotte': 2, 'madden': 2, 'ruz': 7, 'halfway': 2, 'predominately': 6, 'contrary': 6, '4th': 1, 'starbucks': 6, 'pricey': 1, 'seattle': 8, 'briskly': 1, 'hamburgers': 2, 'drinkers': 2, 'avoiding': 1, 'ballad': 1, 'hey': 2, 'pharrell': 1, 'download': 2, 'downloads': 1, 'themed': 1, 'hamburg': 2, 'yazidi': 2, 'sinjar': 2, 'reference': 15, 'qasim': 2, 'dakhil': 1, 'yazidis': 1, 'saeedi': 2, 'gentry': 1, 'disorders': 2, '1772': 1, 'partitioned': 3, 'prussia': 2, '1795': 3, '279': 1, 'levies': 2, 'unlawfully': 3, 'kivu': 11, 'nioka': 2, 'hillah': 5, 'spied': 2, 'beheading': 5, 'tolerant': 3, 'comparatively': 1, '850': 8, 'amisom': 2, 'burundian': 1, 'logistics': 14, 'ochami': 1, 'onyango': 1, 'omollo': 1, 'entitled': 6, 'bahonar': 2, 'vahidi': 2, 'vahid': 2, 'dastjerdi': 1, 'marzieh': 1, 'shock': 7, 'dilapidated': 2, 'transform': 5, 'underclass': 1, 'caretaker': 12, 'indulge': 1, 'sajjad': 2, 'bakhsh': 1, 'fakhar': 1, 'seeduzzaman': 1, 'personalities': 4, 'soomro': 1, 'elahi': 1, 'siddiqui': 3, 'ubaydi': 3, 'curtain': 6, 'caches': 4, 'sharki': 2, 'shadid': 2, 'eldest': 5, 'yonhap': 14, 'dignitary': 1, 'undated': 1, 'krzanich': 1, 'ho': 4, 'chi': 1, 'minh': 1, 'hayat': 5, 'thoroughly': 2, 'depots': 2, 'quality': 12, 'pneumonia': 7, 'evaluates': 1, 'culmination': 2, '80th': 4, 'belatedly': 2, 'gala': 2, 'presenter': 1, 'nicaraguan': 10, 'ortega': 12, 'culminate': 3, 'danes': 2, 'equitable': 4, 'solutions': 5, 'raw': 15, 'zin': 1, 'bo': 1, 'ohn': 1, 'khun': 1, 'sai': 1, 'disbanded': 11, '937': 2, 'wrongfully': 2, 'purged': 2, 'nib': 1, 'yucatan': 8, 'cancun': 12, 'emptied': 3, 'bused': 1, 'rafts': 2, 'banjul': 2, 'communiqué': 1, 'undermining': 10, 'privatizing': 2, 'simplify': 2, 'innovative': 5, 'boom': 18, 'autopsy': 2, 'shinobu': 1, 'fukusho': 1, 'autopsies': 1, 'pathology': 1, 'hasegawa': 1, 'halemi': 1, 'chaman': 3, 'frustration': 6, 'disillusioned': 1, 'torch': 34, 'relay': 28, 'flame': 12, 'everest': 9, 'ron': 5, 'zonen': 1, 'survivor': 5, 'melville': 1, 'rodney': 1, 'extort': 3, 'cyclical': 2, 'nights': 4, 'djibouti': 23, 'firimbi': 1, 'ganey': 1, 'daschle': 3, 'thune': 2, 'employer': 2, 'rendell': 1, '111th': 1, 'ed': 2, 'survive': 7, 'daxing': 1, 'xingning': 2, 'roles': 10, 'mayors': 6, 'meizhou': 1, 'drunk': 5, 'apologize': 8, 'loved': 11, 'harmonized': 2, 'mutahida': 2, 'amal': 3, 'majlis': 4, 'betterment': 2, 'qazi': 6, 'masses': 6, 'enriching': 16, 'npt': 3, 'breaches': 6, 'arguing': 12, 'perez': 11, 'petroleos': 4, 'retaining': 2, 'portfolio': 4, 'consolidated': 2, 'forever': 5, 'domineering': 1, 'minded': 5, 'impending': 4, 'ratio': 7, 'baked': 2, 'tub': 2, 'hugh': 4, 'whittaker': 1, 'weddings': 2, 'notting': 1, 'swung': 3, 'insurer': 3, '306': 1, '415': 1, 'underperforming': 1, 'basotho': 1, 'sacu': 6, 'dependency': 9, 'royalties': 8, 'milling': 2, 'jute': 2, 'canning': 1, 'leather': 2, 'mineworkers': 1, 'herding': 1, 'drawback': 1, '362': 1, 'contributor': 4, 'precipitously': 1, 'sinchulu': 1, 'bhutan': 8, 'ceding': 1, '1907': 2, 'bhutanese': 3, 'whereby': 1, 'criteria': 5, 'krone': 1, '1947': 13, 'indo': 3, 'defined': 3, 'formalized': 2, 'unhcr': 2, 'introduce': 5, 'wangchuck': 2, 'jigme': 2, 'singye': 1, 'throne': 16, 'abdicated': 2, 'namgyel': 1, 'khesar': 1, 'renegotiated': 2, 'thimphu': 1, 'loosening': 1, 'socialism': 11, 'alleviate': 4, 'inefficiencies': 3, 'preferential': 2, 'vegetables': 7, 'pigs': 9, 'licensing': 6, 'venturing': 1, 'peacocks': 3, 'moulting': 1, 'jay': 5, 'walk': 12, '1902': 3, 'administer': 3, 'strutted': 1, 'striding': 1, 'pecked': 1, 'cheat': 1, 'plucked': 1, 'plumes': 1, 'borrowed': 2, 'annoyed': 4, 'behaviour': 1, 'jays': 1, 'equally': 6, 'gullet': 1, 'bolted': 3, 'seagull': 1, 'richly': 2, 'kite': 7, 'cloak': 1, 'pawned': 1, 'spendthrift': 1, 'nose': 12, 'admit': 9, 'solemnly': 1, 'sour': 2, 'inch': 4, 'bougainville': 1, 'teeth': 5, 'claws': 4, 'cleanup': 6, 'skimming': 2, 'vents': 2, 'cleaned': 5, 'separating': 11, 'liters': 5, 'oily': 1, 'skimmers': 1, 'halting': 6, 'containment': 2, 'hookup': 1, '1890s': 1, 'pensacola': 1, 'lisa': 3, 'leased': 1, 'gushing': 1, '322': 1, 'auditors': 4, 'intensify': 9, 'dues': 1, 'guesthouse': 1, 'vanuatu': 8, 'examples': 3, 'inland': 3, 'tegua': 1, 'waterborne': 4, 'accelerating': 7, 'manifestation': 1, 'executing': 5, 'minors': 2, 'executes': 1, 'juveniles': 2, 'gallows': 2, 'stamped': 1, 'headscarf': 3, 'crying': 5, 'newscaster': 1, 'malfeasance': 1, 'reestablishing': 1, 'allan': 1, 'kemakeza': 1, 'sir': 4, 'unreasonable': 4, 'tiananmen': 10, 'spearheaded': 4, 'ramsi': 1, 'cats': 4, 'pet': 7, 'asians': 4, 'spacewalk': 13, 'garan': 1, 'fossum': 1, 'nitrogen': 3, 'panels': 7, 'analysis': 8, 'fig': 2, 'ridiculed': 3, 'olive': 8, 'seasons': 4, 'akihiko': 1, 'astronaut': 9, 'yasuo': 3, 'hoshide': 2, 'robotic': 5, 'plaintiffs': 2, 'foliage': 1, 'shower': 1, 'despoiling': 1, 'kiir': 6, 'mayardit': 2, 'salva': 5, 'injure': 4, 'denuded': 1, 'radicalism': 3, 'deceive': 1, 'wider': 5, 'democratizing': 1, 'bossaso': 1, 'vaunting': 1, 'fearlessness': 1, 'subscribers': 2, 'purity': 2, 'pained': 1, 'infectious': 6, 'afable': 1, 'lumpur': 8, 'moro': 2, 'milf': 3, 'manila': 22, 'kuala': 8, 'silvestre': 1, 'renounced': 7, 'enterprising': 1, 'fearless': 1, 'pure': 3, 'modernizes': 1, 'disappearing': 2, 'arts': 8, 'schearf': 1, 'airs': 3, 'folly': 2, 'reasoned': 1, 'expenses': 7, 'slumping': 4, 'swicord': 1, 'expression': 15, 'supplement': 6, 'pu': 1, 'rui': 1, 'signatories': 1, 'mao': 5, 'houze': 1, 'zhu': 1, 'discover': 5, 'prosperity': 11, 'endeavoured': 1, '191': 6, '199': 2, 'keeps': 10, 'lundestad': 1, '168': 1, 'spaceship': 3, 'cattle': 22, 'roswell': 1, 'aliens': 5, 'selections': 2, 'bono': 2, 'geldof': 3, 'u2': 4, 'surviving': 8, 'anh': 1, 'ha': 2, 'tay': 1, 'duong': 1, 'vinh': 2, 'gore': 13, '1948': 12, '167': 5, 'kalam': 4, 'kerala': 5, 'nicobar': 6, 'pondicherry': 1, 'grim': 3, 'nadu': 2, 'refers': 3, 'clears': 4, 'stakes': 5, 'rails': 1, 'mehrabpur': 1, 'sindh': 6, 'contraction': 14, 'welded': 1, 'darkness': 1, 'meterologists': 1, 'tammy': 3, 'canaveral': 6, 'stan': 10, 'dissipating': 2, 'hollywood': 12, 'wizard': 3, 'oz': 2, 'mgm': 2, 'roaring': 1, 'distinctive': 4, 'restructured': 3, 'movies': 8, 'spyglass': 1, 'entertainment': 10, 'outdo': 1, 'triumphs': 1, 'carl': 4, 'icahn': 1, 'dvds': 2, 'tickets': 11, 'rosales': 3, 'addresses': 9, 'pennetta': 3, 'plavia': 1, 'streak': 4, 'safarova': 6, 'lucie': 5, 'safina': 6, 'dinara': 5, 'patterson': 2, 'gregory': 4, 'firearm': 2, 'recruiting': 23, 'levar': 1, 'conspirator': 2, "jam'iyyat": 1, 'saheeh': 1, 'ul': 7, 'hammad': 2, 'psychiatric': 4, 'samana': 1, 'latina': 1, 'scapegoating': 1, 'zulia': 4, 'hilda': 2, 'solis': 3, 'alienating': 1, 'criminalizes': 1, 'compassionate': 3, 'charm': 6, 'flocking': 1, 'facelift': 1, 'floral': 3, 'makeover': 2, 'warner': 3, 'modernity': 1, 'cubanization': 1, 'bloodiest': 9, 'dhahran': 2, 'vigilance': 3, 'urges': 8, 'frequented': 2, 'amhed': 1, 'mounted': 10, 'gustavo': 2, 'dominguez': 4, 'braves': 1, 'feeder': 1, 'diamondbacks': 1, 'castillo': 1, 'francisely': 1, 'pitches': 2, 'osbek': 1, 'bueno': 1, 'alabama': 7, 'ilyas': 1, 'shurpayev': 2, 'reestablish': 2, 'repressed': 1, 'hernan': 1, 'arboleda': 2, 'inguri': 1, 'ruptured': 3, 'khetaguri': 1, 'malfunctioned': 2, 'tbilisi': 14, 'strangled': 2, 'raced': 4, 'wta': 6, 'jaw': 1, 'airplanes': 11, 'ridden': 5, 'dagestan': 7, 'extensively': 1, 'signings': 1, 'untapped': 2, 'nickel': 5, 'repayment': 3, 'economically': 5, 'dismiss': 18, 'unconstitutional': 8, 'astana': 3, 'holovaty': 3, 'serhiy': 3, 'exceeding': 6, 'betrayal': 1, 'pretty': 4, 'definite': 1, 'suitable': 3, 'datta': 3, 'khel': 4, 'shana': 1, 'garhi': 1, 'natanz': 6, 'observation': 2, 'ransacking': 1, 'crowds': 23, 'airlifting': 4, 'familiar': 8, 'piled': 2, 'bula': 1, 'hawo': 1, 'ahern': 3, 'belfast': 3, 'bertie': 3, 'photographic': 1, 'protestants': 2, 'overturning': 3, 'vandalized': 2, 'baran': 1, 'formality': 3, 'solemn': 3, 'helmet': 1, 'hoisting': 1, 'illusion': 1, 'confront': 13, 'sergio': 2, 'mello': 2, 'viera': 2, 'sherpao': 1, 'yasir': 3, 'aftab': 1, 'adil': 2, 'deported': 13, 'arrival': 25, 'lodi': 1, 'wirayuda': 2, 'albar': 2, 'diplomatically': 2, 'solve': 13, 'background': 8, 'accidental': 10, 'metropolitan': 3, 'disturbances': 2, 'zazai': 1, 'manhattan': 8, 'morgenthau': 3, 'jennifer': 9, 'muniz': 1, 'marco': 4, 'errors': 8, 'unaware': 2, 'maywand': 1, 'glorifies': 3, 'households': 10, 'tolls': 2, 'mishandling': 3, 'hosada': 1, 'misunderstandings': 2, 'guangzhou': 10, 'mobbed': 1, 'supermarket': 2, 'shenzhen': 3, 'textbooks': 3, 'eviction': 6, 'canes': 1, 'butts': 1, 'openly': 10, 'vazquez': 5, 'tabare': 2, 'uruguayan': 3, 'montevideo': 5, 'jorge': 8, 'oncologist': 1, 'meddles': 1, 'meddle': 2, 'interferes': 1, 'suppresses': 1, 'heydari': 2, 'inevitable': 2, 'shaping': 2, 'nevada': 5, 'balances': 2, 'fathers': 1, 'checks': 13, 'retaliate': 7, 'suggest': 12, 'voicing': 2, 'tours': 4, 'vested': 2, 'factual': 2, 'electronically': 2, 'email': 2, 'transcripts': 6, 'kashmiris': 3, 'uighurs': 5, 'acceptance': 3, 'turkic': 2, 'uighur': 5, 'guise': 3, 'judith': 1, 'dateline': 1, 'explores': 2, 'latham': 1, 'minerals': 11, 'isro': 1, 'griffin': 3, 'nair': 1, 'madhavan': 1, 'applicants': 4, 'tighter': 6, 'chandrayaan': 2, 'sensors': 2, 'array': 1, 'resting': 7, 'salvation': 1, 'sayidat': 1, 'nejat': 1, 'netted': 3, 'golding': 4, 'bruce': 4, 'borrowers': 6, 'structuring': 2, 'iscuande': 2, 'chartered': 8, 'mcguffin': 1, 'maan': 6, 'metric': 14, 'ocampo': 2, 'kushayb': 1, 'harun': 1, 'slip': 3, 'uzair': 1, 'paracha': 3, 'plotted': 3, 'duped': 2, 'nathan': 4, 'solicited': 1, 'reassure': 2, 'mashhadani': 3, 'sulaimaniya': 2, 'irbil': 3, 'foe': 2, 'disagreed': 3, 'aspects': 3, 'dublin': 3, 'seekers': 12, 'frustrated': 6, 'ecolog': 1, 'summon': 1, 'mustaqbal': 1, 'sessions': 7, 'fruitful': 5, 'hebei': 7, 'tangshan': 1, 'liuguantun': 1, 'helland': 1, 'quarterfinals': 11, 'flushing': 3, 'meadows': 1, 'stanilas': 1, 'warwrinka': 1, 'gulbis': 1, 'ernest': 3, 'jul': 10, 'henin': 8, 'serena': 3, 'justine': 4, 'database': 8, 'quadrupled': 2, 'spellings': 1, 'aliases': 2, 'compilation': 1, 'banco': 1, 'championed': 1, 'conditional': 2, 'zabi': 1, 'taifi': 2, 'commuters': 4, 'automobile': 8, 'carmaker': 5, "gm's": 1, 'combine': 3, 'conventional': 8, 'slump': 9, 'automakers': 7, '54': 20, 'mongolia': 13, 'baotou': 2, 'nanhai': 1, 'miner': 4, 'yachts': 3, 'crj': 3, 'commuter': 9, 'bombardier': 1, 'backpack': 3, 'robot': 5, 'client': 16, 'bakr': 2, 'wiretapping': 4, 'intercepting': 2, 'wiretaps': 4, 'das': 4, 'wiretapped': 1, 'intercepted': 18, 'semana': 1, 'wrongful': 2, 'pilar': 1, 'hurtado': 2, 'imprisoning': 3, 'assured': 21, 'seizures': 8, 'shengyou': 1, 'clubs': 7, 'thugs': 1, 'feigning': 1, 'roth': 2, 'mandated': 6, 'murderous': 2, 'aquatic': 3, 'builders': 2, '976': 2, 'buro': 1, 'hok': 1, 'happold': 1, 'oda': 1, 'gateway': 4, '476': 1, 'aquatics': 1, 'reconfigured': 1, 'balfour': 1, 'beatty': 1, 'chattisgarh': 1, 'peasants': 4, 'landless': 8, 'roots': 4, 'heritage': 9, 'malls': 2, 'developers': 2, 'homosexuality': 4, 'sin': 1, 'buttiglione': 1, 'rocco': 1, 'description': 3, 'primerica': 4, '472': 1, 'valued': 9, 'delisted': 1, 'duluth': 1, 'ga': 1, 'subsidiaries': 2, 'marketed': 3, 'liabilities': 2, 'fiji': 9, 'endowed': 4, 'fijians': 2, 'harmed': 5, 'author': 10, 'ruler': 16, 'realize': 2, 'adept': 1, 'smarter': 2, 'arrivals': 9, 'dipped': 3, 'landholders': 1, 'jubilee': 1, 'unmasking': 1, 'secretive': 4, 'relied': 4, 'mcc': 2, 'benefiting': 3, 'opted': 4, 'multilateral': 13, 'hipc': 9, 'civic': 3, 'macro': 1, 'violently': 2, 'regulated': 2, 'flourishes': 2, 'unrwa': 1, 'underestimate': 1, 'outsiders': 5, 'astrology': 1, 'handicapped': 1, 'km': 7, 'stream': 10, 'gum': 2, 'astute': 1, 'flesh': 2, 'meat': 24, 'fiercely': 7, 'esteem': 1, 'mortals': 1, 'sculptor': 3, 'disguise': 4, 'statues': 2, 'juno': 2, 'statue': 4, 'messenger': 1, 'sum': 4, 'certainly': 3, 'gods': 2, 'fling': 1, 'journeying': 1, 'tall': 7, 'waited': 8, 'nearer': 2, 'loosen': 1, 'wood': 14, 'companions': 6, 'faggot': 1, 'outrun': 2, 'anticipations': 1, 'realities': 2, 'pursuer': 1, 'strand': 1, 'hot': 13, 'tumultous': 1, 'recollecting': 1, 'hollow': 3, "'t": 1, 'ai': 3, 'skipper': 1, 'heartless': 1, 'rang': 6, 'sadly': 2, 'soul': 8, 'convergent': 1, 'sat': 8, 'murmured': 1, 'marooned': 1, 'shareman': 1, 'ample': 2, 'hauls': 1, 'screened': 3, 'pudong': 1, 'temperature': 5, 'mistreated': 7, 'deportees': 1, 'deportation': 13, 'qatada': 1, 'retrieve': 2, 'projectiles': 3, 'lerner': 1, 'nahal': 1, 'sufa': 1, 'alzheimer': 3, 'illinois': 16, 'antihistamine': 1, 'spray': 2, 'lifestyle': 2, 'heredity': 1, 'determining': 8, 'batter': 1, 'vero': 1, 'southward': 1, 'sellers': 3, 'diving': 5, 'rong': 1, 'ticketing': 1, 'utilized': 1, 'oversold': 1, 'skidded': 3, '165': 6, 'divides': 5, 'lobbed': 2, 'blackhawk': 1, 'causalities': 2, 'registration': 15, 'tshisekedi': 1, 'etienne': 1, 'militarily': 2, 'mingled': 2, 'veracruz': 8, 'pemex': 7, 'weakness': 1, 'fertilizer': 14, 'fireball': 2, 'coahuila': 1, 'charai': 1, 'persists': 1, 'bills': 15, 'item': 5, 'tacked': 1, 'wasteful': 2, 'additions': 1, 'earmarks': 1, 'gauteng': 1, 'tb': 8, 'xdr': 4, 'surprises': 1, 'clarification': 4, 'angrily': 4, 'reacted': 10, 'quo': 2, 'manama': 1, 'attitude': 3, 'dialog': 1, 'namibian': 6, 'namibians': 2, 'grimes': 2, 'frederic': 1, 'piry': 1, 'telegram': 3, 'angelo': 2, 'sodano': 2, 'cow': 15, 'mad': 10, 'spinal': 4, 'abe': 9, 'shinzo': 5, 'veal': 3, 'reimposed': 4, 'vladivostok': 2, 'navies': 2, 'kwazulu': 1, 'natal': 2, 'select': 11, 'hajim': 1, 'hassani': 2, 'mento': 1, 'tshabalala': 4, 'msimang': 1, 'uncensored': 3, 'blacked': 1, 'underscoring': 1, 'stoned': 2, 'daglas': 1, 'torched': 9, 'ghassan': 1, 'blazing': 1, 'vary': 4, 'settler': 10, 'retaliating': 1, 'caravan': 1, 'belaroussi': 2, 'muttawakil': 2, 'wakil': 2, 'rank': 7, 'hardcore': 1, 'admitting': 3, 'wielgus': 5, 'stanislaw': 2, 'conscious': 1, 'rafiqi': 1, 'hayabullah': 1, 'collaborated': 3, 'qabail': 1, 'izarra': 2, 'telesur': 5, 'mouthpiece': 3, 'rhetoric': 4, 'scared': 7, 'solders': 1, 'theaters': 7, 'attach': 5, 'fiction': 4, 'disclaimer': 3, 'vinci': 3, '520': 2, 'repsol': 1, 'britney': 9, 'spears': 8, 'federline': 8, 'probed': 2, 'norma': 1, 'lapd': 2, 'nonspecific': 1, 'eimiller': 2, 'uncorroborated': 1, 'jayden': 2, 'bode': 5, 'maier': 3, 'strobl': 2, 'hermann': 1, 'daron': 2, 'fritz': 3, 'walchhofer': 6, 'rahlves': 5, 'countryman': 7, 'skates': 1, 'anton': 5, 'apollo': 1, 'ohno': 1, 'friesinger': 1, 'pechstein': 1, 'anni': 1, 'stressing': 2, 'zoeggeler': 1, 'armin': 1, 'forensics': 3, 'daud': 2, 'cooperative': 2, 'harmonious': 3, 'resolves': 1, 'strive': 2, 'forgo': 2, 'propose': 9, 'forbidding': 3, 'qala': 11, 'overran': 3, 'appointing': 5, 'super': 12, 'sciri': 1, 'sway': 4, 'echoes': 1, 'supplying': 14, 'chemet': 1, 'metallurgy': 1, 'ounion': 1, 'limmt': 2, 'zibo': 1, 'norinco': 1, 'hongdu': 1, 'aero': 2, 'walt': 1, '660': 1, 'miramax': 2, 'filmyard': 3, 'nonrefundable': 1, 'deposit': 3, 'holdings': 8, 'tutor': 1, 'pulp': 2, 'shakespeare': 2, 'pixar': 1, 'marvel': 1, 'iger': 1, 'bidders': 2, 'harvey': 1, 'weinstein': 2, 'beast': 4, 'bloodthirsty': 1, 'stanizai': 2, 'hated': 1, 'monster': 1, 'mccellan': 1, 'provocative': 6, 'ronnie': 2, 'ultimatum': 5, 'andry': 2, 'rajoelina': 4, 'mujahedin': 6, 'najibullah': 2, 'mwangura': 2, 'seafarers': 2, 'container': 5, 'mombasa': 3, 'kismayo': 2, '740': 1, 'receving': 1, 'inconceivable': 2, 'advocated': 4, 'ecuadoreans': 1, 'proven': 9, 'outraged': 6, 'fradkov': 2, 'tachilek': 1, 'pills': 3, '760': 2, 'proposing': 2, 'textiles': 9, 'byrd': 2, 'sebastien': 2, 'stretching': 4, 'loeb': 7, 'noel': 1, 'choong': 2, 'hijack': 6, 'imb': 1, 'alerted': 7, 'warship': 6, 'melted': 4, 'solberg': 3, 'petter': 3, 'subaru': 4, 'auc': 3, 'plagues': 3, 'demobilization': 4, 'hurriyah': 2, 'ambushes': 8, 'marcus': 2, 'gronholm': 1, 'thirteen': 6, 'lahiya': 3, 'immunizing': 1, 'zahar': 4, 'duaik': 1, 'charger': 1, 'malfunction': 2, 'gigi': 1, 'galli': 1, 'turbo': 1, 'mitsubishi': 1, 'valves': 1, 'guajira': 2, 'connects': 2, 'maracaibo': 1, 'pdvsa': 8, 'haaretz': 6, 'gazan': 1, 'constituted': 1, 'inquest': 2, 'admission': 7, 'guilt': 1, 'stripped': 6, 'bickering': 1, 'monoply': 1, 'plays': 13, 'xp': 1, 'routing': 2, 'saakashvili': 30, 'gurgenidze': 3, 'mikheil': 5, 'lado': 1, 'reformist': 9, 'mgalobishvili': 1, 'grigol': 1, 'markko': 1, 'banker': 4, 'technocrat': 1, 'newhouse': 1, 'barricade': 3, 'bernama': 2, 'asean': 19, 'jabaliya': 3, 'gearing': 4, 'swing': 7, 'advising': 6, 'patricia': 2, 'sezibera': 1, 'massing': 2, 'atenco': 2, 'qassim': 1, 'tamin': 2, 'sleeping': 4, 'nuristani': 2, 'nuristan': 11, 'branco': 1, 'scathing': 1, 'charsadda': 4, 'giliani': 1, 'heinous': 5, 'commerical': 1, 'traumatized': 1, 'veneman': 3, 'untreated': 1, 'adwa': 1, 'underscores': 2, 'wlodzimierz': 1, 'odzimierz': 1, 'cimoszewicz': 2, 'untouched': 2, 'tarnished': 2, 'searchers': 2, 'leftists': 5, 'bravo': 5, 'leopoldo': 1, 'taiana': 1, 'aires': 8, 'buenos': 8, 'parliaments': 2, 'dabaa': 2, 'kam': 4, 'barot': 2, 'dhiren': 1, 'qaisar': 1, 'tarmohammed': 1, 'nadeem': 1, 'shaffi': 1, 'hindi': 7, 'esa': 1, 'prudential': 5, 'newark': 2, 'citicorp': 1, 'subcommittee': 7, 'trucking': 3, 'littered': 3, 'unexploded': 4, 'vigil': 3, 'myers': 9, 'julie': 2, 'oaxaca': 7, 'graffiti': 1, 'painted': 4, 'ruiz': 3, 'resigns': 2, 'ulises': 2, 'elephant': 7, 'culling': 7, 'elephants': 10, 'thin': 5, 'turner': 1, 'durand': 1, 'noses': 1, 'strikers': 1, 'stomachs': 1, 'contacted': 13, 'lauder': 1, 'schneider': 1, 'anxiety': 2, 'instances': 9, 'semitism': 2, 'semitic': 3, 'interfaith': 2, 'inforadio': 1, 'daylam': 2, 'blasting': 5, 'bushehr': 13, 'camilo': 2, 'reyes': 7, 'premeditated': 3, 'strayed': 6, 'jarrin': 1, 'oswaldo': 1, 'nyan': 3, 'razali': 2, 'intelogic': 5, 'trace': 4, 'unaffiliated': 1, 'edelman': 4, 'ackerman': 4, 'asher': 2, 'datapoint': 1, 'maximize': 2, 'marty': 3, 'finfish': 1, 'krill': 2, 'harvesting': 4, 'licenses': 9, 'cruise': 9, 'specialized': 2, 'manpower': 4, 'administrations': 3, 'purely': 4, 'instabilities': 1, 'guiana': 4, 'mayotte': 1, 'guadeloupe': 3, 'martinique': 1, 'reunion': 3, 'overpopulated': 1, 'inefficiently': 1, 'resilient': 6, 'fy10': 1, 'totaling': 5, 'garment': 7, 'fy09': 1, 'fourths': 3, 'pulses': 1, 'sugarcane': 4, 'hampers': 2, 'mw': 1, 'scope': 9, 'susceptibility': 2, 'brook': 2, 'plank': 2, 'grasping': 1, 'beware': 3, 'lest': 2, 'mossad': 3, 'teach': 9, 'stating': 5, 'idf': 1, 'dynamics': 2, 'strelets': 1, 'hrytsenko': 2, 'anatoliy': 1, 'theoretically': 1, 'catering': 2, 'debated': 4, 'signatures': 6, 'symbolically': 2, 'chinook': 9, 'ch': 2, 'tamara': 3, 'lawrence': 7, 'tishrin': 1, 'torrential': 11, 'sincerity': 1, 'zhouqu': 1, '254': 4, 'gansu': 3, 'nasrallah': 9, 'worsening': 8, 'climbing': 4, 'watchers': 1, 'emotional': 4, 'jammed': 1, 'lobbyist': 4, 'disgraced': 10, 'abramoff': 10, 'think': 26, 'knowing': 4, 'ethics': 8, 'resurgent': 11, 'wrongly': 8, 'harper': 10, 'reprinting': 4, 'depiction': 4, 'haider': 7, 'muttahida': 6, 'quami': 3, 'mqm': 11, 'reprinted': 4, 'foment': 2, 'tashkent': 9, 'revolutions': 2, 'jones': 22, 'dow': 14, 'pashtuns': 4, 'urdu': 5, 'awami': 8, 'termed': 9, 'teammate': 4, 'fouled': 1, 'rican': 10, 'narvaez': 1, 'gymnasium': 1, 'yi': 6, 'jianlian': 1, 'insults': 3, 'spilled': 7, 'shielding': 2, 'locker': 1, 'deplored': 4, 'waved': 8, 'marchers': 7, 'shirts': 8, 't': 7, 'wore': 1, "dvd's": 1, 'sassou': 5, 'nguesso': 4, 'denis': 6, 'palma': 3, 'chilitepic': 1, 'sola': 1, '270': 6, 'baby': 13, 'medan': 1, 'cluster': 8, '147': 7, 'bekasi': 2, 'dispatch': 6, 'okinawa': 2, 'galle': 4, 'flash': 17, 'lankans': 4, 'tahseen': 1, 'abhorrent': 2, 'poul': 1, 'nielsen': 1, 'caring': 2, 'undecided': 7, 'farabaugh': 1, 'tong': 5, 'reebok': 1, 'vines': 3, 'alston': 5, 'don': 5, 'nord': 1, 'eclair': 1, 'gerais': 2, 'gobernador': 1, 'valadares': 1, 'casket': 2, 'movements': 14, 'berenson': 7, 'lori': 2, 'alejandro': 5, 'toledo': 5, 'tupac': 2, 'amaru': 2, 'airmen': 3, 'emergencies': 3, 'biloxi': 1, 'keesler': 2, 'rotate': 3, 'expletive': 1, 'laughed': 4, 'oskar': 1, 'kaibyshev': 3, 'bashkortostan': 1, 'patented': 2, 'grateful': 5, 'alekseyeva': 1, 'outrageous': 2, 'framed': 2, 'bannu': 4, 'rewriting': 1, 'andrade': 1, 'diaz': 7, 'gereida': 1, 'dishonest': 2, 'lauderdale': 3, 'formalizing': 1, 'reservations': 3, 'cursed': 4, 'odd': 2, 'unlimited': 2, 'totalitarian': 1, 'resembles': 3, 'pinchuk': 1, 'guilin': 1, 'mei': 3, 'wolong': 1, 'andrei': 5, 'nesterenko': 2, 'proceeding': 2, 'nestrenko': 1, 'ritual': 6, 'ashura': 11, 'farsi': 3, 'mehdi': 8, 'mousavi': 15, 'mir': 15, 'hossein': 14, 'karroubi': 4, 'mourino': 2, 'tellez': 2, 'recordings': 4, 'boxes': 6, 'paseo': 1, 'avenue': 3, 'reforma': 1, 'vasconcelos': 1, 'tahab': 1, 'nadi': 1, 'ghulam': 4, 'hizb': 2, 'mujahedeen': 3, 'snarled': 1, 'inception': 1, 'choking': 4, 'congestion': 1, 'steven': 4, 'briefing': 12, 'whitcomb': 2, 'underneath': 3, 'servicemembers': 2, 'hunan': 8, 'conjunction': 1, 'simulated': 1, 'kot': 6, 'tarin': 1, 'neumann': 3, 'phoned': 3, 'speeding': 5, 'purim': 1, 'galloway': 1, 'luther': 8, 'rejects': 14, 'reverend': 7, 'barter': 1, 'disrepair': 1, 'portrays': 3, 'galleries': 2, 'showcasing': 3, 'showcase': 3, 'mort': 3, 'reelection': 4, 'myuran': 2, 'denpasar': 1, 'sukumaran': 2, 'stephens': 1, 'czugaj': 1, 'jalawla': 2, 'renae': 1, 'earliest': 3, 'burial': 9, 'crypt': 3, 'lambasting': 1, 'adoring': 1, 'divisiveness': 1, 'harshly': 3, 'transparent': 10, 'embraced': 2, 'antalya': 2, 'henochowicz': 2, 'canister': 1, 'emily': 2, 'flotilla': 9, 'canisters': 4, 'sparingly': 1, 'bartering': 2, 'catching': 4, 'besides': 6, 'conspirators': 3, '236': 1, 'quezon': 2, 'emptying': 2, 'reap': 1, 'domenici': 1, 'inspections': 15, 'empowered': 2, 'pulwama': 3, 'katsav': 8, 'vetoed': 1, 'secularists': 5, 'telerate': 6, 'est': 1, '576': 1, 'tendered': 5, 'barron': 1, 'alluvial': 2, 'namibia': 16, 'gem': 4, 'backyard': 4, 'cereal': 3, 'coefficient': 1, 'distributions': 1, 'hides': 2, 'gini': 1, 'rand': 4, 'allotments': 1, 'germanic': 1, '1806': 2, '1815': 4, '1866': 1, 'shortcomings': 3, 'colonized': 4, 'macau': 13, 'sar': 3, 'practiced': 1, 'annals': 1, 'evolution': 5, '1951': 4, 'phenomenon': 3, 'supranational': 1, 'dynastic': 3, 'norm': 2, 'cede': 3, 'entity': 6, 'overarching': 1, 'orientation': 3, 'exodus': 2, 'yemenis': 1, 'subdued': 4, 'kooyong': 3, 'delimitation': 1, 'tentative': 3, 'zaydi': 1, 'huthi': 1, 'revitalized': 1, 'socioeconomic': 2, "sana'a": 1, 'unifying': 2, 'hardened': 2, 'strangle': 2, 'sprain': 1, 'racquetball': 1, 'loosed': 1, 'coil': 1, 'prey': 9, 'poison': 11, 'irritated': 1, 'ignorant': 1, 'aloft': 5, 'rustic': 1, 'goose': 14, 'thereupon': 2, 'induce': 1, 'mused': 1, 'bother': 2, 'noticed': 6, 'ilyushin': 1, 'rosoboronexport': 1, 'izvestia': 3, 'sukhoi': 2, 'ranked': 22, 'balboa': 1, 'detailing': 2, 'nightline': 1, 'carriers': 9, 'embarking': 1, '232nd': 1, 'reminded': 5, 'risking': 1, 'proud': 5, 'roche': 14, 'juste': 1, 'narrated': 5, 'moradi': 1, 'prolong': 3, 'allotted': 2, 'geoffrey': 2, 'inked': 2, 'avoidance': 1, 'taxation': 7, 'hayashi': 1, 'yoshimasa': 1, 'praising': 6, 'johndroe': 4, 'pacifist': 2, 'hangzhou': 2, 'zhejiang': 7, 'xi': 4, 'jinping': 1, 'sachs': 6, 'goldman': 7, 'undervalued': 1, 'yuan': 10, 'ninevah': 3, 'wipe': 3, 'reinforcement': 2, '502nd': 1, 'infantry': 6, '2nd': 1, 'battalion': 4, 'scholarships': 1, 'specter': 1, 'contradicted': 1, 'tolerated': 4, 'nsanje': 1, 'depot': 7, 'expired': 14, 'gah': 7, 'pepfar': 1, 'maroua': 1, 'laborer': 2, 'lobbying': 8, 'modify': 2, 'mutation': 3, 'rania': 1, 'joyous': 1, 'somber': 2, 'ruins': 10, 'eni': 1, 'wintry': 1, 'dictates': 1, 'otunbayeva': 7, 'roza': 6, 'bishkek': 13, 'tenure': 5, 'bakiyev': 17, 'kurmanbek': 9, 'uzbeks': 6, 'intercontinental': 6, 'teikovo': 1, 'topol': 8, 'ivanovo': 1, 'payload': 2, 'countering': 1, 'interceptors': 3, 'radar': 13, 'macarthur': 1, 'bidding': 10, 'parcels': 1, 'fragmented': 2, 'undemocratic': 2, 'zahraa': 1, 'etman': 1, 'fatma': 2, 'camped': 4, 'incentive': 2, 'compel': 1, 'choreographer': 4, 'kidd': 3, 'broadway': 2, 'exuberant': 1, 'guys': 1, 'dolls': 2, '1954': 5, 'brides': 1, 'choreography': 2, 'vientiane': 3, 'chanthalangsy': 1, 'sawang': 1, '112': 5, 'hafez': 2, 'cracking': 7, 'frigid': 1, 'dikweneh': 1, 'ablaze': 3, 'ambulances': 1, 'parisian': 2, 'connect': 7, 'paddick': 1, 'russell': 1, 'kerik': 9, 'abruptly': 7, 'nanny': 2, 'madaen': 1, 'marjah': 2, 'thefts': 2, 'complicating': 3, 'intermediaries': 1, 'kuwaitis': 1, 'mutairi': 1, 'representation': 15, 'nobody': 4, 'traced': 4, 'degrading': 4, 'lieu': 1, 'bloemfontein': 1, '720': 3, 'soup': 5, 'conjured': 1, 'slovak': 4, 'gasparovic': 1, 'bratislava': 5, 'czechoslovakia': 7, 'shed': 2, 'slashing': 9, 'wholesalers': 1, 'madoff': 10, 'swindler': 2, '162': 6, 'koufax': 1, 'mets': 2, 'legendary': 6, 'wilpon': 1, 'citigroup': 7, 'pyramid': 4, 'causality': 1, 'chanting': 15, 'nour': 13, 'ghad': 7, 'aboul': 5, 'gheit': 7, 'grandchild': 2, 'baladiyat': 1, 'medically': 2, 'anesthesia': 1, 'mor': 1, 'shlomo': 1, 'hadassah': 5, 'dosage': 1, 'sedated': 1, 'unconsciousness': 1, 'hemorrhage': 5, 'function': 5, 'chances': 7, '481': 2, 'elimination': 3, 'painting': 4, 'farouk': 4, 'vase': 1, 'kelantan': 1, 'sutham': 1, 'saengprathum': 1, 'weighing': 4, 'polytechnic': 1, 'adb': 3, 'backcountry': 1, 'hindus': 4, 'birthplace': 4, 'babri': 1, 'rama': 1, 'shiv': 1, 'sena': 1, 'safer': 6, 'irving': 8, 'historian': 5, 'hitler': 6, '784': 1, 'atrophy': 4, 'heishan': 1, 'seyoum': 1, 'mesfin': 1, 'guangxi': 2, 'naeem': 2, 'noor': 2, 'awan': 1, 'tanzanian': 3, 'cayenne': 1, 'brace': 2, 'damrey': 5, 'khanun': 1, 'talim': 1, 'dheere': 3, 'disfigured': 1, 'wins': 15, 'guarding': 8, 'mats': 1, 'professor': 15, 'talal': 1, 'sattar': 1, 'qassem': 2, 'suha': 1, 'kidwa': 6, 'asks': 6, 'dordain': 1, 'payloads': 2, 'updates': 5, 'exomars': 2, 'capped': 4, '260': 5, 'slick': 13, 'shoigu': 1, 'songhua': 9, 'flowed': 2, 'poured': 9, 'harbin': 10, 'poisons': 3, 'benzene': 11, 'meteorological': 9, 'foul': 1, 'interruption': 1, 'diluted': 3, 'outed': 1, 'wilson': 15, 'sad': 1, 'revelation': 1, 'underscored': 3, 'defiled': 1, 'diligence': 1, 'commended': 4, 'advisory': 10, 'adopting': 2, 'resolutely': 3, 'consultative': 2, 'scrapping': 2, 'choco': 1, 'exploratory': 3, 'euros': 5, 'wines': 1, 'dreamliner': 3, '00e': 1, 'zuloaga': 4, 'globovision': 2, 'airasia': 1, 'nippon': 3, 'hamdania': 3, 'pendleton': 2, 'assaults': 12, 'trigger': 7, 'gomhouria': 1, 'trumped': 2, 'ralia': 1, 'thrived': 1, 'frequency': 5, 'mhz': 1, 'khz': 2, '1030': 1, 'helpful': 6, 'cranks': 1, 'kadhim': 1, 'suraiwi': 2, 'thayer': 1, 'abid': 2, 'defacate': 1, 'chained': 2, 'allege': 14, 'finely': 1, 'orbiter': 3, 'armchairs': 1, 'punitive': 1, 'orbit': 8, 'theorize': 1, 'harassing': 3, 'petro': 3, 'verbytsky': 1, 'crimean': 5, 'succumbed': 3, 'embankment': 2, 'winfield': 1, 'sandbag': 1, 'reinforced': 5, 'levee': 7, 'peyton': 4, 'inundate': 1, 'embankments': 1, 'ruining': 1, 'soybeans': 1, 'skyrocketed': 1, 'royalists': 1, 'sahafi': 1, 'onboard': 4, 'stadiums': 9, 'abundance': 3, 'oakland': 3, 'nfl': 6, 'mentions': 2, 'involve': 10, 'equus': 3, 'preference': 3, 'liquidation': 1, 'accrue': 2, 'dividends': 1, 'paso': 5, 'boots': 2, 'redeemed': 1, '1652': 1, 'spice': 1, 'boers': 2, 'trekked': 1, 'subjugation': 1, '1886': 2, 'encroachments': 1, 'boer': 1, 'afrikaners': 1, '1910': 6, '1899': 2, 'mandela': 14, 'anc': 9, 'nelson': 9, 'boycotts': 2, 'ushered': 12, 'imbalances': 5, 'decent': 5, 'kgalema': 1, 'motlanthe': 1, 'locomotives': 3, 'santos': 8, 'promarket': 1, 'underemployment': 5, 'narcotrafficking': 1, 'exporters': 8, 'oceans': 8, 'delimited': 1, 'waterways': 4, 'thinned': 2, 'dukedom': 2, 'constitutionally': 3, '1603': 2, 'shogunate': 1, 'tokugawa': 1, 'flowering': 1, 'intensively': 3, 'kanagawa': 1, 'modernize': 2, '1854': 2, 'industrialize': 1, 'sakhalin': 2, 'formosa': 1, '1931': 1, 'manchuria': 1, '1937': 6, 'honshu': 1, 'saibou': 1, 'standstill': 3, 'bare': 4, 'col': 6, 'gupta': 2, 'madhuri': 1, 'duration': 1, 'minimal': 4, 'sahel': 1, 'agrarian': 4, 'nigerien': 1, 'tuareg': 5, 'hay': 8, 'snapping': 1, 'oxen': 4, 'growling': 1, 'selfish': 2, 'gentle': 1, 'tyrannical': 1, 'wrathful': 1, 'reign': 3, 'panther': 1, 'wolf': 21, 'lamb': 4, 'amity': 1, 'oh': 4, 'shall': 6, 'longed': 2, 'devouring': 2, 'swooped': 3, 'coils': 1, 'enabling': 4, 'spat': 1, 'thirst': 4, 'exertions': 2, 'slake': 1, 'draught': 1, 'deserves': 3, 'certificate': 4, 'softening': 4, 'juror': 1, 'afflicted': 3, 'excused': 2, 'gentleman': 2, 'housecat': 1, 'enlist': 2, 'furred': 1, 'aswan': 2, 'setback': 9, 'abul': 2, 'appreciates': 2, 'pretext': 4, 'unimaginably': 1, 'armistice': 6, 'lurking': 1, 'denunciations': 2, 'yitzhak': 1, 'leah': 1, 'rabin': 2, 'paint': 6, 'herzl': 1, 'neo': 2, 'hail': 5, 'defaced': 1, 'theodore': 2, 'beilin': 1, 'gurion': 1, 'graveyard': 1, 'vandalism': 4, 'rooting': 7, 'sadah': 1, 'newlands': 2, 'chanderpaul': 2, 'shivnarine': 1, 'samuels': 3, '214': 1, 'crease': 3, '297': 2, 'dale': 4, 'ntini': 1, 'makhaya': 1, 'wickets': 14, 'steyn': 2, 'durban': 5, 'sung': 8, 'gov': 2, 'www': 2, 'cn': 1, 'bucca': 1, 'biographies': 1, 'reassured': 4, 'buyers': 7, 'aig': 6, 'mv': 9, 'towed': 3, 'miscommunication': 1, 'offloaded': 1, 'tighten': 14, 'cetacean': 1, 'crewmembers': 3, 'acid': 6, 'boarding': 6, 'antarctica': 11, 'forbid': 4, 'cabeza': 1, 'gonzales': 22, 'vaca': 1, 'prompt': 6, 'photographed': 6, 'publications': 7, 'livingstone': 2, 'lusaka': 2, 'shoko': 1, 'cooked': 7, 'improperly': 5, 'unconditionally': 3, 'mediated': 6, 'muallem': 1, 'sheiria': 1, 'retake': 3, 'effigies': 2, 'qualified': 11, 'baluch': 9, 'experiences': 3, 'warmer': 5, 'humidity': 2, 'transmitting': 2, 'interrupt': 3, 'jailing': 3, 'seif': 2, 'riad': 1, 'faulting': 2, 'energetic': 2, 'franks': 3, 'tommy': 8, 'menem': 6, 'thales': 2, 'spectrum': 5, 'dovonou': 1, 'porto': 1, 'adjarra': 1, 'cotonou': 2, 'novo': 2, '7th': 2, 'precautionary': 2, 'infecting': 4, 'wiesenthal': 7, 'scheussel': 1, 'tributes': 2, 'tireless': 3, 'immunity': 8, 'eichmann': 2, 'adolf': 3, 'bombardment': 5, 'pelting': 1, 'eggs': 16, 'incapacitating': 1, 'azhar': 6, 'haifa': 5, 'shiller': 2, 'poors': 1, 'subprime': 2, 'homeowners': 13, 'shrinking': 5, 'detroit': 9, 'solving': 2, 'paused': 1, 'usaid': 4, 'oxfam': 10, 'grenoble': 2, 'streetcar': 1, 'robber': 2, 'monde': 5, 'longwang': 4, 'swirling': 2, 'pounding': 5, 'valenzuela': 3, 'departs': 1, 'tecnica': 1, 'loja': 1, 'andes': 1, 'universidad': 1, 'cartagena': 4, 'kleinkirchheim': 2, 'klammer': 2, 'nike': 1, '068472222': 1, 'franz': 3, 'michaela': 2, 'dorfmeister': 3, 'downhills': 1, 'finishes': 2, 'anja': 2, '882': 1, 'paerson': 4, 'differ': 4, '592': 1, 'khristenko': 2, 'versions': 3, 'panetta': 2, 'obeidi': 3, 'bribe': 3, 'catch': 16, 'installment': 4, 'evacuees': 9, 'presses': 1, 'lembe': 2, 'bukavu': 2, 'kenyon': 2, 'jensen': 1, 'methods': 13, 'phuket': 1, 'downplaying': 3, 'tornadoes': 2, 'tornado': 3, 'yazoo': 1, 'haley': 3, 'counties': 2, 'barbour': 3, 'incomplete': 1, 'reburied': 2, 'anup': 1, 'requiring': 8, 'raj': 1, 'sharma': 4, 'flush': 9, 'ngos': 6, 'abdulkadir': 5, 'jabril': 1, 'abdulle': 1, 'salaam': 5, 'es': 5, 'dar': 6, 'malindi': 3, 'mauritian': 1, 'rodrigues': 2, 'scares': 2, 'grouper': 1, 'malachite': 2, 'determines': 2, 'eels': 1, 'fattah': 1, 'chaka': 1, 'fills': 1, 'rhode': 2, 'vermont': 3, 'gunfights': 1, 'copacabana': 1, 'ipanema': 1, 'legalize': 1, 'shen': 1, 'kuo': 3, 'tai': 2, 'bergersen': 3, 'gregg': 1, 'jawid': 1, 'qiang': 2, 'unexpected': 7, 'wei': 5, 'reshuffle': 8, 'tichaona': 1, 'jokonya': 5, 'moyo': 7, 'traitors': 1, 'katzenellenbogen': 2, 'withered': 1, 'dongzhou': 3, 'escorting': 4, 'reckless': 4, 'helpe': 1, 'monument': 14, 'savannah': 3, 'erect': 2, 'volontaires': 1, 'regiment': 5, 'domingue': 1, 'chasseurs': 1, '1779': 1, 'tremendous': 5, 'debut': 7, 'georgetown': 3, 'churns': 3, 'beta': 10, 'sofia': 4, 'ulent': 1, 'turb': 1, 'osce': 5, 'passy': 1, 'surpass': 2, '2035': 1, 'regardless': 5, 'keidel': 2, 'albert': 4, 'carnegie': 2, 'endowment': 2, 'tampering': 6, 'negligence': 8, 'sahrawi': 1, 'coordinating': 7, 'casablanca': 5, 'stupak': 1, 'gauging': 2, 'bart': 1, 'pricing': 6, 'camila': 2, 'vegas': 3, 'guerra': 2, 'grammyawards': 1, 'las': 7, 'mario': 6, 'domm': 1, 'mientes': 1, 'samo': 1, 'amar': 1, 'dejarte': 1, 'comprised': 4, 'parra': 1, 'guerraas': 1, 'fukuoko': 1, 'grammys': 4, 'bachata': 1, 'univision': 1, 'hilary': 6, 'duff': 3, 'incessant': 2, 'judgmental': 1, 'starlet': 1, 'realizes': 3, 'girlfriend': 9, 'gypsy': 2, 'dignity': 4, 'richie': 1, 'deter': 11, 'logs': 2, 'mindful': 3, 'jiaxuan': 1, 'orderly': 4, 'councilor': 1, 'issuing': 5, 'lehman': 1, 'ubs': 1, 'stearns': 1, 'toure': 9, 'renowed': 1, 'farka': 1, 'sounds': 3, 'guitar': 4, 'genre': 1, 'praise': 11, 'legend': 8, 'ry': 2, 'cooder': 2, 'timbuktu': 1, 'grammy': 6, 'beloved': 2, 'belet': 1, 'weyn': 1, 'clans': 5, 'anarchy': 3, 'brick': 2, 'walls': 14, 'rooms': 7, 'tasks': 3, 'dextre': 3, 'destiny': 2, 'endeavour': 4, 'compartment': 1, 'latifiya': 1, 'hafidh': 1, 'closes': 3, 'salvadoran': 4, 'deferring': 1, 'disband': 2, 'amani': 1, 'havoc': 3, 'lanes': 2, 'wreaking': 1, 'faire': 1, 'laissez': 1, 'intellectual': 4, 'archaic': 1, 'entrepot': 1, 'rebuilt': 4, 'enterprises': 11, 'receipt': 7, 'rafiq': 4, 'reining': 3, 'expenditures': 7, 'ballooning': 1, 'conditioned': 1, 'exploitable': 4, 'mayen': 1, 'bbl': 1, 'manigat': 3, 'savimbi': 3, 'jonas': 2, 'arrears': 2, 'accrued': 1, 'peg': 4, 'kwanza': 1, 'depreciated': 1, 'angolan': 5, 'possesses': 3, 'fishery': 1, 'imbalance': 8, 'rutile': 1, 'revival': 3, 'protectorates': 1, '1942': 3, 'baker': 6, 'malaya': 1, '1957': 4, 'borneo': 2, 'sarawak': 1, 'mahathir': 9, 'razak': 3, 'najib': 8, 'armourer': 1, 'wanderings': 2, 'pricked': 2, 'glided': 2, 'wrath': 4, 'fangs': 1, 'dart': 1, 'insensible': 1, 'useless': 2, 'salesmen': 1, 'dina': 1, 'cody': 1, 'slumps': 1, 'troubles': 7, 'bamako': 3, 'hammered': 4, 'min': 2, 'oceania': 1, 'ashr': 2, 'jem': 3, 'khalil': 3, 'incidences': 1, 'forcible': 1, 'funerals': 6, 'notices': 3, 'interpol': 12, 'mabhouh': 1, 'circulate': 1, 'hydara': 2, 'deyda': 1, 'indirectly': 5, 'zakayev': 1, 'akhmed': 1, 'berezovsky': 1, 'soothe': 1, 'greenest': 1, 'zelaya': 12, 'tsang': 5, 'h9n2': 1, 'subtypes': 1, 'hoax': 6, 'martinez': 2, 'wardak': 7, 'warren': 3, 'buffett': 3, 'micheletti': 4, 'intent': 5, 'mansehra': 1, 'balakot': 2, 'reclusive': 4, 'frees': 2, 'sort': 6, 'emerges': 1, 'jade': 2, 'lots': 1, 'nargis': 6, 'rubies': 1, 'earner': 4, 'auctions': 1, 'jewelry': 6, 'paulo': 10, 'nishin': 1, 'seemed': 7, 'poised': 6, 'ying': 2, 'ma': 10, 'jeou': 2, 'hsieh': 3, 'jeopardy': 2, 'impeachment': 12, 'legality': 6, 'andras': 3, 'batiz': 3, 'ruegen': 5, '012': 2, 'maharastra': 2, 'guirassy': 2, 'asphyxiated': 1, 'senegalese': 9, 'salvatore': 1, 'sagues': 1, 'prosecutions': 1, 'thorough': 6, 'rattled': 3, 'kandill': 1, 'bingol': 3, 'karliova': 2, 'atop': 9, 'tectonic': 2, 'faultline': 1, 'mercenaries': 2, 'chenembiri': 1, 'bhunu': 1, 'ranged': 1, 'teodoro': 2, 'nguema': 1, 'obiang': 1, 'unspoiled': 1, 'nowicki': 1, 'maciej': 1, 'eagles': 3, 'wolves': 2, 'rospuda': 1, 'lynx': 1, 'pristine': 1, 'pillars': 2, 'baltica': 2, 'bog': 1, 'peat': 2, 'augustow': 1, 'abductees': 5, 'nadia': 3, 'fayoum': 1, 'michalak': 1, 'thuan': 1, 'clouded': 1, 'harass': 1, 'venues': 3, 'theo': 4, 'hofstad': 1, 'bouyeri': 4, 'nansan': 1, 'kokang': 3, 'brader': 1, 'levin': 3, 'sates': 2, 'soothing': 1, 'normalization': 4, 'hui': 2, 'teng': 1, 'sighted': 4, 'frontieres': 3, 'medecins': 4, 'sans': 3, 'perpetrating': 1, 'cohesion': 2, 'labado': 3, 'bihar': 4, 'erkki': 1, 'tuomioja': 1, 'influx': 3, 'insulza': 3, 'derbez': 4, 'eighteen': 4, 'oas': 9, 'recessed': 3, 'stored': 9, 'haisori': 1, 'destabilization': 1, 'ngabo': 3, 'kayumba': 1, 'faustin': 1, 'rugigana': 1, 'nyamwasa': 4, 'ottawa': 5, 'forbes': 7, 'benn': 4, 'develops': 1, 'douri': 1, 'izzat': 1, 'hasina': 3, 'inhuman': 3, 'dock': 4, 'ration': 1, 'chiao': 2, 'cosmonaut': 1, 'salizhan': 2, 'leroy': 2, 'sharipov': 2, 'professors': 3, 'scorpions': 4, 'males': 6, 'branko': 2, 'grujic': 1, 'octopus': 3, 'decay': 2, 'decadence': 1, 'aspire': 1, 'psychic': 2, 'perfection': 1, 'oberhausen': 1, 'legged': 1, 'mussels': 1, 'creature': 3, 'susanne': 5, 'osthoff': 6, 'archaeologist': 6, 'peacemaker': 1, 'reconstruct': 4, 'parks': 4, 'ideas': 9, 'reconstructing': 1, 'prone': 8, 'counternarcotics': 3, 'shaanxi': 6, 'zichang': 1, 'wayaobao': 1, 'expeditions': 3, 'howells': 1, '277': 1, 'automatically': 8, 'supamongkhon': 1, 'kantathi': 1, 'garlicky': 1, 'zero': 11, 'kimchi': 2, 'epitomizes': 1, 'spicy': 1, 'cabbage': 2, 'dish': 2, 'achin': 2, 'adamou': 1, 'cuvette': 1, 'yada': 1, 'alzouma': 1, 'hemorrhagic': 6, 'bleeding': 7, 'heath': 1, 'sarath': 2, 'bandaranaike': 1, 'amunugama': 1, 'anura': 1, 'portfolios': 2, 'peril': 1, 'mediocre': 1, 'essay': 2, 'isaiah': 1, 'planner': 4, 'toppling': 3, 'unpublished': 1, 'memoir': 4, 'authored': 1, '294': 1, 'surpasses': 1, '2927': 1, 'rear': 2, 'firecrackers': 3, 'downward': 11, 'polarize': 1, 'symbolic': 4, 'renault': 6, 'interlagos': 1, '060092593': 1, 'mclaren': 2, 'mercedes': 2, 'wurz': 2, 'clocked': 2, '050474537': 1, 'kimi': 1, 'raikkonen': 2, '193': 4, 'trails': 2, 'realistically': 1, 'ferrari': 5, 'finish': 20, 'schumacher': 1, 'falcon': 2, 'quails': 1, 'intrusion': 6, 'heels': 7, 'drifted': 2, 'prolific': 1, 'hispaniola': 4, 'bore': 4, 'frayed': 2, 'shunned': 2, 'summits': 4, 'jovic': 3, 'functional': 1, 'joao': 1, 'vieira': 3, 'billboard': 1, 'vocals': 1, 'lamm': 1, 'loughnane': 1, 'trumpet': 2, 'larry': 4, 'daya': 2, 'sandagiri': 1, 'malam': 1, 'bacai': 1, 'nino': 2, 'sanha': 2, 'bandung': 2, 'toddler': 1, '698': 2, 'qinghai': 9, 'yushu': 1, 'guangrong': 1, 'thoughts': 5, 'kamau': 1, 'luggage': 3, 'pistols': 3, 'artur': 1, 'trafficker': 3, 'salinas': 2, 'edgar': 2, 'marina': 1, 'otalora': 1, 'finances': 9, 'norte': 2, 'contrasted': 2, 'ludicrous': 1, 'condoleeza': 1, 'campo': 2, 'raiding': 2, 'barricaded': 1, 'shangla': 1, 'naeemi': 1, 'sarfraz': 1, 'dera': 12, 'dd': 4, 'cara': 2, 'unicorp': 2, 'kingsbridge': 1, 'donuts': 1, "dunkin'": 4, 'pill': 8, 'dunkin': 1, 'delaware': 6, '468': 1, 'randolph': 1, 'wishing': 11, 'relax': 3, 'defection': 2, 'fiber': 3, 'virtually': 11, 'casinos': 1, 'cepa': 1, 'pataca': 1, 'palm': 4, 'profited': 2, 'petronas': 2, 'riskier': 1, 'decreasing': 4, 'malays': 2, 'revisions': 1, 'polynesian': 5, '1889': 1, '1925': 2, 'ccamlr': 1, '586': 1, 'euphausia': 1, '396': 1, 'toothfish': 2, '027': 1, '591': 1, 'eleginoides': 1, 'superba': 1, '910': 1, 'dissostichus': 1, 'bass': 1, 'patagonian': 2, '376': 2, 'unregulated': 1, 'overflights': 3, 'iaato': 1, 'operators': 8, '552': 2, '799': 1, '213': 3, 'guarantor': 2, 'uniosil': 1, 'sideline': 1, 'stamping': 2, 'furthering': 1, '1936': 4, 'dynamic': 5, 'franco': 4, 'fatherland': 1, 'curled': 1, 'wintertime': 1, 'kites': 3, 'tee': 1, 'olden': 1, 'enchanted': 1, 'imitate': 1, 'neigh': 1, 'dull': 1, 'industrious': 1, 'indolent': 2, 'brilliant': 2, 'writers': 5, 'compiler': 1, 'poetry': 1, 'hog': 2, 'sixteen': 4, 'seventy': 4, 'honoured': 1, 'volumes': 1, 'tabulated': 1, 'crowed': 2, 'lustily': 1, 'cocks': 2, 'skulked': 2, 'hawk': 9, 'goeth': 1, 'behold': 1, 'vanquished': 3, 'calamitously': 1, 'boasting': 2, 'cock': 3, 'dwell': 1, 'prowl': 1, 'whichever': 1, 'quarrelling': 1, 'corner': 4, '138': 3, 'pep': 1, 'marjayoun': 2, 'tzipi': 4, 'livni': 10, 'redirect': 1, 'groomed': 1, 'spam': 5, 'sim': 1, 'telecom': 3, 'unicom': 1, 'somewhere': 9, 'gallon': 4, 'declarations': 2, '628': 1, 'westerly': 1, 'kewell': 3, 'referee': 4, 'animated': 4, 'aussies': 3, 'siegler': 1, 'liverpool': 6, 'inconsistent': 3, 'markus': 2, 'insulted': 2, 'merk': 3, 'fouls': 1, 'stuff': 3, 'handelsblad': 1, 'morsink': 2, 'nrc': 1, 'henk': 1, 'thank': 5, 'forested': 1, 'hyderabad': 5, 'zedong': 3, 'lays': 1, 'empress': 3, 'akihito': 5, 'michiko': 2, 'saipan': 2, '1944': 5, 'mudslide': 8, 'slide': 8, 'fading': 2, 'shanxi': 6, 'reservoir': 5, 'plowed': 3, 'torrent': 2, 'vista': 2, 'buena': 1, 'orlando': 1, 'bassist': 2, 'cachaito': 1, 'prostate': 3, 'glory': 5, 'segundo': 1, 'singers': 4, 'compay': 1, 'gonzalez': 2, 'ruben': 2, 'acclaim': 2, 'bright': 3, 'chopan': 3, 'dai': 3, 'manhunt': 6, 'nurja': 1, 'memin': 2, 'pinguin': 2, 'exaggerated': 3, 'lips': 4, 'depicts': 3, 'racist': 3, 'jesse': 3, 'contribution': 12, 'purchases': 8, 'sa': 4, 'sooner': 6, 'armitage': 7, 'exxonmobil': 8, 'conocophillips': 5, 'nationalize': 12, 'unwanted': 4, 'reality': 9, 'euthanized': 1, 'pets': 1, 'immensely': 2, 'expertise': 4, 'clinics': 5, 'dwyer': 6, 'ruth': 3, 'turk': 5, 'cares': 1, 'grief': 5, 'knows': 8, 'offended': 2, 'demeanor': 2, 'hindawi': 1, 'duluiya': 1, 'conservationists': 2, 'projected': 10, 'machinea': 1, 'yabello': 2, 'shakiso': 2, 'borena': 2, 'arero': 2, 'guji': 2, 'taadhii': 1, 'jaatanni': 1, 'sided': 5, 'virunga': 2, 'segway': 2, 'scooter': 3, 'upright': 1, 'gyroscopes': 1, 'tricky': 1, 'scooters': 1, 'eavesdropping': 5, 'boundaries': 7, 'warrantless': 1, 'minster': 8, 'lowers': 2, 'jing': 2, 'mascots': 1, 'poaching': 3, 'bunia': 3, 'floribert': 1, 'integrationist': 1, 'ndjabu': 1, 'precedence': 1, 'rigoberta': 1, 'menchu': 1, 'guatemalan': 4, 'rulings': 5, 'miroslav': 4, 'bralo': 3, 'jokers': 1, 'forcers': 1, 'nastase': 4, 'contradicting': 1, 'lifetime': 7, 'dwain': 2, 'cheats': 1, 'najim': 3, 'dhia': 1, 'freelance': 2, 'pensioners': 4, 'rodina': 2, 'mironov': 3, 'maneuver': 4, 'impaired': 2, 'visually': 1, 'assimilating': 1, 'blind': 6, 'ancic': 5, 'ordina': 1, 'bosch': 4, 'den': 1, 'taping': 1, 'llodra': 2, 'klara': 1, 'countrywoman': 2, 'koukalova': 2, 'macedonian': 10, 'boskovski': 4, 'ljube': 1, 'ljubotno': 1, 'skopje': 1, 'johan': 2, 'tarulovski': 2, 'foodstuffs': 2, 'malcolm': 2, 'radisson': 1, 'reception': 2, 'confession': 8, 'chee': 4, 'hwa': 4, 'tung': 17, 'cppcc': 3, 'useful': 3, 'deploys': 2, 'retaliatory': 3, 'militarization': 1, 'categorically': 3, 'telecast': 1, 'hekmatyar': 5, 'gulbuddin': 3, 'islami': 4, 'weaponization': 1, 'achievement': 7, 'conferring': 2, 'singnaghi': 1, 'striving': 2, 'aspirations': 6, 'tugboats': 1, 'mavi': 1, 'marmaraout': 1, 'ashdod': 2, 'commandeered': 3, 'emmanuel': 5, 'akitani': 5, 'neuilly': 1, 'olympio': 2, 'gilchrist': 2, 'andiwal': 2, 'marja': 1, 'jalani': 1, 'apologizes': 2, 'kish': 1, 'levinson': 2, 'duffle': 1, 'locating': 2, '218': 4, '243': 2, '131': 3, 'ashwell': 1, 'overs': 9, 'bowler': 8, 'jerome': 1, 'dwayne': 1, 'centurion': 2, 'germ': 1, 'anthrax': 2, 'amash': 1, 'saleh': 12, 'rihab': 1, 'huda': 1, 'marwa': 1, 'schultz': 3, 'demonstrates': 3, 'conventions': 4, 'brookings': 1, 'impacts': 2, 'camaraderie': 1, 'outdoors': 1, 'noormohammadi': 1, 'monaliza': 1, 'endangers': 3, 'porous': 1, 'husaybah': 5, '320': 5, 'thunderous': 1, 'arish': 3, 'dynamite': 2, 'ashkelon': 4, 'sderot': 2, 'uthmani': 1, 'funnel': 1, 'southerners': 2, 'pavarotti': 3, 'opera': 7, 'luciano': 1, 'modena': 1, 'polyclinic': 1, 'pancreatic': 1, 'tenor': 1, 'aficionados': 1, 'celebrity': 6, 'cutoff': 2, 'beattie': 4, 'reassuring': 2, 'boiled': 2, 'wang': 5, 'minghe': 1, 'upstream': 3, 'salad': 1, 'nur': 4, 'mohamud': 5, 'adaado': 3, 'posting': 5, 'sixty': 5, 'talent': 2, 'crooner': 1, 'suggestion': 8, 'shrugged': 1, 'suichuan': 1, 'jiangxi': 3, 'shaikan': 1, 'akre': 1, 'sarta': 1, 'dihok': 1, 'rovi': 1, 'mala': 1, 'bijeel': 1, 'shorish': 1, 'aegean': 4, 'measuring': 5, 'quakes': 2, 'chimneys': 1, 'embarrased': 1, 'halftime': 1, 'jacksonville': 1, 'performer': 1, 'janet': 6, 'timberlake': 4, 'costume': 3, 'mccarthy': 1, 'facets': 1, 'sporting': 6, 'contenders': 1, 'baya': 1, 'beiji': 2, 'faraj': 2, 'paulos': 2, 'rahho': 1, 'nineveh': 2, 'blackmailed': 2, 'archeologist': 1, 'energize': 1, 'verifiably': 1, 'catherine': 7, 'baber': 2, 'mike': 16, 'mcdaniel': 2, 'floodwaters': 13, 'spills': 6, 'pontchartrain': 1, 'ecosystems': 2, 'megumi': 1, 'yokota': 2, 'faking': 1, 'mocks': 1, 'convene': 3, 'duarte': 3, '340': 2, 'alfonso': 2, 'logos': 2, '223': 3, 'ripping': 4, 'mobilize': 7, 'hamesh': 1, 'koreb': 1, 'detonators': 2, 'trawler': 1, 'hmawbi': 1, 'township': 4, 'faiths': 7, 'joyful': 5, 'gracious': 1, 'bijbehera': 1, 'almagro': 1, 'amor': 1, 'newmont': 3, 'buyat': 1, 'arsenic': 2, 'ghazi': 5, 'aridi': 1, 'bugojno': 1, 'diver': 2, 'hamadi': 4, 'parole': 5, 'focal': 3, 'twa': 1, 'stethem': 1, 'lovers': 1, '410': 1, 'guinness': 1, 'yerevan': 1, 'candy': 3, 'alessandria': 1, '587': 1, 'dufour': 1, 'novi': 1, 'piemonte': 1, 'elah': 1, 'savin': 2, 'cent': 3, 'nonrecurring': 1, 'conn': 2, 'adjustments': 2, 'valuation': 1, 'magnified': 1, 'stamford': 1, 'segments': 4, 'principally': 4, 'geodynamic': 1, 'astypaleia': 1, 'kos': 1, 'excels': 1, 'sufficiency': 1, 'contractions': 1, 'stimulated': 2, 'surpluses': 3, 'adjustment': 4, 'khmers': 1, 'descendants': 7, 'angkor': 1, 'cambodians': 6, 'cham': 1, 'ushering': 2, '1863': 6, '1887': 1, 'indochina': 2, 'phnom': 5, 'penh': 5, 'pot': 1, 'hardships': 2, 'pol': 3, 'normalcy': 1, 'semblance': 2, 'contending': 1, 'sihamoni': 1, 'sihanouk': 1, 'norodom': 1, 'aboriginal': 2, '1770': 2, 'capt': 2, 'alam': 4, 'qom': 2, 'ageing': 1, 'amounted': 3, 'fy06': 1, 'earns': 1, 'ascension': 2, 'falklands': 3, 'dhekelia': 4, 'perceiving': 2, 'fold': 3, 'penning': 1, 'tortoise': 9, 'antagonist': 1, 'leisurely': 1, 'fatigue': 4, 'wayside': 3, 'sauntering': 1, 'cheer': 1, 'foxes': 3, 'schemed': 1, 'deprivation': 2, 'shame': 3, 'tailless': 1, 'brush': 1, 'interrupting': 4, 'spider': 3, 'spun': 1, 'thriller': 2, 'grossing': 2, 'shahab': 1, 'tallies': 3, 'entries': 3, 'greg': 3, 'schulte': 3, 'pedophile': 4, 'ideological': 2, 'easter': 12, 'gossip': 2, 'colom': 6, 'hamilton': 2, 'hayek': 2, 'valentina': 1, 'paloma': 1, 'pinault': 3, 'salma': 1, 'henri': 4, 'ugly': 3, 'goldwyn': 1, 'metro': 6, 'ventanazul': 1, 'labels': 1, 'gucci': 1, 'balenciaga': 1, 'ppr': 1, 'puma': 1, 'divorce': 6, 'dismissing': 3, '159th': 1, 'paynesville': 1, 'congotown': 1, 'compatible': 2, 'volt': 2, 'myth': 5, 'antagonizes': 1, 'uncalculated': 1, 'needlessly': 1, 'melih': 1, 'gokcek': 1, 'drapchi': 1, 'nuns': 5, '16s': 2, 'oscar': 5, 'arias': 4, 'manual': 2, 'otton': 1, 'cafta': 10, 'comprises': 8, 'statute': 3, 'backdrop': 4, '351': 2, '187': 1, 'breeding': 3, 'heraldo': 1, 'munoz': 1, 'manipur': 2, 'concealed': 4, 'imphal': 1, 'okram': 1, 'ibobi': 1, '282': 1, 'symonds': 4, 'lbw': 1, 'hussey': 1, 'typically': 4, 'yousef': 3, 'phrase': 3, 'defenseless': 2, 'luo': 3, 'meng': 1, 'wrestler': 4, 'diuretic': 1, 'purge': 4, 'hua': 1, 'offenders': 7, 'michele': 5, 'alliot': 6, 'athlete': 2, 'ouyang': 1, 'swimmer': 1, 'steroid': 4, 'kunpeng': 1, '281': 1, 'gayle': 2, 'pitch': 3, 'paced': 1, 'fours': 5, 'batsman': 5, '267': 1, 'internationals': 2, 'authorites': 1, 'occured': 2, 'hilla': 8, 'outlaws': 1, 'bulava': 1, 'mutates': 3, 'penetrate': 2, 'stavropol': 1, 'rogge': 5, 'coe': 1, 'delanoe': 1, 'sebastian': 5, 'balkenende': 2, 'suite': 1, 'audiences': 12, 'colorful': 6, 'yimou': 1, 'dragons': 2, 'extravagant': 3, 'civilization': 3, 'theme': 6, 'torchbearer': 2, 'cauldron': 4, 'constructively': 2, 'jowhar': 2, 'perina': 1, 'slim': 6, 'simultaneously': 4, 'mohsen': 8, 'lowering': 5, 'seems': 7, 'spaniards': 6, 'artibonite': 1, 'medusa': 2, 'refocus': 1, 'beknazarov': 1, 'azimbek': 1, 'nepotism': 1, 'maksim': 2, 'rewards': 7, 'adolescence': 1, 'awakening': 2, 'sexuality': 2, 'examines': 2, 'adolescents': 1, 'elaine': 7, 'mok': 3, 'zheng': 2, 'advertisements': 5, 'ads': 2, 'reciprocate': 1, 'ad': 5, 'lipsky': 3, 'tower': 6, 'verifiable': 1, 'denuclearization': 2, 'donkey': 2, 'unanswered': 2, 'pouring': 4, 'locate': 10, 'pumps': 3, 'wanzhou': 1, 'hubei': 6, 'yichang': 1, 'downpours': 1, 'abkhaz': 6, 'mujhava': 1, 'outspoken': 8, 'chlorine': 4, 'commonplace': 1, 'reshape': 1, 'broadening': 1, 'ojedokun': 1, 'screeners': 1, 'baggage': 1, 'glut': 1, 'unsold': 1, 'henricsson': 2, 'ulf': 2, 'claudio': 2, 'fava': 1, 'giovanni': 1, 'tenet': 2, 'goss': 12, 'lawmakes': 1, 'porter': 6, 'voluntary': 3, 'overdrafts': 1, 'unwarranted': 2, 'kallenberger': 1, 'undisclosed': 11, 'bellinger': 1, 'deactivated': 2, 'suitcase': 4, 'anfo': 1, 'ammonium': 1, 'nitrate': 2, 'backups': 1, 'deactivate': 1, 'nikiforov': 2, 'allowance': 4, 'staffing': 2, 'uefa': 5, 'maccabi': 1, 'dinamo': 1, 'betar': 1, 'sums': 4, 'postponing': 5, 'nikolas': 1, 'clichy': 1, 'sous': 2, 'bois': 2, 'napolitano': 4, 'radicalization': 2, 'businesswomen': 1, 'flatly': 4, 'sinking': 3, 'kumar': 4, 'madhav': 2, 'memento': 1, 'sherpa': 1, 'unfurled': 1, 'banner': 6, 'rededicate': 1, 'undertaken': 3, 'fundraisers': 1, 'commemorated': 1, 'commemorate': 12, 'acquisitions': 3, 'yadana': 2, 'dharmeratnam': 3, 'sivaram': 7, 'tamilnet': 3, 'columnist': 3, 'mirror': 6, 'realtors': 2, 'chevron': 10, 'itinerary': 1, 'acknowledges': 7, 'helgesen': 2, 'thamilselvan': 1, 'vidar': 1, 'staying': 3, 'mackay': 2, 'contrasts': 2, 'vacationing': 7, 'rests': 4, 'gagra': 1, 'sukhumi': 2, 'snowstorm': 4, 'subzero': 1, 'nebraska': 6, 'kansas': 5, 'slippery': 1, 'motels': 1, 'disbursing': 3, 'millenium': 2, 'exclusion': 1, 'ndc': 4, 'haruna': 1, 'idrissu': 1, 'abidjan': 7, 'protectionist': 2, 'qin': 3, 'underwear': 2, 'knit': 2, 'trousers': 2, 'safeguards': 4, 'softened': 1, 'adopts': 1, 'jaua': 1, 'vedomosti': 2, 'speculating': 1, 'muifa': 2, 'paralyzing': 1, 'thien': 2, 'quang': 2, 'ngai': 2, 'thua': 2, 'tri': 1, 'hue': 2, 'daklak': 1, 'bonfoh': 2, 'boko': 6, 'suicidal': 1, 'detection': 3, 'nonprofit': 3, 'allots': 1, 'immorality': 1, 'offending': 2, 'repealed': 1, 'shoichi': 1, 'nakagawa': 1, 'penn': 1, 'speculated': 3, 'fights': 3, 'roundly': 1, 'testifies': 1, 'chertoff': 12, 'oversees': 8, 'kozulin': 8, 'hooliganism': 3, 'incitement': 4, 'hadson': 2, 'reimbursed': 1, 'amortization': 1, 'slave': 5, 'lingered': 1, 'melnichenko': 2, 'precipitated': 1, '1623': 1, 'rebelled': 1, 'anguilla': 4, 'nevis': 6, 'sekou': 1, 'unwillingness': 2, 'culminated': 4, 'conde': 1, 'konate': 1, 'sekouba': 1, 'pattern': 7, 'impressive': 8, 'nafta': 1, 'absorbs': 1, 'buffeted': 1, 'capitalization': 1, 'enthusiasm': 2, 'aux': 1, 'couch': 2, 'stuffing': 1, 'unabated': 4, 'dames': 1, 'quills': 1, 'damn': 3, 'echo': 2, 'wearily': 1, 'robbers': 3, 'wallets': 1, 'tellers': 2, 'etc': 1, 'gagged': 3, 'brutally': 5, 'whispers': 2, 'replies': 2, 'owe': 4, 'teacher': 13, 'plaster': 1, 'shirt': 6, 'noticeable': 1, 'busied': 1, 'rowdy': 1, 'classroom': 3, 'confidently': 1, 'stapled': 1, 'stapler': 1, 'flap': 1, 'breeze': 2, 'parkinson': 5, 'debilitating': 1, 'addendum': 1, 'duelfer': 5, 'policymakers': 4, '126': 3, 'debriefing': 1, 'villager': 3, 'discriminatory': 2, "b'tselem": 3, 'mekorot': 1, 'peoples': 14, 'rabat': 3, 'concerted': 4, 'bursa': 1, 'compatriots': 1, 'modifies': 1, 'enhances': 1, 'worry': 13, 'detriment': 1, 'enshrines': 2, '375': 3, 'ishac': 1, 'diwan': 1, 'wahab': 1, 'qusai': 1, 'bahrainian': 1, 'adds': 9, 'satisfies': 1, 'verhofstadt': 1, 'guy': 2, 'preaching': 7, 'sol': 1, 'tuzla': 1, 'gazans': 2, 'streamed': 2, 'gijs': 1, 'vries': 1, 'fostering': 2, 'muriel': 1, 'degauque': 1, 'yousaf': 2, 'anyama': 1, 'gendarmes': 1, 'newman': 1, 'connie': 3, 'flagrantly': 1, 'semester': 1, 'sizably': 2, 'tulane': 1, 'trailers': 1, 'seminar': 4, 'alerts': 2, 'moinuddin': 1, 'karnataka': 5, 'puri': 1, 'nationalization': 10, 'affirming': 1, 'giza': 1, 'fayyum': 1, 'eradicated': 3, 'lobbied': 2, "d'affaires": 2, 'velach': 2, 'filip': 1, 'tkachev': 1, 'krasnodar': 1, 'mtetwa': 2, 'beatrice': 1, 'norton': 1, 'dismantle': 20, 'gulu': 2, 'accreditation': 5, 'toby': 1, 'simmonds': 1, 'harnden': 1, 'budapest': 5, 'workings': 1, 'vetoes': 1, 'cooperated': 2, 'sorting': 1, 'ghalib': 2, 'kubba': 2, 'sabotaging': 1, 'adhere': 5, 'investigates': 2, 'schmit': 1, 'gestures': 5, 'zabayda': 1, 'chitchai': 1, 'wannasathit': 1, 'haiphong': 2, 'durango': 1, 'breakdown': 3, 'resembled': 1, 'benedetti': 2, 'incendiary': 1, 'pardo': 1, 'bab': 3, 'sharjee': 1, 'ragoussis': 1, 'frangiskos': 1, 'hudson': 4, 'blige': 2, 'jamie': 4, 'foxx': 3, 'pasadena': 2, 'unpredictable': 3, 'dreamgirls': 1, 'castoff': 1, 'cinderella': 1, 'naacp': 2, 'miro': 1, 'ashdown': 3, 'yousifiyah': 1, 'simplifying': 1, 'ohio': 14, 'ler': 1, 'csw': 2, 'minesweepers': 1, 'airdrops': 1, 'scaling': 3, 'karkh': 1, 'cropper': 3, 'arrange': 8, 'patrolled': 6, 'ogero': 1, 'automobiles': 3, 'hardenne': 7, 'compromised': 1, 'regaining': 1, 'ghangzhou': 1, 'ping': 1, 'homage': 3, 'chiang': 2, 'kun': 1, 'nangjing': 1, 'sen': 4, 'yat': 1, 'slideshow': 1, 'sgt': 1, 'whitney': 2, 'mounts': 1, 'newest': 6, 'surprisingly': 2, 'hate': 10, '74th': 1, 'biennial': 2, 'weaver': 6, 'jumbo': 2, 'researching': 1, 'overturn': 5, 'evaluation': 3, 'jagged': 1, 'malwiya': 1, 'architecture': 2, 'minarets': 2, 'abbassid': 1, 'spiral': 4, 'hatem': 2, 'edict': 3, 'effectiveness': 5, 'budgets': 2, 'recipient': 3, 'relieved': 5, 'baton': 3, 'thad': 3, 'hovered': 2, 'recalling': 4, 'alps': 1, 'unjustly': 2, 'closeness': 1, 'studded': 1, 'inducted': 3, 'multitudes': 1, 'transfusion': 1, 'traumatic': 2, 'explains': 4, '655': 2, 'understandable': 2, 'vincennes': 1, 'bandar': 6, 'lynyrd': 1, 'skynyrd': 1, 'inductees': 3, 'rockers': 1, 'kievenaar': 1, 'butch': 1, 'blondie': 1, 'opener': 5, 'warmup': 4, 'competent': 3, 'herb': 1, 'alpert': 1, 'trumpeter': 1, 'founders': 1, 'moss': 1, 'reside': 2, 'arghandab': 2, 'prematurely': 7, 'mitrovica': 3, 'seceded': 3, 'feith': 2, 'websites': 6, 'reunited': 5, 'lafayette': 1, 'novosibirsk': 1, 'disadvantaged': 3, 'stymied': 1, 'geese': 9, 'revoked': 7, 'scheuer': 2, 'inspires': 1, 'hubris': 1, 'anonymously': 1, 'inflamed': 2, 'flocks': 5, 'processed': 5, 'christ': 9, 'scouts': 2, 'kicking': 4, 'drums': 4, 'festive': 1, 'decked': 1, 'zhanjun': 1, 'blacklist': 2, 'accredited': 1, 'liu': 10, 'binjie': 1, 'amorim': 5, 'celso': 3, 'embraer': 1, 'ramping': 1, "'re": 4, 'sulphur': 2, "sh'ite": 1, 'huaren': 1, 'sheng': 2, 'booming': 10, 'simmons': 1, 'manas': 3, 'decisive': 4, 'fayyaz': 1, 'baqubah': 2, 'cobbled': 1, 'gillard': 2, 'mortgages': 1, 'foreclosures': 7, 'properties': 5, 'refinancing': 1, 'customized': 1, 'simulates': 1, 'sint': 6, 'fifths': 5, 'maarten': 5, 'juliana': 1, 'shiloh': 2, 'weisgan': 1, 'harbors': 2, 'antilles': 5, 'widening': 6, 'bahamian': 1, 'constitute': 5, 'enactment': 3, 'nyasaland': 1, '1964': 5, '1891': 2, 'kamuzu': 1, 'hastings': 1, 'dpp': 2, '1888': 2, '1900': 2, 'umpire': 1, 'sprat': 1, 'strolling': 2, 'lofty': 2, 'bunch': 3, 'vine': 4, 'orchard': 1, 'ripening': 1, 'quoth': 2, 'quench': 1, 'paces': 1, 'tempting': 1, 'morsel': 2, 'despise': 1, 'butlers': 1, 'seldom': 2, 'chauffeurs': 1, 'k': 7, 'drawer': 2, 'fifteen': 7, 'clone': 6, "'d": 2, 'backfired': 1, 'obscene': 1, 'mood': 4, 'tourniquet': 1, 'noticing': 1, 'muentefering': 2, 'recruiter': 2, 'slyly': 1, 'smiled': 3, 'mules': 2, 'dividing': 8, 'fractions': 1, 'hitched': 2, 'mule': 4, 'uncle': 6, 'nahles': 1, 'wasserhoevel': 1, 'andrea': 1, 'kajo': 1, 'trawlers': 2, 'solbes': 1, 'natsios': 6, 'constructing': 1, 'firehouses': 1, 'sonia': 5, 'titan': 7, 'presley': 3, 'memphis': 3, 'handgun': 4, 'elvis': 2, 'wesson': 1, 'mm': 1, 'graceland': 3, 'visitor': 1, 'unfastened': 1, 'beside': 5, 'journeyed': 1, 'magerovskiy': 2, 'sergey': 1, 'tiffany': 1, 'stiegler': 1, 'dancer': 2, "ba'ath": 2, 'naturalization': 1, 'rebecca': 1, 'tanith': 1, 'belbin': 1, 'transmitter': 2, 'shariat': 2, 'hymns': 3, 'shagh': 1, 'rages': 2, 'listeners': 3, 'flurry': 5, 'initialed': 1, 'valdes': 2, 'diarra': 1, 'colombant': 3, 'nico': 2, 'seydou': 1, 'thundershowers': 1, 'boeta': 1, 'nel': 1, 'rounder': 1, 'dippenaar': 1, 'bowled': 3, 'wong': 3, 'cannes': 6, 'wai': 1, 'sci': 1, 'stylized': 1, '2047': 1, 'fi': 1, 'bernando': 1, 'vavuniya': 1, 'beatle': 2, 'bogdanchikov': 1, 'yuganskneftegaz': 6, 'anorexia': 1, 'versace': 2, 'allegra': 1, 'donatella': 2, 'zimmerman': 1, 'estanged': 1, 'beck': 4, 'syndicated': 2, 'gaunt': 2, 'insider': 2, 'correspond': 1, 'ferrero': 4, 'ortiz': 4, 'mishandled': 2, 'batumi': 2, 'reforming': 3, 'inject': 1, 'facilitate': 7, 'qomi': 3, 'kazemi': 4, 'otri': 1, 'naji': 3, 'additionally': 7, 'pramoni': 1, 'plaque': 1, 'frechette': 1, 'louise': 2, 'courageous': 7, 'araque': 2, 'kiryenko': 2, 'shurja': 2, '616': 1, 'eden': 3, 'kolkata': 2, 'wasim': 2, 'jaffer': 4, 'laxman': 3, 'scoring': 8, 'tanvir': 1, 'delighted': 1, 'sohail': 1, 'holing': 1, 'dhoni': 1, 'wicket': 3, 'mahendra': 2, 'keeper': 2, 'waw': 1, 'vomiting': 6, 'kutesa': 3, 'barge': 1, 'looted': 2, 'trudging': 1, 'yorkers': 2, 'jogged': 1, 'biked': 1, 'defying': 4, 'museums': 4, 'costing': 3, 'earn': 5, 'nathalie': 2, 'dechy': 2, 'marta': 3, 'marrero': 2, 'golovin': 3, 'emilie': 1, 'pascual': 2, 'marion': 3, 'ruano': 1, 'loit': 1, 'bartoli': 2, 'bleach': 1, 'panicked': 4, 'restated': 4, 'cereals': 1, 'smerdon': 1, 'brink': 3, 'vukovar': 6, 'awaited': 4, 'veselin': 1, 'mile': 4, 'mrksic': 1, 'radic': 1, 'sljivancanin': 1, 'eprdf': 4, '537': 2, '534': 1, 'fossil': 4, 'fuels': 6, 'delegate': 3, 'reforestation': 1, 'cheating': 2, 'robby': 1, 'ginepri': 2, 'muller': 2, 'gilles': 3, 'yeu': 1, 'tzuoo': 1, 'paradorn': 2, 'srichaphan': 3, 'hero': 9, 'neurosurgeon': 1, 'fuji': 4, 'keizai': 1, 'nihon': 1, 'pioneered': 1, 'economical': 1, 'daimlerchrysler': 4, 'gwangju': 1, 'precaution': 6, 'h7': 2, '206': 3, 'congratulatory': 2, 'nikolic': 1, 'tomislav': 1, 'qua': 1, 'quagga': 2, 'extinction': 3, 'hunted': 2, 'zebra': 4, 'reinhold': 1, 'rau': 1, 'subspecies': 1, 'endeavor': 2, 'impeach': 2, 'yearlong': 2, 'pakitka': 1, 'thrust': 1, 'zabol': 2, 'submitting': 1, 'observance': 8, 'diocese': 1, 'rituals': 3, 'oils': 2, 'commemorating': 6, 'apostles': 2, 'supper': 2, 'biblical': 3, 'feet': 14, 'wash': 1, 'crucifixion': 3, 'liturgy': 1, 'resurrection': 2, 'frances': 5, 'copei': 4, 'liberians': 3, 'booth': 2, 'unchallenged': 3, 'disapproved': 1, 'almaty': 2, 'bolstering': 3, 'clamping': 1, 'peretz': 16, 'rightists': 1, 'coaltion': 1, 'ceramic': 1, 'enlisted': 3, 'jenita': 1, 'murugupillai': 1, 'couples': 4, 'jeyarajah': 1, 'crested': 1, 'myna': 2, 'galam': 1, 'obvious': 4, 'playground': 1, 'aviaries': 1, 'fabricated': 3, 'ghanem': 1, 'antoine': 2, 'deflecting': 1, 'llagostera': 1, 'nuria': 1, 'vives': 1, 'flavia': 2, 'schnyder': 2, 'patty': 2, 'draws': 6, 'pinera': 5, 'aip': 1, 'hidayat': 1, 'aryanto': 1, 'boedihardjo': 1, 'regency': 1, 'ciamis': 1, 'comparing': 6, 'firdaus': 1, 'salik': 1, 'misno': 1, 'arraigned': 2, 'lauro': 1, 'channels': 9, 'martinis': 1, 'fictional': 3, 'stirred': 6, 'savors': 1, 'clearance': 3, 'gary': 2, 'chef': 1, 'freer': 1, 'rep': 1, '558': 1, 'files': 4, 'clotting': 1, 'defendents': 1, 'zubeidi': 1, 'waivers': 2, 'debenture': 1, 'hasbrouk': 1, 'rms': 3, 'distributes': 2, '666': 1, '158': 1, 'zalkin': 1, 'austin': 4, '967': 1, '608': 1, '809': 1, '413': 1, 'principalities': 2, 'absorb': 1, 'mongol': 3, 'muscovy': 1, 'romanov': 1, '1682': 1, '1725': 1, 'hegemony': 1, 'russo': 3, '1904': 3, 'defeats': 1, 'lenin': 1, 'iosif': 1, 'stalin': 4, 'perestroika': 1, 'splintered': 2, 'inadvertently': 2, 'stagnated': 4, 'glasnost': 1, 'gorbachev': 2, 'legitimacy': 3, 'shifted': 3, 'buttressed': 1, 'carefully': 10, 'dislocation': 1, 'seaports': 1, '122': 4, 'uninhabitable': 3, 'antigua': 2, 'barbuda': 1, 'constrained': 7, 'bedding': 2, 'spencer': 1, 'topped': 3, 'antiguan': 1, 'niue': 4, 'yawning': 1, 'disadvantages': 1, 'abject': 1, 'engagement': 7, 'hemispheric': 2, 'equaling': 1, 'gardening': 1, 'reengagement': 1, 'mis': 1, 'outlays': 1, 'ant': 4, 'cream': 4, 'lime': 3, 'coconut': 1, 'passion': 3, 'honey': 4, 'bough': 1, 'pitied': 1, 'dove': 3, 'fowling': 1, 'lambs': 1, 'whelp': 2, 'pupil': 1, 'lookout': 1, 'famishing': 1, 'cottage': 3, 'babe': 1, 'sneered': 1, 'collectors': 2, 'compliment': 1, "i'd": 1, 'battlefield': 4, 'durbin': 3, 'machar': 3, 'riek': 1, 'homestead': 1, 'nyongwa': 1, 'ankunda': 1, 'proxy': 2, 'remarked': 1, 'weakly': 1, 'jolt': 1, 'aftershocks': 4, 'bakool': 4, 'hudur': 2, 'fy08': 1, 'masorin': 2, 'sevastopol': 4, 'tartus': 1, 'decimated': 1, 'doodipora': 1, 'azad': 1, 'nabi': 4, 'seated': 5, 'rezaei': 3, 'registers': 2, 'qalibaf': 2, 'mostafa': 3, 'moin': 2, 'baqer': 1, 'stun': 3, 'constitutions': 1, 'ecumenical': 1, 'constantinople': 3, 'saints': 5, 'relics': 5, 'bartholomew': 2, 'nazianzen': 1, 'chrysostom': 1, 'insurmountable': 2, '1054': 1, 'crusaders': 2, 'sacking': 1, '1204': 1, 'zurabishvili': 1, 'salome': 1, 'brunei': 9, 'inforamtion': 1, 'daunting': 3, 'institutionalizing': 1, 'najam': 2, 'weeklong': 5, 'clause': 3, 'collaborate': 2, 'dose': 2, 'squibb': 1, 'gilead': 2, 'briston': 2, 'doses': 6, 'sustiva': 1, 'viread': 1, 'emtriva': 1, 'replication': 1, 'apec': 18, 'malaki': 1, 'hiker': 1, 'shourd': 7, 'untied': 1, 'bittersweet': 1, 'bauer': 5, 'hikers': 8, 'fattal': 4, 'concertacion': 1, 'foxley': 1, 'velasco': 3, 'harvard': 2, 'conquered': 5, '1824': 1, 'yaqoob': 2, 'bot': 3, 'notari': 1, 'ne': 2, 'involuntarily': 1, 'tubes': 1, 'tombs': 6, 'artifacts': 2, 'zahi': 3, 'hawass': 5, 'servant': 2, 'saqqara': 3, 'murals': 1, 'excavation': 1, 'mummies': 1, 'coffins': 2, 'scribe': 1, 'motorized': 1, 'rickshaw': 1, 'enormous': 5, 'jurist': 2, 'abortion': 18, 'asfandyar': 2, 'marriott': 3, 'idle': 2, 'hello': 1, 'milk': 3, 'collectivization': 1, 'nationalizing': 2, 'russert': 3, 'prodemocracy': 1, 'chronicled': 1, 'eager': 5, 'suppressed': 5, 'rankings': 7, 'maternity': 2, 'skated': 1, 'undefeated': 3, 'moallem': 1, 'appalled': 1, 'barbarism': 1, 'husseinov': 5, 'elmar': 3, 'oppressive': 3, 'procession': 12, 'aliyev': 3, 'ilham': 4, 'provocation': 5, 'garnering': 2, 'inherit': 3, 'huygens': 5, 'saturn': 7, 'relaying': 3, 'cassini': 3, 'composition': 1, 'evolved': 7, 'docks': 1, 'rooftops': 3, 'armstrong': 4, 'banbury': 1, 'appointees': 2, 'roque': 5, 'lenghty': 1, 'hayam': 1, 'zahri': 1, 'genetic': 5, 'borroto': 1, 'feasibility': 3, 'expresses': 6, 'caldwell': 2, 'contemplating': 1, 'glittering': 1, 'egg': 5, 'electorate': 2, 'trick': 4, 'gemelli': 1, 'inflammation': 1, 'engagements': 3, 'muscles': 2, 'minya': 1, 'delight': 2, 'unsanitary': 1, 'helmets': 2, 'simulation': 1, 'followup': 1, 'chelyabinsk': 2, 'nonproliferation': 4, 'solidifies': 1, 'overpass': 3, "o'er": 1, 'reaches': 7, 'oft': 1, 'earning': 4, 'oprah': 1, 'jagger': 1, 'winfrey': 1, 'rounding': 2, 'woods': 3, 'hostess': 3, 'golfer': 1, 'descending': 1, 'mick': 1, 'longevity': 3, 'consitutional': 1, 'spearker': 1, 'misfortune': 2, 'doe': 2, 'breakthroughs': 3, 'abandoning': 5, 'reversible': 1, 'ghor': 3, 'invasive': 2, 'minimally': 1, 'embolization': 1, 'uterine': 2, 'fibroid': 2, 'hysterectomy': 1, 'tumors': 4, 'shrinks': 1, 'rowed': 1, 'fibroids': 1, 'heineman': 2, 'dave': 2, 'alimport': 1, 'qilla': 1, 'saifullah': 2, 'madrassa': 3, 'belongings': 2, 'uproot': 2, 'arrogance': 1, 'sponsor': 9, 'foxy': 3, 'rapper': 3, 'marchand': 1, 'inga': 1, 'spitting': 1, 'probation': 2, 'counseling': 1, 'manicurists': 1, 'schoolgirl': 3, 'plotter': 1, 'slightest': 1, 'indianapolis': 3, 'reassessed': 1, 'maligned': 1, '384': 1, 'supremacy': 1, 'unify': 2, 'malta': 13, 'horizons': 4, 'bereavement': 1, '2a': 2, 'haswa': 1, 'chalabi': 8, 'sii': 2, 'rightly': 5, 'flexibility': 6, 'jib': 1, 'dial': 1, 'stefan': 1, 'mellar': 1, 'deniers': 1, 'profane': 2, 'hikmet': 1, 'cetin': 2, 'rotation': 2, '°c': 1, 'upside': 3, 'pen': 2, 'writes': 3, 'chiyangwa': 3, 'mashonaland': 1, 'kenny': 3, 'tendai': 1, 'itai': 1, 'marchi': 1, 'matambanadzo': 1, 'godfrey': 1, 'dzvairo': 1, 'karidza': 1, 'naad': 1, 'inspecting': 2, 'mangwana': 1, 'disapproval': 3, 'krygyz': 1, 'sampling': 5, 'decorated': 2, 'dubrovka': 1, 'meridian': 5, 'feniger': 1, 'maclellan': 1, 'haden': 1, 'surrey': 1, '1912': 2, '1939': 5, 'partisans': 2, 'xenophobic': 1, 'combative': 1, 'deficiencies': 2, 'judged': 3, 'lsi': 1, 'dp': 5, 'sp': 1, 'wallachia': 1, 'suzerainty': 1, '1856': 2, '1859': 1, '1862': 1, 'moldavia': 1, '1878': 2, 'transylvania': 1, 'axis': 1, 'soviets': 1, 'abdication': 1, 'draconian': 2, 'securitate': 1, 'nicolae': 1, 'ceausescu': 2, 'principe': 3, 'concessional': 1, 'rescheduling': 1, 'prgf': 2, 'bonuses': 4, '1876': 1, 'nomadic': 2, 'incredible': 3, 'tsarist': 1, '1916': 2, 'akaev': 1, 'bakiev': 5, 'manipulated': 3, 'otunbaeva': 1, 'interethnic': 1, 'lark': 1, 'uninterred': 1, 'crest': 1, 'hillock': 1, 'monstrous': 1, 'stuck': 4, 'refrained': 1, 'answering': 4, 'mosquitoes': 2, 'lasting': 16, 'spraying': 2, 'ziemer': 2, 'posthumous': 1, 'comedian': 3, 'pryor': 3, 'deft': 1, 'nerve': 4, 'degenerative': 1, '1938': 2, 'haggard': 1, 'bowie': 1, 'merle': 1, 'jessye': 1, 'rent': 4, 'yonts': 2, 'latif': 6, 'hakimi': 10, 'chinooks': 1, 'rotor': 1, 'sandstorm': 4, 'hodan': 1, 'wadajir': 1, 'florence': 3, 'reconciling': 1, 'seacoast': 1, 'ambulance': 2, 'kusadasi': 1, 'tak': 2, 'qassam': 2, 'ezzedin': 1, 'conducts': 3, 'ravaging': 2, 'spawned': 2, 'smoldering': 1, 'parched': 1, 'smog': 1, 'brasilia': 5, 'proposes': 6, 'sonthi': 1, 'boonyaratglin': 1, 'extraditing': 3, 'influencing': 2, 'tolo': 3, 'rezayee': 2, 'shaima': 1, 'char': 1, 'bowing': 1, 'shaer': 3, '360': 3, 'bombard': 1, 'sheriff': 7, 'booked': 1, 'fingerprinted': 1, 'booking': 2, 'shrunk': 1, 'layoff': 1, 'citi': 1, 'replenish': 3, 'merrill': 2, 'brokerage': 2, 'lynch': 2, 'walks': 1, 'waning': 1, 'ferencevych': 3, 'memories': 2, 'turbulent': 5, 'mint': 1, 'definitive': 3, 'naha': 1, 'mouknass': 1, 'hartmann': 2, 'brig': 1, 'sexy': 1, 'pertains': 1, 'salim': 5, 'hamdan': 2, 'mental': 5, 'nasty': 1, 'spessart': 1, 'fsg': 1, 'pfalz': 1, 'rheinland': 1, 'frigate': 4, 'pak': 6, 'ui': 2, 'catwalk': 1, 'strutting': 1, 'models': 4, 'pittsburgh': 4, 'mellon': 1, 'imran': 3, 'siddiqi': 1, 'retained': 8, 'usual': 8, 'kyiv': 3, 'finalizing': 4, 'beechcraft': 1, 'turboprop': 2, 'zhulyany': 1, 'wooden': 6, 'phyu': 2, 'szmajdzinski': 3, 'jerzy': 4, 'chant': 2, 'confronted': 5, 'zionism': 3, 'ruhollah': 3, 'intoning': 1, 'khomeini': 3, 'stanisic': 3, 'mica': 1, 'outs': 3, 'adde': 1, 'peregrine': 1, 'zsolt': 1, 'gabcikovo': 1, 'grebe': 1, 'slovakian': 1, 'robredo': 4, 'upsets': 1, 'philipp': 2, 'kohlschreiber': 1, 'transformer': 1, 'kayettuli': 1, 'ayad': 4, 'gloomier': 1, 'crafting': 3, 'haris': 1, 'unsealed': 5, 'sadequee': 1, 'ehsanul': 1, 'coerced': 2, 'blackmail': 3, 'positioning': 1, 'iraqiya': 4, 'bheri': 1, 'surkhet': 1, 'cables': 3, 'takirambudde': 1, 'totals': 2, 'khalilzad': 12, 'zalmay': 8, 'firefighting': 1, 'arid': 3, 'razed': 2, 'workshop': 3, 'carpentry': 1, 'provoke': 6, 'kuomintang': 1, 'khim': 1, 'hsiao': 1, 'bi': 2, 'safehouse': 2, 'bombmaking': 1, 'blasphemy': 3, 'perwiz': 1, 'kambakhsh': 2, 'defaming': 3, 'reexamine': 1, 'punishable': 3, 'stipulated': 1, 'saarc': 3, 'dissolving': 2, '142': 3, 'baribari': 1, 'shoreline': 2, 'campaigns': 9, 'confusion': 3, 'alhurra': 1, 'repel': 1, 'foreclosure': 5, 'simkins': 3, 'barretto': 3, 'percussionist': 1, 'conga': 1, 'puente': 1, 'spanned': 2, 'gillespie': 1, 'dizzy': 1, 'tito': 2, 'celia': 1, 'cruz': 5, 'rhythm': 3, 'salsa': 1, 'maduro': 8, 'incomprehensible': 3, 'beached': 2, 'spit': 3, 'forty': 4, 'refloating': 1, 'hopeless': 1, 'refloat': 1, 'settings': 1, 'claus': 4, 'mythical': 1, 'anticipate': 3, 'martti': 2, 'ahtisaari': 3, 'trillions': 2, 'winding': 1, 'bonus': 4, 'borg': 1, 'risky': 1, 'bets': 2, 'farris': 1, 'redrawing': 1, 'separates': 1, 'bathrooms': 2, 'ging': 3, 'pierced': 1, 'cultivated': 3, 'fend': 5, 'attributes': 1, 'sahab': 1, 'rivalries': 3, 'alerting': 2, 'chase': 8, 'europrean': 1, 'greens': 2, 'kuhn': 1, 'renate': 1, 'kuenast': 1, 'batasuna': 1, 'du': 3, 'qinglin': 1, 'lambert': 3, 'kano': 6, 'nigerians': 2, 'mersin': 1, 'counterkidnapping': 1, 'leonel': 3, 'momcilo': 2, 'perisic': 4, 'cinema': 2, 'thatcher': 1, 'dj': 1, 'productions': 1, 'pathe': 1, 'helen': 3, 'frears': 1, 'mirren': 1, 'weisfield': 3, 'outbid': 1, 'ratners': 5, 'qalqilya': 3, 'ratner': 1, 'gerald': 3, 'sweetened': 1, 'acceptances': 1, 'aug': 2, 'rupee': 1, 'overvalued': 1, 'amortizing': 1, 'downgrading': 2, 'eff': 1, 'profitability': 5, 'fluctuates': 3, 'erratic': 4, 'erm2': 1, 'preceding': 4, 'tipped': 2, 'bounce': 1, 'peers': 2, 'payroll': 3, 'revamp': 2, 'mixture': 3, 'supplanted': 1, 'shortfalls': 6, 'overstaffing': 1, 'mortgaged': 1, 'renewing': 5, 'presides': 4, 'stimulating': 2, 'uneasy': 2, 'specializes': 1, 'enhance': 11, 'conformity': 1, 'prefecture': 5, '245': 1, 'qamdo': 1, 'prized': 1, 'bern': 1, 'sizable': 9, 'secrecy': 4, 'consequently': 2, 'incorporate': 2, 'awaits': 2, 'behest': 1, 'aftershock': 6, 'atoll': 4, 'mururoa': 1, 'polynesia': 2, 'vendors': 3, 'microenterprises': 1, 'distinguished': 2, 'deficient': 2, 'shipwrecked': 2, 'buffetings': 1, 'awoke': 2, 'reproaches': 1, 'plow': 2, 'calmness': 1, 'lash': 4, 'fury': 1, 'assuming': 6, 'sorry': 5, 'cd': 2, 'demolished': 5, 'issawiya': 1, 'daoud': 5, 'tainting': 1, 'criminalizing': 1, 'erich': 1, 'scrambled': 2, 'intercept': 4, 'circumventing': 1, 'interrogating': 3, 'rumbek': 1, 'spector': 11, 'phil': 8, 'superior': 2, 'fidler': 1, 'reconvene': 2, 'cutler': 1, 'technique': 4, 'lana': 3, 'daughters': 5, 'fadillah': 2, 'siti': 4, 'probability': 2, 'supari': 4, 'akmatbayev': 2, 'tynychbek': 1, 'transferable': 1, 'blackout': 9, 'subways': 4, 'outage': 5, 'substation': 2, 'chubais': 2, 'anatoly': 5, 'tula': 1, 'repelled': 7, 'escravos': 1, 'entitlements': 1, 'chookiat': 1, 'ophaswongse': 1, 'wesley': 2, 'moodie': 6, 'legg': 2, 'mason': 2, '86th': 2, 'querrey': 2, 'aces': 3, 'grosjean': 1, 'danai': 1, 'udomchoke': 1, '43rd': 2, 'oudsema': 1, 'nightfall': 2, 'scan': 1, 'proclaim': 1, 'accordingly': 1, 'purification': 1, 'thirteenth': 1, 'jailings': 1, 'batted': 1, 'bat': 2, 'shaharyar': 1, 'preside': 3, 'org': 2, 'perkins': 3, 'moveon': 1, 'funneling': 4, 'muqdadiyah': 2, 'haq': 5, 'inzamam': 1, 'stoning': 4, 'hajj': 3, 'mina': 1, 'devil': 4, 'jamarat': 1, 'grueling': 1, 'bodied': 1, 'communion': 1, 'converge': 2, 'wasit': 1, 'kazakh': 2, 'assassins': 2, '\x92s': 8, 'obote': 3, 'ugandans': 4, 'milton': 3, 'mwesige': 1, 'kampala': 5, 'shaka': 1, 'ssali': 1, 'murat': 3, 'sutalinov': 1, 'objectionable': 1, 'ancestral': 2, 'cancelation': 1, 'herman': 1, 'rompuy': 1, 'refrain': 7, 'insolent': 2, 'capitalized': 4, 'edinburgh': 1, 'undetermined': 5, 'descended': 3, 'dervis': 2, 'talat': 11, 'eroglu': 2, 'reunify': 3, 'favors': 8, 'cypriots': 12, 'hinge': 2, 'chhattisgarh': 1, 'raipur': 1, 'jharkhand': 1, 'schumer': 3, 'fratto': 1, 'indices': 1, 'donating': 7, 'jilin': 3, 'dehui': 1, 'giri': 1, 'tulsi': 1, 'kadima': 16, 'accuracy': 4, 'inaccuracies': 4, 'tillman': 2, 'pat': 5, 'mostafavi': 1, 'culprit': 2, 'genes': 1, 'protein': 5, 'jude': 1, 'proteins': 1, 'sampled': 2, 'chihuahua': 6, 'josefa': 1, 'demolitions': 2, 'chepas': 2, 'baeza': 1, 'aston': 1, 'mulally': 1, 'mallahi': 2, 'khamis': 1, 'accomplice': 7, 'tawhid': 2, 'wal': 15, '117': 4, 'dahab': 1, 'compiled': 3, '192': 3, '255': 2, 'sachin': 1, 'kartik': 1, 'dravid': 6, 'dinesh': 2, 'tendulkar': 2, 'rahul': 3, 'tallied': 1, 'anchored': 3, '136': 3, 'brar': 1, 'shamsbari': 1, 'doda': 2, 'mart': 14, 'wreaked': 1, 'blockage': 1, 'sidakan': 1, '132': 5, 'atlantis': 6, 'walheim': 1, 'rex': 1, 'orbital': 1, 'hans': 3, 'schlegel': 2, 'outing': 1, 'crewmates': 1, 'krajina': 2, 'mladen': 3, 'markac': 3, 'cermak': 3, 'eyharts': 1, 'leopold': 1, 'floated': 3, 'deadlines': 4, 'sony': 10, 'ericsson': 3, 'stabbed': 4, 'plundered': 1, 'handsets': 1, 'umpires': 1, 'smear': 2, 'din': 4, 'leaflets': 3, 'circulating': 6, 'restarted': 7, 'charkhi': 4, 'hashimzai': 1, 'sketchy': 4, 'obeyed': 1, 'raging': 2, 'mourns': 2, 'selecting': 1, 'nsc': 1, 'adversaries': 3, 'explicit': 1, 'theatergoers': 1, 'blades': 3, 'suspense': 1, 'disturbiaunseated': 1, 'labeouf': 1, 'starring': 3, 'shia': 2, 'morse': 1, 'peeping': 1, 'takers': 5, 'disturbia': 2, 'distributor': 1, 'dreamworks': 1, 'lebeouf': 1, 'surf': 2, 'transformers': 1, 'penguin': 2, 'hualan': 1, 'mutating': 3, 'plassnik': 2, 'ursula': 4, 'pluralistic': 2, 'heartland': 2, 'nad': 3, 'satan': 1, 'interwoven': 1, 'salif': 1, 'sud': 2, 'sadio': 3, 'ousamane': 1, 'ngom': 1, 'taraba': 1, 'grappling': 3, 'orellana': 2, 'suspends': 1, 'encampment': 1, 'disturbed': 5, 'equity': 3, 'afflicting': 1, 'imperative': 1, 'prohibit': 4, 'defamation': 3, 'oblivious': 1, 'hospitality': 4, 'destitute': 1, 'huts': 1, 'inspire': 5, 'firestorm': 2, 'condoms': 2, 'homily': 2, 'clouds': 3, 'wolfowitz': 10, 'supplemental': 1, 'recounted': 1, 'domino': 3, 'fats': 4, 'nickname': 3, 'fat': 6, 'blueberry': 1, 'hits': 3, 'uncover': 3, 'portrait': 3, 'handsome': 3, 'erasmus': 1, 'avenging': 1, 'dangerously': 2, '246': 1, 'ivchenko': 1, 'ukrainy': 1, 'oleksiy': 1, 'doboj': 2, 'kujundzic': 3, 'apprehended': 3, 'predrag': 1, 'verifying': 4, 'widen': 5, "shi'te": 1, 'dulles': 1, 'ahram': 3, 'omission': 1, 'rostock': 1, 'heiligendamm': 1, 'rostok': 1, 'pig': 4, 'streptococcus': 2, 'suis': 2, 'bacteria': 5, '174': 2, 'pork': 6, 'provocations': 4, 'anwar': 10, 'gargash': 1, 'sift': 1, 'passages': 1, 'displaying': 1, 'bara': 1, 'kazimierz': 3, 'marcinkiewicz': 3, 'caps': 5, 'nuys': 1, 'calif': 1, 'realestate': 1, 'fluctuation': 1, '361': 3, 'millennia': 2, 'rok': 3, 'dprk': 5, '1950': 5, 'demilitarized': 3, '38th': 1, 'bak': 5, 'myung': 5, 'punctuated': 1, 'cheonan': 2, 'bartolomeo': 1, '1648': 3, '1493': 3, 'gustavia': 1, '1784': 1, 'repurchased': 1, 'coat': 2, 'appellations': 1, 'collectivity': 1, 'populace': 1, 'hereditary': 1, 'premiers': 1, 'ensuing': 4, 'assumption': 3, 'promulgation': 1, 'overruled': 1, 'plurality': 1, 'leninist': 2, 'khanal': 1, 'jhala': 1, 'gridlock': 1, 'guyana': 5, 'indentured': 1, 'abolition': 5, 'importation': 3, 'plantations': 3, 'persisted': 3, 'ethnocultural': 1, 'cheddi': 1, 'jagan': 2, 'bharrat': 1, 'jagdeo': 1, '1923': 1, 'rhodesia': 1, '1920s': 4, 'unequivocally': 2, 'anticorruption': 3, 'usd': 2, 'chiluba': 2, 'abrupt': 3, 'spratly': 1, 'reefs': 3, 'reef': 1, 'overlaps': 1, 'placard': 2, 'shopkeeper': 1, 'salesman': 2, 'draped': 4, 'pozarevac': 2, 'mira': 1, 'vucelic': 1, 'markovic': 1, 'milorad': 2, 'remembrances': 1, 'surgical': 6, 'mash': 2, 'trauma': 1, 'beja': 3, 'vandalizing': 2, 'shebaa': 2, 'blockbuster': 1, 'thermopylae': 1, 'grossed': 2, '480': 2, 'copied': 1, 'disciplined': 4, 'amnesties': 1, 'absolve': 1, 'guerillas': 7, 'zhao': 6, 'ziyang': 1, 'watchful': 1, 'magnolia': 1, 'downplayed': 5, 'auspices': 5, 'themes': 2, 'kosumi': 6, 'bajram': 4, 'sejdiu': 1, 'agim': 2, 'ceku': 4, 'fatmir': 1, 'waseem': 1, 'goth': 1, 'sohrab': 1, 'cartographers': 1, 'currencies': 11, 'lutfi': 2, 'haziri': 1, 'commands': 3, 'ethic': 1, 'foster': 4, 'angering': 2, 'puntland': 1, 'bargal': 2, 'dams': 2, 'elyor': 1, 'ganiyev': 1, '173': 3, 'elsa': 3, 'alvarezes': 1, 'encryption': 2, 'shortwave': 3, 'janata': 4, '261': 1, '543': 2, 'bharatiya': 2, '159': 3, 'dissent': 6, 'thanking': 2, 'kwanzaa': 1, 'wishes': 7, 'wintery': 1, 'valleys': 3, 'jawad': 3, 'bolani': 2, 'waili': 2, 'jassim': 1, 'qader': 1, 'sherwan': 1, 'gaulle': 1, 'azur': 2, 'aigle': 2, 'qarabaugh': 1, 'stifling': 2, 'klerk': 1, 'robben': 2, 'denktash': 3, 'jafaari': 1, 'commend': 1, 'torshin': 1, 'shepel': 1, 'klebnikov': 3, 'writings': 2, 'ereli': 11, 'hanover': 3, 'centrifuge': 2, 'disapproves': 1, 'evangelist': 2, 'wholly': 2, 'childhood': 7, 'cdc': 4, 'declassified': 3, 'gimble': 1, 'assertions': 2, 'telephoned': 6, 'godfather': 2, 'papa': 1, 'autobiography': 3, 'sensible': 2, 'boel': 1, 'mariann': 1, 'breakers': 1, 'zhenchuan': 1, 'hurling': 1, "n'guesso": 1, 'statutes': 2, 'norms': 3, 'permissible': 1, 'mistreatment': 10, 'definition': 3, 'inflicted': 6, 'gvero': 1, 'corridor': 4, 'trans': 5, 'interstate': 2, 'fingerprint': 2, 'mocny': 1, 'fingerprinting': 2, 'apply': 18, 'trunk': 1, 'accosted': 1, 'idrac': 1, 'anne': 2, 'overuse': 3, 'sustains': 1, 'contamination': 7, 'landfills': 1, 'infants': 4, 'isaias': 3, 'afeworki': 1, 'backward': 4, 'sufficiently': 2, 'bakery': 5, 'rethink': 1, 'organic': 1, 'susy': 2, 'tekunan': 2, 'recycled': 2, 'cookies': 1, 'quest': 2, 'baking': 2, 'unfriendly': 2, 'deserters': 2, 'nasariyah': 1, 'lattes': 1, 'untitled': 1, 'retailer': 8, 'cobos': 1, 'julio': 4, 'thriving': 2, 'lublin': 3, 'synagogue': 3, 'schudrich': 1, 'scrolls': 1, 'rabinnical': 1, 'videos': 8, 'papua': 6, 'merbau': 1, 'undisturbed': 1, 'hifikepunye': 2, 'pohamba': 6, 'windhoek': 2, 'nujoma': 5, 'swapo': 2, 'admhaiyah': 1, 'designation': 4, 'momen': 1, 'ye': 1, 'dawei': 4, 'legislatures': 3, 'heliodoro': 1, 'construct': 5, 'reclassify': 1, 'canals': 2, 'dot': 1, 'bloating': 1, 'riverbanks': 1, 'irrawaddy': 2, 'exorbitant': 2, 'profiteers': 1, 'scarce': 5, 'essentials': 1, 'lecturers': 1, 'knu': 1, 'ghazaliyah': 1, 'policing': 1, 'hovers': 1, 'kph': 2, 'chequers': 1, 'outer': 5, 'peoria': 2, 'mich': 1, 'kalamazoo': 1, 'managers': 12, 'severance': 3, 'maumoon': 1, 'gayoom': 2, 'embark': 1, 'legalized': 4, 'nasheed': 2, 'elevation': 2, 'defeating': 3, 'tsz': 2, 'remotely': 2, 'badme': 1, 'tracts': 2, 'demarcated': 2, 'coordinates': 2, 'eebc': 1, 'immersed': 1, 'gdr': 2, 'advent': 1, 'frg': 2, 'expended': 1, 'heel': 1, 'geographical': 3, 'corporations': 4, 'intervening': 2, 'mitigating': 1, 'radicova': 1, 'boar': 3, 'agonies': 1, 'fiercer': 1, 'vultures': 2, 'renewal': 5, 'crows': 2, 'unto': 1, 'gasping': 1, 'subjects': 4, 'helpless': 3, 'grudges': 1, 'gored': 2, 'tusks': 1, 'bull': 5, 'growled': 1, 'majesty': 1, 'cowards': 1, 'marry': 1, 'proliferating': 1, 'fan': 3, 'vanallen': 2, 'belts': 3, 'ichiro': 1, 'ozawa': 3, 'audiotapes': 1, 'doug': 1, 'wead': 2, 'discusses': 4, 'pathologically': 1, 'liar': 1, '179': 1, '343': 1, 'csongrad': 1, 'brett': 3, 'tea': 8, '196': 1, 'triangular': 2, '3rd': 2, 'mall': 8, 'abnormally': 1, 'mortality': 4, 'ratnayke': 1, 'prabhakaran': 3, 'velupillai': 2, 'whittington': 9, 'bruised': 1, 'wyoming': 3, 'chahine': 3, 'renowned': 4, 'cosmopolitan': 1, 'imperialism': 3, 'multiculturalism': 1, 'lakhdaria': 2, 'etihad': 1, 'fomenting': 2, 'bastille': 1, 'inalienable': 1, 'vigilantes': 1, 'instructor': 1, 'basij': 1, 'azov': 1, 'kerch': 2, 'volganeft': 1, '139': 4, 'sulfur': 1, 'cary': 1, 'porpoises': 1, 'tuneup': 1, 'arena': 4, 'leaning': 4, 'nashville': 2, 'fallouj': 1, 'conglomerate': 1, 'petrodar': 1, 'rawalpindi': 7, 'quarterfinal': 6, 'khaznawi': 4, 'yekiti': 2, 'maashouq': 2, 'kameshli': 2, 'basir': 1, 'arbab': 1, 'encountering': 2, 'converts': 2, 'rajah': 1, 'solaiman': 3, 'zamboanga': 1, 'ferry': 12, 'unocal': 6, 'cnooc': 3, 'forceful': 3, 'bidder': 2, 'inlets': 1, 'fumes': 1, 'petrechemical': 1, 'pollutants': 3, 'bearing': 4, 'interpreting': 1, 'taitung': 1, 'sepat': 3, 'gusts': 4, 'plowing': 1, 'kurd': 7, 'guardedly': 1, 'defining': 2, 'grips': 1, 'regrettably': 1, 'channeled': 1, 'culls': 1, '555': 1, 'mentioning': 5, 'wisely': 1, 'krugman': 1, 'weathered': 3, 'trichet': 1, 'boao': 1, 'rigging': 9, 'rubaie': 1, 'mowaffaq': 1, 'anfal': 5, 'formulas': 1, 'fade': 2, 'urgently': 7, 'phillips': 1, 'comfort': 2, 'ciudad': 6, 'battleground': 1, 'juarez': 9, 'goers': 2, 'sinaloa': 1, 'lags': 2, 'emitted': 1, 'disqualified': 5, 'nosedive': 1, 'shortened': 5, '646': 2, 'creators': 1, '890': 1, 'tariceanu': 2, 'calin': 2, 'popov': 1, 'siirt': 2, 'diyarbakir': 4, 'perspective': 4, 'voyage': 3, 'mariam': 3, 'suffocation': 5, 'poncet': 1, 'buffer': 6, 'barcode': 1, 'barcodes': 5, 'inventory': 3, 'clerks': 1, 'scanners': 2, 'exactly': 9, 'chewing': 1, 'barcoded': 1, 'invalidated': 1, 'massed': 2, 'redoing': 1, 'glasgow': 7, 'trainees': 2, 'nails': 1, 'cylinders': 2, 'mack': 3, 'sabeel': 3, 'kafeel': 3, 'haneef': 3, 'macapagal': 4, 'jam': 1, 'refiling': 1, 'soliciting': 3, 'everybody': 2, 'sacrilegious': 1, 'valdivia': 1, 'clot': 3, 'ultrasound': 1, 'rada': 1, 'verkhovna': 1, 'unicameral': 1, 'clots': 2, 'immobility': 1, 'destined': 3, 'suits': 4, 'informer': 3, 'nycz': 1, 'dreaded': 1, 'apparatus': 4, 'burying': 1, 'uthai': 1, 'petrovka': 1, 'smashing': 3, 'bakyt': 1, 'seitov': 1, 'secretariat': 2, 'ramechhap': 1, 'schiavone': 1, 'trailed': 1, 'francesca': 2, 'kasparov': 1, 'kasyanov': 1, 'chess': 2, 'liberals': 2, 'magnet': 1, 'disparate': 1, 'transcends': 1, 'superseded': 1, 'trump': 5, 'cohen': 3, 'blond': 1, 'haired': 1, 'emomali': 2, 'rakhmon': 2, 'telephones': 1, 'hazards': 3, 'tajik': 4, 'jokhang': 1, 'andreev': 3, 'igor': 6, 'gongga': 1, 'rabdhure': 1, 'inflate': 1, 'appease': 3, 'amin': 6, 'finalists': 1, 'finisher': 2, 'mexicans': 2, 'underscore': 4, 'stockholders': 1, 'coleco': 3, 'reorganization': 2, 'chapter': 6, 'owed': 5, 'unsecured': 2, 'ranger': 1, 'reorganized': 2, 'glitches': 1, 'avon': 1, 'patch': 1, 'craze': 1, 'anjouan': 3, 'moheli': 1, 'fomboni': 1, 'azali': 3, 'rotates': 1, 'sambi': 1, 'bacar': 1, 'anjouanais': 1, 'effected': 2, 'comoran': 1, 'dioceses': 2, 'curia': 1, 'mementos': 1, 'moreover': 3, 'countercoups': 1, '1825': 2, 'consisted': 2, 'widest': 1, 'empower': 1, 'bolivians': 5, 'lowlands': 1, 'amerindian': 2, 'entrant': 1, 'wavered': 1, 'tallinn': 1, 'bubble': 2, 'bursting': 3, '1861': 3, 'sicily': 2, 'fascist': 3, 'benito': 3, 'mussolini': 3, 'azerbaijani': 3, 'eec': 2, 'forefront': 1, 'outperformed': 1, 'encamped': 3, 'notch': 1, 'continuity': 1, 'boediono': 1, 'impediments': 3, 'redd': 1, 'peatlands': 1, 'conserving': 2, 'trailblazing': 1, 'ostrich': 4, 'choked': 2, 'suppose': 1, 'err': 1, 'testament': 1, 'resigning': 2, 'interred': 1, 'instituting': 2, 'kheir': 2, 'terrorizing': 1, 'negate': 2, 'morally': 1, 'reprehensible': 4, 'silvan': 5, 'chodo': 3, 'firings': 2, 'commute': 1, 'basing': 1, 'grigory': 1, 'karasin': 2, 'lighthouses': 1, 'bachelor': 1, 'rewarded': 1, 'toussaint': 2, '93rd': 1, 'maronite': 3, 'sfeir': 2, 'unfiltered': 2, 'redirected': 1, 'click': 2, 'hack': 1, 'redirecting': 1, 'drummond': 1, 'blog': 3, 'firecracker': 1, 'diwali': 4, 'ignited': 2, 'pallipat': 1, 'lamps': 1, 'snacks': 1, 'sweets': 1, 'ilo': 3, 'bilis': 2, '518': 1, 'hun': 4, '346': 1, 'unverified': 1, '24th': 3, 'rizkar': 1, 'pawn': 1, 'steadfast': 1, 'covenant': 1, 'deposited': 1, 'byelection': 1, 'disbarred': 1, 'amsterdam': 2, 'rollout': 1, 'ab': 1, 'hennes': 1, 'mauritz': 1, 'purses': 1, 'bras': 1, 'risque': 1, 'conical': 1, 'fellowships': 1, 'orphanages': 1, 'discounts': 4, 'reliability': 1, 'recalls': 1, 'honda': 1, 'hyundai': 2, 'kifaya': 2, 'tomorrow': 3, 'forgery': 2, 'sniffing': 1, 'sherry': 1, 'fining': 1, 'misquoting': 2, 'informing': 4, 'padilla': 5, 'classification': 1, 'beijings': 1, 'goddess': 1, 'capitol': 11, 'memorials': 3, 'postsays': 2, 'hacksaws': 1, 'transports': 1, 'muse': 1, 'lone': 5, 'merging': 2, 'nizar': 2, 'trabelsi': 2, 'bakara': 3, '620': 1, 'kochi': 1, '777': 1, 'baziv': 1, 'vasil': 2, 'yevhen': 1, 'kushnaryov': 1, 'slauta': 1, 'kharkiv': 1, 'yuschenko': 3, 'instructors': 2, 'rousing': 1, 'nasiriyah': 3, 'ansa': 3, 'ryzhkov': 1, 'ivashov': 2, 'primakov': 1, 'yevgeny': 1, 'phan': 1, 'khai': 1, 'duc': 2, 'tran': 1, 'luong': 2, 'burundians': 4, 'sized': 3, 'giustra': 1, 'bellerive': 3, 'spreads': 3, 'osterholm': 1, 'nagasaki': 4, 'hiroshima': 9, 'atom': 4, 'katsuya': 1, 'okada': 1, 'kan': 1, 'naoto': 1, 'processes': 6, 'chipmaker': 2, 'shaft': 5, 'golborne': 2, 'laurence': 1, 'analyze': 3, 'boring': 1, 'shafts': 2, 'mardi': 5, 'literally': 1, 'gras': 5, 'precedes': 2, 'purple': 2, 'feather': 1, 'beads': 2, 'boas': 1, 'connick': 1, 'batlle': 2, 'chao': 1, 'congratuatlions': 1, 'rud': 1, 'posht': 1, 'artemisinin': 1, 'bala': 1, 'boluk': 1, 'midestern': 1, 'vx': 3, 'newport': 1, 'drained': 2, 'sodium': 2, 'hydroxide': 1, 'escorts': 2, 'reverses': 1, 'definitely': 1, 'falciparum': 1, 'posters': 8, 'plastered': 1, 'simmering': 2, "ba'athists": 1, 'blueprints': 2, 'sui': 1, 'nawab': 1, 'maliky': 1, 'risked': 2, 'ngwira': 5, 'kandani': 2, 'miyazaki': 2, 'emergence': 3, 'nahim': 1, 'jaghato': 1, 'pajhwok': 2, 'blechschmidt': 2, 'malawian': 1, 'consolidating': 2, 'yulija': 2, 'plenary': 3, 'mwaluka': 1, 'willy': 1, 'zones': 6, 'artisans': 1, 'kirk': 3, 'tops': 3, 'hotspots': 1, 'consequence': 4, 'adijon': 1, 'kosachev': 2, 'luncheon': 3, 'awake': 2, 'warrior': 1, 'malignant': 2, 'tumor': 4, '99': 10, 'tendering': 1, 'jon': 2, 'guber': 3, 'peters': 3, 'litigation': 2, 'eparses': 2, 'taaf': 1, 'integral': 2, 'iles': 3, 'archipelagos': 1, 'crozet': 1, 'kerguelen': 2, 'ile': 1, 'fauna': 1, 'adelie': 1, 'slice': 1, '1840': 3, 'eligibility': 3, 'satisfying': 2, 'gondwe': 1, 'exhibited': 1, 'goodall': 1, "mutharika's": 1, 'unreliable': 3, 'oresund': 1, 'kiel': 3, 'seaway': 1, 'bosporus': 1, 'hydrographic': 3, 'latitude': 3, 'delimit': 4, 'toroitich': 1, 'kenyatta': 2, 'jomo': 1, 'kanu': 4, 'dislodge': 2, 'fractured': 2, 'rainbow': 1, 'multiethnic': 1, 'uhuru': 1, 'narc': 2, 'conciliatory': 1, 'odm': 1, 'powersharing': 1, 'eliminates': 1, 'coral': 7, 'uninhabited': 6, 'islets': 1, 'willis': 1, 'beacons': 1, 'lighthouse': 2, 'automated': 3, 'dolphin': 5, 'ought': 3, 'gladly': 1, 'traitor': 1, 'nay': 1, 'drunken': 3, 'wallow': 1, 'converged': 1, 'disparities': 4, 'owning': 1, 'jeungsan': 1, 'quoting': 3, 'dhi': 1, 'qar': 2, 'nautical': 1, '7115': 1, 'voanews': 1, 'listen': 1, '9885': 1, '11705': 1, '11725': 1, 'solvent': 2, 'depressing': 1, 'argentinean': 1, 'sanclemente': 1, 'valenica': 1, 'angie': 1, 'vakhitov': 2, 'airat': 1, 'korans': 2, 'drifting': 2, 'hae': 1, 'panmunjom': 1, 'ashraf': 6, 'sara': 2, 'meadowbrook': 1, 'sanabel': 1, 'ozlam': 1, 'obsessed': 1, 'chorus': 3, 'alexeyenko': 1, 'mathew': 2, 'stewart': 5, 'surveying': 2, 'ordnance': 1, 'swiergosz': 1, 'chelsea': 1, 'goats': 5, 'cows': 2, 'filtering': 3, 'worded': 3, 'vaguely': 1, 'lawbreakers': 2, 'regulating': 3, 'hotmail': 2, 'fortinet': 1, 'irawaddy': 1, 'bio': 2, 'valery': 1, 'sitnikov': 1, '336': 2, 'enacts': 1, 'fitzpatrick': 1, 'habbaniya': 1, 'yields': 3, 'mandates': 1, 'donation': 5, 'airlifts': 1, 'benghazi': 1, 'heightening': 1, 'bok': 1, 'choe': 3, 'thae': 1, 'hon': 2, 'su': 4, 'okah': 2, 'deceased': 4, 'mandarin': 1, 'tuo': 1, 'bisphenol': 2, 'bpa': 1, 'liver': 2, 'rachid': 2, 'abdelkader': 1, 'ilhami': 1, 'gafir': 1, 'oxygen': 2, 'preacher': 5, 'loyalties': 1, 'nimnim': 1, 'jamal': 8, 'kyodo': 4, 'grieves': 1, 'talangama': 1, 'michaella': 2, 'peng': 3, '36th': 2, 'shuai': 1, 'krajicek': 7, 'wessels': 2, 'kiefer': 2, 'stosur': 1, 'arthurs': 1, 'groenefeld': 4, 'lena': 4, 'dent': 2, 'preservation': 2, '1850s': 1, 'clemency': 4, 'disrespectful': 1, 'kai': 4, 'dissipated': 1, 'inundated': 4, 'stanley': 3, 'tookie': 1, 'infamous': 5, 'zidane': 10, 'zinedine': 3, 'inspirational': 1, 'playmaker': 1, 'juventus': 4, 'grouped': 2, 'unnerved': 2, 'westinghouse': 2, 'competed': 6, 'wrecking': 1, 'clunkers': 4, 'fulayfill': 1, 'suez': 4, 'geyer': 1, 'cavalry': 1, '157': 3, 'préval': 2, 'rené': 1, 'jeb': 1, 'sanderson': 1, 'comprise': 4, 'rony': 1, 'petraeus': 9, 'saves': 3, 'trooper': 1, 'embezzling': 8, 'sese': 1, 'seko': 1, 'mobutu': 2, 'shajoy': 1, 'helsoe': 1, 'jesper': 1, 'feyzabad': 1, 'yazdi': 1, 'tirin': 3, 'mahendranagar': 1, 'legitimizing': 2, 'sham': 4, 'bager': 1, 'uspi': 1, 'asadullah': 2, 'seismologists': 5, 'guardians': 1, 'libbi': 3, 'farraj': 3, 'shiekh': 2, 'insein': 3, 'lntelligence': 1, 'alcantara': 2, 'technicians': 2, 'altcantara': 1, 'vsv': 1, 'pad': 3, 'equator': 3, 'voto': 1, 'convey': 3, 'bernales': 1, 'likening': 1, 'gear': 1, 'pervasive': 2, 'practitioners': 1, 'asserted': 3, 'tarnish': 1, 'stalls': 3, 'depict': 2, 'disastrous': 7, 'wishers': 1, 'detractors': 1, 'iskander': 2, 'paralympic': 3, 'paralympics': 1, 'chongqing': 3, 'tianjin': 5, 'urumqi': 2, 'statisticians': 1, 'gymnasiums': 1, 'oversized': 1, 'chrome': 1, 'wheels': 3, 'sleek': 2, 'velocity': 1, '575': 1, 'streaked': 1, 'levitates': 1, '581': 1, 'magnetic': 2, '515': 1, 'alstom': 1, 'decker': 3, 'horsepower': 1, 'prototype': 3, '786': 1, 'rental': 2, 'boosters': 3, 'alleviation': 1, 'borrower': 2, 'citibank': 1, '295': 2, 'revelations': 1, 'britons': 3, 'laying': 9, 'mayo': 1, '70s': 3, 'environments': 1, '\x92': 1, '\x91': 1, 'kickbacks': 6, 'kollock': 2, 'paige': 2, 'chester': 2, 'gunsmoke': 2, 'qualifications': 2, 'decathlon': 1, 'emmy': 2, 'limping': 1, 'mccloud': 1, 'starred': 3, 'taher': 2, 'ani': 1, 'busta': 2, 'rhymes': 3, 'lectures': 1, 'trevor': 1, 'uncooperative': 2, 'jianchao': 1, 'amateur': 2, 'recreational': 2, 'lounderma': 1, 'unnerve': 1, '550': 5, 'hanun': 1, 'jumping': 4, 'spoiled': 1, 'inaccurate': 2, 'explanations': 1, 'casas': 1, 'cristobal': 1, 'uribana': 1, 'panzhihua': 1, 'tit': 1, 'tat': 1, 'recall': 8, 'kanyarukiga': 3, 'gaspard': 1, 'bulldozing': 1, 'nyange': 1, 'xerox': 3, 'crum': 3, 'forster': 3, 'schooled': 1, 'comparison': 3, '492': 1, 'cutthroat': 1, 'staunchly': 2, 'freight': 2, 'transshipment': 3, 'hooper': 3, 'overfishing': 2, 'mutineers': 2, 'pitcairn': 4, '1767': 1, '1790': 1, 'tahitian': 2, 'vestige': 2, 'outmigration': 1, '233': 1, 'akrotiri': 4, 'southernmost': 3, 'melanesians': 1, 'melanesian': 1, 'ensured': 3, 'cemented': 1, 'fijian': 1, 'qarase': 2, 'laisenia': 1, 'voreqe': 1, 'bainimarama': 2, 'commodore': 1, 'favoring': 6, 'embroiled': 4, 'cpa': 2, 'influxes': 1, 'obstructed': 3, 'undertook': 4, 'sprang': 1, 'huntsmen': 3, 'hounds': 2, 'snatch': 1, 'brawny': 1, 'refrigerator': 1, 'doorbell': 1, 'revolutionaries': 3, 'kung': 2, 'pin': 2, '1911': 2, 'sibaie': 1, 'hani': 2, 'moustafa': 1, 'yafei': 1, 'condone': 1, 'unveil': 3, 'amil': 1, 'adamiya': 1, 'stick': 3, 'calabar': 2, 'villa': 1, 'andré': 1, 'korea\x92s': 1, 'nesnera': 1, 'degradation': 4, 'slot': 6, 'kirilenko': 2, 'pectoral': 1, 'booed': 1, 'domachowska': 1, 'davenport': 4, 'venus': 10, 'seemingly': 2, 'happen': 12, 'beachside': 2, 'berms': 1, 'happens': 3, 'surfboard': 1, 'exciting': 2, 'surfers': 1, 'emphasize': 1, 'swells': 1, 'removes': 3, 'posses': 1, 'convenient': 1, 'mutually': 4, 'gholamhossein': 1, 'elham': 2, 'tribunals': 3, 'protects': 5, 'fundamentally': 2, '540': 3, 'referees': 5, 'practical': 4, 'theoretical': 1, 'sepp': 2, 'blatter': 2, '6000': 1, 'trim': 2, 'daimler': 1, 'bouterse': 4, 'desi': 1, 'dunem': 3, 'discharges': 3, 'cheeks': 3, 'gere': 7, 'shetty': 8, 'shilpa': 4, 'kissed': 4, 'kissing': 2, 'varanasi': 1, 'meerut': 1, 'undocked': 1, 'endeavourhas': 1, 'looped': 2, 'exterior': 1, 'hatches': 1, 'goodbyes': 1, 'recycling': 1, 'yen': 11, 'expectant': 1, 'cords': 4, 'umbilical': 1, 'cord': 4, 'troedsson': 2, 'colder': 5, 'tends': 2, 'suna': 1, 'afewerki': 1, 'ontario': 1, 'worn': 4, 'belief': 5, 'jabr': 3, 'bayan': 3, 'coastguard': 1, 'alloceans': 2, 'ariana': 4, 'spyros': 1, 'smigun': 2, 'kristina': 1, 'marit': 1, 'bjoergen': 1, 'hilde': 1, 'pedersen': 1, 'snowboard': 2, 'temples': 4, 'genders': 1, 'speedskating': 1, 'ebrahim': 1, 'sheibani': 2, 'saderat': 2, 'jabalya': 2, 'collins': 4, 'frail': 3, 'arthritis': 2, 'warhead': 4, 'akash': 2, 'orissa': 4, 'bhubaneshwar': 2, 'chandipur': 2, 'congestive': 2, 'drinan': 2, 'directive': 3, 'supersedes': 1, 'defer': 1, 'overlooks': 1, 'textbook': 1, 'calculation': 1, 'cynical': 1, 'characterization': 1, '880': 1, 'azzedine': 1, 'belkadi': 1, 'kerimli': 1, 'saadat': 1, 'pflp': 1, 'rehavam': 1, 'zeevi': 1, 'splattered': 1, 'pettigrew': 1, 'pierre': 9, 'handover': 10, 'unsure': 2, 'gibbs': 6, 'bowling': 1, 'otoniel': 1, 'guevara': 1, 'rolando': 1, 'aharonot': 3, 'samahdna': 1, 'runup': 1, 'chileans': 3, "o'higgins": 1, 'libertador': 1, 'valparaiso': 1, 'shaking': 2, 'auckland': 5, 'stratfor': 2, 'turkestan': 2, 'intelcenter': 1, 'adumin': 2, 'westernmost': 2, 'heeding': 1, 'rocking': 2, 'energized': 1, 'kiraitu': 1, 'murungi': 3, 'githongo': 2, 'leasing': 5, 'anglo': 7, 'asghar': 2, 'payday': 1, 'michoacan': 3, 'shihri': 3, 'divine': 1, 'everywhere': 2, 'abdulmutallab': 2, 'umar': 3, 'summed': 1, 'barbecues': 1, 'bulandshahr': 1, 'leil': 1, 'commuted': 3, 'nottingham': 3, 'atheists': 1, 'lambeth': 1, 'cloning': 2, 'hwang': 10, 'suk': 2, 'misappropriation': 1, 'veterinarian': 1, 'deceiving': 2, 'ningbo': 4, 'flour': 5, 'veils': 1, 'wives': 6, 'scuffles': 2, 'khori': 1, 'skier': 6, 'wengen': 2, 'lauberhorn': 1, 'alleys': 1, 'bib': 1, 'raich': 3, '706': 2, '589': 1, '106': 5, 'practically': 2, 'plates': 2, 'rittipakdee': 1, 'soonthorn': 3, 'ganei': 2, 'botched': 3, 'unvetted': 1, 'gorges': 1, 'yuanmu': 1, 'overpopulation': 1, 'oic': 2, 'socio': 3, 'mecca': 6, 'caved': 2, 'racing': 4, 'delegitimize': 1, 'drastic': 2, 'newer': 4, 'amrullah': 1, 'tyre': 2, 'militaristic': 1, 'motoyasu': 1, 'lanterns': 2, 'souls': 2, 'candlelit': 1, 'horrific': 2, 'lantern': 1, 'bowed': 3, 'janakpur': 2, 'itahari': 1, 'fonseka': 3, 'rajapaksa': 2, 'astonished': 1, 'hongyao': 1, 'arafura': 1, 'visual': 2, 'merauke': 1, 'upjohn': 9, 'headcount': 1, 'schroder': 1, 'wertheim': 1, 'gelles': 1, '875': 1, 'apiece': 2, 'suitors': 1, 'igad': 1, 'trimmed': 1, 'kubilius': 1, 'cutback': 1, 'eskom': 1, 'shedding': 2, 'necessitating': 1, 'reaped': 2, 'empowerment': 1, 'attaining': 2, 'fiscally': 2, 'guano': 1, '1857': 2, '1898': 6, 'navassa': 1, 'biodiversity': 2, 'expedition': 3, '1903': 2, '1914': 3, 'rested': 1, 'panamanians': 1, '1811': 1, 'chaco': 4, 'lowland': 1, '1932': 5, 'alfredo': 2, 'stroessner': 1, 'celtic': 2, 'omra': 1, 'pilgrimage': 4, 'boru': 1, '1014': 1, 'norsemen': 1, 'rebellions': 2, 'repressions': 1, '1921': 4, 'ulster': 2, 'andrews': 1, 'trotted': 1, 'hart': 9, 'scudded': 1, 'noise': 5, 'optimist': 1, 'pessimist': 1, '857': 1, '772': 1, 'wellington': 3, '107': 3, 'loder': 1, 'crusader': 1, 'sobriety': 1, 'sutherland': 4, 'misdemeanor': 1, 'maiberger': 2, 'misdmeanor': 1, 'keifer': 1, 'obscenity': 3, 'metropolis': 1, 'bei': 5, 'cadmium': 6, 'baishiyao': 1, 'dilute': 3, 'smelter': 2, 'administers': 3, 'politicizing': 2, 'friedan': 4, 'groundwork': 2, 'mystique': 2, 'feminine': 2, 'feminism': 1, 'psychology': 1, 'housewife': 1, 'groundbreaking': 2, 'unfulfilled': 1, 'husbands': 2, 'caucus': 5, 'abdouramane': 1, 'goushmane': 2, 'conviasa': 1, 'controllers': 3, 'sidor': 1, 'margarita': 1, 'ordaz': 3, 'heroes': 3, 'defections': 1, 'portraits': 1, 'privileges': 5, 'yvon': 1, 'privert': 1, 'jocelerme': 1, 'neptune': 1, 'protestors': 1, 'naryn': 1, 'onshore': 1, 'pour': 1, 'changbei': 1, 'petrochina': 1, 'unreleased': 1, 'pneumonic': 1, '2500': 1, 'infect': 4, 'tawilla': 1, 'cannon': 4, 'widens': 1, 'trimester': 1, 'abortions': 4, 'incest': 1, 'obstruction': 3, 'addington': 1, 'hannah': 1, 'canaries': 1, 'whipping': 1, 'utor': 1, 'huddled': 1, 'mindoro': 2, 'albay': 2, 'mayon': 2, 'recommend': 8, 'sludge': 1, 'walayo': 1, 'loaning': 2, 'engineered': 4, 'hepatitis': 1, 'paves': 2, 'cerda': 1, 'disappearances': 1, 'blockades': 2, 'belkhadem': 1, 'destabilizing': 4, 'ugalde': 1, 'gatlin': 5, 'eugene': 2, 'sprinter': 3, 'magistrates': 1, 'prescribed': 2, 'milky': 1, 'magellanic': 1, 'fragmentary': 1, 'cloud': 6, 'azima': 3, 'parnaz': 1, 'esfandiari': 1, 'haleh': 1, 'woodrow': 1, 'tajbakhsh': 1, 'kian': 1, 'shakeri': 3, 'malibu': 1, 'aided': 5, 'concentrating': 1, 'hyde': 2, 'ohlmert': 1, 'qasr': 1, 'irrigation': 2, 'uncomplicated': 1, 'samawa': 3, 'patu': 1, 'cull': 1, 'extolling': 1, 'diyar': 1, 'altitudes': 2, 'nourished': 1, 'rainstorms': 2, 'napa': 2, 'swelling': 2, 'sonoma': 1, 'indict': 2, 'receded': 1, 'rained': 1, '1955': 5, 'backlash': 5, 'shieh': 1, 'jhy': 1, 'wey': 1, 'tu': 2, 'cheng': 1, 'shu': 1, 'ezzedine': 1, 'elusive': 2, 'contemplate': 1, 'dairy': 4, 'prithvi': 2, 'aggravate': 1, 'haradinaj': 11, 'ramush': 4, 'guerilla': 3, 'idriz': 1, 'lahi': 1, 'brahimaj': 1, 'balaj': 1, 'subordinates': 3, 'persecuted': 2, 'pune': 1, '816': 1, '503': 1, 'scarcely': 3, 'misquoted': 1, 'careful': 4, 'dyes': 2, 'conceal': 2, 'kuril': 2, 'tremors': 3, 'magnitudes': 1, 'coastlines': 4, 'destructive': 3, 'disagreement': 9, '561': 2, 'exceeds': 4, 'regev': 3, 'taxpayers': 2, 'aia': 2, 'hakkari': 2, 'thiam': 1, 'tidjane': 1, 'hoon': 4, 'falkland': 6, 'landfill': 3, 'wilmington': 2, 'wheeler': 5, 'exiting': 1, 'backers': 7, 'recife': 1, 'gabgbo': 1, 'sculpture': 1, 'interactive': 3, 'gadgets': 1, 'matteo': 1, 'duran': 1, 'graduation': 2, 'walder': 1, 'benita': 2, 'mercosur': 13, 'breaststroke': 2, 'brendan': 1, 'kitajima': 1, 'kosuke': 1, 'omaha': 1, 'medley': 1, 'katie': 1, 'hoff': 1, 'freestyle': 1, 'regents': 1, 'bencomo': 3, 'verez': 3, 'innovations': 1, 'meningitis': 2, 'themba': 1, 'nyathi': 3, 'agca': 5, 'demirbag': 1, 'forgave': 1, 'midler': 2, 'caesar': 1, 'celine': 2, 'headliner': 2, 'dion': 2, 'bette': 1, 'bawdy': 1, 'inaugurating': 2, 'elton': 5, 'colosseum': 2, 'probable': 1, 'meglen': 1, 'insiders': 1, 'cher': 1, 'vaile': 2, 'vuvuzelas': 2, 'filter': 3, 'trumpets': 1, '545': 1, 'reduces': 1, 'commentary': 4, 'ambient': 1, 'camel': 4, 'racers': 1, 'jockeys': 3, 'experimenting': 1, 'robots': 2, 'lighter': 2, 'underage': 1, 'underfed': 1, 'jockey': 1, 'horne': 3, 'johnny': 1, 'unpremeditated': 1, 'garbage': 1, 'misery': 4, 'tolipkhodzhayev': 1, 'akhrorkhodzha': 1, 'chikunova': 2, 'offline': 1, 'danforth': 2, 'statesmen': 1, 'tearfully': 1, 'georges': 3, 'chesnot': 2, 'malbrunot': 2, 'doaba': 1, 'hangu': 1, 'klaus': 1, 'toepfer': 1, 'poisonous': 5, 'riverside': 1, 'wazirstan': 2, 'profiles': 1, 'inaccessible': 4, 'accessing': 2, '377': 1, 'rak': 2, 'mushtaq': 1, 'garrison': 4, 'bechuanaland': 1, 'uninterrupted': 2, 'preserves': 1, 'dominates': 3, '1895': 1, 'reverted': 1, 'democratized': 1, 'yams': 3, 'watermelons': 1, 'bind': 3, 'unload': 2, 'occupies': 3, 'earners': 2, 'quarry': 1, 'subsuming': 1, 'legislated': 1, 'reasoning': 1, 'overgrazing': 1, 'tramp': 3, 'linen': 1, 'travelling': 1, 'characteristic': 1, 'unconcern': 1, 'contemptuously': 1, 'superb': 1, 'genius': 2, 'smooth': 5, 'carved': 3, 'bark': 1, 'gump': 1, 'birch': 1, 'correctness': 2, 'overheard': 1, 'pie': 1, 'disbursed': 1, 'verification': 3, 'saiki': 2, 'akitaka': 1, 'hammering': 1, 'creatively': 1, 'bwakira': 2, 'wet': 4, 'tankbuster': 1, 'baaz': 1, 'kheyal': 1, 'sherzai': 2, 'gardez': 1, 'weed': 2, 'cantarell': 1, 'administrators': 1, 'ourselves': 4, 'narcotic': 2, 'ilayan': 1, 'bribed': 2, 'mendonca': 1, 'duda': 1, 'beds': 2, 'floors': 1, 'crumbled': 1, 'inferior': 1, 'correcting': 1, 'dengue': 1, 'fanning': 2, 'larvae': 1, 'breed': 1, '284': 2, 'woon': 1, 'chul': 1, '626': 1, "n'zerekore": 1, 'yentai': 1, 'kapilvastu': 2, 'bhaikaji': 1, 'ghimire': 1, 'directing': 2, 'prostitution': 2, 'prostitutes': 1, 'handicaps': 1, 'pioneering': 1, 'lagham': 1, 'mengistu': 3, 'haile': 2, 'politicize': 2, 'chaudry': 1, '156': 3, 'veloso': 1, 'sebastiao': 1, 'mutilating': 2, 'archives': 3, 'preview': 3, 'abdali': 1, 'arak': 2, 'keg': 1, 'powder': 1, 'servicmen': 1, 'jennings': 1, 'securities': 5, 'trader': 3, 'borrows': 1, 'brokers': 1, 'cuomo': 1, 'deporting': 3, 'wemple': 1, 'stoffel': 1, 'clarified': 1, 'kerem': 1, 'chacon': 3, 'lapi': 1, 'yaracuy': 1, 'salas': 1, 'henrique': 1, 'carabobo': 1, 'akerson': 3, 'turnaround': 3, 'jailbreak': 1, 'banghazi': 1, 'ensures': 2, 'unbeatable': 1, 'abdi': 1, 'dagoberto': 1, 'swirled': 1, 'torah': 2, 'enclaves': 8, 'narrowing': 1, 'redistributed': 1, 'overcrowding': 2, 'discriminate': 1, 'witch': 1, 'mutua': 3, 'shoddy': 3, 'francis': 5, 'muthaura': 1, 'resignations': 6, 'kospi': 1, 'tine': 2, 'noureddine': 1, 'deshu': 2, 'kakar': 2, 'yam': 1, 'marshmallow': 1, 'sibghatullah': 1, 'mujaddedi': 2, 'marshmallows': 1, 'cpp': 2, 'bel': 3, 'intersection': 3, 'benigno': 6, 'aquino': 11, 'broadband': 3, 'hilario': 1, 'davide': 1, 'alluded': 1, 'carreno': 1, 'psychiatrist': 1, 'backyards': 1, 'sughayr': 1, 'talhi': 1, 'abdallah': 4, 'khashiban': 1, 'freezes': 1, 'forbids': 7, 'qari': 5, 'frantic': 1, 'ilbo': 2, 'chosun': 1, 'outline': 4, 'saud': 9, 'continuation': 3, 'abubakar': 5, 'abdulhamid': 1, 'cough': 1, 'kuduna': 1, 'lubroth': 1, 'borrow': 5, 'collateral': 1, 'sinbad': 3, 'wikipedia': 3, 'demise': 1, 'erroneous': 2, 'forwarded': 2, 'adkins': 4, 'rosh': 2, 'hashanah': 2, 'awe': 1, 'strangling': 2, 'meshesha': 1, 'wolde': 1, 'ozcan': 1, 'icelandic': 2, 'disrupts': 1, 'ash': 7, 'aena': 1, 'belching': 1, 'abrasive': 1, 'cordoned': 5, 'minar': 1, 'kye': 3, 'lecture': 1, 'stanford': 2, 'haas': 5, 'yongbyong': 1, 'dinners': 3, 'parachute': 1, 'temptations': 1, 'chesney': 2, 'chill': 2, 'gatemouth': 2, 'clarence': 2, 'zydeco': 1, 'cajun': 1, 'dokie': 1, 'stomp': 1, 'dandy': 1, 'okie': 1, 'supervisor': 3, 'zaw': 1, 'masud': 1, 'shmatko': 2, 'malda': 2, 'murshidabad': 1, 'tyranny': 2, 'greatness': 1, 'bullring': 1, 'levan': 2, 'gachechiladze': 2, 'theofilos': 1, 'malenchenko': 1, 'flowstations': 2, 'kula': 1, 'chevrontexaco': 2, 'eindhoven': 2, 'psv': 1, 'afellay': 1, 'babel': 1, 'sota': 1, 'hirayama': 1, '68th': 1, 'omotoyossi': 1, '32nd': 1, 'fargo': 2, '59th': 1, '5th': 1, 'outposts': 3, 'squatters': 2, 'hilltops': 1, 'evictions': 1, 'thuggish': 1, 'aa': 1, 'bbb': 1, 'fitch': 1, 'intact': 3, 'lbc': 1, 'shidiac': 1, 'judging': 1, 'configuring': 1, 'plainclothes': 2, 'homecoming': 4, 'brining': 1, 'eloy': 1, '820': 1, 'nazarov': 1, 'talbak': 1, 'dushanbe': 2, 'rakhmonov': 1, 'purina': 1, 'ralston': 6, '422': 1, 'seafood': 2, 'cake': 2, 'greenville': 1, 'cincinnati': 1, 'rechargeable': 2, 'volume': 2, 'bread': 3, 'eveready': 1, '1494': 1, 'taino': 3, 'exterminated': 2, '1655': 1, '1834': 2, 'geographically': 2, 'dahlan': 3, 'mohmmed': 1, 'bolstered': 4, 'ethanol': 2, 'expiration': 2, '461': 1, 'distortions': 1, 'statist': 2, 'workshops': 2, 'rigidities': 1, 'weigh': 4, 'mahmud': 3, 'nejad': 1, 'digit': 6, 'excluding': 3, '41st': 2, 'ace': 1, 'fended': 1, 'hydrocarbons': 5, 'limitations': 3, 'decaying': 1, 'neglected': 1, 'telecoms': 1, 'crunch': 3, 'kazakhstani': 1, 'tenge': 1, 'overreliance': 1, 'petrochemicals': 1, 'lucayan': 1, '1492': 4, '1647': 1, 'geography': 2, 'inca': 1, '1533': 1, 'conquest': 5, '1717': 1, 'viceroyalty': 2, '1822': 2, 'offspring': 2, 'handsomest': 2, 'monkey': 2, 'tenderness': 2, 'hairless': 1, 'nosed': 1, 'saluted': 3, 'laugh': 1, 'allot': 2, 'dearest': 1, 'additives': 1, 'paths': 4, 'scattering': 2, 'recede': 1, 'jixi': 1, '828': 1, 'nehe': 1, 'fataki': 1, 'barbey': 1, 'floruan': 1, '154': 3, 'demobilize': 2, 'aiff': 2, 'choudhury': 4, 'pradip': 1, 'houghton': 3, 'fernandes': 1, 'carmo': 1, 'negatively': 4, 'morale': 2, 'deandre': 1, 'soulja': 3, 'crank': 1, 'itunes': 1, 'z': 2, 'pinpoint': 1, 'globalized': 1, 'futures': 4, 'refine': 2, 'abductee': 1, 'admited': 1, 'reparation': 2, 'militarism': 2, 'contrite': 1, 'curtailed': 6, 'stumbling': 2, 'solider': 4, 'sidelined': 3, 'funk': 1, 'recorders': 3, 'divers': 2, 'khayrat': 1, 'shater': 1, 'linguists': 1, 'theoneste': 1, 'bagosora': 3, 'codefendants': 1, '200s': 1, 'bancoro': 2, 'giordani': 1, 'liquidity': 2, 'nationalized': 7, 'recoverable': 1, 'halliburton': 5, '538': 2, 'azeglio': 1, 'ciampi': 1, 'carlo': 4, 'prestige': 1, 'fadilah': 2, 'immunized': 3, '264': 1, 'inhumanely': 1, 'corporal': 4, 'payne': 2, 'receptionist': 2, 'manslaughter': 6, 'routed': 3, 'occupiers': 2, 'aaron': 1, 'nandrolone': 1, 'galindo': 1, 'confrontational': 2, 'somebody': 1, 'rebirth': 1, 'advocating': 5, 'unwavering': 1, 'favorite': 5, 'arinze': 1, 'easterly': 1, '1800': 1, 'converging': 4, 'aktham': 1, 'naisse': 2, 'ennals': 1, 'somalian': 1, 'hatching': 1, 'naise': 1, 'embodied': 1, 'sparrows': 3, 'perfectly': 1, 'bussereau': 1, 'plummets': 1, 'giants': 4, 'finalizes': 1, 'modification': 1, 'barney': 1, 'danielle': 5, 'leeward': 3, 'disseminating': 1, 'usher': 4, 'platinum': 1, 'stylist': 1, 'tameka': 1, 'smalls': 1, 'mtv': 7, 'associations': 4, '311': 2, 'carme': 1, 'yoadimadji': 1, 'yoadimnadji': 3, 'wilds': 1, 'dell': 3, 'silicon': 1, 'outsourcing': 1, 'numbering': 1, '\x96': 6, 'veerman': 3, 'capua': 1, 'emphasizing': 3, 'shih': 1, 'chien': 1, 'golo': 1, 'marra': 3, 'wahid': 1, 'jebel': 2, 'moun': 1, 'omari': 1, '276': 1, 'farooq': 3, 'tap': 2, 'lashing': 2, 'sociology': 1, 'razumkov': 1, 'manning': 5, 'croatians': 1, 'zadar': 1, 'dense': 3, 'zabihullah': 1, 'mujahed': 1, 'canyons': 1, 'naxalites': 1, 'exemplifies': 1, 'massacres': 2, 'interagency': 1, 'welcometousa': 1, 'zahedan': 2, 'guides': 1, 'leaner': 1, 'trimming': 1, 'underreporting': 1, 'moratinos': 1, 'melilla': 8, 'ceuta': 7, 'scaled': 3, 'fences': 5, 'razor': 6, 'novelist': 2, 'booker': 2, 'kiran': 1, 'desai': 4, 'novel': 3, 'inheritance': 1, 'breadth': 1, 'wisdom': 4, 'humane': 3, 'acuteness': 1, 'magnificent': 1, 'granddaughter': 2, 'crumbling': 1, 'orphaned': 2, 'anita': 1, 'blagojevic': 3, 'vidoje': 2, 'genocidal': 1, 'embera': 1, 'jundollah': 2, 'jihadists': 1, 'logical': 3, 'adapt': 2, 'blend': 1, 'receptive': 1, 'norfolk': 1, 'dictators': 1, 'multiply': 1, 'auditorium': 1, 'ogun': 2, 'decontaminating': 1, 'torun': 2, 'boone': 1, 'pickens': 6, 'oilman': 1, 'reno': 1, 'betraying': 3, 'jafarzadeh': 3, 'laser': 1, 'revisit': 2, 'sadrists': 1, 'shakes': 1, 'loach': 1, "d'or": 1, 'barley': 2, 'palme': 1, 'flanders': 1, 'inarritu': 1, 'collectively': 1, 'volver': 1, 'cauvin': 2, 'narino': 1, 'gangchuan': 1, 'cao': 1, 'butheetaung': 1, 'quintana': 2, 'rakhine': 3, 'ojea': 2, 'oo': 4, 'hogan': 2, 'hulk': 1, 'median': 3, 'supra': 1, 'bollea': 4, 'clearwater': 2, 'shelor': 2, 'extricated': 1, 'graziano': 2, 'bayfront': 1, 'jassar': 3, 'rawi': 1, 'jibouri': 1, 'speedskater': 3, 'cheek': 8, 'joey': 2, 'emphasizes': 2, 'champ': 1, 'johann': 1, 'koss': 1, 'zetas': 4, 'hidalgo': 1, 'cardenas': 5, 'lazcano': 1, 'heriberto': 1, 'endure': 3, 'samoa': 2, 'retaken': 1, 'primitive': 1, 'caroline': 1, 'nkurunziza': 2, 'pastured': 1, 'bulls': 1, 'guileful': 1, 'feasted': 1, '237': 1, 'lease': 4, 'overland': 2, 'bentegeat': 1, 'refusals': 1, 'rahama': 1, 'granville': 1, 'abdelrahman': 1, 'confessing': 1, 'disgrace': 2, 'ireju': 1, 'bares': 1, 'furious': 1, 'messaging': 1, 'teaches': 3, 'dalailama': 1, 'evan': 1, 'floundering': 1, 'reasonably': 3, 'besigye': 15, 'kizza': 4, 'gwan': 2, 'commandoes': 2, 'script': 1, 'mauled': 2, 'lip': 5, "xi'an": 1, 'xijing': 1, 'eyebrow': 1, 'recluse': 1, 'disfigurement': 1, 'loose': 6, 'sadness': 4, 'tirelessly': 1, 'beings': 2, 'denuclearize': 1, 'papacy': 6, 'fini': 2, 'gianfranco': 1, 'bezoti': 1, 'marshburn': 1, '40th': 1, 'kopra': 3, 'wakata': 2, 'koichi': 2, 'tuning': 1, 'macchiavello': 1, 'overthrowing': 1, 'patterns': 2, 'crossroads': 2, 'guaranteeing': 3, 'narrows': 1, 'rohmer': 5, 'relationships': 7, 'philosophical': 2, 'maud': 1, 'claire': 2, 'unionist': 3, 'paisley': 4, 'fein': 4, 'sinn': 4, 'chastelain': 1, 'unitary': 1, 'pristina': 6, 'borys': 1, '247': 2, 'lutsenko': 1, 'tsushko': 1, 'staunchest': 1, 'despises': 1, 'fodder': 3, 'tabloids': 1, 'operas': 1, 'soap': 1, 'alien': 1, 'confer': 3, 'fulfills': 2, 'ruggiero': 3, 'straits': 2, '982': 1, 'hafeez': 2, 'ascend': 2, 'chrysanthemum': 2, 'heir': 2, 'recommending': 4, 'vovk': 1, 'rs': 1, 'yar': 1, '12m': 1, 'kapustin': 1, 'teaching': 6, 'rosa': 2, 'floats': 1, 'strings': 1, 'lesser': 7, 'climatic': 2, 'lenten': 1, 'packs': 1, 'grassland': 1, 'demostrators': 1, 'cannons': 3, 'slutskaya': 2, 'evgeny': 1, 'skate': 1, 'plushenko': 1, 'skaters': 2, 'entrants': 2, 'volchkova': 1, 'sokolova': 1, 'elena': 2, 'viktoria': 1, 'kostomarov': 1, 'navka': 1, 'dancers': 1, 'technocrats': 2, 'creativity': 2, 'greener': 1, 'equalize': 1, 'utiashvili': 1, 'shota': 1, 'turbine': 1, 'poti': 1, 'antics': 1, 'fonda': 3, 'scolded': 3, 'jane': 3, 'publicized': 4, 'capp': 1, 'massey': 3, 'wooten': 1, 'raleigh': 1, 'charleston': 3, 'manchin': 1, 'gazette': 1, 'sago': 1, 'define': 1, 'fractious': 1, 'distinguish': 2, 'absent': 5, 'marker': 1, 'ruutel': 1, 'estonian': 1, 'andrus': 1, 'ansip': 3, 'juhan': 1, 'shevchenko': 1, 'andriy': 1, 'ronaldo': 2, 'balking': 1, 'qeshm': 1, 'unhitches': 1, '1600': 1, 'unintentional': 1, 'molded': 1, 'demonized': 1, 'explaining': 3, 'roseneft': 2, 'yugansk': 3, 'managerial': 1, 'asses': 3, 'mate': 4, 'plouffe': 1, 'erik': 5, 'solheim': 5, 'misallocation': 1, 'renegotiate': 1, 'wmd': 1, 'fiercest': 2, 'blanketed': 3, 'adhamiyah': 1, 'waziriyah': 1, 'muscular': 1, 'vahiuddin': 1, 'shafi': 1, 'calcutta': 2, 'toiba': 6, 'ghettos': 2, 'inward': 1, 'cartoonists': 1, 'depictions': 4, 'civilizations': 1, 'bandage': 1, 'kulov': 4, 'runners': 4, 'combs': 6, 'mogul': 2, 'diddy': 2, 'rechnitzer': 3, 'salvage': 2, 'retreating': 1, 'bosco': 2, 'katutsi': 2, 'iowa': 17, 'gamma': 3, 'dissipate': 1, 'coasts': 1, 'coppola': 1, 'limon': 1, 'elgon': 3, 'sirisia': 1, 'sabaot': 1, 'exempt': 3, 'goverment': 1, 'beitenu': 1, 'yisrael': 1, 'avigdor': 3, 'calf': 1, 'kulyab': 2, 'displeased': 1, 'ordinance': 2, 'newfoundland': 1, '348': 2, 'sq': 1, 'subsidizes': 2, 'cashew': 1, 'haunch': 1, 'mutton': 1, 'hut': 1, 'shepherds': 3, 'kernels': 1, 'peanuts': 2, 'undp': 1, 'dahomey': 1, '1872': 4, 'clamor': 1, 'yayi': 3, 'boni': 2, 'outsider': 1, 'cumulative': 1, 'dampened': 2, 'nigh': 1, 'squid': 2, 'furnish': 1, 'wool': 2, 'dane': 2, 'dampen': 1, 'commercially': 4, 'abated': 1, 'eco': 2, 'perch': 1, 'envy': 2, 'jackdaw': 2, 'emulate': 3, 'fleece': 1, 'entangled': 1, 'fluttered': 1, 'wings': 7, 'whir': 2, 'clipped': 1, 'daw': 2, 'woodcutter': 1, 'expedient': 1, 'importunities': 1, 'fearfully': 4, 'extract': 3, 'suitor': 1, 'cheerfully': 1, 'assented': 2, 'clawless': 1, 'woodman': 1, 'toothless': 1, 'hemp': 3, 'hopping': 2, 'sowing': 2, 'repent': 1, 'heed': 3, 'despised': 2, 'messed': 1, 'aisle': 1, 'crapping': 1, 'filsi': 1, 'tayr': 1, 'recommends': 3, 'rehnquist': 7, 'pausing': 2, 'nixon': 2, 'roberts': 9, 'panhandle': 2, 'solemly': 1, 'hallandale': 2, 'boating': 2, 'obsolete': 1, 'shalikashvili': 1, 'inscribed': 2, 'romano': 3, 'prodi': 5, 'tacitly': 1, 'cardboard': 1, 'reinstatement': 3, 'deliberating': 1, 'todovic': 3, 'savo': 1, 'comprehend': 1, 'timoshenko': 2, 'distracted': 1, 'balikesir': 1, 'rezko': 4, 'antoin': 1, 'foca': 4, 'dom': 1, 'interrogations': 5, 'afrik': 1, 'chariot': 6, 'tutankhamun': 1, 'antiquities': 7, 'tut': 2, '1922': 4, 'mussa': 6, 'taif': 2, 'skyline': 1, 'stephanie': 2, 'demerits': 1, 'misused': 1, 'breaching': 2, 'blanked': 1, 'emmen': 1, 'argentines': 2, '52nd': 1, 'harrison': 2, 'otalvaro': 1, 'lionel': 1, 'messi': 1, 'taye': 1, 'taiwo': 1, 'doetinchem': 1, 'g7': 4, 'kolesnikov': 1, '331': 1, 'wirajuda': 1, 'bahlul': 2, 'medic': 3, 'sassi': 1, '347': 1, 'oviedo': 1, 'lammert': 1, 'norbert': 1, 'diminish': 2, 'girona': 1, 'bigots': 1, 'horror': 4, 'apure': 1, 'catalonians': 1, 'luton': 2, 'backpacks': 2, 'esteban': 1, 'rocio': 1, 'cooperativa': 1, 'boniface': 1, 'alexandre': 3, 'ramazan': 2, 'localized': 1, 'wikileaks': 3, 'aftenposten': 1, 'arian': 3, 'sami': 2, 'noneducational': 1, 'understood': 2, 'reschedule': 3, 'proliferate': 1, 'novin': 2, 'mesbah': 2, 'judgment': 8, 'regina': 1, 'reichenm': 1, 'reassurance': 1, 'douglas': 2, 'infomation': 1, '569': 1, 'badakshan': 2, 'depth': 3, 'mohean': 1, 'vladislav': 3, 'ardzinba': 4, 'khadjimba': 4, 'khashba': 1, 'nodar': 1, 'bagapsh': 8, 'voided': 1, '186': 2, 'gali': 1, 'majmaah': 1, 'kharj': 2, 'assorted': 1, 'vicious': 3, 'rodon': 1, 'sinmun': 2, 'somaliland': 2, 'hargeisa': 1, 'maigao': 1, 'paksitan': 1, 'pollsters': 3, '1718': 1, 'safta': 1, '2013': 3, 'forge': 3, 'cynics': 1, 'naysayers': 1, 'strenuously': 1, 'disappear': 3, 'renovations': 2, 'balancing': 1, 'reauthorize': 2, 'redeployment': 2, 'assumptions': 1, 'disturbingly': 1, 'hezb': 2, 'olympia': 2, 'relays': 1, 'patan': 2, 'merajudeen': 1, 'stanishev': 2, 'reprocessing': 3, 'odom': 2, 'squander': 1, 'invading': 3, 'compounded': 3, '181': 2, 'hospitalizations': 1, 'diverting': 2, 'gripped': 1, 'hurry': 1, '50th': 1, 'ysidro': 1, 'embolden': 2, 'adjust': 5, 'diameter': 1, 'fushun': 2, 'sinuiju': 1, 'portman': 1, 'rob': 2, 'iiss': 1, 'hails': 1, 'tipping': 1, 'badaun': 1, 'organiation': 1, 'pedestrians': 4, 'shahawar': 1, 'brooklyn': 1, 'siraj': 3, 'stoked': 1, 'matin': 1, 'impress': 1, 'eldawoody': 2, 'flagship': 2, 'frazier': 2, 'observes': 3, "qur'an": 1, 'scud': 1, 'showering': 1, 'machines': 8, 'heist': 2, 'thankful': 1, 'agendas': 1, 'discretion': 2, 'bradford': 1, 'disburses': 1, 'ernst': 1, 'kasami': 1, 'shab': 1, 'barat': 1, 'malegaon': 2, '1621': 1, 'sophistication': 1, 'cpsc': 1, 'toys': 4, 'dallas': 4, 'minivan': 2, 'trailer': 2, 'undocumented': 2, 'darfuri': 1, 'chieftain': 1, 'eissa': 1, 'rizeigat': 2, 'aliu': 2, 'mas': 3, 'ghazal': 1, 'bahr': 4, 'hinges': 2, 'tandem': 1, 'jebaliya': 1, 'podemos': 1, 'akylbek': 1, 'sariyev': 2, 'ricans': 1, 'plebiscites': 1, 'blatantly': 1, 'guei': 1, 'disaffected': 1, 'linas': 1, 'marcoussis': 1, 'soro': 2, 'guillaume': 1, 'ouagadougou': 1, 'reintegration': 1, 'dramane': 1, 'pleasant': 1, 'nonpolluting': 1, 'thrives': 1, 'jurisdictions': 1, 'monopolies': 1, 'tamper': 1, 'computerized': 1, 'biometric': 1, 'reassert': 1, 'telescopes': 1, 'optician': 1, 'annapolis': 2, 'munificent': 1, 'patronage': 1, 'languishing': 2, 'desirous': 1, 'kangaroo': 1, 'bulky': 2, 'awkwardly': 1, 'pouch': 1, 'deceitful': 1, 'insupportable': 1, 'smiling': 2, 'wit': 3, 'consciousness': 5, 'cheerless': 1, 'implored': 1, 'unappreciated': 1, 'endow': 1, 'wag': 2, 'creator': 2, 'thereof': 1, 'resentment': 1, 'affection': 4, 'fulness': 1, 'chin': 1, 'incaudate': 1, 'wags': 1, 'gratification': 1, 'conferred': 5, 'pluto': 5, 'charon': 1, 'geology': 1, 'kuiper': 1, 'payenda': 1, 'rocketed': 1, 'caves': 1, 'subverting': 1, 'issoufou': 1, 'mahamadou': 1, 'sarturday': 1, 'hassas': 1, 'shahidi': 2, 'checkpost': 1, 'khela': 1, 'barbershops': 1, 'jabber': 1, 'belgaum': 1, 'alias': 3, 'gurage': 1, 'mohamuud': 1, 'musse': 1, 'dastardly': 1, 'responsibilty': 1, 'ossetian': 1, 'katsina': 1, 'annulment': 2, 'acquittals': 1, 'convict': 1, 'hostilities': 6, 'dormant': 2, 'mazda': 1, 'outsold': 1, 'volkswagen': 1, 'agadez': 1, 'uninsured': 2, 'initials': 3, 'mnj': 1, 'bales': 2, 'immobilized': 1, 'pastures': 2, 'crusted': 1, 'drifts': 1, 'injection': 4, 'siding': 1, 'antonin': 1, 'scalia': 1, 'hermogenes': 1, 'esperon': 1, 'patek': 1, 'dulmatin': 1, 'suspiciously': 1, 'behaving': 1, 'insistence': 4, 'stopper': 1, 'saberi': 6, 'roxana': 2, 'evin': 1, 'genetically': 4, 'cobs': 1, 'hats': 1, 'fillon': 5, 'recognizable': 1, 'pests': 1, 'monsanto': 1, 'frattini': 1, 'konare': 6, 'oumar': 4, 'deterioration': 4, 'detached': 2, 'strangely': 1, 'chairwoman': 2, 'colllins': 1, 'ineffectively': 1, 'galveston': 1, 'aurangabad': 1, 'laredo': 9, 'berrones': 1, 'casings': 2, 'lao': 3, 'apache': 2, 'abusive': 5, 'yankee': 2, 'colors': 1, 'handkerchiefs': 1, 'dazzled': 1, 'filmmakers': 3, 'documentaries': 1, 'restless': 1, 'frontline': 2, 'pbs': 2, 'suli': 3, 'katyusha': 3, 'controller': 1, 'nevzlin': 4, 'sunda': 1, 'encircling': 1, 'arc': 2, 'volcanos': 1, 'grace': 2, 'val': 4, 'gaule': 1, 'dabaan': 1, 'nuri': 2, 'baalbek': 1, 'naqoura': 3, 'swearing': 4, 'ural': 1, 'distorted': 1, 'potent': 2, 'unbiased': 1, 'inquiries': 2, 'migrate': 1, 'roshan': 1, 'interpretations': 2, 'pepsico': 6, 'pepsi': 1, 'nooyi': 3, 'indra': 1, 'undergraduate': 1, 'madras': 1, 'graduate': 1, 'yale': 1, 'skillfully': 1, 'sightings': 1, 'congregate': 1, 'surroundings': 2, 'lunchtime': 2, 'grower': 1, 'masjid': 1, 'eyewitness': 1, 'excrement': 2, 'preachers': 2, 'forgiving': 1, 'motivation': 2, 'geologist': 1, 'geofizyka': 1, 'krakow': 1, 'stanczak': 1, 'piotr': 1, 'contributors': 1, 'rehearsing': 1, 'revelry': 2, 'penitence': 1, 'unami': 1, 'rancher': 2, 'apondi': 2, 'delamere': 1, 'muga': 1, 'cholmondeley': 3, 'harbored': 1, 'sympathetic': 3, 'nonassociated': 1, '2022': 1, 'causeway': 1, 'maastricht': 2, 'amerindians': 1, 'annihilated': 1, '1697': 2, 'revolted': 1, "l'ouverture": 1, '1804': 2, 'inaugurate': 1, 'postponements': 2, 'neighbours': 1, 'avarice': 1, 'neighbour': 3, 'avaricious': 1, 'envious': 1, 'vices': 1, 'farmyard': 3, 'grievously': 2, 'spectator': 1, 'shake': 3, 'devoured': 2, 'scratched': 1, 'surfeited': 2, 'jog': 1, 'nursing': 2, 'enables': 1, 'kalfin': 1, 'ivaylo': 1, 'calculated': 1, 'preparatory': 1, 'sogo': 3, 'seibu': 3, 'convenience': 1, 'afghani': 1, 'magloire': 1, 'consult': 5, 'chains': 2, 'retailing': 1, 'diary': 3, 'kezerashvili': 2, 'davit': 1, 'nomura': 1, 'parachinar': 4, 'sahibzada': 2, 'anis': 2, 'constatin': 1, '217': 1, 'occidental': 1, 'kasai': 1, 'kanow': 2, 'burials': 1, 'irin': 1, 'ears': 5, 'dominating': 1, 'ilya': 1, 'kovalchuk': 1, 'goaltender': 1, 'evgeni': 1, 'shutout': 1, 'nabokov': 1, 'surging': 6, 'clinched': 2, 'suleimaniya': 1, 'suspecting': 3, 'abating': 3, 'abolishes': 1, 'blacklisted': 3, 'tier': 1, '482': 1, '203': 4, 'salami': 2, 'tor': 2, 'm1': 1, 'belfort': 1, 'skied': 2, 'whistler': 1, 'ivica': 2, 'zurbriggen': 1, '100ths': 2, 'dlamini': 1, 'nkosazana': 1, 'transitioning': 1, 'extraditions': 1, 'revert': 1, 'mamoun': 1, 'darkazanli': 2, 'sphere': 4, 'enlargement': 3, 'unstoppable': 1, 'paule': 1, 'kieny': 1, 'obesity': 6, 'irreversible': 1, 'zuckerberg': 1, 'entrepreneur': 2, 'billionaires': 1, 'unfolding': 2, 'ubiquitous': 1, 'bacterium': 1, 'guni': 1, 'rusere': 1, 'frenk': 1, 'revoking': 2, 'otto': 1, 'molina': 5, 'finishers': 1, 'cpj': 1, '090': 1, 'prosper': 1, 'alcoa': 1, 'santuario': 1, 'guatica': 1, 'restrepo': 1, 'reintegrate': 1, 'downbeat': 1, 'hausa': 1, 'impressions': 1, 'showered': 1, 'unprotected': 1, 'undermines': 2, 'intercourse': 1, 'ibaraki': 2, 'contingency': 1, 'sabawi': 2, 'jvp': 2, 'sars': 3, 'clinching': 1, 'verge': 1, 'huckabee': 1, 'sayyed': 1, 'tantawi': 1, 'bioterrorism': 1, 'accommodating': 1, 'purifying': 1, 'emptive': 3, 'bleak': 2, 'unhelpful': 2, 'navtej': 1, 'sarna': 1, 'trapping': 4, 'decorations': 6, 'billboards': 3, 'fascists': 1, 'swastika': 2, 'neon': 1, 'sizes': 1, 'underfunding': 1, 'baltasar': 1, 'garzon': 1, 'binalshibh': 1, 'ramzi': 1, 'ezirouali': 1, 'tahar': 1, 'heptathlon': 1, 'blonska': 3, 'dobrynska': 1, 'natalia': 1, 'tamimi': 2, 'alwan': 1, 'baquoba': 1, 'motorcycles': 6, 'shaways': 3, 'rowsch': 2, 'doumgor': 1, 'hourmadji': 1, 'chadians': 1, 'duekoue': 4, 'zia': 5, 'khaleda': 1, 'taka': 1, 'tarique': 1, 'zhuang': 1, 'collaborative': 1, 'cabezas': 1, 'unsatisfactory': 1, 'inflationary': 2, 'zeros': 1, 'dzhennet': 1, 'abdurakhmanova': 1, 'umalat': 1, 'magomedov': 1, 'ustarkhanova': 2, 'markha': 1, 'ramzan': 4, 'kadyrov': 6, 'videotapes': 4, 'inflame': 2, 'leashes': 1, 'blogger': 6, 'kareem': 3, 'illustrates': 1, 'relented': 1, 'reappointing': 1, 'aberrahman': 1, 'hamed': 3, 'unprofessional': 2, 'epsilon': 4, 'catastrophes': 1, 'happening': 1, 'doctrine': 4, 'penetrating': 2, 'envisions': 2, 'busters': 1, 'tauran': 2, 'zaragoza': 4, 'gmt': 1, 'denisov': 1, 'underline': 1, 'andrey': 1, '373': 3, 'erode': 3, "madai'ni": 1, 'overrunning': 1, '249': 1, 'particulate': 1, 'cavic': 1, 'pluralism': 2, 'lodin': 1, 'azizullah': 1, 'evangelical': 1, 'nita': 2, 'zelenak': 1, 'nipayia': 1, 'asir': 1, 'handwriting': 1, 'raouf': 3, 'shorja': 1, 'inflaming': 1, 'uedf': 1, 'cud': 3, 'vaccinating': 1, 'goot': 1, 'jeanet': 1, 'infectiousness': 1, 'thereby': 1, 'inoculation': 1, '1765': 1, 'isle': 5, 'hebrides': 3, 'gaelic': 1, 'manx': 1, 'extinct': 2, 'cultures': 3, 'idi': 1, 'promulgated': 1, 'amending': 1, 'loosened': 2, 'respectable': 1, 'ratsiraka': 2, 'didier': 3, 'antananarivo': 1, 'lessened': 2, 'simplification': 1, 'mitigated': 1, 'graduates': 1, 'bedside': 3, 'vineyards': 1, 'mattocks': 1, 'spades': 1, 'repaid': 1, 'superabundant': 1, 'mechanisms': 1, 'melody': 2, 'preserved': 4, 'pleases': 1, 'surety': 1, 'begging': 1, 'coward': 1, 'wheelchair': 4, 'waist': 1, 'stool': 2, 'cookstown': 1, 'paralysed': 1, 'tyrone': 1, 'newell': 2, 'negligent': 1, 'letten': 2, 'levees': 10, 'misconduct': 3, 'bernazzani': 1, 'nacion': 1, 'kenjic': 2, 'mladjen': 1, 'vojkovici': 1, 'fraught': 1, 'detachment': 3, 'petitioning': 2, 'kiwayu': 1, 'pitted': 2, 'sleng': 1, 'tuol': 1, 'duch': 1, 'ta': 1, 'amezcua': 2, 'adan': 1, 'stimulant': 6, 'minds': 2, 'mirrored': 1, 'despondent': 1, 'boulevard': 1, 'hobart': 3, 'filipchuk': 1, 'vasilily': 1, 'shakespeareans': 1, 'thespians': 1, 'introduces': 3, 'zaher': 1, 'sardar': 2, 'barometer': 2, 'lifeline': 1, 'georgians': 2, 'vaccinated': 1, 'guideline': 1, 'rosal': 1, 'gregorio': 2, 'deserts': 1, 'greenery': 1, 'blick': 1, 'sonntags': 1, 'reassigned': 1, 'millerwise': 1, 'dyck': 1, 'hierarchical': 1, 'alec': 2, 'staffed': 1, 'krasnokamensk': 1, 'chita': 2, 'pleshkov': 1, 'forays': 1, 'reprisal': 3, '\x93': 2, 'harcharik': 1, 'faisal': 8, 'angioplasty': 1, 'pakhtunkhwa': 2, 'impeding': 1, 'schedules': 1, 'hadjiya': 1, 'handcuffed': 2, 'skirmishes': 3, 'bands': 2, 'terrorize': 1, 'fldr': 1, 'canine': 1, 'soiling': 1, 'handler': 1, 'ketzer': 1, 'discharge': 2, 'emptively': 1, 'halfun': 1, 'bartlett': 2, 'weaker': 4, 'spiked': 2, 'haroon': 2, 'aswat': 5, '30s': 1, 'niyazov': 1, 'schulz': 1, 'corroborate': 1, 'shek': 1, 'chevallier': 1, 'jarrah': 1, 'ayalon': 1, 'danny': 1, 'rude': 1, 'coldplay': 1, 'paltrow': 2, 'gwyneth': 3, 'opined': 1, 'folha': 2, 'paolo': 1, 'nicest': 1, 'testy': 1, 'ringtone': 1, '068171296': 1, '068217593': 1, 'edging': 1, 'aufdenblatten': 1, '068263889': 1, 'fraenzi': 2, '068275463': 1, '685': 1, '782': 1, 'redesigned': 1, 'slope': 1, 'sangju': 1, '4000': 1, 'paz': 6, 'mexicali': 1, 'marghzar': 1, 'underfunded': 1, 'unprepared': 1, 'comrade': 1, 'infromation': 2, 'nigerla': 1, 'shoppertrak': 1, 'rct': 1, 'wielding': 5, 'nutritional': 2, 'blanket': 2, 'quietly': 1, 'elaborating': 1, 'chaparhar': 1, 'sprawling': 2, 'radioactivity': 1, 'expatriates': 2, 'narim': 1, 'alcolac': 1, 'vwr': 1, 'fisher': 3, 'thermo': 1, 'fujian': 7, 'tributary': 2, 'yangtze': 2, 'avert': 4, 'maruf': 1, 'bakhit': 1, 'fayez': 1, 'vest': 2, 'ncri': 2, '630': 2, '55th': 2, 'minia': 1, 'sights': 1, 'reaping': 1, 'exxon': 1, 'sanchez': 4, 'hers': 1, 'baloyi': 2, 'remixed': 1, 'hop': 2, 'cheadle': 1, 'portray': 3, 'benham': 1, 'natagehi': 1, 'paratroopers': 1, 'excursion': 1, 'retreated': 3, 'landale': 1, 'mirko': 1, 'ademi': 1, 'norac': 1, 'medak': 1, 'gall': 1, 'bladder': 1, 'raffarin': 5, 'reims': 1, 'penjwin': 1, 'mukasey': 5, 'durham': 1, 'taxiways': 1, 'hornafrik': 1, 'abdirahman': 1, 'dinari': 1, 'sreten': 1, 'lukic': 3, 'vascular': 1, '1726': 1, '1828': 1, 'tupamaros': 1, 'yearend': 2, 'frente': 1, 'amplio': 1, 'freest': 1, 'pa': 3, 'intifada': 2, 'fayyad': 1, 'salam': 1, 'uptick': 1, 'mandeb': 1, 'hormuz': 1, 'malacca': 1, 'cranes': 1, 'plowlands': 1, 'sling': 2, 'brandishing': 1, 'earnest': 1, 'forsook': 1, 'liliput': 1, 'suffice': 1, 'crept': 4, 'oak': 2, 'hearty': 2, 'lament': 3, 'groan': 1, 'cries': 3, 'escutcheon': 3, 'orator': 3, 'unblotted': 3, 'organ': 2, 'finger': 1, 'scorn': 2, 'whitewash': 1, 'misdeeds': 1, 'mortification': 1, 'tired': 2, 'partitioning': 2, 'unsettling': 1, 'buyout': 1, 'breakout': 1, 'machetes': 2, 'kuru': 1, 'wareng': 1, 'ladi': 1, 'barakin': 1, 'jos': 5, 'haram': 2, 'assumes': 4, 'anwarul': 1, 'foy': 3, 'ghani': 2, 'usman': 1, 'phases': 2, 'unseated': 1, 'shoving': 4, 'railed': 1, 'undetected': 2, 'wadia': 1, 'jaish': 1, 'hafsa': 1, 'misusing': 3, 'hiked': 1, 'busier': 1, 'quelled': 1, 'harbi': 4, 'yaounde': 1, 'debriefed': 2, 'cancels': 1, 'bed': 4, 'fasted': 1, 'saada': 1, 'nidal': 1, 'amona': 1, 'overstaying': 1, 'zenani': 2, 'culpable': 1, 'homicide': 4, 'grandchildren': 1, 'browsing': 2, 'hrd': 1, 'computing': 2, 'laptop': 9, 'sibal': 3, 'conferencing': 2, 'kapil': 1, 'gadget': 2, 'ipad': 4, 'linux': 1, 'campuses': 3, 'glacier': 7, 'perito': 1, 'glacieres': 1, 'spectacular': 1, 'breakup': 6, 'khorramshahi': 1, 'abdolsamad': 1, 'shatters': 1, 'dharamsala': 3, 'martic': 3, 'interception': 1, 'arjangi': 1, 'conson': 2, 'elliott': 1, 'abrams': 1, 'welch': 1, 'catandunes': 1, 'floodwalls': 2, 'berkeley': 2, 'minke': 2, 'crusade': 1, 'satirical': 1, 'eternal': 4, 'anesthetic': 2, 'bharti': 2, 'duke': 3, 'shopkeepers': 2, 'rockefeller': 1, 'zaldivar': 1, 'detainment': 3, 'treacherous': 1, 'gusting': 1, 'pile': 1, 'trnava': 1, 'unaffected': 2, 'compensating': 1, 'ethem': 2, 'erdagi': 2, 'tuition': 1, 'horses': 2, 'biak': 1, 'yearly': 4, 'tremendously': 1, 'amazed': 2, 'deception': 2, 'substantiate': 1, 'supposition': 1, 'involuntary': 1, 'callup': 1, 'chimango': 1, 'asgiriya': 1, '188': 1, 'kandy': 1, 'panesar': 3, 'toss': 3, 'seam': 1, 'spinner': 1, 'monty': 1, 'hoggard': 3, 'collingwood': 1, 'sangakkara': 2, 'prasanna': 1, 'alastair': 1, 'jayawardene': 1, 'gana': 1, 'kingibe': 1, 'baba': 3, 'divisive': 2, 'unintended': 1, 'pregnancies': 2, 'intrude': 1, 'roe': 2, 'legalizing': 2, 'liken': 1, 'conception': 1, 'latifullah': 1, 'banditry': 3, 'sha': 2, 'zukang': 1, 'dictate': 3, 'shawel': 1, 'hailu': 2, 'abqaiq': 3, 'mosaic': 1, 'farthest': 1, 'bang': 1, 'snapshot': 1, 'turbans': 1, 'mature': 1, 'sorts': 1, 'galactic': 1, 'clarity': 1, 'ammar': 2, 'nom': 1, 'guerre': 1, 'muqataa': 1, 'masons': 1, 'royalty': 2, 'morality': 1, 'wearers': 1, 'headdress': 1, 'detector': 2, 'irreparable': 2, 'escalates': 1, 'grievances': 6, 'shaaban': 1, 'muntadar': 2, 'nhk': 2, 'arbor': 2, 'tsa': 2, 'grassroots': 2, 'plantings': 1, 'overshadows': 2, 'headgear': 1, 'medicare': 6, 'prescription': 8, 'seniors': 4, 'preventative': 1, 'enroll': 2, 'pronounces': 1, 'subjecting': 2, 'commons': 2, 'protégé': 1, 'suited': 1, 'kalla': 1, 'jusuf': 1, 'mukmin': 1, 'mammy': 1, 'maiduguri': 4, 'fare': 1, 'mohtarem': 1, 'manzur': 1, 'births': 2, 'disengage': 1, 'rowhani': 7, 'adamant': 1, 'muntazer': 2, 'zaidi': 5, 'ducked': 1, 'masterminded': 1, 'murderers': 3, 'hangings': 1, 'khazaee': 1, 'invalid': 2, 'tightens': 1, 'reluctantly': 1, '1713': 1, 'utrecht': 1, 'gibraltarians': 1, 'tripartite': 1, 'cooperatively': 1, 'noncolonial': 1, 'sporadically': 1, '1747': 1, 'durrani': 1, 'empires': 3, 'notional': 1, '1919': 2, 'experiment': 1, 'tottering': 1, 'relentless': 1, 'ladin': 1, 'bonn': 1, 'manipulation': 3, 'sandinista': 5, 'contra': 3, 'saavedra': 1, 'mitch': 1, 'depressed': 6, 'polarized': 2, 'buoyant': 1, 'rope': 3, 'soaked': 2, 'strange': 1, 'lacerated': 1, 'faint': 2, 'scampered': 2, 'woe': 2, 'belabored': 1, 'tonight': 1, 'toil': 1, 'rut': 1, 'waggon': 1, 'hercules': 5, 'exertion': 1, 'tuba': 1, 'firmn': 1, 'hotbeds': 1, 'mitsumi': 1, 'overtime': 2, 'quran': 2, 'pastor': 3, 'puli': 1, 'terry': 2, 'obscure': 1, 'mizhar': 1, '618': 1, 'varema': 1, 'divorced': 3, 'pummeling': 1, 'northwesterly': 1, 'therapies': 1, 'lobby': 4, 'vitamin': 1, 'retroviral': 3, 'pitting': 3, 'matthias': 1, 'rath': 2, 'defamatory': 2, 'tac': 3, 'nutrients': 3, 'vorachit': 1, 'bounnyang': 1, 'ome': 1, 'taj': 1, 'nenbutsushu': 2, 'macedonians': 1, '463': 1, '109': 4, 'counterattack': 1, 'hogg': 4, 'yuganskeneftegaz': 1, 'mahmanparast': 1, 'ramin': 1, 'mobs': 2, 'dimona': 3, 'adhaim': 1, 'waxman': 2, 'emissary': 1, 'censors': 1, 'sudharmono': 4, 'suharto': 2, 'golkar': 1, 'jonah': 1, 'brass': 1, 'viewing': 2, 'unopposed': 2, 'nasdaq': 3, '532': 1, 'cac': 3, 'dax': 3, '864': 1, '406': 1, 'ounce': 3, 'electricidad': 1, 'edc': 1, 'aes': 2, 'nationalizations': 1, 'mouthing': 1, 'articulated': 1, 'iadb': 1, 'ramda': 5, 'crimping': 1, 'breakdowns': 2, 'tomohito': 3, 'mikasa': 1, 'emperors': 2, 'heirs': 2, 'concubines': 1, 'trusted': 2, 'counterfeit': 3, 'rsf': 5, 'photographers': 4, 'bighi': 1, 'saidi': 1, 'somaieh': 1, 'nosrati': 1, 'tohid': 1, 'matinpour': 1, 'henghameh': 1, 'concede': 5, '239': 1, 'sinopec': 2, 'vitoria': 2, 'espirito': 1, 'catu': 1, 'bahia': 1, 'gasene': 1, 'datafolha': 2, 'zoran': 2, 'djindjic': 5, 'covic': 2, 'nebojsa': 1, 'milenkovic': 2, 'dejan': 1, 'bugsy': 1, 'seselj': 4, 'instrumental': 1, 'baluyevsky': 2, 'hajime': 1, 'massaki': 1, 'mutalib': 1, 'convening': 1, 'procedural': 1, 'clyburn': 2, 'gamble': 1, 'affordable': 3, 'baki': 3, 'andar': 1, 'rejoining': 1, 'dunham': 3, 'madelyn': 1, 'impregnating': 1, 'dusan': 2, 'tesic': 2, 'matabeleland': 1, 'counterfeiting': 1, 'hargreaves': 2, 'geologists': 1, 'ranges': 2, 'confessions': 1, 'censored': 1, 'piot': 1, 'pears': 1, 'shoe': 1, 'baitullah': 1, 'perpetuating': 1, 'ericq': 1, 'alexis': 3, 'edouard': 2, 'cayes': 1, 'les': 1, 'attainable': 1, 'augustin': 1, 'haro': 3, 'welt': 1, 'saroki': 1, 'overheating': 3, 'predictions': 3, 'bolder': 1, 'cautions': 2, 'cutbacks': 3, 'curve': 4, 'mountainside': 2, 'morin': 1, 'herve': 1, 'areva': 1, 'stupid': 2, 'gay': 12, 'californian': 1, 'silbert': 1, 'marriages': 2, 'gujarat': 2, 'hamel': 1, 'chuck': 1, 'corrosion': 1, 'prudhoe': 1, 'diseased': 2, 'implanted': 1, 'manufactures': 1, 'syncardia': 1, 'tucson': 2, 'eskisehir': 1, 'nabbed': 2, 'kiliclar': 1, 'nazer': 1, 'osprey': 1, 'reacts': 1, 'garner': 2, 'concluding': 3, 'obey': 2, 'sticking': 5, 'unfold': 1, 'blogs': 1, 'weblogs': 1, 'saadi': 3, 'aleim': 1, 'gothenburg': 1, 'scandinavia': 1, 'gungoren': 1, 'savage': 1, 'olusegan': 1, 'ahronoth': 3, 'yedioth': 3, 'trouncing': 1, 'prefers': 1, 'taker': 1, 'denials': 2, '444': 4, 'slaying': 4, 'younes': 1, 'megawatt': 1, 'dinar': 1, 'deutchmark': 1, 'privatized': 3, 'infusion': 1, 'criminality': 1, 'insecurity': 5, 'fundamentals': 3, 'chernobyl': 1, 'lows': 3, 'expansionary': 5, 'riverine': 1, 'semidesert': 1, 'adherence': 2, 'devaluation': 1, 'cfa': 2, 'jeopardized': 2, 'predominate': 1, 'breadfruit': 2, 'tomatoes': 4, 'melons': 1, 'ranches': 1, 'exemptions': 1, 'booty': 1, 'modestly': 2, 'spoil': 2, 'heap': 2, 'witnessing': 1, 'learns': 1, 'misfortunes': 2, 'requite': 1, 'kindness': 1, 'beg': 1, 'flattered': 1, 'yours': 2, 'eats': 1, 'messes': 1, 'behaves': 1, 'cubapetroleo': 1, 'cnbc': 1, 'bell': 2, 'coercive': 1, 'conniving': 1, 'dismisses': 1, 'protocols': 2, 'doubting': 1, 'chests': 1, 'beltran': 1, 'shyloh': 1, 'giddens': 2, 'barman': 2, 'naqba': 2, 'entangle': 1, '405': 1, 've': 1, 'plummeting': 3, 'counterinsurgency': 2, 'falluja': 1, 'conduit': 1, 'ravix': 2, 'remissaninthe': 1, 'roared': 1, 'formulations': 1, 'differing': 1, '2050': 1, 'polluting': 1, 'mortally': 3, 'makhachkala': 2, 'bitarov': 1, 'bitar': 1, 'adilgerei': 1, 'magomedtagirov': 1, 'xianghe': 1, 'salang': 1, 'avalanches': 3, 'belgians': 1, 'returnees': 2, 'statoil': 1, 'consisting': 3, 'krasnoyarsk': 1, 'assortment': 1, 'firearms': 4, 'subtracted': 1, 'masah': 1, 'mawlawi': 1, 'ulema': 1, 'cambridge': 1, 'incapacitated': 2, 'azocar': 1, 'moviemaking': 2, 'reinforces': 2, 'bollywood': 1, 'kiteswere': 1, 'tarnishing': 2, 'silverman': 1, 'misguided': 3, 'deifies': 1, 'militarist': 1, 'gao': 3, 'shara': 3, 'demonstrably': 1, 'antony': 1, 'jayprakash': 1, 'dal': 1, 'ambareesh': 1, 'rashtriya': 1, 'narain': 1, 'jabel': 1, 'wasting': 2, '20s': 3, 'creutzfeldt': 1, 'bovine': 1, 'spongiform': 1, 'encephalopathy': 1, 'alienation': 1, 'victimize': 1, 'loosely': 1, 'smash': 1, 'delivers': 1, 'dream': 3, 'samho': 1, 'filipinos': 5, 'qater': 1, 'frontal': 1, 'aboutorabi': 1, 'fard': 1, 'pretending': 3, 'seeker': 1, 'joongang': 1, 'esfandiar': 1, 'mashaie': 5, 'arg': 2, 'heater': 2, 'exits': 1, 'fang': 1, 'llam': 1, 'impassioned': 1, 'vandemoortele': 1, 'constituency': 5, 'defies': 1, 'kakdwip': 2, '1004': 1, '101': 5, 'bricks': 6, 'maghazi': 1, 'thun': 1, 'statistical': 1, 'methodology': 2, 'variation': 3, 'makubalo': 1, 'lindy': 1, 'attache': 2, 'olesgun': 1, 'miserable': 1, 'turkana': 1, 'rongai': 1, 'mogotio': 1, 'marigat': 1, 'corresponding': 1, 'hawks': 3, 'mesa': 8, 'hammers': 1, 'unsound': 1, 'sledge': 1, 'structurally': 1, 'seabees': 1, 'exploding': 2, 'shujaat': 1, 'zamir': 1, 'asef': 1, 'shawkat': 1, 'escapees': 2, 'firebase': 1, 'bumpy': 1, 'ripley': 1, 'baskin': 1, 'bypassed': 1, 'resorting': 2, 'inroads': 1, 'wefaq': 1, 'orchids': 1, 'botanic': 1, 'partnered': 1, 'brilliance': 1, 'splash': 1, 'kohlu': 2, 'shahid': 1, 'rivalry': 4, 'bangla': 1, 'durjoy': 1, 'bogra': 1, 'spouse': 1, 'demographer': 1, 'guangzhong': 1, 'mu': 1, 'outlaw': 3, 'fetus': 1, 'passover': 2, 'heartened': 1, 'motivating': 2, 'shies': 1, 'kanan': 1, 'duffy': 1, 'trent': 4, 'balah': 1, 'deir': 2, 'caterpillar': 2, 'swerve': 1, 'aweil': 1, 'hanifa': 1, '733': 1, 'g20': 1, 'azizi': 1, 'jalali': 1, 'benoit': 1, 'maimed': 1, 'waaq': 1, 'ceel': 1, 'vacationers': 2, 'campsites': 1, 'schoolgirls': 1, 'motorbikes': 1, 'bundled': 1, 'iapa': 1, 'entitlement': 1, 'overhauling': 1, 'exceedingly': 1, 'explorers': 3, 'samoan': 1, 'pago': 1, 'togoland': 1, 'facade': 1, 'rpt': 1, 'continually': 1, 'capitalism': 3, 'tile': 1, 'gardener': 2, 'watered': 2, 'prospering': 1, 'tilemaker': 1, 'shine': 1, 'breakfast': 5, 'bolting': 1, 'fork': 1, 'pickle': 1, 'abstraction': 1, 'lens': 1, 'spectacles': 1, 'wharf': 1, 'needless': 1, 'swamp': 2, 'stumped': 1, 'alarming': 2, 'predator': 1, 'chemist': 2, 'coupled': 2, 'brewed': 1, 'adhesive': 1, 'togetherness': 1, 'monosodium': 1, 'glue': 1, 'uhm': 4, 'shimbun': 1, 'valero': 1, 'prejudice': 1, 'tarcisio': 1, 'bertone': 2, 'priestly': 1, 'furor': 1, 'celibacy': 1, 'galo': 1, 'chiriboga': 1, 'rejoined': 3, 'dries': 1, 'buehring': 1, 'gujri': 1, 'mudasir': 2, 'gopal': 1, 'slams': 1, 'tolerating': 1, 'animists': 1, 'heineken': 1, 'pingdingshan': 1, 'notoriously': 2, 'sarin': 2, 'correspondence': 1, 'coded': 1, 'informant': 2, 'deciphering': 1, 'reaffirm': 3, 'endeavouris': 1, 'endeavourundocked': 1, 'spacewalks': 4, 'educate': 1, '26th': 2, 'hell': 1, 'invaders': 2, 'stern': 5, 'wook': 1, 'gainers': 1, 'performace': 1, 'concacaf': 1, 'frisked': 2, 'slots': 4, 'hamarjajab': 1, 'schuessel': 3, 'bridged': 1, 'khatibi': 1, 'communicate': 2, 'conscience': 2, 'flourish': 1, 'curbs': 2, 'abilities': 1, 'literacy': 2, 'rangeen': 1, 'refueling': 2, 'milomir': 1, 'stakic': 2, 'sample': 3, 'droppings': 1, 'h7n3': 1, 'thyroid': 1, '47s': 1, 'warranted': 2, 'slayings': 1, 'kharazi': 2, 'zvyagintsev': 1, 'parulski': 1, 'tusk': 6, 'smolensk': 1, 'djinnit': 1, 'civility': 2, 'unacceptably': 1, 'reconstructive': 2, 'sliced': 1, 'grossman': 1, 'aisha': 3, 'hacked': 1, 'canons': 1, 'glad': 1, 'assailed': 3, 'fabric': 1, 'unravel': 1, 'betting': 4, 'calculators': 1, 'bookmaking': 1, 'kalmykia': 1, 'khurul': 1, 'expectation': 1, 'ipsos': 2, 'midterm': 2, 'exams': 5, 'answers': 2, 'haidallah': 1, 'khouna': 1, 'maaouiya': 1, 'taya': 3, 'advertisement': 1, 'expansive': 1, 'communicated': 1, 'fares': 1, 'firmest': 1, 'mediating': 1, 'pepper': 1, 'truncheons': 1, 'beyazit': 1, 'activated': 2, 'bluefin': 4, 'wwf': 2, 'sushi': 1, 'leigh': 3, 'liang': 3, 'sever': 2, 'eighties': 2, 'jinling': 1, 'identifies': 3, 'shadi': 3, 'spiraled': 1, 'uji': 1, 'hagino': 1, 'unaccounted': 3, 'townspeople': 2, 'deserter': 2, 'shfaram': 1, 'renen': 1, 'schorr': 1, 'shooter': 1, 'kach': 1, 'belhadj': 1, 'sibneft': 2, 'inoculate': 1, 'measles': 3, 'nomads': 2, 'ziyad': 1, 'karbouli': 4, 'desouki': 1, 'vaccinators': 1, 'macheke': 1, 'chigwedere': 1, 'aeneas': 1, 'comatose': 2, 'unconscious': 2, 'lci': 1, 'initiating': 2, 'react': 1, 'relate': 1, 'incur': 1, 'directives': 1, 'jameson': 4, 'grill': 5, 'maybe': 2, 'raiser': 1, 'picnic': 1, 'creek': 1, 'volleyball': 1, 'kickball': 1, 'archery': 2, 'crafts': 1, 'roast': 1, 'fixings': 1, 'raffle': 3, 'drinks': 4, 'dreams': 2, 'enclosed': 2, 'aol': 3, 'bray': 2, 'jcfundrzr': 1, '241': 1, '2661': 1, 'colonizers': 1, 'distinct': 3, 'linguistic': 2, '1906': 1, 'condominium': 1, '963': 1, '1839': 2, 'knife': 2, 'benelux': 1, 'bolshevik': 2, 'agrochemicals': 1, 'aral': 1, 'stagnation': 1, 'curtailment': 1, '1935': 1, 'ferdinand': 2, 'edsa': 2, 'corazon': 2, 'estrada': 3, 'insurgencies': 1, 'exultingly': 1, 'conqueror': 1, 'flapped': 1, 'pounced': 1, 'undisputed': 1, 'comission': 1, 'sportsmen': 1, 'coho': 1, 'kowal': 2, 'walleye': 1, 'reproduced': 1, 'kowalski': 1, 'muskie': 1, 'laotian': 1, 'franken': 2, 'harkin': 1, 'dispose': 1, 'waiver': 1, 'suicides': 1, 'malpractice': 3, 'premiums': 3, 'standby': 6, 'baiji': 7, 'numaniya': 1, 'uday': 1, 'domed': 1, 'cykla': 1, 'assailant': 1, 'nuaimi': 1, 'fathi': 1, 'void': 1, 'firmer': 1, 'fiery': 5, 'jufeng': 1, 'jiang': 1, 'skull': 6, 'bildnewspaper': 1, 'josef': 4, 'ornament': 1, 'fastening': 1, 'exposing': 3, 'align': 1, 'propped': 1, 'robustly': 1, 'getters': 1, 'mizarkhel': 1, 'layoffs': 2, '747': 5, '737s': 1, 'rashad': 1, '356': 1, 'defaulting': 1, 'tareen': 3, 'disbursements': 1, 'pontificate': 1, 'inserted': 3, 'delegated': 1, 'urbi': 1, 'et': 2, 'orbi': 1, 'supremacist': 1, 'mahlangu': 1, "terre'blanche": 2, 'afrikaner': 1, 'bludgeoned': 1, 'stemmed': 2, 'seal': 10, 'fenced': 1, 'abbott': 3, '259': 1, 'labs': 1, 'kaletra': 1, 'generic': 1, 'overpricing': 1, 'muted': 1, 'malaysians': 1, 'vacations': 1, 'caucasian': 1, 'borcz': 1, 'teresa': 1, 'harkat': 1, 'zihad': 1, 'communal': 2, 'mailed': 2, 'ranbir': 1, 'dayers': 1, 'poroshenko': 2, 'acrimonious': 1, 'chrysostomos': 1, 'heretic': 1, 'poachers': 1, 'skins': 1, 'imperialist': 1, 'crucified': 1, 'relaxing': 2, 'bakri': 5, 'overflowed': 4, 'neiva': 1, 'kilimo': 2, 'jebii': 1, '1582': 1, 'xii': 3, 'noncompliant': 1, 'yunlin': 1, 'lebedev': 4, 'platon': 3, 'mariane': 1, 'habib': 2, 'odierno': 1, 'prout': 1, 'bobbie': 1, 'zctu': 2, 'vihemina': 1, 'wrought': 1, 'czar': 2, 'singling': 1, 'seige': 1, 'chibebe': 1, 'okala': 2, 'tobie': 1, 'brownback': 2, 'hicks': 9, 'cosatu': 5, 'homosexual': 1, 'massouda': 2, 'enthusiastic': 2, 'alexeyev': 3, 'discriminated': 1, 'insights': 1, 'kappes': 3, 'sulik': 1, 'rebut': 1, 'broncos': 1, 'touchdown': 6, 'rod': 2, 'yards': 1, 'undrafted': 1, 'quarterback': 3, 'plummer': 1, 'kicker': 1, 'elam': 1, 'jake': 1, 'samie': 1, 'receiver': 1, 'corpse': 1, 'kits': 1, 'repaired': 4, 'reopening': 6, 'kaman': 2, 'hasten': 2, 'relaunch': 2, 'monk': 6, 'yury': 2, 'luzhkov': 1, 'satanic': 1, 'ashin': 1, 'htay': 1, 'thu': 3, 'gambira': 2, 'mandalay': 2, 'hkamti': 1, 'sagaing': 1, 'willian': 1, 'orchestrating': 2, 'sadiq': 1, 'defy': 4, 'bandundu': 2, 'mende': 3, 'merchandise': 2, 'navigate': 2, 'nickelodeon': 2, 'slimy': 1, 'dispenser': 1, 'goo': 1, 'messiest': 1, 'stiller': 1, 'sandler': 1, 'wannabe': 1, 'signifies': 2, 'youthful': 1, 'lighthearted': 1, 'imprisioned': 1, 'trivial': 2, 'meltdown': 2, '1745': 1, 'cozumel': 3, 'zu': 2, 'theodor': 2, 'guttenberg': 3, 'schneiderhan': 3, 'xinqian': 1, 'ou': 2, 'adjusted': 1, 'asadollah': 1, 'sabouri': 2, 'obligates': 1, 'disposition': 2, 'leftwing': 1, 'relates': 1, 'buraydah': 1, 'cheapest': 3, 'tata': 8, 'singur': 1, 'uttarakhand': 1, 'chapfika': 1, 'zvakwana': 1, 'tuvalu': 7, 'atolls': 3, 'seamen': 2, 'ttf': 2, 'nz': 2, 'vulnerability': 2, 'remoteness': 2, 'lobster': 2, 'anguillan': 1, 'intercommunal': 1, 'trnc': 1, 'impetus': 1, 'reuniting': 2, 'acquis': 1, 'forbade': 1, 'altered': 3, 'flute': 2, 'tunes': 1, 'projecting': 1, 'haul': 3, 'perverse': 1, 'creatures': 4, 'merrily': 1, 'leaping': 1, 'signboard': 2, 'goblet': 1, 'dashed': 2, 'unwittingly': 2, 'jarring': 1, 'terribly': 2, 'zeal': 1, 'naturedly': 1, 'benefactor': 1, 'gnawed': 1, 'playoffs': 4, 'orioles': 1, 'baltimore': 1, 'outfield': 1, 'reindeer': 2, 'deserved': 2, 'rudolph': 2, 'glowing': 1, 'proboscis': 1, 'pinna': 1, 'cylinder': 2, 'origins': 3, 'jumpers': 2, 'stefano': 1, 'beltrame': 2, 'chiapolino': 2, 'predazzo': 1, 'spleen': 1, 'belluno': 1, 'cavalese': 1, 'concussion': 2, 'fractures': 2, 'roofs': 4, 'montas': 2, 'someday': 1, 'zwelinzima': 1, 'skewed': 2, 'vavi': 1, 'uz': 1, 'mushahid': 1, 'squadron': 1, 'brightest': 1, 'shawn': 1, 'gymnast': 3, 'enming': 1, 'aspiring': 1, 'olympian': 1, 'sagging': 1, 'backgrounds': 3, 'gdps': 1, 'arawak': 2, '1672': 1, 'inevitably': 1, 'slows': 2, 'simmers': 1, 'policharki': 1, 'khaisor': 1, 'siphiwe': 2, 'safa': 1, 'sedibe': 2, 'memorable': 2, 'symbolism': 1, 'heighten': 1, 'enviable': 1, 'curator': 3, 'xinmiao': 1, '1644': 1, 'qing': 1, 'spanning': 2, 'zaken': 2, 'shula': 3, 'andijon': 4, 'cascade': 2, 'adwar': 1, 'rabiyaa': 1, 'faisalabad': 2, 'hasib': 1, 'shehzad': 1, 'sidique': 2, 'tanweer': 1, 'sidon': 2, 'slid': 1, 'shehade': 2, 'housekeeper': 2, 'uncovering': 1, 'taser': 1, 'giuliani': 1, 'patriots': 2, 'lubanga': 7, 'hema': 1, 'utmost': 2, 'acutely': 1, 'brammertz': 2, 'serge': 1, 'laughing': 3, 'ilna': 1, 'sajedinia': 1, 'karoubi': 1, 'rightful': 2, 'geagea': 2, 'liquids': 2, 'believing': 3, 'liner': 1, "ma'ariv": 1, 'nazif': 2, 'heidelberg': 1, 'intestine': 1, 'mullen': 5, 'hoekstra': 1, 'feinstein': 1, 'chambliss': 3, 'diane': 1, 'saxby': 2, 'waking': 2, 'comfortably': 2, 'dozing': 1, 'videolink': 1, 'bailey': 1, 'neil': 1, 'abercrombie': 2, 'rohrabacher': 3, 'silenced': 2, 'horrifying': 1, 'owl': 4, 'acorn': 1, 'sprout': 2, 'counseled': 1, 'heroism': 1, 'ignace': 1, 'schops': 1, "voa's": 1, 'ivanic': 2, 'mistletoe': 1, 'acorns': 1, 'irremediable': 1, 'insure': 1, 'mikerevic': 1, 'dismissals': 1, 'pluck': 2, 'boded': 1, 'flax': 1, 'sadeh': 1, "pe'at": 1, 'lastly': 1, 'darts': 1, 'dormitories': 1, 'disobey': 1, 'credence': 1, '321': 2, 'stubb': 1, 'wisest': 1, 'odde': 1, 'leaflet': 1, 'solitude': 1, 'mixing': 1, 'nagano': 2, 'ayyub': 1, 'khakrez': 1, 'touts': 1, 'haleem': 1, 'traded': 5, '121': 3, '329': 1, '913': 1, '781': 1, 'abdulaziz': 2, 'intending': 2, 'colts': 2, 'lions': 3, 'touchdowns': 3, 'cowboys': 1, 'julius': 1, 'phillip': 2, 'madhya': 1, 'buzzard': 1, 'hotline': 2, 'outstrip': 1, '9942': 1, '205': 1, 'vaeidi': 1, 'soltanieh': 1, 'provincal': 1, 'zinjibar': 2, 'crazy': 1, 'pest': 1, 'fouling': 1, 'insect': 1, 'extracting': 1, 'cesar': 1, 'abderrahaman': 1, 'infidel': 1, 'noaman': 2, 'gomaa': 2, 'swear': 2, 'confiscate': 1, 'affiliates': 5, 'aqaba': 2, 'ashland': 1, 'scam': 4, 'gyeong': 1, 'nuptials': 1, 'bryant': 1, 'sita': 1, 'di': 4, 'rubina': 2, 'miliatry': 1, 'aspiazu': 2, 'garikoitz': 1, 'pyrenees': 1, 'tablet': 3, 'wireless': 1, 'txeroki': 1, 'myricks': 1, 'demotion': 1, 'samsun': 1, 'seaside': 3, 'dimensional': 2, 'vertigo': 1, '3d': 3, 'heralds': 1, 'modell': 2, 'filmmaking': 1, 'physiology': 1, 'replicating': 1, 'nausea': 2, '715': 1, '1607': 1, 'onward': 1, '1278': 1, "d'urgell": 1, 'seu': 1, 'andorrans': 1, 'feudal': 2, 'titular': 1, 'benefitted': 1, 'hellenic': 1, 'inequities': 1, 'ohrid': 2, 'fronts': 3, '1609': 2, 'keeling': 1, '1820s': 2, 'clunie': 1, 'cocos': 1, '1841': 1, 'meshes': 1, 'skillful': 1, 'exacted': 1, 'promissory': 1, '747s': 1, 'raft': 2, 'locator': 1, 'chopper': 1, 'renouncing': 1, 'unknowingly': 2, 'crisp': 1, 'overpaid': 1, 'dilemma': 1, 'athar': 1, 'phukphasuk': 1, 'chalit': 1, 'serirangsan': 1, 'thirapat': 1, 'paulino': 1, 'matip': 1, 'splm': 2, 'bossa': 2, 'reinhardt': 2, 'django': 2, 'aneurysm': 2, 'ventura': 1, 'dans': 1, 'mon': 1, 'jobim': 1, 'acronym': 1, 'expending': 1, 'deviated': 1, 'cerberus': 2, 'fragility': 1, 'waive': 3, 'seminars': 1, 'enrollment': 1, 'confuse': 1, 'straightened': 2, 'nissan': 6, 'mckiernan': 1, 'unites': 1, 'solitary': 4, 'buhriz': 2, 'taza': 1, 'dutton': 1, 'buchan': 1, 'gasses': 2, 'unscheduled': 2, 'curbed': 1, 'shrines': 3, 'babil': 2, 'victors': 1, 'qurans': 2, 'peacekeeers': 1, 'mcadams': 2, 'demented': 1, 'storming': 3, 'invoke': 1, 'emission': 1, 'brokering': 2, 'fledged': 1, 'kien': 1, 'premiering': 1, 'dramatizes': 1, 'integrating': 1, 'dishonor': 1, 'indifferent': 1, 'comeback': 4, 'pararajasingham': 1, 'undertake': 1, 'yuli': 1, 'tamir': 2, 'soleil': 2, 'ronit': 1, 'tirosh': 1, 'pieced': 1, 'reconstructed': 1, 'presidencies': 1, 'persson': 2, 'freivalds': 2, 'goran': 1, 'laila': 2, 'tempered': 1, 'sharpest': 2, 'gag': 1, 'colby': 1, 'cerveny': 1, 'vokey': 1, 'mcnuggets': 1, 'abdurrahman': 1, 'dtp': 2, 'yalcinkaya': 1, 'fda': 5, 'salmonella': 2, 'budny': 1, 'unger': 1, 'marxists': 1, 'rash': 2, 'miliband': 6, 'jacqui': 1, 'headless': 1, 'jamaat': 2, 'fitna': 1, 'quotations': 1, 'wilders': 1, 'geert': 1, 'summoning': 2, 'stein': 4, 'bloom': 1, 'narthiwat': 1, 'kolok': 1, 'sungai': 1, 'carrasquero': 1, 'kumgang': 1, 'reunions': 1, 'defected': 12, 'mclaughlin': 1, 'disrespectfully': 1, 'tabulation': 1, 'moines': 1, 'des': 2, 'intuitive': 1, 'talker': 1, 'dismal': 1, 'saudis': 3, 'shuttered': 1, 'humphreys': 1, 'kozloduy': 1, 'reinsurer': 1, 'zurich': 3, 'reinsurers': 1, 'jetliner': 1, 'cargolux': 1, 'quieter': 1, 'utilize': 1, '380': 3, 'superjumbo': 3, 'alkhanov': 6, 'alu': 3, 'danilovich': 2, 'justly': 1, 'dahoud': 1, 'militaries': 1, 'withstood': 1, 'idled': 2, 'dos': 2, 'mpla': 2, 'unita': 2, 'tristan': 1, 'cunha': 1, 'helena': 1, 'agriculturally': 1, 'tasalouti': 1, 'expectancy': 1, 'vertical': 1, 'clusters': 1, 'horizontal': 1, 'mitigate': 1, 'outreach': 1, '1667': 1, 'nominally': 1, '1650': 1, 'saeedlou': 1, 'precipice': 1, 'endeavoring': 1, 'willful': 1, 'funny': 1, 'windy': 1, 'wig': 2, 'mane': 1, 'sisters': 1, 'blandly': 1, 'charming': 1, 'billiard': 1, 'bald': 2, 'gust': 1, 'foolish': 4, 'glistening': 1, 'embarrassed': 1, 'woodchopper': 1, 'besought': 1, 'mahsouli': 1, 'sadeq': 3, 'salivated': 1, 'thoughtless': 1, 'deity': 1, 'thorn': 4, 'amphitheatre': 1, 'devour': 1, 'claimant': 1, 'honourably': 1, 'abstained': 2, 'catcher': 1, 'yogi': 2, 'stocky': 1, 'horrified': 3, 'berra': 1, 'miraculous': 1, '1850': 2, 'bathtub': 2, 'invented': 2, '1875': 2, 'bothered': 1, 'miscreants': 1, 'toad': 1, 'evicting': 1, 'query': 1, 'reply': 4, "ha'aretz": 1, 'utilization': 1, 'interaction': 1, 'unsolicited': 1, 'spammers': 2, 'kramer': 3, 'server': 1, 'disabling': 1, 'upgrading': 2, 'faraa': 1, 'yamoun': 1, 'mohammadi': 2, 'parental': 1, 'jeffery': 2, 'jeffrey': 4, 'hamstrung': 1, 'orion': 2, 'workhorse': 1, 'subhadra': 1, 'dhanush': 1, 'moiseyev': 4, 'balletic': 1, 'dances': 2, 'acrobatic': 1, 'ghazl': 1, 'souq': 1, 'inoculations': 1, 'surfaces': 2, 'rye': 1, 'bluegrass': 1, 'turf': 2, 'billed': 1, 'mown': 1, 'fertilized': 1, 'successors': 2, 'flares': 1, 'abizaid': 3, 'reyna': 5, 'ligament': 2, 'sprained': 1, 'saddamists': 1, 'gianni': 1, 'magazzeni': 2, 'piles': 1, 'echoupal': 4, 'expensively': 1, 'kiosks': 1, 'rafidain': 1, 'finsbury': 1, 'stirring': 4, 'sermons': 2, 'notoriety': 1, 'earl': 1, 'christa': 2, 'mcauliffe': 2, 'liftoff': 2, 'booster': 3, 'columbiadisintegred': 1, 'disciplines': 1, 'showings': 1, 'rowing': 3, 'cycling': 2, 'steele': 5, 'drugged': 1, 'adolfo': 3, 'scilingo': 2, 'recanted': 2, 'embracing': 2, 'cult': 1, 'headlining': 1, 'differed': 1, 'erian': 1, 'acceptable': 5, 'complains': 1, 'poster': 1, 'ezzat': 1, 'itv1': 1, 'salah': 1, 'suleimaniyah': 1, 'reservist': 1, 'genitals': 1, 'kerkorian': 1, 'ghosn': 1, 'contry': 1, 'patzi': 2, 'transporation': 1, '263': 1, '299': 1, 'diagnostic': 1, 'irrelevant': 1, 'utterly': 1, 'interahamwe': 2, 'dean': 4, 'orjiako': 1, 'hiva': 1, 'abdolvahed': 1, 'botimar': 1, 'hassanpour': 1, 'asou': 1, 'zhou': 2, 'guocong': 1, 'grossly': 1, 'rings': 2, 'jamuna': 1, 'witty': 5, '051655093': 1, 'hilton': 2, 'camille': 1, 'karolina': 1, 'sprem': 1, '580': 2, 'harkleroad': 1, 'smyr': 3, 'petrova': 2, 'mujahadeen': 1, 'adjourns': 1, 'gaudio': 1, 'gaston': 1, 'kanaan': 1, 'postcard': 2, 'qaumi': 2, 'parlemannews': 1, 'crackdowns': 2, 'alyaty': 1, 'melange': 1, 'edison': 1, 'confidentiality': 3, 'baptiste': 2, 'natama': 1, 'unavoidable': 1, 'wannian': 1, 'whereas': 1, 'hanyuan': 1, 'zhengyu': 1, 'dung': 1, 'halo': 1, 'skulls': 3, 'trenches': 1, 'lining': 1, 'specialize': 1, 'cumple': 1, 'si': 1, 'validated': 1, 'rumbo': 2, 'propio': 2, 'mvr': 1, 'depressions': 1, '1851': 1, 'courier': 2, 'rustlers': 1, 'samburu': 2, 'pokot': 1, 'letimalo': 1, 'combing': 1, 'bend': 1, 'oceanic': 3, 'wilderness': 1, 'archipelagoes': 1, 'boasts': 2, 'aquarium': 1, 'turtles': 3, 'buckovski': 4, 'vlado': 1, 'majtenyi': 1, 'cathy': 1, 'theatre': 1, 'uwezo': 1, '1870s': 2, 'equatoria': 3, 'colonize': 1, 'mahdist': 1, 'facilitated': 3, 'distilling': 1, 'rum': 1, 'croix': 1, 'mindaugas': 1, '1236': 2, '1386': 1, '1569': 1, 'abortive': 1, 'variations': 1, 'banana': 3, 'gonsalves': 1, 'flea': 4, 'dare': 2, 'limbs': 1, 'notarized': 1, 'hint': 1, 'pinions': 1, 'pugiliste': 1, 'biceps': 1, 'ladders': 1, 'stepstools': 1, 'demontrators': 1, 'unregistered': 2, 'qidwa': 1, 'shaath': 1, 'pall': 1, 'sugars': 1, 'clinical': 4, 'diet': 1, 'shelley': 1, 'schlender': 1, 'staggered': 2, 'hammond': 1, 'obamas': 1, 'tayeb': 1, 'musbah': 1, 'abstentions': 2, 'pilgrimages': 1, 'inflicting': 1, 'khar': 1, 'santander': 1, 'hacari': 1, 'honked': 1, 'circled': 1, 'unleaded': 1, 'spite': 2, 'kalameh': 1, 'sabz': 1, 'refiner': 1, 'sk': 2, 'dahuk': 1, 'bazian': 1, 'uloum': 3, 'impulses': 1, 'injustices': 1, 'disloyalty': 1, 'adulthood': 2, 'olds': 1, 'juristictions': 1, 'camouflage': 2, 'kimonos': 1, 'ud': 1, 'gabla': 1, 'animosity': 1, 'concocted': 1, 'berman': 3, 'lowey': 2, 'appropriations': 1, 'jalalzadeh': 1, 'guo': 1, 'quiang': 1, 'cai': 1, 'gunpowder': 1, 'firework': 1, 'designing': 1, 'guggenheim': 1, 'yiru': 1, '7000': 1, 'aka': 3, 'tanoh': 1, 'bouna': 1, 'vezzaz': 1, 'abderahmane': 1, 'tintane': 1, 'acapulco': 3, 'otay': 1, 'yunesi': 2, 'interrogate': 1, 'offerings': 1, 'banged': 1, 'moken': 1, 'loftis': 1, 'schilling': 1, 'rood': 3, 'usain': 1, 'asafa': 1, 'jamaicans': 2, 'bolt': 4, 'haniyah': 1, 'farhat': 1, 'cautioning': 1, 'rematch': 1, 'cabins': 1, 'gels': 1, '100th': 2, 'bermet': 2, 'akayeva': 3, 'aidar': 1, 'reactions': 3, 'sequence': 1, 'tyson': 1, 'sonora': 1, 'anxiously': 1, 'steam': 2, 'approves': 3, 'shenzhou': 4, 'liwei': 1, 'earthen': 1, 'naser': 1, 'raba': 1, 'borderline': 1, 'spearheading': 1, 'genoino': 3, 'genro': 1, 'tarso': 1, 'treasurer': 1, 'dirceu': 1, 'reshuffling': 1, 'nestle': 3, 'trickle': 2, 'worshipers': 4, 'timer': 1, 'coexist': 2, 'props': 1, 'gaudy': 1, 'muqudadiyah': 1, 'silo': 1, 'kicks': 1, 'relais': 1, 'bruhin': 3, 'schoch': 3, 'prommegger': 1, 'heinz': 1, 'inniger': 1, 'jaquet': 1, '460': 1, 'kohli': 1, 'daniela': 1, 'meuli': 1, 'pomagalski': 1, 'blanc': 1, 'isabelle': 1, 'kamel': 2, 'ibb': 1, 'baptist': 3, 'jibla': 1, 'gollnisch': 2, 'bruno': 1, 'disputing': 2, 'nullify': 2, 'acholi': 1, 'bhatiasevi': 1, 'aphaluck': 1, '292': 1, 'saliva': 1, 'vomit': 1, 'shalabi': 1, 'dhess': 1, 'dagma': 1, 'djamel': 1, 'discotheques': 2, 'dusseldorf': 1, 'abdalla': 1, 'trademark': 2, 'trademarks': 1, 'vuitton': 2, 'counterfeiters': 1, 'alois': 1, 'soldaten': 1, 'cavernous': 1, 'armory': 1, 'bernd': 2, 'lekki': 1, 'decommission': 1, 'resent': 1, 'acehnese': 1, 'nogaideli': 2, 'burdzhanadze': 1, '42nd': 1, 'murphy': 2, 'translates': 1, 'shaleyste': 1, 'cart': 1, 'reconcilation': 1, 'berkin': 1, 'alimov': 1, 'chaika': 1, 'emigre': 1, 'pavel': 1, 'ryaguzov': 1, 'anglican': 2, 'rowan': 2, 'canterbury': 3, 'mostar': 2, 'krzyzewski': 2, 'grocery': 2, 'annualized': 1, 'coalitions': 2, 'jalbire': 1, 'retires': 1, '265': 2, 'shearer': 1, 'tremor': 2, 'pius': 4, 'archive': 3, 'middleman': 1, 'initiators': 1, 'lupolianski': 1, 'marshal': 1, 'slavonia': 1, 'downturns': 1, 'interlarded': 1, 'vagaries': 1, 'statutory': 1, 'gateways': 1, 'ltte': 5, 'alleviated': 1, 'vulnerabilities': 1, 'eater': 1, 'halter': 2, 'courted': 2, 'hairs': 1, 'ashamed': 1, 'admirer': 1, 'zealous': 1, 'dazzling': 1, 'boisterous': 1, 'tilt': 1, 'kalachen': 1, 'rapporteur': 1, '557': 1, '439': 2, '522': 1, 'enroute': 1, 'whatsoever': 1, 'kaohsiung': 1, 'xiamen': 1, 'lambasted': 1, 'unsavory': 1, 'filthy': 1, 'dunking': 1, 'workable': 1, 'jocic': 1, 'cimpl': 2, 'flashing': 3, 'floodwater': 1, 'getty': 2, 'marble': 1, 'bc': 2, 'sculpted': 1, 'tombstone': 3, 'voulgarakis': 1, 'popovkin': 1, 'fayfi': 2, 'jabir': 1, 'jubran': 1, 'barzani': 11, 'veiled': 2, 'manar': 3, 'iqbal': 2, 'machinists': 1, 'gene': 3, 'glucose': 1, 'destroys': 2, 'insulin': 1, 'snipers': 1, 'carrizales': 1, 'atal': 1, 'bihari': 1, 'vajpayee': 4, 'bhartiya': 1, 'bjp': 2, 'prosecutorial': 1, 'earle': 1, 'pornographic': 1, 'passwords': 1, 'proliferators': 1, 'axum': 5, 'obelisk': 7, 'teshome': 1, 'toga': 1, 'structur': 1, 'granite': 2, 'unsaturated': 1, 'clog': 1, 'applauding': 1, 'hubs': 1, 'artistic': 1, 'evangelina': 1, 'sepehri': 1, 'sahar': 1, 'elizondo': 1, 'phantom': 1, '915': 1, 'konarak': 1, 'prediction': 2, 'spaces': 1, 'furnished': 1, 'wmo': 1, 'factored': 1, 'salaheddin': 1, 'talib': 1, 'nader': 1, 'shaban': 1, 'seaport': 1, '1750': 1, 'slovaks': 6, 'lithuanians': 1, 'conducive': 3, 'chipaque': 1, 'policymaking': 1, 'yakubov': 3, 'sabirjon': 1, 'derivative': 1, 'mbalax': 1, "n'dour": 1, 'youssou': 1, 'kwaito': 1, 'ovation': 2, 'stiffly': 1, 'fracturing': 1, 'stumbled': 2, 'inequalities': 1, 'batna': 1, 'likened': 2, 'roulette': 1, 'irresponsibly': 1, 'sittwe': 1, 'birdflu': 1, 'sparrowhawk': 1, 'jubilation': 1, 'unfunded': 1, 'specializing': 1, 'warriors': 2, "qu'ran": 1, "jama'at": 1, 'rusafa': 1, 'nahda': 1, 'decisively': 2, 'latifiyah': 1, 'intercede': 1, 'aps': 1, '151': 1, 'austere': 1, 'pachyderm': 1, 'foments': 1, 'favoritism': 1, 'perception': 1, 'crimp': 1, 'pastrana': 5, 'arbour': 1, 'indiscriminate': 2, 'nomadism': 1, 'pastoral': 1, 'sedentary': 1, 'quarreled': 1, 'literate': 1, 'indebtedness': 1, 'saa': 1, 'duhalde': 1, 'peso': 3, 'bottomed': 1, 'audacious': 1, 'understating': 1, 'exacerbating': 1, 'dominica': 1, 'ecotourism': 2, 'geothermal': 1, 'lightening': 1, 'loads': 2, 'luxuries': 1, 'overburdened': 1, 'traveler': 4, 'shining': 1, 'intensely': 1, 'afforded': 3, 'galloped': 2, 'quarreling': 1, 'hopped': 1, 'antagonists': 1, 'groaned': 3, 'comb': 1, 'emroz': 1, 'alamzai': 2, 'fahim': 1, 'ajmal': 2, 'kohdamani': 2, 'didadgah': 1, 'rodong': 1, 'wenesday': 1, 'organizational': 1, 'faulted': 1, 'responders': 1, 'toilets': 2, 'gijira': 1, 'fdic': 1, 'tartous': 1, 'rainstorm': 1, 'nirvana': 2, 'cobain': 4, 'bean': 1, 'wed': 2, 'flannel': 1, 'smells': 1, 'sweaters': 1, 'patat': 1, 'jabor': 1, 'dalian': 1, 'meishan': 1, 'neng': 1, 'tian': 1, 'vent': 1, 'reformer': 1, 'lukewarm': 1, 'realistic': 2, 'puebla': 2, 'mosleshi': 1, '637': 1, 'texmelucan': 1, 'rehn': 3, 'herceptin': 2, 'drugmaker': 2, 'postpones': 1, 'ayar': 1, 'lazio': 1, 'fiorentina': 1, 'manipulate': 3, 'handpicking': 1, 'serie': 1, 'relegation': 1, 'demotions': 1, '164': 1, 'herdsmen': 1, 'fulani': 1, 'martyrdom': 4, 'hamm': 6, 'intelligent': 2, 'creationism': 1, 'gymnastics': 1, 'healed': 1, 'ternate': 1, 'molucca': 1, 'vivanews': 1, 'pm': 2, 'radius': 2, 'rotator': 1, 'cuff': 1, 'keywords': 1, 'chat': 1, 'alluding': 2, 'nsa': 5, 'accessed': 1, 'storing': 1, 'wiretap': 1, 'pbsnewshour': 1, 'alternates': 1, 'mighty': 1, 'najjar': 1, 'badawi': 2, 'demonizing': 3, 'meza': 1, 'horobets': 1, 'oleksander': 1, 'despicable': 1, 'wallet': 1, 'biographical': 1, 'rfid': 1, 'bezsmertniy': 1, 'terrence': 1, 'craybas': 4, 'morariu': 1, 'corina': 1, 'mashona': 1, 'obliged': 2, 'qutbi': 1, 'dome': 5, 'tahhar': 2, 'toluca': 1, 'pietersen': 2, 'alistair': 1, '367': 1, '323': 1, 'isturiz': 1, 'aristolbulo': 1, 'timergarah': 1, 'sibbi': 1, 'streamlines': 1, 'siphon': 1, 'pullouts': 1, 'rouen': 1, 'haze': 3, 'islamophobia': 2, 'manifestations': 1, 'xenophobia': 2, 'disproportionally': 1, 'overrepresented': 1, 'pekanbara': 1, 'underdocumented': 1, 'islamophobic': 1, 'underreported': 1, 'hexaflouride': 1, 'tackling': 1, 'tindouf': 1, 'lugar': 3, 'yudhyono': 1, 'peipah': 2, 'lekima': 1, 'guanglie': 1, 'mesopotamia': 1, 'abnormalities': 1, 'papadopoulos': 4, 'tassos': 2, 'haves': 1, 'nots': 1, 'satisfy': 3, 'ashes': 2, 'keith': 1, 'snorted': 1, 'ingesting': 1, 'nme': 2, 'cremated': 1, 'dad': 3, 'cared': 1, 'bert': 1, 'luck': 7, 'famously': 1, 'petitions': 1, 'edmund': 1, 'dissenting': 1, 'vacated': 1, 'strikingly': 1, 'sews': 1, 'devra': 1, 'robitaille': 1, 'washingtonians': 1, 'fliers': 1, 'designs': 5, 'mousa': 1, "'80s": 1, 'irrelevent': 1, 'valuables': 1, 'confiscation': 2, 'bengali': 4, 'khagrachhari': 2, 'statments': 1, 'baghaichhari': 1, 'karradah': 2, 'gravesite': 1, 'zelenovic': 2, 'mansiisk': 1, 'khanty': 1, 'padded': 1, 'burdensome': 1, 'psl': 1, 'po': 1, 'straddling': 1, 'overexploitation': 1, 'deepwater': 1, '110474537': 1, 'kjetil': 1, 'aamodt': 1, 'concessionary': 1, 'globally': 2, 'ladder': 1, 'deepened': 1, 'broadened': 3, 'parity': 1, 'aggravating': 2, '110625': 1, 'maaouya': 1, 'cheikh': 2, 'sidi': 2, 'abdallahi': 2, "qa'ida": 1, 'afro': 2, 'moor': 1, 'mauritanians': 1, 'berber': 1, 'aqim': 1, 'combi': 1, 'jar': 1, 'smeared': 1, 'suffocated': 1, 'expiring': 1, 'nurtures': 1, 'hates': 1, 'fondles': 1, 'neglects': 1, 'nurtured': 1, 'smothered': 1, 'caressed': 1, 'banjo': 1, 'halifax': 2, 'passaro': 7, 'plotters': 1, 'simulating': 2, 'goldschmidt': 2, 'alishar': 1, 'rizgar': 2, 'arsala': 3, 'mirajuddin': 1, 'giro': 1, 'melt': 4, 'pole': 4, 'sheet': 3, 'rignot': 1, 'glaciers': 3, 'christi': 3, 'corpus': 3, 'kenedy': 1, 'neal': 1, 'redraw': 3, 'unmonitored': 1, 'josslyn': 1, 'aberle': 1, 'incarceration': 1, 'unpaid': 2, 'readied': 1, 'homayun': 1, 'interpret': 2, 'combining': 3, 'khamail': 1, 'reassess': 1, 'playoff': 1, 'wnba': 1, 'hawi': 1, 'parma': 4, 'peeling': 1, 'khao': 1, 'agitated': 3, 'rides': 1, 'lak': 1, 'trumpeting': 1, 'trunks': 1, 'calamities': 1, 'dodger': 1, 'perishable': 1, 'millionaire': 2, 'hopkins': 2, 'walikale': 3, 'alison': 1, 'forges': 1, 'karate': 2, 'enzo': 2, 'eddie': 2, 'sportscar': 1, 'irwindale': 1, 'speedway': 1, 'redline': 2, 'deuce': 1, 'bigalow': 1, 'sadek': 1, 'exotic': 3, 'enzos': 1, 'busch': 4, 'bottler': 1, 'anheuser': 4, 'brewing': 1, 'budweiser': 2, 'immense': 2, 'brewer': 1, 'caldera': 4, 'coltan': 1, 'cassiterite': 1, 'diaoyu': 1, 'unguarded': 1, 'perimeter': 1, 'leftover': 1, 'maaleh': 3, 'nullified': 3, 'retroactively': 1, 'shifts': 2, 'hib': 1, 'shuffle': 1, '541': 1, 'proportions': 1, 'dharmendra': 1, 'rajaratnam': 1, 'placards': 2, 'radak': 1, 'sasa': 1, 'ovcara': 1, 'mukakibibi': 2, 'saidati': 1, 'umurabyo': 2, 'publishers': 1, 'hatf': 2, 'disobedience': 1, 'uwimana': 1, 'agnes': 1, '462': 1, '224': 3, '745': 1, 'arenas': 1, 'uladi': 1, 'jusoh': 1, 'mazlan': 1, 'resonated': 1, 'deepens': 1, 'asb': 2, 'srebotnik': 5, 'katarina': 1, 'shinboue': 1, 'asagoe': 2, 'lubiani': 1, 'leanne': 1, '87th': 1, 'a380': 1, 'qantas': 4, 'a380s': 2, 'midair': 1, 'turbines': 1, 'rolls': 1, 'royce': 1, 'volleys': 1, 'bani': 1, 'navarre': 1, 'corpsman': 1, 'kor': 1, 'affirmation': 1, 'yankham': 1, 'kengtung': 1, 'silently': 2, 'countdown': 1, 'manan': 1, '452': 1, 'edible': 1, 'kavkazcenter': 1, 'sadulayev': 1, 'khalim': 1, 'rivaling': 1, 'telethons': 1, 'kalma': 2, 'acropolis': 2, 'unesco': 1, 'parthenon': 1, '485': 1, '392': 1, 'clamped': 1, 'drywall': 1, 'helipad': 1, 'kruif': 1, 'bedfordshire': 1, 'khayam': 3, 'bedford': 1, 'skyjackers': 1, 'mourn': 5, 'straying': 2, 'expedite': 2, '726': 1, 'atwood': 2, 'artificially': 2, 'chang': 4, 'bestseller': 1, 'nanking': 1, 'cola': 3, 'pesticides': 1, 'cse': 3, 'residue': 1, 'statistically': 1, 'stringent': 3, 'onassis': 1, 'kennedys': 1, 'stillborn': 1, 'jacqueline': 1, 'repose': 1, 'hkd': 1, 'konstanz': 1, 'hajdib': 2, 'suitcases': 1, 'koblenz': 1, 'dortmund': 1, 'kazmi': 1, 'outnumbering': 1, '8000': 1, 'hycamtin': 2, 'cisplatin': 1, 'organs': 3, 'cervix': 1, 'prolonging': 1, 'jutarnji': 1, 'miljus': 3, 'hina': 1, 'bats': 1, 'diligently': 1, 'loj': 2, 'shy': 1, 'cowboy': 2, 'casual': 1, 'bankrupt': 3, 'grinstein': 1, 'destinations': 2, 'rafat': 1, 'ecc': 2, 'costliest': 1, 'burdens': 1, 'rmi': 1, 'downsizing': 1, 'cooled': 1, 'lackluster': 2, 'psa': 1, 'nagorno': 1, 'karabakh': 1, 'chiefdoms': 1, 'springboard': 1, '1844': 1, 'dominicans': 1, 'leonidas': 1, '1930': 2, 'unsettled': 2, 'trujillo': 1, 'balaguer': 3, 'popes': 2, '1870': 1, 'circumscribed': 1, 'lateran': 1, 'catholicism': 3, 'primacy': 1, 'concordat': 1, 'interreligious': 2, 'profess': 1, 'ungrudgingly': 1, 'commiserated': 1, 'surely': 1, 'vasp': 3, 'celma': 1, 'slater': 2, 'inhabitant': 1, 'mcqueen': 2, 'illustrious': 1, 'papillon': 1, 'bullitt': 1, "'70s": 1, 'mc': 1, 'boyhood': 1, 'memorabilia': 1, 'yung': 1, 'meaningless': 1, 'graz': 1, 'saturated': 2, 'nozari': 1, 'dormitory': 2, 'hamshahri': 1, 'shargh': 1, 'unforgivable': 2, 'chow': 1, 'panyu': 2, 'chilled': 1, 'fuzhou': 1, 'antibiotics': 3, 'jessica': 1, 'fiance': 1, 'raviglione': 3, 'xtr': 1, 'ludford': 1, 'hanjiang': 1, 'mashjid': 1, 'jalna': 1, 'falluji': 1, 'osaid': 1, 'najdi': 1, 'sawt': 1, 'newsletter': 1, 'disseminate': 1, 'jihadist': 1, 'khaleeq': 1, 'tumbling': 1, 'kel': 1, 'fela': 1, 'seun': 1, 'kuti': 1, 'moweta': 1, 'nnamdi': 1, 'leo': 1, 'cheaply': 1, 'undercutting': 1, 'bomblets': 1, 'hyper': 1, 'eavesdrop': 3, 'clearances': 1, 'assistants': 1, 'dubs': 1, 'vivanco': 2, 'disregarding': 1, 'discriminating': 1, 'laguna': 1, 'aynin': 1, 'haaretzthat': 1, 'gumushane': 1, 'zigana': 1, 'semiarid': 1, 'intersect': 1, 'surround': 1, 'arla': 1, 'loses': 1, 'chretien': 1, 'secularist': 1, 'toptan': 1, 'koksal': 1, 'rim': 1, 'disowning': 1, 'lustiger': 2, 'dame': 1, 'notre': 1, 'patriarchal': 1, 'khalayleh': 1, 'doomsday': 1, 'gobe': 1, 'squadrons': 1, 'uprooting': 1, 'admits': 2, 'conclusive': 1, 'waded': 1, 'unprovoked': 1, 'faults': 2, 'shargudud': 2, 'habsadeh': 2, 'hamidreza': 1, 'thalia': 1, 'estefan': 1, 'feliciano': 2, 'jamrud': 1, 'venevision': 1, 'ovidio': 1, 'cuesta': 1, 'baramullah': 1, 'srinigar': 1, 'assembling': 1, 'bikaner': 1, 'sketch': 1, 'kimunya': 2, 'amos': 1, 'esmat': 1, 'klain': 1, '599': 1, 'emanate': 1, 'froeshaug': 1, 'dahl': 1, '4x5': 1, 'atiku': 1, 'lai': 1, 'immoral': 1, 'yambio': 1, 'memene': 1, 'seyi': 1, 'veil': 1, 'kerosene': 1, 'ramdi': 1, 'deepak': 1, 'gurung': 1, '256': 3, 'voyages': 1, 'bago': 1, 'ein': 2, 'kyauk': 1, 'kanyutkwin': 1, 'allert': 1, 'behave': 2, '428': 1, 'sounding': 1, 'caufield': 1, 'byers': 1, 'blossomed': 1, 'kleiner': 1, 'lockerbie': 2, 'nurses': 5, 'machakos': 1, 'makutano': 1, 'brew': 2, 'drank': 1, 'methanol': 1, "chang'aa": 1, 'akhmad': 2, 'palpa': 1, 'bitlis': 1, 'sulaimaniyah': 2, 'pejak': 1, 'hyperinflation': 2, 'cessation': 1, 'nwr': 2, 'midway': 3, 'hawaiian': 1, 'papahanaumokuakea': 1, 'refuges': 2, 'terrestrial': 1, 'vegetation': 2, 'insects': 1, 'seabirds': 1, 'corals': 1, 'shellfish': 1, 'colon': 1, 'traverse': 1, 'firsts': 1, 'aruban': 1, 'aruba': 2, 'dip': 1, 'footing': 2, 'fuss': 1, 'lamentation': 1, 'frequenting': 1, 'batsmen': 1, 'jaques': 1, 'harbhajan': 2, '493': 1, '332': 2, 'shbak': 3, 'intimidating': 1, 'intra': 1, 'sucre': 1, 'gasquet': 3, 'mirnyi': 3, 'rochus': 1, 'olivier': 1, 'ramstein': 1, 'pontifical': 1, 'castel': 1, 'poupard': 1, 'gandolfo': 1, 'byzantine': 3, 'nun': 5, 'theorized': 1, 'othman': 1, 'swank': 1, 'guild': 3, 'aviator': 2, 'portrayal': 1, 'katherine': 1, 'freeman': 1, 'cate': 3, 'hepburn': 1, 'blanchett': 3, 'orbach': 1, 'shaloub': 1, 'sideways': 2, 'quirky': 1, 'ensemble': 1, 'duress': 2, 'jpmorgan': 1, 'materazzi': 4, 'midfielder': 1, 'ramming': 1, 'verbally': 2, 'wael': 1, 'gunning': 2, 'rubaei': 1, 'izzariya': 1, 'arafa': 2, 'schatten': 2, 'embryonic': 2, 'embryos': 1, 'coercion': 1, 'underlings': 1, 'preclude': 1, 'impropriety': 1, '127': 1, 'fadela': 1, 'chaib': 1, 'sufferer': 1, '315': 1, 'beleaguered': 2, 'kandili': 1, 'anatolianews': 1, 'erzurum': 1, 'governorates': 1, 'qods': 1, 'explosively': 2, 'efps': 2, 'shorten': 1, 'healing': 2, 'adhamiya': 2, 'significance': 2, 'editorials': 1, 'gye': 1, 'wafd': 1, 'europol': 3, 'baleno': 1, 'newseum': 4, 'fatigues': 1, 'revote': 1, 'aleg': 1, 'picnicking': 1, 'badghis': 5, 'qadis': 1, 'iveta': 2, 'benesova': 4, 'na': 1, 'gajdosova': 1, 'jarmila': 1, 'ting': 1, 'tiantian': 1, 'bild': 4, 'rtl': 2, 'scandalized': 1, 'anymore': 1, 'downriver': 2, 'mains': 1, 'mechanics': 1, 'ana': 1, 'rauchenstein': 1, 'sabaa': 1, 'inflexible': 2, 'spelled': 1, 'guzzling': 1, 'drel': 2, '46th': 2, 'contradict': 2, 'lobes': 1, 'sedatives': 1, "l'aquila": 4, 'caricatures': 2, 'soir': 1, 'acquaint': 1, 'acosta': 4, 'inflammable': 1, 'consumes': 1, 'philanthropy': 1, 'mikati': 4, 'loopholes': 1, 'nhs': 3, 'hutton': 1, 'paktiawal': 3, 'disgust': 2, 'forgivable': 1, 'faras': 1, 'jabouri': 2, 'aqidi': 1, '163': 3, 'moore': 6, 'joanne': 1, 'fahad': 1, 'cured': 1, 'verveer': 3, 'melanne': 1, 'epidemics': 1, 'invests': 2, 'yomiuri': 2, 'nanjing': 1, 'haerbaling': 1, 'divulge': 1, 'crafted': 1, 'naimi': 3, 'pennies': 1, 'counselor': 2, 'tsholotsho': 1, 'chinotimba': 1, 'inbalance': 1, 'madero': 1, 'otis': 1, 'powerlines': 1, 'whiskey': 1, 'gunshots': 2, 'banderas': 4, '710': 2, 'abolishing': 1, 'convicts': 2, 'commutes': 1, 'footprints': 1, 'khuzestan': 1, 'saudia': 1, 'jules': 1, 'guere': 1, 'influnce': 1, 'majzoub': 1, 'samkelo': 1, 'mokhine': 1, 'mirza': 1, 'jehangir': 1, 'enthusiastically': 1, 'acknowledgment': 1, 'muqrin': 2, 'thrifty': 1, 'handmade': 1, 'micronesians': 1, 'polynesians': 1, 'ellice': 2, 'tonga': 3, 'vanilla': 1, 'squash': 2, 'tongan': 1, 'upturn': 1, 'tanganyika': 1, 'zanzibar': 3, 'minimizing': 1, 'negation': 1, '1010': 1, 'tifa': 1, 'fashioning': 1, 'twigs': 2, 'fowler': 3, 'thrush': 1, 'intently': 1, 'fitting': 4, 'trod': 2, 'upwards': 1, 'viper': 4, 'myself': 3, 'purposed': 1, 'swoon': 1, 'unawares': 1, 'snares': 1, 'ahmedou': 1, 'kouk': 1, 'ashayier': 1, "sa'dun": 1, 'hamduni': 1, 'foreman': 4, 'talha': 2, 'hmas': 1, 'fitted': 1, 'turnbull': 1, 'awantipora': 1, 'saldanha': 1, 'lateef': 1, 'adegbite': 1, 'tenets': 1, 'betrayed': 2, 'ulemas': 2, 'darkest': 1, 'zarqwai': 1, 'scrubbed': 1, 'hangar': 1, 'dovish': 2, 'annex': 1, 'blocs': 2, 'unilaterally': 2, 'annexing': 1, 'sabri': 1, 'disengagement': 3, 'shas': 2, 'abolish': 3, '335': 1, 'treetops': 1, 'compliments': 1, 'obaidullah': 1, 'akhund': 2, 'advani': 4, 'lal': 1, 'subcontinent': 1, 'jinnah': 1, 'patched': 1, 'kononov': 3, 'vassily': 1, 'slandered': 1, 'yoshie': 1, 'reese': 2, 'sato': 1, 'yokosuka': 1, 'kitty': 1, 'assessors': 1, 'vimpelcom': 1, 'jaunpur': 1, 'patna': 1, 'rdx': 2, 'hexogen': 1, 'unclaimed': 1, 'bavaria': 1, 'allay': 1, 'aggressions': 1, '58th': 1, 'wrecked': 1, 'shadows': 1, 'shines': 1, 'gomes': 2, 'aijalon': 1, 'unhindered': 1, 'renunciation': 2, 'kasab': 1, 'taiba': 2, 'faizullah': 1, 'youm': 1, 'akhbar': 1, 'sects': 1, 'solecki': 3, 'jamia': 1, 'americanism': 1, '049': 1, 'verizon': 2, 'sukhorenko': 2, 'stepan': 1, 'kgb': 2, 'proclaiming': 2, 'parvanov': 1, 'prolongs': 1, 'georgi': 1, 'normalized': 1, 'hennadiy': 1, 'vasylyev': 1, 'epa': 1, 'sandwich': 2, 'swahili': 1, 'ecweru': 1, 'mulongoti': 1, 'bududa': 1, 'header': 1, 'khedira': 1, 'mesut': 1, '82nd': 1, 'oezil': 1, 'edinson': 1, 'equalized': 1, 'mueller': 6, 'cavani': 1, 'marcell': 1, 'forlan': 1, 'jansen': 1, 'montagnards': 3, 'resettling': 1, 'smearing': 2, 'unsubstantiated': 1, 'categorized': 1, 'karo': 1, 'shegag': 1, 'hudaidah': 1, '411': 1, 'luca': 2, 'badoer': 1, 'maranello': 1, 'reggio': 1, 'emilia': 1, 'venice': 1, 'ignites': 1, 'scoop': 1, 'footprint': 1, 'subsurface': 1, '679': 1, 'cantv': 2, '447': 1, 'buyukanit': 1, 'yasar': 2, 'tokage': 3, 'tolling': 2, 'wadowice': 1, 'wept': 2, 'knees': 1, 'worshipped': 1, 'readies': 1, 'gurirab': 1, 'redistribution': 1, 'sensing': 1, 'kindergarten': 3, 'petting': 1, 'binyamina': 1, 'symptom': 1, 'chilling': 1, 'echoed': 2, 'bullying': 1, 'deprive': 1, 'mastering': 1, 'wajama': 2, 'wabho': 1, 'electricial': 1, 'jankovic': 5, 'gojko': 2, 'trusting': 1, 'flourished': 2, 'triumf': 1, 'riza': 2, 'greenland': 5, 'vikings': 1, 'easternmost': 1, 'kwajalein': 1, 'usaka': 1, '1881': 1, 'bourguiba': 2, 'offender': 1, 'paroled': 1, 'joachim': 1, 'ruecker': 1, 'unmatched': 1, 'repressing': 1, 'abidine': 1, 'zine': 1, 'tunis': 1, 'ghannouchi': 1, "m'bazaa": 1, 'viable': 2, 'fluctuated': 1, 'terrible': 1, 'alderman': 2, 'zoological': 1, 'raccoon': 2, 'tales': 1, 'graze': 1, 'tidings': 1, 'hound': 3, 'entertained': 1, 'whence': 1, 'boatmen': 1, 'outpouring': 2, 'emotion': 1, 'yielding': 1, 'gasped': 1, 'perilous': 1, 'wretched': 1, 'clauses': 3, 'renoun': 1, 'subordinate': 1, 'elves': 1, 'leonardo': 1, 'dicaprio': 2, 'overaggressive': 1, 'sparing': 2, 'falun': 5, 'gong': 5, 'hsi': 1, 'ching': 1, 'mistreats': 1, 'kafr': 1, 'baltim': 1, 'zigazag': 1, 'entebbe': 1, 'kakooza': 2, 'jovica': 1, 'simatovic': 1, 'nihilism': 1, 'fanatic': 1, 'insidious': 1, 'copts': 1, 'nightly': 1, 'renegotiating': 1, 'karabilah': 4, 'cone': 1, 'dye': 2, 'insulation': 2, 'foam': 1, 'disintegration': 1, 'goldsmith': 1, "u'zayra": 1, 'larkin': 1, 'khayber': 1, 'hyuck': 1, 'format': 1, 'worthless': 1, 'satterfield': 4, '617': 2, 'gulag': 2, 'ri': 2, 'dae': 1, 'jo': 3, 'hamash': 1, 'levied': 1, 'bextra': 2, 'prescriptions': 1, 'somavia': 1, 'passersby': 2, 'spelling': 1, 'sayings': 1, 'donetsk': 2, 'borjomi': 1, 'jaca': 1, 'pyeongchang': 1, 'salzburg': 1, 'questionnaire': 1, 'accommodations': 1, '6th': 1, 'belligerence': 2, 'negotiation': 3, 'polonnaruwa': 1, 'circa': 1, 'buddhism': 1, '1200': 1, 'anuradhapura': 1, '1070': 1, 'mcchrystal': 1, 'pinerolo': 1, '1500s': 1, 'glides': 1, 'sliding': 4, 'circular': 1, 'norpro': 1, 'matesi': 3, 'briquettes': 1, 'tenaris': 2, 'xingguang': 1, '1802': 1, '1796': 1, 'pellegrini': 1, 'alain': 1, 'ceylon': 1, 'lubero': 1, 'guehenno': 1, 'eelam': 1, 'overshadow': 1, 'natasha': 2, 'kofoworola': 1, 'quist': 3, 'petkoff': 3, 'anguish': 1, 'cual': 1, 'borges': 1, 'ojeda': 1, 'proficiency': 1, 'indoctrinate': 1, 'bounds': 1, 'weekends': 1, 'gravel': 1, 'kihonge': 1, 'supervising': 1, 'dacic': 1, 'yugoslavian': 1, 'wreaths': 1, 'larose': 3, 'colleen': 1, 'drillings': 1, 'arising': 3, 'pedraz': 1, 'couso': 2, 'portsyuk': 1, 'taras': 1, 'maori': 2, 'buenaventura': 1, 'waitangi': 1, 'chieftains': 1, 'npa': 1, '1843': 1, 'tsotne': 1, 'gamsakhurdia': 4, 'zviad': 1, 'hajan': 1, 'coleman': 2, 'lapsed': 1, 'printemps': 1, '464': 1, 'dispatching': 1, 'brandished': 2, 'vampire': 1, 'rigorous': 2, 'refill': 1, 'usage': 2, 'wraps': 2, 'trespassing': 1, 'botanical': 2, 'seini': 1, 'sperling': 4, 'leniency': 1, 'rebuke': 1, 'khamas': 1, 'hajem': 1, 'depended': 3, 'denigrates': 1, 'bole': 1, 'nihal': 1, 'ruble': 1, 'steward': 1, 'codes': 1, 'göteborg': 1, 'krisztina': 1, 'nagy': 1, 'anp': 3, 'tiechui': 1, 'paramedics': 1, 'irrawady': 1, 'fazul': 1, 'stanezai': 1, 'buster': 1, 'impervious': 1, 'lioness': 1, 'harden': 1, 'drift': 1, 'easley': 1, 'laszlo': 1, 'solyom': 1, 'hares': 3, 'mufti': 1, 'shuttles': 1, 'jalula': 1, 'yourselves': 1, 'amerli': 1, 'petrol': 1, 'invariably': 1, 'passers': 1, 'leveraged': 1, 'maltese': 1, 'faroe': 2, 'faroese': 1, 'dynasties': 1, 'moorish': 1, '258': 3, "sa'adi": 1, '1578': 1, 'alaouite': 1, '1860': 1, 'tangier': 1, 'internationalized': 1, 'bicameral': 1, 'taboo': 2, 'moderately': 1, 'hobbles': 1, 'overborrowing': 1, 'overspending': 1, 'realizing': 3, 'favourite': 2, 'stables': 1, 'licked': 1, 'lapdog': 4, 'dainty': 1, 'blinking': 1, 'lap': 2, 'stroked': 1, 'prancing': 1, 'commenced': 1, 'pitchforks': 1, 'joke': 1, 'jesting': 1, 'clumsy': 1, 'summers': 1, 'natured': 1, 'shibis': 1, 'dhimbil': 2, 'hawiye': 2, 'llasa': 1, 'achouri': 1, 'radhia': 1, 'trily': 1, 'showdowns': 1, 'reprimands': 1, 'sanaa': 1, 'limburg': 1, 'coleand': 1, 'supertanker': 1, 'fawzi': 2, 'supervises': 1, 'dmitrivev': 1, 'prestwick': 1, 'geithner': 2, 'jagdish': 1, 'tytler': 2, 'yongbyon': 1, 'renaissance': 1, 'kedallah': 1, 'younous': 1, 'purging': 1, 'demeans': 1, 'gratuitous': 1, 'instructional': 1, 'kuta': 1, 'eide': 6, 'taormina': 1, 'suggestive': 1, 'taboos': 1, 'menstrual': 1, 'unidentifed': 1, 'provocateurs': 1, 'enticing': 2, '385': 1, 'gration': 3, 'falsifying': 1, 'saqib': 3, 'shootouts': 1, 'eastward': 1, 'alternately': 1, 'taatgen': 1, 'hendrik': 1, 'ambrosio': 1, 'intakes': 1, 'wastewater': 1, 'yingde': 1, 'reservoirs': 1, 'neurological': 1, 'knockout': 1, 'strongmen': 1, 'nazareth': 3, 'yardenna': 1, 'dwelling': 1, 'courtyard': 1, 'annunciation': 1, 'copenhagen': 1, 'enacting': 1, 'snub': 1, 'siachen': 1, 'equate': 2, 'kalikot': 1, 'seiken': 1, 'sugiura': 2, 'spouses': 1, 'torres': 1, 'yake': 1, '867': 1, 'headbands': 1, '577': 1, '453': 2, '969': 1, '729': 1, 'twisted': 1, 'jamaican': 3, 'lewthwaite': 2, 'germaine': 1, 'fanatics': 1, 'holdouts': 1, 'lamivudine': 1, 'zidovudine': 1, 'nevirapine': 6, 'aurobindo': 1, 'pharma': 1, 'regimen': 1, 'patents': 1, 'sharpened': 1, 'daley': 1, 'rahm': 1, 'lid': 2, 'repays': 1, 'liempde': 1, 'kesbir': 1, 'nuriye': 1, 'climbs': 1, 'cot': 1, 'greeks': 2, 'breasts': 1, 'exam': 1, 'malakhel': 1, 'osmani': 1, 'aubenas': 1, 'reopens': 1, 'xinjuan': 1, 'bruises': 1, 'trondheim': 1, 'georg': 1, 'vague': 1, 'eidsvig': 2, 'bernt': 1, 'choir': 2, 'pedophilia': 1, 'takeifa': 1, 'jac': 2, 'keita': 1, 'ricci': 2, 'shyrock': 1, 'hulya': 2, 'kocyigit': 2, '675': 1, 'architectural': 1, 'profound': 2, 'burgeoning': 1, 'hinting': 1, 'managua': 1, 'disavowed': 1, 'murr': 1, 'shameful': 2, 'thuggery': 1, 'michuki': 1, 'bitten': 1, 'doghmush': 2, 'bijeljina': 1, 'neskovic': 1, 'gebran': 2, 'amagasaki': 1, 'osaka': 1, 'milososki': 1, 'beneficial': 1, 'gruevski': 1, 'crvenkovski': 1, 'mia': 1, 'nikola': 2, 'swerved': 1, '930': 1, 'althing': 1, 'askja': 1, 'emigrated': 3, 'paracel': 2, 'pattle': 1, 'woody': 1, '1788': 1, 'islanders': 1, 'mechanized': 1, 'bailouts': 2, 'rutte': 1, 'dwindling': 2, 'industrialization': 1, 'soles': 1, 'fasten': 1, 'idols': 1, 'unlucky': 1, 'pedestal': 1, 'hardly': 1, 'splendour': 1, 'sultans': 1, 'dukeness': 1, 'liege': 1, 'gleaming': 1, 'catarrh': 1, 'badgesty': 1, 'gorgeous': 1, 'jewel': 1, 'overtaken': 2, 'rabbit': 1, 'amusement': 2, 'obstructs': 1, 'showers': 1, 'cheyenne': 1, 'wednesdays': 1, 'upholding': 1, 'abbasali': 1, 'kadkhodai': 1, 'tahreer': 1, 'compare': 2, 'peshmerga': 1, 'ludlam': 2, 'christman': 1, 'starved': 1, 'menoufia': 1, '43nd': 1, 'unasur': 1, 'clarifies': 3, 'basile': 1, 'casmoussa': 1, 'rodier': 1, 'guenael': 1, 'attributing': 1, 'glacial': 2, 'cachet': 1, 'colonia': 1, 'burrow': 1, 'casassa': 1, 'gino': 1, 'repeals': 1, 'segun': 1, 'nfa': 1, 'salisu': 1, 'odegbami': 1, 'hendarso': 1, 'danuri': 2, 'sumatran': 1, 'religiously': 1, '269': 1, 'alienate': 1, 'blears': 1, 'hazel': 1, 'khalidiyah': 1, 'bakwa': 1, 'asghari': 5, 'ziba': 1, 'sharq': 1, 'awsat': 1, 'tempers': 1, 'designating': 1, 'excited': 1, 'spontaneous': 2, 'motel': 1, 'dietary': 1, 'hauled': 1, 'pragmatists': 1, 'copes': 1, 'revisited': 1, 'asteroid': 5, 'wd5': 1, 'stargazers': 1, '1908': 2, 'megaton': 1, 'exhumation': 1, 'glorious': 1, 'auwaerter': 2, 'johns': 1, 'fabrizio': 1, 'meoni': 3, 'kiffa': 1, 'motorcyclists': 1, 'charter97': 1, 'despres': 1, 'cyril': 2, 'ktm': 1, '8956': 1, 'ceremon': 1, 'flabbergasted': 1, 'asparagus': 1, 'acidic': 1, 'dirt': 2, 'vapor': 1, 'cassettes': 1, 'augment': 1, 'mcbride': 1, 'vocalist': 1, 'mcgraw': 1, 'wiseman': 1, 'nichols': 1, 'peformed': 1, 'craig': 1, 'gretchen': 1, 'horizon': 1, 'kristofferson': 1, 'kris': 1, 'zamani': 1, 'benisede': 3, 'speedboats': 1, 'coherent': 1, 'nyein': 1, 'ko': 1, 'naing': 1, 'appropriated': 1, 'fadhila': 1, 'perverted': 1, 'cctv': 1, 'nozzles': 1, 'salomi': 1, 'reads': 1, 'blackwater': 1, 'incited': 2, 'ire': 1, 'benedicto': 1, 'commercio': 1, 'jimenez': 3, 'zevallos': 1, 'boersma': 1, 'dee': 1, 'serfdom': 1, 'dumitru': 1, 'newsroom': 1, 'formalities': 1, 'dens': 1, 'airdrop': 1, 'ingmar': 1, 'bergman': 3, 'faro': 1, 'actresses': 1, 'ullman': 1, 'bibi': 1, 'andersson': 1, 'strawberries': 1, 'madness': 1, 'tinged': 1, 'melancholy': 1, 'humor': 1, 'iconic': 1, 'knight': 1, 'bylaw': 1, 'thg': 1, 'tetrahydrogestrinone': 1, 'boa': 1, 'mailbox': 1, 'nist': 4, 'measurer': 1, 'machining': 1, 'invent': 1, 'braille': 1, 'nanotechnology': 1, 'cybersecurity': 1, 'grid': 1, 'hoses': 1, 'standardized': 1, 'mummified': 1, 'mummy': 2, 'earthenware': 1, 'sarcophagus': 2, 'teir': 1, 'atoun': 1, 'theyab': 2, 'beatified': 2, 'habsburg': 1, 'emmerick': 1, 'mel': 1, 'visions': 1, 'sainthood': 2, 'gibson': 1, 'ludovica': 1, 'angelis': 1, 'beatification': 1, 'directorate': 1, 'touted': 1, 'prizren': 2, 'handovers': 1, 'vigilante': 1, 'idema': 3, 'edwin': 1, 'lakers': 2, 'hornets': 3, 'workload': 1, 'rezai': 3, 'principlists': 1, 'khazaal': 1, 'zeid': 1, 'reem': 1, 'gheorghe': 1, 'flutur': 1, 'caraorman': 1, 'leavitt': 2, 'huy': 1, 'preventive': 3, 'nga': 1, 'locus': 1, 'interwar': 2, 'slavic': 1, 'dniester': 1, 'transnistria': 1, 'moldovan': 1, 'voronin': 2, 'pcrm': 1, 'aie': 2, 'reconstituted': 1, 'barren': 1, '1755': 1, 'heyday': 1, 'crocodile': 1, 'murderer': 1, 'bosom': 1, 'grin': 1, 'coals': 1, 'coldness': 1, 'civilly': 1, 'thawed': 1, 'pleasures': 1, 'foreshadows': 1, '940': 1, 'budgeted': 2, 'tedder': 2, 'bedingfield': 1, 'onerepublic': 2, 'dreaming': 1, 'beatboxing': 2, 'beatboxers': 1, 'adverse': 4, 'dispenses': 1, 'anybody': 1, 'machage': 1, 'joshua': 1, 'kutuny': 1, 'wilfred': 1, 'kapondi': 1, 'mistaking': 2, 'dragmulla': 1, 'hurl': 1, 'marts': 1, 'pierce': 6, '29th': 3, 'gucht': 1, 'elvira': 1, 'karel': 2, 'nabiullina': 1, 'shoaib': 1, 'okonkwo': 1, 'festus': 1, 'attends': 1, 'amed': 1, 'snag': 1, 'olpc': 3, 'exclusively': 1, 'loophole': 1, 'kordofan': 1, 'ghubaysh': 1, 'sla': 1, 'federations': 1, 'edel': 1, 'checkup': 1, 'espinoso': 1, 'osvaldo': 2, 'rivero': 1, 'chepe': 1, 'poet': 1, 'conspired': 1, 'uddin': 1, 'nabeel': 1, 'gulzar': 1, 'shamin': 1, 'skill': 2, 'treasures': 1, 'dakhlallah': 1, 'ideals': 1, 'hortefeux': 2, 'brice': 1, 'flashlight': 1, 'interrogator': 1, 'bishara': 5, 'azmi': 1, 'enkhbayar': 1, 'samper': 3, 'cali': 1, 'barco': 1, 'bogata': 1, 'headache': 1, 'tagging': 1, 'mastered': 1, 'methane': 3, 'doused': 1, 'unfolded': 2, 'blizzards': 1, 'herders': 1, 'liban': 1, 'afder': 1, 'gode': 1, 'deteriorate': 1, 'atomstroyexport': 1, 'blowtorch': 1, 'deteriorates': 1, 'kisangani': 1, 'temur': 1, 'kidnapper': 1, 'pasted': 1, 'krivtsov': 1, 'iraqna': 1, 'faeldon': 2, 'makati': 1, 'posh': 1, 'nicanor': 2, 'deluxe': 1, 'ghorian': 1, 'navfor': 1, 'harardhere': 1, 'sacrificial': 1, 'altar': 2, 'ubt': 1, 'sardenberg': 2, 'validity': 2, 'trampling': 1, 'keck': 1, 'vortex': 3, 'infrared': 2, 'vortices': 1, 'tf': 2, 'teenaged': 1, 'jungles': 2, 'mastung': 1, 'voltage': 2, 'naushki': 1, 'wellhead': 1, 'cawthorne': 1, 'shams': 2, 'dignified': 1, 'emails': 1, 'weird': 1, 'flakus': 1, 'mingora': 1, 'neolithic': 1, 'republished': 1, 'alok': 1, 'tomar': 2, 'lauded': 1, 'palocci': 1, 'rato': 1, 'ringing': 1, 'tet': 1, 'hermosa': 1, 'myitsone': 1, 'northernmost': 2, 'kachin': 1, 'humam': 1, 'hammoudi': 1, 'narayan': 1, 'pokhrel': 3, 'mora': 2, 'huerta': 1, 'announces': 2, 'knut': 1, 'ahnlund': 3, 'elfriede': 1, 'jelinek': 1, 'descriptions': 2, 'irreparably': 1, 'engdahl': 1, 'horace': 1, 'madison': 1, 'glasses': 1, 'tinted': 1, 'lennon': 1, 'goldberg': 1, 'whoopie': 1, 'taupin': 1, 'lyricist': 1, 'serenaded': 1, 'comedians': 2, 'portraying': 1, 'waldron': 2, 'shaheen': 1, 'nexus': 1, 'watni': 1, 'cheecha': 1, 'corvettes': 1, 'overruns': 1, 'sipah': 1, 'sahaba': 1, 'husbandry': 1, 'terrain': 4, 'upscale': 5, 'conscientious': 1, 'entrepreneurship': 1, 'encompasses': 2, 'supplements': 1, 'pretended': 1, 'lameness': 1, 'butcher': 2, 'pulse': 1, 'vexation': 1, 'mouthful': 1, 'insert': 1, 'flagon': 1, 'sup': 1, 'requital': 1, 'anesthetist': 1, 'pager': 1, 'stethoscope': 1, 'dangling': 1, 'ellison': 4, 'hardfought': 1, 'mcneill': 2, '286': 1, '252': 1, 'tuncay': 1, 'seyranlioglu': 2, 'heroic': 1, 'tabinda': 1, 'kamiya': 1, 'astros': 2, 'clemens': 3, 'leagues': 1, 'sosa': 1, 'sammy': 1, 'outfielders': 1, 'hander': 1, 'strikeouts': 1, 'cy': 1, 'rippled': 1, 'deauville': 1, 'comerio': 2, 'kouguell': 1, 'compares': 2, 'padang': 1, 'daraga': 1, 'catanduanes': 1, 'durian': 1, 'enezi': 1, 'kut': 1, 'rescind': 1, 'ryon': 1, 'dawoud': 1, 'rasheed': 1, 'thawing': 1, 'predictor': 1, 'punxsutawney': 2, 'stump': 1, 'groundhog': 4, 'candlemas': 1, 'carryover': 1, 'copa': 2, 'wwii': 1, 'blared': 1, 'dabbagh': 1, 'keynote': 1, 'complementary': 1, 'genome': 1, 'dripped': 1, 'notion': 1, 'atttacks': 1, 'hurriya': 1, 'kufa': 1, 'boodai': 1, 'swamped': 1, 'tearfund': 1, 'andrhra': 1, 'ar': 1, 'rutbah': 1, 'khurram': 1, 'bing': 1, 'msn': 1, 'mehran': 1, 'sufi': 1, 'heretics': 1, 'sufis': 1, 'turkman': 1, 'qaratun': 1, 'khairuddin': 1, 'nassif': 2, '590': 1, 'yair': 1, 'klein': 2, 'landlords': 1, 'mykolaiv': 1, 'maariv': 2, 'rohingya': 2, 'chasing': 1, 'loyalist': 1, 'lvf': 1, 'oppress': 1, 'tyrants': 1, 'rocker': 1, 'springsteen': 1, 'repertoire': 1, 'bmg': 2, 'idolwinner': 1, 'kabylie': 2, 'refrigerated': 1, 'abass': 1, 'ieds': 2, 'barbero': 1, 'neutralizing': 1, 'jowell': 1, 'tessa': 1, 'expose': 1, 'baojing': 1, 'jianhao': 1, 'blackmailing': 1, 'blackmailer': 2, '88th': 1, 'floquet': 2, 'maurice': 1, 'somme': 1, 'triomphe': 1, 'pongpa': 1, 'lek': 2, 'alimony': 2, 'wasser': 1, 'backup': 1, 'irreconcilable': 1, 'cavaliers': 2, 'mcgrady': 2, 'tracy': 1, 'lebron': 1, 'pacers': 1, 'assists': 2, 'croshere': 1, 'steals': 1, 'dunleavy': 1, 'wam': 1, 'kani': 1, 'faridullah': 1, 'interivew': 1, 'jianzhong': 1, 'surkh': 1, 'nangahar': 1, 'empowers': 2, 'penalize': 1, 'baptismal': 1, 'arranges': 1, 'shutters': 1, 'portico': 1, 'rites': 1, 'asserting': 1, 'sarabjit': 1, 'moods': 1, 'retirements': 1, 'politicized': 1, 'derg': 1, 'selassie': 1, 'mayan': 2, 'kartli': 1, 'kingdoms': 1, 'colchis': 1, 'iberia': 1, '330s': 1, 'persians': 2, 'shevardnadze': 2, 'acc': 2, 'circumpolar': 1, 'oceanographic': 1, 'ecologic': 1, 'convergence': 4, 'mingle': 1, 'discrete': 1, 'concentrates': 1, 'approximates': 1, 'imply': 2, '1631': 1, 'retook': 1, '1633': 1, 'amongst': 1, 'frightening': 3, 'roamed': 1, 'amused': 1, 'frighten': 1, 'fool': 2, 'perched': 1, 'beak': 1, 'wily': 1, 'stratagem': 1, 'complexion': 1, 'deservedly': 1, 'deceitfully': 1, 'refute': 1, 'housedog': 3, 'reproached': 1, 'luxuriate': 1, 'depicted': 1, 'zuhair': 1, 'reapprove': 1, 'abiya': 1, 'chitigov': 1, 'shali': 1, 'rizvan': 1, 'rescinded': 1, 'judo': 1, 'repatriation': 1, 'ticona': 2, 'esperanza': 1, 'copiapo': 1, 'chute': 2, 'libel': 2, 'shadowed': 1, 'zyazikov': 1, 'nazran': 1, 'sodomy': 2, 'jafri': 1, 'venomous': 1, 'vindicated': 1, 'samara': 1, 'arsamokov': 1, 'ingush': 1, 'condominiums': 1, 'equipping': 1, 'interacted': 1, 'ahvaz': 2, 'kayhan': 1, 'pinas': 1, 'alabang': 1, 'schoof': 1, 'ayala': 1, 'baikonur': 1, 'cosmodrome': 1, 'pythons': 1, 'wedded': 1, 'cage': 1, 'kroung': 1, 'kandal': 1, 'chamrouen': 2, 'pich': 1, 'python': 4, 'inanimate': 1, 'inhabit': 1, 'animism': 1, 'subscribe': 1, 'snakes': 1, 'vy': 1, 'neth': 1, 'dharmsala': 1, 'acknowledging': 1, 'worthwhile': 1, 'rosaries': 1, 'colleges': 2, 'venom': 1, 'scorpion': 1, 'selectively': 1, '516': 1, 'collier': 3, 'enlarged': 1, 'howell': 1, 'franchises': 1, 'echocardiograms': 1, 'eddy': 1, 'knicks': 1, 'curry': 1, 'heartbeat': 1, 'irregular': 1, 'hoiberg': 1, 'guenter': 1, 'verheugen': 1, 'sonntag': 2, 'sheeting': 1, 'coveted': 1, 'lithium': 4, 'entitle': 1, 'salar': 1, 'uyuni': 1, 'sics': 2, 'aweys': 1, 'dahir': 1, 'ousting': 3, 'powerless': 1, 'tvn': 1, 'stainmeier': 1, 'butmir': 1, 'eufor': 1, 'karameh': 1, 'sapporo': 2, 'dwarfed': 1, 'pressewednesday': 1, 'jeremic': 2, 'selig': 1, 'bud': 1, 'fehr': 1, 'sox': 1, 'sama': 1, 'murillo': 1, 'hokkaido': 1, 'stiffer': 1, 'carphedon': 1, 'hondo': 4, 'cyclist': 2, 'murcia': 1, 'gerolsteiner': 1, 'issori': 1, 'scourge': 1, 'duggal': 2, 'atmospheric': 2, 'buoys': 1, 'thammararoj': 1, 'killer': 1, 'cerkez': 5, 'dario': 1, 'kordic': 1, 'adewale': 1, 'ganiyu': 1, 'badoosh': 1, 'zibari': 1, 'babaker': 1, 'dodik': 2, 'lajcak': 1, 'clamps': 1, 'anand': 1, 'sterilized': 1, 'balasingham': 2, 'siripala': 1, 'nimal': 1, 'salvaging': 1, 'cabs': 1, 'greeting': 3, 'fortunate': 1, 'feasts': 1, 'pacheco': 2, 'costan': 1, 'merchants': 2, 'emphatically': 1, 'ashtiani': 2, 'sakineh': 1, 'lashes': 2, 'adultery': 2, 'starve': 1, 'inexplicable': 1, '5\xa0storm': 1, 'measurement': 1, 'measurements': 1, 'outcries': 1, 'zim': 2, 'shinsei': 1, 'maru': 1, 'compensatiion': 1, 'krisnamurthi': 1, 'bayu': 1, 'davoodi': 1, 'parviz': 1, 'odense': 1, 'vollsmose': 1, 'conversions': 1, 'evangelization': 1, 'merapi': 3, 'volcanologist': 1, 'surano': 2, 'eruptions': 2, 'lene': 1, 'espersen': 2, 'baghran': 2, 'merimee': 3, 'understates': 1, 'thankot': 1, 'bhairahawa': 1, 'geophysical': 1, 'sparse': 1, 'guernsey': 4, 'congratulation': 1, 'summarizes': 1, 'flaring': 2, 'pollutes': 1, 'afghanis': 1, 'waistcoat': 1, 'stained': 1, 'fayaz': 4, 'biakand': 1, 'sunnah': 1, '215': 1, 'aulnay': 1, 'eker': 1, 'mellot': 1, 'korangal': 1, 'commissions': 2, 'meckfessel': 1, 'shon': 1, 'fadlallah': 1, 'dispel': 1, 'kahar': 2, 'koyair': 1, 'drunkedness': 1, 'documenting': 2, 'screaming': 2, 'crosses': 1, 'hossam': 1, 'zaki': 1, 'sadat': 1, 'outstripped': 1, 'legia': 1, 'cummings': 2, 'elijah': 1, 'missteps': 1, 'sacirbey': 2, 'maas': 1, 'muhamed': 1, 'garza': 1, 'superdome': 1, 'workouts': 1, 'trinity': 1, 'alamodome': 1, 'herbal': 1, 'palnoo': 1, 'kulgam': 1, 'detonator': 2, 'resembling': 2, 'siasi': 1, 'unprincipled': 1, 'coldest': 1, 'mikulski': 1, 'shyster': 1, 'uhstad': 1, 'mahn': 2, 'thura': 2, 'hardliner': 1, 'indicative': 1, '3700': 1, 'bermel': 1, 'ghormley': 2, 'slumped': 1, 'bargains': 1, 'resales': 1, 'karpinski': 4, 'janice': 2, 'kabungulu': 2, 'kibembi': 1, 'shryock': 1, 'carcasses': 1, 'manifest': 1, 'portoroz': 1, 'obita': 1, 'rostov': 1, 'flagrant': 2, 'checkups': 1, 'dhangadi': 1, 'reclaimed': 1, 'interesting': 2, 'fragmentation': 1, 'cruiser': 1, 'chabanenko': 1, 'nesterenk': 1, 'rearming': 1, 'harith': 1, 'tayseer': 1, 'endeavouron': 1, 'spacewalking': 1, 'reaffirms': 1, 'bergner': 1, 'rish': 1, 'kayani': 1, 'parvez': 1, 'ashfaq': 1, 'bensouda': 1, 'fatou': 1, 'hemingway': 1, 'herrington': 2, 'brighton': 1, 'everado': 1, 'tobasco': 1, 'montiel': 1, 'handpicked': 1, 'ofakim': 1, 'unbeaten': 2, 'resurrected': 1, 'tajouri': 1, 'campaigner': 1, 'polska': 1, 'diminishes': 1, 'obsession': 1, 'junoon': 2, 'falu': 2, 'ethnomusicologist': 1, 'q': 1, 'nabarro': 1, 'mirwaiz': 1, 'kucinich': 1, 'vilsack': 1, 'rodham': 2, 'dulaymi': 2, 'nationalizes': 1, 'poleo': 2, 'mezerhane': 2, 'anez': 1, 'nunez': 1, 'romani': 1, 'eugenio': 1, 'distract': 1, 'lately': 1, 'morshed': 1, 'ldp': 1, 'responsive': 1, 'jawed': 1, 'ludin': 1, 'sordid': 1, 'horticulture': 1, 'evolving': 1, 'derive': 1, 'onerous': 1, 'jdx': 1, 'multilaterals': 1, 'exacerbates': 1, 'judaidah': 1, 'tarmiyah': 1, 'ratios': 1, 'mingling': 1, 'lott': 2, 'witt': 2, 'zalinge': 1, 'demoralized': 1, 'minimized': 1, 'disinvest': 1, 'digits': 2, 'demirel': 3, 'suleyman': 1, 'bourgas': 1, 'aysegul': 1, 'esenler': 1, 'egebank': 1, 'joblessness': 1, 'haridy': 1, 'redmond': 2, 'mirdamadi': 1, 'pakhtoonkhaw': 1, 'iteere': 1, 'tracing': 1, 'almazbek': 1, 'atambayev': 2, 'absalon': 2, 'appointee': 1, 'hashem': 1, 'ashibli': 1, 'clerq': 1, 'manie': 1, 'torching': 1, 'jumbled': 1, 'acclaimed': 1, 'garde': 1, 'avant': 1, 'yakov': 1, 'chernikhov': 1, 'antiques': 1, '274': 1, 'hermitage': 1, 'gilded': 1, 'ladle': 1, 'gastrostomy': 1, 'yass': 1, 'zoba': 1, 'aalam': 3, 'saloumi': 1, 'nkunda': 1, 'rutshuru': 1, 'disproportionate': 1, 'opel': 4, 'fiat': 3, 'cordero': 1, 'della': 1, 'sera': 1, 'montezemolo': 1, 'corriere': 1, 'kamin': 3, 'disregards': 1, 'kotkai': 2, 'bajur': 1, 'resealed': 1, 'squeezing': 1, 'workforces': 1, 'democratia': 1, 'datanalisis': 2, 'channu': 1, 'torchbearers': 1, 'piazza': 2, 'massaua': 1, 'livio': 1, 'berruti': 1, 'palazzo': 1, 'citta': 1, 'tchangai': 1, 'walla': 1, 'kissem': 1, 'lome': 1, 'emmanual': 1, 'townsend': 1, 'jackets': 1, 'schwarzenburg': 1, 'frenchmen': 1, 'jin': 1, 'choi': 1, 'youn': 1, 'spilt': 1, 'unjustifiable': 1, 'beruit': 1, 'odyssey': 1, 'quds': 2, 'indignation': 1, 'boulder': 1, 'zwally': 1, 'bioethics': 1, 'sperm': 1, 'rois': 1, 'darmawan': 2, 'kompas': 1, 'iwan': 1, 'underlined': 1, 'ridding': 1, 'untrue': 1, '1452': 1, '459': 1, 'magazines': 2, 'pamphlets': 3, 'decried': 1, 'vadym': 1, 'chuprun': 1, 'preach': 1, 'namik': 1, 'infuriated': 1, 'bumper': 2, 'shahin': 1, 'abdulla': 1, 'pegs': 1, 'pagonis': 1, 'habila': 1, 'bluefields': 1, 'steelers': 1, 'chargers': 1, 'rams': 2, 'seahawks': 1, 'falcons': 2, 'invalidate': 2, 'kubis': 2, 'tarija': 1, '308': 1, 'tain': 2, 'ahafo': 1, 'afari': 1, 'djan': 1, 'brong': 1, 'npp': 1, 'duping': 1, 'marinellis': 2, 'fleeced': 1, 'gilgit': 1, 'ziauddin': 1, 'conoco': 1, 'gela': 1, 'bezhuashvili': 1, 'abiding': 1, 'rebuffing': 1, 'ghost': 3, '602': 1, 'universally': 1, 'soundly': 2, 'latgalians': 1, 'originates': 1, 'panabaj': 1, 'moviegoers': 1, 'irate': 1, 'propriety': 1, 'bhagwagar': 1, 'sensationalizing': 1, 'revalue': 1, 'churn': 1, '345': 1, 'anatol': 1, 'liabedzka': 1, 'krasovsky': 1, '1776': 1, 'confederacy': 1, 'buoyed': 1, 'indisputably': 1, 'desolate': 1, '1614': 1, 'trappers': 1, 'beerenberg': 1, 'victorian': 1, 'winters': 1, 'bengalis': 2, 'awkward': 1, 'wajed': 1, 'harangued': 1, 'nineva': 1, 'dahal': 1, 'dasain': 1, 'pushpa': 1, 'predetermined': 1, 'stunned': 1, 'reverberated': 1, 'downfall': 1, 'prabtibha': 1, 'patil': 1, 'fallujans': 1, 'swazis': 1, 'mswati': 3, 'grudgingly': 1, 'backslid': 1, 'mattel': 4, 'gagging': 1, 'cesme': 1, 'ksusadasi': 1, 'bassel': 1, 'fleihan': 4, 'sanitary': 1, 'typhoid': 1, 'aguilar': 3, 'zinser': 3, 'morelos': 1, 'disdain': 1, 'lugansville': 1, 'espiritu': 1, 'bankroll': 1, 'fundraiser': 2, '1845': 1, 'chandpur': 1, 'apologies': 1, 'exhort': 1, 'giorgio': 1, 'darwin': 1, 'resurrect': 1, 'adana': 1, 'disparage': 1, 'enraged': 1, 'idolatry': 1, 'eventide': 1, 'goatherd': 2, 'pulikovsky': 2, 'lively': 2, 'selective': 1, 'snowed': 1, 'herd': 2, 'strangers': 1, 'abundantly': 1, 'qubad': 1, 'sonata': 2, 'follower': 2, 'ingratitude': 1, 'restriction': 1, 'hindrance': 1, 'iwc': 1, 'hunts': 1, 'puteh': 2, 'nahrawan': 1, "sa'eed": 1, 'becher': 1, 'comptroller': 1, 'feeble': 1, 'compassionately': 1, 'advertisers': 1, 'nursery': 2, 'abayi': 1, 'abia': 2, 'kourou': 1, 'moans': 1, 'nutrient': 1, 'tonic': 1, 'helios': 1, 'optical': 1, 'parasol': 1, 'halts': 1, 'ge': 2, 'hints': 2, 'mistrust': 1, 'fixation': 1, 'reminder': 1, 'tunisian': 2, 'boudhiba': 4, 'hedi': 1, 'planners': 1, 'yousseff': 1, 'mentally': 2, 'trench': 5, 'fanned': 1, 'andreu': 1, 'hearse': 1, 'volodymyr': 2, 'lytvyn': 1, 'ulrich': 1, 'wilhelm': 1, 'rebelde': 1, 'juventud': 1, 'conjwayo': 1, 'zapata': 1, 'tiempo': 1, 'mauricio': 1, 'arauca': 4, 'bernal': 2, 'sununu': 1, 'tripura': 5, 'dangabari': 1, 'svalbard': 1, 'wainganga': 1, 'wangari': 1, 'maathai': 1, 'environmentalist': 1, 'stoltenberg': 1, 'jens': 1, 'capsize': 1, 'bhandara': 1, '925': 1, 'janan': 1, 'souray': 1, 'gloom': 1, 'lashkargah': 1, 'tables': 1, 'pham': 1, 'thrive': 1, 'painstakingly': 1, 'anchor': 1, 'ulsan': 1, 'sopranos': 2, 'hangout': 1, 'satriale': 1, 'soprano': 3, 'mobster': 1, 'costeira': 3, 'manny': 1, 'condominum': 1, 'authentication': 1, 'serial': 1, 'pals': 1, 'demolishing': 1, 'episode': 1, 'reprocess': 2, 'gref': 1, 'pai': 1, 'f15': 1, 'dohuk': 1, 'okay': 1, 'insurers': 1, 'caters': 1, 'caymanians': 1, 'czechs': 4, 'preoccupied': 1, 'ruthenians': 1, 'sudeten': 1, 'reich': 1, 'truncated': 1, 'ruthenia': 1, 'velvet': 2, 'siam': 1, 'chinnawat': 1, 'mohanna': 1, 'shahdi': 1, 'wetchachiwa': 1, 'udd': 2, 'confiscating': 1, 'politic': 1, 'cleavages': 1, 'marginal': 1, 'underinvestment': 1, 'vitality': 1, 'dombrovskis': 1, 'meekness': 1, 'gentleness': 1, 'temper': 1, 'bridle': 1, 'altogether': 1, 'boldness': 1, 'dread': 1, 'valynkin': 1, 'mainichi': 1, 'amelia': 1, 'rowers': 3, 'mura': 2, 'falash': 2, 'renting': 1, 'rabbis': 3, 'lehava': 1, 'rentals': 1, 'lifestyles': 1, 'muttur': 1, 'hanssen': 1, 'indescribable': 1, 'horrors': 1, 'miracles': 1, 'pingtung': 1, 'e2': 1, 'azizuddin': 3, 'flattening': 1, 'shanties': 1, 'songwriters': 2, 'inductee': 1, 'browne': 1, 'teddy': 1, 'burgie': 1, 'masser': 1, 'randazzo': 1, 'bobby': 1, 'dylan': 1, 'catalog': 1, 'nominating': 2, 'induction': 1, 'abac': 7, 'vindicates': 1, 'septum': 1, 'cerebral': 1, 'balart': 2, 'piling': 1, 'orenburg': 1, 'beiring': 4, '2017': 1, 'polo': 1, 'rugby': 1, 'roller': 1, 'equestrian': 1, 'equine': 1, 'hallams': 1, 'muammar': 1, 'antwerp': 2, 'divas': 1, 'potts': 1, 'jolie': 5, 'angelina': 1, 'pitt': 3, 'naankuse': 1, 'dedication': 1, 'baboons': 1, 'leopards': 1, 'itzik': 1, 'dalia': 1, 'seasonally': 1, 'diouf': 3, 'tropics': 1, 'climates': 1, 'modifying': 1, 'acidity': 1, 'salinity': 1, 'henman': 2, 'idaho': 1, 'taepodong': 1, 'geoscience': 1, 'spewing': 1, 'plume': 1, 'gulfport': 1, 'sancha': 1, 'changqi': 1, 'waterworks': 1, 'jubilant': 1, 'neve': 1, 'dekalim': 1, 'commissioning': 1, 'mowlam': 5, 'mo': 1, 'layered': 1, 'hospice': 1, 'radiotherapy': 1, 'shelve': 1, 'iskan': 1, 'harithiya': 1, 'brazen': 1, 'mashhad': 2, 'nourollah': 1, 'niaraki': 1, 'fuselage': 1, 'dousing': 1, 'airtour': 1, 'tupolev': 1, 'silos': 2, 'logjams': 1, 'ballenas': 1, 'implicate': 2, 'defrauding': 1, 'congressmen': 2, 'psychologically': 1, 'unfurling': 1, 'ayham': 1, 'sammarei': 2, 'mujaheddin': 1, 'distinction': 1, 'radhika': 1, 'coomaraswamy': 1, 'westminster': 2, 'cormac': 1, 'delmas': 1, 'challengerspace': 1, 'minuteman': 3, 'donovan': 1, 'watan': 1, 'blackburn': 1, 'basically': 1, 'sneaking': 1, 'torkham': 2, 'burqa': 1, 'ono': 1, 'yoshinori': 1, 'hauts': 1, 'yvelines': 1, 'oise': 1, "d'": 1, 'seine': 1, 'zheijiang': 1, 'forklift': 2, 'inscriptions': 1, 'etched': 1, 'phoenixes': 1, 'porcelain': 1, 'mirrors': 3, 'avril': 2, 'lavigne': 2, 'whibley': 1, 'derek': 1, 'sauna': 1, 'bedrooms': 1, 'disarms': 1, 'noranit': 1, 'setabutr': 1, 'amass': 1, 'sigou': 1, 'salvadorans': 1, 'solidaria': 1, 'vulgar': 1, 'gniljane': 1, 'selim': 1, 'krasniqi': 2, 'trident': 1, 'transocean': 1, 'channeling': 1, 'indus': 1, 'fused': 1, 'aryan': 1, 'scythians': 1, 'satisfactorily': 1, 'marginalization': 1, 'rocky': 1, 'brightens': 1, 'microchips': 1, 'nicaraguans': 2, 'clinging': 1, 'drunkard': 1, 'idiot': 1, 'chinchilla': 1, 'eurasian': 1, 'khaan': 1, 'chinggis': 1, 'mongols': 3, 'steppe': 1, 'mongolians': 1, 'mprp': 4, 'mpp': 2, 'waemu': 1, 'disbursement': 2, 'bakers': 1, 'concealment': 1, 'tendrils': 1, 'nibble': 1, 'rustling': 1, 'arrow': 1, 'maltreated': 1, 'partridge': 2, 'partridges': 1, 'recompense': 1, 'earnestly': 2, 'scruple': 1, 'hen': 3, 'pondered': 1, 'matched': 1, '425': 1, 'touches': 1, 'closings': 1, 'nasab': 1, 'mohaqiq': 2, 'haqooq': 1, 'zan': 1, 'popovic': 1, 'vujadin': 1, 'ojdanic': 1, 'sainovic': 1, 'lazarevic': 2, 'milutinovic': 1, 'dragoljub': 1, 'moses': 1, 'bittok': 3, 'lottery': 1, 'refinances': 1, 'cyanide': 1, 'cashed': 1, 'echeverria': 4, 'smiley': 1, 'tavis': 1, 'imagine': 1, 'conscription': 1, 'evading': 1, 'abeto': 2, 'asmara': 1, 'adi': 2, 'qadir': 1, 'sinak': 1, 'muadham': 1, 'brillantes': 1, 'kilju': 1, 'cabin': 1, 'spinetta': 1, 'cricketer': 1, 'mauro': 1, 'vechchio': 1, 'sharks': 1, 'stalking': 1, 'crittercam': 1, 'saola': 1, 'hachijo': 1, 'habibur': 1, 'gaddafi': 1, 'tharanga': 1, 'samaraweera': 1, 'thilan': 1, 'paranavithana': 1, 'misleading': 1, 'purposely': 2, 'culprits': 2, 'devise': 1, 'sisli': 1, 'netting': 2, 'compounding': 1, 'agence': 1, 'presse': 1, 'razuri': 6, 'jaime': 2, 'riskiest': 1, 'womb': 1, 'veterinarians': 1, 'farhatullah': 1, 'kypriano': 1, 'kristin': 1, 'halvorsen': 1, 'crave': 1, 'leong': 1, 'lazy': 1, 'mandera': 1, 'prep': 1, 'skelleftea': 1, 'lloyd': 2, 'carli': 1, 'footed': 1, 'dribbled': 1, 'ricocheted': 1, 'pia': 1, 'sundhage': 1, 'cups': 1, 'olmo': 2, 'severity': 1, 'kazemeini': 1, 'disapora': 1, 'speeds': 1, 'parallels': 1, 'lagunas': 1, 'lazaro': 2, 'chacahua': 1, 'cabo': 1, 'corrientes': 1, 'guatemalans': 1, 'tabasco': 1, 'chanet': 2, 'mengal': 1, 'salem': 1, 'ushakov': 2, 'respectfully': 1, '355': 1, 'overtake': 1, 'erase': 1, 'latvians': 1, 'clinch': 1, 'certificates': 4, 'kyat': 1, 'siddiq': 2, 'ronco': 1, 'doled': 1, 'highness': 1, 'surkhanpha': 2, 'kiriyenko': 2, 'megawatts': 2, 'royals': 1, 'scimitar': 1, 'accompany': 1, 'sandhurst': 1, 'graduating': 1, 'isna': 1, 'zolfaghari': 2, 'nie': 1, 'frailty': 1, 'decriminalizing': 1, 'sweltering': 1, 'massa': 1, 'tursunov': 1, 'edgardo': 1, 'phau': 1, 'bjorn': 1, 'gremelmayr': 1, 'teesta': 1, 'ecology': 1, 'ganges': 1, 'damadola': 1, 'scold': 1, 'cuzco': 1, 'reckon': 1, 'incwala': 1, 'nhlanhla': 1, 'nhlabatsi': 1, 'swazi': 1, 'mbabane': 1, 'hms': 1, 'seawater': 1, 'qanuni': 4, 'yunus': 1, 'tadjoura': 1, 'loudspeakers': 1, 'pines': 1, 'ophir': 1, 'relinquish': 1, 'ney': 3, 'pradel': 1, 'rosemond': 1, 'certainty': 2, 'kostiw': 2, 'indiscretion': 1, 'distraction': 2, 'shoplifting': 1, 'tetanus': 2, 'husseindoust': 1, 'destablize': 1, 'dabous': 2, 'yanbu': 2, 'howlwadaag': 2, 'streamlined': 1, 'weiner': 1, 'lobbyists': 1, 'tenzin': 1, 'taklha': 1, 'attribute': 1, 'vials': 1, 'profiting': 1, 'ciampino': 1, 'martha': 1, 'karua': 2, 'goldenberg': 1, 'murungaru': 2, 'meek': 2, 'kendrick': 1, 'cong': 1, 'superhighway': 1, 'shady': 1, 'loitering': 1, 'johanns': 2, 'boyle': 1, '1860s': 1, 'sughd': 1, 'ssr': 1, 'deluge': 1, 'khujand': 1, 'rasht': 1, 'basins': 1, 'sedimentary': 1, 'garments': 1, 'overflowing': 1, 'damsel': 1, 'reclining': 1, 'forgetting': 1, 'nurture': 1, 'truss': 2, 'curious': 1, 'sandbags': 1, 'learnt': 1, 'escapes': 1, 'chevy': 1, 'gallegos': 1, 'sheldon': 1, 'quail': 3, 'shotgun': 4, 'katharine': 2, 'pellets': 2, 'avid': 2, '232': 1, 'honesty': 1, '728': 1, 'aleman': 1, 'arnoldo': 1, 'discredited': 1, 'sandanista': 1, '54th': 1, 'dikes': 1, 'vicinity': 1, 'flashpoints': 1, 'osnabrueck': 1, 'montiglio': 2, 'processor': 1, 'westland': 1, 'hallmark': 1, 'slaughterhouse': 2, 'usda': 1, 'vani': 1, 'contreras': 1, 'averting': 1, 'jeopardizes': 1, '34th': 1, 'socially': 1, 'hatim': 1, '1657': 1, 'megrahi': 1, 'basset': 1, 'neighbourhood': 1, 'saydiyah': 1, 'mans': 1, 'lightened': 1, 'lateral': 1, 'limped': 1, 'edt': 1, '2100': 1, 'doubly': 1, 'aoun': 1, 'sulieman': 1, 'lisjak': 1, 'dushevina': 2, 'gaz': 1, 'ivana': 1, 'byes': 1, 'kveta': 1, 'peschke': 1, 'virginie': 1, 'razzano': 2, 'pironkova': 1, 'tsvetana': 1, 'bareli': 1, 'rae': 1, '70th': 1, 'rajiv': 1, 'tarmac': 2, 'safeguarding': 1, 'refurbished': 1, 'bike': 1, 'walkways': 1, 'sorted': 1, 'crawl': 1, 'unidentifiied': 1, 'gruesome': 1, "'n": 1, 'caliber': 1, 'jaoko': 1, 'chairperson': 1, 'recounting': 1, 'episodes': 1, 'pheasants': 1, 'townships': 1, 'reinvigorate': 1, 'doi': 1, 'talaeng': 1, 'quadriplegic': 1, 'clips': 1, 'sunspots': 1, 'streaming': 1, 'outward': 1, 'disembark': 2, '489': 1, 'forgotten': 1, 'jaber': 1, 'wafa': 1, 'saboor': 1, 'hadhar': 1, 'leatherback': 1, 'fascinate': 1, 'nesting': 1, 'roams': 1, 'leatherbacks': 1, 'phrases': 3, 'tubigan': 1, 'joko': 1, 'suyono': 1, 'intensifies': 1, 'apostates': 1, 'qais': 1, 'shameri': 1, 'rekindle': 1, 'sabotages': 1, 'lawfully': 1, 'empt': 1, 'unleash': 1, 'otari': 1, 'seche': 1, 'miscalculated': 1, 'zap': 1, 'ararat': 1, 'mazlum': 1, 'mattei': 1, 'nuimei': 1, 'listened': 1, '\x97': 1, 'monuc': 1, 'anyplace': 1, 'catapulted': 1, 'showcased': 1, 'madhoun': 1, 'leveling': 1, 'kumtor': 1, 'cis': 1, 'recapitalization': 1, 'cycles': 1, 'grytviken': 2, 'fated': 1, 'shackleton': 1, 'nm': 1, 'aviary': 1, 'wont': 1, 'nightingale': 3, 'peacock': 1, 'accham': 1, 'bajura': 1, 'kohistani': 1, 'ahadi': 1, 'locusts': 1, 'zinder': 1, 'caste': 2, 'castes': 1, 'fortis': 1, 'stella': 1, 'artois': 1, 'radek': 1, 'stepanek': 2, 'johansson': 1, 'tulkarm': 1, 'maclang': 1, 'batad': 1, 'banaue': 1, 'ifugao': 1, 'legaspi': 1, 'starmagazine': 1, 'distorts': 1, 'muasher': 1, 'safehaven': 1, 'wachira': 1, 'waruru': 1, '73rd': 1, 'holodomor': 1, 'kiwis': 1, 'scorers': 1, '201': 1, 'ashraful': 1, 'napier': 1, 'kindhearts': 3, 'cobra': 1, 'lilienfeld': 2, 'detective': 1, 'revolver': 1, 'colt': 1, 'definitively': 1, 'holster': 1, 'aussie': 1, 'flyway': 1, 'yuanshi': 1, 'xianliang': 1, 'sprees': 1, 'gedo': 1, 'udf': 2, 'muluzi': 1, 'bakili': 1, '365': 1, 'yuvraj': 1, 'araft': 1, 'goodbye': 1, 'saca': 1, 'shaowu': 1, 'chairmen': 1, 'mumps': 1, 'rubella': 1, 'autism': 1, 'upali': 1, 'ealier': 1, 'affiliations': 1, 'hypocrisy': 1, 'gardena': 1, 'buechel': 4, 'guay': 1, 'aksel': 1, '417': 1, '442': 1, 'svindal': 1, '420': 1, 'configured': 1, 'nahum': 1, 'repeating': 1, 'costello': 2, 'petrasevic': 1, 'aleksandar': 1, 'pera': 1, 'vukov': 1, 'csatia': 1, 'metullah': 1, 'teeple': 1, 'unspent': 1, 'helmund': 1, 'hushiar': 1, 'facilitates': 1, 'loveland': 1, 'butchers': 1, 'unionize': 1, 'yevpatoria': 2, 'basement': 1, 'acetylene': 1, 'monsoons': 1, 'bong': 1, 'rhee': 3, 'rumbling': 2, 'saqlawiyah': 1, 'rappers': 2, 'nampo': 1, 'samad': 1, 'achakzai': 2, 'kojak': 1, 'sulaiman': 1, 'milli': 1, 'pashtoonkhwa': 1, 'cholily': 1, '221': 1, 'chakul': 1, 'consent': 1, 'abune': 1, 'sbs': 3, 'squares': 1, 'sicko': 1, 'humaneness': 1, 'harshest': 1, 'wealthier': 1, 'heeds': 1, 'celebritiesforcharity': 1, 'raffles': 1, 'https': 1, 'cfm': 1, 'netraffle': 1, 'airfare': 1, 'costumed': 1, 'urchins': 1, 'gravelly': 1, 'beggars': 1, 'porch': 1, 'treaters': 1, 'decorating': 1, 'halloween': 2, 'upshot': 1, 'ghouls': 1, 'dusk': 1, 'starlets': 1, 'distasteful': 1, 'nisr': 1, 'khantumani': 1, '178': 1, 'moravia': 1, 'magyarization': 1, 'issas': 1, 'afars': 2, 'gouled': 1, 'aptidon': 1, 'guelleh': 1, 'socializing': 1, 'terrified': 1, 'pounce': 1, 'slew': 1, 'remedy': 1, 'doosnoswair': 1, 'oliveira': 1, 'onion': 1, 'eggplant': 1, '567': 1, 'smoked': 1, 'trout': 1, 'spaghetti': 1, 'spinach': 1, 'parmesan': 1, 'flavors': 2, 'chili': 1, 'avocado': 1, 'lipstick': 2, 'prints': 3, 'custodian': 1, 'squeegee': 1, 'educators': 1, 'séances': 1, 'believer': 1, 'ear': 1, 'fooling': 1, 'poke': 1, 'merriment': 1, 'gullible': 1, 'scenic': 1, 'mansions': 1, '314': 1, 'inoperable': 3, 'amharic': 1, 'murtha': 3, 'stinging': 1, 'misled': 1, 'rotfeld': 1, 'surrenders': 1, 'onal': 1, 'ulus': 1, 'cabello': 1, 'youssifiyeh': 1, 'kamdesh': 1, 'abbreviations': 1, 'translations': 1, 'transplanting': 1, 'casualities': 1, 'rawhi': 1, 'fattouh': 1, 'corners': 1, '407': 1, 'francs': 1, 'abetting': 2, 'jokic': 1, 'murghab': 1, 'cellist': 2, 'conductor': 1, 'mstislav': 1, 'rostropovich': 3, 'constrain': 2, 'moderating': 1, '39th': 1, 'houngbedji': 1, 'adrien': 1, 'vishnevskaya': 1, 'laughable': 1, 'laiyan': 1, 'jiaotong': 1, 'tp': 1, 'rovnag': 1, 'abdullayev': 1, 'filya': 1, 'bypassing': 1, 'nabucco': 1, 'implications': 1, 'colchester': 1, 'shabat': 1, 'jabbar': 1, 'hobbs': 1, 'jeremy': 1, 'khasavyurt': 1, 'longyan': 1, 'shawal': 2, 'mensehra': 1, 'dialed': 1, 'gibb': 1, 'guadalupe': 1, 'escamilla': 1, 'mota': 1, 'syvkovych': 2, 'rajapakshe': 1, 'kentung': 1, 'leonella': 1, 'sgorbati': 1, 'disable': 1, 'amerzeb': 1, 'beats': 1, 'irresistable': 1, 'slinging': 1, 'shrek': 1, 'grosses': 1, 'bourj': 1, 'abi': 1, 'haidar': 1, 'puccio': 1, 'vince': 1, 'spadea': 1, 'taik': 1, 'proliferator': 1, 'melli': 1, 'agartala': 1, 'sébastien': 1, 'monte': 3, 'wrc': 1, 'citroën': 1, 'xsara': 1, 'duval': 1, 'grönholm': 1, 'auriol': 1, 'submachine': 1, 'eminem': 2, 'mathers': 2, 'hailie': 1, 'estranged': 1, 'remarried': 1, 'recouped': 1, 'continents': 1, 'waldner': 1, 'tycze': 1, 'substantiated': 1, 'establishments': 1, 'ventilation': 1, 'passive': 1, 'kamchatskii': 1, 'petropavlosk': 1, 'fedora': 1, 'adventurer': 1, 'spielberg': 1, 'paramount': 1, 'indy': 1, 'mangos': 1, 'avocados': 1, '1634': 1, 'bonaire': 1, 'refineria': 1, 'isla': 1, 'lied': 1, 'burner': 1, 'housekeeping': 1, 'fuller': 2, 'blacken': 1, 'whiten': 1, 'tormented': 1, 'harnessed': 1, 'heifer': 3, 'yoke': 1, 'smile': 1, 'idleness': 1, 'grasshoppers': 2, 'lighten': 1, 'labors': 1, 'hive': 1, 'honeycomb': 1, 'tasted': 1, 'brute': 1, 'brayed': 1, 'fright': 1, 'cudgelling': 1, 'irrationality': 1, 'lakeside': 1, 'vijay': 1, 'nambiar': 2, 'silly': 1, 'approximation': 2, 'equations': 2, 'clapping': 1, 'luay': 1, 'zaid': 1, 'sander': 1, 'maddalena': 1, 'sardinian': 1, 'humberto': 1, 'valbuena': 1, 'caqueta': 1, 'gospel': 2, 'pilgrim': 2, '1890': 1, 'dorsey': 1, 'mahalia': 1, '13010': 1, 'majdal': 1, 'druze': 2, 'matara': 1, 'atwah': 1, 'matwali': 1, 'muhsin': 1, 'intoxicating': 1, 'damaluji': 1, 'maysoon': 1, 'massum': 1, 'homeowner': 1, 'micky': 1, 'rosenfeld': 1, 'talansky': 1, 'congratulations': 1, 'tokar': 2, 'barka': 1, 'fay': 2, 'kousalyan': 1, 'shipyards': 1, 'disillusionment': 1, 'pictured': 1, 'plastinina': 1, 'kira': 1, 'mikhailova': 1, 'emma': 1, 'sarrata': 1, 'chronicle': 1, 'jamahiriyah': 1, 'pariah': 1, "l'aquilla": 2, 'walled': 1, 'sevilla': 1, 'middlesbrough': 2, '78th': 1, '84th': 1, 'maresca': 1, 'fabiano': 1, 'frédéric': 1, 'kanouté': 1, 'collemaggio': 1, 'tarsyuk': 1, 'adriatic': 1, 'slovenians': 1, 'dagger': 1, 'stashes': 1, 'worm': 3, 'unleashing': 1, 'atilla': 1, 'essebar': 2, 'ekici': 2, 'onna': 1, 'shkin': 1, 'abruzzo': 1, 'cruelty': 1, 'amicable': 1, 'fades': 1, 'remnant': 1, 'animist': 1, 'gil': 1, 'hoffman': 1, 'kohl': 2, 'reunifying': 1, 'helmut': 1, '238': 1, 'verbeke': 1, 'intercepts': 1, 'annul': 2, 'symbolize': 1, 'nazari': 1, 'alako': 1, 'rahmatullah': 1, 'ishaq': 1, 'robinsons': 1, 'grindhouse': 1, 'tarantino': 1, 'debuted': 1, 'quentin': 1, 'dergarabedian': 1, 'tracker': 1, 'takhar': 1, 'icelanders': 1, 'bootleg': 1, 'reykjavik': 1, 'marginalize': 1})
len(encoded_docs[0])
23
NewX = pad_sequences(maxlen=max_len, sequences=encoded_docs, padding="post", value=0)
NewX[15]
array([ 110, 33, 26, 98, 56, 1649, 834, 32, 5699, 5, 780,
3788, 76, 4, 543, 5, 4299, 6304, 2, 30, 1720, 1121,
84, 1236, 8, 5, 3083, 2845, 1275, 52, 97, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0], dtype=int32)
from sklearn.model_selection import train_test_split
X_tr, X_te, y_tr, y_te = train_test_split(NewX, y, test_size=0.1, random_state=2018)
X_tr, X_val = X_tr[:1213*batch_size], X_tr[-135*batch_size:]
y_tr, y_val = y_tr[:1213*batch_size], y_tr[-135*batch_size:]
y_tr = y_tr.reshape(y_tr.shape[0], y_tr.shape[1], 1)
y_val = y_val.reshape(y_val.shape[0], y_val.shape[1], 1)
from keras.layers import Embedding
input_text = Input(shape=(max_len,))
embedding = Embedding(input_dim=10000, output_dim=1024, mask_zero=True)(input_text)
# Complete the model architecture here:
x = Bidirectional(LSTM(units=512, return_sequences=True,
recurrent_dropout=0.2, dropout=0.2))(embedding)
x_rnn = Bidirectional(LSTM(units=512, return_sequences=True,
recurrent_dropout=0.2, dropout=0.2))(x)
x = add([x, x_rnn]) # residual connection to the first biLSTM
out = TimeDistributed(Dense(n_tags, activation="softmax"))(x)
model2 = Model(input_text, out)
model2.compile(optimizer="adam", loss="sparse_categorical_crossentropy", metrics=["accuracy"])
model2.fit(np.array(X_tr), y_tr, validation_data=(np.array(X_val), y_val),
batch_size=batch_size, epochs=5, verbose=1)
Train on 38816 samples, validate on 4320 samples
Epoch 1/5
38816/38816 [==============================] - 325s 8ms/step - loss: 0.1924 - accuracy: 0.9462 - val_loss: 0.1502 - val_accuracy: 0.9532
Epoch 2/5
38816/38816 [==============================] - 323s 8ms/step - loss: 0.1361 - accuracy: 0.9569 - val_loss: 0.1384 - val_accuracy: 0.9572
Epoch 3/5
38816/38816 [==============================] - 323s 8ms/step - loss: 0.1165 - accuracy: 0.9618 - val_loss: 0.1383 - val_accuracy: 0.9576
Epoch 4/5
38816/38816 [==============================] - 322s 8ms/step - loss: 0.0995 - accuracy: 0.9663 - val_loss: 0.1418 - val_accuracy: 0.9582
Epoch 5/5
38816/38816 [==============================] - 320s 8ms/step - loss: 0.0836 - accuracy: 0.9708 - val_loss: 0.1514 - val_accuracy: 0.9579
<keras.callbacks.callbacks.History at 0x7f0bcfa64f98>
X_te = X_te[:149*batch_size,:]
test_pred = model2.predict(np.array(X_te), verbose=1)
4768/4768 [==============================] - 18s 4ms/step
idx2tag = {i: w for w, i in tags2index.items()}
def pred2label(pred):
out = []
for pred_i in pred:
out_i = []
for p in pred_i:
p_i = np.argmax(p)
out_i.append(idx2tag[p_i].replace("PADword", "O"))
out.append(out_i)
return out
def test2label(pred):
out = []
for pred_i in pred:
out_i = []
for p in pred_i:
out_i.append(idx2tag[p].replace("PADword", "O"))
out.append(out_i)
return out
pred_labels = pred2label(test_pred)
test_labels = test2label(y_te[:149*32])
print("F1-score: {:.1%}".format(f1_score(test_labels, pred_labels)))
F1-score: 54.5%
print(classification_report(test_labels, pred_labels))
precision recall f1-score support
geo 0.63 0.52 0.57 3720
per 0.51 0.46 0.48 1677
tim 0.59 0.50 0.54 2148
gpe 0.80 0.68 0.73 1591
org 0.49 0.38 0.42 2061
eve 0.20 0.12 0.15 33
art 0.08 0.02 0.03 49
nat 0.19 0.18 0.19 22
micro avg 0.60 0.50 0.55 11301
macro avg 0.60 0.50 0.54 11301
Note the performance drop in this case!
Let’s see the predictions for the same sentenced analysed before:
i = 390
p = model2.predict(np.array(X_te[i:i+batch_size]))[0]
p = np.argmax(p, axis=-1)
print("{:15} {:5}: ({})".format("Word", "Pred", "True"))
print("="*30)
for w, true, pred in zip(X_te[i], y_te[i], p):
if w != "PADword":
print("{:15}:{:5} ({})".format(w, tags[pred], tags[true]))
Word Pred : (True)
==============================
2327:O (O)
5:O (O)
1604:O (O)
144:O (O)
21:O (O)
1:O (O)
14:O (B-org)
20:B-geo (I-org)
34:O (O)
8632:I-org (O)
383:I-org (O)
1:O (B-org)
49:B-org (I-org)
436:I-org (I-org)
784:I-org (I-org)
16:O (O)
95:O (B-tim)
1:O (O)
1890:O (O)
2436:O (O)
4:O (O)
109:O (O)
155:O (O)
583:O (O)
88:O (O)
6:O (O)
356:O (O)
583:O (O)
88:O (O)
1559:O (B-geo)
5:O (O)
125:O (O)
3:O (O)
60:O (O)
7:O (O)
827:O (O)
90:O (B-tim)
589:O (I-tim)
79:O (O)
1:I-tim (O)
369:I-tim (O)
137:O (O)
116:O (O)
0:O (O)
0:O (O)
0:O (O)
0:O (O)
0:O (O)
0:O (O)
0:O (O)